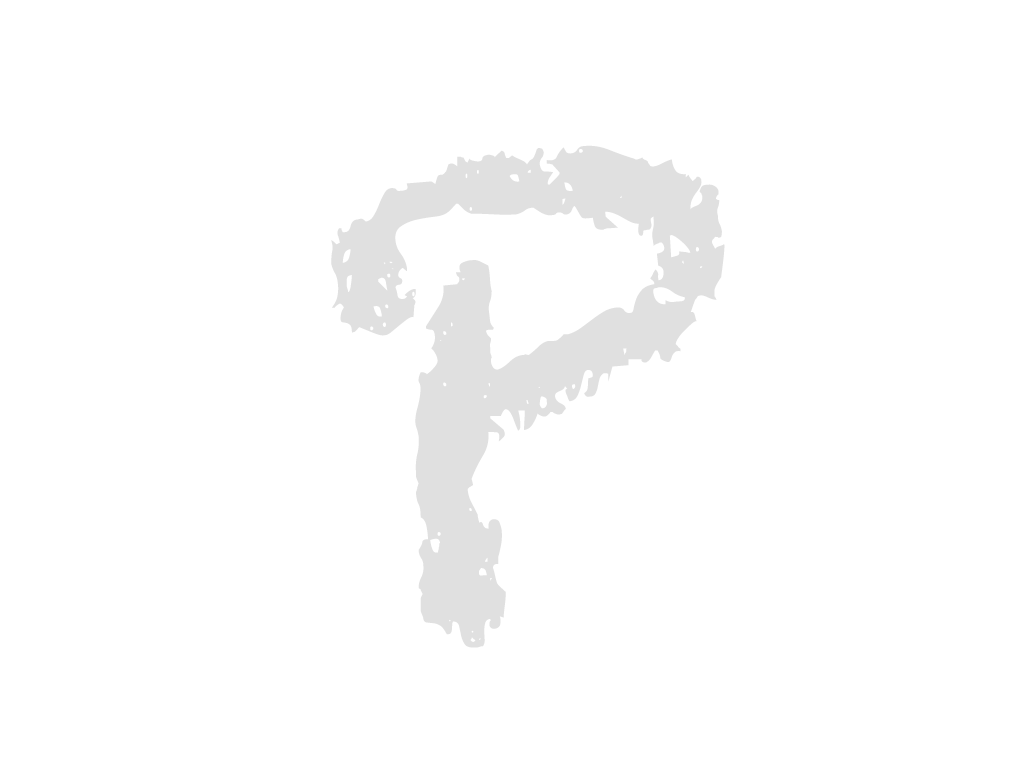
--- streaming_url_updator.py
+++ streaming_url_updator.py
... | ... | @@ -1,11 +1,15 @@ |
1 |
+import json |
|
1 | 2 |
import requests |
3 |
+import textwrap |
|
2 | 4 |
from ITS.api import gather_cctv_list |
5 |
+import urllib.parse |
|
3 | 6 |
|
4 | 7 |
from flask import Flask |
5 | 8 |
from flask_restx import Api |
6 | 9 |
from flask_cors import CORS |
7 | 10 |
from apscheduler.schedulers.background import BackgroundScheduler |
8 | 11 |
from apscheduler.triggers.interval import IntervalTrigger |
12 |
+from requests_toolbelt.utils import dump |
|
9 | 13 |
|
10 | 14 |
API_ENDPOINT = "http://165.229.169.148:8080/EquipmentUrlChanger.json" |
11 | 15 |
|
... | ... | @@ -21,14 +25,31 @@ |
21 | 25 |
) |
22 | 26 |
|
23 | 27 |
|
28 |
+def print_request(req): |
|
29 |
+ data = dump.dump_all(req) |
|
30 |
+ print(data.decode('utf-8')) |
|
31 |
+ |
|
24 | 32 |
def url_list_sender(): |
25 | 33 |
df = gather_cctv_list(129.2, 129.3, 35.9, 36.07, 1, "its", 1) |
26 | 34 |
df = df.drop("roadsectionid", axis=1) |
27 | 35 |
df = df.drop("cctvresolution", axis=1) |
28 | 36 |
df = df.drop("filecreatetime", axis=1) |
29 |
- payload = df.T.to_json(force_ascii=False) |
|
30 |
- respond = requests.post(API_ENDPOINT, json=payload) |
|
31 |
- print(respond) |
|
37 |
+ |
|
38 |
+ headers = { |
|
39 |
+ 'Content-Type': 'application/json', |
|
40 |
+ # 'User-Agent': 'PostmanRuntime/7.39.0', |
|
41 |
+ 'Accept': '*/*', |
|
42 |
+ # 'Postman-Token': 'c9688f7d-228b-4ac7-80d2-217dec302bc8', |
|
43 |
+ 'Accept-Encoding': 'gzip, deflate, br', |
|
44 |
+ 'Connection': 'keep-alive', |
|
45 |
+ # 'Content-Length': '5465' |
|
46 |
+ } |
|
47 |
+ |
|
48 |
+ payload = df.T.to_dict() |
|
49 |
+ payload = list(payload.values()) |
|
50 |
+ payload = json.dumps(payload, indent=2)#, ensure_ascii=False) # the server in question is not using UTF-8, change it when it does. |
|
51 |
+ response = requests.post(API_ENDPOINT, headers=headers, data=payload) |
|
52 |
+ print_request(response) |
|
32 | 53 |
|
33 | 54 |
url_list_sender() |
34 | 55 |
scheduler = BackgroundScheduler() |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?