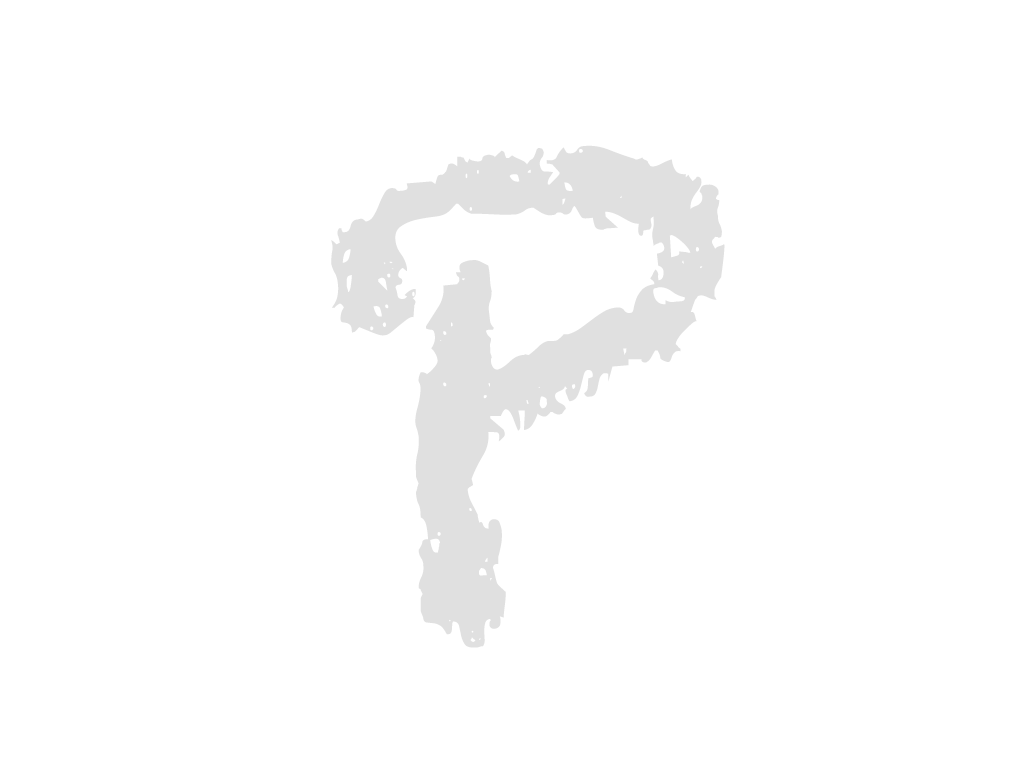
--- algorithms/voronoi.py
+++ algorithms/voronoi.py
... | ... | @@ -15,52 +15,28 @@ |
15 | 15 |
coords = list(geom.exterior.coords) |
16 | 16 |
return (coords) |
17 | 17 |
|
18 |
-# def vor_ver(furthest_pnt, i): |
|
19 |
-# return furthest_pnt[i].vertices |
|
18 |
+def find_lec_for_polygon(points, polygon): |
|
19 |
+ voronoi = Voronoi(points, furthest_site=False) |
|
20 |
+ furthest_pnt = voronoi.vertices |
|
21 |
+ |
|
22 |
+ voronoi_nodes = points_from_xy(extract(furthest_pnt, 0), extract(furthest_pnt, 1)) |
|
23 |
+ voronoi_nodes_if_inside = polygon.contains(voronoi_nodes) |
|
24 |
+ voronoi_nodes = list(compress(voronoi_nodes, voronoi_nodes_if_inside)) |
|
25 |
+ leccc = [item for sublist in extract_coord(voronoi_nodes) for item in sublist] |
|
26 |
+ |
|
27 |
+ dist = cdist(np.array([list(item) for item in points]), np.array([list(item) for item in leccc])) |
|
28 |
+ voronoi_nodes_dist = [min(dist[:, j]) for j in range(dist.shape[1])] |
|
29 |
+ lecc_ind = np.argmax(voronoi_nodes_dist) |
|
30 |
+ lecc = leccc[lecc_ind] |
|
31 |
+ lecc_dist = np.max(voronoi_nodes_dist) |
|
32 |
+ |
|
33 |
+ return lecc, lecc_dist |
|
20 | 34 |
|
21 | 35 |
def get_lecc(shp): |
22 |
- """ |
|
23 |
- This functions finds largest enclosed circle center from shape file. |
|
24 |
- It is used for optimizing text placement and size using Voronoi diagram in this project |
|
25 |
- Arguments: |
|
26 |
- shp -- shape object |
|
36 |
+ polygons_coord = [coord_lister(geom) for geom in shp.geometry] |
|
27 | 37 |
|
28 |
- returns - |
|
29 |
- lecc : which is largest enclosed circle |
|
30 |
- lecc_dist : which is radius of largest enclosed circle |
|
31 |
- """ |
|
32 |
- num_area = len(shp) |
|
33 |
- polygons_coord = shp.geometry.apply(coord_lister) |
|
34 |
- furthest_pnt = [None] * num_area |
|
35 |
- voronoi_ori = [None] * num_area |
|
36 |
- for i, points in enumerate(polygons_coord): |
|
37 |
- voronoi_ori[i] = Voronoi(points, furthest_site=False) |
|
38 |
- furthest_pnt[i] = voronoi_ori[i].vertices |
|
38 |
+ with ProcessPoolExecutor() as executor: |
|
39 |
+ results = list(executor.map(find_lec_for_polygon, polygons_coord, shp.geometry)) |
|
39 | 40 |
|
40 |
- voronoi_nodes = [None] * num_area |
|
41 |
- leccc = [None] * num_area |
|
42 |
- # get voronoi nodes that is inside the polygon. |
|
43 |
- for i in range(num_area): |
|
44 |
- voronoi_nodes[i] = points_from_xy(extract(furthest_pnt[i], 0), extract(furthest_pnt[i], 1)) |
|
45 |
- voronoi_nodes_if_inside = shp.geometry[i].contains(voronoi_nodes[i]) |
|
46 |
- voronoi_nodes[i] = list(compress(voronoi_nodes[i], voronoi_nodes_if_inside)) |
|
47 |
- leccc[i] = [item for sublist in extract_coord(voronoi_nodes[i]) for item in sublist] |
|
48 |
- |
|
49 |
- dist = [None] * num_area |
|
50 |
- for i, points in enumerate(polygons_coord): |
|
51 |
- # explaining this mess : I need to get the LEC from candidate I got before, but the thing is, |
|
52 |
- # cdist from scipy only accepts exact dimension with array like object, |
|
53 |
- # which means I need to explicitly set the shape of ndarray, convert list of points into ndarray |
|
54 |
- dist[i] = cdist(np.array([list(item) for item in points]), np.array([list(item) for item in leccc[i]])) |
|
55 |
- |
|
56 |
- lecc = [None] * num_area |
|
57 |
- lecc_dist = [None] * num_area |
|
58 |
- for i in range(num_area): |
|
59 |
- voronoi_nodes_dist = [None] * (dist[i].shape[1]) |
|
60 |
- for j in range(dist[i].shape[1]): |
|
61 |
- voronoi_nodes_dist[j] = min(dist[i][:, j]) |
|
62 |
- lecc_ind = np.argmax(voronoi_nodes_dist) |
|
63 |
- lecc[i] = leccc[i][lecc_ind] |
|
64 |
- lecc_dist[i] = np.max(voronoi_nodes_dist) |
|
65 |
- |
|
41 |
+ lecc, lecc_dist = zip(*results) |
|
66 | 42 |
return lecc, lecc_dist(No newline at end of file) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?