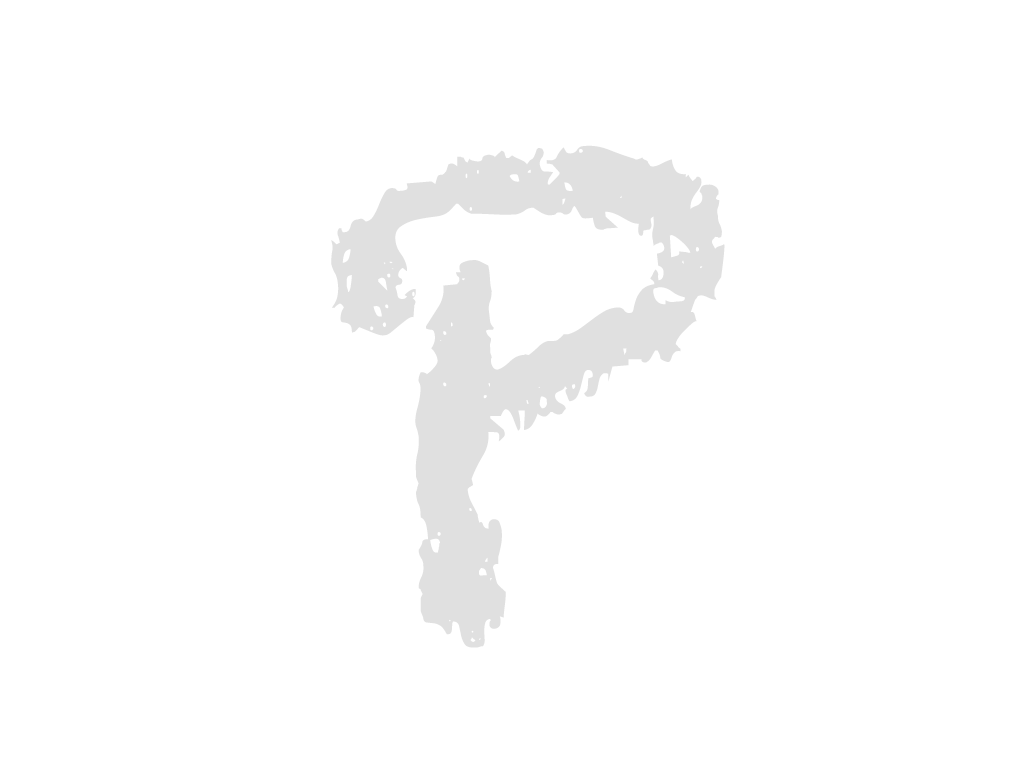
--- yoloseg/inference_.py
+++ yoloseg/inference_.py
... | ... | @@ -207,7 +207,7 @@ |
207 | 207 |
|
208 | 208 |
# Path to your ONNX model and classes text file |
209 | 209 |
model_path = 'yoloseg/weight/best.onnx' |
210 |
- classes_txt_file = 'yoloseg/config_files/yolo_config.txt' |
|
210 |
+ classes_txt_file = 'config_files/yolo_config.txt' |
|
211 | 211 |
image_path = 'yoloseg/img3.jpg' |
212 | 212 |
|
213 | 213 |
model_input_shape = (640, 640) |
... | ... | @@ -254,50 +254,51 @@ |
254 | 254 |
cv2.waitKey(0) |
255 | 255 |
cv2.destroyAllWindows() |
256 | 256 |
|
257 |
-def test2(): |
|
258 |
- import time |
|
259 |
- import glob |
|
260 |
- |
|
261 |
- # Path to your ONNX model and classes text file |
|
262 |
- model_path = 'yoloseg/weight/best.onnx' |
|
263 |
- classes_txt_file = 'yoloseg/config_files/yolo_config.txt' |
|
264 |
- |
|
265 |
- model_input_shape = (640, 640) |
|
266 |
- inference_engine = Inference( |
|
267 |
- onnx_model_path=model_path, |
|
268 |
- model_input_shape=model_input_shape, |
|
269 |
- classes_txt_file=classes_txt_file, |
|
270 |
- run_with_cuda=True |
|
271 |
- ) |
|
272 |
- |
|
273 |
- image_dir = glob.glob("/home/juni/사진/sample_data/ex1/*.png") |
|
274 |
- |
|
275 |
- for iteration, image_path in enumerate(image_dir): |
|
276 |
- img = cv2.imread(image_path) |
|
277 |
- if img is None: |
|
278 |
- print("Error loading image_binary") |
|
279 |
- return |
|
280 |
- img = cv2.resize(img, model_input_shape) |
|
281 |
- # Run inference |
|
282 |
- t1 = time.time() |
|
283 |
- detections, mask_maps = inference_engine.run_inference(img) |
|
284 |
- t2 = time.time() |
|
285 |
- |
|
286 |
- print(t2-t1) |
|
287 |
- |
|
288 |
- # Display results |
|
289 |
- # for detection in detections: |
|
290 |
- # x, y, w, h = detection['box'] |
|
291 |
- # class_name = detection['class_name'] |
|
292 |
- # confidence = detection['confidence'] |
|
293 |
- # cv2.rectangle(img, (x, y), (x+w, y+h), detection['color'], 2) |
|
294 |
- # label = f"{class_name}: {confidence:.2f}" |
|
295 |
- # cv2.putText(img, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, detection['color'], 2) |
|
296 |
- # |
|
297 |
- # if len(mask_maps) > 0 : |
|
298 |
- # seg_image = overlay_mask(img, mask_maps[0], color=(0, 255, 0), alpha=0.3) |
|
299 |
- # cv2.imwrite(f"result/{iteration}.png", seg_image) |
|
257 |
+# def test2(): |
|
258 |
+# import time |
|
259 |
+# import glob |
|
260 |
+# |
|
261 |
+# # Path to your ONNX model and classes text file |
|
262 |
+# model_path = 'yoloseg/weight/best.onnx' |
|
263 |
+# classes_txt_file = 'config_files/yolo_config.txt' |
|
264 |
+# |
|
265 |
+# model_input_shape = (640, 640) |
|
266 |
+# inference_engine = Inference( |
|
267 |
+# onnx_model_path=model_path, |
|
268 |
+# model_input_shape=model_input_shape, |
|
269 |
+# classes_txt_file=classes_txt_file, |
|
270 |
+# run_with_cuda=True |
|
271 |
+# ) |
|
272 |
+# |
|
273 |
+# image_dir = glob.glob("/home/juni/사진/sample_data/ex1/*.png") |
|
274 |
+# |
|
275 |
+# for iteration, image_path in enumerate(image_dir): |
|
276 |
+# img = cv2.imread(image_path) |
|
277 |
+# if img is None: |
|
278 |
+# print("Error loading image_binary") |
|
279 |
+# return |
|
280 |
+# img = cv2.resize(img, model_input_shape) |
|
281 |
+# # Run inference |
|
282 |
+# t1 = time.time() |
|
283 |
+# detections, mask_maps = inference_engine.run_inference(img) |
|
284 |
+# t2 = time.time() |
|
285 |
+# |
|
286 |
+# print(t2-t1) |
|
287 |
+# |
|
288 |
+# # Display results |
|
289 |
+# # for detection in detections: |
|
290 |
+# # x, y, w, h = detection['box'] |
|
291 |
+# # class_name = detection['class_name'] |
|
292 |
+# # confidence = detection['confidence'] |
|
293 |
+# # cv2.rectangle(img, (x, y), (x+w, y+h), detection['color'], 2) |
|
294 |
+# # label = f"{class_name}: {confidence:.2f}" |
|
295 |
+# # cv2.putText(img, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, detection['color'], 2) |
|
296 |
+# # |
|
297 |
+# if len(mask_maps) > 0 : |
|
298 |
+# seg_image = overlay_mask(img, mask_maps[0], color=(0, 255, 0), alpha=0.3) |
|
299 |
+# cv2.imwrite(f"result/{iteration}.png", seg_image) |
|
300 | 300 |
|
301 | 301 |
|
302 | 302 |
if __name__ == "__main__": |
303 |
- test2()(파일 끝에 줄바꿈 문자 없음) |
|
303 |
+ pass |
|
304 |
+ # test2()(파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?