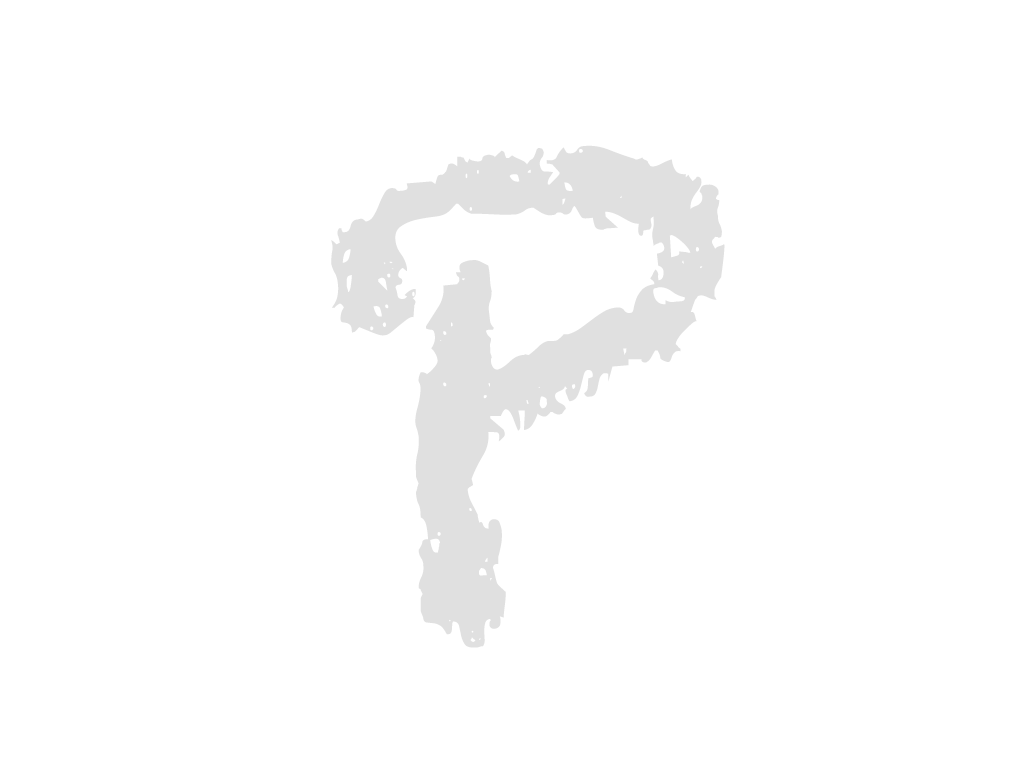
--- config/cctv_list.csv
+++ config_files/cctv_list.csv
No changes |
--- config/hls_streaming_config.py
+++ config_files/hls_streaming_config.py
No changes |
--- config/yolo_config.py
+++ config_files/yolo_config.py
No changes |
--- config/yolo_config.txt
+++ config_files/yolo_config.txt
No changes |
--- hls_streaming/hls.py
+++ hls_streaming/hls.py
... | ... | @@ -100,7 +100,7 @@ |
100 | 100 |
header = { |
101 | 101 |
'Content-Type': f'image/{image_type}', |
102 | 102 |
'x-time-sent': time_sent, |
103 |
- 'x-cctv-info': base64.b64encode(str(self.cctvid).encode('utf-8')).decode('ascii'), |
|
103 |
+ 'x-cctv-name': base64.b64encode(str(self.cctvid).encode('utf-8')).decode('ascii'), |
|
104 | 104 |
'x-cctv-latitude' : str(self.lat), |
105 | 105 |
'x-cctv-longitude' : str(self.lon), |
106 | 106 |
} |
--- inference_endpoint.py
+++ inference_endpoint.py
... | ... | @@ -1,3 +1,4 @@ |
1 |
+import numpy as np |
|
1 | 2 |
from flask import Flask, request |
2 | 3 |
from flask_restx import Api, Resource, fields |
3 | 4 |
import os |
... | ... | @@ -5,6 +6,7 @@ |
5 | 6 |
from yoloseg.inference_ import Inference, overlay_mask |
6 | 7 |
import cv2 |
7 | 8 |
import time |
9 |
+import base64 |
|
8 | 10 |
|
9 | 11 |
app = Flask(__name__) |
10 | 12 |
api = Api(app, version='1.0', title='CCTV Image Upload API', |
... | ... | @@ -14,7 +16,7 @@ |
14 | 16 |
ns = api.namespace('cctv', description='CCTV operations') |
15 | 17 |
|
16 | 18 |
model_path = 'yoloseg/weight/best.onnx' |
17 |
-classes_txt_file = 'config/yolo_config.txt' |
|
19 |
+classes_txt_file = 'config_files/yolo_config.txt' |
|
18 | 20 |
image_path = 'yoloseg/img3.jpg' |
19 | 21 |
|
20 | 22 |
model_input_shape = (640, 640) |
... | ... | @@ -48,32 +50,27 @@ |
48 | 50 |
if 'file' not in request.files: |
49 | 51 |
ns.abort(400, 'No image part in the request') |
50 | 52 |
image = request.files['file'] |
51 |
- cctv_info = request.headers.get('x-cctv-info', '') |
|
53 |
+ cctv_info = base64.b64decode(request.headers.get('x-cctv-name', '')).decode('UTF-8') |
|
52 | 54 |
time_sent = request.headers.get('x-time-sent', '') |
53 | 55 |
cctv_latitude = request.headers.get('x-cctv-latitude', 'Not provided') |
54 | 56 |
cctv_longitude = request.headers.get('x-cctv-longitude', 'Not provided') |
55 | 57 |
|
56 | 58 |
timestamp = datetime.now().strftime("%Y%m%d_%H%M%S") |
57 |
- image = cv2.imdecode(image) |
|
59 |
+ image = image.read() |
|
60 |
+ image = np.frombuffer(image, np.uint8) |
|
61 |
+ image = cv2.imdecode(image, cv2.IMREAD_COLOR) |
|
58 | 62 |
filename = f"{timestamp}_{cctv_info}.png" |
59 | 63 |
|
60 | 64 |
t1 = time.time() |
61 | 65 |
detections, mask_maps = inference_engine.run_inference(image) |
62 | 66 |
t2 = time.time() |
63 | 67 |
|
64 |
- if mask_maps is not None: |
|
68 |
+ print(t2 - t1) |
|
69 |
+ |
|
70 |
+ if len(mask_maps) != 0: |
|
65 | 71 |
seg_image = overlay_mask(image, mask_maps[0], color=(0, 255, 0), alpha=0.3) |
66 |
- |
|
67 |
- if image.filename == '': |
|
68 |
- ns.abort(400, 'No selected image') |
|
69 |
- |
|
70 |
- # Use current timestamp to avoid filename conflicts |
|
71 |
- # image_path = os.path.join(IMAGE_DIR, filename) |
|
72 |
- # image.save(image_path) |
|
73 | 72 |
|
74 | 73 |
return {"message": f"Image {filename} uploaded successfully!"} |
75 | 74 |
|
76 |
- def send_result(self): |
|
77 |
- pass |
|
78 | 75 |
if __name__ == '__main__': |
79 | 76 |
app.run(debug=True, port=12345) |
--- streaming_process.py
+++ streaming_process.py
... | ... | @@ -25,7 +25,7 @@ |
25 | 25 |
|
26 | 26 |
cctv_ind = args.cctv_num |
27 | 27 |
|
28 |
- cctv_info = pd.read_csv("config/cctv_list.csv") |
|
28 |
+ cctv_info = pd.read_csv("config_files/cctv_list.csv") |
|
29 | 29 |
cctv_info = cctv_info.iloc[cctv_ind] |
30 | 30 |
|
31 | 31 |
lat = cctv_info["coordx"] |
--- test.py
+++ test.py
... | ... | @@ -35,7 +35,7 @@ |
35 | 35 |
if 'file' not in request.files: |
36 | 36 |
ns.abort(400, 'No image part in the request') |
37 | 37 |
image = request.files['file'] |
38 |
- cctv_info = base64.b64decode(request.headers.get('x-cctv-info', '')).decode('UTF-8') |
|
38 |
+ cctv_info = base64.b64decode(request.headers.get('x-cctv-name', '')).decode('UTF-8') |
|
39 | 39 |
print(cctv_info) |
40 | 40 |
time_sent = request.headers.get('x-time-sent', '') |
41 | 41 |
cctv_latitude = request.headers.get('x-cctv-latitude', 'Not provided') |
--- yoloseg/inference_.py
+++ yoloseg/inference_.py
... | ... | @@ -1,7 +1,7 @@ |
1 | 1 |
import cv2 |
2 | 2 |
import numpy as np |
3 | 3 |
import random |
4 |
-from config import CLASS_NAME, CLASS_NUM |
|
4 |
+from config_files.yolo_config import CLASS_NAME, CLASS_NUM |
|
5 | 5 |
|
6 | 6 |
class Inference: |
7 | 7 |
def __init__(self, onnx_model_path, model_input_shape, classes_txt_file, run_with_cuda): |
... | ... | @@ -188,7 +188,7 @@ |
188 | 188 |
|
189 | 189 |
# Path to your ONNX model and classes text file |
190 | 190 |
model_path = 'yoloseg/weight/best.onnx' |
191 |
- classes_txt_file = 'yoloseg/config/yolo_config.txt' |
|
191 |
+ classes_txt_file = 'yoloseg/config_files/yolo_config.txt' |
|
192 | 192 |
image_path = 'yoloseg/img3.jpg' |
193 | 193 |
|
194 | 194 |
model_input_shape = (640, 640) |
... | ... | @@ -241,7 +241,7 @@ |
241 | 241 |
|
242 | 242 |
# Path to your ONNX model and classes text file |
243 | 243 |
model_path = 'yoloseg/weight/best.onnx' |
244 |
- classes_txt_file = 'yoloseg/config/yolo_config.txt' |
|
244 |
+ classes_txt_file = 'yoloseg/config_files/yolo_config.txt' |
|
245 | 245 |
|
246 | 246 |
model_input_shape = (640, 640) |
247 | 247 |
inference_engine = Inference( |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?