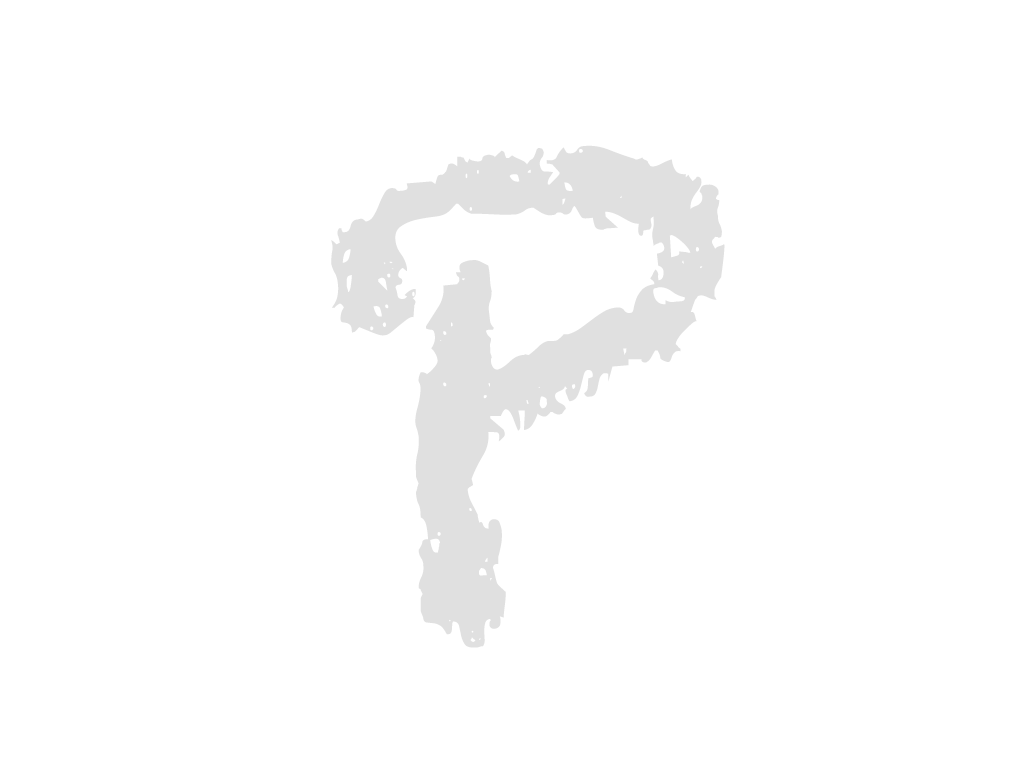
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React, { useState } from 'react';
import { View, TextInput, Text, TouchableOpacity, Alert, StyleSheet } from 'react-native';
import Api from '../api/ApiUtils';
import AsyncStorage from '@react-native-async-storage/async-storage';
import { useNavigation } from '@react-navigation/native';
const LoginScreen = () => {
const [userId, setUserId] = useState('');
const [password, setPassword] = useState('');
const navigation = useNavigation();
const handleLogin = async () => {
const credentials = {
id: userId,
password: password,
};
try {
const response = await Api.login(credentials);
console.log(response);
if(response.result === 'success'){
await AsyncStorage.setItem('token', JSON.stringify({accessToken:response.token}));
await AsyncStorage.setItem('user_id', credentials.id);
navigation.navigate('Selection');
}else{
Alert.alert('로그인 정보를 다시 한번 확인해주세요.');
}
} catch (error) {
Alert.alert('로그인 실패', error.message);
}
};
return (
<View style={styles.container}>
<Text style={styles.logo}>로그인</Text>
<TextInput
style={styles.input}
placeholder="아이디"
value={userId}
onChangeText={setUserId}
/>
<TextInput
style={styles.input}
placeholder="비밀번호"
secureTextEntry
value={password}
onChangeText={setPassword}
/>
<TouchableOpacity style={styles.button} onPress={handleLogin}>
<Text style={styles.buttonText}>로그인</Text>
</TouchableOpacity>
<View style={styles.footer}>
<TouchableOpacity
onPress={() => navigation.navigate('Agreement')}
>
<Text style={styles.footerText}>회원가입</Text>
</TouchableOpacity>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
padding: 20,
backgroundColor: '#F7F7F7',
},
logo: {
fontSize: 28,
color: '#007AFF',
fontWeight: 'bold',
marginBottom: 30,
textAlign: 'center',
},
input: {
height: 50,
borderColor: '#CED4DA',
borderWidth: 1,
borderRadius: 10,
paddingHorizontal: 15,
marginBottom: 15,
backgroundColor: '#FFFFFF',
fontSize: 16,
},
button: {
backgroundColor: '#007AFF',
paddingVertical: 15,
borderRadius: 10,
alignItems: 'center',
marginBottom: 20,
},
buttonText: {
color: '#FFFFFF',
fontSize: 16,
fontWeight: 'bold',
},
footer: {
alignItems: 'center',
marginTop: 20,
},
footerText: {
fontSize: 16,
color: '#666',
marginBottom: 10,
},
});
export default LoginScreen;