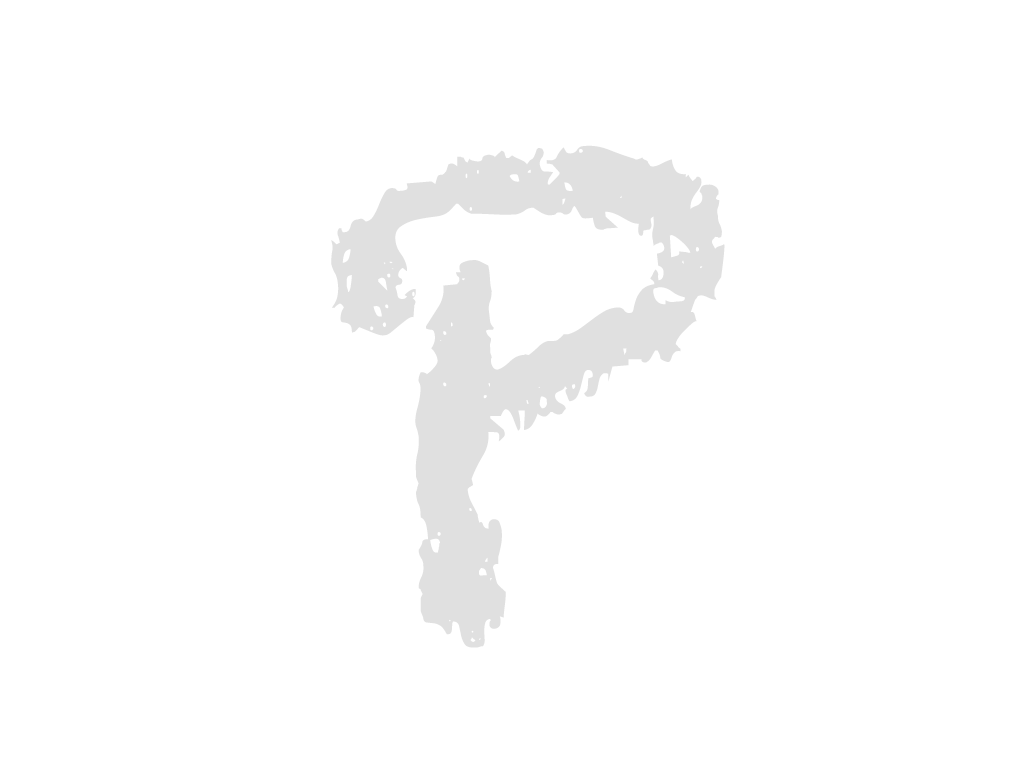
--- binary mask map test.py
+++ binary mask map test.py
... | ... | @@ -1,3 +1,4 @@ |
1 |
+import concurrent.futures |
|
1 | 2 |
import numpy as np |
2 | 3 |
import cv2 |
3 | 4 |
import glob |
... | ... | @@ -11,7 +12,7 @@ |
11 | 12 |
|
12 | 13 |
return shifted |
13 | 14 |
|
14 |
-def binary_diff_mask(clean, dirty, thresold=0.2): |
|
15 |
+def binary_diff_mask(clean, dirty, thresold=0.3): |
|
15 | 16 |
# this parts corrects gamma, and always remember, sRGB values are not in linear scale with lights intensity, |
16 | 17 |
clean = np.power(clean, 1/2.2) |
17 | 18 |
dirty = np.power(dirty, 1/2.2) |
... | ... | @@ -24,20 +25,25 @@ |
24 | 25 |
|
25 | 26 |
return bin_diff |
26 | 27 |
|
27 |
-clean = glob.glob("data/source/clean/*.png") |
|
28 |
+clean = glob.glob("data/source/Oxford_raindrop_dataset/clean/*.png") |
|
28 | 29 |
clean = sorted(clean) |
29 |
-dirty = glob.glob("data/source/dirty/*.png") |
|
30 |
+dirty = glob.glob("data/source/Oxford_raindrop_dataset/dirty/*.png") |
|
30 | 31 |
dirty = sorted(dirty) |
31 | 32 |
|
32 |
-clean_img = cv2.imread(clean[0]) |
|
33 |
-dirty_img = cv2.imread(dirty[0]) |
|
33 |
+clean_img = cv2.imread(clean[34]) |
|
34 |
+dirty_img = cv2.imread(dirty[34]) |
|
34 | 35 |
|
35 | 36 |
binary_diff_mask_img = binary_diff_mask(dirty_img/255, clean_img/255, thresold=0.05) |
36 | 37 |
|
37 |
-k = 40 |
|
38 |
+k = 20 |
|
38 | 39 |
|
39 |
-for i in range(k*2): |
|
40 |
+def process(i, j): |
|
40 | 41 |
print(i) |
41 |
- clean_img_copy = shift_img(clean_img, (i-k)/4, 0) |
|
42 |
- binary_diff_mask_img = binary_diff_mask(dirty_img / 255, clean_img_copy / 255, thresold=0.2) |
|
43 |
- cv2.imwrite(f"test/test_img{(i-k)/4}.png", binary_diff_mask_img*255) |
|
42 |
+ clean_img_copy = shift_img(clean_img, (i-k)/4, (j-k)/4) |
|
43 |
+ binary_diff_mask_img = binary_diff_mask(dirty_img / 255, clean_img_copy / 255, threshold=0.2) |
|
44 |
+ cv2.imwrite(f"test/test_img{(i-k)/4}-{(j-k)/4}.png", binary_diff_mask_img*255) |
|
45 |
+ |
|
46 |
+with concurrent.futures.ProcessPoolExecutor() as executor: |
|
47 |
+ for i in range(k*2): |
|
48 |
+ for j in range(k*2): |
|
49 |
+ executor.submit(process, i, j) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?