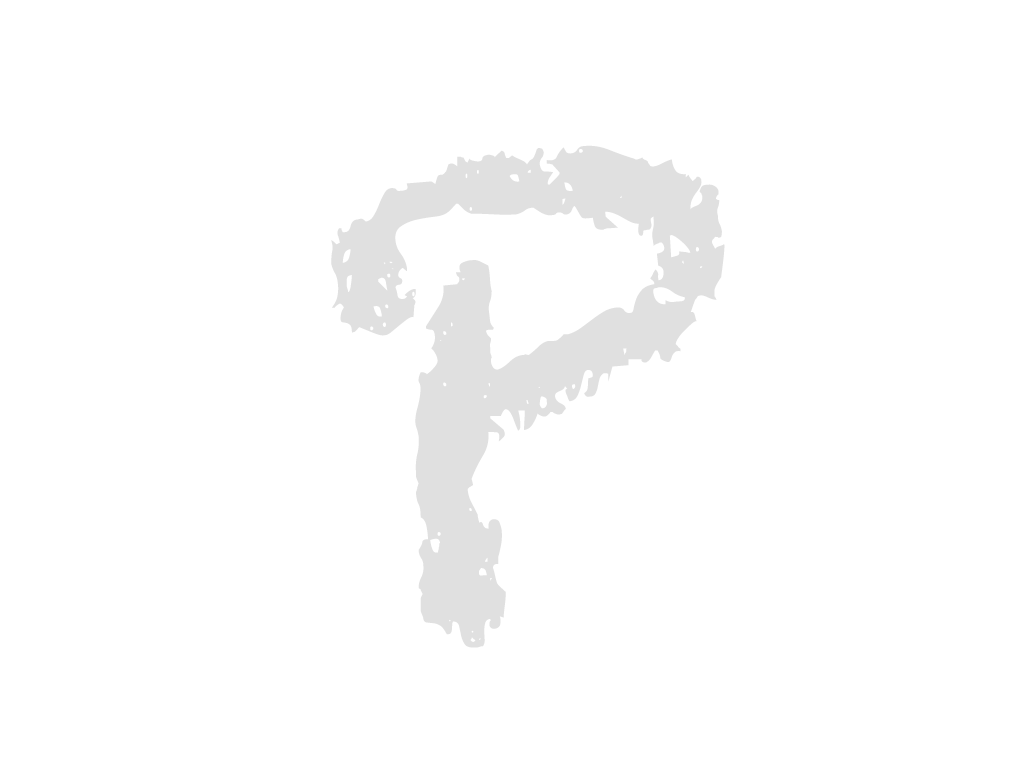
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import { StyleSheet, View, Text, Image } from 'react-native';
import React from 'react';
import AnalysisComponent from '../component/AnalysisComponent';
// 더미데이터
const data = {
score: 95,
distance: 36,
time: 100,
};
export default function AnalysisScreen() {
return (
<View style={styles.container}>
<Score score={data.score} distance={data.distance} time={data.time} />
<View style={styles.totalBoxView}>
<TotalBox distance={data.distance} />
<TotalBox time={data.time} />
</View>
<View style={styles.summaryContainer}>
<AnalysisComponent />
</View>
</View>
);
}
// 운행 점수 컴포넌트
function Score({ score }) {
return (
<View style={styles.scoreContainer}>
<View style={styles.scoreTextBox}>
<Text style={styles.scoreText}>'이용자'님의 운행 점수</Text>
<Text style={styles.score}>
<Text style={styles.boldText}>{score}</Text> 점
</Text>
</View>
<Image source={require('../asset/bicycle.png')} style={styles.image} />
</View>
);
}
// 총 이동거리, 주행시간 컴포넌트
function TotalBox({ distance, time }) {
return (
<View style={styles.totalBoxContainer}>
<View style={styles.totalBoxLabelContainer}>
{distance !== undefined ? (
<>
<Text style={styles.totalBoxLabel}>총 이동거리</Text>
<Text style={styles.totalBoxValue}>
{distance} <Text style={styles.totalBoxLabel}> km</Text>
</Text>
</>
) : (
<>
<Text style={styles.totalBoxLabel}>총 주행시간</Text>
<Text style={styles.totalBoxValue}>
{time} <Text style={styles.totalBoxLabel}> 시간</Text>
</Text>
</>
)}
</View>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#ffffff',
padding: 20,
},
scoreContainer: {
width: '100%',
marginTop: 20,
backgroundColor: '#5488FF',
borderRadius: 10,
paddingVertical: 15,
paddingHorizontal: 20,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'space-between',
},
scoreTextBox: {
flexDirection: 'column',
justifyContent: 'space-between',
},
scoreText: {
fontSize: 20,
color: '#ffffff',
},
boldText: {
fontSize: 65,
fontWeight: 'bold',
},
score: {
color: '#ffffff',
fontSize: 40,
fontWeight: 'bold',
},
image: {
width: 120,
height: 130,
marginLeft: 10,
},
totalBoxView: {
flexDirection: 'row',
justifyContent: 'space-between',
flexWrap: 'wrap',
},
totalBoxContainer: {
marginVertical: 20,
paddingHorizontal: 10,
paddingVertical: 10,
backgroundColor: '#ffffff',
borderRadius: 10,
elevation: 5,
width: '48%',
},
totalBoxLabelContainer: {
flexDirection: 'column',
justifyContent: 'space-between',
alignItems: 'flex-start',
},
totalBoxLabel: {
color: '#000000',
fontSize: 16,
},
totalBoxValue: {
color: '#5488FF',
fontSize: 24,
fontWeight: '600',
textAlign: 'left',
},
summaryContainer: {
flex: 4,
marginTop: 10,
},
});