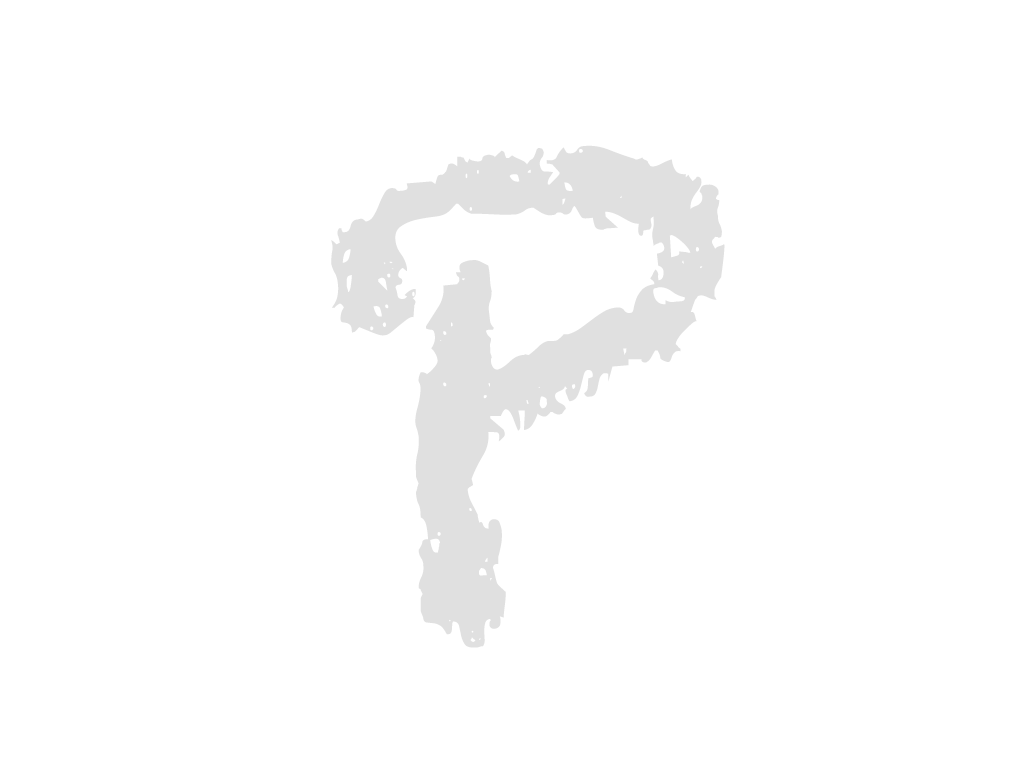
--- .gitignore
+++ .gitignore
... | ... | @@ -1,1 +1,2 @@ |
1 | 1 |
/hls_streaming/captured_frame_/ |
2 |
+/config_files/MAIN_DB_ENDPOINT.json(파일 끝에 줄바꿈 문자 없음) |
+++ config_files/MAIN_DB_ENDPOINT.json
... | ... | @@ -0,0 +1,19 @@ |
1 | +{ | |
2 | + "address" : "210.180.118.83", | |
3 | + "port" : "5423", | |
4 | + "id" : "takensoft", | |
5 | + "password" : "tts96314728!@", | |
6 | + "table_name" : "flooding_detect_event", | |
7 | + "columns" : [ | |
8 | + "ocrn_dt", | |
9 | + "eqpmn_id", | |
10 | + "flooding_result", | |
11 | + "flooding_per", | |
12 | + "image", | |
13 | + "image_seg", | |
14 | + "eqpmn_lat", | |
15 | + "eqpmn_lon", | |
16 | + "flooding_y", | |
17 | + "flooding_x" | |
18 | + ] | |
19 | +}(파일 끝에 줄바꿈 문자 없음) |
--- postprocess_draft.py
+++ postprocess_draft.py
... | ... | @@ -33,10 +33,22 @@ |
33 | 33 |
|
34 | 34 |
class StreamSources(): |
35 | 35 |
def __init__(self, buffer_size, normal_send_interval, failure_mode_thres, failure_mode_check_past_n, normal_mode_thres, normal_mode_check_past_n): |
36 |
- assert failure_mode_thres <= failure_mode_check_past_n, f"failure_mode checker condition is invaild!, failure_mode needs {failure_mode_thres} fails in {failure_mode_check_past_n}, which is not possible!" |
|
37 |
- assert normal_mode_thres <= normal_mode_check_past_n, f"normal_mode checker condition is invaild!, normal_mode needs {normal_mode_thres} fails in {normal_mode_check_past_n}, which is not possible!" |
|
38 |
- assert buffer_size >= failure_mode_check_past_n, f"'buffer_size' is smaller then failure_mode_thres! This is not possible! This will cause program to never enter failure mode!! \nPrinting relevent args and shutting down!\n buffer_size : {buffer_size}\n failure_mode_thres : {failure_mode_thres}" |
|
39 |
- assert buffer_size >= normal_mode_check_past_n, f"'buffer_size' is smaller then normal_mode_thres! This is will cause the program to never revert back to normal mode!! \nPrinting relevent args and shutting down!\n buffer_size : {buffer_size}\n normal_mode_thres : {normal_mode_thres}" |
|
36 |
+ assert failure_mode_thres <= failure_mode_check_past_n,\ |
|
37 |
+ (f"failure_mode checker condition is invaild!," |
|
38 |
+ f" failure_mode needs {failure_mode_thres} fails in {failure_mode_check_past_n}, which is not possible!") |
|
39 |
+ assert normal_mode_thres <= normal_mode_check_past_n,\ |
|
40 |
+ (f"normal_mode checker condition is invaild!," |
|
41 |
+ f" normal_mode needs {normal_mode_thres} fails in {normal_mode_check_past_n}, which is not possible!") |
|
42 |
+ assert buffer_size >= failure_mode_check_past_n,\ |
|
43 |
+ (f"'buffer_size' is smaller then failure_mode_thres! This is not possible!" |
|
44 |
+ f" This will cause program to never enter failure mode!! \n" |
|
45 |
+ f"Printing relevent args and shutting down!\n" |
|
46 |
+ f" buffer_size : {buffer_size}\n failure_mode_thres : {failure_mode_thres}") |
|
47 |
+ assert buffer_size >= normal_mode_check_past_n,\ |
|
48 |
+ (f"'buffer_size' is smaller then normal_mode_thres!" |
|
49 |
+ f" This is will cause the program to never revert back to normal mode!! \n" |
|
50 |
+ f"Printing relevent args and shutting down!\n" |
|
51 |
+ f" buffer_size : {buffer_size}\n normal_mode_thres : {normal_mode_thres}") |
|
40 | 52 |
|
41 | 53 |
self.sources = {} |
42 | 54 |
self.buffer_size = buffer_size |
... | ... | @@ -84,7 +96,8 @@ |
84 | 96 |
return self.sources |
85 | 97 |
|
86 | 98 |
def add_status(self, source, status): |
87 |
- assert status in ["OK", "FAIL"], f"Invalid status was given!, status must be one of 'OK' or 'FAIL', but given '{status}'!" |
|
99 |
+ assert status in ["OK", "FAIL"],\ |
|
100 |
+ f"Invalid status was given!, status must be one of 'OK' or 'FAIL', but given '{status}'!" |
|
88 | 101 |
|
89 | 102 |
if source not in self.sources: |
90 | 103 |
raise ValueError(f"No key found for source. Did you forgot to add it? \n source : {source}") |
... | ... | @@ -125,6 +138,7 @@ |
125 | 138 |
flag_send_event = True |
126 | 139 |
self.sources[source]["normal_to_failure_mode_change_alert"] = True |
127 | 140 |
|
141 |
+ # regular interval message logic |
|
128 | 142 |
if self.sources[source]["last_send_before"] > self.normal_send_interval: |
129 | 143 |
flag_send_event =True |
130 | 144 |
else : |
+++ streaming_url_updator.py
... | ... | @@ -0,0 +1,40 @@ |
1 | +import requests | |
2 | +from ITS.api import gather_cctv_list | |
3 | + | |
4 | +from flask import Flask | |
5 | +from flask_restx import Api | |
6 | +from apscheduler.schedulers.background import BackgroundScheduler | |
7 | +from apscheduler.triggers.interval import IntervalTrigger | |
8 | + | |
9 | +API_ENDPOINT = "" | |
10 | + | |
11 | +app = Flask(__name__) | |
12 | +print("ITS API Updater START") | |
13 | + | |
14 | +api = Api(app, | |
15 | + version='0.1', | |
16 | + title="monitoring", | |
17 | + description="API Server", | |
18 | + terms_url="/", | |
19 | + contact="", | |
20 | + ) | |
21 | + | |
22 | + | |
23 | +def url_list_sender(): | |
24 | + df = gather_cctv_list(129.2, 129.3, 35.9, 36.07, 1, "its", 1) | |
25 | + df = df.drop("roadsectionid", axis=1) | |
26 | + df = df.drop("cctvresolution", axis=1) | |
27 | + df = df.drop("filecreatetime", axis=1) | |
28 | + payload = df.T.to_json() | |
29 | + requests.post(API_ENDPOINT, json=payload) | |
30 | + | |
31 | +url_list_sender() | |
32 | +scheduler = BackgroundScheduler() | |
33 | +scheduler.start() | |
34 | +scheduler.add_job( | |
35 | + func=url_list_sender, | |
36 | + trigger=IntervalTrigger(hours=10), | |
37 | + id='weather_data_update', | |
38 | + name='update weather time every 6 hours', | |
39 | + replace_existing=True | |
40 | +) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?