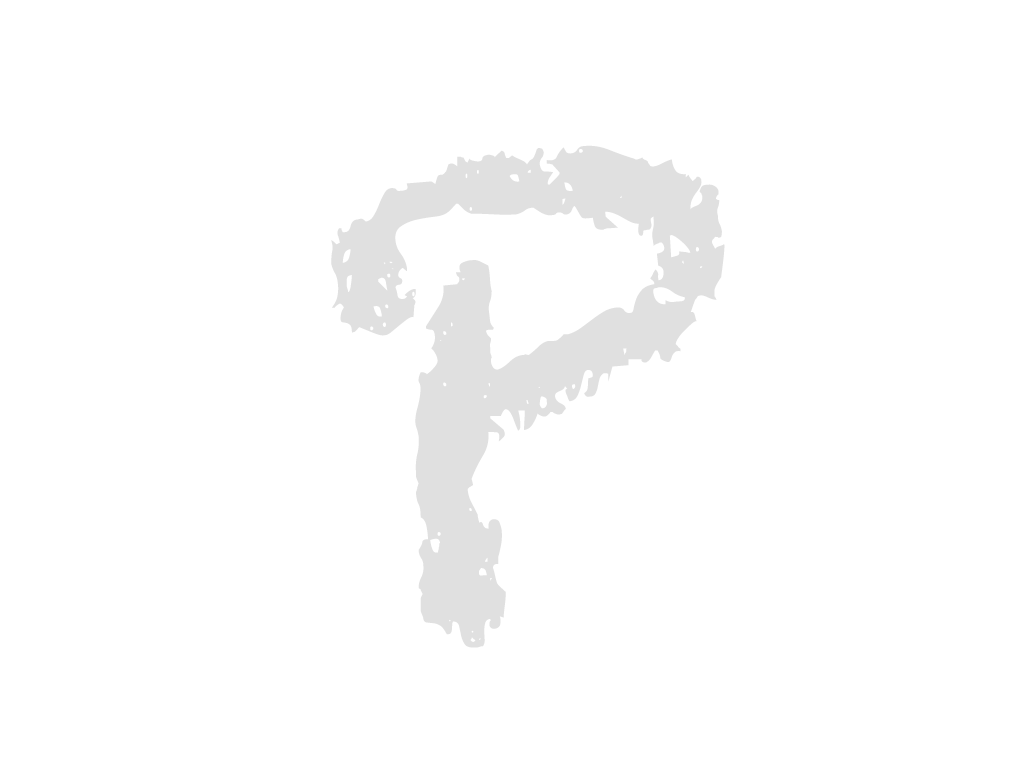
--- hls_streaming/hls.py
+++ hls_streaming/hls.py
... | ... | @@ -9,6 +9,7 @@ |
9 | 9 |
from threading import Lock, Thread, Event |
10 | 10 |
|
11 | 11 |
|
12 |
+ |
|
12 | 13 |
class FrameCapturer: |
13 | 14 |
def __init__( |
14 | 15 |
self, |
... | ... | @@ -46,7 +47,7 @@ |
46 | 47 |
self.video_stream = next(s for s in self.input_stream.streams if s.type == 'video') |
47 | 48 |
self.fps = self.video_stream.guessed_rate.numerator |
48 | 49 |
self.capture_interval = 1 / self.fps |
49 |
- |
|
50 |
+ print(cctv_name) |
|
50 | 51 |
self.cctvid = cctv_name |
51 | 52 |
self.time_zone = ZoneInfo(time_zone) |
52 | 53 |
self.endpoint = endpoint |
... | ... | @@ -82,13 +83,13 @@ |
82 | 83 |
# print(len(self.frame_buffer)) |
83 | 84 |
self.current_frame = buffered_frame.to_image() |
84 | 85 |
self.current_frame = cv2.cvtColor(np.array(self.current_frame), cv2.COLOR_RGB2BGR) |
85 |
- frame_name = f"captured_frame_{self.captured_frame_count}.jpg" |
|
86 |
+ frame_name = f"captured_frame_{self.cctvid}_{self.captured_frame_count}.png" |
|
86 | 87 |
img_binary = cv2.imencode('.png', self.current_frame) |
87 | 88 |
img_binary = img_binary[1].tobytes() |
88 | 89 |
self.send_image_to_server(img_binary) |
89 |
- cv2.imwrite(f'hls_streaming/captured_frame_/{datetime.now()}_{frame_name}', self.current_frame) |
|
90 |
+ cv2.imwrite(f'hls_streaming/captured_frame_/{self.cctvid}_{datetime.now()}_{frame_name}', self.current_frame) |
|
90 | 91 |
self.last_capture_time = current_time |
91 |
- print(f"Captured {frame_name} at time: {current_time - self.start_time:.2f}s") |
|
92 |
+ print(f"Captured {frame_name} of {self.cctvid} at time: {current_time - self.start_time:.2f}s") |
|
92 | 93 |
self.captured_frame_count +=1 |
93 | 94 |
|
94 | 95 |
time.sleep(0.1) |
... | ... | @@ -99,8 +100,8 @@ |
99 | 100 |
'Content-Type': f'image/{image_type}', |
100 | 101 |
'x-time-sent': time_sent, |
101 | 102 |
'x-cctv-info': str(self.cctvid), |
102 |
- 'x-cctv-latitude' : self.lat, |
|
103 |
- 'x-cctv-longitude' : self.lon, |
|
103 |
+ 'x-cctv-latitude' : str(self.lat), |
|
104 |
+ 'x-cctv-longitude' : str(self.lon), |
|
104 | 105 |
} |
105 | 106 |
session = requests.Session() |
106 | 107 |
try: |
... | ... | @@ -132,20 +133,20 @@ |
132 | 133 |
self.input_stream.close() |
133 | 134 |
|
134 | 135 |
|
135 |
-# Example usage |
|
136 |
-if __name__ == "__main__": |
|
137 |
- capturer = FrameCapturer( |
|
138 |
- 'http://cctvsec.ktict.co.kr/5545/LFkDslDT81tcSYh3G4306+mcGlLb3yShF9rx2vcPfltwUL4+I950kcBlD15uWm6K0cKCtAMlxsIptMkCDo5lGQiLlARP+SyUloz8vIMNB18=', |
|
139 |
- 101, 10, 5 |
|
140 |
- ) |
|
141 |
- t1 = time.time() |
|
142 |
- try: |
|
143 |
- capturer.start() |
|
144 |
- time.sleep(600000) |
|
145 |
- finally: |
|
146 |
- capturer.stop() |
|
147 |
- del capturer |
|
148 |
- t2 = time.time() |
|
149 |
- with open("result.txt", "w") as file: |
|
150 |
- file.write(f'{t2-t1} seconds before terminating') |
|
151 |
- exit() |
|
136 |
+# # Example usage |
|
137 |
+# if __name__ == "__main__": |
|
138 |
+# capturer = FrameCapturer( |
|
139 |
+# 'http://cctvsec.ktict.co.kr/5545/LFkDslDT81tcSYh3G4306+mcGlLb3yShF9rx2vcPfltwUL4+I950kcBlD15uWm6K0cKCtAMlxsIptMkCDo5lGQiLlARP+SyUloz8vIMNB18=', |
|
140 |
+# 101, 10, 5 |
|
141 |
+# ) |
|
142 |
+# t1 = time.time() |
|
143 |
+# try: |
|
144 |
+# capturer.start() |
|
145 |
+# time.sleep(600000) |
|
146 |
+# finally: |
|
147 |
+# capturer.stop() |
|
148 |
+# del capturer |
|
149 |
+# t2 = time.time() |
|
150 |
+# with open("result.txt", "w") as file: |
|
151 |
+# file.write(f'{t2-t1} seconds before terminating') |
|
152 |
+# exit() |
--- run_image_anal_backend.sh
+++ run_image_anal_backend.sh
... | ... | @@ -6,9 +6,9 @@ |
6 | 6 |
PYTHONPATH="/home/juni/PycharmProjects/segmentation_overlapping" |
7 | 7 |
|
8 | 8 |
# Start multiple Python processes in the background |
9 |
-python hls_streaming/hls.py --cctv_num 0 & |
|
9 |
+python streaming_process.py --cctv_num 0 & |
|
10 | 10 |
pids+=($!) |
11 |
-python hls_streaming/hls.py --cctv_num 1 & |
|
11 |
+python streaming_process.py --cctv_num 1 & |
|
12 | 12 |
pids+=($!) |
13 | 13 |
|
14 | 14 |
python test.py |
--- hls_streaming/streaming_process.py
+++ streaming_process.py
... | ... | @@ -3,13 +3,6 @@ |
3 | 3 |
# This is by design, and is a way to circumvent GIL of python, because the server needs to handle multiple video streaming at once. |
4 | 4 |
|
5 | 5 |
if __name__ == "__main__": |
6 |
- from dotenv import load_dotenv |
|
7 |
- import os |
|
8 |
- |
|
9 |
- load_dotenv() |
|
10 |
- # print("PYTHONPATH:", os.getenv("PYTHONPATH")) |
|
11 |
- # print("_____") |
|
12 |
- # print(os.path.dirname(os.path.abspath(__file__))) |
|
13 | 6 |
from ITS.api import gather_cctv_list |
14 | 7 |
from hls_streaming.hls import FrameCapturer |
15 | 8 |
|
... | ... | @@ -33,7 +26,7 @@ |
33 | 26 |
cctv_ind = args.cctv_num |
34 | 27 |
|
35 | 28 |
cctv_info = pd.read_csv("config/cctv_list.csv") |
36 |
- cctv_info = cctv_info.iloc[0] |
|
29 |
+ cctv_info = cctv_info.iloc[cctv_ind] |
|
37 | 30 |
|
38 | 31 |
lat = cctv_info["coordx"] |
39 | 32 |
lon = cctv_info["coordy"] |
... | ... | @@ -47,6 +40,7 @@ |
47 | 40 |
while True: |
48 | 41 |
|
49 | 42 |
cctv_url = refresh_hls_address(lat, lon, ) |
43 |
+ print(name) |
|
50 | 44 |
hls_streaming = FrameCapturer(cctv_url, name, lat, lon, 5, 15, 300, "Asia/Seoul", |
51 | 45 |
endpoint= "http://localhost:12345/cctv/infer") |
52 | 46 |
hls_streaming.start() |
--- test.py
+++ test.py
... | ... | @@ -2,7 +2,7 @@ |
2 | 2 |
from flask_restx import Api, Resource, fields |
3 | 3 |
import os |
4 | 4 |
from datetime import datetime |
5 |
-from yoloseg.inference_ import Inference |
|
5 |
+# from yoloseg.inference_ import Inference |
|
6 | 6 |
|
7 | 7 |
app = Flask(__name__) |
8 | 8 |
api = Api(app, version='1.0', title='CCTV Image Upload API', |
... | ... | @@ -35,6 +35,7 @@ |
35 | 35 |
ns.abort(400, 'No image part in the request') |
36 | 36 |
image = request.files['file'] |
37 | 37 |
cctv_info = request.headers.get('x-cctv-info', '') |
38 |
+ print(cctv_info) |
|
38 | 39 |
time_sent = request.headers.get('x-time-sent', '') |
39 | 40 |
cctv_latitude = request.headers.get('x-cctv-latitude', 'Not provided') |
40 | 41 |
cctv_longitude = request.headers.get('x-cctv-longitude', 'Not provided') |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?