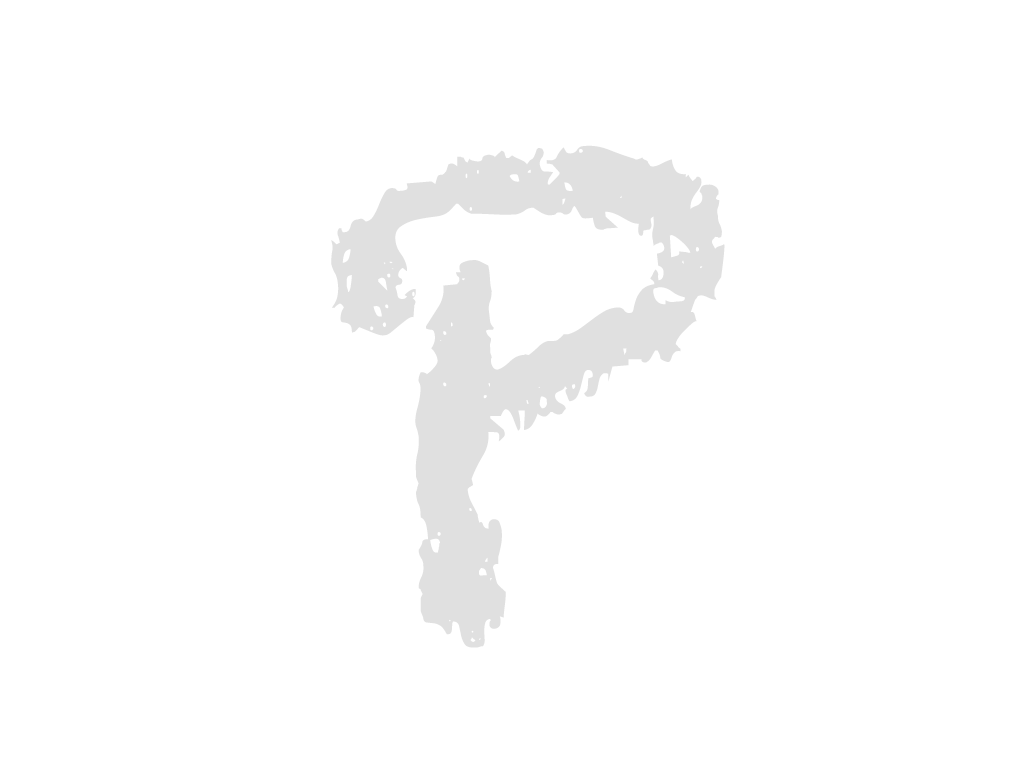
+++ tools/algo/ARIMA.py
... | ... | @@ -0,0 +1,2 @@ |
1 | +import numpy as np | |
2 | +import pandas as pd(파일 끝에 줄바꿈 문자 없음) |
--- tools/algo/interpolation.py
+++ tools/algo/interpolation.py
... | ... | @@ -1,1 +1,61 @@ |
1 |
-import scipy(파일 끝에 줄바꿈 문자 없음) |
|
1 |
+import numpy as np |
|
2 |
+from scipy.interpolate import CubicSpline |
|
3 |
+from datetime import datetime, timedelta |
|
4 |
+import pandas as pd |
|
5 |
+import matplotlib.pyplot as plt |
|
6 |
+ |
|
7 |
+ |
|
8 |
+def interpolate_value(df, timestamp, col_key, k=12, show_graph=False): |
|
9 |
+ """ |
|
10 |
+ :param df: DataFrame with 'timestamp' and a specified column key. |
|
11 |
+ :param timestamp: String of timestamp in format '%Y%m%d%H%M' to interpolate value for. |
|
12 |
+ :param col_key: The key/column name in df for which value needs to be interpolated. |
|
13 |
+ :param k: Number of hours before and after the target timestamp to be considered for interpolation. |
|
14 |
+ :return: Interpolated value for the given timestamp. |
|
15 |
+ """ |
|
16 |
+ # Convert timestamp to datetime object |
|
17 |
+ target_time = datetime.strptime(timestamp, '%Y%m%d%H%M') |
|
18 |
+ |
|
19 |
+ # Get nearest left 'o clock' time |
|
20 |
+ left_o_clock = target_time.replace(minute=0) |
|
21 |
+ |
|
22 |
+ # Prepare a list of relevant times for interpolation: k before and k after |
|
23 |
+ relevant_times = [left_o_clock + timedelta(hours=i) for i in range(-k, k + 1)] |
|
24 |
+ |
|
25 |
+ # Convert them to string format for matching with the DataFrame |
|
26 |
+ relevant_times_str = [dt.strftime('%Y%m%d%H%M') for dt in relevant_times] |
|
27 |
+ relevant_times_str = np.array(relevant_times_str, dtype=int) |
|
28 |
+ # Extract relevant rows from DataFrame |
|
29 |
+ relevant_df = df[df['관측시각'].isin(relevant_times_str)].sort_values(by='관측시각') |
|
30 |
+ |
|
31 |
+ # Convert datetime to numerical format: -k to k |
|
32 |
+ x = [i for i in range(-k, k+1)] |
|
33 |
+ y = relevant_df[col_key].values |
|
34 |
+ |
|
35 |
+ # Find the x value for the target timestamp |
|
36 |
+ x_target = (target_time - left_o_clock).total_seconds() / 3600 |
|
37 |
+ |
|
38 |
+ # Create a cubic spline interpolation function |
|
39 |
+ cs = CubicSpline(x, y) |
|
40 |
+ |
|
41 |
+ if show_graph: |
|
42 |
+ # For visualization |
|
43 |
+ x_dense = np.linspace(min(x), max(x), 400) # Densely sampled x values |
|
44 |
+ y_dense = cs(x_dense) # Interpolated values |
|
45 |
+ |
|
46 |
+ fig, ax = plt.subplots(figsize=(6.5, 4)) |
|
47 |
+ ax.plot(x, y, 'o', label='Data') |
|
48 |
+ ax.plot(x_dense, y_dense, label='Cubic Spline Interpolation') |
|
49 |
+ ax.axvline(x_target, color='red', linestyle='--', label='Target Timestamp') # Marking the target timestamp |
|
50 |
+ ax.legend() |
|
51 |
+ plt.show() |
|
52 |
+ |
|
53 |
+ # Return the interpolated value at x_target |
|
54 |
+ return cs(x_target) |
|
55 |
+ |
|
56 |
+ |
|
57 |
+if __name__ == '__main__': |
|
58 |
+ df = pd.read_csv("/home/juni/PycharmProjects/failure_analysis/data/weather/202007010000_202308010000.csv", |
|
59 |
+ encoding='utf-8') |
|
60 |
+ timestamp = '202307271015' |
|
61 |
+ print(interpolate_value(df, timestamp, '기온', show_graph=True))(파일 끝에 줄바꿈 문자 없음) |
--- tools/visualizer/visualizer.py
+++ tools/visualizer/visualizer.py
... | ... | @@ -2,4 +2,26 @@ |
2 | 2 |
import pandas as pd |
3 | 3 |
import plotly.graph_objects as go |
4 | 4 |
|
5 |
+def parse_gcode(file_path): |
|
6 |
+ movements = [] |
|
7 |
+ with open(file_path, 'r') as file: |
|
8 |
+ for line in file: |
|
9 |
+ # (parse the line to extract commands and parameters) |
|
10 |
+ cmd, x, y, z = ... # Simplified for brevity |
|
11 |
+ movements.append((cmd, x, y, z)) |
|
12 |
+ return movements |
|
5 | 13 |
|
14 |
+def plot_gcode(movements): |
|
15 |
+ fig, ax = plt.subplots() |
|
16 |
+ x_data, y_data = [], [] |
|
17 |
+ |
|
18 |
+ for cmd, x, y, z in movements: |
|
19 |
+ if cmd in ['G0', 'G1']: |
|
20 |
+ x_data.append(x) |
|
21 |
+ y_data.append(y) |
|
22 |
+ |
|
23 |
+ ax.plot(x_data, y_data) |
|
24 |
+ plt.show() |
|
25 |
+ |
|
26 |
+movements = parse_gcode('path_to_gcode_file.gcode') |
|
27 |
+plot_gcode(movements)(파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?