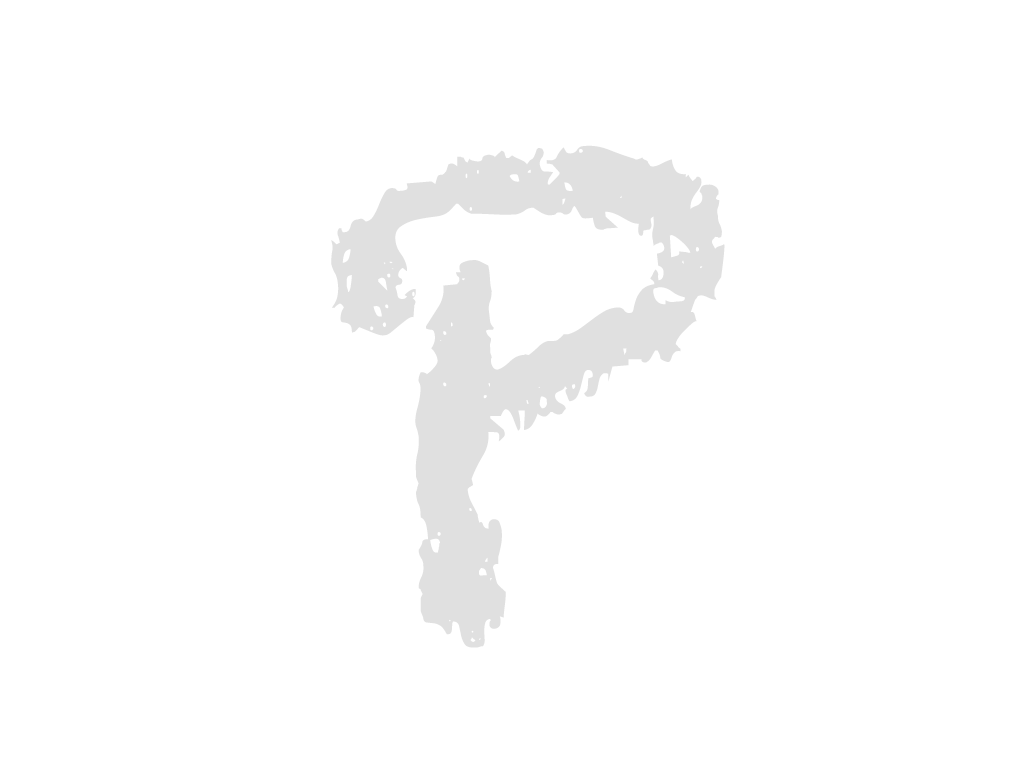
bug fix : wrong operation on segmentation masks (resize on entire proto mask?;;;)
@9b92e6481b61d0fab11babee734d23e45844e653
--- yoloseg/inference_gpu_.py
+++ yoloseg/inference_gpu_.py
... | ... | @@ -166,10 +166,10 @@ |
166 | 166 |
# for now, plural batch operation is not supported, and this is the point where you should start. |
167 | 167 |
# instead of hardcoded proto_masks[0], do some iterative/vectorize operation |
168 | 168 |
mask = np.tensordot(coeffs, proto_masks[0], axes=[0, 0]) # Dot product along the number of prototypes |
169 |
- |
|
169 |
+ resized_mask = cv2.resize(mask,(image_shape[0], image_shape[1])) |
|
170 | 170 |
# Resize mask to the bounding box size, using sigmoid to normalize |
171 |
- resized_mask = cv2.resize(mask, (w, h)) |
|
172 |
- resized_mask = self.sigmoid(resized_mask) |
|
171 |
+ cropped_mask = resized_mask[y1:y1+h, x1:x1+w] |
|
172 |
+ resized_mask = self.sigmoid(cropped_mask) |
|
173 | 173 |
|
174 | 174 |
# Threshold to create a binary mask |
175 | 175 |
final_mask = (resized_mask > 0.5).astype(np.uint8) |
... | ... | @@ -250,14 +250,15 @@ |
250 | 250 |
|
251 | 251 |
def test(): |
252 | 252 |
import time |
253 |
- |
|
253 |
+ import glob |
|
254 |
+ import os |
|
254 | 255 |
# Path to your ONNX model and classes text file |
255 | 256 |
model_path = 'yoloseg/weight/best.onnx' |
256 | 257 |
classes_txt_file = 'config_files/yolo_config.txt' |
257 | 258 |
# image_path = 'yoloseg/img3.jpg' |
258 |
- image_path = 'yoloseg/img3.jpg' |
|
259 |
+ image_path = 'yoloseg/img.jpg' |
|
259 | 260 |
|
260 |
- model_input_shape = (640, 640) |
|
261 |
+ model_input_shape = (480, 480) |
|
261 | 262 |
inference_engine = Inference( |
262 | 263 |
onnx_model_path=model_path, |
263 | 264 |
model_input_shape=model_input_shape, |
... | ... | @@ -273,36 +274,44 @@ |
273 | 274 |
img = cv2.resize(img, model_input_shape) |
274 | 275 |
# Run inference |
275 | 276 |
|
276 |
- for i in range(10): |
|
277 |
+ # for i in range(10): |
|
278 |
+ # t1 = time.time() |
|
279 |
+ # detections, mask_maps = inference_engine.run_inference(img) |
|
280 |
+ # t2 = time.time() |
|
281 |
+ # print(t2 - t1) |
|
282 |
+ |
|
283 |
+ images = glob.glob("/home/juni/사진/flood/out-/*.jpg") |
|
284 |
+ images = sorted(images) |
|
285 |
+ for k, image in enumerate(images): |
|
286 |
+ image = cv2.imread(image) |
|
277 | 287 |
t1 = time.time() |
278 |
- detections, mask_maps = inference_engine.run_inference(img) |
|
288 |
+ image = cv2.resize(image, model_input_shape) |
|
289 |
+ detections, mask_maps = inference_engine.run_inference(image) |
|
290 |
+ |
|
291 |
+ # Display results |
|
292 |
+ for detection in detections: |
|
293 |
+ x, y, w, h = detection['box'] |
|
294 |
+ class_name = detection['class_name'] |
|
295 |
+ confidence = detection['confidence'] |
|
296 |
+ cv2.rectangle(image, (x, y), (x+w, y+h), detection['color'], 2) |
|
297 |
+ label = f"{class_name}: {confidence:.2f}" |
|
298 |
+ cv2.putText(image, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, detection['color'], 2) |
|
299 |
+ if len(mask_maps) != 0: |
|
300 |
+ for i in range(mask_maps.shape[2]): # Iterate over each mask |
|
301 |
+ seg_image = overlay_mask(image, mask_maps[:, :, i], color=(0, 255, 0), alpha=0.3) |
|
302 |
+ # cv2.imshow(f"Segmentation {i + 1}", seg_image) |
|
303 |
+ # cv2.waitKey(0) # Wait for a key press before showing the next mask |
|
304 |
+ # cv2.destroyAllWindows() |
|
279 | 305 |
t2 = time.time() |
280 | 306 |
print(t2 - t1) |
281 |
- |
|
282 |
- |
|
283 |
- |
|
284 |
- # Display results |
|
285 |
- for detection in detections: |
|
286 |
- x, y, w, h = detection['box'] |
|
287 |
- class_name = detection['class_name'] |
|
288 |
- confidence = detection['confidence'] |
|
289 |
- cv2.rectangle(img, (x, y), (x+w, y+h), detection['color'], 2) |
|
290 |
- label = f"{class_name}: {confidence:.2f}" |
|
291 |
- cv2.putText(img, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, detection['color'], 2) |
|
307 |
+ cv2.imwrite( f"/home/juni/사진/flood/infer/{k}.jpg", seg_image) |
|
292 | 308 |
|
293 | 309 |
# Show the image_binary |
294 | 310 |
# cv2.imshow('Detections', img) |
295 | 311 |
# cv2.waitKey(0) |
296 | 312 |
# cv2.destroyAllWindows() |
297 | 313 |
|
298 |
- # If you also want to display segmentation maps, you would need additional handling here |
|
299 |
- # Example for displaying first mask if available: |
|
300 |
- if len(mask_maps) != 0: |
|
301 | 314 |
|
302 |
- seg_image = overlay_mask(img, mask_maps[:,:,0], color=(0, 255, 0), alpha=0.3) |
|
303 |
- cv2.imshow("segmentation", seg_image) |
|
304 |
- cv2.waitKey(0) |
|
305 |
- cv2.destroyAllWindows() |
|
306 | 315 |
|
307 | 316 |
|
308 | 317 |
if __name__ == "__main__": |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?