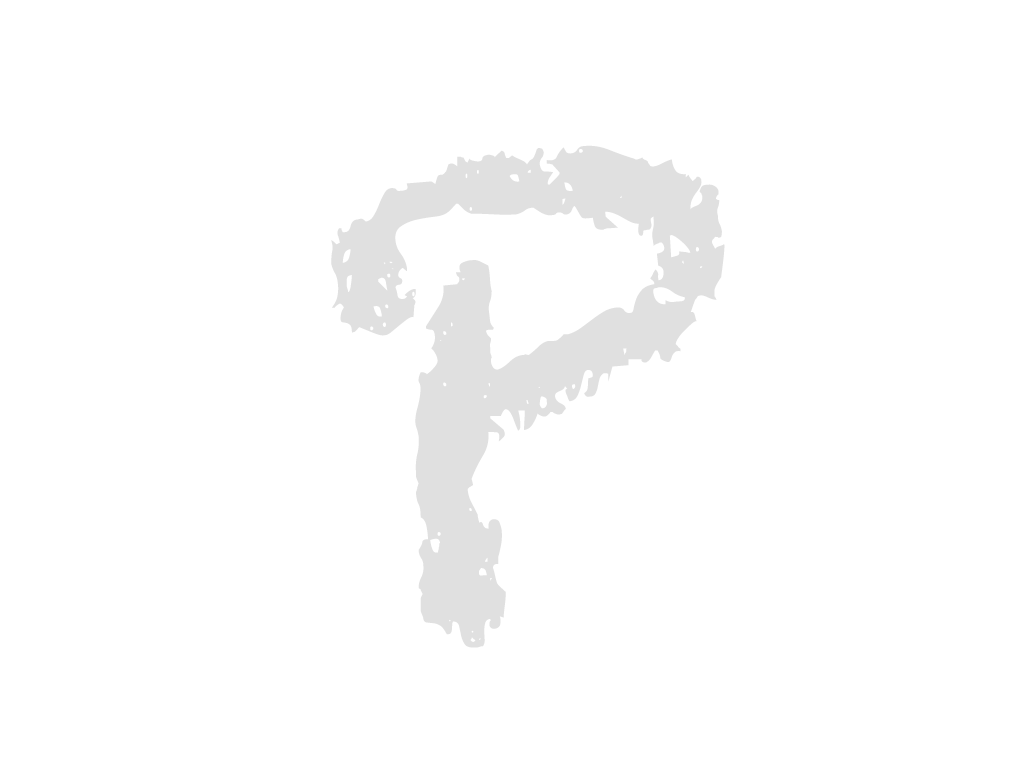
Bug fix and refactoring for inferenece_.py
1. handling xywh <-> xyxy is now a separate method 2. this doubles as a bug fix for occasional dimension mismatch between ```full_masks``` and ```full_mask```
@c8654885df50cdbd58d4f88f48ba4e34a432c7cc
--- inference_endpoint.py
+++ inference_endpoint.py
... | ... | @@ -82,13 +82,11 @@ |
82 | 82 |
|
83 | 83 |
t2 = time.time() |
84 | 84 |
if len(self.mask) > 0: |
85 |
- print(self.mask.shape) |
|
86 |
- print(type(self.mask)) |
|
87 | 85 |
self.mask_blob = cv2.imencode('.png', self.mask) |
88 | 86 |
self.mask_blob = self.mask.tobytes() |
89 | 87 |
self.mask = cv2.resize(self.mask, (image.shape[0], image.shape[1])) |
90 | 88 |
|
91 |
- # print(t2 - t1) |
|
89 |
+ print(t2 - t1) |
|
92 | 90 |
|
93 | 91 |
if len(self.mask) != 0: |
94 | 92 |
seg_image = overlay_mask(image, self.mask[0], color=(0, 255, 0), alpha=0.3) |
--- yoloseg/inference_.py
+++ yoloseg/inference_.py
... | ... | @@ -2,6 +2,7 @@ |
2 | 2 |
import numpy as np |
3 | 3 |
import random |
4 | 4 |
from config_files.yolo_config import CLASS_NAME, CLASS_NUM |
5 |
+from typing import List, Tuple |
|
5 | 6 |
|
6 | 7 |
class Inference: |
7 | 8 |
def __init__(self, onnx_model_path, model_input_shape, classes_txt_file, run_with_cuda): |
... | ... | @@ -99,31 +100,16 @@ |
99 | 100 |
if not detections: |
100 | 101 |
return [] |
101 | 102 |
|
102 |
- batch_size, num_protos, proto_height, proto_width = proto_masks.shape # Correct shape unpacking |
|
103 |
+ batch_size, num_protos, proto_height, proto_width = proto_masks.shape |
|
103 | 104 |
full_masks = np.zeros((len(detections), image_shape[0], image_shape[1]), dtype=np.float32) |
104 | 105 |
|
105 | 106 |
for idx, det in enumerate(detections): |
106 | 107 |
box = det['box'] |
107 |
- x1, y1, w, h = box |
|
108 |
- # print(f"x1 : {x1}, y1 : {y1}, w: {w}, h: {h}") |
|
109 | 108 |
|
110 |
- x1, y1, x2, y2 = x1, y1, x1 + w, y1 + h |
|
109 |
+ x1, y1, w, h = self.adjust_box_coordinates(box, (image_shape[0], image_shape[1])) |
|
111 | 110 |
|
112 |
- #... why the model outputs ... negative values?... |
|
113 |
- if x1 <= 0 : |
|
114 |
- w += x1 |
|
115 |
- x1 = 0 |
|
116 |
- if y1 <= 0 : |
|
117 |
- h += y1 |
|
118 |
- y1 = 0 |
|
119 |
- |
|
120 |
- # To handle edge cases where you get bboxes that pass beyond the original size of image_binary |
|
121 |
- if y2 > image_shape[1]: |
|
122 |
- h = image_shape[1] - y1 |
|
123 |
- if x2 > image_shape[0]: |
|
124 |
- w = image_shape[1] - y1 |
|
125 |
- |
|
126 |
- # print(f"x2: {x2}, y2 : {y2}") |
|
111 |
+ if w <=1 or h <= 1: |
|
112 |
+ continue |
|
127 | 113 |
|
128 | 114 |
# Get the corresponding mask coefficients for this detection |
129 | 115 |
coeffs = det["mask_coefficients"] |
... | ... | @@ -147,15 +133,38 @@ |
147 | 133 |
# print(f"x2: {x2}, y2 : {y2}") |
148 | 134 |
# print(final_mask.shape) |
149 | 135 |
# print(full_mask[y1:y2, x1:x2].shape) |
150 |
- full_mask[y1:y2, x1:x2] = final_mask |
|
136 |
+ full_mask[y1:y1+h, x1:x1+w] = final_mask |
|
151 | 137 |
|
152 | 138 |
# Combine the mask with the masks of other detections |
153 | 139 |
full_masks[idx] = full_mask |
140 |
+ |
|
141 |
+ |
|
154 | 142 |
all_mask = full_masks.sum(axis=0) |
155 |
- # Append a dimension so that cv2 can understand this as an image. |
|
143 |
+ all_mask = np.clip(all_mask, 0, 1) |
|
144 |
+ # Append a dimension so that cv2 can understand ```all_mask``` argument as an image. |
|
156 | 145 |
all_mask = all_mask.reshape((image_shape[0], image_shape[1], 1)) |
157 | 146 |
return all_mask.astype(np.uint8) |
158 | 147 |
|
148 |
+ def adjust_box_coordinates(self, box: List[int], image_shape: Tuple[int, int]) -> Tuple[int, int, int, int]: |
|
149 |
+ """ |
|
150 |
+ Adjusts bounding box coordinates to ensure they lie within image boundaries. |
|
151 |
+ """ |
|
152 |
+ x1, y1, w, h = box |
|
153 |
+ x2, y2 = x1 + w, y1 + h |
|
154 |
+ |
|
155 |
+ # Clamp coordinates to image boundaries |
|
156 |
+ x1 = max(0, x1) |
|
157 |
+ y1 = max(0, y1) |
|
158 |
+ x2 = min(image_shape[1], x2) |
|
159 |
+ y2 = min(image_shape[0], y2) |
|
160 |
+ |
|
161 |
+ # Recalculate width and height |
|
162 |
+ w = x2 - x1 |
|
163 |
+ h = y2 - y1 |
|
164 |
+ |
|
165 |
+ return x1, y1, w, h |
|
166 |
+ |
|
167 |
+ |
|
159 | 168 |
def load_classes_from_file(self): |
160 | 169 |
with open(self.classes_path, 'r') as f: |
161 | 170 |
self.classes = f.read().strip().split('\n') |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?