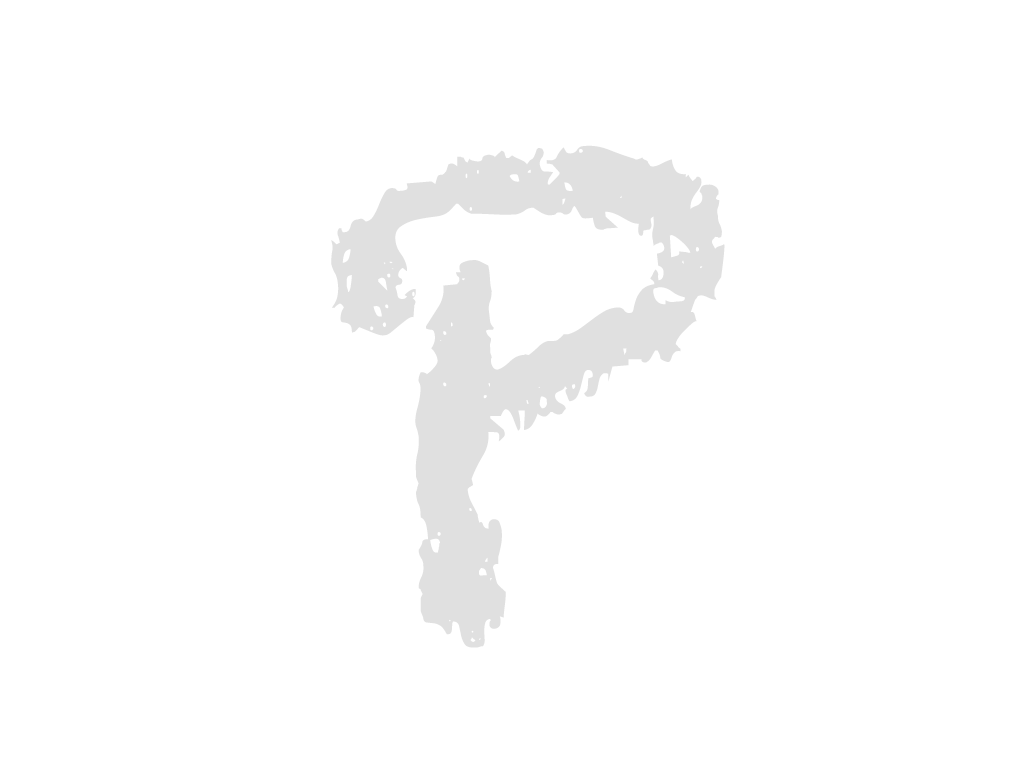
+++ binary mask map test.py
... | ... | @@ -0,0 +1,43 @@ |
1 | +import numpy as np | |
2 | +import cv2 | |
3 | +import glob | |
4 | + | |
5 | +def shift_img(img, x, y): | |
6 | + M = np.float32([[1, 0, x], | |
7 | + | |
8 | + [0, 1, y]]) | |
9 | + | |
10 | + shifted = cv2.warpAffine(img, M, (img.shape[1], img.shape[0])) | |
11 | + | |
12 | + return shifted | |
13 | + | |
14 | +def binary_diff_mask(clean, dirty, thresold=0.2): | |
15 | + # this parts corrects gamma, and always remember, sRGB values are not in linear scale with lights intensity, | |
16 | + clean = np.power(clean, 1/2.2) | |
17 | + dirty = np.power(dirty, 1/2.2) | |
18 | + | |
19 | + # averaged_per_pixel = np.abs(dirty / shift_img(clean, 5, 0) - 1) | |
20 | + # print(averaged_per_pixel) | |
21 | + diff = np.abs(clean - dirty) | |
22 | + | |
23 | + bin_diff = (diff > thresold).astype(np.uint8) | |
24 | + | |
25 | + return bin_diff | |
26 | + | |
27 | +clean = glob.glob("data/source/clean/*.png") | |
28 | +clean = sorted(clean) | |
29 | +dirty = glob.glob("data/source/dirty/*.png") | |
30 | +dirty = sorted(dirty) | |
31 | + | |
32 | +clean_img = cv2.imread(clean[0]) | |
33 | +dirty_img = cv2.imread(dirty[0]) | |
34 | + | |
35 | +binary_diff_mask_img = binary_diff_mask(dirty_img/255, clean_img/255, thresold=0.05) | |
36 | + | |
37 | +k = 40 | |
38 | + | |
39 | +for i in range(k*2): | |
40 | + print(i) | |
41 | + clean_img_copy = shift_img(clean_img, (i-k)/4, 0) | |
42 | + binary_diff_mask_img = binary_diff_mask(dirty_img / 255, clean_img_copy / 255, thresold=0.2) | |
43 | + cv2.imwrite(f"test/test_img{(i-k)/4}.png", binary_diff_mask_img*255) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?