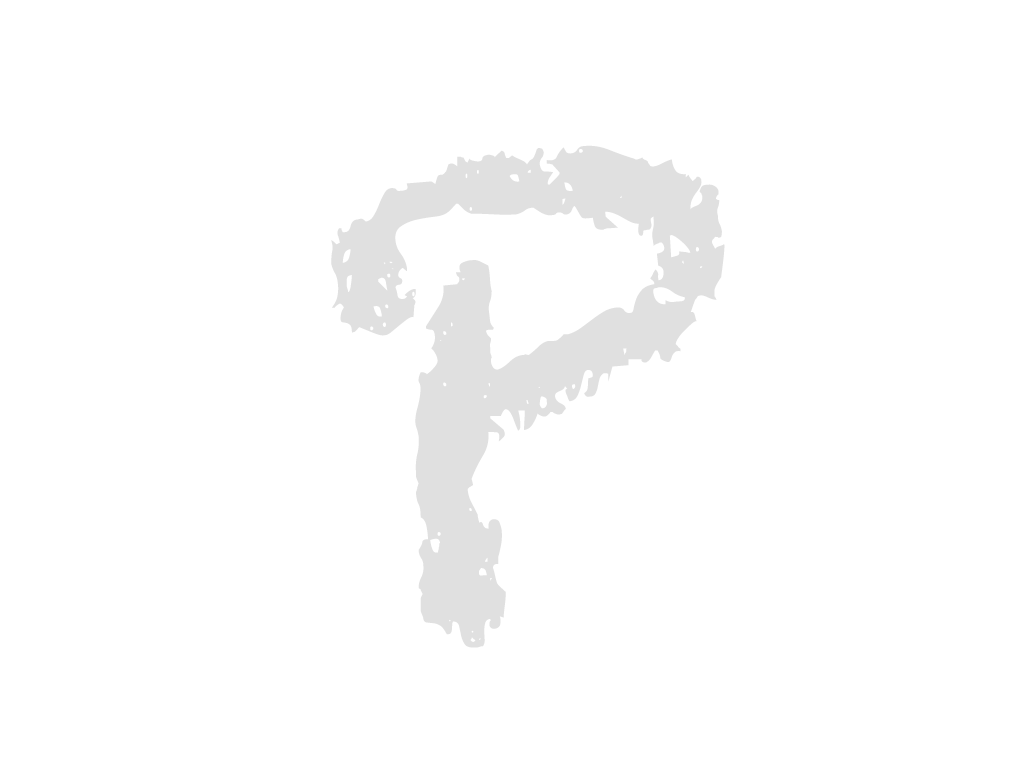
bug fix : mismatch in request file fields name causing postprocess_draft.py not reading segmented image
@ae76301f2f7396b39a4c8b4f61a163b3feef0a2c
--- ITS/api.py
+++ ITS/api.py
... | ... | @@ -48,8 +48,6 @@ |
48 | 48 |
:return: pandas DataFrame 형태로 반환 |
49 | 49 |
주요 컬럼은 다음과 같음 |
50 | 50 |
coordx coordy cctvtype cctvformat cctvname cctvurl |
51 |
- |
|
52 |
- |
|
53 | 51 |
''' |
54 | 52 |
dotenv = load_dotenv() |
55 | 53 |
apiKey= os.getenv("ITS_API") |
... | ... | @@ -68,8 +66,14 @@ |
68 | 66 |
|
69 | 67 |
url = create_url(apiKey, roadType, cctvType, |
70 | 68 |
x, x_next, y, y_next) |
71 |
- response = requests.get(url) |
|
69 |
+ try : |
|
70 |
+ response = requests.get(url) |
|
71 |
+ except : |
|
72 |
+ df_temp = pd.DataFrame([x,x_next,y,y_next], columns=["x_min", "x_max", "y_min", "y_max"]) |
|
73 |
+ df_temp.to_csv(f"ITS/list/error/{x}_{x_next}_{y}_{y_next}.csv") |
|
74 |
+ continue |
|
72 | 75 |
response_json = json.loads(response.text) |
76 |
+ print(response) |
|
73 | 77 |
if response_json['response']['datacount'] == 1: |
74 | 78 |
new_df = pd.json_normalize(response_json['response']['data']) |
75 | 79 |
all_data_df = pd.concat([all_data_df, new_df], ignore_index=True) |
... | ... | @@ -80,7 +84,7 @@ |
80 | 84 |
# df.to_csv(f"result/pohang/listofcctv_포항_{x}_{y}.csv", index=False) |
81 | 85 |
time.sleep(1) |
82 | 86 |
print(f"{i}, {j}") |
83 |
- all_data_df.to_csv(f"result/{xmin}_{xmax}_y{ymin}_{ymax}.csv") |
|
87 |
+ all_data_df.to_csv(f"ITS/list/{xmin}_{xmax}_y{ymin}_{ymax}.csv") |
|
84 | 88 |
return all_data_df |
85 | 89 |
|
86 | 90 |
def get_jpeg(url): |
... | ... | @@ -92,6 +96,6 @@ |
92 | 96 |
|
93 | 97 |
|
94 | 98 |
if __name__ == "__main__": |
95 |
- df = gather_cctv_list(127.9, 128.3, 35.9, 36.07, 1, "its", 1) |
|
99 |
+ df = gather_cctv_list(125, 130, 33, 39, 2, "ex", 1) |
|
96 | 100 |
pass |
97 | 101 |
# get_jpeg("http://cctvsec.ktict.co.kr:8090/74236/IM2NQs4/uHZcgnvJo3V/mjo3tswwgUj87kpcYZfR/BPxaQ4lk9agnl8ARIB9lhlgOD87VBx6RDHFl423kLkqHQ==")(파일 끝에 줄바꿈 문자 없음) |
+++ ITS/api_list_gatherer.py
... | ... | @@ -0,0 +1,114 @@ |
1 | +import requests | |
2 | +import pandas as pd | |
3 | +import numpy as np | |
4 | +import os | |
5 | +import json | |
6 | +import time | |
7 | +from dotenv import load_dotenv | |
8 | +import cv2 | |
9 | + | |
10 | + | |
11 | +def create_url(apiKey, roadType, cctvType, minX, maxX, minY, maxY, getType="json", | |
12 | + baseurl="https://openapi.its.go.kr:9443/cctvInfo"): | |
13 | + ''' | |
14 | + 국가교통정보센터 api 예제 실행 코드, 더 자세한 내용은 | |
15 | + https://www.its.go.kr/opendata/openApiEx?service=cctv 참고 | |
16 | + :param apiKey: ``str`` 국가교통정보센터에서 발급받은 api 키 | |
17 | + :param roadType: ``str`` 도로 유형 ('ex' : 고속도로, 'its' : 국도) | |
18 | + :param cctvType: ``int`` CCTV 유형 (1. 실시간 스트리밍(HLS) / 2. 동영상 파일(m3u8) / 3. 정지 영상(JPEG)) | |
19 | + :param minX: 최소 경도 영역 | |
20 | + :param maxX: 최대 경도 영역 | |
21 | + :param minY: 최소 위도 영역 | |
22 | + :param maxY: 최대 경도 영역 | |
23 | + :param getType: 출력 결과 형식 ("xml" or "json") | |
24 | + :return: api 요청 url | |
25 | + ''' | |
26 | + assert roadType != "ex" or "its", 'Error! roadType should be either "ex" or "its"' | |
27 | + assert cctvType != 1 or 2 or 3, 'Error! cctvType should be one of 1, 2, 3!' | |
28 | + assert getType != "json" or "xml", 'Error! gettype should be either "json" or "xml"!' | |
29 | + return ( | |
30 | + f"{baseurl}?" | |
31 | + f"apiKey={apiKey}&" | |
32 | + f"type={roadType}&" | |
33 | + f"cctvType={cctvType}&" | |
34 | + f"minX={minX}&maxX={maxX}&minY={minY}&maxY={maxY}&" | |
35 | + f"getType={getType}" | |
36 | + ) | |
37 | + | |
38 | + | |
39 | +def gather_cctv_list(xmin, xmax, ymin, ymax, intervals, roadType, cctvType, depth=0, max_depth=5): | |
40 | + ''' | |
41 | + :param minX: 최소 경도 영역 | |
42 | + :param maxX: 최대 경도 영역 | |
43 | + :param minY: 최소 위도 영역 | |
44 | + :param maxY: 최대 경도 영역 | |
45 | + :param intervals: api를 통해서 cctv 목록을 불러올때 위경도 격자 간격 | |
46 | + :param roadType: ``str`` 도로 유형 ('ex' : 고속도로, 'its' : 국도) | |
47 | + :param cctvType: ``int`` CCTV 유형 (1. 실시간 스트리밍(HLS) / 2. 동영상 파일(m3u8) / 3. 정지 영상(JPEG)) | |
48 | + :param depth: ``int`` 재귀적으로 불러온 함수의 깊이 (절대로 직접 넣지 말것.) | |
49 | + :param max_depth: ``int`` 재귀 함수 깊이 제한 | |
50 | + :return: pandas DataFrame 형태로 반환 | |
51 | + 주요 컬럼은 다음과 같음 | |
52 | + coordx coordy cctvtype cctvformat cctvname cctvurl | |
53 | + ''' | |
54 | + dotenv = load_dotenv() | |
55 | + apiKey = os.getenv("ITS_API") | |
56 | + x_values = np.linspace(xmin, xmax, intervals + 1) | |
57 | + y_values = np.linspace(ymin, ymax, intervals + 1) | |
58 | + all_data_df = pd.DataFrame() | |
59 | + | |
60 | + if depth > max_depth: | |
61 | + return all_data_df # Avoids excessive recursion depth | |
62 | + | |
63 | + for i in range(len(x_values) - 1): | |
64 | + for j in range(len(y_values) - 1): | |
65 | + x = x_values[i] | |
66 | + y = y_values[j] | |
67 | + x_next = x_values[i + 1] | |
68 | + y_next = y_values[j + 1] | |
69 | + | |
70 | + url = create_url(apiKey, roadType, cctvType, x, x_next, y, y_next) | |
71 | + try: | |
72 | + response = requests.get(url) | |
73 | + response_json = json.loads(response.text) | |
74 | + if response_json['response']['datacount'] == 0: | |
75 | + continue | |
76 | + new_df = pd.json_normalize(response_json['response']['data']) | |
77 | + all_data_df = pd.concat([all_data_df, new_df], ignore_index=True) | |
78 | + print(f"x: {i}, y: {j}, d: {depth}") | |
79 | + except Exception as e: | |
80 | + # Splitting the area into four if an exception occurs (likely due to too many entries) | |
81 | + if depth < max_depth: | |
82 | + mid_x = (x + x_next) / 2 | |
83 | + mid_y = (y + y_next) / 2 | |
84 | + all_data_df = pd.concat([ | |
85 | + all_data_df, | |
86 | + gather_cctv_list(x, mid_x, y, mid_y, intervals, roadType, cctvType, depth + 1, max_depth), | |
87 | + gather_cctv_list(mid_x, x_next, y, mid_y, intervals, roadType, cctvType, depth + 1, max_depth), | |
88 | + gather_cctv_list(x, mid_x, mid_y, y_next, intervals, roadType, cctvType, depth + 1, max_depth), | |
89 | + gather_cctv_list(mid_x, x_next, mid_y, y_next, intervals, roadType, cctvType, depth + 1, max_depth) | |
90 | + ], ignore_index=True) | |
91 | + time.sleep(1) # To prevent hitting the API rate limit | |
92 | + return all_data_df | |
93 | + | |
94 | + | |
95 | +if __name__ == "__main__": | |
96 | + list2= [ | |
97 | + [129.37762, 36.318485, 'its'], | |
98 | + [129.381293, 36.339985, 'its'], | |
99 | + [129.3459, 36.11983, 'its'], | |
100 | + [129.200930555555, 35.772125, 'its'], | |
101 | + [129.347244, 36.286135, 'its'], | |
102 | + [128.03166, 36.011665, 'its'], | |
103 | + [128.840556, 35.384722, 'ex'] | |
104 | + ] | |
105 | + delta = 0.01 | |
106 | + all_data_df = pd.DataFrame() | |
107 | + for i in list2: | |
108 | + x = i[0] | |
109 | + y = i[1] | |
110 | + ty = i[2] | |
111 | + df = gather_cctv_list(x-delta,x+delta, y-delta, y+delta, 1, ty, 1) | |
112 | + all_data_df = pd.concat((all_data_df,df), ignore_index=True) | |
113 | + # df = gather_cctv_list(127, 130, 33, 39, 8, "its", 1) | |
114 | + all_data_df.to_csv("cctv_data.csv")(파일 끝에 줄바꿈 문자 없음) |
--- config_files/cctv_list.csv
+++ config_files/cctv_list.csv
... | ... | @@ -2,4 +2,11 @@ |
2 | 2 |
,129.245312,35.920346,,,1,HLS,[국도 7호선] 모아초교,http://cctvsec.ktict.co.kr/4463/6SqphQj32sxeB2nYIMerulhkk0HdDKP/aSEgp7hHbUY9iwTDb+UQZfTbag9pXmM/yo3a7l1usm64GwBH77/SCVEel3JF3g9BZDc+ws1es2w= |
3 | 3 |
,129.2005,35.921825,,,1,HLS,[국도20호선] 검단산업단지,http://cctvsec.ktict.co.kr/71187/bWDrL7fpStZDeDZgCybpJH8gagWJOynbaA/l91ExpmUPKzc3bCsHJtIblDkzG3TfmkwkLj7+PPdYAHSYBXxem4SZJpaAYFU0CtDtr5rz7DY= |
4 | 4 |
,128.06396,36.047108,,,1,HLS,[국도3호선] 송죽교차로,http://cctvsec.ktict.co.kr/71208/Z9Acztz6CYwzuUXQFreRR0Kdewba8Ml4CNxR0Jsp9uSCWmvL9W/BO1w2XIPxtxs9kn58qVvtHREs6vU305JaRa40F1m/P6f13gpiz9C+46M= |
5 |
-,128.2671,35.91457,,,1,HLS,[국도33호선] 대흥교차로,http://cctvsec.ktict.co.kr/72593/mTZfGWopuLSKGZsBABx2whdCW4FLmBl6wi4dCvp0MxMpKF6c6ydtf40G8dVXQcAGlDug1DIE/E4MVUyoa3UuD18aYxP6ZCMD5zISEiDxXTQ=(파일 끝에 줄바꿈 문자 없음) |
|
5 |
+,128.2671,35.91457,,,1,HLS,[국도33호선] 대흥교차로,http://cctvsec.ktict.co.kr/72593/mTZfGWopuLSKGZsBABx2whdCW4FLmBl6wi4dCvp0MxMpKF6c6ydtf40G8dVXQcAGlDug1DIE/E4MVUyoa3UuD18aYxP6ZCMD5zISEiDxXTQ= |
|
6 |
+,129.378721,36.310334,,,1,HLS,[국도 7호선] 영덕 구계휴게소,http://cctvsec.ktict.co.kr/4459/kAgktFah0VAtMosKMfrJ13d7CBLAa5+M1xhiLgsypRh5NDDIqY0Y7Z2+hkF88PZUhL1uFJTYshn/Afc4FCS67iWpGfxUOoNU8b0ul3flW8c= |
|
7 |
+,129.37762,36.318485,,,1,HLS,"[국도7호선] 영덕 영덕구계항부근(마을주민,해안가)",http://cctvsec.ktict.co.kr/72957/LkVa3bIbXIBudmzokRTyNtAbqEoZCk+DHRZ8i6cs+rL6QIEM7Fv1N+wfJPjSFQOEmn+Sklz5M3zqnjyiuo6lcPORJsjpyig6wn11ZeH+h44= |
|
8 |
+,129.381293,36.339985,,,1,HLS,[국도 7호선] 오션뷰C.C 앞 삼거리,http://cctvsec.ktict.co.kr/4475/0lZW6kXydguhfnjHZgPeVWi/UKIYrPrUGPfVDyXcGXynS/XFEaXHTYGgmLHdj6QwF1AcHSd6JRZnW+/Ecw30OL/L63URGEp3X/DJqKRrzR0= |
|
9 |
+,129.3459,36.11983,,,1,HLS,[국도7호선] 곡강교,http://cctvsec.ktict.co.kr/71249/YZj8q/z+rpoz8wHUZGst7oMp01rLXrsqhKDnbcKcndono98hK4v5LXE3UumA35GOUC3vxTXsvCBdemtPt60aNJsGUbr+NNiMFUZXDoNutF8= |
|
10 |
+,129.193728,35.762614,,,1,HLS,[국도7호선] 부지교차로,http://cctvsec.ktict.co.kr/72311/kdgcZLCWMjfPL2B06+sReFWMMB+6gW6i1XePhxb0jT/S3jhoQGFbl4nM8fnNunVxbfcv+gtwwve5/Alut8ExIXo8I9h3kV5tNjcqr2ai8d4= |
|
11 |
+,129.200930555555,35.772125,,,1,HLS,[국도 35호선] 용장교,http://cctvsec.ktict.co.kr/5569/7Wk7xI0iDot4My7wDolq5X3jnW9Pgsp7eqT4fmbF1G1dWh248yZuO0r6DZGPJc0lFupmPdL6nCMDyuYljTRHmm7FxLfEMZ9OOKcycSLyHIk= |
|
12 |
+,128.03166,36.011665,,,1,HLS,[국도3호선] 방산교,http://cctvsec.ktict.co.kr/72618/tJq3wZnEySy1HdQLbvzJhD6i+QrqP0HIopbzDMD/h21V/QmMVP26YJUOZsU9WnMsSrPMHfzgTP54irzJjPv+4kCcFJuqvRM63JNFmolU2tE= |
--- inference_endpoint.py
+++ inference_endpoint.py
... | ... | @@ -103,7 +103,6 @@ |
103 | 103 |
self.flag_detected = False |
104 | 104 |
|
105 | 105 |
if self.flag_detected: |
106 |
- print(image.shape) |
|
107 | 106 |
print(self.mask.shape) |
108 | 107 |
self.mask = cv2.resize(self.mask, (self.image.shape[1], self.image.shape[0])) # cv2 saves image with w,h order |
109 | 108 |
self.mask = self.mask[..., np.newaxis] |
... | ... | @@ -151,7 +150,7 @@ |
151 | 150 |
# self.mask_blob, |
152 | 151 |
# f'image/{self.image_type}' |
153 | 152 |
# ), |
154 |
- 'seg_mask' : ( |
|
153 |
+ 'seg_image' : ( |
|
155 | 154 |
f'frame_seg_{self.cctv_name}.{self.image_type}', |
156 | 155 |
seg_binary, |
157 | 156 |
f'image/{self.image_type}' |
--- postprocess_draft.py
+++ postprocess_draft.py
... | ... | @@ -226,7 +226,7 @@ |
226 | 226 |
|
227 | 227 |
memory = StreamSources( |
228 | 228 |
buffer_size=15, |
229 |
- normal_send_interval=1, |
|
229 |
+ normal_send_interval=10, |
|
230 | 230 |
failure_mode_thres=8, |
231 | 231 |
failure_mode_check_past_n=12, |
232 | 232 |
normal_mode_thres=8, |
... | ... | @@ -297,8 +297,8 @@ |
297 | 297 |
|
298 | 298 |
if self.detected: |
299 | 299 |
try: |
300 |
- self.mask = request.files['mask'].read() |
|
301 |
- self.seg_image = request.files['seg_image'].read() |
|
300 |
+ # self.mask = request.files['mask'].read() |
|
301 |
+ self.seg_image = request.files['seg_image'] |
|
302 | 302 |
except: |
303 | 303 |
raise ValueError("Error reading 'mask' and 'seg_mask'") |
304 | 304 |
|
--- run_image_anal_backend.sh
+++ run_image_anal_backend.sh
... | ... | @@ -8,8 +8,24 @@ |
8 | 8 |
# Start multiple Python processes in the background |
9 | 9 |
python streaming_process.py --cctv_num 0 & |
10 | 10 |
pids+=($!) |
11 |
+sleep 1 |
|
11 | 12 |
python streaming_process.py --cctv_num 1 & |
12 | 13 |
pids+=($!) |
14 |
+sleep 1 |
|
15 |
+python streaming_process.py --cctv_num 2 & |
|
16 |
+pids+=($!) |
|
17 |
+sleep 1 |
|
18 |
+python streaming_process.py --cctv_num 3 & |
|
19 |
+pids+=($!) |
|
20 |
+sleep 1 |
|
21 |
+python streaming_process.py --cctv_num 4 & |
|
22 |
+pids+=($!) |
|
23 |
+sleep 1 |
|
24 |
+python streaming_process.py --cctv_num 5 & |
|
25 |
+pids+=($!) |
|
26 |
+sleep 1 |
|
27 |
+python streaming_process.py --cctv_num 6 & |
|
28 |
+pids+=($!) |
|
13 | 29 |
|
14 | 30 |
#python test.py |
15 | 31 |
python inference_endpoint.py |
+++ setup.py
... | ... | @@ -0,0 +1,23 @@ |
1 | +from setuptools import setup | |
2 | + | |
3 | +setup( | |
4 | + name='flood_server', | |
5 | + version='0.9', | |
6 | + packages=[''], | |
7 | + install_requires=[ | |
8 | + 'opencv-python', | |
9 | + 'pandas', | |
10 | + 'numpy', | |
11 | + 'python-dotenv', | |
12 | + 'flask', | |
13 | + 'flask-restx', | |
14 | + 'pyav', | |
15 | + 'requests-toolbelt', | |
16 | + 'onnxrunntime-gpu', | |
17 | + ], | |
18 | + url='', | |
19 | + license='', | |
20 | + author='takensoft-Yoon-Young-Joon', | |
21 | + author_email='[email protected]', | |
22 | + description='', | |
23 | +) |
--- streaming_process.py
+++ streaming_process.py
... | ... | @@ -11,7 +11,7 @@ |
11 | 11 |
import time |
12 | 12 |
|
13 | 13 |
|
14 |
- def refresh_hls_address(lat, lon, lat_lon_interval=0.000001): |
|
14 |
+ def refresh_hls_address(lat, lon, lat_lon_interval=0.0001): |
|
15 | 15 |
api_result = gather_cctv_list( |
16 | 16 |
xmin=lat - lat_lon_interval, ymin=lon - lat_lon_interval, |
17 | 17 |
xmax=lat + lat_lon_interval, ymax=lon + lat_lon_interval, |
--- yoloseg/inference_gpu_.py
+++ yoloseg/inference_gpu_.py
... | ... | @@ -4,7 +4,7 @@ |
4 | 4 |
import numpy as np |
5 | 5 |
import random |
6 | 6 |
import onnxruntime as ort |
7 |
-from config_files.yolo_config import CLASS_NAME, CLASS_NUM |
|
7 |
+from config_files.yolo_config import CLASS_NUM |
|
8 | 8 |
from typing import List, Tuple |
9 | 9 |
|
10 | 10 |
|
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?