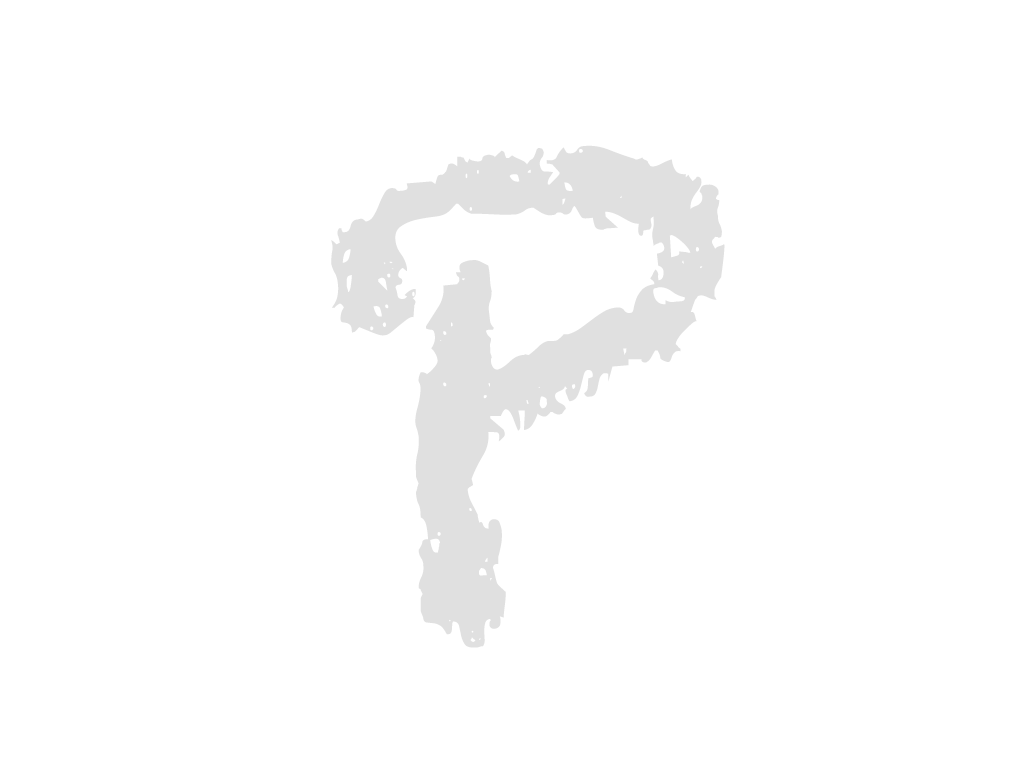
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React, { useState, useEffect } from 'react';
import { View, Text, StyleSheet, Alert, TouchableOpacity } from 'react-native';
import AsyncStorage from '@react-native-async-storage/async-storage';
import crypto from 'crypto-js';
import { startBackgroundTask, stopBackgroundTask, locations } from '../services/LocationService';
import Api from '../api/ApiUtils';
import { useNavigation } from '@react-navigation/native';
import Icon from 'react-native-vector-icons/FontAwesome'; // FontAwesome 아이콘 사용
const GpsScreen = () => {
const [isMeasuring, setIsMeasuring] = useState(false);
const [elapsedTime, setElapsedTime] = useState(0); // 초 단위의 경과 시간
const [tripId, setTripId] = useState(''); // trip_id 상태 추가
const [userId, setUserId] = useState(''); // user_id 상태 추가
const navigation = useNavigation(); // useNavigation 훅으로 navigation 객체 가져오기
useEffect(() => {
let timer;
if (isMeasuring) {
timer = setInterval(() => {
setElapsedTime(prevElapsedTime => prevElapsedTime + 1);
}, 1000); // 1초마다 실행
} else {
clearInterval(timer);
}
return () => {
clearInterval(timer);
};
}, [isMeasuring]);
useEffect(() => {
// 앱이 처음 실행될 때 user_id를 AsyncStorage에서 가져옴
const fetchUserId = async () => {
try {
const storedUserId = await AsyncStorage.getItem('user_id');
if (storedUserId !== null) {
setUserId(storedUserId);
}
} catch (error) {
console.error('Error fetching user_id from AsyncStorage:', error);
}
};
fetchUserId();
}, []);
const generateTripId = () => {
// 해시 값을 생성
const rand = Math.random().toString();
const date = new Date();
const sha256Hash = crypto.SHA256(rand + ',' + date.toString()).toString(crypto.enc.Hex);
return sha256Hash;
};
const handleStart = async () => {
try {
const newTripId = generateTripId();
setTripId(newTripId); // trip_id 상태 업데이트
await startBackgroundTask();
setIsMeasuring(true);
} catch (error) {
console.error('Error starting background task:', error);
Alert.alert('백그라운드 작업 오류', '백그라운드 작업을 시작할 수 없습니다.');
setIsMeasuring(false);
}
};
const handleStop = async () => {
setIsMeasuring(false);
setElapsedTime(0); // 시간 초기화
console.log('Measuring stopped.');
try {
await stopBackgroundTask();
const dataToSend = {
user_id: userId, // AsyncStorage에서 가져온 user_id 사용
trip_id: tripId, // 상태에서 trip_id 가져오기
trip_log: locations,
};
console.log('Data to send:', dataToSend);
// 서버로 전송
try {
const response = await Api.sendTripLog(dataToSend, navigation);
console.log(":::::", response);
} catch (error) {
Alert.alert('로그인 실패', error.message);
}
} catch (error) {
console.error('Error stopping background task:', error);
}
};
const handleLogout = () => {
AsyncStorage.removeItem('token');
navigation.navigate('Login');
Alert.alert('로그아웃', '로그아웃되었습니다.');
};
const handleHistory = () => {
navigation.navigate('Analysis');
Alert.alert('히스토리', '히스토리 화면으로 이동합니다.');
};
// 시간 형식으로 변환
const formatTime = (seconds) => {
const hours = Math.floor(seconds / 3600);
const minutes = Math.floor((seconds % 3600) / 60);
const secs = seconds % 60;
return `${String(hours).padStart(2, '0')}:${String(minutes).padStart(2, '0')}:${String(secs).padStart(2, '0')}`;
};
return (
<View style={styles.container}>
<View style={styles.timerContainer}>
<Text style={styles.time}>{formatTime(elapsedTime)}</Text>
</View>
<View style={styles.buttonRowContainer}>
<TouchableOpacity
style={[styles.button, styles.startButton, isMeasuring && styles.buttonDisabled]}
onPress={handleStart}
disabled={isMeasuring}
>
<Icon name="play" size={20} color="#FFFFFF" />
<Text style={styles.buttonText}>측정 시작</Text>
</TouchableOpacity>
<TouchableOpacity
style={[styles.button, styles.stopButton, !isMeasuring && styles.buttonDisabled]}
onPress={handleStop}
disabled={!isMeasuring}
>
<Icon name="stop" size={20} color="#FFFFFF" />
<Text style={styles.buttonText}>측정 종료</Text>
</TouchableOpacity>
</View>
<View style={styles.fullWidthButtonContainer}>
<TouchableOpacity
style={[styles.fullWidthButton, styles.historyButton]}
onPress={handleHistory}
>
<Text style={styles.fullWidthButtonText}>분석결과</Text>
</TouchableOpacity>
<TouchableOpacity
style={[styles.fullWidthButton, styles.logoutButton]}
onPress={handleLogout}
>
<Text style={styles.blackbuttonText}>로그아웃</Text>
</TouchableOpacity>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
padding: 20,
backgroundColor: '#F7F7F7', // 배경색
},
timerContainer: {
marginVertical: 30,
padding: 20,
borderRadius: 10,
width: '100%',
alignItems: 'center',
},
time: {
fontSize: 68,
fontWeight: 'bold',
color: '#007AFF', // 타이머 색상
},
buttonRowContainer: {
flexDirection: 'row',
width: '100%',
justifyContent: 'space-between',
paddingHorizontal: 20,
marginBottom: 20,
},
button: {
flex: 1,
paddingVertical: 15,
borderRadius: 10,
marginHorizontal: 5,
alignItems: 'center',
justifyContent: 'center', // 중앙 정렬
},
startButton: {
backgroundColor: '#007AFF', // 시작 버튼 색상
},
stopButton: {
backgroundColor: '#FF3B30', // 종료 버튼 색상
},
fullWidthButtonContainer: {
width: '100%',
paddingHorizontal: 20,
},
fullWidthButton: {
paddingVertical: 15,
borderRadius: 10,
marginVertical: 5,
alignItems: 'center',
},
logoutButton: {
backgroundColor: '#eeeeee', // 로그아웃 버튼 색상 (연한 무채색)
},
historyButton: {
backgroundColor: '#CAF4FF', // 분석결과 버튼 색상 (연한 파란색)
},
buttonText: {
color: '#FFFFFF',
fontWeight: 'bold',
fontSize: 16,
},
blackbuttonText: {
fontWeight: 'bold',
fontSize: 16,
},
fullWidthButtonText: {
color:'#007AFF',
fontWeight: 'bold',
fontSize: 16,
},
buttonDisabled: {
opacity: 0.5,
},
});
export default GpsScreen;