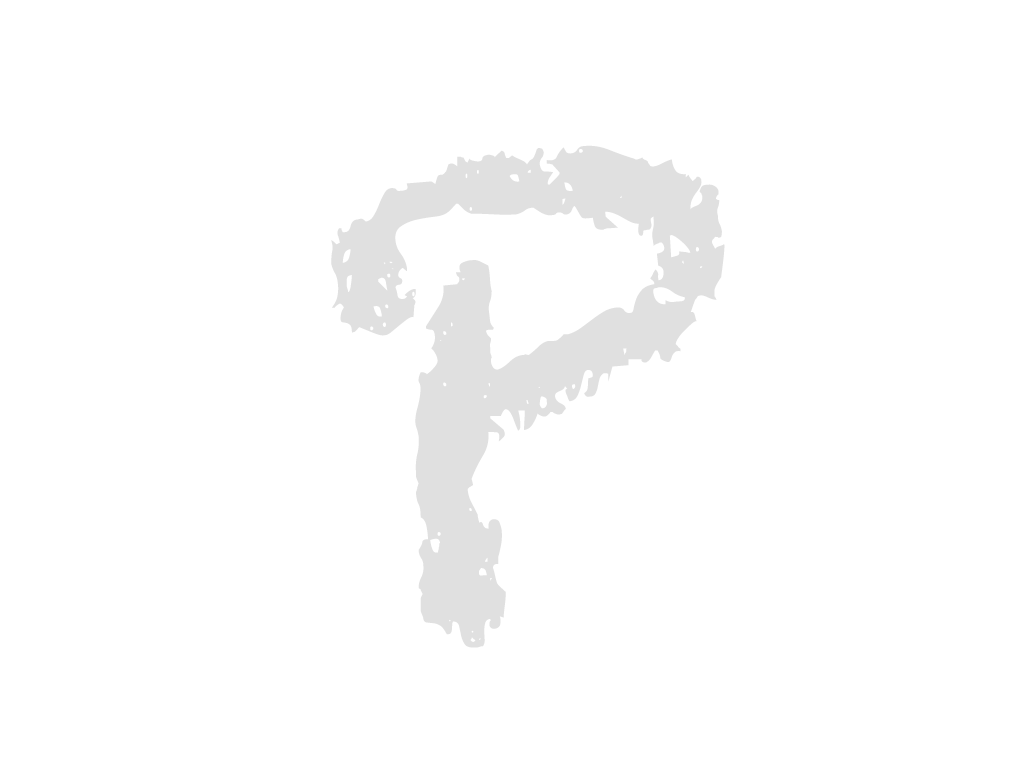
--- ITS/api.py
+++ ITS/api.py
... | ... | @@ -30,7 +30,7 @@ |
30 | 30 |
return ( |
31 | 31 |
f"{baseurl}?" |
32 | 32 |
f"apiKey={apiKey}&" |
33 |
- f"roadType={roadType}&" |
|
33 |
+ f"type={roadType}&" |
|
34 | 34 |
f"cctvType={cctvType}&" |
35 | 35 |
f"minX={minX}&maxX={maxX}&minY={minY}&maxY={maxY}&" |
36 | 36 |
f"getType={getType}" |
... | ... | @@ -92,6 +92,6 @@ |
92 | 92 |
|
93 | 93 |
|
94 | 94 |
if __name__ == "__main__": |
95 |
- df = gather_cctv_list(129.2, 129.3, 35.9, 36.07, 1, 1) |
|
95 |
+ df = gather_cctv_list(129.2, 129.3, 35.9, 36.07, 1, "its", 1) |
|
96 | 96 |
pass |
97 | 97 |
# get_jpeg("http://cctvsec.ktict.co.kr:8090/74236/IM2NQs4/uHZcgnvJo3V/mjo3tswwgUj87kpcYZfR/BPxaQ4lk9agnl8ARIB9lhlgOD87VBx6RDHFl423kLkqHQ==")(파일 끝에 줄바꿈 문자 없음) |
+++ config/cctv_list.csv
... | ... | @@ -0,0 +1,3 @@ |
1 | +,roadsectionid,coordx,coordy,cctvresolution,filecreatetime,cctvtype,cctvformat,cctvname,cctvurl | |
2 | +0,,129.245312,35.920346,,,1,HLS,[국도 7호선] 모아초교,http://cctvsec.ktict.co.kr/4463/6SqphQj32sxeB2nYIMerulhkk0HdDKP/aSEgp7hHbUY9iwTDb+UQZfTbag9pXmM/yo3a7l1usm64GwBH77/SCVEel3JF3g9BZDc+ws1es2w= | |
3 | +1,,129.2005,35.921825,,,1,HLS,[국도20호선] 검단산업단지,http://cctvsec.ktict.co.kr/71187/bWDrL7fpStZDeDZgCybpJH8gagWJOynbaA/l91ExpmUPKzc3bCsHJtIblDkzG3TfmkwkLj7+PPdYAHSYBXxem4SZJpaAYFU0CtDtr5rz7DY=(파일 끝에 줄바꿈 문자 없음) |
--- config/cctv_lists.csv
... | ... | @@ -1,0 +0,0 @@ |
--- hls_streaming/hls.py
+++ hls_streaming/hls.py
... | ... | @@ -1,4 +1,3 @@ |
1 |
-import parser |
|
2 | 1 |
import time |
3 | 2 |
import av |
4 | 3 |
import cv2 |
--- hls_streaming/streaming_process.py
+++ hls_streaming/streaming_process.py
... | ... | @@ -1,14 +1,56 @@ |
1 |
-from ITS.api import gather_cctv_list |
|
2 |
-from hls_streaming.hls import FrameCapturer |
|
3 |
- |
|
4 |
-def refresh_hls_address(lat, lon, lat_lon_interval=0.000001): |
|
5 |
- api_result = gather_cctv_list(xmin=lat-lat_lon_interval, ymin=lon-lat_lon_interval, xmax=lat+lat_lon_interval, ymax=lon+lat_lon_interval, intervals=1, roadType=1) |
|
6 |
- return api_result.loc[0]["cctvurl"] |
|
1 |
+# This script only works if this is the main process. |
|
2 |
+# This script must be executed with bash script that is located in the root directory of this project |
|
3 |
+# This is by design, and is a way to circumvent GIL of python, because the server needs to handle multiple video streaming at once. |
|
7 | 4 |
|
8 | 5 |
if __name__ == "__main__": |
6 |
+ from dotenv import load_dotenv |
|
7 |
+ import os |
|
8 |
+ |
|
9 |
+ load_dotenv() |
|
10 |
+ print("PYTHONPATH:", os.getenv("PYTHONPATH")) |
|
11 |
+ print("_____") |
|
12 |
+ print(os.path.dirname(os.path.abspath(__file__))) |
|
13 |
+ from ITS.api import gather_cctv_list |
|
14 |
+ from hls_streaming.hls import FrameCapturer |
|
15 |
+ |
|
9 | 16 |
import argparse |
10 |
- args = argparse.ArgumentParser |
|
11 |
- args.add_argument("cctv_num", help="index number of cctv, see cctv_list.csv") |
|
17 |
+ import pandas as pd |
|
18 |
+ import time |
|
19 |
+ |
|
20 |
+ |
|
21 |
+ def refresh_hls_address(lat, lon, lat_lon_interval=0.000001): |
|
22 |
+ api_result = gather_cctv_list( |
|
23 |
+ xmin=lat - lat_lon_interval, ymin=lon - lat_lon_interval, |
|
24 |
+ xmax=lat + lat_lon_interval, ymax=lon + lat_lon_interval, |
|
25 |
+ intervals=1, roadType=1, cctvType=1 |
|
26 |
+ ) |
|
27 |
+ return api_result.loc[0]["cctvurl"] |
|
28 |
+ |
|
29 |
+ args = argparse.ArgumentParser() |
|
30 |
+ args.add_argument("--cctv_num", type=int, help="index number of cctv, see cctv_list.csv") |
|
12 | 31 |
args = args.parse_args() |
13 | 32 |
|
14 | 33 |
cctv_ind = args.cctv_num |
34 |
+ |
|
35 |
+ cctv_info = pd.read_csv("config/cctv_list.csv") |
|
36 |
+ cctv_info = cctv_info.iloc[0] |
|
37 |
+ |
|
38 |
+ lat = cctv_info["coordx"] |
|
39 |
+ lon = cctv_info["coordy"] |
|
40 |
+ name = cctv_info["cctvname"] |
|
41 |
+ if name.find("국도"): |
|
42 |
+ roadtype = 'its' |
|
43 |
+ else: |
|
44 |
+ roadtype = 'ex' |
|
45 |
+ |
|
46 |
+ # initiate ITS CCTV streaming via hls |
|
47 |
+ while True: |
|
48 |
+ |
|
49 |
+ cctv_url = refresh_hls_address(lat, lon, ) |
|
50 |
+ hls_streaming = FrameCapturer(cctv_url, name, 5, 15, 300, "Asia/Seoul", |
|
51 |
+ endpoint= "http://localhost:12345/cctv/infer") |
|
52 |
+ hls_streaming.start() |
|
53 |
+ |
|
54 |
+ time.wait(60000) |
|
55 |
+ |
|
56 |
+ hls_streaming.stop() |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?