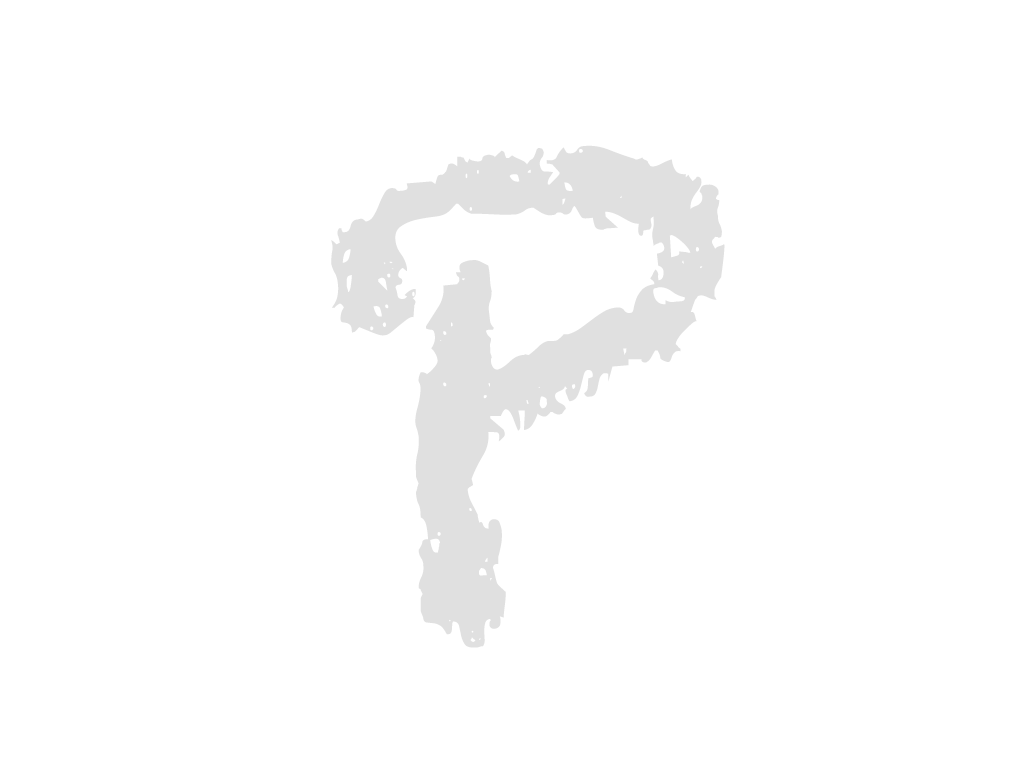
--- hls_streaming/hls.py
+++ hls_streaming/hls.py
... | ... | @@ -90,7 +90,7 @@ |
90 | 90 |
self.send_image_to_server(img_binary) |
91 | 91 |
cv2.imwrite(f'hls_streaming/captured_frame_/{self.cctvid}_{datetime.now()}_{frame_name}', self.current_frame) |
92 | 92 |
self.last_capture_time = current_time |
93 |
- print(f"Captured {frame_name} of {self.cctvid} at time: {current_time - self.start_time:.2f}s") |
|
93 |
+ # print(f"Captured {frame_name} of {self.cctvid} at time: {current_time - self.start_time:.2f}s") |
|
94 | 94 |
self.captured_frame_count +=1 |
95 | 95 |
|
96 | 96 |
time.sleep(0.1) |
--- inference_endpoint.py
+++ inference_endpoint.py
... | ... | @@ -69,8 +69,15 @@ |
69 | 69 |
|
70 | 70 |
if len(mask_maps) != 0: |
71 | 71 |
seg_image = overlay_mask(image, mask_maps[0], color=(0, 255, 0), alpha=0.3) |
72 |
+ area_percent = 0 |
|
73 |
+ else : |
|
74 |
+ area_percent = np.sum(mask_maps) / image.shape[0] * image.shape[1] |
|
72 | 75 |
|
76 |
+ # write another post request for pushing a detection result |
|
73 | 77 |
return {"message": f"Image {filename} uploaded successfully!"} |
74 | 78 |
|
79 |
+ def send_result(self): |
|
80 |
+ pass |
|
81 |
+ |
|
75 | 82 |
if __name__ == '__main__': |
76 | 83 |
app.run(debug=True, port=12345) |
+++ postprocessing.py
... | ... | @@ -0,0 +1,17 @@ |
1 | +import numpy as np | |
2 | +from flask import Flask, request | |
3 | +from flask_restx import Api, Resource, fields | |
4 | +import os | |
5 | +from datetime import datetime | |
6 | +from yoloseg.inference_ import Inference, overlay_mask | |
7 | +import cv2 | |
8 | +import time | |
9 | +import base64 | |
10 | + | |
11 | + | |
12 | +app = Flask(__name__) | |
13 | +api = Api(app, version='1.0', title='Detection postprocessing component', | |
14 | + description='A postprocessing that dynamically decides how frequently send image and detection result') | |
15 | + | |
16 | +# Namespace definition | |
17 | +ns = api.namespace('cctv', description='CCTV operations')(파일 끝에 줄바꿈 문자 없음) |
--- run_image_anal_backend.sh
+++ run_image_anal_backend.sh
... | ... | @@ -11,7 +11,9 @@ |
11 | 11 |
python streaming_process.py --cctv_num 1 & |
12 | 12 |
pids+=($!) |
13 | 13 |
|
14 |
-python test.py |
|
14 |
+ |
|
15 |
+python inference_endpoint.py |
|
16 |
+#gunicorn --workers=6 inference_endpoint:app |
|
15 | 17 |
pids+=($!) |
16 | 18 |
|
17 | 19 |
# Function to kill all processes |
--- yoloseg/inference_.py
+++ yoloseg/inference_.py
... | ... | @@ -58,8 +58,13 @@ |
58 | 58 |
confidence = scores_classification[class_id] |
59 | 59 |
|
60 | 60 |
thres = self.model_score_threshold |
61 |
+ w_thres = 20 |
|
62 |
+ h_thres = 20 |
|
61 | 63 |
if confidence > thres: |
62 | 64 |
x, y, w, h = detection[:4] |
65 |
+ # if bboxes are too small, it just skips, and it is not a bad idea since we do not need to detect small areas |
|
66 |
+ if w < w_thres or h < h_thres: |
|
67 |
+ continue |
|
63 | 68 |
left = int((x - 0.5 * w) * x_factor) |
64 | 69 |
top = int((y - 0.5 * h) * y_factor) |
65 | 70 |
width = int(w * x_factor) |
... | ... | @@ -97,19 +102,25 @@ |
97 | 102 |
for idx, det in enumerate(detections): |
98 | 103 |
box = det['box'] |
99 | 104 |
x1, y1, w, h = box |
105 |
+ print(f"x1 : {x1}, y1 : {y1}, w: {w}, h: {h}") |
|
106 |
+ |
|
107 |
+ x1, y1, x2, y2 = x1, y1, x1 + w, y1 + h |
|
100 | 108 |
|
101 | 109 |
#... why the model outputs ... negative values?... |
102 | 110 |
if x1 <= 0 : |
111 |
+ w += x1 |
|
103 | 112 |
x1 = 0 |
104 | 113 |
if y1 <= 0 : |
114 |
+ h += y1 |
|
105 | 115 |
y1 = 0 |
106 |
- x1, y1, x2, y2 = x1, y1, x1 + w, y1 + h |
|
107 | 116 |
|
108 |
- # To handle edge cases where you get bboxes that pass beyond the original image_binary |
|
117 |
+ # To handle edge cases where you get bboxes that pass beyond the original size of image_binary |
|
109 | 118 |
if y2 > image_shape[1]: |
110 |
- h = h + image_shape[1] - h - y1 |
|
119 |
+ h = image_shape[1] - y1 |
|
111 | 120 |
if x2 > image_shape[0]: |
112 |
- w = w + image_shape[1] - w - y1 |
|
121 |
+ w = image_shape[1] - y1 |
|
122 |
+ |
|
123 |
+ print(f"x2: {x2}, y2 : {y2}") |
|
113 | 124 |
|
114 | 125 |
# Get the corresponding mask coefficients for this detection |
115 | 126 |
coeffs = det["mask_coefficients"] |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?