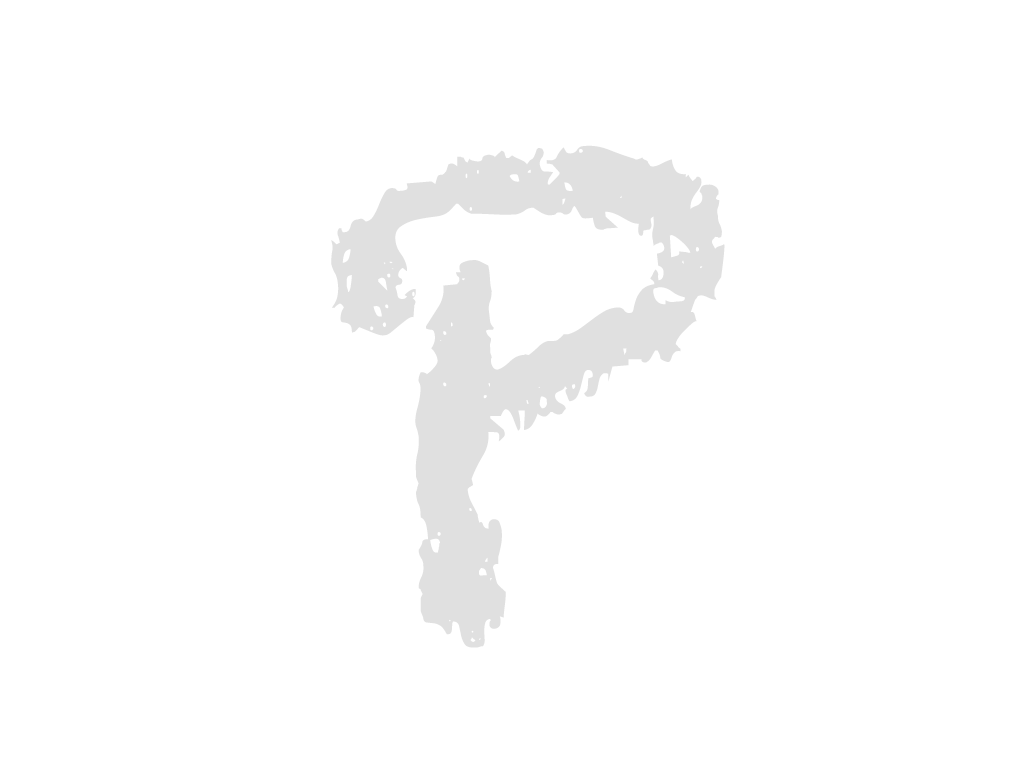
completed functionality, but flask interface needs to be done
@a6f036ff4c32ea16c37cc623c3c56e07c512e448
+++ app.py
... | ... | @@ -0,0 +1,24 @@ |
1 | +from flask import Flask | |
2 | +from flask_restx import Api | |
3 | +from auth import Auth | |
4 | +from action import Action | |
5 | + | |
6 | +app = Flask(__name__) | |
7 | + | |
8 | +print("Api Start") | |
9 | +api = Api( app, | |
10 | + version='0.1', | |
11 | + title="trafficagent", | |
12 | + description="API Server", | |
13 | + terms_url="/", | |
14 | + contact="[email protected]", | |
15 | + license="MIT") | |
16 | + | |
17 | +api.add_namespace(Auth, '/auth') | |
18 | +print("Api Add Auth") | |
19 | + | |
20 | +api.add_namespace(Action, '/action') | |
21 | + | |
22 | +if __name__ == "__main__": | |
23 | + app.run(debug=False, host='0.0.0.0', port=8080) | |
24 | + print("Flask Start")(파일 끝에 줄바꿈 문자 없음) |
--- data/weather/202007010000_202308010000.csv
+++ data/weather/202007010000_202308310000_f.csv
This diff is too big to display. |
--- tools/algo/ARIMA.py
+++ tools/algo/ARIMA.py
... | ... | @@ -1,2 +1,53 @@ |
1 | 1 |
import numpy as np |
2 |
-import pandas as pd(파일 끝에 줄바꿈 문자 없음) |
|
2 |
+import pandas as pd |
|
3 |
+from statsmodels.tsa.statespace.sarimax import SARIMAX |
|
4 |
+import matplotlib.pyplot as plt |
|
5 |
+from statsmodels.graphics.tsaplots import plot_acf, plot_pacf |
|
6 |
+import plotly.graph_objects as go |
|
7 |
+from scipy import signal |
|
8 |
+from datetime import datetime |
|
9 |
+import plotly.express as px |
|
10 |
+from tools.algo.humidity import absolute_humidity |
|
11 |
+ |
|
12 |
+ |
|
13 |
+if __name__ == "__main__": |
|
14 |
+ df = pd.read_csv("/home/juni/PycharmProjects/failure_analysis/data/weather/202007010000_202308310000_f.csv") |
|
15 |
+ ah = absolute_humidity(df["상대습도"], df["기온"]) |
|
16 |
+ df['관측시각'] = df['관측시각'].apply(lambda x: datetime.strptime(f"{x}", '%Y%m%d%H%M')) |
|
17 |
+ df["절대습도"] = ah |
|
18 |
+ # fig = go.Figure() |
|
19 |
+ # |
|
20 |
+ # fig.add_trace( |
|
21 |
+ # go.Scatter(x=df["관측시각"], y=df["절대습도"]) |
|
22 |
+ # ) |
|
23 |
+ # fig.add_trace( |
|
24 |
+ # go.Scatter(x=df["관측시각"], y=signal.savgol_filter( |
|
25 |
+ # df["절대습도"],72,3) |
|
26 |
+ # )) |
|
27 |
+ # fig.show() |
|
28 |
+ # log_df = np.log(df["절대습도"]) |
|
29 |
+ diff_1 = (df["절대습도"].diff(periods=1).iloc[1:]) |
|
30 |
+ diff_2 = diff_1.diff(periods=1).iloc[1:] |
|
31 |
+ # plot_acf(diff_2) |
|
32 |
+ # plot_pacf(diff_2) |
|
33 |
+ plt.show() |
|
34 |
+ model = SARIMAX(df["절대습도"], order=(1,0,2), seasonal_order=(0,1,2,24)) |
|
35 |
+ model_fit = model.fit() |
|
36 |
+ # ARIMA_model = pm.auto_arima(df['절대습도'], |
|
37 |
+ # start_p=1, |
|
38 |
+ # start_q=1, |
|
39 |
+ # test='adf', # use adftest to find optimal 'd' |
|
40 |
+ # max_p=3, max_q=3, # maximum p and q |
|
41 |
+ # m=24, # frequency of series (if m==1, seasonal is set to FALSE automatically) |
|
42 |
+ # d=None, # let model determine 'd' |
|
43 |
+ # D=2, #order of the seasonal differencing |
|
44 |
+ # seasonal=True, # No Seasonality for standard ARIMA |
|
45 |
+ # trace=False, # logs |
|
46 |
+ # error_action='warn', # shows errors ('ignore' silences these) |
|
47 |
+ # suppress_warnings=False, |
|
48 |
+ # stepwise=True) |
|
49 |
+ print(model_fit.summary()) |
|
50 |
+ df['forecast'] = model_fit.predict(start=-100, end=-1, dynamic=True) |
|
51 |
+ # df[['절대습도', 'forecast']].plot(figsize=(12, 8)) |
|
52 |
+ fig = px.line(df[['절대습도', 'forecast']]) |
|
53 |
+ fig.show()(파일 끝에 줄바꿈 문자 없음) |
+++ tools/algo/correlation.py
... | ... | @@ -0,0 +1,39 @@ |
1 | +import numpy as np | |
2 | +import pandas as pd | |
3 | +from scipy.stats import pearsonr, spearmanr, kendalltau, pointbiserialr | |
4 | +import plotly.express as px | |
5 | +import random | |
6 | + | |
7 | +from tools.algo.interpolation import interpolate_value | |
8 | +from tools.algo.humidity import absolute_humidity | |
9 | + | |
10 | +if __name__ == "__main__": | |
11 | + df_weather = pd.read_csv("/home/juni/PycharmProjects/failure_analysis/data/weather/202007010000_202308310000_f.csv") | |
12 | + df_failure = pd.read_excel("/home/juni/PycharmProjects/failure_analysis/data/failure/대옹 작업내용.xlsx") | |
13 | + times = df_failure["작업 시작 시간"] | |
14 | + temp = [] | |
15 | + humidity = [] | |
16 | + i=0 | |
17 | + for time in times: | |
18 | + temp.append(interpolate_value(df_weather, timestamp=time, col_key="기온", k=6)) | |
19 | + humidity.append(interpolate_value(df_weather, timestamp=time, col_key="상대습도", k=6)) | |
20 | + | |
21 | + absolute_humidity = absolute_humidity(humidity, temp) | |
22 | + | |
23 | + df_failure["기온"] = temp | |
24 | + df_failure["상대습도"] = humidity | |
25 | + df_failure["절대습도"] = absolute_humidity | |
26 | + df_failure['불량 여부'] = np.where(df_failure['불량 여부'] == 'F', 0, df_failure['불량 여부']) | |
27 | + df_failure['불량 여부'] = np.where(df_failure['불량 여부'] == 'T', 1, df_failure['불량 여부']) | |
28 | + | |
29 | + | |
30 | + df = df_failure.copy() | |
31 | + correlation_manufacturing_abhumidity = pointbiserialr(df["절대습도"], df["불량 여부"]) | |
32 | + correlation_manufacturing_rehumidity = pointbiserialr(df["상대습도"], df["불량 여부"]) | |
33 | + correlation_manufacturing_temp = pointbiserialr(df["기온"], df["불량 여부"]) | |
34 | + print(correlation_manufacturing_abhumidity) | |
35 | + print(correlation_manufacturing_rehumidity) | |
36 | + print(correlation_manufacturing_temp) | |
37 | + | |
38 | + df_failure.to_csv("merged_data.csv") | |
39 | + pass(파일 끝에 줄바꿈 문자 없음) |
--- tools/algo/humidity.py
+++ tools/algo/humidity.py
... | ... | @@ -7,11 +7,14 @@ |
7 | 7 |
return saturation_vapor_pressure * 1000 # KPa -> Pa |
8 | 8 |
|
9 | 9 |
def absolute_humidity(relative_humidity, temperature): |
10 |
+ relative_humidity = np.array(relative_humidity) |
|
11 |
+ temperature = np.array(temperature) |
|
10 | 12 |
saturation_vapor_pressure = buck_equation(temperature) |
11 | 13 |
# 461.5/Kg Kelvin is specific gas constatnt |
12 | 14 |
return saturation_vapor_pressure * relative_humidity * 0.01 /(461.5 * (temperature + 273.15) ) # g/m^3 |
13 | 15 |
|
14 |
-df = pd.read_csv('data/202007010000_202308010000.csv') |
|
16 |
+if __name__ == "__main__": |
|
17 |
+ df = pd.read_csv('data/202007010000_202308010000.csv') |
|
15 | 18 |
|
16 | 19 |
# 절대 습도 구하기 |
17 | 20 |
# interpolation을 통해 시간 사이의 온습도 구하기 |
--- tools/algo/interpolation.py
+++ tools/algo/interpolation.py
... | ... | @@ -14,7 +14,10 @@ |
14 | 14 |
:return: Interpolated value for the given timestamp. |
15 | 15 |
""" |
16 | 16 |
# Convert timestamp to datetime object |
17 |
- target_time = datetime.strptime(timestamp, '%Y%m%d%H%M') |
|
17 |
+ if type(timestamp) is str: |
|
18 |
+ target_time = datetime.strptime(timestamp, '%Y%m%d%H%M') |
|
19 |
+ else: |
|
20 |
+ target_time = timestamp |
|
18 | 21 |
|
19 | 22 |
# Get nearest left 'o clock' time |
20 | 23 |
left_o_clock = target_time.replace(minute=0) |
... | ... | @@ -30,7 +33,7 @@ |
30 | 33 |
|
31 | 34 |
# Convert datetime to numerical format: -k to k |
32 | 35 |
x = [i for i in range(-k, k+1)] |
33 |
- y = relevant_df[col_key].values |
|
36 |
+ y = relevant_df[col_key] |
|
34 | 37 |
|
35 | 38 |
# Find the x value for the target timestamp |
36 | 39 |
x_target = (target_time - left_o_clock).total_seconds() / 3600 |
... | ... | @@ -55,7 +58,7 @@ |
55 | 58 |
|
56 | 59 |
|
57 | 60 |
if __name__ == '__main__': |
58 |
- df = pd.read_csv("/home/juni/PycharmProjects/failure_analysis/data/weather/202007010000_202308010000.csv", |
|
61 |
+ df = pd.read_csv("/home/juni/PycharmProjects/failure_analysis/data/weather/202007010000_202308310000_f.csv", |
|
59 | 62 |
encoding='utf-8') |
60 | 63 |
timestamp = '202307271015' |
61 | 64 |
print(interpolate_value(df, timestamp, '기온', show_graph=True))(파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?