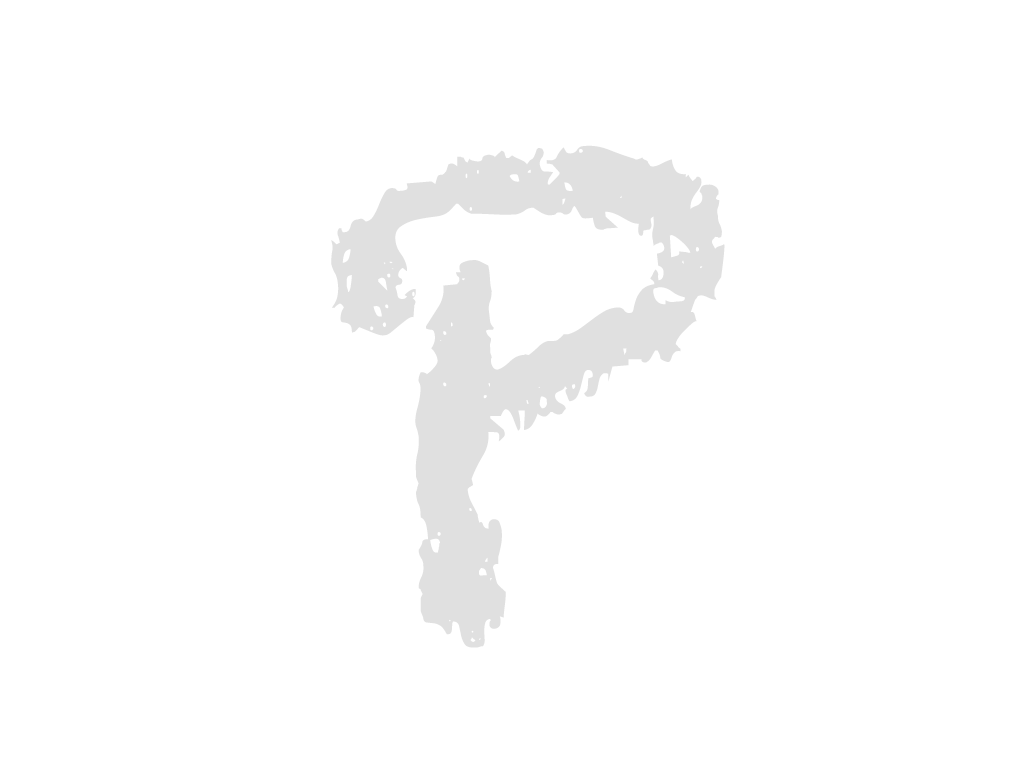
+++ postprocess_draft.py
... | ... | @@ -0,0 +1,71 @@ |
1 | +class StreamSources: | |
2 | + def __init__(self, buffer_size, normal_send_interval, failure_mode_thres, failure_mode_check_past_n, normal_mode_thres, normal_mode_check_past_n): | |
3 | + assert failure_mode_thres <= failure_mode_check_past_n, f"failure_mode checker condition is invaild!, failure_mode needs {failure_mode_thres} fails in {failure_mode_check_past_n}, which is not possible!" | |
4 | + assert failure_mode_thres <= failure_mode_check_past_n, f"normal_mode checker condition is invaild!, normal_mode needs {failure_mode_thres} fails in {normal_mode_check_past_n}, which is not possible!" | |
5 | + assert buffer_size < failure_mode_check_past_n, f"'buffer_size' is smaller then failure_mode_thres! This is not possible! This will cause program to never enter failure mode!! \nPrinting relevent args and shutting down!\n buffer_size : {buffer_size}\n failure_mode_thres : {failure_mode_thres}" | |
6 | + assert buffer_size < normal_mode_thres, f"'buffer_size' is smaller then normal_mode_thres! This is will cause the program to never revert back to normal mode!! \nPrinting relevent args and shutting down!\n buffer_size : {buffer_size}\n normal_mode_thres : {normal_mode_thres}" | |
7 | + | |
8 | + self.sources = {} | |
9 | + self.buffer_size = buffer_size | |
10 | + self.normal_send_interval = normal_send_interval | |
11 | + | |
12 | + if failure_mode_thres == failure_mode_check_past_n: | |
13 | + self.switching_fail_consecutive_mode = True | |
14 | + else: | |
15 | + self.switching_fail_consecutive_mode = False | |
16 | + if normal_mode_thres == normal_mode_check_past_n: | |
17 | + self.switching_normal_consecutive_mode = True | |
18 | + else | |
19 | + self.switching_normal_consecutive_mode = False | |
20 | + | |
21 | + self.failure_mode_thres = failure_mode_thres | |
22 | + self.failure_mode_check_past_n = failure_mode_check_past_n | |
23 | + self.normal_mode_thres = normal_mode_thres | |
24 | + | |
25 | + def __setitem__(self, key): | |
26 | + if key not in self.sources: | |
27 | + self.sources[key] = { | |
28 | + "status_counts": [], | |
29 | + "ok_counts": 0, | |
30 | + "force_send_mode": False | |
31 | + } | |
32 | + else : | |
33 | + raise "Error! Attempting to access that has no key!" | |
34 | + # Update logic here if needed | |
35 | + | |
36 | + def __getitem__(self, key): | |
37 | + return self.sources[key] | |
38 | + | |
39 | + def add_status(self, source, status): | |
40 | + assert status == "OK" or status == "FAIL" , f"Invalid status was given!, status must be one of 'OK' or 'FAIL', but given '{status}'!" | |
41 | + | |
42 | + if source not in self.sources: | |
43 | + self[source] = {} # Initializes source if not existing | |
44 | + self.sources[source]["status_counts"].append(status) | |
45 | + if len(self.sources[source]["status_counts"]) > self.buffer_size): | |
46 | + self.sources[source]["status_counts"].pop(0) | |
47 | + | |
48 | + # Your existing logic for updating counts and checking statuses | |
49 | + if status == 'OK': | |
50 | + self.sources[source]["ok_counts"] += 1 | |
51 | + if self.sources[source]["force_send_mode"] and self.sources[source]["ok_counts"] >= self.normal_mode_thres: | |
52 | + self.sources[source]["force_send_mode"] = False | |
53 | + self.send_message(source, "OK SEND") | |
54 | + else: | |
55 | + self.sources[source]["ok_counts"] = 0 # Reset on FAIL | |
56 | + self.check_failures(source) | |
57 | + | |
58 | + def check_failures(self, source): | |
59 | + if self.switching_fail_consecutive_mode: | |
60 | + if len(self.sources[source]["status_counts"]) >= failure_mode_thres and all(status == 'FAIL' for status in self.sources[source]["status_counts"][-failure_mode_thres:]): | |
61 | + print(f"Source {source} has 5 consecutive FAILs!") | |
62 | + self.sources[source]["force_send_mode"] = True | |
63 | + self.send_message(source, "FORCE SEND") | |
64 | + else : | |
65 | + pass | |
66 | + | |
67 | + def send_message(self, source, message_type): | |
68 | + print(f"Sending message for {source} - Status: {message_type}") | |
69 | + # Reset the count after sending message | |
70 | + self.sources[source]["ok_counts"] = 0 | |
71 | + |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?