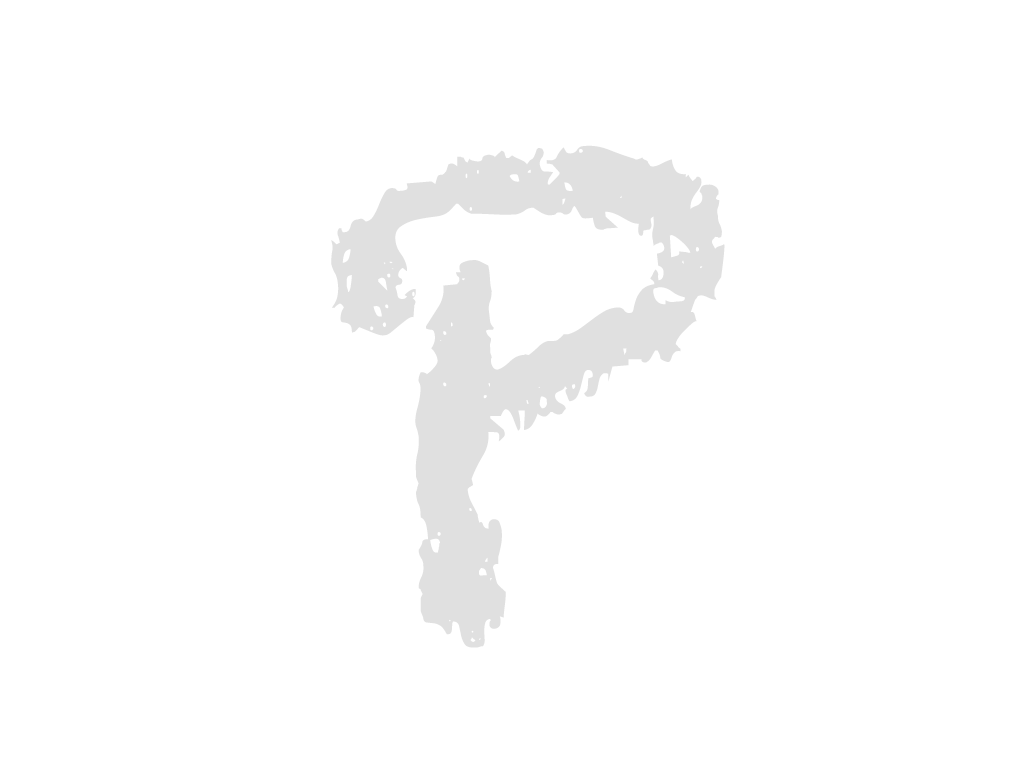
Merge branch 'admin' of http://210.180.118.83/yjryu/senior_care_system into admin
@e8e3b22cd620d358501333fa861deb7a73102984
--- client/views/pages/user_management/UserAuthoriySelect_agency.jsx
+++ client/views/pages/user_management/UserAuthoriySelect_agency.jsx
... | ... | @@ -6,23 +6,24 @@ |
6 | 6 |
import { useNavigate } from "react-router"; |
7 | 7 |
|
8 | 8 |
export default function UserAuthoriySelect_agency() { |
9 |
+ // 화면 진입 시 탭 별 노출될ㄹ 리스트 초기화 |
|
9 | 10 |
const [subjectList, setSubjectList] = React.useState([]); |
10 |
- //초기값 세팅 |
|
11 |
- const [regiNumber, setRegiNumber] = React.useState(""); |
|
11 |
+ const [subjectMyList, setSubjectMyList] = React.useState([]); |
|
12 |
+ |
|
13 |
+ // ------ 등록 버튼 클릭 시 노출되는 모달 데이터 ------// |
|
14 |
+ // 사용자 등록 시 초기값 세팅 |
|
12 | 15 |
const [userName, setUserName] = React.useState(""); |
13 | 16 |
const [gender, setGender] = React.useState(""); |
14 |
- const [brithday, setBrithday] = React.useState(""); |
|
17 |
+ const [brith, setBrith] = React.useState(""); |
|
15 | 18 |
const [telNum, setTelNum] = React.useState(""); |
16 | 19 |
const [homeAddress, setHomeAddress] = React.useState(""); |
17 | 20 |
const [medicineM, setMedicineM] = React.useState(false); |
18 | 21 |
const [medicineL, setMedicineL] = React.useState(false); |
19 | 22 |
const [medicineD, setMedicineD] = React.useState(false); |
23 |
+ const [medication, setMedication] = React.useState(""); |
|
20 | 24 |
const [note, setNote] = React.useState(""); |
21 | 25 |
|
22 | 26 |
// 변경되는 데이터 Handler |
23 |
- const handleRegiNumber = (e) => { |
|
24 |
- setRegiNumber(e.target.value); |
|
25 |
- }; |
|
26 | 27 |
const handleUserName = (e) => { |
27 | 28 |
setUserName(e.target.value); |
28 | 29 |
}; |
... | ... | @@ -30,9 +31,10 @@ |
30 | 31 |
setGender(e.target.value); |
31 | 32 |
}; |
32 | 33 |
const handleBrithday = (e) => { |
33 |
- setBrithday(e.target.value); |
|
34 |
+ setBrith(e.target.value); |
|
34 | 35 |
}; |
35 | 36 |
const handleTelNum = (e) => { |
37 |
+ e.target.value = e.target.value.replace(/[^0-9]/g, '').replace(/^(\d{2,3})(\d{3,4})(\d{4})$/, `$1-$2-$3`); |
|
36 | 38 |
setTelNum(e.target.value); |
37 | 39 |
}; |
38 | 40 |
const handleHomeAddress = (e) => { |
... | ... | @@ -47,49 +49,92 @@ |
47 | 49 |
const handleMedicineD = (e) => { |
48 | 50 |
setMedicineD(e.target.checked); |
49 | 51 |
}; |
52 |
+ const handleMedication = (e) => { |
|
53 |
+ setMedication(e.target.value); |
|
54 |
+ }; |
|
50 | 55 |
const handleNote = (e) => { |
51 | 56 |
setNote(e.target.value); |
52 | 57 |
}; |
53 | 58 |
|
59 |
+ // 대상자 등록 함수 |
|
54 | 60 |
const seniorInsert = () => { |
55 |
- console.log("userName : ", userName); |
|
56 |
- console.log("gender : ", gender); |
|
57 |
- console.log("brithday : ", brithday); |
|
58 |
- console.log("telNum : ", telNum); |
|
59 |
- console.log("homeAddress : ", homeAddress); |
|
60 |
- console.log("note : ", note); |
|
61 |
- console.log("medicineM : ", medicineM); |
|
62 |
- console.log("medicineL : ", medicineL); |
|
63 |
- console.log("medicineD : ", medicineD); |
|
64 |
- // fetch("", { |
|
65 |
- // method: "POST", |
|
66 |
- // headers: { |
|
67 |
- // 'Content-Type': 'application/json; charset=UTF-8' |
|
68 |
- // }, |
|
69 |
- // body: JSON.stringify({ |
|
70 |
- // category: selectCategory, |
|
71 |
- // title: inputTitle, |
|
72 |
- // writer: writer, |
|
73 |
- // }), |
|
74 |
- // }).then((response) => response.json()).then((data) => { |
|
75 |
- // alert("등록 되었습니다."); |
|
76 |
- // }).catch((error) => { |
|
77 |
- // console.log('selectNotice() /Notice/selectNotice.json error : ', error); |
|
78 |
- // }); |
|
61 |
+ // userName,gender,brith, telNum, homeAddress, note, medicineM, medicineL, medicineD |
|
62 |
+ // console.log("userName : ",userName); |
|
63 |
+ // console.log("gender : ",gender); |
|
64 |
+ // console.log("brith : ",brith); |
|
65 |
+ // console.log("telNum : ",telNum); |
|
66 |
+ // console.log("homeAddress : ",homeAddress); |
|
67 |
+ // console.log("note : ",note); |
|
68 |
+ // console.log("medicineM : ",medicineM); |
|
69 |
+ // console.log("medicineL : ",medicineL); |
|
70 |
+ // console.log("medicineD : ",medicineD); |
|
71 |
+ var insertBtn = confirm("등록하시겠습니까?"); |
|
72 |
+ if(insertBtn){ |
|
73 |
+ fetch("/user/userInsert_userMadicationInsert.json", { |
|
74 |
+ method: "POST", |
|
75 |
+ headers: { |
|
76 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
77 |
+ }, |
|
78 |
+ body: JSON.stringify({ |
|
79 |
+ userName:userName, |
|
80 |
+ gender:gender, |
|
81 |
+ brith :brith, |
|
82 |
+ userTel :telNum, |
|
83 |
+ homeAddress:homeAddress, |
|
84 |
+ medicineM:medicineM, |
|
85 |
+ medicineL:medicineL, |
|
86 |
+ medicineD:medicineD, |
|
87 |
+ medication:medication, |
|
88 |
+ note:note, |
|
89 |
+ agancyId:'agency1', |
|
90 |
+ govId:'government1', |
|
91 |
+ userCode : '4' |
|
92 |
+ }), |
|
93 |
+ }).then((response) => response.json()).then((data) => { |
|
94 |
+ alert("등록 되었습니다."); |
|
95 |
+ }).catch((error) => { |
|
96 |
+ console.log('selectNotice() /Notice/selectNotice.json error : ', error); |
|
97 |
+ }); |
|
98 |
+ }else{ |
|
99 |
+ return; |
|
100 |
+ } |
|
101 |
+ |
|
79 | 102 |
}; |
80 |
- const getSubjectSelect = () => { |
|
81 |
- fetch("/user/subjectList.json", { |
|
103 |
+ |
|
104 |
+ const getSelectSubjectList = () => { |
|
105 |
+ fetch("/user/selectSubjectList.json", { |
|
82 | 106 |
method: "POST", |
83 | 107 |
headers: { |
84 | 108 |
'Content-Type': 'application/json; charset=UTF-8' |
85 | 109 |
}, |
86 | 110 |
}).then((response) => response.json()).then((data) => { |
111 |
+ const rowData = data; |
|
87 | 112 |
console.log(data); |
88 |
- // setSubjectList(data.rows); |
|
113 |
+ setSubjectList(rowData) |
|
114 |
+ |
|
89 | 115 |
}).catch((error) => { |
90 |
- console.log('getSubjectSelect() /user/subjectList.json error : ', error); |
|
116 |
+ console.log('getSelectSubjectList() /user/selectSubjectList.json error : ', error); |
|
91 | 117 |
}); |
92 | 118 |
}; |
119 |
+ |
|
120 |
+ const getSelectMySubjectList = () => { |
|
121 |
+ fetch("/user/selectMySubjectList.json", { |
|
122 |
+ method: "POST", |
|
123 |
+ headers: { |
|
124 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
125 |
+ }, |
|
126 |
+ body: JSON.stringify({ |
|
127 |
+ agancyId: 'agency1', |
|
128 |
+ }), |
|
129 |
+ }).then((response) => response.json()).then((data) => { |
|
130 |
+ const rowData = data; |
|
131 |
+ console.log(data); |
|
132 |
+ setSubjectMyList(rowData) |
|
133 |
+ }).catch((error) => { |
|
134 |
+ console.log('getSubjectMySelect() /user/selectMySubjectList.json error : ', error); |
|
135 |
+ }); |
|
136 |
+ }; |
|
137 |
+ |
|
93 | 138 |
const navigate = useNavigate(); |
94 | 139 |
const [modalOpen, setModalOpen] = React.useState(false); |
95 | 140 |
const openModal = () => { |
... | ... | @@ -131,37 +176,26 @@ |
131 | 176 |
const thead1 = [ |
132 | 177 |
"No", |
133 | 178 |
"대상자 이름", |
134 |
- "대상자 등록번호", |
|
135 | 179 |
"대상자 연락처", |
136 | 180 |
"성별", |
137 | 181 |
"생년월일", |
138 | 182 |
"주소", |
139 | 183 |
"보호자", |
184 |
+ |
|
140 | 185 |
]; |
141 |
- const key1 = ["No", "name", "Id", "call", "gender", "birth", "address", "management"]; |
|
142 |
- const content1 = [ |
|
143 |
- { |
|
144 |
- No: 1, |
|
145 |
- name: "김복남", |
|
146 |
- Id: "20230131", |
|
147 |
- call: "010-1234-1234", |
|
148 |
- gender: "여", |
|
149 |
- birth: "1948.11.15", |
|
150 |
- address: "경상북도 군위군 삼국유사면", |
|
151 |
- management: ( |
|
152 |
- <Button |
|
153 |
- className={"btn-small gray-btn"} |
|
154 |
- btnName={"보기"} |
|
155 |
- onClick={openModal} |
|
156 |
- /> |
|
157 |
- ), |
|
158 |
- }, |
|
186 |
+ const key1 = [ |
|
187 |
+ // "No", "name", "Id", "call", "gender", "birth", "address", "management" |
|
188 |
+ "rn", |
|
189 |
+ "user_name", |
|
190 |
+ "user_phonenumber", |
|
191 |
+ "user_gender", |
|
192 |
+ "user_birth", |
|
193 |
+ "user_address", |
|
159 | 194 |
]; |
160 | 195 |
|
161 | 196 |
const thead2 = [ |
162 | 197 |
"No", |
163 | 198 |
"대상자 이름", |
164 |
- "대상자 등록번호", |
|
165 | 199 |
"대상자 연락처", |
166 | 200 |
"성별", |
167 | 201 |
"생년월일", |
... | ... | @@ -170,13 +204,17 @@ |
170 | 204 |
"보호자", |
171 | 205 |
]; |
172 | 206 |
const key2 = [ |
173 |
- "No", "name", "Id", "call", "gender", "birth", "address", "worker", "guardian" |
|
207 |
+ "rn", |
|
208 |
+ "user_name", |
|
209 |
+ "user_phonenumber", |
|
210 |
+ "user_gender", |
|
211 |
+ "user_birth", |
|
212 |
+ "user_address", |
|
174 | 213 |
]; |
175 | 214 |
const content2 = [ |
176 | 215 |
{ |
177 | 216 |
No: 2, |
178 | 217 |
name: "송창덕", |
179 |
- Id: "20230202", |
|
180 | 218 |
call: "010-5555-3573", |
181 | 219 |
gender: "남", |
182 | 220 |
birth: "1951.08.12", |
... | ... | @@ -199,7 +237,6 @@ |
199 | 237 |
{ |
200 | 238 |
No: 1, |
201 | 239 |
name: "김복남", |
202 |
- Id: "20230131", |
|
203 | 240 |
call: "010-1234-1234", |
204 | 241 |
gender: "여", |
205 | 242 |
birth: "1948.11.15", |
... | ... | @@ -302,10 +339,11 @@ |
302 | 339 |
id: 1, |
303 | 340 |
title: "내가 관리하는 대상자", |
304 | 341 |
description: ( |
342 |
+ |
|
305 | 343 |
<Table |
306 | 344 |
className={"protector-user"} |
307 | 345 |
head={thead1} |
308 |
- contents={subjectList} |
|
346 |
+ contents={subjectMyList} |
|
309 | 347 |
contentKey={key1} |
310 | 348 |
/> |
311 | 349 |
), |
... | ... | @@ -317,7 +355,7 @@ |
317 | 355 |
<Table |
318 | 356 |
className={"caregiver-user"} |
319 | 357 |
head={thead2} |
320 |
- contents={content2} |
|
358 |
+ contents={subjectList} |
|
321 | 359 |
contentKey={key2} |
322 | 360 |
/> |
323 | 361 |
), |
... | ... | @@ -327,7 +365,8 @@ |
327 | 365 |
const [index, setIndex] = React.useState(1); |
328 | 366 |
|
329 | 367 |
React.useEffect(() => { |
330 |
- getSubjectSelect(); |
|
368 |
+ getSelectSubjectList(); |
|
369 |
+ getSelectMySubjectList(); |
|
331 | 370 |
|
332 | 371 |
}, []) |
333 | 372 |
return ( |
... | ... | @@ -359,7 +398,7 @@ |
359 | 398 |
<tr> |
360 | 399 |
<th>연락처</th> |
361 | 400 |
<td colSpan={3}> |
362 |
- <input type="text" /> |
|
401 |
+ <input type="input" maxLength="11" /> |
|
363 | 402 |
</td> |
364 | 403 |
</tr> |
365 | 404 |
<tr> |
... | ... | @@ -419,7 +458,7 @@ |
419 | 458 |
<div className="board-wrap"> |
420 | 459 |
<SubTitle explanation={"회원 등록 시 ID는 연락처, 패스워드는 생년월일 8자리입니다."} className="margin-bottom"/> |
421 | 460 |
<table className="margin-bottom2 senior-insert"> |
422 |
- <tr> |
|
461 |
+ {/* <tr> |
|
423 | 462 |
<th>대상자등록번호</th> |
424 | 463 |
<td colSpan={3} className="flex"> |
425 | 464 |
<input type="text" placeholder="생성하기 버튼 클릭 시 자동으로 생성됩니다."/> |
... | ... | @@ -428,7 +467,7 @@ |
428 | 467 |
btnName={"생성하기"} |
429 | 468 |
/> |
430 | 469 |
</td> |
431 |
- </tr> |
|
470 |
+ </tr> */} |
|
432 | 471 |
<tr> |
433 | 472 |
<th>이름</th> |
434 | 473 |
<td> |
... | ... | @@ -450,7 +489,7 @@ |
450 | 489 |
<th>생년월일</th> |
451 | 490 |
<td> |
452 | 491 |
<div className="flex"> |
453 |
- <input type='date' value={brithday} onChange={handleBrithday} /> |
|
492 |
+ <input type='date' value={brith} onChange={handleBrithday} /> |
|
454 | 493 |
</div> |
455 | 494 |
</td> |
456 | 495 |
{/* <th>요양등급</th> |
... | ... | @@ -462,7 +501,7 @@ |
462 | 501 |
<tr> |
463 | 502 |
<th>연락처</th> |
464 | 503 |
<td colSpan={3}> |
465 |
- <input type="text" value={telNum} onChange={handleTelNum}/> |
|
504 |
+ <input type="text" value={telNum} onChange={handleTelNum} /> |
|
466 | 505 |
</td> |
467 | 506 |
</tr> |
468 | 507 |
<tr> |
... | ... | @@ -482,18 +521,19 @@ |
482 | 521 |
</td> |
483 | 522 |
</tr> |
484 | 523 |
<tr> |
485 |
- <th>비고</th> |
|
486 |
- <td colSpan={3}> |
|
487 |
- <textarea className="medicine" cols="30" rows="2" value={note} onChange={handleNote}></textarea> |
|
488 |
- </td> |
|
489 |
- </tr> |
|
490 |
- {/* <tr> |
|
491 | 524 |
<th>복용중인 약</th> |
492 | 525 |
<td colSpan={3}> |
493 |
- <textarea className="medicine" cols="30" rows="2"></textarea> |
|
526 |
+ <textarea className="medicine" cols="30" rows="2" value={medication} onChange={handleMedication}></textarea> |
|
494 | 527 |
</td> |
495 | 528 |
</tr> |
496 | 529 |
<tr> |
530 |
+ <th>비고</th> |
|
531 |
+ <td colSpan={3}> |
|
532 |
+ <textarea className="note" cols="30" rows="2" value={note} onChange={handleNote}></textarea> |
|
533 |
+ </td> |
|
534 |
+ </tr> |
|
535 |
+ |
|
536 |
+ {/* <tr> |
|
497 | 537 |
<th>기저질환</th> |
498 | 538 |
<td colSpan={3}> |
499 | 539 |
<textarea cols="30" rows="10"></textarea> |
... | ... | @@ -505,7 +545,7 @@ |
505 | 545 |
className={"btn-small green-btn"} |
506 | 546 |
btnName={"등록"} |
507 | 547 |
onClick={() => { |
508 |
- seniorInsert(userName,gender,brithday, telNum, homeAddress, note, medicineM, medicineL, medicineD) |
|
548 |
+ seniorInsert(userName,gender,brith, telNum, homeAddress, note, medicineM, medicineL, medicineD) |
|
509 | 549 |
}} |
510 | 550 |
/> |
511 | 551 |
</div> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?