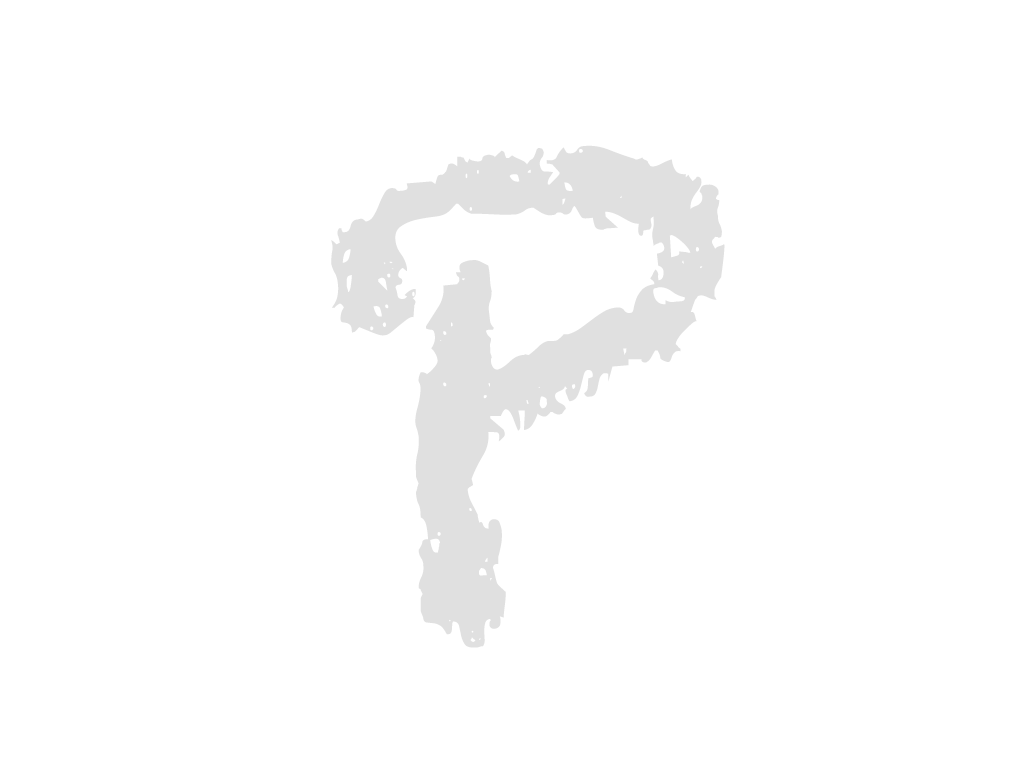
+++ example_GIS_marker.py
... | ... | @@ -0,0 +1,77 @@ |
1 | +from choropleth import choropleth_chart | |
2 | +from choropleth import plotly_fig2array | |
3 | +import plotly.graph_objects as go | |
4 | +import numpy as np | |
5 | +import geopandas as gpd | |
6 | +import pandas as pd | |
7 | +import cv2 | |
8 | + | |
9 | +def extract(lst, i): | |
10 | + return [item[i] for item in lst] | |
11 | + | |
12 | +def is_what(source, compare): | |
13 | + if compare in source: | |
14 | + return True | |
15 | + else: | |
16 | + return False | |
17 | + | |
18 | +shp = gpd.read_file('map/영천시 행정동.shp', encoding='utf-8') | |
19 | +shp = shp.sort_values('EMD_KOR_NM') | |
20 | +shp = shp.reset_index() | |
21 | + | |
22 | +df = np.linspace(0,15,num=16) | |
23 | + | |
24 | +address_book = pd.read_csv('data/영천시병원좌표.csv') | |
25 | + | |
26 | +color = ["#45E646"] * len(address_book['lat']) | |
27 | +shape = ["circle"] * len(address_book['lat']) | |
28 | +size = [12] * len(address_book['lat']) | |
29 | + | |
30 | +for i in range(len(color)): | |
31 | + name = address_book['병원명'][i] | |
32 | + if is_what(name, '보건소'): | |
33 | + color[i]="#FC5BC1" | |
34 | + elif is_what(name, '보건지소'): | |
35 | + color[i]="#9781DB" | |
36 | + elif is_what(name, '보건진료소'): | |
37 | + color[i]="#FC5B48" | |
38 | + elif is_what(name, '한의원'): | |
39 | + color[i]="#A85214" | |
40 | + elif is_what(name, '치과'): | |
41 | + color[i]="#94FFE8" | |
42 | + | |
43 | +for i in range(len(color)): | |
44 | + emergency= address_book["업무구분"][i] | |
45 | + if is_what(emergency, '지역응급의료기관'): | |
46 | + shape[i] = 'star-diamond' | |
47 | + size[i] = 20 | |
48 | + | |
49 | +colorscale = [\ | |
50 | + [0,'#FFEDCF'], | |
51 | + [0.33, '#F0CF2E'], | |
52 | + [0.57, '#F0FAA2'], | |
53 | + [1, '#F0CF2E'] | |
54 | +] | |
55 | + | |
56 | +fig = choropleth_chart(shp, df, '영천시 병원', 'figure/병원', geo_annot_scale=3000, colorscheme=colorscale, adaptive_legend_font_size=True, scale=5, save=False) | |
57 | +fig.add_trace( | |
58 | + go.Scattergeo( | |
59 | + lat=address_book['lat'], lon=address_book['lon'], | |
60 | + marker=dict( | |
61 | + size= size, | |
62 | + color= color, | |
63 | + opacity= 0.6, | |
64 | + symbol=shape | |
65 | + ), | |
66 | + name="", | |
67 | + mode='markers', | |
68 | + text=address_book['병원명'], | |
69 | + ) | |
70 | +) | |
71 | +fig.update( | |
72 | + layout_showlegend=True, | |
73 | + layout_coloraxis_showscale=False | |
74 | +) | |
75 | +scale = 4 | |
76 | + | |
77 | +fig.show()(No newline at end of file) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?