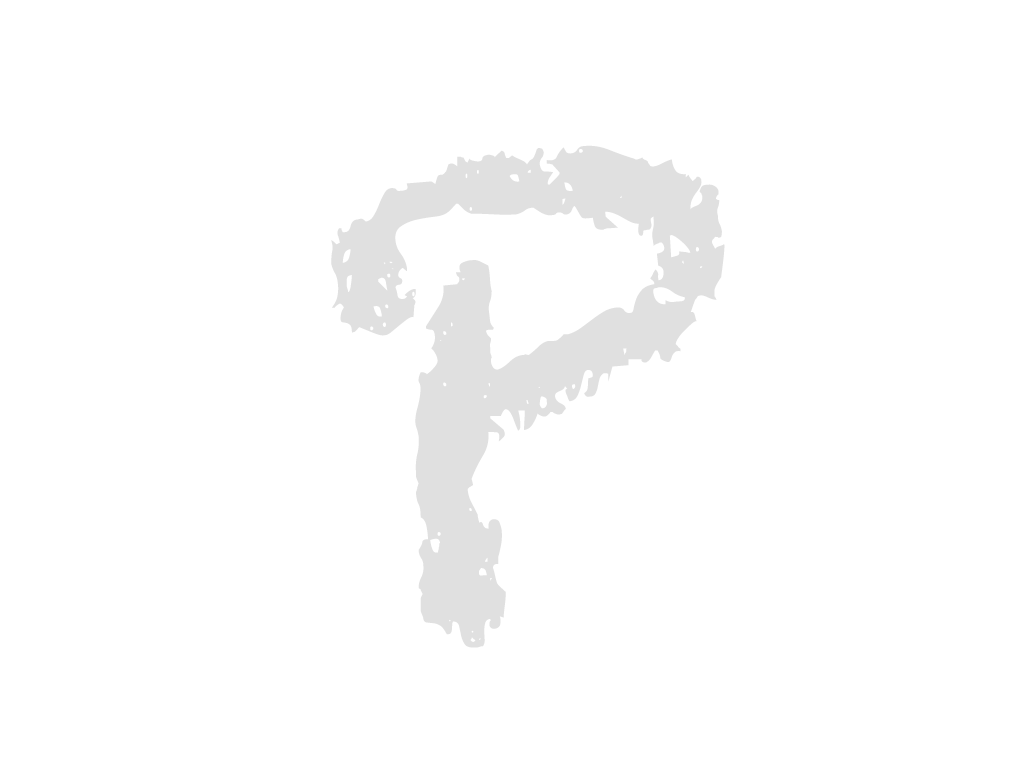
--- client/views/component/Calendar.jsx
+++ client/views/component/Calendar.jsx
... | ... | @@ -3,13 +3,23 @@ |
3 | 3 |
import "react-calendar/dist/Calendar.css"; |
4 | 4 |
import moment from "moment"; |
5 | 5 |
|
6 |
-export default function CalendarComponent(props) { |
|
6 |
+export default function CalendarComponent({ data }) { |
|
7 |
+ console.log('createCalendar data : ', data); |
|
8 |
+ |
|
7 | 9 |
const [value, setValue] = useState(new Date()); |
8 | 10 |
//const mark = ["12-04-2023", "21-04-2023", "05-04-2023", "02-04-2023"]; |
9 |
- const mark = ["2023-04-12", "2023-04-21"]; |
|
11 |
+ //const mark = ["2023-04-12", "2023-06-12"]; |
|
12 |
+ |
|
13 |
+ let marks = []; |
|
14 |
+ for (let i = 0; i < data['visit'].length; i++) { |
|
15 |
+ marks.push(data['visit'][i]['xName']); |
|
16 |
+ } |
|
17 |
+ |
|
18 |
+ const mark = marks |
|
19 |
+ |
|
10 | 20 |
return ( |
11 |
- <> |
|
12 |
- <Calendar |
|
21 |
+ <> |
|
22 |
+ <Calendar |
|
13 | 23 |
onChange={setValue} |
14 | 24 |
value={value} |
15 | 25 |
tileClassName={({ date, view }) => { |
... | ... | @@ -17,7 +27,46 @@ |
17 | 27 |
return "highlight"; |
18 | 28 |
} |
19 | 29 |
}} |
30 |
+ tileContent={({ date, view }) => { |
|
31 |
+ return ( |
|
32 |
+ <> |
|
33 |
+ <div className="flex justify-center items-center absoluteDiv"> |
|
34 |
+ <div> |
|
35 |
+ <p><small>복약량</small></p> |
|
36 |
+ {data['medication'].map((item, idx) => { |
|
37 |
+ return ( |
|
38 |
+ item['xName'] === moment(date).format("YYYY-MM-DD") ? item['sum'] + '/' + item['total'] : null |
|
39 |
+ ) |
|
40 |
+ })} |
|
41 |
+ </div> |
|
42 |
+ <div> |
|
43 |
+ <p><small>온도(℃)</small></p> |
|
44 |
+ {data['temperature'].map((item, idx) => { |
|
45 |
+ return ( |
|
46 |
+ item['xName'] === moment(date).format("YYYY-MM-DD") && item['time'] == '02:00' ? item['temperature'] : null |
|
47 |
+ ) |
|
48 |
+ })}/ |
|
49 |
+ {data['temperature'].map((item, idx) => { |
|
50 |
+ return ( |
|
51 |
+ item['xName'] === moment(date).format("YYYY-MM-DD") && item['time'] == '10:00' ? item['temperature'] : null |
|
52 |
+ ) |
|
53 |
+ })}/ |
|
54 |
+ {data['temperature'].map((item, idx) => { |
|
55 |
+ return ( |
|
56 |
+ item['xName'] === moment(date).format("YYYY-MM-DD") && item['time'] == '14:00' ? item['temperature'] : null |
|
57 |
+ ) |
|
58 |
+ })}/ |
|
59 |
+ {data['temperature'].map((item, idx) => { |
|
60 |
+ return ( |
|
61 |
+ item['xName'] === moment(date).format("YYYY-MM-DD") && item['time'] == '23:00' ? item['temperature'] : null |
|
62 |
+ ) |
|
63 |
+ })} |
|
64 |
+ </div> |
|
65 |
+ </div> |
|
66 |
+ </> |
|
67 |
+ ); |
|
68 |
+ }} |
|
20 | 69 |
/> |
21 |
- </> |
|
70 |
+ </> |
|
22 | 71 |
); |
23 | 72 |
} |
--- client/views/component/Table.jsx
+++ client/views/component/Table.jsx
... | ... | @@ -133,13 +133,13 @@ |
133 | 133 |
navigate(`/QuestionConfirm/${i.qna_idx}`); |
134 | 134 |
} |
135 | 135 |
else if (view == "mySeniorMedicine") { |
136 |
- navigate("/MedicineCareSelectOne"); |
|
136 |
+ navigate("/HealthcareSelectOne"); |
|
137 | 137 |
} |
138 | 138 |
else if (view == "mySeniorTemperature") { |
139 |
- navigate("/TemperatureManagementSelectOne"); |
|
139 |
+ navigate("/HealthcareSelectOne"); |
|
140 | 140 |
} |
141 | 141 |
else if (view == "mySeniorVisit") { |
142 |
- navigate("/VisitSelectOne"); |
|
142 |
+ navigate("/HealthcareSelectOne"); |
|
143 | 143 |
} |
144 | 144 |
else { |
145 | 145 |
return; |
--- client/views/pages/AppRoute.jsx
+++ client/views/pages/AppRoute.jsx
... | ... | @@ -40,6 +40,7 @@ |
40 | 40 |
import EquipmentManagementSelectOne from "./equipment/EquipmentManagementSelectOne.jsx"; |
41 | 41 |
import UserSelect from "./user_management/UserSelect.jsx"; |
42 | 42 |
import AgencySeniorSelect from "./user_management/AgencySeniorSelect.jsx"; |
43 |
+import AgencyAdminSeniorSelect from "./user_management/AgencyAdminSeniorSelect.jsx"; |
|
43 | 44 |
import QandASelect from "./callcenter/QandASelect.jsx"; |
44 | 45 |
import QandASelectOne from "./callcenter/QandASelectOne.jsx"; |
45 | 46 |
import QandAInsert from "./callcenter/QandAInsert.jsx"; |
... | ... | @@ -58,9 +59,10 @@ |
58 | 59 |
import QuestionSelect from "./callcenter/QuestionSelect.jsx"; |
59 | 60 |
import Join from "./join/Join.jsx"; |
60 | 61 |
import Healthcare from "./healthcare/Healthcare.jsx" |
61 |
-import TeamHealthcare from "./healthcare/TeamHealthcare.jsx" |
|
62 |
+import HealthcareAdmin from "./healthcare/HealthcareAdmin.jsx" |
|
62 | 63 |
import Medicalcare from "./healthcare/Medicalcare.jsx" |
63 |
-import TeamMedicalcare from "./healthcare/TeamMedicalcare.jsx" |
|
64 |
+import MedicalcareAdmin from "./healthcare/MedicalcareAdmin.jsx" |
|
65 |
+import HealthcareSelectOne from "./healthcare/HealthcareSelectOne.jsx" |
|
64 | 66 |
|
65 | 67 |
import UserEdit from "./user_management/UserEdit.jsx"; |
66 | 68 |
import AgentSelectOne from "./user_management/AgentSelectOne.jsx"; |
... | ... | @@ -289,7 +291,7 @@ |
289 | 291 |
}, |
290 | 292 |
{ |
291 | 293 |
title: "대상자 관리", |
292 |
- path: "/AgencySeniorSelect", |
|
294 |
+ path: "/AgencyAdminSeniorSelect", |
|
293 | 295 |
icon: ( |
294 | 296 |
<PersonIcon sx={{ fontSize: 20, color: "#333333", marginRight: 1 }} /> |
295 | 297 |
), |
... | ... | @@ -303,14 +305,14 @@ |
303 | 305 |
}, |
304 | 306 |
{ |
305 | 307 |
title: "생활 복지 관리", |
306 |
- path: "/TeamHealthcare", |
|
308 |
+ path: "/HealthcareAdmin", |
|
307 | 309 |
icon: ( |
308 | 310 |
<Diversity1Icon sx={{ fontSize: 20, color: "#333333", marginRight: 1 }} /> |
309 | 311 |
), |
310 | 312 |
}, |
311 | 313 |
{ |
312 | 314 |
title: "건강 관리", |
313 |
- path: "/TeamMedicalcare", |
|
315 |
+ path: "/MedicalcareAdmin", |
|
314 | 316 |
icon: ( |
315 | 317 |
<LocalHospitalIcon sx={{ fontSize: 20, color: "#333333", marginRight: 1 }} /> |
316 | 318 |
), |
... | ... | @@ -329,17 +331,21 @@ |
329 | 331 |
return ( |
330 | 332 |
<Routes> |
331 | 333 |
|
332 |
- <Route path="/TeamMedicalcare" element={<TeamMedicalcare />}></Route> |
|
333 |
- <Route path="/TeamHealthcare" element={<TeamHealthcare />}></Route> |
|
334 |
+ <Route path="/MedicalcareAdmin" element={<MedicalcareAdmin />}></Route> |
|
335 |
+ <Route path="/HealthcareAdmin" element={<HealthcareAdmin />}></Route> |
|
334 | 336 |
<Route path="/Join" element={<Join />}></Route> |
335 | 337 |
<Route path="/QuestionSelect" element={<QuestionSelect />}></Route> |
336 | 338 |
|
337 | 339 |
<Route path="/" element={<Main_agencyAdmin />}></Route> |
338 |
- <Route path="/AgencySeniorSelect" element={<AgencySeniorSelect />}></Route> |
|
340 |
+ <Route path="/AgencyAdminSeniorSelect" element={<AgencyAdminSeniorSelect />}></Route> |
|
339 | 341 |
<Route path="/SeniorEdit" element={<SeniorEdit />}></Route> |
340 | 342 |
<Route path="/SeniorSelectOne" element={<SeniorSelectOne />}></Route> |
341 | 343 |
<Route path="/UserEdit" element={<UserEdit />}></Route> |
342 | 344 |
<Route path="/AgentSelectOne" element={<AgentSelectOne />}></Route> |
345 |
+ <Route |
|
346 |
+ path="/HealthcareSelectOne" |
|
347 |
+ element={<HealthcareSelectOne />} |
|
348 |
+ ></Route> |
|
343 | 349 |
<Route |
344 | 350 |
path="/MedicineCareSelectOne" |
345 | 351 |
element={<MedicineCareSelectOne />} |
... | ... | @@ -428,6 +434,10 @@ |
428 | 434 |
<Route path="/UserEdit" element={<UserEdit />}></Route> |
429 | 435 |
<Route path="/AgentSelectOne" element={<AgentSelectOne />}></Route> |
430 | 436 |
<Route |
437 |
+ path="/HealthcareSelectOne" |
|
438 |
+ element={<HealthcareSelectOne />} |
|
439 |
+ ></Route> |
|
440 |
+ <Route |
|
431 | 441 |
path="/MedicineCareSelectOne" |
432 | 442 |
element={<MedicineCareSelectOne />} |
433 | 443 |
></Route> |
--- client/views/pages/equipment/GovernmentEquipmentSelect.jsx
+++ client/views/pages/equipment/GovernmentEquipmentSelect.jsx
... | ... | @@ -748,7 +748,7 @@ |
748 | 748 |
<div className="board-wrap" style={{ marginTop: "3rem" }} > |
749 | 749 |
<div className="btn-wrap margin-bottom"> |
750 | 750 |
<div className="btn-wrap flex-end margin-bottom "> |
751 |
- <button className={"btn-small gray-btn"} onClick={() => navigate("/AgencySeniorSelect")}>대상자 관리</button> |
|
751 |
+ <button className={"btn-small gray-btn"} onClick={() => (state.loginUser['authority'] == 'ROLE_AGENCYADMIN') ? navigate("/AgencyAdminSeniorSelect") : navigate("/AgencySeniorSelect")}>대상자 관리</button> |
|
752 | 752 |
</div> |
753 | 753 |
{rentalContent} |
754 | 754 |
</div> |
--- client/views/pages/healthcare/Healthcare.jsx
+++ client/views/pages/healthcare/Healthcare.jsx
... | ... | @@ -216,7 +216,7 @@ |
216 | 216 |
{senior.seniorList.map((item, idx) => { |
217 | 217 |
return ( |
218 | 218 |
<tr key={idx} onClick={() => { |
219 |
- navigate("/MedicineCareSelectOne", { |
|
219 |
+ navigate("/HealthcareSelectOne", { |
|
220 | 220 |
state: { |
221 | 221 |
'senior_id': item['user_id'], |
222 | 222 |
'agency_id': item['agency_id'], |
... | ... | @@ -275,19 +275,19 @@ |
275 | 275 |
<th rowSpan={2}>생년월일</th> |
276 | 276 |
<th rowSpan={2}>성별</th> |
277 | 277 |
<th rowSpan={2}>연락처</th> |
278 |
- <th colSpan={12}>최근온도</th> |
|
278 |
+ <th colSpan={12}>최근온도 (℃)</th> |
|
279 | 279 |
</tr> |
280 | 280 |
<tr> |
281 |
- <th colSpan={4}>어제</th> |
|
282 |
- <th colSpan={4}>이틀 전</th> |
|
283 |
- <th colSpan={4}>사흘 전</th> |
|
281 |
+ <th colSpan={4}>최근 하루 전</th> |
|
282 |
+ <th colSpan={4}>최근 이틀 전</th> |
|
283 |
+ <th colSpan={4}>최근 사흘 전</th> |
|
284 | 284 |
</tr> |
285 | 285 |
</thead> |
286 | 286 |
<tbody> |
287 | 287 |
{senior.seniorList.map((item, idx) => { |
288 | 288 |
return ( |
289 | 289 |
<tr key={idx} onClick={() => { |
290 |
- navigate("/TemperatureManagementSelectOne", { |
|
290 |
+ navigate("/HealthcareSelectOne", { |
|
291 | 291 |
state: { |
292 | 292 |
'senior_id': item['user_id'], |
293 | 293 |
'agency_id': item['agency_id'], |
... | ... | @@ -306,7 +306,8 @@ |
306 | 306 |
item['seniorTemperatureList'].map((data, dataIdx) => { |
307 | 307 |
return ( |
308 | 308 |
<td data-label="최근온도"> |
309 |
- {data['temperature']} <small>({data['time']})</small> |
|
309 |
+ {data['temperature']} |
|
310 |
+ {/* <small>({data['time']})</small> */} |
|
310 | 311 |
</td> |
311 | 312 |
) |
312 | 313 |
}) |
... | ... | @@ -355,7 +356,7 @@ |
355 | 356 |
{senior.seniorList.map((item, idx) => { |
356 | 357 |
return ( |
357 | 358 |
<tr key={idx} onClick={() => { |
358 |
- navigate("/VisitSelectOne", { |
|
359 |
+ navigate("/HealthcareSelectOne", { |
|
359 | 360 |
state: { |
360 | 361 |
'senior_id': item['senior_id'], |
361 | 362 |
} |
--- client/views/pages/healthcare/TeamHealthcare.jsx
+++ client/views/pages/healthcare/HealthcareAdmin.jsx
... | ... | @@ -38,8 +38,7 @@ |
38 | 38 |
seniorSelectList(1); |
39 | 39 |
} |
40 | 40 |
|
41 |
- const [seniorNum, setSeniorNum] = React.useState(); |
|
42 |
- const [isMySenior, setIsMySenior] = React.useState(true); |
|
41 |
+ const [isMySenior, setIsMySenior] = React.useState(false); |
|
43 | 42 |
React.useEffect(() => { |
44 | 43 |
searching(); |
45 | 44 |
}, [isMySenior]) |
... | ... | @@ -69,6 +68,7 @@ |
69 | 68 |
data.seniorList.map((item, idx) => { |
70 | 69 |
setSeniorNum(item); |
71 | 70 |
seniorMedicationSelectList(item); |
71 |
+ seniorTemperatureSelectListByDay(item); |
|
72 | 72 |
}) |
73 | 73 |
console.log("돌봄 대상자(시니어) 목록 조회 : ", data); |
74 | 74 |
setSenior(data); |
... | ... | @@ -89,7 +89,6 @@ |
89 | 89 |
}, |
90 | 90 |
body: JSON.stringify(seniorNum), |
91 | 91 |
}).then((response) => response.json()).then((data) => { |
92 |
- console.log("seniorMedicationList data : ", data); |
|
93 | 92 |
setSeniorMedicationList(data, seniorNum); |
94 | 93 |
seniorMedicationSelectListByDay(data, seniorNum); |
95 | 94 |
}).catch((error) => { |
... | ... | @@ -113,7 +112,6 @@ |
113 | 112 |
showMedicationTimeCode[seniorMedicationList[i]] = true; |
114 | 113 |
} |
115 | 114 |
setShowMedicationTimeCode(showMedicationTimeCode); |
116 |
- console.log('showMedicationTimeCode : ', showMedicationTimeCode); |
|
117 | 115 |
|
118 | 116 |
if (CommonUtil.isEmpty(data) == false) { |
119 | 117 |
let _stackChartData = []; |
... | ... | @@ -134,13 +132,55 @@ |
134 | 132 |
continue; |
135 | 133 |
} |
136 | 134 |
} |
137 |
- // _stackChartData.push(chartData); |
|
138 | 135 |
_stackChartData.push({ "sum": sum, "total": counter }) |
139 | 136 |
} |
140 | 137 |
seniorNum.seniorMedicationList = _stackChartData; |
141 | 138 |
} |
142 | 139 |
}).catch((error) => { |
143 | 140 |
console.log('seniorMedicationSelectListByDay() /user/seniorMedicationSelectListByDay.json error : ', error); |
141 |
+ }); |
|
142 |
+ }; |
|
143 |
+ |
|
144 |
+ |
|
145 |
+ const seniorTemperatureList = ['02:00', '10:00', '14:00', '23:00'] |
|
146 |
+ |
|
147 |
+ //특정 대상자의 일별, 시간별 온도 목록 |
|
148 |
+ const [seniorTemperatureListByDay, setSeniorTemperatureListByDay] = React.useState([]); |
|
149 |
+ const [stackTemperatureData, setStackTemperatureData] = React.useState([]); |
|
150 |
+ //특정 대상자의 일별, 시간별 온도 목록 조회 |
|
151 |
+ const seniorTemperatureSelectListByDay = (seniorNum) => { |
|
152 |
+ fetch("/user/seniorTemperatureSelectListByDay.json", { |
|
153 |
+ method: "POST", |
|
154 |
+ headers: { |
|
155 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
156 |
+ }, |
|
157 |
+ body: JSON.stringify(seniorNum), |
|
158 |
+ }).then((response) => response.json()).then((data) => { |
|
159 |
+ console.log("seniorTemperatureListByDay data : ", data); |
|
160 |
+ setSeniorTemperatureListByDay(data); |
|
161 |
+ |
|
162 |
+ if (CommonUtil.isEmpty(data) == false) { |
|
163 |
+ let _stackTemperatureData = []; |
|
164 |
+ let chartData = {}; |
|
165 |
+ for (let i = data.length - 1; i >= (data.length > 12 ? data.length - 12 : 0); i--) { |
|
166 |
+ chartData = { |
|
167 |
+ xName: data[i]['temperature_date'], |
|
168 |
+ }; |
|
169 |
+ chartData['temperature'] = data[i]['temperature_data']; |
|
170 |
+ chartData['time'] = data[i]['temperature_time']; |
|
171 |
+ |
|
172 |
+ if (data[i]['temperature_date'].substr(5, 2) >= 11 || data[i]['temperature_date'].substr(5, 2) <= 2) { |
|
173 |
+ _stackTemperatureData.unshift(chartData); |
|
174 |
+ } else { |
|
175 |
+ _stackTemperatureData.push(chartData); |
|
176 |
+ } |
|
177 |
+ } |
|
178 |
+ |
|
179 |
+ seniorNum.seniorTemperatureList = _stackTemperatureData; |
|
180 |
+ console.log('_stackTemperatureData : ', _stackTemperatureData); |
|
181 |
+ } |
|
182 |
+ }).catch((error) => { |
|
183 |
+ console.log('seniorTemperatureSelectListByDay() /user/seniorTemperatureSelectListByDay.json error : ', error); |
|
144 | 184 |
}); |
145 | 185 |
}; |
146 | 186 |
|
... | ... | @@ -228,21 +268,31 @@ |
228 | 268 |
<table className={"protector-user"}> |
229 | 269 |
<thead> |
230 | 270 |
<tr> |
231 |
- <th>No</th> |
|
232 |
- <th>소속기관명</th> |
|
233 |
- <th>이름</th> |
|
234 |
- <th>생년월일</th> |
|
235 |
- <th>성별</th> |
|
236 |
- <th>연락처</th> |
|
237 |
- <th>최근최저온도</th> |
|
238 |
- <th>최근최고온도</th> |
|
271 |
+ <th rowSpan={2}>No</th> |
|
272 |
+ <th rowSpan={2}>소속기관명</th> |
|
273 |
+ <th rowSpan={2}>이름</th> |
|
274 |
+ <th rowSpan={2}>생년월일</th> |
|
275 |
+ <th rowSpan={2}>성별</th> |
|
276 |
+ <th rowSpan={2}>연락처</th> |
|
277 |
+ <th colSpan={12}>최근온도 (℃)</th> |
|
278 |
+ </tr> |
|
279 |
+ <tr> |
|
280 |
+ <th colSpan={4}>최근 하루 전</th> |
|
281 |
+ <th colSpan={4}>최근 이틀 전</th> |
|
282 |
+ <th colSpan={4}>최근 사흘 전</th> |
|
239 | 283 |
</tr> |
240 | 284 |
</thead> |
241 | 285 |
<tbody> |
242 | 286 |
{senior.seniorList.map((item, idx) => { |
243 | 287 |
return ( |
244 | 288 |
<tr key={idx} onClick={() => { |
245 |
- navigate("/TemperatureManagementSelectOne"); |
|
289 |
+ navigate("/HealthcareSelectOne", { |
|
290 |
+ state: { |
|
291 |
+ 'senior_id': item['user_id'], |
|
292 |
+ 'agency_id': item['agency_id'], |
|
293 |
+ 'government_id': item['government_id'] |
|
294 |
+ } |
|
295 |
+ }) |
|
246 | 296 |
}}> |
247 | 297 |
<td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td> |
248 | 298 |
<td data-label="소속기관명">{item['agency_name']}</td> |
... | ... | @@ -250,18 +300,23 @@ |
250 | 300 |
<td data-label="생년월일">{item['user_birth']}</td> |
251 | 301 |
<td data-label="성별">{item['user_gender']}</td> |
252 | 302 |
<td data-label="연락처">{item['user_phonenumber']}</td> |
253 |
- <td data-label="최근최저온도"> |
|
254 |
- 18℃ |
|
255 |
- </td> |
|
256 |
- <td data-label="최근최고온도"> |
|
257 |
- 26℃ |
|
258 |
- </td> |
|
303 |
+ { |
|
304 |
+ item['seniorTemperatureList'] && item['seniorTemperatureList'].length > 0 ? |
|
305 |
+ item['seniorTemperatureList'].map((data, dataIdx) => { |
|
306 |
+ return ( |
|
307 |
+ <td data-label="최근온도"> |
|
308 |
+ {data['temperature']} |
|
309 |
+ {/* <small>({data['time']})</small> */} |
|
310 |
+ </td> |
|
311 |
+ ) |
|
312 |
+ }) |
|
313 |
+ : null} |
|
259 | 314 |
</tr> |
260 | 315 |
) |
261 | 316 |
})} |
262 | 317 |
{CommonUtil.isEmpty(senior.seniorList) ? |
263 | 318 |
<tr> |
264 |
- <td colSpan={8}>조회된 데이터가 없습니다</td> |
|
319 |
+ <td colSpan={18}>조회된 데이터가 없습니다</td> |
|
265 | 320 |
</tr> |
266 | 321 |
: null} |
267 | 322 |
</tbody> |
... | ... | @@ -300,7 +355,7 @@ |
300 | 355 |
{senior.seniorList.map((item, idx) => { |
301 | 356 |
return ( |
302 | 357 |
<tr key={idx} onClick={() => { |
303 |
- navigate("/VisitSelectOne", { |
|
358 |
+ navigate("/HealthcareSelectOne", { |
|
304 | 359 |
state: { |
305 | 360 |
'senior_id': item['senior_id'], |
306 | 361 |
} |
+++ client/views/pages/healthcare/HealthcareSelectOne.jsx
... | ... | @@ -0,0 +1,213 @@ |
1 | +import React from "react"; | |
2 | +import { useNavigate, useLocation } from "react-router"; | |
3 | +import { useSelector } from "react-redux"; | |
4 | + | |
5 | +import Title from "../../component/Title.jsx"; | |
6 | +import Button from "../../component/Button.jsx"; | |
7 | +import Modal from "../../component/Modal.jsx"; | |
8 | +import Calendar from "../../component/Calendar.jsx"; | |
9 | +import ContentTitle from "../../component/ContentTitle.jsx"; | |
10 | +import TableTitle from "../../component/Tabletitle.jsx"; | |
11 | +import SubTitle from "../../component/SubTitle.jsx"; | |
12 | +import Donut2 from "../../component/chart/Donut2.jsx"; | |
13 | +import LineColor_medicine from "../../component/chart/LineColor_medicine.jsx"; | |
14 | +import MedicationIcon from '@mui/icons-material/Medication'; | |
15 | +import Senior from '../../../resources/files/images/senior.png'; | |
16 | + | |
17 | +import CommonUtil from "../../../resources/js/CommonUtil.js"; | |
18 | + | |
19 | +export default function MedicineCareSelectOne() { | |
20 | + const navigate = useNavigate(); | |
21 | + const location = useLocation(); | |
22 | + | |
23 | + //전역 변수 저장 객체 | |
24 | + const state = useSelector((state) => { return state }); | |
25 | + | |
26 | + //시니어 정보 | |
27 | + const [senior, setSenior] = React.useState({ | |
28 | + 'user_id': state.loginUser['authority'] == 'ROLE_SENIOR' ? state.loginUser['user_id'] : null, | |
29 | + 'user_name': state.loginUser['authority'] == 'ROLE_SENIOR' ? state.loginUser['user_name'] : null, | |
30 | + 'senior_id': state.loginUser['authority'] == 'ROLE_SENIOR' ? state.loginUser['user_id'] : null, | |
31 | + }); | |
32 | + | |
33 | + //시니어 상세 조회 | |
34 | + const seniorSelectOne = () => { | |
35 | + fetch("/user/seniorSelectOne.json", { | |
36 | + method: "POST", | |
37 | + headers: { | |
38 | + 'Content-Type': 'application/json; charset=UTF-8' | |
39 | + }, | |
40 | + body: JSON.stringify(senior), | |
41 | + }).then((response) => response.json()).then((data) => { | |
42 | + console.log("seniorSelectOne data : ", data); | |
43 | + setSenior(data); | |
44 | + }).catch((error) => { | |
45 | + console.log('seniorSelectOne() /user/seniorSelectOne.json error : ', error); | |
46 | + }); | |
47 | + }; | |
48 | + | |
49 | + const handleChangeSelect = (e) => { | |
50 | + setMySenior(e.target.value); | |
51 | + setIsMySenior(true); | |
52 | + }; | |
53 | + | |
54 | + const SelectBox = () => { | |
55 | + if (state['seniorList'].length > 1) { | |
56 | + return ( | |
57 | + <select className="margin-bottom2" onChange={handleChangeSelect} value={mySenior}> | |
58 | + {state['seniorList'].map((item, idx) => { | |
59 | + return ( | |
60 | + <option key={item} value={idx}>{item['user_name']}</option> | |
61 | + ) | |
62 | + })} | |
63 | + </select> | |
64 | + ) | |
65 | + } | |
66 | + } | |
67 | + | |
68 | + | |
69 | + //특정 대상자의 실제 복약 정보 | |
70 | + const [seniorMedicationList, setSeniorMedicationList] = React.useState([]); | |
71 | + const [showMedicationTimeCode, setShowMedicationTimeCode] = React.useState({}); | |
72 | + //특정 대상자의 실제 복약 정보 목록 조회 | |
73 | + const seniorMedicationSelectList = (seniorNum) => { | |
74 | + fetch("/user/seniorMedicationSelectList.json", { | |
75 | + method: "POST", | |
76 | + headers: { | |
77 | + 'Content-Type': 'application/json; charset=UTF-8' | |
78 | + }, | |
79 | + body: JSON.stringify(seniorNum), | |
80 | + }).then((response) => response.json()).then((data) => { | |
81 | + console.log("seniorMedicationList data : ", data); | |
82 | + setSeniorMedicationList(data); | |
83 | + seniorMedicationSelectListByDay(data, seniorNum); | |
84 | + }).catch((error) => { | |
85 | + console.log('seniorMedicationSelectList() /user/seniorMedicationSelectList.json error : ', error); | |
86 | + }); | |
87 | + }; | |
88 | + | |
89 | + //특정 대상자의 일별, 복약시간별 복약 목록 | |
90 | + const [seniorMedicationListByDay, setSeniorMedicationListByDay] = React.useState([]); | |
91 | + const [stackChartData, setStackChartData] = React.useState([]); | |
92 | + //특정 대상자의 일별, 복약시간별 복약 목록 조회 | |
93 | + const seniorMedicationSelectListByDay = (seniorMedicationList, seniorNum) => { | |
94 | + fetch("/user/seniorMedicationSelectListByDay.json", { | |
95 | + method: "POST", | |
96 | + headers: { | |
97 | + 'Content-Type': 'application/json; charset=UTF-8' | |
98 | + }, | |
99 | + body: JSON.stringify(seniorNum), | |
100 | + }).then((response) => response.json()).then((data) => { | |
101 | + console.log("seniorMedicationListByDay data : ", data); | |
102 | + setSeniorMedicationListByDay(data); | |
103 | + | |
104 | + let showMedicationTimeCode = {}; | |
105 | + for (let i = 0; i < seniorMedicationList.length; i++) { | |
106 | + showMedicationTimeCode[seniorMedicationList[i]] = true; | |
107 | + } | |
108 | + setShowMedicationTimeCode(showMedicationTimeCode); | |
109 | + console.log('showMedicationTimeCode : ', showMedicationTimeCode); | |
110 | + | |
111 | + if (CommonUtil.isEmpty(data) == false) { | |
112 | + let _stackChartData = []; | |
113 | + for (let i = 0; i < data.length; i++) { | |
114 | + let sum = 0; // 실제 복약량 | |
115 | + let counter = 0; // 복약해야하는 양 | |
116 | + for (let j = 0; j < data[i]['medication_time_code_list'].length; j++) { | |
117 | + if (CommonUtil.isEmpty(showMedicationTimeCode[data[i]['medication_time_code_list'][j]]) == false) { | |
118 | + counter++; | |
119 | + if (i > 0) { | |
120 | + sum += data[i]['medication_time_code_count_list'][j]; | |
121 | + } | |
122 | + } else { | |
123 | + continue; | |
124 | + } | |
125 | + } | |
126 | + _stackChartData.push({ "xName": data[i]['medication_default_date'], "sum": sum, "total": counter }) | |
127 | + } | |
128 | + setStackChartData(_stackChartData); | |
129 | + console.log('_stackChartData : ', _stackChartData); | |
130 | + } | |
131 | + | |
132 | + }).catch((error) => { | |
133 | + console.log('seniorMedicationSelectListByDay() /user/seniorMedicationSelectListByDay.json error : ', error); | |
134 | + }); | |
135 | + }; | |
136 | + | |
137 | + const seniorTemperatureList = ['02:00', '10:00', '14:00', '23:00'] | |
138 | + | |
139 | + //특정 대상자의 일별, 시간별 온도 목록 | |
140 | + const [seniorTemperatureListByDay, setSeniorTemperatureListByDay] = React.useState([]); | |
141 | + const [stackTemperatureData, setStackTemperatureData] = React.useState([]); | |
142 | + //특정 대상자의 일별, 시간별 온도 목록 조회 | |
143 | + const seniorTemperatureSelectListByDay = (seniorNum) => { | |
144 | + fetch("/user/seniorTemperatureSelectListByDay.json", { | |
145 | + method: "POST", | |
146 | + headers: { | |
147 | + 'Content-Type': 'application/json; charset=UTF-8' | |
148 | + }, | |
149 | + body: JSON.stringify(seniorNum), | |
150 | + }).then((response) => response.json()).then((data) => { | |
151 | + console.log("seniorTemperatureListByDay data : ", data); | |
152 | + setSeniorTemperatureListByDay(data); | |
153 | + | |
154 | + if (CommonUtil.isEmpty(data) == false) { | |
155 | + let _stackTemperatureData = []; | |
156 | + let chartData = {}; | |
157 | + for (let i = 0; i < data.length; i++) { | |
158 | + chartData = { | |
159 | + xName: data[i]['temperature_date'], | |
160 | + }; | |
161 | + chartData['temperature'] = data[i]['temperature_data']; | |
162 | + chartData['time'] = data[i]['temperature_time']; | |
163 | + | |
164 | + _stackTemperatureData.push(chartData); | |
165 | + } | |
166 | + setStackTemperatureData(_stackTemperatureData); | |
167 | + console.log('_stackTemperatureData : ', _stackTemperatureData); | |
168 | + } | |
169 | + }).catch((error) => { | |
170 | + console.log('seniorTemperatureSelectListByDay() /user/seniorTemperatureSelectListByDay.json error : ', error); | |
171 | + }); | |
172 | + }; | |
173 | + | |
174 | + //방문 기록 정보 | |
175 | + const [visitRecordList, setVisitRecordList] = React.useState({}); | |
176 | + //방문 기록 목록 조회 | |
177 | + const visitRecordSelectList = (seniorNum) => { | |
178 | + fetch("/welfare/visitRecordSelectList.json", { | |
179 | + method: "POST", | |
180 | + headers: { | |
181 | + 'Content-Type': 'application/json; charset=UTF-8' | |
182 | + }, | |
183 | + body: JSON.stringify(seniorNum), | |
184 | + }).then((response) => response.json()).then((data) => { | |
185 | + console.log("방문 기록 목록 조회 결과(건수) : ", data); | |
186 | + let _stackVisitData = []; | |
187 | + let chartData = {}; | |
188 | + for (let i = 0; i < data['visitRecordList'].length; i++) { | |
189 | + chartData = { | |
190 | + xName: data['visitRecordList'][i]['visit_date'], | |
191 | + }; | |
192 | + chartData['reason'] = data['visitRecordList'][i]['visit_reason']; | |
193 | + _stackVisitData.push(chartData); | |
194 | + } | |
195 | + setVisitRecordList(_stackVisitData); | |
196 | + console.log('_stackVisitData : ', _stackVisitData); | |
197 | + }).catch((error) => { | |
198 | + console.log('visitRecordSelectList() /user/visitRecordSelectList.json error : ', error); | |
199 | + }); | |
200 | + } | |
201 | + | |
202 | + return ( | |
203 | + <> | |
204 | + <main className="pink"> | |
205 | + <SelectBox /> | |
206 | + <div className="flex-start main-guardian"><img src={Senior} alt="" /> | |
207 | + <Title title={`${senior['user_name']} 어르신`} explanation={"방문, 복약, 온도, 배터리 현황을 확인하세요."} /> | |
208 | + </div> | |
209 | + <Calendar data={{ medication: stackChartData, temperature: stackTemperatureData, visit: visitRecordList }} /> | |
210 | + </main> | |
211 | + </> | |
212 | + ); | |
213 | +}(No newline at end of file) |
+++ client/views/pages/healthcare/MedicalcareAdmin.jsx
... | ... | @@ -0,0 +1,1740 @@ |
1 | +import React from "react"; | |
2 | +import { useNavigate, useLocation } from "react-router"; | |
3 | +import { useSelector } from "react-redux"; | |
4 | + | |
5 | + | |
6 | +import SubTitle from "../../component/SubTitle.jsx"; | |
7 | +import Modal from "../../component/Modal.jsx"; | |
8 | +import DetailTitle from "../../component/DetailTitle.jsx"; | |
9 | +import Pagination from "../../component/Pagination.jsx"; | |
10 | +import CommonFile from "../../component/file/CommonFile.jsx"; | |
11 | + | |
12 | +import CommonUtil from "../../../resources/js/CommonUtil.js"; | |
13 | + | |
14 | + | |
15 | +export default function UserAuthoriySelect() { | |
16 | + const navigate = useNavigate(); | |
17 | + const location = useLocation(); | |
18 | + | |
19 | + //전역 변수 저장 객체 | |
20 | + const state = useSelector((state) => { return state }); | |
21 | + const defaultUserId = CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['user_id']; | |
22 | + | |
23 | + //검색(엔터) | |
24 | + const searchingEnter = (key) => { | |
25 | + if (key == 'Enter') { | |
26 | + searching(); | |
27 | + } else { | |
28 | + return; | |
29 | + } | |
30 | + } | |
31 | + //검색 | |
32 | + const searching = () => { | |
33 | + if (CommonUtil.isEmpty(state.loginUser) == false | |
34 | + && state.loginUser['authority'] == 'ROLE_AGENCY' && isMySenior) { | |
35 | + senior.search['agent_id'] = state.loginUser['user_id']; | |
36 | + } else { | |
37 | + senior.search['agent_id'] = null; | |
38 | + } | |
39 | + | |
40 | + setSenior({ ...senior }); | |
41 | + | |
42 | + seniorSelectList(1); | |
43 | + } | |
44 | + | |
45 | + const [isMySenior, setIsMySenior] = React.useState(false); | |
46 | + React.useEffect(() => { | |
47 | + searching(); | |
48 | + }, [isMySenior]) | |
49 | + //보호사(간호사)의 돌봄 대상자(시니어) | |
50 | + const [senior, setSenior] = React.useState({ | |
51 | + seniorList: [], seniorListCount: 0, search: { | |
52 | + 'government_id': CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['government_id'], | |
53 | + 'agency_id': CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['agency_id'], | |
54 | + 'searchType': null, | |
55 | + 'searchText': null, | |
56 | + 'currentPage': 1, | |
57 | + 'perPage': 10, | |
58 | + } | |
59 | + }); | |
60 | + //보호사(간호사)의 돌봄 대상자(시니어) 목록 조회 | |
61 | + const seniorSelectList = (currentPage) => { | |
62 | + senior.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; | |
63 | + | |
64 | + fetch("/user/seniorSelectList.json", { | |
65 | + method: "POST", | |
66 | + headers: { | |
67 | + 'Content-Type': 'application/json; charset=UTF-8' | |
68 | + }, | |
69 | + body: JSON.stringify(senior.search), | |
70 | + }).then((response) => response.json()).then((data) => { | |
71 | + data.search = senior.search; | |
72 | + console.log("돌봄 대상자(시니어) 목록 조회 : ", data); | |
73 | + setSenior(data); | |
74 | + }).catch((error) => { | |
75 | + console.log('seniorSelectList() /user/seniorSelectList.json error : ', error); | |
76 | + }); | |
77 | + } | |
78 | + | |
79 | + | |
80 | + //현재 선택한 대상자 정보 | |
81 | + const [targetSenior, setTargetSenior] = React.useState({ 'senior_id': null }); | |
82 | + | |
83 | + //문진표 | |
84 | + const [modalOpen, setModalOpen] = React.useState(false); | |
85 | + const openModal = (senior) => { | |
86 | + targetSenior['senior_id'] = senior['senior_id']; | |
87 | + setTargetSenior(senior); | |
88 | + questionnaireSelectList(); | |
89 | + | |
90 | + setModalOpen(true); | |
91 | + }; | |
92 | + const closeModal = () => { | |
93 | + setTargetSenior({ 'senior_id': null }); | |
94 | + setModalOpen(false); | |
95 | + }; | |
96 | + //내원기록 | |
97 | + const [modalOpen2, setModalOpen2] = React.useState(false); | |
98 | + const openModal2 = (senior) => { | |
99 | + targetSenior['senior_id'] = senior['senior_id']; | |
100 | + setTargetSenior(senior); | |
101 | + hospitalMedicalRecordSelectList(); | |
102 | + | |
103 | + setModalOpen2(true); | |
104 | + }; | |
105 | + const closeModal2 = () => { | |
106 | + setTargetSenior({ 'senior_id': null }); | |
107 | + setModalOpen2(false); | |
108 | + }; | |
109 | + //심전도 | |
110 | + const [modalOpen3, setModalOpen3] = React.useState(false); | |
111 | + const openModal3 = (senior) => { | |
112 | + targetSenior['senior_id'] = senior['senior_id']; | |
113 | + setTargetSenior(senior); | |
114 | + ecgSelectList(); | |
115 | + | |
116 | + setModalOpen3(true); | |
117 | + }; | |
118 | + const closeModal3 = () => { | |
119 | + setTargetSenior({ 'senior_id': null }); | |
120 | + setModalOpen3(false); | |
121 | + }; | |
122 | + //혈압 | |
123 | + const [modalOpen4, setModalOpen4] = React.useState(false); | |
124 | + const openModal4 = (senior) => { | |
125 | + targetSenior['senior_id'] = senior['senior_id']; | |
126 | + setTargetSenior(senior); | |
127 | + bloodPressureSelectList(); | |
128 | + | |
129 | + setModalOpen4(true); | |
130 | + }; | |
131 | + const closeModal4 = () => { | |
132 | + setTargetSenior({ 'senior_id': null }); | |
133 | + setModalOpen4(false); | |
134 | + }; | |
135 | + | |
136 | + /****************** 문진표 (시작) ******************/ | |
137 | + const questionnaireInit = { | |
138 | + 'senior_id': null, | |
139 | + 'questionnaire_record_idx': null, | |
140 | + 'weight_change_amount': 0, | |
141 | + 'past_history': null, | |
142 | + 'agent_id': defaultUserId, | |
143 | + 'medical_record_idx': null, | |
144 | + | |
145 | + 'smoke_type': false, | |
146 | + 'no_smoke_period': null, | |
147 | + 'smoke_period': null, | |
148 | + 'daily_smoke_amount': null, | |
149 | + | |
150 | + 'drink_type': false, | |
151 | + 'weekly_drink_amount': null, | |
152 | + 'onetime_alcoholic_kind': null, | |
153 | + 'onetime_bottle_amount': null, | |
154 | + 'onetime_cup_amount': null, | |
155 | + | |
156 | + 'exercise_type': false, | |
157 | + 'weekly_exercise_amount': null, | |
158 | + 'onetime_exercise_hour': null, | |
159 | + 'onetime_exercise_minute': null, | |
160 | + 'onetime_exercise_kind': null, | |
161 | + | |
162 | + 'medication_pill': '', | |
163 | + 'medication_pill_etc': '', | |
164 | + }; | |
165 | + //문진표 정보 | |
166 | + const [questionnaire, setQuestionnaire] = React.useState({ ...questionnaireInit }); | |
167 | + const questionnaireRef = React.useRef({ ...questionnaireInit }); | |
168 | + | |
169 | + //문진표 등록 | |
170 | + const questionnaireInsert = () => { | |
171 | + questionnaire['senior_id'] = targetSenior['senior_id']; | |
172 | + questionnaire['agent_id'] = defaultUserId; | |
173 | + setQuestionnaire({ ...questionnaire }); | |
174 | + | |
175 | + fetch("/hospital/questionnaireInsert.json", { | |
176 | + method: "POST", | |
177 | + headers: { | |
178 | + 'Content-Type': 'application/json; charset=UTF-8' | |
179 | + }, | |
180 | + body: JSON.stringify(questionnaire), | |
181 | + }).then((response) => response.json()).then((data) => { | |
182 | + console.log("문진표 등록 결과(건수) : ", data); | |
183 | + if (data > 0) { | |
184 | + alert("저장완료"); | |
185 | + closeModal(); | |
186 | + } else { | |
187 | + alert("저장에 실패하였습니다. 관리자에게 문의바랍니다."); | |
188 | + } | |
189 | + }).catch((error) => { | |
190 | + console.log('questionnaireInsert() /hospital/questionnaireInsert.json error : ', error); | |
191 | + }); | |
192 | + } | |
193 | + | |
194 | + //문진표 수정 | |
195 | + const questionnaireUpdate = () => { | |
196 | + questionnaire['senior_id'] = targetSenior['senior_id']; | |
197 | + questionnaire['agent_id'] = defaultUserId; | |
198 | + setQuestionnaire({ ...questionnaire }); | |
199 | + | |
200 | + fetch("/hospital/questionnaireUpdate.json", { | |
201 | + method: "POST", | |
202 | + headers: { | |
203 | + 'Content-Type': 'application/json; charset=UTF-8' | |
204 | + }, | |
205 | + body: JSON.stringify(questionnaire), | |
206 | + }).then((response) => response.json()).then((data) => { | |
207 | + console.log("문진표 수정 결과(건수) : ", data); | |
208 | + if (data > 0) { | |
209 | + alert("저장완료"); | |
210 | + closeModal(); | |
211 | + } else { | |
212 | + alert("저장에 실패하였습니다. 관리자에게 문의바랍니다."); | |
213 | + } | |
214 | + }).catch((error) => { | |
215 | + console.log('questionnaireUpdate() /hospital/questionnaireUpdate.json error : ', error); | |
216 | + }); | |
217 | + } | |
218 | + | |
219 | + //문진표 목록 조회 | |
220 | + const questionnaireSelectList = () => { | |
221 | + fetch("/hospital/questionnaireSelectList.json", { | |
222 | + method: "POST", | |
223 | + headers: { | |
224 | + 'Content-Type': 'application/json; charset=UTF-8' | |
225 | + }, | |
226 | + body: JSON.stringify(targetSenior), | |
227 | + }).then((response) => response.json()).then((data) => { | |
228 | + console.log("문진표 목록 조회 결과(건수) : ", data); | |
229 | + if (CommonUtil.isEmpty(data.questionnaireList) == false) { | |
230 | + let item = data.questionnaireList[0];//최신순으로 가지고오기 때문에, 0 인텍스가 젤 최신 | |
231 | + if (CommonUtil.isEmpty(item['medication_pill'])) { | |
232 | + item['medication_pill'] = ''; | |
233 | + } else { | |
234 | + const startCheckWord = '기타「'; | |
235 | + const endCheckWord = '」'; | |
236 | + if (item['medication_pill'].indexOf(startCheckWord) > -1) { | |
237 | + let startIndex = item['medication_pill'].indexOf(startCheckWord); | |
238 | + let lastIndex = item['medication_pill'].lastIndexOf(endCheckWord); | |
239 | + console.log("000000 item['medication_pill_etc'] : ", item['medication_pill_etc'], "item['medication_pill'] : ", item['medication_pill']); | |
240 | + item['medication_pill_etc'] = item['medication_pill'].substring(startIndex + startCheckWord.length, lastIndex); | |
241 | + let medicationPillFront = item['medication_pill'].substring(0, startIndex + startCheckWord.length); | |
242 | + let medicationPillBack = item['medication_pill'].substring(lastIndex); | |
243 | + item['medication_pill'] = medicationPillFront + medicationPillBack; | |
244 | + console.log("333333 item['medication_pill_etc'] : ", item['medication_pill_etc'], "item['medication_pill'] : ", item['medication_pill']); | |
245 | + } else { | |
246 | + item['medication_pill_etc'] = ''; | |
247 | + } | |
248 | + } | |
249 | + | |
250 | + setQuestionnaire(item); | |
251 | + } | |
252 | + }).catch((error) => { | |
253 | + console.log('questionnaireSelectList() /hospital/questionnaireSelectList.json error : ', error); | |
254 | + }); | |
255 | + } | |
256 | + /****************** 문진표 (종료) ******************/ | |
257 | + | |
258 | + | |
259 | + | |
260 | + /****************** 병원 진료 기록 (시작) ******************/ | |
261 | + const hospitalMedicalRecordInit = { | |
262 | + 'senior_id': null, | |
263 | + 'medical_record_idx': null, | |
264 | + 'medical_date': CommonUtil.getDate(), | |
265 | + 'medical_reason': '', | |
266 | + 'medical_content': '', | |
267 | + 'agent_id': defaultUserId | |
268 | + }; | |
269 | + //병원 진료 기록 정보 | |
270 | + const [hospitalMedicalRecord, setHospitalMedicalRecord] = React.useState({ ...hospitalMedicalRecordInit }); | |
271 | + const hospitalMedicalRecordRef = React.useRef({ ...hospitalMedicalRecordInit }); | |
272 | + | |
273 | + //병원 진료 기록 유효성 검사 | |
274 | + const hospitalMedicalRecordValidation = () => { | |
275 | + const target = hospitalMedicalRecord; | |
276 | + const targetRef = hospitalMedicalRecordRef; | |
277 | + | |
278 | + if (CommonUtil.isEmpty(target['medical_date']) == true) { | |
279 | + targetRef.current['medical_date'].focus(); | |
280 | + alert("진료 일자를 선택해 주세요."); | |
281 | + return false; | |
282 | + } | |
283 | + if (CommonUtil.isEmpty(target['medical_reason']) == true) { | |
284 | + targetRef.current['medical_reason'].focus(); | |
285 | + alert("진료 사유를 입력해 주세요."); | |
286 | + return false; | |
287 | + } | |
288 | + if (CommonUtil.isEmpty(target['medical_content']) == true) { | |
289 | + targetRef.current['medical_content'].focus(); | |
290 | + alert("진료 내용을 입력해 주세요."); | |
291 | + return false; | |
292 | + } | |
293 | + | |
294 | + return true; | |
295 | + } | |
296 | + | |
297 | + | |
298 | + //병원 진료 기록 등록 | |
299 | + const hospitalMedicalRecordInsert = () => { | |
300 | + if (hospitalMedicalRecordValidation() == false) { | |
301 | + return; | |
302 | + } | |
303 | + | |
304 | + hospitalMedicalRecord['senior_id'] = targetSenior['senior_id']; | |
305 | + hospitalMedicalRecord['agent_id'] = defaultUserId; | |
306 | + setHospitalMedicalRecord({ ...hospitalMedicalRecord }); | |
307 | + | |
308 | + fetch("/hospital/hospitalMedicalRecordInsert.json", { | |
309 | + method: "POST", | |
310 | + headers: { | |
311 | + 'Content-Type': 'application/json; charset=UTF-8' | |
312 | + }, | |
313 | + body: JSON.stringify(hospitalMedicalRecord), | |
314 | + }).then((response) => response.json()).then((data) => { | |
315 | + console.log("병원 진료 기록 등록 결과(건수) : ", data); | |
316 | + if (data > 0) { | |
317 | + setHospitalMedicalRecordInit(); | |
318 | + hospitalMedicalRecordSelectList(); | |
319 | + //closeModal2(); | |
320 | + alert("등록완료"); | |
321 | + | |
322 | + } else { | |
323 | + alert("등록에 실패하였습니다. 관리자에게 문의바랍니다."); | |
324 | + } | |
325 | + }).catch((error) => { | |
326 | + console.log('hospitalMedicalRecordInsert() /hospital/hospitalMedicalRecordInsert.json error : ', error); | |
327 | + }); | |
328 | + } | |
329 | + | |
330 | + //병원 진료 기록 수정 | |
331 | + const hospitalMedicalRecordUpdate = () => { | |
332 | + if (hospitalMedicalRecordValidation() == false) { | |
333 | + return; | |
334 | + } | |
335 | + | |
336 | + hospitalMedicalRecord['senior_id'] = targetSenior['senior_id']; | |
337 | + hospitalMedicalRecord['agent_id'] = defaultUserId; | |
338 | + setHospitalMedicalRecord({ ...hospitalMedicalRecord }); | |
339 | + | |
340 | + fetch("/hospital/hospitalMedicalRecordUpdate.json", { | |
341 | + method: "POST", | |
342 | + headers: { | |
343 | + 'Content-Type': 'application/json; charset=UTF-8' | |
344 | + }, | |
345 | + body: JSON.stringify(hospitalMedicalRecord), | |
346 | + }).then((response) => response.json()).then((data) => { | |
347 | + console.log("병원 진료 기록 수정 결과(건수) : ", data); | |
348 | + if (data > 0) { | |
349 | + setHospitalMedicalRecordInit(); | |
350 | + hospitalMedicalRecordSelectList(); | |
351 | + //closeModal2(); | |
352 | + alert("수정완료"); | |
353 | + } else { | |
354 | + alert("수정에 실패하였습니다. 관리자에게 문의바랍니다."); | |
355 | + } | |
356 | + }).catch((error) => { | |
357 | + console.log('hospitalMedicalRecordUpdate() /hospital/hospitalMedicalRecordUpdate.json error : ', error); | |
358 | + }); | |
359 | + } | |
360 | + | |
361 | + //병원 진료 기록 삭제 | |
362 | + const hospitalMedicalRecordDelete = () => { | |
363 | + if (confirm('진료 기록을 삭제하시겠습니까?') == false) { | |
364 | + return; | |
365 | + } | |
366 | + | |
367 | + fetch("/hospital/hospitalMedicalRecordDelete.json", { | |
368 | + method: "POST", | |
369 | + headers: { | |
370 | + 'Content-Type': 'application/json; charset=UTF-8' | |
371 | + }, | |
372 | + body: JSON.stringify(hospitalMedicalRecord), | |
373 | + }).then((response) => response.json()).then((data) => { | |
374 | + console.log("병원 진료 기록 삭제 결과(건수) : ", data); | |
375 | + if (data > 0) { | |
376 | + setHospitalMedicalRecordInit(); | |
377 | + hospitalMedicalRecordSelectList(); | |
378 | + //closeModal2(); | |
379 | + alert("삭제완료"); | |
380 | + } else { | |
381 | + alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다."); | |
382 | + } | |
383 | + }).catch((error) => { | |
384 | + console.log('hospitalMedicalRecordDelete() /hospital/hospitalMedicalRecordDelete.json error : ', error); | |
385 | + }); | |
386 | + } | |
387 | + | |
388 | + //초기화 취소 | |
389 | + const setHospitalMedicalRecordInit = () => { | |
390 | + setHospitalMedicalRecord({ ...hospitalMedicalRecordInit }); | |
391 | + } | |
392 | + | |
393 | + //병원 진료 기록 정보 | |
394 | + const [hospitalMedicalRecordList, setHospitalMedicalRecordList] = React.useState({ hospitalMedicalRecordList: [], hospitalMedicalRecordListCount: 0, search: { currentPage: 1, perPage: 5 } }); | |
395 | + //병원 진료 기록 목록 조회 | |
396 | + const hospitalMedicalRecordSelectList = (currentPage) => { | |
397 | + hospitalMedicalRecordList.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; | |
398 | + hospitalMedicalRecordList.search['senior_id'] = targetSenior['senior_id']; | |
399 | + setHospitalMedicalRecordList({ ...hospitalMedicalRecordList }); | |
400 | + | |
401 | + fetch("/hospital/hospitalMedicalRecordSelectList.json", { | |
402 | + method: "POST", | |
403 | + headers: { | |
404 | + 'Content-Type': 'application/json; charset=UTF-8' | |
405 | + }, | |
406 | + body: JSON.stringify(hospitalMedicalRecordList.search), | |
407 | + }).then((response) => response.json()).then((data) => { | |
408 | + console.log("병원 진료 기록 목록 조회 결과(건수) : ", data); | |
409 | + data.search = hospitalMedicalRecordList.search; | |
410 | + setHospitalMedicalRecordList(data); | |
411 | + }).catch((error) => { | |
412 | + console.log('hospitalMedicalRecordSelectList() /hospital/hospitalMedicalRecordSelectList.json error : ', error); | |
413 | + }); | |
414 | + } | |
415 | + /****************** 병원 진료 기록 (종료) ******************/ | |
416 | + | |
417 | + | |
418 | + | |
419 | + /****************** 심전도 (시작) ******************/ | |
420 | + const ecgInit = { | |
421 | + 'senior_id': null, | |
422 | + 'ecg_record_idx': null, | |
423 | + 'bradycardia_count': 0, | |
424 | + 'tachycardia_count': 0, | |
425 | + 'max_heart_rate': 0.0, | |
426 | + 'min_heart_rate': 0.0, | |
427 | + 'abnormal_heart_rate': 0.0, | |
428 | + 'average_heart_rate': 0.0, | |
429 | + 'max_rr': 0.0, | |
430 | + 'min_rr': 0.0, | |
431 | + 'average_rr': 0.0, | |
432 | + 'ecg_reading_date': CommonUtil.getDate(), | |
433 | + 'ecg_finding_type': '', | |
434 | + 'ecg_finding_content': '', | |
435 | + 'agent_id': defaultUserId, | |
436 | + 'medical_record_idx': null, | |
437 | + 'common_group_file_idx': null, | |
438 | + commonFileList: [],//심전도 업로드 파일 목록 | |
439 | + | |
440 | + isEdit: false,//직접 수정일 때, true (수정 or 등록 상관없음); | |
441 | + }; | |
442 | + //심전도 정보 | |
443 | + const [ecg, setEcg] = React.useState({ ...ecgInit }); | |
444 | + const ecgRef = React.useRef({ ...ecgInit }); | |
445 | + | |
446 | + //심전도 유효성 검사 | |
447 | + const ecgValidation = () => { | |
448 | + const target = ecg; | |
449 | + const targetRef = ecgRef; | |
450 | + | |
451 | + if (CommonUtil.isEmpty(target['ecg_reading_date']) == true) { | |
452 | + targetRef.current['ecg_reading_date'].focus(); | |
453 | + alert("판독 소견 측정 일자를 선택해 주세요."); | |
454 | + return false; | |
455 | + } | |
456 | + if (CommonUtil.isEmpty(target['ecg_finding_type']) == true) { | |
457 | + targetRef.current['ecg_finding_type'].focus(); | |
458 | + alert("판독 소견 측정 종류를 입력해 주세요."); | |
459 | + return false; | |
460 | + } | |
461 | + if (CommonUtil.isEmpty(target['ecg_finding_content']) == true) { | |
462 | + targetRef.current['ecg_finding_content'].focus(); | |
463 | + alert("판독 소견 측정 내용을 입력해 주세요."); | |
464 | + return false; | |
465 | + } | |
466 | + //파일 입력 | |
467 | + if (target.isEdit == false) { | |
468 | + if (CommonUtil.isEmpty(target['commonFileList']) == true) { | |
469 | + //targetRef.current['commonFileList'].focus(); | |
470 | + alert("심전도 측정 파일을 올려주세요."); | |
471 | + return false; | |
472 | + } | |
473 | + } else {//직접 입력 | |
474 | + if (CommonUtil.isEmpty(target['bradycardia_count']) == true) { | |
475 | + targetRef.current['bradycardia_count'].focus(); | |
476 | + alert("서맥 횟수를 입력해 주세요."); | |
477 | + return false; | |
478 | + } | |
479 | + if (CommonUtil.isEmpty(target['tachycardia_count']) == true) { | |
480 | + targetRef.current['tachycardia_count'].focus(); | |
481 | + alert("빈맥 횟수 입력해 주세요."); | |
482 | + return false; | |
483 | + } | |
484 | + if (CommonUtil.isEmpty(target['max_heart_rate']) == true) { | |
485 | + targetRef.current['average_heart_rate'].focus(); | |
486 | + alert("최대 심박수를 입력해 주세요."); | |
487 | + return false; | |
488 | + } | |
489 | + if (CommonUtil.isEmpty(target['min_heart_rate']) == true) { | |
490 | + targetRef.current['average_heart_rate'].focus(); | |
491 | + alert("최소 심박수를 입력해 주세요."); | |
492 | + return false; | |
493 | + } | |
494 | + if (CommonUtil.isEmpty(target['average_heart_rate']) == true) { | |
495 | + targetRef.current['average_heart_rate'].focus(); | |
496 | + alert("평균 심박수를 입력해 주세요."); | |
497 | + return false; | |
498 | + } | |
499 | + if (CommonUtil.isEmpty(target['abnormal_heart_rate']) == true) { | |
500 | + targetRef.current['abnormal_heart_rate'].focus(); | |
501 | + alert("이상 심박수를 입력해 주세요."); | |
502 | + return false; | |
503 | + } | |
504 | + if (CommonUtil.isEmpty(target['max_rr']) == true) { | |
505 | + targetRef.current['max_rr'].focus(); | |
506 | + alert("최대 R-R를 입력해 주세요."); | |
507 | + return false; | |
508 | + } | |
509 | + if (CommonUtil.isEmpty(target['min_rr']) == true) { | |
510 | + targetRef.current['min_rr'].focus(); | |
511 | + alert("최소 R-R를 입력해 주세요."); | |
512 | + return false; | |
513 | + } | |
514 | + if (CommonUtil.isEmpty(target['average_rr']) == true) { | |
515 | + targetRef.current['average_rr'].focus(); | |
516 | + alert("평균 R-R를 입력해 주세요."); | |
517 | + return false; | |
518 | + } | |
519 | + } | |
520 | + | |
521 | + return true; | |
522 | + } | |
523 | + | |
524 | + | |
525 | + //심전도 등록 | |
526 | + const ecgInsert = () => { | |
527 | + if (ecgValidation() == false) { | |
528 | + return; | |
529 | + } | |
530 | + | |
531 | + ecg['senior_id'] = targetSenior['senior_id']; | |
532 | + ecg['agent_id'] = defaultUserId; | |
533 | + setEcg({ ...ecg }); | |
534 | + | |
535 | + fetch("/hospital/ecgInsert.json", { | |
536 | + method: "POST", | |
537 | + headers: { | |
538 | + 'Content-Type': 'application/json; charset=UTF-8' | |
539 | + }, | |
540 | + body: JSON.stringify(ecg), | |
541 | + }).then((response) => response.json()).then((data) => { | |
542 | + console.log("심전도 등록 결과(건수) : ", data); | |
543 | + if (data > 0) { | |
544 | + setEcgInit(); | |
545 | + ecgSelectList(); | |
546 | + //closeModal3(); | |
547 | + alert("등록완료"); | |
548 | + | |
549 | + } else { | |
550 | + alert("등록에 실패하였습니다. 관리자에게 문의바랍니다."); | |
551 | + } | |
552 | + }).catch((error) => { | |
553 | + console.log('ecgInsert() /hospital/ecgInsert.json error : ', error); | |
554 | + }); | |
555 | + } | |
556 | + | |
557 | + //심전도 수정 | |
558 | + const ecgUpdate = () => { | |
559 | + if (ecgValidation() == false) { | |
560 | + return; | |
561 | + } | |
562 | + | |
563 | + ecg['senior_id'] = targetSenior['senior_id']; | |
564 | + ecg['agent_id'] = defaultUserId; | |
565 | + setEcg({ ...ecg }); | |
566 | + | |
567 | + fetch("/hospital/ecgUpdate.json", { | |
568 | + method: "POST", | |
569 | + headers: { | |
570 | + 'Content-Type': 'application/json; charset=UTF-8' | |
571 | + }, | |
572 | + body: JSON.stringify(ecg), | |
573 | + }).then((response) => response.json()).then((data) => { | |
574 | + console.log("심전도 수정 결과(건수) : ", data); | |
575 | + if (data > 0) { | |
576 | + setEcgInit(); | |
577 | + ecg.commonFileList = []; | |
578 | + setEcg({ ...ecg }); | |
579 | + ecgSelectList(); | |
580 | + //closeModal3(); | |
581 | + alert("수정완료"); | |
582 | + } else { | |
583 | + alert("수정에 실패하였습니다. 관리자에게 문의바랍니다."); | |
584 | + } | |
585 | + }).catch((error) => { | |
586 | + console.log('ecgUpdate() /hospital/ecgUpdate.json error : ', error); | |
587 | + }); | |
588 | + } | |
589 | + | |
590 | + //심전도 삭제 | |
591 | + const ecgDelete = () => { | |
592 | + if (confirm('심전도 판독 소견을 삭제하시겠습니까?') == false) { | |
593 | + return; | |
594 | + } | |
595 | + | |
596 | + fetch("/hospital/ecgDelete.json", { | |
597 | + method: "POST", | |
598 | + headers: { | |
599 | + 'Content-Type': 'application/json; charset=UTF-8' | |
600 | + }, | |
601 | + body: JSON.stringify(ecg), | |
602 | + }).then((response) => response.json()).then((data) => { | |
603 | + console.log("심전도 삭제 결과(건수) : ", data); | |
604 | + if (data > 0) { | |
605 | + setEcgInit(); | |
606 | + ecg.commonFileList = []; | |
607 | + setEcg({ ...ecg }); | |
608 | + ecgSelectList(); | |
609 | + //closeModal3(); | |
610 | + alert("삭제완료"); | |
611 | + } else { | |
612 | + alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다."); | |
613 | + } | |
614 | + }).catch((error) => { | |
615 | + console.log('ecgDelete() /hospital/ecgDelete.json error : ', error); | |
616 | + }); | |
617 | + } | |
618 | + | |
619 | + //초기화 취소 | |
620 | + const setEcgInit = () => { | |
621 | + setEcg({ ...ecgInit }); | |
622 | + } | |
623 | + | |
624 | + //심전도 정보 | |
625 | + const [ecgList, setEcgList] = React.useState({ ecgList: [], ecgListCount: 0, search: { currentPage: 1, perPage: 5 } }); | |
626 | + //심전도 목록 조회 | |
627 | + const ecgSelectList = (currentPage) => { | |
628 | + ecgList.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; | |
629 | + ecgList.search['senior_id'] = targetSenior['senior_id']; | |
630 | + setEcgList({ ...ecgList }); | |
631 | + | |
632 | + fetch("/hospital/ecgSelectList.json", { | |
633 | + method: "POST", | |
634 | + headers: { | |
635 | + 'Content-Type': 'application/json; charset=UTF-8' | |
636 | + }, | |
637 | + body: JSON.stringify(ecgList.search), | |
638 | + }).then((response) => response.json()).then((data) => { | |
639 | + console.log("심전도 목록 조회 결과(건수) : ", data); | |
640 | + data.search = ecgList.search; | |
641 | + setEcgList(data); | |
642 | + }).catch((error) => { | |
643 | + console.log('ecgSelectList() /hospital/ecgSelectList.json error : ', error); | |
644 | + }); | |
645 | + } | |
646 | + /****************** 심전도 기록 (종료) ******************/ | |
647 | + | |
648 | + | |
649 | + | |
650 | + /****************** 혈압 (시작) ******************/ | |
651 | + const bloodPressureInit = { | |
652 | + 'senior_id': null, | |
653 | + 'blood_pressure_record_idx': null, | |
654 | + 'max_blood_pressure': null, | |
655 | + 'min_blood_pressure': null, | |
656 | + 'pulse_rate': null, | |
657 | + 'medical_record_idx': null, | |
658 | + 'blood_pressure_record_date': CommonUtil.getDate(), | |
659 | + 'agent_id': defaultUserId | |
660 | + }; | |
661 | + //혈압 정보 | |
662 | + const [bloodPressure, setBloodPressure] = React.useState({ ...bloodPressureInit }); | |
663 | + const bloodPressureRef = React.useRef({ ...bloodPressureInit }); | |
664 | + | |
665 | + //혈압 유효성 검사 | |
666 | + const bloodPressureValidation = () => { | |
667 | + const target = bloodPressure; | |
668 | + const targetRef = bloodPressureRef; | |
669 | + | |
670 | + if (CommonUtil.isEmpty(target['blood_pressure_record_date']) == true) { | |
671 | + targetRef.current['blood_pressure_record_date'].focus(); | |
672 | + alert("진료 일자를 선택해 주세요."); | |
673 | + return false; | |
674 | + } | |
675 | + if (CommonUtil.isEmpty(target['max_blood_pressure']) == true) { | |
676 | + targetRef.current['max_blood_pressure'].focus(); | |
677 | + alert("최고 혈압을 입력해 주세요."); | |
678 | + return false; | |
679 | + } | |
680 | + if (CommonUtil.isEmpty(target['min_blood_pressure']) == true) { | |
681 | + targetRef.current['min_blood_pressure'].focus(); | |
682 | + alert("최저 혈압을 입력해 주세요."); | |
683 | + return false; | |
684 | + } | |
685 | + if (CommonUtil.isEmpty(target['pulse_rate']) == true) { | |
686 | + targetRef.current['pulse_rate'].focus(); | |
687 | + alert("맥박수를 입력해 주세요."); | |
688 | + return false; | |
689 | + } | |
690 | + | |
691 | + return true; | |
692 | + } | |
693 | + | |
694 | + | |
695 | + //혈압 등록 | |
696 | + const bloodPressureInsert = () => { | |
697 | + if (bloodPressureValidation() == false) { | |
698 | + return; | |
699 | + } | |
700 | + | |
701 | + bloodPressure['senior_id'] = targetSenior['senior_id']; | |
702 | + bloodPressure['agent_id'] = defaultUserId; | |
703 | + setBloodPressure({ ...bloodPressure }); | |
704 | + | |
705 | + fetch("/hospital/bloodPressureInsert.json", { | |
706 | + method: "POST", | |
707 | + headers: { | |
708 | + 'Content-Type': 'application/json; charset=UTF-8' | |
709 | + }, | |
710 | + body: JSON.stringify(bloodPressure), | |
711 | + }).then((response) => response.json()).then((data) => { | |
712 | + console.log("혈압 등록 결과(건수) : ", data); | |
713 | + if (data > 0) { | |
714 | + setBloodPressureInit(); | |
715 | + bloodPressureSelectList(); | |
716 | + //closeModal2(); | |
717 | + alert("등록완료"); | |
718 | + | |
719 | + } else { | |
720 | + alert("등록에 실패하였습니다. 관리자에게 문의바랍니다."); | |
721 | + } | |
722 | + }).catch((error) => { | |
723 | + console.log('bloodPressureInsert() /hospital/bloodPressureInsert.json error : ', error); | |
724 | + }); | |
725 | + } | |
726 | + | |
727 | + //혈압 수정 | |
728 | + const bloodPressureUpdate = () => { | |
729 | + if (bloodPressureValidation() == false) { | |
730 | + return; | |
731 | + } | |
732 | + | |
733 | + bloodPressure['senior_id'] = targetSenior['senior_id']; | |
734 | + bloodPressure['agent_id'] = defaultUserId; | |
735 | + setBloodPressure({ ...bloodPressure }); | |
736 | + | |
737 | + fetch("/hospital/bloodPressureUpdate.json", { | |
738 | + method: "POST", | |
739 | + headers: { | |
740 | + 'Content-Type': 'application/json; charset=UTF-8' | |
741 | + }, | |
742 | + body: JSON.stringify(bloodPressure), | |
743 | + }).then((response) => response.json()).then((data) => { | |
744 | + console.log("혈압 수정 결과(건수) : ", data); | |
745 | + if (data > 0) { | |
746 | + setBloodPressureInit(); | |
747 | + bloodPressureSelectList(); | |
748 | + //closeModal2(); | |
749 | + alert("수정완료"); | |
750 | + } else { | |
751 | + alert("수정에 실패하였습니다. 관리자에게 문의바랍니다."); | |
752 | + } | |
753 | + }).catch((error) => { | |
754 | + console.log('bloodPressureUpdate() /hospital/bloodPressureUpdate.json error : ', error); | |
755 | + }); | |
756 | + } | |
757 | + | |
758 | + //혈압 삭제 | |
759 | + const bloodPressureDelete = () => { | |
760 | + if (confirm('혈압 측정 정보를 삭제하시겠습니까?') == false) { | |
761 | + return; | |
762 | + } | |
763 | + | |
764 | + fetch("/hospital/bloodPressureDelete.json", { | |
765 | + method: "POST", | |
766 | + headers: { | |
767 | + 'Content-Type': 'application/json; charset=UTF-8' | |
768 | + }, | |
769 | + body: JSON.stringify(bloodPressure), | |
770 | + }).then((response) => response.json()).then((data) => { | |
771 | + console.log("혈압 삭제 결과(건수) : ", data); | |
772 | + if (data > 0) { | |
773 | + setBloodPressureInit(); | |
774 | + bloodPressureSelectList(); | |
775 | + //closeModal2(); | |
776 | + alert("삭제완료"); | |
777 | + } else { | |
778 | + alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다."); | |
779 | + } | |
780 | + }).catch((error) => { | |
781 | + console.log('bloodPressureDelete() /hospital/bloodPressureDelete.json error : ', error); | |
782 | + }); | |
783 | + } | |
784 | + | |
785 | + //초기화 취소 | |
786 | + const setBloodPressureInit = () => { | |
787 | + setBloodPressure({ ...bloodPressureInit }); | |
788 | + } | |
789 | + | |
790 | + //혈압 정보 | |
791 | + const [bloodPressureList, setBloodPressureList] = React.useState({ bloodPressureList: [], bloodPressureListCount: 0, search: { currentPage: 1, perPage: 5 } }); | |
792 | + //혈압 목록 조회 | |
793 | + const bloodPressureSelectList = (currentPage) => { | |
794 | + bloodPressureList.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; | |
795 | + bloodPressureList.search['senior_id'] = targetSenior['senior_id']; | |
796 | + setBloodPressureList({ ...bloodPressureList }); | |
797 | + | |
798 | + fetch("/hospital/bloodPressureSelectList.json", { | |
799 | + method: "POST", | |
800 | + headers: { | |
801 | + 'Content-Type': 'application/json; charset=UTF-8' | |
802 | + }, | |
803 | + body: JSON.stringify(bloodPressureList.search), | |
804 | + }).then((response) => response.json()).then((data) => { | |
805 | + console.log("혈압 목록 조회 결과(건수) : ", data); | |
806 | + data.search = bloodPressureList.search; | |
807 | + setBloodPressureList(data); | |
808 | + }).catch((error) => { | |
809 | + console.log('bloodPressureSelectList() /hospital/bloodPressureSelectList.json error : ', error); | |
810 | + }); | |
811 | + } | |
812 | + /****************** 혈압 (종료) ******************/ | |
813 | + | |
814 | + React.useEffect(() => { | |
815 | + searching(); | |
816 | + }, []); | |
817 | + | |
818 | + | |
819 | + //현재 탭 Index | |
820 | + const [tabIndex, setTabIndex] = React.useState(0); | |
821 | + //탭 초기화 | |
822 | + const tab = [{ | |
823 | + title: `문진표관리`, | |
824 | + content: ( | |
825 | + <div> | |
826 | + <div className="flex equip-tab"> | |
827 | + <SubTitle explanation={"돌봄 대상자의 병원 내원 기록 및 문진표를 관리할 수 있습니다."} /> | |
828 | + </div> | |
829 | + <table className={"protector-user"}> | |
830 | + <thead> | |
831 | + <tr> | |
832 | + <th>No</th> | |
833 | + <th>소속기관명</th> | |
834 | + <th>이름</th> | |
835 | + <th>생년월일</th> | |
836 | + <th>성별</th> | |
837 | + <th>연락처</th> | |
838 | + <th>문진표관리</th> | |
839 | + <th>내원기록관리</th> | |
840 | + </tr> | |
841 | + </thead> | |
842 | + <tbody> | |
843 | + {senior.seniorList.map((item, idx) => { | |
844 | + return ( | |
845 | + <tr key={idx}> | |
846 | + <td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td> | |
847 | + <td data-label="소속기관명">{item['agency_name']}</td> | |
848 | + <td data-label="이름">{item['user_name']}</td> | |
849 | + <td data-label="생년월일">{item['user_birth']}</td> | |
850 | + <td data-label="성별">{item['user_gender']}</td> | |
851 | + <td data-label="연락처">{item['user_phonenumber']}</td> | |
852 | + <td data-label="문진표관리"> | |
853 | + <button className="btn-small gray-btn" onClick={() => openModal(item)}>작성하기</button> | |
854 | + </td> | |
855 | + <td data-label="내원기록관리"> | |
856 | + <button className="btn-small gray-btn" onClick={() => openModal2(item)}>작성하기</button> | |
857 | + </td> | |
858 | + </tr> | |
859 | + ) | |
860 | + })} | |
861 | + {CommonUtil.isEmpty(senior.seniorList) ? | |
862 | + <tr> | |
863 | + <td colSpan={8}>조회된 데이터가 없습니다</td> | |
864 | + </tr> | |
865 | + : null} | |
866 | + </tbody> | |
867 | + </table> | |
868 | + <Pagination | |
869 | + currentPage={senior.search.currentPage} | |
870 | + perPage={senior.search.perPage} | |
871 | + totalCount={senior.seniorListCount} | |
872 | + maxRange={5} | |
873 | + click={seniorSelectList} | |
874 | + /> | |
875 | + </div> | |
876 | + ) | |
877 | + }, { | |
878 | + title: `심전도관리`, | |
879 | + content: ( | |
880 | + <div> | |
881 | + <div className="flex equip-tab"> | |
882 | + <SubTitle explanation={"돌봄 대상자의 심전도 정보를 관리할 수 있습니다."} /> | |
883 | + </div> | |
884 | + <table className={"protector-user"}> | |
885 | + <thead> | |
886 | + <tr> | |
887 | + <th>No</th> | |
888 | + <th>소속기관명</th> | |
889 | + <th>이름</th> | |
890 | + <th>생년월일</th> | |
891 | + <th>성별</th> | |
892 | + <th>연락처</th> | |
893 | + <th>심전도관리</th> | |
894 | + </tr> | |
895 | + </thead> | |
896 | + <tbody> | |
897 | + {senior.seniorList.map((item, idx) => { | |
898 | + return ( | |
899 | + <tr key={idx}> | |
900 | + <td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td> | |
901 | + <td data-label="소속기관명">{item['agency_name']}</td> | |
902 | + <td data-label="이름">{item['user_name']}</td> | |
903 | + <td data-label="생년월일">{item['user_birth']}</td> | |
904 | + <td data-label="성별">{item['user_gender']}</td> | |
905 | + <td data-label="연락처">{item['user_phonenumber']}</td> | |
906 | + <td data-label="심전도관리"> | |
907 | + <button className="btn-small gray-btn" onClick={() => openModal3(item)}>심전도관리</button> | |
908 | + </td> | |
909 | + </tr> | |
910 | + ) | |
911 | + })} | |
912 | + {CommonUtil.isEmpty(senior.seniorList) ? | |
913 | + <tr> | |
914 | + <td colSpan={7}>조회된 데이터가 없습니다</td> | |
915 | + </tr> | |
916 | + : null} | |
917 | + </tbody> | |
918 | + </table> | |
919 | + <Pagination | |
920 | + currentPage={senior.search.currentPage} | |
921 | + perPage={senior.search.perPage} | |
922 | + totalCount={senior.seniorListCount} | |
923 | + maxRange={5} | |
924 | + click={seniorSelectList} | |
925 | + /> | |
926 | + </div> | |
927 | + ) | |
928 | + }, { | |
929 | + title: `혈압관리`, | |
930 | + content: ( | |
931 | + <div> | |
932 | + <div className="flex equip-tab"> | |
933 | + <SubTitle explanation={"돌봄 대상자의 혈압 정보를 관리할 수 있습니다."} /> | |
934 | + </div> | |
935 | + <table className={"protector-user"}> | |
936 | + <thead> | |
937 | + <tr> | |
938 | + <th>No</th> | |
939 | + <th>소속기관명</th> | |
940 | + <th>이름</th> | |
941 | + <th>생년월일</th> | |
942 | + <th>성별</th> | |
943 | + <th>연락처</th> | |
944 | + <th>혈압관리</th> | |
945 | + </tr> | |
946 | + </thead> | |
947 | + <tbody> | |
948 | + {senior.seniorList.map((item, idx) => { | |
949 | + return ( | |
950 | + <tr key={idx}> | |
951 | + <td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td> | |
952 | + <td data-label="소속기관명">{item['agency_name']}</td> | |
953 | + <td data-label="이름">{item['user_name']}</td> | |
954 | + <td data-label="생년월일">{item['user_birth']}</td> | |
955 | + <td data-label="성별">{item['user_gender']}</td> | |
956 | + <td data-label="연락처">{item['user_phonenumber']}</td> | |
957 | + <td data-label="혈압관리"> | |
958 | + <button className="btn-small gray-btn" onClick={() => openModal4(item)}>혈압관리</button> | |
959 | + </td> | |
960 | + </tr> | |
961 | + ) | |
962 | + })} | |
963 | + {CommonUtil.isEmpty(senior.seniorList) ? | |
964 | + <tr> | |
965 | + <td colSpan={7}>조회된 데이터가 없습니다</td> | |
966 | + </tr> | |
967 | + : null} | |
968 | + </tbody> | |
969 | + </table> | |
970 | + <Pagination | |
971 | + currentPage={senior.search.currentPage} | |
972 | + perPage={senior.search.perPage} | |
973 | + totalCount={senior.seniorListCount} | |
974 | + maxRange={5} | |
975 | + click={seniorSelectList} | |
976 | + /> | |
977 | + </div> | |
978 | + ) | |
979 | + }]; | |
980 | + | |
981 | + return ( | |
982 | + <main> | |
983 | + | |
984 | + {/* <Modal_Questionnaire open={modalOpen} close={closeModal} /> */} | |
985 | + {/* <Modal_MedicalHistory open={modalOpen2} close={closeModal2} /> */} | |
986 | + {/* <Modal_ECG open={modalOpen3} close={closeModal3} /> */} | |
987 | + {/* <Modal_Blood open={modalOpen4} close={closeModal4} /> */} | |
988 | + | |
989 | + <div className="search-management flex-start margin-bottom2"> | |
990 | + <select style={{ maxWidth: '150px' }} | |
991 | + onChange={(e) => { senior.search.searchType = e.target.value; setSenior({ ...senior }); }}> | |
992 | + <option value="" selected={CommonUtil.isEmpty(senior.search.searchType)}>전체</option> | |
993 | + <option value="user_name" selected={senior.search.searchType == 'user_name'}>이름</option> | |
994 | + <option value="user_id" selected={senior.search.searchType == 'user_id'}>아이디</option> | |
995 | + <option value="user_phonenumber" selected={senior.search.searchType == 'user_phonenumber'}>연락처</option> | |
996 | + </select> | |
997 | + <input type="text" className="senior-search" value={senior.search.searchText} | |
998 | + onChange={(e) => { senior.search.searchText = e.target.value; setSenior({ ...senior }); }} | |
999 | + onKeyUp={(e) => searchingEnter(e.key)} /> | |
1000 | + <button className="btn-small gray-btn" onClick={searching}>검색</button> | |
1001 | + </div> | |
1002 | + | |
1003 | + <div className="content-wrap"> | |
1004 | + <DetailTitle contentTitle={"대상자의 문진표 / 심전도 / 혈압 관리를 할 수 있습니다."} /> | |
1005 | + | |
1006 | + <div style={{ height: "calc(100% - 61px)" }}> | |
1007 | + <div className="right" style={{ height: "100%", }}> | |
1008 | + <div style={{ height: "100%" }}> | |
1009 | + <div className="tab-container" style={{ marginTop: "5rem" }}> | |
1010 | + {/* {CommonUtil.isEmpty(state.loginUser) == false && state.loginUser['authority'] == 'ROLE_AGENCY' ? | |
1011 | + <div className="flex-end margin-bottom"> | |
1012 | + <div className="flex searchselect" style={{width: 'auto'}}> | |
1013 | + | |
1014 | + <input type="radio" id="my_senior" name="senior" checked={isMySenior} | |
1015 | + onChange={(e) => {e.target.checked ? setIsMySenior(true) : null}}/> | |
1016 | + <label for="my_senior" style={{marginRight: '3rem'}}>나의 대상자 보기</label> | |
1017 | + | |
1018 | + <input type="radio" id="all_senior" name="senior" checked={!isMySenior} | |
1019 | + onChange={(e) => {e.target.checked ? setIsMySenior(false) : null}}/> | |
1020 | + <label for="all_senior" style={{marginRight: '0'}}>전체 대상자 보기</label> | |
1021 | + | |
1022 | + </div> | |
1023 | + </div> | |
1024 | + :null} */} | |
1025 | + <ul className="tab-menu flex-end"> | |
1026 | + {tab.map((item, idx) => { | |
1027 | + return ( | |
1028 | + <li onClick={() => setTabIndex(idx)} className={idx == tabIndex ? 'active' : null}> | |
1029 | + {item.title} | |
1030 | + </li> | |
1031 | + ) | |
1032 | + })} | |
1033 | + </ul> | |
1034 | + <div className="content-wrap userlist"> | |
1035 | + | |
1036 | + <ul className="tab-content"> | |
1037 | + <li> | |
1038 | + {tab[tabIndex].content} | |
1039 | + </li> | |
1040 | + </ul> | |
1041 | + </div> | |
1042 | + </div> | |
1043 | + </div> | |
1044 | + </div> | |
1045 | + </div> | |
1046 | + </div> | |
1047 | + | |
1048 | + | |
1049 | + <Modal open={modalOpen} close={closeModal} header="문진표 작성"> | |
1050 | + <div className="board-wrap"> | |
1051 | + <div> | |
1052 | + <table className="margin-bottom2 questionnaire-table"> | |
1053 | + <tr> | |
1054 | + <th>흡연을 하십니까?</th> | |
1055 | + <td className="flex-start"> | |
1056 | + <input type="radio" name="smoke" id="smoke-true" | |
1057 | + checked={questionnaire['smoke_type'] == true} | |
1058 | + onChange={(e) => { | |
1059 | + if (e.target.checked) { | |
1060 | + questionnaire['smoke_type'] = true; | |
1061 | + setQuestionnaire({ ...questionnaire }); | |
1062 | + } | |
1063 | + } | |
1064 | + } | |
1065 | + /> | |
1066 | + <label for="smoke-true">예</label> | |
1067 | + <input type="radio" name="smoke" id="smoke-false" | |
1068 | + checked={questionnaire['smoke_type'] == false} | |
1069 | + onChange={(e) => { | |
1070 | + if (e.target.checked) { | |
1071 | + questionnaire['smoke_type'] = false; | |
1072 | + setQuestionnaire({ ...questionnaire }); | |
1073 | + } | |
1074 | + } | |
1075 | + } | |
1076 | + /> | |
1077 | + <label for="smoke-false">아니요</label> | |
1078 | + </td> | |
1079 | + </tr> | |
1080 | + <tr> | |
1081 | + <th>음주를 하십니까?</th> | |
1082 | + <td className="flex-start"> | |
1083 | + <input type="radio" name="drink" id="drink-true" | |
1084 | + checked={questionnaire['drink_type'] == true} | |
1085 | + onChange={(e) => { | |
1086 | + if (e.target.checked) { | |
1087 | + questionnaire['drink_type'] = true; | |
1088 | + setQuestionnaire({ ...questionnaire }); | |
1089 | + } | |
1090 | + } | |
1091 | + } | |
1092 | + /> | |
1093 | + <label for="drink-true">예</label> | |
1094 | + <input type="radio" name="drink" id="drink-false" | |
1095 | + checked={questionnaire['drink_type'] == false} | |
1096 | + onChange={(e) => { | |
1097 | + if (e.target.checked) { | |
1098 | + questionnaire['drink_type'] = false; | |
1099 | + setQuestionnaire({ ...questionnaire }); | |
1100 | + } | |
1101 | + } | |
1102 | + } | |
1103 | + /> | |
1104 | + <label for="drink-false">아니요</label> | |
1105 | + </td> | |
1106 | + </tr> | |
1107 | + <tr> | |
1108 | + <th>일주일에 운동을 몇회 하십니까?</th> | |
1109 | + <td className="flex-start"> | |
1110 | + <input type="radio" name="exercise" id="exercise-false" | |
1111 | + checked={questionnaire['exercise_type'] == false} | |
1112 | + onChange={(e) => { | |
1113 | + if (e.target.checked) { | |
1114 | + questionnaire['exercise_type'] = false; | |
1115 | + setQuestionnaire({ ...questionnaire }); | |
1116 | + } | |
1117 | + } | |
1118 | + } | |
1119 | + /> | |
1120 | + <label for="exercise-false">안함</label> | |
1121 | + | |
1122 | + <input type="radio" name="exercise" id="exercise-1" | |
1123 | + checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 1} | |
1124 | + onChange={(e) => { | |
1125 | + if (e.target.checked) { | |
1126 | + questionnaire['exercise_type'] = true; | |
1127 | + questionnaire['weekly_exercise_amount'] = 1; | |
1128 | + setQuestionnaire({ ...questionnaire }); | |
1129 | + } | |
1130 | + } | |
1131 | + } | |
1132 | + /> | |
1133 | + <label for="exercise-1">1회</label> | |
1134 | + | |
1135 | + <input type="radio" name="exercise" id="exercise-2" | |
1136 | + checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 2} | |
1137 | + onChange={(e) => { | |
1138 | + if (e.target.checked) { | |
1139 | + questionnaire['exercise_type'] = true; | |
1140 | + questionnaire['weekly_exercise_amount'] = 2; | |
1141 | + setQuestionnaire({ ...questionnaire }); | |
1142 | + } | |
1143 | + } | |
1144 | + } | |
1145 | + /> | |
1146 | + <label for="exercise-2">2회</label> | |
1147 | + | |
1148 | + <input type="radio" name="exercise" id="exercise-3" | |
1149 | + checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 3} | |
1150 | + onChange={(e) => { | |
1151 | + if (e.target.checked) { | |
1152 | + questionnaire['exercise_type'] = true; | |
1153 | + questionnaire['weekly_exercise_amount'] = 3; | |
1154 | + setQuestionnaire({ ...questionnaire }); | |
1155 | + } | |
1156 | + } | |
1157 | + } | |
1158 | + /> | |
1159 | + <label for="exercise-3">3회</label> | |
1160 | + | |
1161 | + <input type="radio" name="exercise" id="exercise-4" | |
1162 | + checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 4} | |
1163 | + onChange={(e) => { | |
1164 | + if (e.target.checked) { | |
1165 | + questionnaire['exercise_type'] = true; | |
1166 | + questionnaire['weekly_exercise_amount'] = 4; | |
1167 | + setQuestionnaire({ ...questionnaire }); | |
1168 | + } | |
1169 | + } | |
1170 | + } | |
1171 | + /> | |
1172 | + <label for="exercise-4">4회</label> | |
1173 | + | |
1174 | + <input type="radio" name="exercise" id="exercise-5" | |
1175 | + checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 5} | |
1176 | + onChange={(e) => { | |
1177 | + if (e.target.checked) { | |
1178 | + questionnaire['exercise_type'] = true; | |
1179 | + questionnaire['weekly_exercise_amount'] = 5; | |
1180 | + setQuestionnaire({ ...questionnaire }); | |
1181 | + } | |
1182 | + } | |
1183 | + } | |
1184 | + /> | |
1185 | + <label for="exercise-5">5회</label> | |
1186 | + | |
1187 | + <input type="radio" name="exercise" id="exercise-6" | |
1188 | + checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 6} | |
1189 | + onChange={(e) => { | |
1190 | + if (e.target.checked) { | |
1191 | + questionnaire['exercise_type'] = true; | |
1192 | + questionnaire['weekly_exercise_amount'] = 6; | |
1193 | + setQuestionnaire({ ...questionnaire }); | |
1194 | + } | |
1195 | + } | |
1196 | + } | |
1197 | + /> | |
1198 | + <label for="exercise-6">6회</label> | |
1199 | + | |
1200 | + <input type="radio" name="exercise" id="exercise-7" | |
1201 | + checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 7} | |
1202 | + onChange={(e) => { | |
1203 | + if (e.target.checked) { | |
1204 | + questionnaire['exercise_type'] = true; | |
1205 | + questionnaire['weekly_exercise_amount'] = 7; | |
1206 | + setQuestionnaire({ ...questionnaire }); | |
1207 | + } | |
1208 | + } | |
1209 | + } | |
1210 | + /> | |
1211 | + <label for="exercise-7">매일</label> | |
1212 | + </td> | |
1213 | + </tr> | |
1214 | + <tr> | |
1215 | + <th>최근 3개월 동안 갑작스런 체중 변화가 있었습니까?</th> | |
1216 | + <td className="flex-start"> | |
1217 | + <input type="radio" name="weight" id="weight-up" | |
1218 | + checked={questionnaire['weight_change_amount'] == 1} | |
1219 | + onChange={(e) => { | |
1220 | + if (e.target.checked) { | |
1221 | + questionnaire['weight_change_amount'] = 1; | |
1222 | + setQuestionnaire({ ...questionnaire }); | |
1223 | + } | |
1224 | + } | |
1225 | + } | |
1226 | + /> | |
1227 | + <label for="weight-up">예 - 증가</label> | |
1228 | + | |
1229 | + <input type="radio" name="weight" id="weight-down" | |
1230 | + checked={questionnaire['weight_change_amount'] == -1} | |
1231 | + onChange={(e) => { | |
1232 | + if (e.target.checked) { | |
1233 | + questionnaire['weight_change_amount'] = -1; | |
1234 | + setQuestionnaire({ ...questionnaire }); | |
1235 | + } | |
1236 | + } | |
1237 | + } | |
1238 | + /> | |
1239 | + <label for="weight-down">예 - 감소</label> | |
1240 | + | |
1241 | + <input type="radio" name="weight" id="weight-false" | |
1242 | + checked={questionnaire['weight_change_amount'] == 0} | |
1243 | + onChange={(e) => { | |
1244 | + if (e.target.checked) { | |
1245 | + questionnaire['weight_change_amount'] = 0; | |
1246 | + setQuestionnaire({ ...questionnaire }); | |
1247 | + } | |
1248 | + } | |
1249 | + } | |
1250 | + /> | |
1251 | + <label for="weight-false">아니요</label> | |
1252 | + </td> | |
1253 | + </tr> | |
1254 | + <tr> | |
1255 | + <th>현재 복용중인 약이 있으면 체크를 해주세요.</th> | |
1256 | + <td className="flex-start"> | |
1257 | + | |
1258 | + <input type="checkbox" name="pills" id="pills-1" | |
1259 | + checked={questionnaire['medication_pill'].indexOf('아스피린(항혈소판제)') > -1} | |
1260 | + onChange={(e) => { | |
1261 | + if (e.target.checked) { | |
1262 | + questionnaire['medication_pill'] += '아스피린(항혈소판제),'; | |
1263 | + setQuestionnaire({ ...questionnaire }); | |
1264 | + } else { | |
1265 | + questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('아스피린(항혈소판제),', ''); | |
1266 | + setQuestionnaire({ ...questionnaire }); | |
1267 | + } | |
1268 | + } | |
1269 | + } | |
1270 | + /> | |
1271 | + <label for="pills-1">아스피린(항혈소판제)</label> | |
1272 | + | |
1273 | + <input type="checkbox" name="pills" id="pills-2" | |
1274 | + checked={questionnaire['medication_pill'].indexOf('당뇨약') > -1} | |
1275 | + onChange={(e) => { | |
1276 | + if (e.target.checked) { | |
1277 | + questionnaire['medication_pill'] += '당뇨약,'; | |
1278 | + setQuestionnaire({ ...questionnaire }); | |
1279 | + } else { | |
1280 | + questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('당뇨약,', ''); | |
1281 | + setQuestionnaire({ ...questionnaire }); | |
1282 | + } | |
1283 | + } | |
1284 | + } | |
1285 | + /> | |
1286 | + <label for="pills-2">당뇨약</label> | |
1287 | + | |
1288 | + <input type="checkbox" name="pills" id="pills-3" | |
1289 | + checked={questionnaire['medication_pill'].indexOf('고혈압약') > -1} | |
1290 | + onChange={(e) => { | |
1291 | + if (e.target.checked) { | |
1292 | + questionnaire['medication_pill'] += '고혈압약,'; | |
1293 | + setQuestionnaire({ ...questionnaire }); | |
1294 | + } else { | |
1295 | + questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('고혈압약,', ''); | |
1296 | + setQuestionnaire({ ...questionnaire }); | |
1297 | + } | |
1298 | + } | |
1299 | + } | |
1300 | + /> | |
1301 | + <label for="pills-3">고혈압약</label> | |
1302 | + | |
1303 | + <input type="checkbox" name="pills" id="pills-etc" | |
1304 | + checked={questionnaire['medication_pill'].indexOf('기타「」,') > -1} | |
1305 | + onChange={(e) => { | |
1306 | + if (e.target.checked) { | |
1307 | + questionnaire['medication_pill'] += '기타「」,'; | |
1308 | + setQuestionnaire({ ...questionnaire }); | |
1309 | + } else { | |
1310 | + questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('기타「」,', ''); | |
1311 | + questionnaire['medication_pill_etc'] = ''; | |
1312 | + setQuestionnaire({ ...questionnaire }); | |
1313 | + } | |
1314 | + } | |
1315 | + } | |
1316 | + /> | |
1317 | + <label for="pills-etc">기타</label> | |
1318 | + <input type="text" style={{ width: 'max-content', marginLeft: '1rem' }} | |
1319 | + disabled={questionnaire['medication_pill'].indexOf('기타「」,') == -1} | |
1320 | + value={questionnaire['medication_pill_etc']} | |
1321 | + onChange={(e) => { | |
1322 | + questionnaire['medication_pill_etc'] = e.target.value; | |
1323 | + setQuestionnaire({ ...questionnaire }); | |
1324 | + }} | |
1325 | + /> | |
1326 | + | |
1327 | + </td> | |
1328 | + </tr> | |
1329 | + </table> | |
1330 | + </div> | |
1331 | + <div className="flex-center"> | |
1332 | + {CommonUtil.isEmpty(questionnaire['questionnaire_record_idx']) | |
1333 | + ? <button className="btn-small red-btn" onClick={questionnaireInsert}>저장</button> | |
1334 | + : <button className="btn-small red-btn" onClick={questionnaireUpdate}>저장</button> | |
1335 | + } | |
1336 | + </div> | |
1337 | + </div> | |
1338 | + </Modal> | |
1339 | + | |
1340 | + | |
1341 | + <Modal open={modalOpen2} close={closeModal2} header="내원기록 관리 및 조회"> | |
1342 | + <div className="board-wrap"> | |
1343 | + <table className="margin-bottom2 senior-insert "> | |
1344 | + <tr> | |
1345 | + <th>진료 일자</th> | |
1346 | + <td> | |
1347 | + <input type="date" | |
1348 | + value={hospitalMedicalRecord['medical_date']} | |
1349 | + onChange={(e) => { | |
1350 | + hospitalMedicalRecord['medical_date'] = e.target.value; | |
1351 | + setHospitalMedicalRecord({ ...hospitalMedicalRecord }); | |
1352 | + }} | |
1353 | + ref={el => hospitalMedicalRecordRef.current['medical_date'] = el} | |
1354 | + /> | |
1355 | + </td> | |
1356 | + </tr> | |
1357 | + <tr> | |
1358 | + <th>진료 사유</th> | |
1359 | + <td className="flex-start "> | |
1360 | + <input type="text" | |
1361 | + value={hospitalMedicalRecord['medical_reason']} | |
1362 | + onChange={(e) => { | |
1363 | + hospitalMedicalRecord['medical_reason'] = e.target.value; | |
1364 | + setHospitalMedicalRecord({ ...hospitalMedicalRecord }); | |
1365 | + }} | |
1366 | + ref={el => hospitalMedicalRecordRef.current['medical_reason'] = el} | |
1367 | + /> | |
1368 | + </td> | |
1369 | + </tr> | |
1370 | + <tr> | |
1371 | + <th>진료 내용</th> | |
1372 | + <td colSpan={3}> | |
1373 | + <textarea className="medicine" cols="30" rows="2" | |
1374 | + value={hospitalMedicalRecord['medical_content']} | |
1375 | + onChange={(e) => { | |
1376 | + hospitalMedicalRecord['medical_content'] = e.target.value; | |
1377 | + setHospitalMedicalRecord({ ...hospitalMedicalRecord }); | |
1378 | + }} | |
1379 | + ref={el => hospitalMedicalRecordRef.current['medical_content'] = el} | |
1380 | + ></textarea> | |
1381 | + </td> | |
1382 | + </tr> | |
1383 | + </table> | |
1384 | + <div className="btn-wrap flex-center margin-bottom5"> | |
1385 | + {CommonUtil.isEmpty(hospitalMedicalRecord['medical_record_idx']) | |
1386 | + ? <button className="btn-small red-btn" onClick={hospitalMedicalRecordInsert}>등록</button> | |
1387 | + | |
1388 | + : <> | |
1389 | + <button className="btn-small gray-btn" onClick={setHospitalMedicalRecordInit}>수정취소</button> | |
1390 | + <button className="btn-small red-btn" onClick={hospitalMedicalRecordUpdate}>수정</button> | |
1391 | + <button className="btn-small red-btn" onClick={hospitalMedicalRecordDelete}>삭제</button> | |
1392 | + </> | |
1393 | + } | |
1394 | + </div> | |
1395 | + <div> | |
1396 | + <table className="caregiver-user senior-insert senior-table"> | |
1397 | + <thead> | |
1398 | + <tr> | |
1399 | + <th>No</th> | |
1400 | + <th>진료 일자</th> | |
1401 | + <th>진료 사유</th> | |
1402 | + <th>진료 내용</th> | |
1403 | + <th>기록 작성자</th> | |
1404 | + </tr> | |
1405 | + </thead> | |
1406 | + <tbody> | |
1407 | + {hospitalMedicalRecordList.hospitalMedicalRecordList.map((item, idx) => { | |
1408 | + return ( | |
1409 | + <tr key={idx} onClick={() => { setHospitalMedicalRecord(item) }}> | |
1410 | + <td data-label="No">{hospitalMedicalRecordList.hospitalMedicalRecordListCount - idx - (hospitalMedicalRecordList.search.currentPage - 1) * hospitalMedicalRecordList.search.perPage}</td> | |
1411 | + <td data-label="진료 일자">{item['medical_date']}</td> | |
1412 | + <td data-label="진료 내용">{item['medical_reason']}</td> | |
1413 | + <td data-label="진료 사유">{item['medical_content']}</td> | |
1414 | + <td data-label="기록 작성자">{item['insert_user_name']}</td> | |
1415 | + </tr> | |
1416 | + ) | |
1417 | + })} | |
1418 | + {CommonUtil.isEmpty(hospitalMedicalRecordList.hospitalMedicalRecordList) ? | |
1419 | + <tr> | |
1420 | + <td colSpan={5}>조회된 데이터가 없습니다</td> | |
1421 | + </tr> | |
1422 | + : null} | |
1423 | + </tbody> | |
1424 | + </table> | |
1425 | + <Pagination | |
1426 | + currentPage={hospitalMedicalRecordList.search.currentPage} | |
1427 | + perPage={hospitalMedicalRecordList.search.perPage} | |
1428 | + totalCount={hospitalMedicalRecordList.hospitalMedicalRecordListCount} | |
1429 | + maxRange={5} | |
1430 | + click={hospitalMedicalRecordSelectList} | |
1431 | + /> | |
1432 | + </div> | |
1433 | + </div> | |
1434 | + </Modal> | |
1435 | + | |
1436 | + | |
1437 | + <Modal open={modalOpen3} close={closeModal3} header="심전도판독소견 관리 및 조회"> | |
1438 | + <div className="board-wrap"> | |
1439 | + <table className="margin-bottom2 senior-insert "> | |
1440 | + <tr> | |
1441 | + <th>측정 일자</th> | |
1442 | + <td colSpan={5}> | |
1443 | + <input type="date" value={ecg['ecg_reading_date']} | |
1444 | + onChange={(e) => { | |
1445 | + ecg['ecg_reading_date'] = e.target.value; | |
1446 | + setEcg({ ...ecg }); | |
1447 | + }} | |
1448 | + ref={el => ecgRef.current['ecg_reading_date'] = el} | |
1449 | + /> | |
1450 | + </td> | |
1451 | + </tr> | |
1452 | + <tr> | |
1453 | + <th>판독 소견 종류</th> | |
1454 | + <td colSpan={5}> | |
1455 | + <input type="text" value={ecg['ecg_finding_type']} | |
1456 | + onChange={(e) => { | |
1457 | + ecg['ecg_finding_type'] = e.target.value; | |
1458 | + setEcg({ ...ecg }); | |
1459 | + }} | |
1460 | + ref={el => ecgRef.current['ecg_finding_type'] = el} | |
1461 | + /> | |
1462 | + </td> | |
1463 | + </tr> | |
1464 | + <tr> | |
1465 | + <th>판독 소견 내용</th> | |
1466 | + <td colSpan={5}> | |
1467 | + <textarea className="medicine" cols="30" rows="2" | |
1468 | + value={ecg['ecg_finding_content']} | |
1469 | + onChange={(e) => { | |
1470 | + ecg['ecg_finding_content'] = e.target.value; | |
1471 | + setEcg({ ...ecg }); | |
1472 | + }} | |
1473 | + ref={el => ecgRef.current['ecg_finding_content'] = el} | |
1474 | + ></textarea> | |
1475 | + </td> | |
1476 | + </tr> | |
1477 | + {ecg.isEdit == false | |
1478 | + ? <tr> | |
1479 | + <th>측정 파일</th> | |
1480 | + <td colSpan={5}> | |
1481 | + <CommonFile commonFileList={ecg['commonFileList']} multiple={false} accept={'.dat, .ecg'} /> | |
1482 | + </td> | |
1483 | + </tr> | |
1484 | + : <> | |
1485 | + <tr> | |
1486 | + <th>서맥 횟수</th> | |
1487 | + <td> | |
1488 | + <input type="number" value={ecg['bradycardia_count']} | |
1489 | + onChange={(e) => { | |
1490 | + ecg['bradycardia_count'] = e.target.value; | |
1491 | + setEcg({ ...ecg }); | |
1492 | + }} | |
1493 | + ref={el => ecgRef.current['bradycardia_count'] = el} | |
1494 | + /> | |
1495 | + </td> | |
1496 | + <th>빈맥 횟수</th> | |
1497 | + <td> | |
1498 | + <input type="number" value={ecg['tachycardia_count']} | |
1499 | + onChange={(e) => { | |
1500 | + ecg['tachycardia_count'] = e.target.value; | |
1501 | + setEcg({ ...ecg }); | |
1502 | + }} | |
1503 | + ref={el => ecgRef.current['tachycardia_count'] = el} | |
1504 | + /> | |
1505 | + </td> | |
1506 | + <th>이상 심박수</th> | |
1507 | + <td> | |
1508 | + <input type="number" value={ecg['abnormal_heart_rate']} | |
1509 | + onChange={(e) => { | |
1510 | + ecg['abnormal_heart_rate'] = e.target.value; | |
1511 | + setEcg({ ...ecg }); | |
1512 | + }} | |
1513 | + ref={el => ecgRef.current['abnormal_heart_rate'] = el} | |
1514 | + /> | |
1515 | + </td> | |
1516 | + </tr> | |
1517 | + <tr> | |
1518 | + <th>최대 심박수</th> | |
1519 | + <td> | |
1520 | + <input type="number" value={ecg['max_heart_rate']} | |
1521 | + onChange={(e) => { | |
1522 | + ecg['max_heart_rate'] = e.target.value; | |
1523 | + setEcg({ ...ecg }); | |
1524 | + }} | |
1525 | + ref={el => ecgRef.current['max_heart_rate'] = el} | |
1526 | + /> | |
1527 | + </td> | |
1528 | + <th>최소 심박수</th> | |
1529 | + <td> | |
1530 | + <input type="number" value={ecg['min_heart_rate']} | |
1531 | + onChange={(e) => { | |
1532 | + ecg['min_heart_rate'] = e.target.value; | |
1533 | + setEcg({ ...ecg }); | |
1534 | + }} | |
1535 | + ref={el => ecgRef.current['min_heart_rate'] = el} | |
1536 | + /> | |
1537 | + </td> | |
1538 | + <th>평균 심박수</th> | |
1539 | + <td> | |
1540 | + <input type="number" value={ecg['average_heart_rate']} | |
1541 | + onChange={(e) => { | |
1542 | + ecg['average_heart_rate'] = e.target.value; | |
1543 | + setEcg({ ...ecg }); | |
1544 | + }} | |
1545 | + ref={el => ecgRef.current['average_heart_rate'] = el} | |
1546 | + /> | |
1547 | + </td> | |
1548 | + </tr> | |
1549 | + <tr> | |
1550 | + <th>최대 R-R</th> | |
1551 | + <td> | |
1552 | + <input type="number" value={ecg['max_rr']} | |
1553 | + onChange={(e) => { | |
1554 | + ecg['max_rr'] = e.target.value; | |
1555 | + setEcg({ ...ecg }); | |
1556 | + }} | |
1557 | + ref={el => ecgRef.current['max_rr'] = el} | |
1558 | + /> | |
1559 | + </td> | |
1560 | + <th>최소 R-R</th> | |
1561 | + <td> | |
1562 | + <input type="number" value={ecg['min_rr']} | |
1563 | + onChange={(e) => { | |
1564 | + ecg['min_rr'] = e.target.value; | |
1565 | + setEcg({ ...ecg }); | |
1566 | + }} | |
1567 | + ref={el => ecgRef.current['min_rr'] = el} | |
1568 | + /> | |
1569 | + </td> | |
1570 | + <th>평균 R-R</th> | |
1571 | + <td> | |
1572 | + <input type="number" value={ecg['average_rr']} | |
1573 | + onChange={(e) => { | |
1574 | + ecg['average_rr'] = e.target.value; | |
1575 | + setEcg({ ...ecg }); | |
1576 | + }} | |
1577 | + ref={el => ecgRef.current['average_rr'] = el} | |
1578 | + /> | |
1579 | + </td> | |
1580 | + </tr> | |
1581 | + </>} | |
1582 | + </table> | |
1583 | + <div className="btn-wrap flex-center margin-bottom5"> | |
1584 | + {CommonUtil.isEmpty(ecg['ecg_record_idx']) | |
1585 | + ? <button className="btn-small red-btn" onClick={ecgInsert}>등록</button> | |
1586 | + : <> | |
1587 | + <button className="btn-small gray-btn" onClick={setEcgInit}>수정취소</button> | |
1588 | + <button className="btn-small red-btn" onClick={ecgUpdate}>수정</button> | |
1589 | + <button className="btn-small red-btn" onClick={ecgDelete}>삭제</button> | |
1590 | + </> | |
1591 | + } | |
1592 | + </div> | |
1593 | + <div> | |
1594 | + <table className="caregiver-user senior-table"> | |
1595 | + <thead> | |
1596 | + <tr> | |
1597 | + <th>No</th> | |
1598 | + <th>소견 작성 일자</th> | |
1599 | + <th>판독 소견 종류</th> | |
1600 | + <th>소견 작성자</th> | |
1601 | + </tr> | |
1602 | + </thead> | |
1603 | + <tbody> | |
1604 | + {ecgList.ecgList.map((item, idx) => { | |
1605 | + return ( | |
1606 | + <tr key={idx} onClick={() => { item.isEdit = false; setEcg(item); }}> | |
1607 | + <td data-label="No">{ecgList.ecgListCount - idx - (ecgList.search.currentPage - 1) * ecgList.search.perPage}</td> | |
1608 | + <td data-label="소견 작성 일자">{item['ecg_reading_date']}</td> | |
1609 | + <td data-label="판독 소견 종류">{item['ecg_finding_type']}</td> | |
1610 | + <td data-label="소견 작성자">{item['insert_user_name']}</td> | |
1611 | + </tr> | |
1612 | + ) | |
1613 | + })} | |
1614 | + {CommonUtil.isEmpty(ecgList.ecgList) ? | |
1615 | + <tr> | |
1616 | + <td colSpan={4}>조회된 데이터가 없습니다</td> | |
1617 | + </tr> | |
1618 | + : null} | |
1619 | + </tbody> | |
1620 | + </table> | |
1621 | + <Pagination | |
1622 | + currentPage={ecgList.search.currentPage} | |
1623 | + perPage={ecgList.search.perPage} | |
1624 | + totalCount={ecgList.ecgListCount} | |
1625 | + maxRange={5} | |
1626 | + click={ecgSelectList} | |
1627 | + /> | |
1628 | + </div> | |
1629 | + </div> | |
1630 | + </Modal> | |
1631 | + | |
1632 | + | |
1633 | + <Modal open={modalOpen4} close={closeModal4} header="혈압측정결과 관리 및 조회"> | |
1634 | + <div className="board-wrap"> | |
1635 | + <table className="margin-bottom2 senior-insert "> | |
1636 | + <tr> | |
1637 | + <th>측정일자</th> | |
1638 | + <td> | |
1639 | + <input type="date" value={bloodPressure['blood_pressure_record_date']} | |
1640 | + onChange={(e) => { | |
1641 | + bloodPressure['blood_pressure_record_date'] = e.target.value; | |
1642 | + setBloodPressure({ ...bloodPressure }); | |
1643 | + }} | |
1644 | + ref={el => bloodPressureRef.current['blood_pressure_record_date'] = el} | |
1645 | + /> | |
1646 | + </td> | |
1647 | + </tr> | |
1648 | + <tr> | |
1649 | + <th>최고혈압</th> | |
1650 | + <td> | |
1651 | + <input type="number" value={bloodPressure['max_blood_pressure']} | |
1652 | + onChange={(e) => { | |
1653 | + bloodPressure['max_blood_pressure'] = e.target.value; | |
1654 | + setBloodPressure({ ...bloodPressure }); | |
1655 | + }} | |
1656 | + ref={el => bloodPressureRef.current['max_blood_pressure'] = el} | |
1657 | + /> | |
1658 | + </td> | |
1659 | + </tr> | |
1660 | + <tr> | |
1661 | + <th>최저혈압</th> | |
1662 | + <td> | |
1663 | + <input type="number" value={bloodPressure['min_blood_pressure']} | |
1664 | + onChange={(e) => { | |
1665 | + bloodPressure['min_blood_pressure'] = e.target.value; | |
1666 | + setBloodPressure({ ...bloodPressure }); | |
1667 | + }} | |
1668 | + ref={el => bloodPressureRef.current['min_blood_pressure'] = el} | |
1669 | + /> | |
1670 | + </td> | |
1671 | + </tr> | |
1672 | + <tr> | |
1673 | + <th>맥박수</th> | |
1674 | + <td> | |
1675 | + <input type="number" value={bloodPressure['pulse_rate']} | |
1676 | + onChange={(e) => { | |
1677 | + bloodPressure['pulse_rate'] = e.target.value; | |
1678 | + setBloodPressure({ ...bloodPressure }); | |
1679 | + }} | |
1680 | + ref={el => bloodPressureRef.current['pulse_rate'] = el} | |
1681 | + /> | |
1682 | + </td> | |
1683 | + </tr> | |
1684 | + </table> | |
1685 | + <div className="btn-wrap flex-center margin-bottom5"> | |
1686 | + {CommonUtil.isEmpty(bloodPressure['blood_pressure_record_idx']) | |
1687 | + ? <button className="btn-small red-btn" onClick={bloodPressureInsert}>등록</button> | |
1688 | + : <> | |
1689 | + <button className="btn-small gray-btn" onClick={setBloodPressureInit}>수정취소</button> | |
1690 | + <button className="btn-small red-btn" onClick={bloodPressureUpdate}>수정</button> | |
1691 | + <button className="btn-small red-btn" onClick={bloodPressureDelete}>삭제</button> | |
1692 | + </> | |
1693 | + } | |
1694 | + </div> | |
1695 | + <div> | |
1696 | + <table className="caregiver-user senior-insert senior-table"> | |
1697 | + <thead> | |
1698 | + <tr> | |
1699 | + <th>No</th> | |
1700 | + <th>기록 작성일</th> | |
1701 | + <th>최고 혈압</th> | |
1702 | + <th>최저 혈압</th> | |
1703 | + <th>맥박수</th> | |
1704 | + <th>기록 작성자</th> | |
1705 | + </tr> | |
1706 | + </thead> | |
1707 | + <tbody> | |
1708 | + {bloodPressureList.bloodPressureList.map((item, idx) => { | |
1709 | + return ( | |
1710 | + <tr key={idx} onClick={() => { setBloodPressure(item); }}> | |
1711 | + <td data-label="No">{bloodPressureList.bloodPressureListCount - idx - (bloodPressureList.search.currentPage - 1) * bloodPressureList.search.perPage}</td> | |
1712 | + <td data-label="기록 작성일">{item['blood_pressure_record_date']}</td> | |
1713 | + <td data-label="최고 혈압">{item['max_blood_pressure']}</td> | |
1714 | + <td data-label="최저 혈압">{item['min_blood_pressure']}</td> | |
1715 | + <td data-label="맥박수">{item['pulse_rate']}</td> | |
1716 | + <td data-label="기록 작성자">{item['insert_user_name']}</td> | |
1717 | + </tr> | |
1718 | + ) | |
1719 | + })} | |
1720 | + {CommonUtil.isEmpty(bloodPressureList.bloodPressureList) ? | |
1721 | + <tr> | |
1722 | + <td colSpan={6}>조회된 데이터가 없습니다</td> | |
1723 | + </tr> | |
1724 | + : null} | |
1725 | + </tbody> | |
1726 | + </table> | |
1727 | + <Pagination | |
1728 | + currentPage={bloodPressureList.search.currentPage} | |
1729 | + perPage={bloodPressureList.search.perPage} | |
1730 | + totalCount={bloodPressureList.bloodPressureListCount} | |
1731 | + maxRange={5} | |
1732 | + click={bloodPressureSelectList} | |
1733 | + /> | |
1734 | + </div> | |
1735 | + </div> | |
1736 | + </Modal> | |
1737 | + | |
1738 | + </main> | |
1739 | + ); | |
1740 | +} |
--- client/views/pages/healthcare/TeamMedicalcare.jsx
... | ... | @@ -1,1705 +0,0 @@ |
1 | -import React from "react"; | |
2 | -import { useNavigate, useLocation } from "react-router"; | |
3 | -import { useSelector } from "react-redux"; | |
4 | - | |
5 | - | |
6 | -import SubTitle from "../../component/SubTitle.jsx"; | |
7 | -import Modal from "../../component/Modal.jsx"; | |
8 | -import DetailTitle from "../../component/DetailTitle.jsx"; | |
9 | -import Pagination from "../../component/Pagination.jsx"; | |
10 | -import CommonFile from "../../component/file/CommonFile.jsx"; | |
11 | - | |
12 | -import CommonUtil from "../../../resources/js/CommonUtil.js"; | |
13 | - | |
14 | - | |
15 | -export default function UserAuthoriySelect() { | |
16 | - const navigate = useNavigate(); | |
17 | - const location = useLocation(); | |
18 | - | |
19 | - //전역 변수 저장 객체 | |
20 | - const state = useSelector((state) => {return state}); | |
21 | - const defaultUserId = CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['user_id']; | |
22 | - | |
23 | - //검색(엔터) | |
24 | - const searchingEnter = (key) => { | |
25 | - if (key == 'Enter') { | |
26 | - searching(); | |
27 | - } else { | |
28 | - return; | |
29 | - } | |
30 | - } | |
31 | - //검색 | |
32 | - const searching = () => { | |
33 | - if (CommonUtil.isEmpty(state.loginUser) == false | |
34 | - && state.loginUser['authority'] == 'ROLE_AGENCY' && isMySenior) { | |
35 | - senior.search['agent_id'] = state.loginUser['user_id']; | |
36 | - } else { | |
37 | - senior.search['agent_id'] = null; | |
38 | - } | |
39 | - | |
40 | - setSenior({...senior}); | |
41 | - | |
42 | - seniorSelectList(1); | |
43 | - } | |
44 | - | |
45 | - const [isMySenior, setIsMySenior] = React.useState(true); | |
46 | - React.useEffect(() => { | |
47 | - searching(); | |
48 | - }, [isMySenior]) | |
49 | - //보호사(간호사)의 돌봄 대상자(시니어) | |
50 | - const [senior, setSenior] = React.useState({seniorList: [], seniorListCount: 0, search: { | |
51 | - 'government_id': CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['government_id'], | |
52 | - 'agency_id': CommonUtil.isEmpty(state.loginUser) ? null : state.loginUser['agency_id'], | |
53 | - 'searchType': null, | |
54 | - 'searchText': null, | |
55 | - 'currentPage': 1, | |
56 | - 'perPage': 10, | |
57 | - }}); | |
58 | - //보호사(간호사)의 돌봄 대상자(시니어) 목록 조회 | |
59 | - const seniorSelectList = (currentPage) => { | |
60 | - senior.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; | |
61 | - | |
62 | - fetch("/user/seniorSelectList.json", { | |
63 | - method: "POST", | |
64 | - headers: { | |
65 | - 'Content-Type': 'application/json; charset=UTF-8' | |
66 | - }, | |
67 | - body: JSON.stringify(senior.search), | |
68 | - }).then((response) => response.json()).then((data) => { | |
69 | - data.search = senior.search; | |
70 | - console.log("돌봄 대상자(시니어) 목록 조회 : ", data); | |
71 | - setSenior(data); | |
72 | - }).catch((error) => { | |
73 | - console.log('seniorSelectList() /user/seniorSelectList.json error : ', error); | |
74 | - }); | |
75 | - } | |
76 | - | |
77 | - | |
78 | - //현재 선택한 대상자 정보 | |
79 | - const [targetSenior, setTargetSenior] = React.useState({'senior_id': null}); | |
80 | - | |
81 | - //문진표 | |
82 | - const [modalOpen, setModalOpen] = React.useState(false); | |
83 | - const openModal = (senior) => { | |
84 | - targetSenior['senior_id'] = senior['senior_id']; | |
85 | - setTargetSenior(senior); | |
86 | - questionnaireSelectList(); | |
87 | - | |
88 | - setModalOpen(true); | |
89 | - }; | |
90 | - const closeModal = () => { | |
91 | - setTargetSenior({'senior_id': null}); | |
92 | - setModalOpen(false); | |
93 | - }; | |
94 | - //내원기록 | |
95 | - const [modalOpen2, setModalOpen2] = React.useState(false); | |
96 | - const openModal2 = (senior) => { | |
97 | - targetSenior['senior_id'] = senior['senior_id']; | |
98 | - setTargetSenior(senior); | |
99 | - hospitalMedicalRecordSelectList(); | |
100 | - | |
101 | - setModalOpen2(true); | |
102 | - }; | |
103 | - const closeModal2 = () => { | |
104 | - setTargetSenior({'senior_id': null}); | |
105 | - setModalOpen2(false); | |
106 | - }; | |
107 | - //심전도 | |
108 | - const [modalOpen3, setModalOpen3] = React.useState(false); | |
109 | - const openModal3 = (senior) => { | |
110 | - targetSenior['senior_id'] = senior['senior_id']; | |
111 | - setTargetSenior(senior); | |
112 | - ecgSelectList(); | |
113 | - | |
114 | - setModalOpen3(true); | |
115 | - }; | |
116 | - const closeModal3 = () => { | |
117 | - setTargetSenior({'senior_id': null}); | |
118 | - setModalOpen3(false); | |
119 | - }; | |
120 | - //혈압 | |
121 | - const [modalOpen4, setModalOpen4] = React.useState(false); | |
122 | - const openModal4 = (senior) => { | |
123 | - targetSenior['senior_id'] = senior['senior_id']; | |
124 | - setTargetSenior(senior); | |
125 | - bloodPressureSelectList(); | |
126 | - | |
127 | - setModalOpen4(true); | |
128 | - }; | |
129 | - const closeModal4 = () => { | |
130 | - setTargetSenior({'senior_id': null}); | |
131 | - setModalOpen4(false); | |
132 | - }; | |
133 | - | |
134 | - /****************** 문진표 (시작) ******************/ | |
135 | - const questionnaireInit = { | |
136 | - 'senior_id': null, | |
137 | - 'questionnaire_record_idx': null, | |
138 | - 'weight_change_amount': 0, | |
139 | - 'past_history': null, | |
140 | - 'agent_id': defaultUserId, | |
141 | - 'medical_record_idx': null, | |
142 | - | |
143 | - 'smoke_type': false, | |
144 | - 'no_smoke_period': null, | |
145 | - 'smoke_period': null, | |
146 | - 'daily_smoke_amount': null, | |
147 | - | |
148 | - 'drink_type': false, | |
149 | - 'weekly_drink_amount': null, | |
150 | - 'onetime_alcoholic_kind': null, | |
151 | - 'onetime_bottle_amount': null, | |
152 | - 'onetime_cup_amount': null, | |
153 | - | |
154 | - 'exercise_type': false, | |
155 | - 'weekly_exercise_amount': null, | |
156 | - 'onetime_exercise_hour': null, | |
157 | - 'onetime_exercise_minute': null, | |
158 | - 'onetime_exercise_kind': null, | |
159 | - | |
160 | - 'medication_pill': '', | |
161 | - 'medication_pill_etc': '', | |
162 | - }; | |
163 | - //문진표 정보 | |
164 | - const [questionnaire, setQuestionnaire] = React.useState({...questionnaireInit}); | |
165 | - const questionnaireRef = React.useRef({...questionnaireInit}); | |
166 | - | |
167 | - //문진표 등록 | |
168 | - const questionnaireInsert = () => { | |
169 | - questionnaire['senior_id'] = targetSenior['senior_id']; | |
170 | - questionnaire['agent_id'] = defaultUserId; | |
171 | - setQuestionnaire({...questionnaire}); | |
172 | - | |
173 | - fetch("/hospital/questionnaireInsert.json", { | |
174 | - method: "POST", | |
175 | - headers: { | |
176 | - 'Content-Type': 'application/json; charset=UTF-8' | |
177 | - }, | |
178 | - body: JSON.stringify(questionnaire), | |
179 | - }).then((response) => response.json()).then((data) => { | |
180 | - console.log("문진표 등록 결과(건수) : ", data); | |
181 | - if (data > 0) { | |
182 | - alert("저장완료"); | |
183 | - closeModal(); | |
184 | - } else { | |
185 | - alert("저장에 실패하였습니다. 관리자에게 문의바랍니다."); | |
186 | - } | |
187 | - }).catch((error) => { | |
188 | - console.log('questionnaireInsert() /hospital/questionnaireInsert.json error : ', error); | |
189 | - }); | |
190 | - } | |
191 | - | |
192 | - //문진표 수정 | |
193 | - const questionnaireUpdate = () => { | |
194 | - questionnaire['senior_id'] = targetSenior['senior_id']; | |
195 | - questionnaire['agent_id'] = defaultUserId; | |
196 | - setQuestionnaire({...questionnaire}); | |
197 | - | |
198 | - fetch("/hospital/questionnaireUpdate.json", { | |
199 | - method: "POST", | |
200 | - headers: { | |
201 | - 'Content-Type': 'application/json; charset=UTF-8' | |
202 | - }, | |
203 | - body: JSON.stringify(questionnaire), | |
204 | - }).then((response) => response.json()).then((data) => { | |
205 | - console.log("문진표 수정 결과(건수) : ", data); | |
206 | - if (data > 0) { | |
207 | - alert("저장완료"); | |
208 | - closeModal(); | |
209 | - } else { | |
210 | - alert("저장에 실패하였습니다. 관리자에게 문의바랍니다."); | |
211 | - } | |
212 | - }).catch((error) => { | |
213 | - console.log('questionnaireUpdate() /hospital/questionnaireUpdate.json error : ', error); | |
214 | - }); | |
215 | - } | |
216 | - | |
217 | - //문진표 목록 조회 | |
218 | - const questionnaireSelectList = () => { | |
219 | - fetch("/hospital/questionnaireSelectList.json", { | |
220 | - method: "POST", | |
221 | - headers: { | |
222 | - 'Content-Type': 'application/json; charset=UTF-8' | |
223 | - }, | |
224 | - body: JSON.stringify(targetSenior), | |
225 | - }).then((response) => response.json()).then((data) => { | |
226 | - console.log("문진표 목록 조회 결과(건수) : ", data); | |
227 | - if (CommonUtil.isEmpty(data.questionnaireList) == false) { | |
228 | - let item = data.questionnaireList[0];//최신순으로 가지고오기 때문에, 0 인텍스가 젤 최신 | |
229 | - if (CommonUtil.isEmpty(item['medication_pill'])) { | |
230 | - item['medication_pill'] = ''; | |
231 | - } else { | |
232 | - const startCheckWord = '기타「'; | |
233 | - const endCheckWord = '」'; | |
234 | - if (item['medication_pill'].indexOf(startCheckWord) > -1) { | |
235 | - let startIndex = item['medication_pill'].indexOf(startCheckWord); | |
236 | - let lastIndex = item['medication_pill'].lastIndexOf(endCheckWord); | |
237 | - console.log("000000 item['medication_pill_etc'] : ", item['medication_pill_etc'], "item['medication_pill'] : ", item['medication_pill']); | |
238 | - item['medication_pill_etc'] = item['medication_pill'].substring(startIndex + startCheckWord.length, lastIndex); | |
239 | - let medicationPillFront = item['medication_pill'].substring(0, startIndex + startCheckWord.length); | |
240 | - let medicationPillBack = item['medication_pill'].substring(lastIndex); | |
241 | - item['medication_pill'] = medicationPillFront + medicationPillBack; | |
242 | - console.log("333333 item['medication_pill_etc'] : ", item['medication_pill_etc'], "item['medication_pill'] : ", item['medication_pill']); | |
243 | - } else { | |
244 | - item['medication_pill_etc'] = ''; | |
245 | - } | |
246 | - } | |
247 | - | |
248 | - setQuestionnaire(item); | |
249 | - } | |
250 | - }).catch((error) => { | |
251 | - console.log('questionnaireSelectList() /hospital/questionnaireSelectList.json error : ', error); | |
252 | - }); | |
253 | - } | |
254 | - /****************** 문진표 (종료) ******************/ | |
255 | - | |
256 | - | |
257 | - | |
258 | - /****************** 병원 진료 기록 (시작) ******************/ | |
259 | - const hospitalMedicalRecordInit = { | |
260 | - 'senior_id': null, | |
261 | - 'medical_record_idx': null, | |
262 | - 'medical_date': CommonUtil.getDate(), | |
263 | - 'medical_reason': '', | |
264 | - 'medical_content': '', | |
265 | - 'agent_id': defaultUserId | |
266 | - }; | |
267 | - //병원 진료 기록 정보 | |
268 | - const [hospitalMedicalRecord, setHospitalMedicalRecord] = React.useState({...hospitalMedicalRecordInit}); | |
269 | - const hospitalMedicalRecordRef = React.useRef({...hospitalMedicalRecordInit}); | |
270 | - | |
271 | - //병원 진료 기록 유효성 검사 | |
272 | - const hospitalMedicalRecordValidation = () => { | |
273 | - const target = hospitalMedicalRecord; | |
274 | - const targetRef = hospitalMedicalRecordRef; | |
275 | - | |
276 | - if (CommonUtil.isEmpty(target['medical_date']) == true) { | |
277 | - targetRef.current['medical_date'].focus(); | |
278 | - alert("진료 일자를 선택해 주세요."); | |
279 | - return false; | |
280 | - } | |
281 | - if (CommonUtil.isEmpty(target['medical_reason']) == true) { | |
282 | - targetRef.current['medical_reason'].focus(); | |
283 | - alert("진료 사유를 입력해 주세요."); | |
284 | - return false; | |
285 | - } | |
286 | - if (CommonUtil.isEmpty(target['medical_content']) == true) { | |
287 | - targetRef.current['medical_content'].focus(); | |
288 | - alert("진료 내용을 입력해 주세요."); | |
289 | - return false; | |
290 | - } | |
291 | - | |
292 | - return true; | |
293 | - } | |
294 | - | |
295 | - | |
296 | - //병원 진료 기록 등록 | |
297 | - const hospitalMedicalRecordInsert = () => { | |
298 | - if (hospitalMedicalRecordValidation() == false) { | |
299 | - return; | |
300 | - } | |
301 | - | |
302 | - hospitalMedicalRecord['senior_id'] = targetSenior['senior_id']; | |
303 | - hospitalMedicalRecord['agent_id'] = defaultUserId; | |
304 | - setHospitalMedicalRecord({...hospitalMedicalRecord}); | |
305 | - | |
306 | - fetch("/hospital/hospitalMedicalRecordInsert.json", { | |
307 | - method: "POST", | |
308 | - headers: { | |
309 | - 'Content-Type': 'application/json; charset=UTF-8' | |
310 | - }, | |
311 | - body: JSON.stringify(hospitalMedicalRecord), | |
312 | - }).then((response) => response.json()).then((data) => { | |
313 | - console.log("병원 진료 기록 등록 결과(건수) : ", data); | |
314 | - if (data > 0) { | |
315 | - setHospitalMedicalRecordInit(); | |
316 | - hospitalMedicalRecordSelectList(); | |
317 | - //closeModal2(); | |
318 | - alert("등록완료"); | |
319 | - | |
320 | - } else { | |
321 | - alert("등록에 실패하였습니다. 관리자에게 문의바랍니다."); | |
322 | - } | |
323 | - }).catch((error) => { | |
324 | - console.log('hospitalMedicalRecordInsert() /hospital/hospitalMedicalRecordInsert.json error : ', error); | |
325 | - }); | |
326 | - } | |
327 | - | |
328 | - //병원 진료 기록 수정 | |
329 | - const hospitalMedicalRecordUpdate = () => { | |
330 | - if (hospitalMedicalRecordValidation() == false) { | |
331 | - return; | |
332 | - } | |
333 | - | |
334 | - hospitalMedicalRecord['senior_id'] = targetSenior['senior_id']; | |
335 | - hospitalMedicalRecord['agent_id'] = defaultUserId; | |
336 | - setHospitalMedicalRecord({...hospitalMedicalRecord}); | |
337 | - | |
338 | - fetch("/hospital/hospitalMedicalRecordUpdate.json", { | |
339 | - method: "POST", | |
340 | - headers: { | |
341 | - 'Content-Type': 'application/json; charset=UTF-8' | |
342 | - }, | |
343 | - body: JSON.stringify(hospitalMedicalRecord), | |
344 | - }).then((response) => response.json()).then((data) => { | |
345 | - console.log("병원 진료 기록 수정 결과(건수) : ", data); | |
346 | - if (data > 0) { | |
347 | - setHospitalMedicalRecordInit(); | |
348 | - hospitalMedicalRecordSelectList(); | |
349 | - //closeModal2(); | |
350 | - alert("수정완료"); | |
351 | - } else { | |
352 | - alert("수정에 실패하였습니다. 관리자에게 문의바랍니다."); | |
353 | - } | |
354 | - }).catch((error) => { | |
355 | - console.log('hospitalMedicalRecordUpdate() /hospital/hospitalMedicalRecordUpdate.json error : ', error); | |
356 | - }); | |
357 | - } | |
358 | - | |
359 | - //병원 진료 기록 삭제 | |
360 | - const hospitalMedicalRecordDelete = () => { | |
361 | - if (confirm('진료 기록을 삭제하시겠습니까?') == false) { | |
362 | - return; | |
363 | - } | |
364 | - | |
365 | - fetch("/hospital/hospitalMedicalRecordDelete.json", { | |
366 | - method: "POST", | |
367 | - headers: { | |
368 | - 'Content-Type': 'application/json; charset=UTF-8' | |
369 | - }, | |
370 | - body: JSON.stringify(hospitalMedicalRecord), | |
371 | - }).then((response) => response.json()).then((data) => { | |
372 | - console.log("병원 진료 기록 삭제 결과(건수) : ", data); | |
373 | - if (data > 0) { | |
374 | - setHospitalMedicalRecordInit(); | |
375 | - hospitalMedicalRecordSelectList(); | |
376 | - //closeModal2(); | |
377 | - alert("삭제완료"); | |
378 | - } else { | |
379 | - alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다."); | |
380 | - } | |
381 | - }).catch((error) => { | |
382 | - console.log('hospitalMedicalRecordDelete() /hospital/hospitalMedicalRecordDelete.json error : ', error); | |
383 | - }); | |
384 | - } | |
385 | - | |
386 | - //초기화 취소 | |
387 | - const setHospitalMedicalRecordInit = () => { | |
388 | - setHospitalMedicalRecord({...hospitalMedicalRecordInit}); | |
389 | - } | |
390 | - | |
391 | - //병원 진료 기록 정보 | |
392 | - const [hospitalMedicalRecordList, setHospitalMedicalRecordList] = React.useState({hospitalMedicalRecordList: [], hospitalMedicalRecordListCount:0, search: {currentPage: 1, perPage: 5}}); | |
393 | - //병원 진료 기록 목록 조회 | |
394 | - const hospitalMedicalRecordSelectList = (currentPage) => { | |
395 | - hospitalMedicalRecordList.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; | |
396 | - hospitalMedicalRecordList.search['senior_id'] = targetSenior['senior_id']; | |
397 | - setHospitalMedicalRecordList({...hospitalMedicalRecordList}); | |
398 | - | |
399 | - fetch("/hospital/hospitalMedicalRecordSelectList.json", { | |
400 | - method: "POST", | |
401 | - headers: { | |
402 | - 'Content-Type': 'application/json; charset=UTF-8' | |
403 | - }, | |
404 | - body: JSON.stringify(hospitalMedicalRecordList.search), | |
405 | - }).then((response) => response.json()).then((data) => { | |
406 | - console.log("병원 진료 기록 목록 조회 결과(건수) : ", data); | |
407 | - data.search = hospitalMedicalRecordList.search; | |
408 | - setHospitalMedicalRecordList(data); | |
409 | - }).catch((error) => { | |
410 | - console.log('hospitalMedicalRecordSelectList() /hospital/hospitalMedicalRecordSelectList.json error : ', error); | |
411 | - }); | |
412 | - } | |
413 | - /****************** 병원 진료 기록 (종료) ******************/ | |
414 | - | |
415 | - | |
416 | - | |
417 | - /****************** 심전도 (시작) ******************/ | |
418 | - const ecgInit = { | |
419 | - 'senior_id': null, | |
420 | - 'ecg_record_idx': null, | |
421 | - 'bradycardia_count': 0, | |
422 | - 'tachycardia_count': 0, | |
423 | - 'max_heart_rate': 0.0, | |
424 | - 'min_heart_rate': 0.0, | |
425 | - 'abnormal_heart_rate': 0.0, | |
426 | - 'average_heart_rate': 0.0, | |
427 | - 'max_rr': 0.0, | |
428 | - 'min_rr': 0.0, | |
429 | - 'average_rr': 0.0, | |
430 | - 'ecg_reading_date': CommonUtil.getDate(), | |
431 | - 'ecg_finding_type': '', | |
432 | - 'ecg_finding_content': '', | |
433 | - 'agent_id': defaultUserId, | |
434 | - 'medical_record_idx': null, | |
435 | - 'common_group_file_idx': null, | |
436 | - commonFileList: [],//심전도 업로드 파일 목록 | |
437 | - | |
438 | - isEdit: false,//직접 수정일 때, true (수정 or 등록 상관없음); | |
439 | - }; | |
440 | - //심전도 정보 | |
441 | - const [ecg, setEcg] = React.useState({...ecgInit}); | |
442 | - const ecgRef = React.useRef({...ecgInit}); | |
443 | - | |
444 | - //심전도 유효성 검사 | |
445 | - const ecgValidation = () => { | |
446 | - const target = ecg; | |
447 | - const targetRef = ecgRef; | |
448 | - | |
449 | - if (CommonUtil.isEmpty(target['ecg_reading_date']) == true) { | |
450 | - targetRef.current['ecg_reading_date'].focus(); | |
451 | - alert("판독 소견 측정 일자를 선택해 주세요."); | |
452 | - return false; | |
453 | - } | |
454 | - if (CommonUtil.isEmpty(target['ecg_finding_type']) == true) { | |
455 | - targetRef.current['ecg_finding_type'].focus(); | |
456 | - alert("판독 소견 측정 종류를 입력해 주세요."); | |
457 | - return false; | |
458 | - } | |
459 | - if (CommonUtil.isEmpty(target['ecg_finding_content']) == true) { | |
460 | - targetRef.current['ecg_finding_content'].focus(); | |
461 | - alert("판독 소견 측정 내용을 입력해 주세요."); | |
462 | - return false; | |
463 | - } | |
464 | - //파일 입력 | |
465 | - if (target.isEdit == false) { | |
466 | - if (CommonUtil.isEmpty(target['commonFileList']) == true) { | |
467 | - //targetRef.current['commonFileList'].focus(); | |
468 | - alert("심전도 측정 파일을 올려주세요."); | |
469 | - return false; | |
470 | - } | |
471 | - } else {//직접 입력 | |
472 | - if (CommonUtil.isEmpty(target['bradycardia_count']) == true) { | |
473 | - targetRef.current['bradycardia_count'].focus(); | |
474 | - alert("서맥 횟수를 입력해 주세요."); | |
475 | - return false; | |
476 | - } | |
477 | - if (CommonUtil.isEmpty(target['tachycardia_count']) == true) { | |
478 | - targetRef.current['tachycardia_count'].focus(); | |
479 | - alert("빈맥 횟수 입력해 주세요."); | |
480 | - return false; | |
481 | - } | |
482 | - if (CommonUtil.isEmpty(target['max_heart_rate']) == true) { | |
483 | - targetRef.current['average_heart_rate'].focus(); | |
484 | - alert("최대 심박수를 입력해 주세요."); | |
485 | - return false; | |
486 | - } | |
487 | - if (CommonUtil.isEmpty(target['min_heart_rate']) == true) { | |
488 | - targetRef.current['average_heart_rate'].focus(); | |
489 | - alert("최소 심박수를 입력해 주세요."); | |
490 | - return false; | |
491 | - } | |
492 | - if (CommonUtil.isEmpty(target['average_heart_rate']) == true) { | |
493 | - targetRef.current['average_heart_rate'].focus(); | |
494 | - alert("평균 심박수를 입력해 주세요."); | |
495 | - return false; | |
496 | - } | |
497 | - if (CommonUtil.isEmpty(target['abnormal_heart_rate']) == true) { | |
498 | - targetRef.current['abnormal_heart_rate'].focus(); | |
499 | - alert("이상 심박수를 입력해 주세요."); | |
500 | - return false; | |
501 | - } | |
502 | - if (CommonUtil.isEmpty(target['max_rr']) == true) { | |
503 | - targetRef.current['max_rr'].focus(); | |
504 | - alert("최대 R-R를 입력해 주세요."); | |
505 | - return false; | |
506 | - } | |
507 | - if (CommonUtil.isEmpty(target['min_rr']) == true) { | |
508 | - targetRef.current['min_rr'].focus(); | |
509 | - alert("최소 R-R를 입력해 주세요."); | |
510 | - return false; | |
511 | - } | |
512 | - if (CommonUtil.isEmpty(target['average_rr']) == true) { | |
513 | - targetRef.current['average_rr'].focus(); | |
514 | - alert("평균 R-R를 입력해 주세요."); | |
515 | - return false; | |
516 | - } | |
517 | - } | |
518 | - | |
519 | - return true; | |
520 | - } | |
521 | - | |
522 | - | |
523 | - //심전도 등록 | |
524 | - const ecgInsert = () => { | |
525 | - if (ecgValidation() == false) { | |
526 | - return; | |
527 | - } | |
528 | - | |
529 | - ecg['senior_id'] = targetSenior['senior_id']; | |
530 | - ecg['agent_id'] = defaultUserId; | |
531 | - setEcg({...ecg}); | |
532 | - | |
533 | - fetch("/hospital/ecgInsert.json", { | |
534 | - method: "POST", | |
535 | - headers: { | |
536 | - 'Content-Type': 'application/json; charset=UTF-8' | |
537 | - }, | |
538 | - body: JSON.stringify(ecg), | |
539 | - }).then((response) => response.json()).then((data) => { | |
540 | - console.log("심전도 등록 결과(건수) : ", data); | |
541 | - if (data > 0) { | |
542 | - setEcgInit(); | |
543 | - ecgSelectList(); | |
544 | - //closeModal3(); | |
545 | - alert("등록완료"); | |
546 | - | |
547 | - } else { | |
548 | - alert("등록에 실패하였습니다. 관리자에게 문의바랍니다."); | |
549 | - } | |
550 | - }).catch((error) => { | |
551 | - console.log('ecgInsert() /hospital/ecgInsert.json error : ', error); | |
552 | - }); | |
553 | - } | |
554 | - | |
555 | - //심전도 수정 | |
556 | - const ecgUpdate = () => { | |
557 | - if (ecgValidation() == false) { | |
558 | - return; | |
559 | - } | |
560 | - | |
561 | - ecg['senior_id'] = targetSenior['senior_id']; | |
562 | - ecg['agent_id'] = defaultUserId; | |
563 | - setEcg({...ecg}); | |
564 | - | |
565 | - fetch("/hospital/ecgUpdate.json", { | |
566 | - method: "POST", | |
567 | - headers: { | |
568 | - 'Content-Type': 'application/json; charset=UTF-8' | |
569 | - }, | |
570 | - body: JSON.stringify(ecg), | |
571 | - }).then((response) => response.json()).then((data) => { | |
572 | - console.log("심전도 수정 결과(건수) : ", data); | |
573 | - if (data > 0) { | |
574 | - setEcgInit(); | |
575 | - ecg.commonFileList = []; | |
576 | - setEcg({...ecg}); | |
577 | - ecgSelectList(); | |
578 | - //closeModal3(); | |
579 | - alert("수정완료"); | |
580 | - } else { | |
581 | - alert("수정에 실패하였습니다. 관리자에게 문의바랍니다."); | |
582 | - } | |
583 | - }).catch((error) => { | |
584 | - console.log('ecgUpdate() /hospital/ecgUpdate.json error : ', error); | |
585 | - }); | |
586 | - } | |
587 | - | |
588 | - //심전도 삭제 | |
589 | - const ecgDelete = () => { | |
590 | - if (confirm('심전도 판독 소견을 삭제하시겠습니까?') == false) { | |
591 | - return; | |
592 | - } | |
593 | - | |
594 | - fetch("/hospital/ecgDelete.json", { | |
595 | - method: "POST", | |
596 | - headers: { | |
597 | - 'Content-Type': 'application/json; charset=UTF-8' | |
598 | - }, | |
599 | - body: JSON.stringify(ecg), | |
600 | - }).then((response) => response.json()).then((data) => { | |
601 | - console.log("심전도 삭제 결과(건수) : ", data); | |
602 | - if (data > 0) { | |
603 | - setEcgInit(); | |
604 | - ecg.commonFileList = []; | |
605 | - setEcg({...ecg}); | |
606 | - ecgSelectList(); | |
607 | - //closeModal3(); | |
608 | - alert("삭제완료"); | |
609 | - } else { | |
610 | - alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다."); | |
611 | - } | |
612 | - }).catch((error) => { | |
613 | - console.log('ecgDelete() /hospital/ecgDelete.json error : ', error); | |
614 | - }); | |
615 | - } | |
616 | - | |
617 | - //초기화 취소 | |
618 | - const setEcgInit = () => { | |
619 | - setEcg({...ecgInit}); | |
620 | - } | |
621 | - | |
622 | - //심전도 정보 | |
623 | - const [ecgList, setEcgList] = React.useState({ecgList: [], ecgListCount:0, search: {currentPage: 1, perPage: 5}}); | |
624 | - //심전도 목록 조회 | |
625 | - const ecgSelectList = (currentPage) => { | |
626 | - ecgList.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; | |
627 | - ecgList.search['senior_id'] = targetSenior['senior_id']; | |
628 | - setEcgList({...ecgList}); | |
629 | - | |
630 | - fetch("/hospital/ecgSelectList.json", { | |
631 | - method: "POST", | |
632 | - headers: { | |
633 | - 'Content-Type': 'application/json; charset=UTF-8' | |
634 | - }, | |
635 | - body: JSON.stringify(ecgList.search), | |
636 | - }).then((response) => response.json()).then((data) => { | |
637 | - console.log("심전도 목록 조회 결과(건수) : ", data); | |
638 | - data.search = ecgList.search; | |
639 | - setEcgList(data); | |
640 | - }).catch((error) => { | |
641 | - console.log('ecgSelectList() /hospital/ecgSelectList.json error : ', error); | |
642 | - }); | |
643 | - } | |
644 | - /****************** 심전도 기록 (종료) ******************/ | |
645 | - | |
646 | - | |
647 | - | |
648 | - /****************** 혈압 (시작) ******************/ | |
649 | - const bloodPressureInit = { | |
650 | - 'senior_id': null, | |
651 | - 'blood_pressure_record_idx': null, | |
652 | - 'max_blood_pressure': null, | |
653 | - 'min_blood_pressure': null, | |
654 | - 'pulse_rate': null, | |
655 | - 'medical_record_idx': null, | |
656 | - 'blood_pressure_record_date': CommonUtil.getDate(), | |
657 | - 'agent_id': defaultUserId | |
658 | - }; | |
659 | - //혈압 정보 | |
660 | - const [bloodPressure, setBloodPressure] = React.useState({...bloodPressureInit}); | |
661 | - const bloodPressureRef = React.useRef({...bloodPressureInit}); | |
662 | - | |
663 | - //혈압 유효성 검사 | |
664 | - const bloodPressureValidation = () => { | |
665 | - const target = bloodPressure; | |
666 | - const targetRef = bloodPressureRef; | |
667 | - | |
668 | - if (CommonUtil.isEmpty(target['blood_pressure_record_date']) == true) { | |
669 | - targetRef.current['blood_pressure_record_date'].focus(); | |
670 | - alert("진료 일자를 선택해 주세요."); | |
671 | - return false; | |
672 | - } | |
673 | - if (CommonUtil.isEmpty(target['max_blood_pressure']) == true) { | |
674 | - targetRef.current['max_blood_pressure'].focus(); | |
675 | - alert("최고 혈압을 입력해 주세요."); | |
676 | - return false; | |
677 | - } | |
678 | - if (CommonUtil.isEmpty(target['min_blood_pressure']) == true) { | |
679 | - targetRef.current['min_blood_pressure'].focus(); | |
680 | - alert("최저 혈압을 입력해 주세요."); | |
681 | - return false; | |
682 | - } | |
683 | - if (CommonUtil.isEmpty(target['pulse_rate']) == true) { | |
684 | - targetRef.current['pulse_rate'].focus(); | |
685 | - alert("맥박수를 입력해 주세요."); | |
686 | - return false; | |
687 | - } | |
688 | - | |
689 | - return true; | |
690 | - } | |
691 | - | |
692 | - | |
693 | - //혈압 등록 | |
694 | - const bloodPressureInsert = () => { | |
695 | - if (bloodPressureValidation() == false) { | |
696 | - return; | |
697 | - } | |
698 | - | |
699 | - bloodPressure['senior_id'] = targetSenior['senior_id']; | |
700 | - bloodPressure['agent_id'] = defaultUserId; | |
701 | - setBloodPressure({...bloodPressure}); | |
702 | - | |
703 | - fetch("/hospital/bloodPressureInsert.json", { | |
704 | - method: "POST", | |
705 | - headers: { | |
706 | - 'Content-Type': 'application/json; charset=UTF-8' | |
707 | - }, | |
708 | - body: JSON.stringify(bloodPressure), | |
709 | - }).then((response) => response.json()).then((data) => { | |
710 | - console.log("혈압 등록 결과(건수) : ", data); | |
711 | - if (data > 0) { | |
712 | - setBloodPressureInit(); | |
713 | - bloodPressureSelectList(); | |
714 | - //closeModal2(); | |
715 | - alert("등록완료"); | |
716 | - | |
717 | - } else { | |
718 | - alert("등록에 실패하였습니다. 관리자에게 문의바랍니다."); | |
719 | - } | |
720 | - }).catch((error) => { | |
721 | - console.log('bloodPressureInsert() /hospital/bloodPressureInsert.json error : ', error); | |
722 | - }); | |
723 | - } | |
724 | - | |
725 | - //혈압 수정 | |
726 | - const bloodPressureUpdate = () => { | |
727 | - if (bloodPressureValidation() == false) { | |
728 | - return; | |
729 | - } | |
730 | - | |
731 | - bloodPressure['senior_id'] = targetSenior['senior_id']; | |
732 | - bloodPressure['agent_id'] = defaultUserId; | |
733 | - setBloodPressure({...bloodPressure}); | |
734 | - | |
735 | - fetch("/hospital/bloodPressureUpdate.json", { | |
736 | - method: "POST", | |
737 | - headers: { | |
738 | - 'Content-Type': 'application/json; charset=UTF-8' | |
739 | - }, | |
740 | - body: JSON.stringify(bloodPressure), | |
741 | - }).then((response) => response.json()).then((data) => { | |
742 | - console.log("혈압 수정 결과(건수) : ", data); | |
743 | - if (data > 0) { | |
744 | - setBloodPressureInit(); | |
745 | - bloodPressureSelectList(); | |
746 | - //closeModal2(); | |
747 | - alert("수정완료"); | |
748 | - } else { | |
749 | - alert("수정에 실패하였습니다. 관리자에게 문의바랍니다."); | |
750 | - } | |
751 | - }).catch((error) => { | |
752 | - console.log('bloodPressureUpdate() /hospital/bloodPressureUpdate.json error : ', error); | |
753 | - }); | |
754 | - } | |
755 | - | |
756 | - //혈압 삭제 | |
757 | - const bloodPressureDelete = () => { | |
758 | - if (confirm('혈압 측정 정보를 삭제하시겠습니까?') == false) { | |
759 | - return; | |
760 | - } | |
761 | - | |
762 | - fetch("/hospital/bloodPressureDelete.json", { | |
763 | - method: "POST", | |
764 | - headers: { | |
765 | - 'Content-Type': 'application/json; charset=UTF-8' | |
766 | - }, | |
767 | - body: JSON.stringify(bloodPressure), | |
768 | - }).then((response) => response.json()).then((data) => { | |
769 | - console.log("혈압 삭제 결과(건수) : ", data); | |
770 | - if (data > 0) { | |
771 | - setBloodPressureInit(); | |
772 | - bloodPressureSelectList(); | |
773 | - //closeModal2(); | |
774 | - alert("삭제완료"); | |
775 | - } else { | |
776 | - alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다."); | |
777 | - } | |
778 | - }).catch((error) => { | |
779 | - console.log('bloodPressureDelete() /hospital/bloodPressureDelete.json error : ', error); | |
780 | - }); | |
781 | - } | |
782 | - | |
783 | - //초기화 취소 | |
784 | - const setBloodPressureInit = () => { | |
785 | - setBloodPressure({...bloodPressureInit}); | |
786 | - } | |
787 | - | |
788 | - //혈압 정보 | |
789 | - const [bloodPressureList, setBloodPressureList] = React.useState({bloodPressureList: [], bloodPressureListCount:0, search: {currentPage: 1, perPage: 5}}); | |
790 | - //혈압 목록 조회 | |
791 | - const bloodPressureSelectList = (currentPage) => { | |
792 | - bloodPressureList.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; | |
793 | - bloodPressureList.search['senior_id'] = targetSenior['senior_id']; | |
794 | - setBloodPressureList({...bloodPressureList}); | |
795 | - | |
796 | - fetch("/hospital/bloodPressureSelectList.json", { | |
797 | - method: "POST", | |
798 | - headers: { | |
799 | - 'Content-Type': 'application/json; charset=UTF-8' | |
800 | - }, | |
801 | - body: JSON.stringify(bloodPressureList.search), | |
802 | - }).then((response) => response.json()).then((data) => { | |
803 | - console.log("혈압 목록 조회 결과(건수) : ", data); | |
804 | - data.search = bloodPressureList.search; | |
805 | - setBloodPressureList(data); | |
806 | - }).catch((error) => { | |
807 | - console.log('bloodPressureSelectList() /hospital/bloodPressureSelectList.json error : ', error); | |
808 | - }); | |
809 | - } | |
810 | - /****************** 혈압 (종료) ******************/ | |
811 | - | |
812 | - React.useEffect(() => { | |
813 | - searching(); | |
814 | - }, []); | |
815 | - | |
816 | - | |
817 | - //현재 탭 Index | |
818 | - const [tabIndex, setTabIndex] = React.useState(0); | |
819 | - //탭 초기화 | |
820 | - const tab = [{ | |
821 | - title: `문진표관리`, | |
822 | - content: ( | |
823 | - <div> | |
824 | - <div className="flex equip-tab"> | |
825 | - <SubTitle explanation={"돌봄 대상자의 병원 내원 기록 및 문진표를 관리할 수 있습니다."} /> | |
826 | - </div> | |
827 | - <table className={"protector-user"}> | |
828 | - <thead> | |
829 | - <tr> | |
830 | - <th>No</th> | |
831 | - <th>소속기관명</th> | |
832 | - <th>이름</th> | |
833 | - <th>생년월일</th> | |
834 | - <th>성별</th> | |
835 | - <th>연락처</th> | |
836 | - <th>문진표관리</th> | |
837 | - <th>내원기록관리</th> | |
838 | - </tr> | |
839 | - </thead> | |
840 | - <tbody> | |
841 | - {senior.seniorList.map((item, idx) => { return ( | |
842 | - <tr key={idx}> | |
843 | - <td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td> | |
844 | - <td data-label="소속기관명">{item['agency_name']}</td> | |
845 | - <td data-label="이름">{item['user_name']}</td> | |
846 | - <td data-label="생년월일">{item['user_birth']}</td> | |
847 | - <td data-label="성별">{item['user_gender']}</td> | |
848 | - <td data-label="연락처">{item['user_phonenumber']}</td> | |
849 | - <td data-label="문진표관리"> | |
850 | - <button className="btn-small gray-btn" onClick={() => openModal(item)}>작성하기</button> | |
851 | - </td> | |
852 | - <td data-label="내원기록관리"> | |
853 | - <button className="btn-small gray-btn" onClick={() => openModal2(item)}>작성하기</button> | |
854 | - </td> | |
855 | - </tr> | |
856 | - )})} | |
857 | - {CommonUtil.isEmpty(senior.seniorList) ? | |
858 | - <tr> | |
859 | - <td colSpan={8}>조회된 데이터가 없습니다</td> | |
860 | - </tr> | |
861 | - : null} | |
862 | - </tbody> | |
863 | - </table> | |
864 | - <Pagination | |
865 | - currentPage={senior.search.currentPage} | |
866 | - perPage={senior.search.perPage} | |
867 | - totalCount={senior.seniorListCount} | |
868 | - maxRange={5} | |
869 | - click={seniorSelectList} | |
870 | - /> | |
871 | - </div> | |
872 | - ) | |
873 | - }, { | |
874 | - title: `심전도관리`, | |
875 | - content: ( | |
876 | - <div> | |
877 | - <div className="flex equip-tab"> | |
878 | - <SubTitle explanation={"돌봄 대상자의 심전도 정보를 관리할 수 있습니다."} /> | |
879 | - </div> | |
880 | - <table className={"protector-user"}> | |
881 | - <thead> | |
882 | - <tr> | |
883 | - <th>No</th> | |
884 | - <th>소속기관명</th> | |
885 | - <th>이름</th> | |
886 | - <th>생년월일</th> | |
887 | - <th>성별</th> | |
888 | - <th>연락처</th> | |
889 | - <th>심전도관리</th> | |
890 | - </tr> | |
891 | - </thead> | |
892 | - <tbody> | |
893 | - {senior.seniorList.map((item, idx) => { return ( | |
894 | - <tr key={idx}> | |
895 | - <td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td> | |
896 | - <td data-label="소속기관명">{item['agency_name']}</td> | |
897 | - <td data-label="이름">{item['user_name']}</td> | |
898 | - <td data-label="생년월일">{item['user_birth']}</td> | |
899 | - <td data-label="성별">{item['user_gender']}</td> | |
900 | - <td data-label="연락처">{item['user_phonenumber']}</td> | |
901 | - <td data-label="심전도관리"> | |
902 | - <button className="btn-small gray-btn" onClick={() => openModal3(item)}>심전도관리</button> | |
903 | - </td> | |
904 | - </tr> | |
905 | - )})} | |
906 | - {CommonUtil.isEmpty(senior.seniorList) ? | |
907 | - <tr> | |
908 | - <td colSpan={7}>조회된 데이터가 없습니다</td> | |
909 | - </tr> | |
910 | - : null} | |
911 | - </tbody> | |
912 | - </table> | |
913 | - <Pagination | |
914 | - currentPage={senior.search.currentPage} | |
915 | - perPage={senior.search.perPage} | |
916 | - totalCount={senior.seniorListCount} | |
917 | - maxRange={5} | |
918 | - click={seniorSelectList} | |
919 | - /> | |
920 | - </div> | |
921 | - ) | |
922 | - }, { | |
923 | - title: `혈압관리`, | |
924 | - content: ( | |
925 | - <div> | |
926 | - <div className="flex equip-tab"> | |
927 | - <SubTitle explanation={"돌봄 대상자의 혈압 정보를 관리할 수 있습니다."} /> | |
928 | - </div> | |
929 | - <table className={"protector-user"}> | |
930 | - <thead> | |
931 | - <tr> | |
932 | - <th>No</th> | |
933 | - <th>소속기관명</th> | |
934 | - <th>이름</th> | |
935 | - <th>생년월일</th> | |
936 | - <th>성별</th> | |
937 | - <th>연락처</th> | |
938 | - <th>혈압관리</th> | |
939 | - </tr> | |
940 | - </thead> | |
941 | - <tbody> | |
942 | - {senior.seniorList.map((item, idx) => { return ( | |
943 | - <tr key={idx}> | |
944 | - <td data-label="No">{senior.seniorListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td> | |
945 | - <td data-label="소속기관명">{item['agency_name']}</td> | |
946 | - <td data-label="이름">{item['user_name']}</td> | |
947 | - <td data-label="생년월일">{item['user_birth']}</td> | |
948 | - <td data-label="성별">{item['user_gender']}</td> | |
949 | - <td data-label="연락처">{item['user_phonenumber']}</td> | |
950 | - <td data-label="혈압관리"> | |
951 | - <button className="btn-small gray-btn" onClick={() => openModal4(item)}>혈압관리</button> | |
952 | - </td> | |
953 | - </tr> | |
954 | - )})} | |
955 | - {CommonUtil.isEmpty(senior.seniorList) ? | |
956 | - <tr> | |
957 | - <td colSpan={7}>조회된 데이터가 없습니다</td> | |
958 | - </tr> | |
959 | - : null} | |
960 | - </tbody> | |
961 | - </table> | |
962 | - <Pagination | |
963 | - currentPage={senior.search.currentPage} | |
964 | - perPage={senior.search.perPage} | |
965 | - totalCount={senior.seniorListCount} | |
966 | - maxRange={5} | |
967 | - click={seniorSelectList} | |
968 | - /> | |
969 | - </div> | |
970 | - ) | |
971 | - }]; | |
972 | - | |
973 | - return ( | |
974 | - <main> | |
975 | - | |
976 | - {/* <Modal_Questionnaire open={modalOpen} close={closeModal} /> */} | |
977 | - {/* <Modal_MedicalHistory open={modalOpen2} close={closeModal2} /> */} | |
978 | - {/* <Modal_ECG open={modalOpen3} close={closeModal3} /> */} | |
979 | - {/* <Modal_Blood open={modalOpen4} close={closeModal4} /> */} | |
980 | - | |
981 | - <div className="search-management flex-start margin-bottom2"> | |
982 | - <select style={{maxWidth: '150px'}} | |
983 | - onChange={(e) => {senior.search.searchType = e.target.value; setSenior({...senior});}}> | |
984 | - <option value="" selected={CommonUtil.isEmpty(senior.search.searchType)}>전체</option> | |
985 | - <option value="user_name" selected={senior.search.searchType == 'user_name'}>이름</option> | |
986 | - <option value="user_id" selected={senior.search.searchType == 'user_id'}>아이디</option> | |
987 | - <option value="user_phonenumber" selected={senior.search.searchType == 'user_phonenumber'}>연락처</option> | |
988 | - </select> | |
989 | - <input type="text" className="senior-search" value={senior.search.searchText} | |
990 | - onChange={(e) => {senior.search.searchText = e.target.value; setSenior({...senior});}} | |
991 | - onKeyUp={(e) => searchingEnter(e.key)}/> | |
992 | - <button className="btn-small gray-btn" onClick={searching}>검색</button> | |
993 | - </div> | |
994 | - | |
995 | - <div className="content-wrap"> | |
996 | - <DetailTitle contentTitle={"대상자의 문진표 / 심전도 / 혈압 관리를 할 수 있습니다."} /> | |
997 | - | |
998 | - <div style={{ height: "calc(100% - 61px)" }}> | |
999 | - <div className="right" style={{ height: "100%", }}> | |
1000 | - <div style={{ height: "100%" }}> | |
1001 | - <div className="tab-container" style={{ marginTop: "5rem"}}> | |
1002 | - {/* {CommonUtil.isEmpty(state.loginUser) == false && state.loginUser['authority'] == 'ROLE_AGENCY' ? | |
1003 | - <div className="flex-end margin-bottom"> | |
1004 | - <div className="flex searchselect" style={{width: 'auto'}}> | |
1005 | - | |
1006 | - <input type="radio" id="my_senior" name="senior" checked={isMySenior} | |
1007 | - onChange={(e) => {e.target.checked ? setIsMySenior(true) : null}}/> | |
1008 | - <label for="my_senior" style={{marginRight: '3rem'}}>나의 대상자 보기</label> | |
1009 | - | |
1010 | - <input type="radio" id="all_senior" name="senior" checked={!isMySenior} | |
1011 | - onChange={(e) => {e.target.checked ? setIsMySenior(false) : null}}/> | |
1012 | - <label for="all_senior" style={{marginRight: '0'}}>전체 대상자 보기</label> | |
1013 | - | |
1014 | - </div> | |
1015 | - </div> | |
1016 | - :null} */} | |
1017 | - <ul className="tab-menu flex-end"> | |
1018 | - {tab.map((item, idx) => { return ( | |
1019 | - <li onClick={() => setTabIndex(idx)} className={idx == tabIndex ? 'active' : null}> | |
1020 | - {item.title} | |
1021 | - </li> | |
1022 | - )})} | |
1023 | - </ul> | |
1024 | - <div className="content-wrap userlist"> | |
1025 | - | |
1026 | - <ul className="tab-content"> | |
1027 | - <li> | |
1028 | - {tab[tabIndex].content} | |
1029 | - </li> | |
1030 | - </ul> | |
1031 | - </div> | |
1032 | - </div> | |
1033 | - </div> | |
1034 | - </div> | |
1035 | - </div> | |
1036 | - </div> | |
1037 | - | |
1038 | - | |
1039 | - <Modal open={modalOpen} close={closeModal} header="문진표 작성"> | |
1040 | - <div className="board-wrap"> | |
1041 | - <div> | |
1042 | - <table className="margin-bottom2 questionnaire-table"> | |
1043 | - <tr> | |
1044 | - <th>흡연을 하십니까?</th> | |
1045 | - <td className="flex-start"> | |
1046 | - <input type="radio" name="smoke" id="smoke-true" | |
1047 | - checked={questionnaire['smoke_type'] == true} | |
1048 | - onChange={(e) => { | |
1049 | - if (e.target.checked) { | |
1050 | - questionnaire['smoke_type'] = true; | |
1051 | - setQuestionnaire({...questionnaire}); | |
1052 | - }} | |
1053 | - } | |
1054 | - /> | |
1055 | - <label for="smoke-true">예</label> | |
1056 | - <input type="radio" name="smoke" id="smoke-false" | |
1057 | - checked={questionnaire['smoke_type'] == false} | |
1058 | - onChange={(e) => { | |
1059 | - if (e.target.checked) { | |
1060 | - questionnaire['smoke_type'] = false; | |
1061 | - setQuestionnaire({...questionnaire}); | |
1062 | - }} | |
1063 | - } | |
1064 | - /> | |
1065 | - <label for="smoke-false">아니요</label> | |
1066 | - </td> | |
1067 | - </tr> | |
1068 | - <tr> | |
1069 | - <th>음주를 하십니까?</th> | |
1070 | - <td className="flex-start"> | |
1071 | - <input type="radio" name="drink" id="drink-true" | |
1072 | - checked={questionnaire['drink_type'] == true} | |
1073 | - onChange={(e) => { | |
1074 | - if (e.target.checked) { | |
1075 | - questionnaire['drink_type'] = true; | |
1076 | - setQuestionnaire({...questionnaire}); | |
1077 | - }} | |
1078 | - } | |
1079 | - /> | |
1080 | - <label for="drink-true">예</label> | |
1081 | - <input type="radio" name="drink" id="drink-false" | |
1082 | - checked={questionnaire['drink_type'] == false} | |
1083 | - onChange={(e) => { | |
1084 | - if (e.target.checked) { | |
1085 | - questionnaire['drink_type'] = false; | |
1086 | - setQuestionnaire({...questionnaire}); | |
1087 | - }} | |
1088 | - } | |
1089 | - /> | |
1090 | - <label for="drink-false">아니요</label> | |
1091 | - </td> | |
1092 | - </tr> | |
1093 | - <tr> | |
1094 | - <th>일주일에 운동을 몇회 하십니까?</th> | |
1095 | - <td className="flex-start"> | |
1096 | - <input type="radio" name="exercise" id="exercise-false" | |
1097 | - checked={questionnaire['exercise_type'] == false} | |
1098 | - onChange={(e) => { | |
1099 | - if (e.target.checked) { | |
1100 | - questionnaire['exercise_type'] = false; | |
1101 | - setQuestionnaire({...questionnaire}); | |
1102 | - }} | |
1103 | - } | |
1104 | - /> | |
1105 | - <label for="exercise-false">안함</label> | |
1106 | - | |
1107 | - <input type="radio" name="exercise" id="exercise-1" | |
1108 | - checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 1} | |
1109 | - onChange={(e) => { | |
1110 | - if (e.target.checked) { | |
1111 | - questionnaire['exercise_type'] = true; | |
1112 | - questionnaire['weekly_exercise_amount'] = 1; | |
1113 | - setQuestionnaire({...questionnaire}); | |
1114 | - }} | |
1115 | - } | |
1116 | - /> | |
1117 | - <label for="exercise-1">1회</label> | |
1118 | - | |
1119 | - <input type="radio" name="exercise" id="exercise-2" | |
1120 | - checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 2} | |
1121 | - onChange={(e) => { | |
1122 | - if (e.target.checked) { | |
1123 | - questionnaire['exercise_type'] = true; | |
1124 | - questionnaire['weekly_exercise_amount'] = 2; | |
1125 | - setQuestionnaire({...questionnaire}); | |
1126 | - }} | |
1127 | - } | |
1128 | - /> | |
1129 | - <label for="exercise-2">2회</label> | |
1130 | - | |
1131 | - <input type="radio" name="exercise" id="exercise-3" | |
1132 | - checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 3} | |
1133 | - onChange={(e) => { | |
1134 | - if (e.target.checked) { | |
1135 | - questionnaire['exercise_type'] = true; | |
1136 | - questionnaire['weekly_exercise_amount'] = 3; | |
1137 | - setQuestionnaire({...questionnaire}); | |
1138 | - }} | |
1139 | - } | |
1140 | - /> | |
1141 | - <label for="exercise-3">3회</label> | |
1142 | - | |
1143 | - <input type="radio" name="exercise" id="exercise-4" | |
1144 | - checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 4} | |
1145 | - onChange={(e) => { | |
1146 | - if (e.target.checked) { | |
1147 | - questionnaire['exercise_type'] = true; | |
1148 | - questionnaire['weekly_exercise_amount'] = 4; | |
1149 | - setQuestionnaire({...questionnaire}); | |
1150 | - }} | |
1151 | - } | |
1152 | - /> | |
1153 | - <label for="exercise-4">4회</label> | |
1154 | - | |
1155 | - <input type="radio" name="exercise" id="exercise-5" | |
1156 | - checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 5} | |
1157 | - onChange={(e) => { | |
1158 | - if (e.target.checked) { | |
1159 | - questionnaire['exercise_type'] = true; | |
1160 | - questionnaire['weekly_exercise_amount'] = 5; | |
1161 | - setQuestionnaire({...questionnaire}); | |
1162 | - }} | |
1163 | - } | |
1164 | - /> | |
1165 | - <label for="exercise-5">5회</label> | |
1166 | - | |
1167 | - <input type="radio" name="exercise" id="exercise-6" | |
1168 | - checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 6} | |
1169 | - onChange={(e) => { | |
1170 | - if (e.target.checked) { | |
1171 | - questionnaire['exercise_type'] = true; | |
1172 | - questionnaire['weekly_exercise_amount'] = 6; | |
1173 | - setQuestionnaire({...questionnaire}); | |
1174 | - }} | |
1175 | - } | |
1176 | - /> | |
1177 | - <label for="exercise-6">6회</label> | |
1178 | - | |
1179 | - <input type="radio" name="exercise" id="exercise-7" | |
1180 | - checked={questionnaire['exercise_type'] == true && questionnaire['weekly_exercise_amount'] == 7} | |
1181 | - onChange={(e) => { | |
1182 | - if (e.target.checked) { | |
1183 | - questionnaire['exercise_type'] = true; | |
1184 | - questionnaire['weekly_exercise_amount'] = 7; | |
1185 | - setQuestionnaire({...questionnaire}); | |
1186 | - }} | |
1187 | - } | |
1188 | - /> | |
1189 | - <label for="exercise-7">매일</label> | |
1190 | - </td> | |
1191 | - </tr> | |
1192 | - <tr> | |
1193 | - <th>최근 3개월 동안 갑작스런 체중 변화가 있었습니까?</th> | |
1194 | - <td className="flex-start"> | |
1195 | - <input type="radio" name="weight" id="weight-up" | |
1196 | - checked={questionnaire['weight_change_amount'] == 1} | |
1197 | - onChange={(e) => { | |
1198 | - if (e.target.checked) { | |
1199 | - questionnaire['weight_change_amount'] = 1; | |
1200 | - setQuestionnaire({...questionnaire}); | |
1201 | - }} | |
1202 | - } | |
1203 | - /> | |
1204 | - <label for="weight-up">예 - 증가</label> | |
1205 | - | |
1206 | - <input type="radio" name="weight" id="weight-down" | |
1207 | - checked={questionnaire['weight_change_amount'] == -1} | |
1208 | - onChange={(e) => { | |
1209 | - if (e.target.checked) { | |
1210 | - questionnaire['weight_change_amount'] = -1; | |
1211 | - setQuestionnaire({...questionnaire}); | |
1212 | - }} | |
1213 | - } | |
1214 | - /> | |
1215 | - <label for="weight-down">예 - 감소</label> | |
1216 | - | |
1217 | - <input type="radio" name="weight" id="weight-false" | |
1218 | - checked={questionnaire['weight_change_amount'] == 0} | |
1219 | - onChange={(e) => { | |
1220 | - if (e.target.checked) { | |
1221 | - questionnaire['weight_change_amount'] = 0; | |
1222 | - setQuestionnaire({...questionnaire}); | |
1223 | - }} | |
1224 | - } | |
1225 | - /> | |
1226 | - <label for="weight-false">아니요</label> | |
1227 | - </td> | |
1228 | - </tr> | |
1229 | - <tr> | |
1230 | - <th>현재 복용중인 약이 있으면 체크를 해주세요.</th> | |
1231 | - <td className="flex-start"> | |
1232 | - | |
1233 | - <input type="checkbox" name="pills" id="pills-1" | |
1234 | - checked={questionnaire['medication_pill'].indexOf('아스피린(항혈소판제)') > -1} | |
1235 | - onChange={(e) => { | |
1236 | - if (e.target.checked) { | |
1237 | - questionnaire['medication_pill'] += '아스피린(항혈소판제),'; | |
1238 | - setQuestionnaire({...questionnaire}); | |
1239 | - } else { | |
1240 | - questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('아스피린(항혈소판제),', ''); | |
1241 | - setQuestionnaire({...questionnaire}); | |
1242 | - }} | |
1243 | - } | |
1244 | - /> | |
1245 | - <label for="pills-1">아스피린(항혈소판제)</label> | |
1246 | - | |
1247 | - <input type="checkbox" name="pills" id="pills-2" | |
1248 | - checked={questionnaire['medication_pill'].indexOf('당뇨약') > -1} | |
1249 | - onChange={(e) => { | |
1250 | - if (e.target.checked) { | |
1251 | - questionnaire['medication_pill'] += '당뇨약,'; | |
1252 | - setQuestionnaire({...questionnaire}); | |
1253 | - } else { | |
1254 | - questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('당뇨약,', ''); | |
1255 | - setQuestionnaire({...questionnaire}); | |
1256 | - }} | |
1257 | - } | |
1258 | - /> | |
1259 | - <label for="pills-2">당뇨약</label> | |
1260 | - | |
1261 | - <input type="checkbox" name="pills" id="pills-3" | |
1262 | - checked={questionnaire['medication_pill'].indexOf('고혈압약') > -1} | |
1263 | - onChange={(e) => { | |
1264 | - if (e.target.checked) { | |
1265 | - questionnaire['medication_pill'] += '고혈압약,'; | |
1266 | - setQuestionnaire({...questionnaire}); | |
1267 | - } else { | |
1268 | - questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('고혈압약,', ''); | |
1269 | - setQuestionnaire({...questionnaire}); | |
1270 | - }} | |
1271 | - } | |
1272 | - /> | |
1273 | - <label for="pills-3">고혈압약</label> | |
1274 | - | |
1275 | - <input type="checkbox" name="pills" id="pills-etc" | |
1276 | - checked={questionnaire['medication_pill'].indexOf('기타「」,') > -1} | |
1277 | - onChange={(e) => { | |
1278 | - if (e.target.checked) { | |
1279 | - questionnaire['medication_pill'] += '기타「」,'; | |
1280 | - setQuestionnaire({...questionnaire}); | |
1281 | - } else { | |
1282 | - questionnaire['medication_pill'] = questionnaire['medication_pill'].replace('기타「」,', ''); | |
1283 | - questionnaire['medication_pill_etc'] = ''; | |
1284 | - setQuestionnaire({...questionnaire}); | |
1285 | - }} | |
1286 | - } | |
1287 | - /> | |
1288 | - <label for="pills-etc">기타</label> | |
1289 | - <input type="text" style={{width: 'max-content', marginLeft: '1rem'}} | |
1290 | - disabled={questionnaire['medication_pill'].indexOf('기타「」,') == -1} | |
1291 | - value={questionnaire['medication_pill_etc']} | |
1292 | - onChange={(e) => { | |
1293 | - questionnaire['medication_pill_etc'] = e.target.value; | |
1294 | - setQuestionnaire({...questionnaire}); | |
1295 | - }} | |
1296 | - /> | |
1297 | - | |
1298 | - </td> | |
1299 | - </tr> | |
1300 | - </table> | |
1301 | - </div> | |
1302 | - <div className="flex-center"> | |
1303 | - {CommonUtil.isEmpty(questionnaire['questionnaire_record_idx']) | |
1304 | - ? <button className="btn-small red-btn" onClick={questionnaireInsert}>저장</button> | |
1305 | - : <button className="btn-small red-btn" onClick={questionnaireUpdate}>저장</button> | |
1306 | - } | |
1307 | - </div> | |
1308 | - </div> | |
1309 | - </Modal> | |
1310 | - | |
1311 | - | |
1312 | - <Modal open={modalOpen2} close={closeModal2} header="내원기록 관리 및 조회"> | |
1313 | - <div className="board-wrap"> | |
1314 | - <table className="margin-bottom2 senior-insert "> | |
1315 | - <tr> | |
1316 | - <th>진료 일자</th> | |
1317 | - <td> | |
1318 | - <input type="date" | |
1319 | - value={hospitalMedicalRecord['medical_date']} | |
1320 | - onChange={(e) => { | |
1321 | - hospitalMedicalRecord['medical_date'] = e.target.value; | |
1322 | - setHospitalMedicalRecord({...hospitalMedicalRecord}); | |
1323 | - }} | |
1324 | - ref={el => hospitalMedicalRecordRef.current['medical_date'] = el} | |
1325 | - /> | |
1326 | - </td> | |
1327 | - </tr> | |
1328 | - <tr> | |
1329 | - <th>진료 사유</th> | |
1330 | - <td className="flex-start "> | |
1331 | - <input type="text" | |
1332 | - value={hospitalMedicalRecord['medical_reason']} | |
1333 | - onChange={(e) => { | |
1334 | - hospitalMedicalRecord['medical_reason'] = e.target.value; | |
1335 | - setHospitalMedicalRecord({...hospitalMedicalRecord}); | |
1336 | - }} | |
1337 | - ref={el => hospitalMedicalRecordRef.current['medical_reason'] = el} | |
1338 | - /> | |
1339 | - </td> | |
1340 | - </tr> | |
1341 | - <tr> | |
1342 | - <th>진료 내용</th> | |
1343 | - <td colSpan={3}> | |
1344 | - <textarea className="medicine" cols="30" rows="2" | |
1345 | - value={hospitalMedicalRecord['medical_content']} | |
1346 | - onChange={(e) => { | |
1347 | - hospitalMedicalRecord['medical_content'] = e.target.value; | |
1348 | - setHospitalMedicalRecord({...hospitalMedicalRecord}); | |
1349 | - }} | |
1350 | - ref={el => hospitalMedicalRecordRef.current['medical_content'] = el} | |
1351 | - ></textarea> | |
1352 | - </td> | |
1353 | - </tr> | |
1354 | - </table> | |
1355 | - <div className="btn-wrap flex-center margin-bottom5"> | |
1356 | - {CommonUtil.isEmpty(hospitalMedicalRecord['medical_record_idx']) | |
1357 | - ? <button className="btn-small red-btn" onClick={hospitalMedicalRecordInsert}>등록</button> | |
1358 | - | |
1359 | - : <> | |
1360 | - <button className="btn-small gray-btn" onClick={setHospitalMedicalRecordInit}>수정취소</button> | |
1361 | - <button className="btn-small red-btn" onClick={hospitalMedicalRecordUpdate}>수정</button> | |
1362 | - <button className="btn-small red-btn" onClick={hospitalMedicalRecordDelete}>삭제</button> | |
1363 | - </> | |
1364 | - } | |
1365 | - </div> | |
1366 | - <div> | |
1367 | - <table className="caregiver-user senior-insert senior-table"> | |
1368 | - <thead> | |
1369 | - <tr> | |
1370 | - <th>No</th> | |
1371 | - <th>진료 일자</th> | |
1372 | - <th>진료 사유</th> | |
1373 | - <th>진료 내용</th> | |
1374 | - <th>기록 작성자</th> | |
1375 | - </tr> | |
1376 | - </thead> | |
1377 | - <tbody> | |
1378 | - {hospitalMedicalRecordList.hospitalMedicalRecordList.map((item, idx) => { return ( | |
1379 | - <tr key={idx} onClick={() => {setHospitalMedicalRecord(item)}}> | |
1380 | - <td data-label="No">{hospitalMedicalRecordList.hospitalMedicalRecordListCount - idx - (hospitalMedicalRecordList.search.currentPage - 1) * hospitalMedicalRecordList.search.perPage}</td> | |
1381 | - <td data-label="진료 일자">{item['medical_date']}</td> | |
1382 | - <td data-label="진료 내용">{item['medical_reason']}</td> | |
1383 | - <td data-label="진료 사유">{item['medical_content']}</td> | |
1384 | - <td data-label="기록 작성자">{item['insert_user_name']}</td> | |
1385 | - </tr> | |
1386 | - )})} | |
1387 | - {CommonUtil.isEmpty(hospitalMedicalRecordList.hospitalMedicalRecordList) ? | |
1388 | - <tr> | |
1389 | - <td colSpan={5}>조회된 데이터가 없습니다</td> | |
1390 | - </tr> | |
1391 | - : null} | |
1392 | - </tbody> | |
1393 | - </table> | |
1394 | - <Pagination | |
1395 | - currentPage={hospitalMedicalRecordList.search.currentPage} | |
1396 | - perPage={hospitalMedicalRecordList.search.perPage} | |
1397 | - totalCount={hospitalMedicalRecordList.hospitalMedicalRecordListCount} | |
1398 | - maxRange={5} | |
1399 | - click={hospitalMedicalRecordSelectList} | |
1400 | - /> | |
1401 | - </div> | |
1402 | - </div> | |
1403 | - </Modal> | |
1404 | - | |
1405 | - | |
1406 | - <Modal open={modalOpen3} close={closeModal3} header="심전도판독소견 관리 및 조회"> | |
1407 | - <div className="board-wrap"> | |
1408 | - <table className="margin-bottom2 senior-insert "> | |
1409 | - <tr> | |
1410 | - <th>측정 일자</th> | |
1411 | - <td colSpan={5}> | |
1412 | - <input type="date" value={ecg['ecg_reading_date']} | |
1413 | - onChange={(e) => { | |
1414 | - ecg['ecg_reading_date'] = e.target.value; | |
1415 | - setEcg({...ecg}); | |
1416 | - }} | |
1417 | - ref={el => ecgRef.current['ecg_reading_date'] = el} | |
1418 | - /> | |
1419 | - </td> | |
1420 | - </tr> | |
1421 | - <tr> | |
1422 | - <th>판독 소견 종류</th> | |
1423 | - <td colSpan={5}> | |
1424 | - <input type="text" value={ecg['ecg_finding_type']} | |
1425 | - onChange={(e) => { | |
1426 | - ecg['ecg_finding_type'] = e.target.value; | |
1427 | - setEcg({...ecg}); | |
1428 | - }} | |
1429 | - ref={el => ecgRef.current['ecg_finding_type'] = el} | |
1430 | - /> | |
1431 | - </td> | |
1432 | - </tr> | |
1433 | - <tr> | |
1434 | - <th>판독 소견 내용</th> | |
1435 | - <td colSpan={5}> | |
1436 | - <textarea className="medicine" cols="30" rows="2" | |
1437 | - value={ecg['ecg_finding_content']} | |
1438 | - onChange={(e) => { | |
1439 | - ecg['ecg_finding_content'] = e.target.value; | |
1440 | - setEcg({...ecg}); | |
1441 | - }} | |
1442 | - ref={el => ecgRef.current['ecg_finding_content'] = el} | |
1443 | - ></textarea> | |
1444 | - </td> | |
1445 | - </tr> | |
1446 | - {ecg.isEdit == false | |
1447 | - ? <tr> | |
1448 | - <th>측정 파일</th> | |
1449 | - <td colSpan={5}> | |
1450 | - <CommonFile commonFileList={ecg['commonFileList']} multiple={false} accept={'.dat, .ecg'}/> | |
1451 | - </td> | |
1452 | - </tr> | |
1453 | - : <> | |
1454 | - <tr> | |
1455 | - <th>서맥 횟수</th> | |
1456 | - <td> | |
1457 | - <input type="number" value={ecg['bradycardia_count']} | |
1458 | - onChange={(e) => { | |
1459 | - ecg['bradycardia_count'] = e.target.value; | |
1460 | - setEcg({...ecg}); | |
1461 | - }} | |
1462 | - ref={el => ecgRef.current['bradycardia_count'] = el} | |
1463 | - /> | |
1464 | - </td> | |
1465 | - <th>빈맥 횟수</th> | |
1466 | - <td> | |
1467 | - <input type="number" value={ecg['tachycardia_count']} | |
1468 | - onChange={(e) => { | |
1469 | - ecg['tachycardia_count'] = e.target.value; | |
1470 | - setEcg({...ecg}); | |
1471 | - }} | |
1472 | - ref={el => ecgRef.current['tachycardia_count'] = el} | |
1473 | - /> | |
1474 | - </td> | |
1475 | - <th>이상 심박수</th> | |
1476 | - <td> | |
1477 | - <input type="number" value={ecg['abnormal_heart_rate']} | |
1478 | - onChange={(e) => { | |
1479 | - ecg['abnormal_heart_rate'] = e.target.value; | |
1480 | - setEcg({...ecg}); | |
1481 | - }} | |
1482 | - ref={el => ecgRef.current['abnormal_heart_rate'] = el} | |
1483 | - /> | |
1484 | - </td> | |
1485 | - </tr> | |
1486 | - <tr> | |
1487 | - <th>최대 심박수</th> | |
1488 | - <td> | |
1489 | - <input type="number" value={ecg['max_heart_rate']} | |
1490 | - onChange={(e) => { | |
1491 | - ecg['max_heart_rate'] = e.target.value; | |
1492 | - setEcg({...ecg}); | |
1493 | - }} | |
1494 | - ref={el => ecgRef.current['max_heart_rate'] = el} | |
1495 | - /> | |
1496 | - </td> | |
1497 | - <th>최소 심박수</th> | |
1498 | - <td> | |
1499 | - <input type="number" value={ecg['min_heart_rate']} | |
1500 | - onChange={(e) => { | |
1501 | - ecg['min_heart_rate'] = e.target.value; | |
1502 | - setEcg({...ecg}); | |
1503 | - }} | |
1504 | - ref={el => ecgRef.current['min_heart_rate'] = el} | |
1505 | - /> | |
1506 | - </td> | |
1507 | - <th>평균 심박수</th> | |
1508 | - <td> | |
1509 | - <input type="number" value={ecg['average_heart_rate']} | |
1510 | - onChange={(e) => { | |
1511 | - ecg['average_heart_rate'] = e.target.value; | |
1512 | - setEcg({...ecg}); | |
1513 | - }} | |
1514 | - ref={el => ecgRef.current['average_heart_rate'] = el} | |
1515 | - /> | |
1516 | - </td> | |
1517 | - </tr> | |
1518 | - <tr> | |
1519 | - <th>최대 R-R</th> | |
1520 | - <td> | |
1521 | - <input type="number" value={ecg['max_rr']} | |
1522 | - onChange={(e) => { | |
1523 | - ecg['max_rr'] = e.target.value; | |
1524 | - setEcg({...ecg}); | |
1525 | - }} | |
1526 | - ref={el => ecgRef.current['max_rr'] = el} | |
1527 | - /> | |
1528 | - </td> | |
1529 | - <th>최소 R-R</th> | |
1530 | - <td> | |
1531 | - <input type="number" value={ecg['min_rr']} | |
1532 | - onChange={(e) => { | |
1533 | - ecg['min_rr'] = e.target.value; | |
1534 | - setEcg({...ecg}); | |
1535 | - }} | |
1536 | - ref={el => ecgRef.current['min_rr'] = el} | |
1537 | - /> | |
1538 | - </td> | |
1539 | - <th>평균 R-R</th> | |
1540 | - <td> | |
1541 | - <input type="number" value={ecg['average_rr']} | |
1542 | - onChange={(e) => { | |
1543 | - ecg['average_rr'] = e.target.value; | |
1544 | - setEcg({...ecg}); | |
1545 | - }} | |
1546 | - ref={el => ecgRef.current['average_rr'] = el} | |
1547 | - /> | |
1548 | - </td> | |
1549 | - </tr> | |
1550 | - </>} | |
1551 | - </table> | |
1552 | - <div className="btn-wrap flex-center margin-bottom5"> | |
1553 | - {CommonUtil.isEmpty(ecg['ecg_record_idx']) | |
1554 | - ? <button className="btn-small red-btn" onClick={ecgInsert}>등록</button> | |
1555 | - : <> | |
1556 | - <button className="btn-small gray-btn" onClick={setEcgInit}>수정취소</button> | |
1557 | - <button className="btn-small red-btn" onClick={ecgUpdate}>수정</button> | |
1558 | - <button className="btn-small red-btn" onClick={ecgDelete}>삭제</button> | |
1559 | - </> | |
1560 | - } | |
1561 | - </div> | |
1562 | - <div> | |
1563 | - <table className="caregiver-user senior-table"> | |
1564 | - <thead> | |
1565 | - <tr> | |
1566 | - <th>No</th> | |
1567 | - <th>소견 작성 일자</th> | |
1568 | - <th>판독 소견 종류</th> | |
1569 | - <th>소견 작성자</th> | |
1570 | - </tr> | |
1571 | - </thead> | |
1572 | - <tbody> | |
1573 | - {ecgList.ecgList.map((item, idx) => { return ( | |
1574 | - <tr key={idx} onClick={() => {item.isEdit = false; setEcg(item);}}> | |
1575 | - <td data-label="No">{ecgList.ecgListCount - idx - (ecgList.search.currentPage - 1) * ecgList.search.perPage}</td> | |
1576 | - <td data-label="소견 작성 일자">{item['ecg_reading_date']}</td> | |
1577 | - <td data-label="판독 소견 종류">{item['ecg_finding_type']}</td> | |
1578 | - <td data-label="소견 작성자">{item['insert_user_name']}</td> | |
1579 | - </tr> | |
1580 | - )})} | |
1581 | - {CommonUtil.isEmpty(ecgList.ecgList) ? | |
1582 | - <tr> | |
1583 | - <td colSpan={4}>조회된 데이터가 없습니다</td> | |
1584 | - </tr> | |
1585 | - : null} | |
1586 | - </tbody> | |
1587 | - </table> | |
1588 | - <Pagination | |
1589 | - currentPage={ecgList.search.currentPage} | |
1590 | - perPage={ecgList.search.perPage} | |
1591 | - totalCount={ecgList.ecgListCount} | |
1592 | - maxRange={5} | |
1593 | - click={ecgSelectList} | |
1594 | - /> | |
1595 | - </div> | |
1596 | - </div> | |
1597 | - </Modal> | |
1598 | - | |
1599 | - | |
1600 | - <Modal open={modalOpen4} close={closeModal4} header="혈압측정결과 관리 및 조회"> | |
1601 | - <div className="board-wrap"> | |
1602 | - <table className="margin-bottom2 senior-insert "> | |
1603 | - <tr> | |
1604 | - <th>측정일자</th> | |
1605 | - <td> | |
1606 | - <input type="date" value={bloodPressure['blood_pressure_record_date']} | |
1607 | - onChange={(e) => { | |
1608 | - bloodPressure['blood_pressure_record_date'] = e.target.value; | |
1609 | - setBloodPressure({...bloodPressure}); | |
1610 | - }} | |
1611 | - ref={el => bloodPressureRef.current['blood_pressure_record_date'] = el} | |
1612 | - /> | |
1613 | - </td> | |
1614 | - </tr> | |
1615 | - <tr> | |
1616 | - <th>최고혈압</th> | |
1617 | - <td> | |
1618 | - <input type="number" value={bloodPressure['max_blood_pressure']} | |
1619 | - onChange={(e) => { | |
1620 | - bloodPressure['max_blood_pressure'] = e.target.value; | |
1621 | - setBloodPressure({...bloodPressure}); | |
1622 | - }} | |
1623 | - ref={el => bloodPressureRef.current['max_blood_pressure'] = el} | |
1624 | - /> | |
1625 | - </td> | |
1626 | - </tr> | |
1627 | - <tr> | |
1628 | - <th>최저혈압</th> | |
1629 | - <td> | |
1630 | - <input type="number" value={bloodPressure['min_blood_pressure']} | |
1631 | - onChange={(e) => { | |
1632 | - bloodPressure['min_blood_pressure'] = e.target.value; | |
1633 | - setBloodPressure({...bloodPressure}); | |
1634 | - }} | |
1635 | - ref={el => bloodPressureRef.current['min_blood_pressure'] = el} | |
1636 | - /> | |
1637 | - </td> | |
1638 | - </tr> | |
1639 | - <tr> | |
1640 | - <th>맥박수</th> | |
1641 | - <td> | |
1642 | - <input type="number" value={bloodPressure['pulse_rate']} | |
1643 | - onChange={(e) => { | |
1644 | - bloodPressure['pulse_rate'] = e.target.value; | |
1645 | - setBloodPressure({...bloodPressure}); | |
1646 | - }} | |
1647 | - ref={el => bloodPressureRef.current['pulse_rate'] = el} | |
1648 | - /> | |
1649 | - </td> | |
1650 | - </tr> | |
1651 | - </table> | |
1652 | - <div className="btn-wrap flex-center margin-bottom5"> | |
1653 | - {CommonUtil.isEmpty(bloodPressure['blood_pressure_record_idx']) | |
1654 | - ? <button className="btn-small red-btn" onClick={bloodPressureInsert}>등록</button> | |
1655 | - : <> | |
1656 | - <button className="btn-small gray-btn" onClick={setBloodPressureInit}>수정취소</button> | |
1657 | - <button className="btn-small red-btn" onClick={bloodPressureUpdate}>수정</button> | |
1658 | - <button className="btn-small red-btn" onClick={bloodPressureDelete}>삭제</button> | |
1659 | - </> | |
1660 | - } | |
1661 | - </div> | |
1662 | - <div> | |
1663 | - <table className="caregiver-user senior-insert senior-table"> | |
1664 | - <thead> | |
1665 | - <tr> | |
1666 | - <th>No</th> | |
1667 | - <th>기록 작성일</th> | |
1668 | - <th>최고 혈압</th> | |
1669 | - <th>최저 혈압</th> | |
1670 | - <th>맥박수</th> | |
1671 | - <th>기록 작성자</th> | |
1672 | - </tr> | |
1673 | - </thead> | |
1674 | - <tbody> | |
1675 | - {bloodPressureList.bloodPressureList.map((item, idx) => { return ( | |
1676 | - <tr key={idx} onClick={() => {setBloodPressure(item);}}> | |
1677 | - <td data-label="No">{bloodPressureList.bloodPressureListCount - idx - (bloodPressureList.search.currentPage - 1) * bloodPressureList.search.perPage}</td> | |
1678 | - <td data-label="기록 작성일">{item['blood_pressure_record_date']}</td> | |
1679 | - <td data-label="최고 혈압">{item['max_blood_pressure']}</td> | |
1680 | - <td data-label="최저 혈압">{item['min_blood_pressure']}</td> | |
1681 | - <td data-label="맥박수">{item['pulse_rate']}</td> | |
1682 | - <td data-label="기록 작성자">{item['insert_user_name']}</td> | |
1683 | - </tr> | |
1684 | - )})} | |
1685 | - {CommonUtil.isEmpty(bloodPressureList.bloodPressureList) ? | |
1686 | - <tr> | |
1687 | - <td colSpan={6}>조회된 데이터가 없습니다</td> | |
1688 | - </tr> | |
1689 | - : null} | |
1690 | - </tbody> | |
1691 | - </table> | |
1692 | - <Pagination | |
1693 | - currentPage={bloodPressureList.search.currentPage} | |
1694 | - perPage={bloodPressureList.search.perPage} | |
1695 | - totalCount={bloodPressureList.bloodPressureListCount} | |
1696 | - maxRange={5} | |
1697 | - click={bloodPressureSelectList} | |
1698 | - /> | |
1699 | - </div> | |
1700 | - </div> | |
1701 | - </Modal> | |
1702 | - | |
1703 | - </main> | |
1704 | - ); | |
1705 | -} |
--- client/views/pages/main/Main_agencyAdmin.jsx
+++ client/views/pages/main/Main_agencyAdmin.jsx
... | ... | @@ -22,280 +22,279 @@ |
22 | 22 |
|
23 | 23 |
import "leaflet/dist/leaflet.css"; |
24 | 24 |
|
25 |
-export default function Main_government() { |
|
25 |
+export default function Main_agencyAdmin() { |
|
26 | 26 |
|
27 |
- //전역 변수 저장 객체 |
|
28 |
- const state = useSelector((state) => { return state }); |
|
27 |
+ //전역 변수 저장 객체 |
|
28 |
+ const state = useSelector((state) => { return state }); |
|
29 | 29 |
|
30 |
- const [cityName, setCityName] = useState(state.loginUser['government_name']); |
|
30 |
+ const [cityName, setCityName] = useState(state.loginUser['agency_name']); |
|
31 | 31 |
|
32 |
- //대상자(시니어) 목록 조회 |
|
33 |
- const [senior, setSenior] = React.useState({ userList: [], userListCount: 0 }); |
|
34 |
- const seniorSelectList = () => { |
|
35 |
- fetch("/user/userSelectList.json", { |
|
36 |
- method: "POST", |
|
37 |
- headers: { |
|
38 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
39 |
- }, |
|
40 |
- body: JSON.stringify({ |
|
41 |
- 'government_id': state.loginUser['government_id'], |
|
42 |
- 'agency_id': state.loginUser['agency_id'], |
|
43 |
- 'authority': 'ROLE_SENIOR', |
|
44 |
- }), |
|
45 |
- }).then((response) => response.json()).then((data) => { |
|
46 |
- console.log("대상자(시니어) 목록 조회 : ", data); |
|
47 |
- setSenior(data); |
|
48 |
- }).catch((error) => { |
|
49 |
- console.log('seniorSelectList() /user/userSelectList.json error : ', error); |
|
50 |
- }); |
|
51 |
- } |
|
52 |
- |
|
53 |
- //전체 대상자(시니어) 수 조회 |
|
54 |
- const [seniorCount, setSeniorCount] = React.useState(0); |
|
55 |
- const seniorCountTotal = () => { |
|
56 |
- fetch("/stats/governmentSeniorCount.json", { |
|
57 |
- method: "POST", |
|
58 |
- headers: { |
|
59 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
60 |
- }, |
|
61 |
- body: JSON.stringify({ |
|
62 |
- 'government_id': state.loginUser['government_id'], |
|
63 |
- }), |
|
64 |
- }).then((response) => response.json()).then((data) => { |
|
65 |
- console.log("전체 대상자(시니어) 수 조회 : ", data); |
|
66 |
- setSeniorCount(data); |
|
67 |
- }).catch((error) => { |
|
68 |
- console.log('governmentSeniorCount() /stats/governmentSeniorCount.json error : ', error); |
|
69 |
- }); |
|
70 |
- } |
|
71 |
- |
|
72 |
- //온도 위험 대상자(시니어) 수 조회 |
|
73 |
- const [temperatureCount, setTemperatureCount] = React.useState(0); |
|
74 |
- const temperatureRiskCount = () => { |
|
75 |
- fetch("/stats/governmentTemperatureRisk.json", { |
|
76 |
- method: "POST", |
|
77 |
- headers: { |
|
78 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
79 |
- }, |
|
80 |
- body: JSON.stringify({ |
|
81 |
- 'government_id': state.loginUser['government_id'], |
|
82 |
- }), |
|
83 |
- }).then((response) => response.json()).then((data) => { |
|
84 |
- console.log("온도 위험 대상자(시니어) 수 조회 : ", data); |
|
85 |
- setTemperatureCount(data); |
|
86 |
- }).catch((error) => { |
|
87 |
- console.log('temperatureRiskCount() /stats/governmentTemperatureRisk.json error : ', error); |
|
88 |
- }); |
|
89 |
- } |
|
90 |
- |
|
91 |
- //배터리 부족 대상자(시니어) 수 조회 |
|
92 |
- const [batteryCount, setbatteryCount] = React.useState(0); |
|
93 |
- const batteryRiskCount = () => { |
|
94 |
- fetch("/stats/governmentBatteryRisk.json", { |
|
95 |
- method: "POST", |
|
96 |
- headers: { |
|
97 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
98 |
- }, |
|
99 |
- body: JSON.stringify({ |
|
100 |
- 'government_id': state.loginUser['government_id'], |
|
101 |
- }), |
|
102 |
- }).then((response) => response.json()).then((data) => { |
|
103 |
- console.log("배터리 부족 대상자(시니어) 수 조회 : ", data); |
|
104 |
- setbatteryCount(data); |
|
105 |
- }).catch((error) => { |
|
106 |
- console.log('batteryRiskCount() /stats/governmentBatteryRisk.json error : ', error); |
|
107 |
- }); |
|
108 |
- } |
|
109 |
- |
|
110 |
- //월별 방문 횟수 조회 |
|
111 |
- const [visit, setVisit] = React.useState([]); |
|
112 |
- const visitByMonthList = () => { |
|
113 |
- fetch("/stats/visitByMonthList.json", { |
|
114 |
- method: "POST", |
|
115 |
- headers: { |
|
116 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
117 |
- }, |
|
118 |
- body: JSON.stringify({ |
|
119 |
- 'government_id': state.loginUser['government_id'], |
|
120 |
- }), |
|
121 |
- }).then((response) => response.json()).then((data) => { |
|
122 |
- console.log("월별 방문 횟수 조회 : ", data); |
|
123 |
- setVisit(data); |
|
124 |
- }).catch((error) => { |
|
125 |
- console.log('visitByMonthList() /stats/visitByMonthList.json error : ', error); |
|
126 |
- }); |
|
127 |
- } |
|
128 |
- |
|
129 |
- //시행 기관별 약상자 사용 현황 |
|
130 |
- const [equipmentUsage, setEquipmentUsage] = React.useState([]); |
|
131 |
- const equipmentByAgency = () => { |
|
132 |
- fetch("/stats/equipmentByAgency.json", { |
|
133 |
- method: "POST", |
|
134 |
- headers: { |
|
135 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
136 |
- }, |
|
137 |
- body: JSON.stringify({ |
|
138 |
- 'government_id': state.loginUser['government_id'], |
|
139 |
- }), |
|
140 |
- }).then((response) => response.json()).then((data) => { |
|
141 |
- console.log("시행기관별 약상자 사용 현황 : ", data); |
|
142 |
- let newEquipmentList = updateList(data['agencyList'], data['equipmentList']); |
|
143 |
- console.log("new equipment list", newEquipmentList); |
|
144 |
- setEquipmentUsage(newEquipmentList); |
|
145 |
- }).catch((error) => { |
|
146 |
- console.log('equipmentByAgency() /stats/equipmentByAgency.json error : ', error); |
|
147 |
- }); |
|
148 |
- } |
|
149 |
- |
|
150 |
- //시행 기관별 대상자(시니어) 등록 현황 |
|
151 |
- const [seniorEnroll, setSeniorEnroll] = React.useState([]); |
|
152 |
- const seniorByAgency = () => { |
|
153 |
- fetch("/stats/seniorByAgency.json", { |
|
154 |
- method: "POST", |
|
155 |
- headers: { |
|
156 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
157 |
- }, |
|
158 |
- body: JSON.stringify({ |
|
159 |
- 'government_id': state.loginUser['government_id'], |
|
160 |
- }), |
|
161 |
- }).then((response) => response.json()).then((data) => { |
|
162 |
- console.log("시행기관별 시니어 등록 현황 : ", data); |
|
163 |
- let newSeniorList = updateList(data['agencyList'], data['seniorList']); |
|
164 |
- console.log("new senior list", newSeniorList); |
|
165 |
- setSeniorEnroll(newSeniorList); |
|
166 |
- }).catch((error) => { |
|
167 |
- console.log('seniorByAgency() /stats/seniorByAgency.json error : ', error); |
|
168 |
- }); |
|
169 |
- } |
|
170 |
- |
|
171 |
- function updateList(agencyList, countList) { |
|
172 |
- |
|
173 |
- const result = []; |
|
174 |
- for (let i = 0; i < agencyList.length; i++) { |
|
175 |
- const agency = agencyList[i]; |
|
176 |
- let count = 0; |
|
177 |
- for (let j = 0; j < countList.length; j++) { |
|
178 |
- if (countList[j].agency_id === agency.agency_id) { |
|
179 |
- count = countList[j].count; |
|
180 |
- break; |
|
181 |
- } |
|
182 |
- } |
|
183 |
- result.push({ agency_id: agency.agency_id, agency_name: agency.agency_name, count: count }); |
|
184 |
- } |
|
185 |
- return result; |
|
186 |
- } |
|
187 |
- |
|
188 |
- |
|
189 |
- |
|
190 |
- const iconHouse = new L.Icon({ |
|
191 |
- iconUrl: '/client/resources/files/images/house.png', |
|
192 |
- iconRetinaUrl: '/client/resources/files/images/house.png', |
|
193 |
- iconSize: [20, 20], |
|
194 |
- className: 'leaflet-background-radius-icon'//leaflet-div-icon |
|
32 |
+ //대상자(시니어) 목록 조회 |
|
33 |
+ const [senior, setSenior] = React.useState({ userList: [], userListCount: 0 }); |
|
34 |
+ const seniorSelectList = () => { |
|
35 |
+ fetch("/user/userSelectList.json", { |
|
36 |
+ method: "POST", |
|
37 |
+ headers: { |
|
38 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
39 |
+ }, |
|
40 |
+ body: JSON.stringify({ |
|
41 |
+ 'agency_id': state.loginUser['agency_id'], |
|
42 |
+ 'authority': 'ROLE_SENIOR', |
|
43 |
+ }), |
|
44 |
+ }).then((response) => response.json()).then((data) => { |
|
45 |
+ console.log("대상자(시니어) 목록 조회 : ", data); |
|
46 |
+ setSenior(data); |
|
47 |
+ }).catch((error) => { |
|
48 |
+ console.log('seniorSelectList() /user/userSelectList.json error : ', error); |
|
195 | 49 |
}); |
50 |
+ } |
|
196 | 51 |
|
197 |
- React.useEffect(() => { |
|
198 |
- seniorSelectList(); |
|
199 |
- seniorCountTotal(); |
|
200 |
- temperatureRiskCount(); |
|
201 |
- batteryRiskCount(); |
|
202 |
- visitByMonthList(); |
|
203 |
- equipmentByAgency(); |
|
204 |
- seniorByAgency(); |
|
205 |
- }, []); |
|
52 |
+ //전체 대상자(시니어) 수 조회 |
|
53 |
+ const [seniorCount, setSeniorCount] = React.useState(0); |
|
54 |
+ const seniorCountTotal = () => { |
|
55 |
+ fetch("/stats/agencySeniorCount.json", { |
|
56 |
+ method: "POST", |
|
57 |
+ headers: { |
|
58 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
59 |
+ }, |
|
60 |
+ body: JSON.stringify({ |
|
61 |
+ 'agency_id': state.loginUser['agency_id'], |
|
62 |
+ }), |
|
63 |
+ }).then((response) => response.json()).then((data) => { |
|
64 |
+ console.log("전체 대상자(시니어) 수 조회 : ", data); |
|
65 |
+ setSeniorCount(data); |
|
66 |
+ }).catch((error) => { |
|
67 |
+ console.log('agencySeniorCount() /stats/agencySeniorCount.json error : ', error); |
|
68 |
+ }); |
|
69 |
+ } |
|
70 |
+ |
|
71 |
+ //온도 위험 대상자(시니어) 수 조회 |
|
72 |
+ const [temperatureCount, setTemperatureCount] = React.useState(0); |
|
73 |
+ const temperatureRiskCount = () => { |
|
74 |
+ fetch("/stats/agencyTemperatureRisk.json", { |
|
75 |
+ method: "POST", |
|
76 |
+ headers: { |
|
77 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
78 |
+ }, |
|
79 |
+ body: JSON.stringify({ |
|
80 |
+ 'agency_id': state.loginUser['agency_id'], |
|
81 |
+ }), |
|
82 |
+ }).then((response) => response.json()).then((data) => { |
|
83 |
+ console.log("온도 위험 대상자(시니어) 수 조회 : ", data); |
|
84 |
+ setTemperatureCount(data); |
|
85 |
+ }).catch((error) => { |
|
86 |
+ console.log('temperatureRiskCount() /stats/agencyTemperatureRisk.json error : ', error); |
|
87 |
+ }); |
|
88 |
+ } |
|
89 |
+ |
|
90 |
+ //배터리 부족 대상자(시니어) 수 조회 |
|
91 |
+ const [batteryCount, setbatteryCount] = React.useState(0); |
|
92 |
+ const batteryRiskCount = () => { |
|
93 |
+ fetch("/stats/agencyBatteryRisk.json", { |
|
94 |
+ method: "POST", |
|
95 |
+ headers: { |
|
96 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
97 |
+ }, |
|
98 |
+ body: JSON.stringify({ |
|
99 |
+ 'agency_id': state.loginUser['agency_id'], |
|
100 |
+ }), |
|
101 |
+ }).then((response) => response.json()).then((data) => { |
|
102 |
+ console.log("배터리 부족 대상자(시니어) 수 조회 : ", data); |
|
103 |
+ setbatteryCount(data); |
|
104 |
+ }).catch((error) => { |
|
105 |
+ console.log('batteryRiskCount() /stats/agencyBatteryRisk.json error : ', error); |
|
106 |
+ }); |
|
107 |
+ } |
|
108 |
+ |
|
109 |
+ //월별 방문 횟수 조회 |
|
110 |
+ const [visit, setVisit] = React.useState([]); |
|
111 |
+ const visitByMonthList = () => { |
|
112 |
+ fetch("/stats/visitByMonthList.json", { |
|
113 |
+ method: "POST", |
|
114 |
+ headers: { |
|
115 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
116 |
+ }, |
|
117 |
+ body: JSON.stringify({ |
|
118 |
+ 'agency_id': state.loginUser['agency_id'], |
|
119 |
+ }), |
|
120 |
+ }).then((response) => response.json()).then((data) => { |
|
121 |
+ console.log("월별 방문 횟수 조회 : ", data); |
|
122 |
+ setVisit(data); |
|
123 |
+ }).catch((error) => { |
|
124 |
+ console.log('visitByMonthList() /stats/visitByMonthList.json error : ', error); |
|
125 |
+ }); |
|
126 |
+ } |
|
127 |
+ |
|
128 |
+ //시행 기관별 약상자 사용 현황 |
|
129 |
+ const [equipmentUsage, setEquipmentUsage] = React.useState([]); |
|
130 |
+ const equipmentByAgency = () => { |
|
131 |
+ fetch("/stats/equipmentByAgency.json", { |
|
132 |
+ method: "POST", |
|
133 |
+ headers: { |
|
134 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
135 |
+ }, |
|
136 |
+ body: JSON.stringify({ |
|
137 |
+ 'agency_id': state.loginUser['agency_id'], |
|
138 |
+ }), |
|
139 |
+ }).then((response) => response.json()).then((data) => { |
|
140 |
+ console.log("시행기관별 약상자 사용 현황 : ", data); |
|
141 |
+ let newEquipmentList = updateList(data['agencyList'], data['equipmentList']); |
|
142 |
+ console.log("new equipment list", newEquipmentList); |
|
143 |
+ setEquipmentUsage(newEquipmentList); |
|
144 |
+ }).catch((error) => { |
|
145 |
+ console.log('equipmentByAgency() /stats/equipmentByAgency.json error : ', error); |
|
146 |
+ }); |
|
147 |
+ } |
|
148 |
+ |
|
149 |
+ //시행 기관별 대상자(시니어) 등록 현황 |
|
150 |
+ const [seniorEnroll, setSeniorEnroll] = React.useState([]); |
|
151 |
+ const seniorByAgency = () => { |
|
152 |
+ fetch("/stats/seniorByAgency.json", { |
|
153 |
+ method: "POST", |
|
154 |
+ headers: { |
|
155 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
156 |
+ }, |
|
157 |
+ body: JSON.stringify({ |
|
158 |
+ 'agency_id': state.loginUser['agency_id'], |
|
159 |
+ }), |
|
160 |
+ }).then((response) => response.json()).then((data) => { |
|
161 |
+ console.log("시행기관별 시니어 등록 현황 : ", data); |
|
162 |
+ let newSeniorList = updateList(data['agencyList'], data['seniorList']); |
|
163 |
+ console.log("new senior list", newSeniorList); |
|
164 |
+ setSeniorEnroll(newSeniorList); |
|
165 |
+ }).catch((error) => { |
|
166 |
+ console.log('seniorByAgency() /stats/seniorByAgency.json error : ', error); |
|
167 |
+ }); |
|
168 |
+ } |
|
169 |
+ |
|
170 |
+ function updateList(agencyList, countList) { |
|
171 |
+ |
|
172 |
+ const result = []; |
|
173 |
+ for (let i = 0; i < agencyList.length; i++) { |
|
174 |
+ const agency = agencyList[i]; |
|
175 |
+ let count = 0; |
|
176 |
+ for (let j = 0; j < countList.length; j++) { |
|
177 |
+ if (countList[j].agency_id === agency.agency_id) { |
|
178 |
+ count = countList[j].count; |
|
179 |
+ break; |
|
180 |
+ } |
|
181 |
+ } |
|
182 |
+ result.push({ agency_id: agency.agency_id, agency_name: agency.agency_name, count: count }); |
|
183 |
+ } |
|
184 |
+ return result; |
|
185 |
+ } |
|
206 | 186 |
|
207 | 187 |
|
208 |
- return ( |
|
209 |
- <main> |
|
210 |
- <div className="main-grid-government"> |
|
211 |
- <div className="sub-grid-government"> |
|
212 |
- <ul className="content-box statistics-govern" background="#f7acba"> |
|
213 |
- <li> |
|
214 |
- <p><ElderlyIcon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#bf0629", borderRadius: "50px" }} /></p> |
|
215 |
- <p>{cityName} 전체 대상자</p> |
|
216 |
- <p>{seniorCount}</p> |
|
217 |
- </li> |
|
218 |
- </ul> |
|
219 |
- <ul className="content-box statistics-govern" background="#8ef3d1"> |
|
220 |
- <li> |
|
221 |
- <p><MedicationIcon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#076143", borderRadius: "50px" }} /></p> |
|
222 |
- <p>{cityName} 미복약 위험 대상자</p> |
|
223 |
- <p>11</p> |
|
224 |
- </li> |
|
225 |
- </ul> |
|
226 |
- <ul className="content-box statistics-govern" background="#ebe7b9" > |
|
227 |
- <li> |
|
228 |
- <p><DeviceThermostatIcon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#f1de05", borderRadius: "50px" }} /></p> |
|
229 |
- <p>{cityName} 댁내 온도 위험 대상자</p> |
|
230 |
- <p>{temperatureCount}</p> |
|
231 |
- </li> |
|
232 |
- </ul> |
|
233 |
- <ul className="content-box statistics-govern" background="#5f9af3"> |
|
234 |
- <li> |
|
235 |
- <p><BatteryCharging20Icon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#5f9af3", borderRadius: "50px" }} /></p> |
|
236 |
- <p>{cityName} 배터리 부족 대상자 </p> |
|
237 |
- <p>{batteryCount}</p> |
|
238 |
- </li> |
|
239 |
- </ul> |
|
240 |
- </div> |
|
241 |
- <div className="content-box combine-left-government combine-bottom-government2 main-main"> |
|
242 |
- <div className="flex"> |
|
243 |
- <Title title={"지역별 케어 대상자 분포 현황"} explanation={"지역 선택 시 해당 지역의 대상자리스트가 보여집니다."} /> |
|
244 |
- </div> |
|
245 |
- <div style={{ height: 'calc(100% - 60px)' }}> |
|
246 |
- <MapContainer center={latLng(35.8713802646197, 128.601805491072)} zoom={13} scrollWheelZoom={true} style={{ height: '100%' }}> |
|
247 |
- <TileLayer |
|
248 |
- attribution='© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors' |
|
249 |
- url="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png" |
|
250 |
- /> |
|
251 |
- {/* <Marker position={[128.601405491072, 35.8913802646197]}> |
|
188 |
+ |
|
189 |
+ const iconHouse = new L.Icon({ |
|
190 |
+ iconUrl: '/client/resources/files/images/house.png', |
|
191 |
+ iconRetinaUrl: '/client/resources/files/images/house.png', |
|
192 |
+ iconSize: [20, 20], |
|
193 |
+ className: 'leaflet-background-radius-icon'//leaflet-div-icon |
|
194 |
+ }); |
|
195 |
+ |
|
196 |
+ React.useEffect(() => { |
|
197 |
+ seniorSelectList(); |
|
198 |
+ seniorCountTotal(); |
|
199 |
+ temperatureRiskCount(); |
|
200 |
+ batteryRiskCount(); |
|
201 |
+ visitByMonthList(); |
|
202 |
+ equipmentByAgency(); |
|
203 |
+ seniorByAgency(); |
|
204 |
+ }, []); |
|
205 |
+ |
|
206 |
+ |
|
207 |
+ return ( |
|
208 |
+ <main> |
|
209 |
+ <div className="main-grid-government"> |
|
210 |
+ <div className="sub-grid-government"> |
|
211 |
+ <ul className="content-box statistics-govern" background="#f7acba"> |
|
212 |
+ <li> |
|
213 |
+ <p><ElderlyIcon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#bf0629", borderRadius: "50px" }} /></p> |
|
214 |
+ <p>{cityName} 전체 대상자</p> |
|
215 |
+ <p>{seniorCount}</p> |
|
216 |
+ </li> |
|
217 |
+ </ul> |
|
218 |
+ <ul className="content-box statistics-govern" background="#8ef3d1"> |
|
219 |
+ <li> |
|
220 |
+ <p><MedicationIcon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#076143", borderRadius: "50px" }} /></p> |
|
221 |
+ <p>{cityName} 미복약 위험 대상자</p> |
|
222 |
+ <p>11</p> |
|
223 |
+ </li> |
|
224 |
+ </ul> |
|
225 |
+ <ul className="content-box statistics-govern" background="#ebe7b9" > |
|
226 |
+ <li> |
|
227 |
+ <p><DeviceThermostatIcon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#f1de05", borderRadius: "50px" }} /></p> |
|
228 |
+ <p>{cityName} 댁내 온도 위험 대상자</p> |
|
229 |
+ <p>{temperatureCount}</p> |
|
230 |
+ </li> |
|
231 |
+ </ul> |
|
232 |
+ <ul className="content-box statistics-govern" background="#5f9af3"> |
|
233 |
+ <li> |
|
234 |
+ <p><BatteryCharging20Icon sx={{ width: "50px", height: "50px", color: "#ffffff", background: "#5f9af3", borderRadius: "50px" }} /></p> |
|
235 |
+ <p>{cityName} 배터리 부족 대상자 </p> |
|
236 |
+ <p>{batteryCount}</p> |
|
237 |
+ </li> |
|
238 |
+ </ul> |
|
239 |
+ </div> |
|
240 |
+ <div className="content-box combine-left-government combine-bottom-government2 main-main"> |
|
241 |
+ <div className="flex"> |
|
242 |
+ <Title title={"지역별 케어 대상자 분포 현황"} explanation={"지역 선택 시 해당 지역의 대상자리스트가 보여집니다."} /> |
|
243 |
+ </div> |
|
244 |
+ <div style={{ height: 'calc(100% - 60px)' }}> |
|
245 |
+ <MapContainer center={latLng(35.8713802646197, 128.601805491072)} zoom={13} scrollWheelZoom={true} style={{ height: '100%' }}> |
|
246 |
+ <TileLayer |
|
247 |
+ attribution='© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors' |
|
248 |
+ url="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png" |
|
249 |
+ /> |
|
250 |
+ {/* <Marker position={[128.601405491072, 35.8913802646197]}> |
|
252 | 251 |
<Popup> |
253 | 252 |
A pretty CSS3 popup. <br /> Easily customizable. |
254 | 253 |
</Popup> |
255 | 254 |
</Marker> */} |
256 | 255 |
|
257 |
- <LayerGroup> |
|
258 |
- {senior.userList.map((item, idx) => { |
|
259 |
- return item['y'] != null && item['x'] != null ? ( |
|
260 |
- <Marker position={[item['y'], item['x']]} icon={iconHouse}> |
|
261 |
- <Popup> |
|
262 |
- <div> |
|
263 |
- {item['user_name']}({item['user_birth']} - {item['user_gender']}) |
|
264 |
- </div> |
|
265 |
- </Popup> |
|
266 |
- </Marker> |
|
267 |
- ): null |
|
268 |
- })} |
|
269 |
- </LayerGroup> |
|
270 |
- </MapContainer> |
|
271 |
- </div> |
|
272 |
- {/* <Map setCityName={setCityName} /> */} |
|
273 |
- </div> |
|
274 |
- <div className="content-box combine-all-government combine-bottom-government2"> |
|
275 |
- <div className="flex"> |
|
276 |
- <Title title={`${cityName} 월별 방문 횟수`} explanation={"최근 6개월간 방문 횟수의 변화를 확인할 수 있습니다."} /> |
|
277 |
- </div> |
|
278 |
- <RowChart_govern data={visit} /> |
|
279 |
- </div> |
|
280 |
- <div className="content-box combine-left-government2"> |
|
281 |
- <div className="flex"> |
|
282 |
- <Title title={`${cityName} 복용률 평균`} explanation={"해당 지역의 대상자 복용률이 그래프로 보여집니다."} /> |
|
283 |
- </div> |
|
284 |
- <Chart2_govern /> |
|
285 |
- </div> |
|
286 |
- <div className="content-box combine-right-government2"> |
|
287 |
- <div className="flex"> |
|
288 |
- <Title title={`기관별 대상자 등록 현황`} explanation={"약상자 사용자의 데이터 차트가 보여집니다."} /> |
|
289 |
- </div> |
|
290 |
- <Chart5 data={seniorEnroll} /> |
|
291 |
- </div> |
|
292 |
- <div className="content-box combine-right-government"> |
|
293 |
- <div className="flex"> |
|
294 |
- <Title title={`기관별 약상자 사용 현황`} explanation={""} /> |
|
295 |
- </div> |
|
296 |
- <Donut1_govern data={equipmentUsage} /> |
|
297 |
- </div> |
|
298 |
- </div> |
|
299 |
- </main> |
|
300 |
- ); |
|
256 |
+ <LayerGroup> |
|
257 |
+ {senior.userList.map((item, idx) => { |
|
258 |
+ return item['y'] != null && item['x'] != null ? ( |
|
259 |
+ <Marker position={[item['y'], item['x']]} icon={iconHouse}> |
|
260 |
+ <Popup> |
|
261 |
+ <div> |
|
262 |
+ {item['user_name']}({item['user_birth']} - {item['user_gender']}) |
|
263 |
+ </div> |
|
264 |
+ </Popup> |
|
265 |
+ </Marker> |
|
266 |
+ ) : null |
|
267 |
+ })} |
|
268 |
+ </LayerGroup> |
|
269 |
+ </MapContainer> |
|
270 |
+ </div> |
|
271 |
+ {/* <Map setCityName={setCityName} /> */} |
|
272 |
+ </div> |
|
273 |
+ <div className="content-box combine-all-government combine-bottom-government2"> |
|
274 |
+ <div className="flex"> |
|
275 |
+ <Title title={`${cityName} 월별 방문 횟수`} explanation={"최근 6개월간 방문 횟수의 변화를 확인할 수 있습니다."} /> |
|
276 |
+ </div> |
|
277 |
+ <RowChart_govern data={visit} /> |
|
278 |
+ </div> |
|
279 |
+ <div className="content-box combine-left-government2"> |
|
280 |
+ <div className="flex"> |
|
281 |
+ <Title title={`${cityName} 복용률 평균`} explanation={"해당 지역의 대상자 복용률이 그래프로 보여집니다."} /> |
|
282 |
+ </div> |
|
283 |
+ <Chart2_govern /> |
|
284 |
+ </div> |
|
285 |
+ <div className="content-box combine-right-government2"> |
|
286 |
+ <div className="flex"> |
|
287 |
+ <Title title={`보호사별 대상자 등록 현황`} explanation={"약상자 사용자의 데이터 차트가 보여집니다."} /> |
|
288 |
+ </div> |
|
289 |
+ <Chart5 data={seniorEnroll} /> |
|
290 |
+ </div> |
|
291 |
+ <div className="content-box combine-right-government"> |
|
292 |
+ <div className="flex"> |
|
293 |
+ <Title title={`보호사별 약상자 사용 현황`} explanation={""} /> |
|
294 |
+ </div> |
|
295 |
+ <Donut1_govern data={equipmentUsage} /> |
|
296 |
+ </div> |
|
297 |
+ </div> |
|
298 |
+ </main> |
|
299 |
+ ); |
|
301 | 300 |
} |
--- client/views/pages/main/Main_guardian.jsx
+++ client/views/pages/main/Main_guardian.jsx
... | ... | @@ -37,6 +37,8 @@ |
37 | 37 |
import percent_m_80 from '../../../resources/files/images/percent_m_80.png'; |
38 | 38 |
import percent_m_100 from '../../../resources/files/images/percent_m_100.png'; |
39 | 39 |
|
40 |
+import CommonUtil from "../../../resources/js/CommonUtil.js"; |
|
41 |
+ |
|
40 | 42 |
export default function Main_guardian() { |
41 | 43 |
const navigate = useNavigate(); |
42 | 44 |
const location = useLocation(); |
... | ... | @@ -45,79 +47,87 @@ |
45 | 47 |
const state = useSelector((state) => { return state }); |
46 | 48 |
//console.log('state.seniorList[state.currentSeniorIndex] : ', state.seniorList[state.currentSeniorIndex]); |
47 | 49 |
|
48 |
- const tableHead1 = ["", "", "", "", "", ""]; |
|
49 |
- const Key1 = ["morning", "morning2", "lunch", "lunch2", "dinner", "dinner2"]; |
|
50 |
- const content1 = [ |
|
51 |
- { |
|
52 |
- morning: "아침", |
|
53 |
- morning2: ( |
|
54 |
- <CheckCircleOutlineIcon sx={{ width: "3rem", height: "3rem", color: "#067943", borderRadius: "50px" }} /> |
|
55 |
- ), |
|
56 |
- lunch: "점심", |
|
57 |
- lunch2: ( |
|
58 |
- <CheckCircleOutlineIcon sx={{ width: "3rem", height: "3rem", color: "#067943", borderRadius: "50px" }} /> |
|
59 |
- ), |
|
60 |
- dinner: "저녁", |
|
61 |
- dinner2: ( |
|
62 |
- <CheckCircleOutlineIcon sx={{ width: "3rem", height: "3rem", color: "#067943", borderRadius: "50px" }} /> |
|
63 |
- ), |
|
64 |
- } |
|
65 |
- ]; |
|
66 |
- const tableHead2 = ["", "", "", "", "", ""]; |
|
67 |
- const Key2 = ["morning", "morning2", "lunch", "lunch2", "dinner", "dinner2"]; |
|
68 |
- const content2 = [ |
|
69 |
- { |
|
70 |
- morning: "아침", |
|
71 |
- morning2: ( |
|
72 |
- <ClearIcon sx={{ width: "3rem", height: "3rem", color: "#bf0629", borderRadius: "50px" }} /> |
|
73 |
- ), |
|
74 |
- lunch: "점심", |
|
75 |
- lunch2: ( |
|
76 |
- <ClearIcon sx={{ width: "3rem", height: "3rem", color: "#bf0629", borderRadius: "50px" }} /> |
|
77 |
- ), |
|
78 |
- dinner: "저녁", |
|
79 |
- dinner2: ( |
|
80 |
- <ClearIcon sx={{ width: "3rem", height: "3rem", color: "#bf0629", borderRadius: "50px" }} /> |
|
81 |
- ), |
|
82 |
- } |
|
83 |
- ]; |
|
84 |
- const tableHead3 = ["월", "화", "수", "목", "금", "토", "일"]; |
|
85 |
- const Key3 = ["mon", "tue", "wed", "thu", "fri", "sat", "sun"]; |
|
86 |
- const content3 = [ |
|
87 |
- { |
|
88 |
- mon: "24°C", |
|
89 |
- tue: "24°C", |
|
90 |
- wed: "24°C", |
|
91 |
- thu: "24°C", |
|
92 |
- fri: "24°C", |
|
93 |
- sat: "24°C", |
|
94 |
- sun: "24°C", |
|
95 |
- } |
|
96 |
- ]; |
|
50 |
+ // const tableHead1 = ["", "", "", "", "", ""]; |
|
51 |
+ // const Key1 = ["morning", "morning2", "lunch", "lunch2", "dinner", "dinner2"]; |
|
52 |
+ // const content1 = [ |
|
53 |
+ // { |
|
54 |
+ // morning: "아침", |
|
55 |
+ // morning2: ( |
|
56 |
+ // <CheckCircleOutlineIcon sx={{ width: "3rem", height: "3rem", color: "#067943", borderRadius: "50px" }} /> |
|
57 |
+ // ), |
|
58 |
+ // lunch: "점심", |
|
59 |
+ // lunch2: ( |
|
60 |
+ // <CheckCircleOutlineIcon sx={{ width: "3rem", height: "3rem", color: "#067943", borderRadius: "50px" }} /> |
|
61 |
+ // ), |
|
62 |
+ // dinner: "저녁", |
|
63 |
+ // dinner2: ( |
|
64 |
+ // <CheckCircleOutlineIcon sx={{ width: "3rem", height: "3rem", color: "#067943", borderRadius: "50px" }} /> |
|
65 |
+ // ), |
|
66 |
+ // } |
|
67 |
+ // ]; |
|
68 |
+ // const tableHead2 = ["", "", "", "", "", ""]; |
|
69 |
+ // const Key2 = ["morning", "morning2", "lunch", "lunch2", "dinner", "dinner2"]; |
|
70 |
+ // const content2 = [ |
|
71 |
+ // { |
|
72 |
+ // morning: "아침", |
|
73 |
+ // morning2: ( |
|
74 |
+ // <ClearIcon sx={{ width: "3rem", height: "3rem", color: "#bf0629", borderRadius: "50px" }} /> |
|
75 |
+ // ), |
|
76 |
+ // lunch: "점심", |
|
77 |
+ // lunch2: ( |
|
78 |
+ // <ClearIcon sx={{ width: "3rem", height: "3rem", color: "#bf0629", borderRadius: "50px" }} /> |
|
79 |
+ // ), |
|
80 |
+ // dinner: "저녁", |
|
81 |
+ // dinner2: ( |
|
82 |
+ // <ClearIcon sx={{ width: "3rem", height: "3rem", color: "#bf0629", borderRadius: "50px" }} /> |
|
83 |
+ // ), |
|
84 |
+ // } |
|
85 |
+ // ]; |
|
86 |
+ // const tableHead3 = ["월", "화", "수", "목", "금", "토", "일"]; |
|
87 |
+ // const Key3 = ["mon", "tue", "wed", "thu", "fri", "sat", "sun"]; |
|
88 |
+ // const content3 = [ |
|
89 |
+ // { |
|
90 |
+ // mon: "24°C", |
|
91 |
+ // tue: "24°C", |
|
92 |
+ // wed: "24°C", |
|
93 |
+ // thu: "24°C", |
|
94 |
+ // fri: "24°C", |
|
95 |
+ // sat: "24°C", |
|
96 |
+ // sun: "24°C", |
|
97 |
+ // } |
|
98 |
+ // ]; |
|
97 | 99 |
|
98 | 100 |
//검색 |
99 | 101 |
const searching = () => { |
100 |
- if (state['seniorList'] && state['seniorList'].length > 0) { |
|
101 |
- if (isMySenior) { |
|
102 |
- setSenior(state['seniorList'][isMySenior]['senior_id']); |
|
103 |
- } else if (state['seniorList'].length > 0) { |
|
104 |
- setSenior(state['seniorList'][0]['senior_id']); |
|
105 |
- } |
|
102 |
+ if (CommonUtil.isEmpty(state.loginUser) == false |
|
103 |
+ && state.loginUser['authority'] == 'ROLE_GUARDIAN' & isMySenior) { |
|
104 |
+ console.log('here!!', mySenior); |
|
105 |
+ setSenior(state['seniorList'][mySenior]); |
|
106 |
+ } else if (state['seniorList'] != null && state['seniorList'].length > 0) { |
|
107 |
+ setSenior(state['seniorList'][0]); |
|
106 | 108 |
} |
109 |
+ if (CommonUtil.isEmpty(state.loginUser) == false |
|
110 |
+ && state.loginUser['authority'] == 'ROLE_SENIOR') { |
|
111 |
+ setSenior({ ...senior }); |
|
112 |
+ seniorSelectOne(); |
|
113 |
+ } |
|
114 |
+ |
|
115 |
+ seniorMedicationSelectList(senior); |
|
116 |
+ seniorTemperatureSelectListByDay(senior); |
|
117 |
+ visitRecordSelectList(senior); |
|
107 | 118 |
} |
108 | 119 |
|
109 |
- const [isMySenior, setIsMySenior] = React.useState(true); |
|
120 |
+ const [mySenior, setMySenior] = React.useState(); |
|
121 |
+ const [isMySenior, setIsMySenior] = React.useState(false); |
|
110 | 122 |
React.useEffect(() => { |
111 | 123 |
searching(); |
112 |
- }, [isMySenior]) |
|
124 |
+ }, [mySenior, isMySenior]) |
|
113 | 125 |
|
114 | 126 |
//시니어 정보 |
115 | 127 |
const [senior, setSenior] = React.useState({ |
116 | 128 |
'user_id': state.loginUser['authority'] == 'ROLE_SENIOR' ? state.loginUser['user_id'] : null, |
117 | 129 |
'user_name': state.loginUser['authority'] == 'ROLE_SENIOR' ? state.loginUser['user_name'] : null, |
118 | 130 |
'senior_id': state.loginUser['authority'] == 'ROLE_SENIOR' ? state.loginUser['user_id'] : null, |
119 |
- |
|
120 |
- 'seniorMedicationList': [] |
|
121 | 131 |
}); |
122 | 132 |
|
123 | 133 |
//시니어 상세 조회 |
... | ... | @@ -136,6 +146,159 @@ |
136 | 146 |
}); |
137 | 147 |
}; |
138 | 148 |
|
149 |
+ const handleChangeSelect = (e) => { |
|
150 |
+ setMySenior(e.target.value); |
|
151 |
+ setIsMySenior(true); |
|
152 |
+ }; |
|
153 |
+ |
|
154 |
+ const SelectBox = () => { |
|
155 |
+ if (state['seniorList'].length > 1) { |
|
156 |
+ return ( |
|
157 |
+ <select className="margin-bottom2" onChange={handleChangeSelect} value={mySenior}> |
|
158 |
+ {state['seniorList'].map((item, idx) => { |
|
159 |
+ return ( |
|
160 |
+ <option key={item} value={idx}>{item['user_name']}</option> |
|
161 |
+ ) |
|
162 |
+ })} |
|
163 |
+ </select> |
|
164 |
+ ) |
|
165 |
+ } |
|
166 |
+ } |
|
167 |
+ |
|
168 |
+ |
|
169 |
+ //특정 대상자의 실제 복약 정보 |
|
170 |
+ const [seniorMedicationList, setSeniorMedicationList] = React.useState([]); |
|
171 |
+ const [showMedicationTimeCode, setShowMedicationTimeCode] = React.useState({}); |
|
172 |
+ //특정 대상자의 실제 복약 정보 목록 조회 |
|
173 |
+ const seniorMedicationSelectList = (seniorNum) => { |
|
174 |
+ fetch("/user/seniorMedicationSelectList.json", { |
|
175 |
+ method: "POST", |
|
176 |
+ headers: { |
|
177 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
178 |
+ }, |
|
179 |
+ body: JSON.stringify(seniorNum), |
|
180 |
+ }).then((response) => response.json()).then((data) => { |
|
181 |
+ console.log("seniorMedicationList data : ", data); |
|
182 |
+ setSeniorMedicationList(data); |
|
183 |
+ seniorMedicationSelectListByDay(data, seniorNum); |
|
184 |
+ }).catch((error) => { |
|
185 |
+ console.log('seniorMedicationSelectList() /user/seniorMedicationSelectList.json error : ', error); |
|
186 |
+ }); |
|
187 |
+ }; |
|
188 |
+ |
|
189 |
+ //특정 대상자의 일별, 복약시간별 복약 목록 |
|
190 |
+ const [seniorMedicationListByDay, setSeniorMedicationListByDay] = React.useState([]); |
|
191 |
+ const [stackChartData, setStackChartData] = React.useState([]); |
|
192 |
+ //특정 대상자의 일별, 복약시간별 복약 목록 조회 |
|
193 |
+ const seniorMedicationSelectListByDay = (seniorMedicationList, seniorNum) => { |
|
194 |
+ fetch("/user/seniorMedicationSelectListByDay.json", { |
|
195 |
+ method: "POST", |
|
196 |
+ headers: { |
|
197 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
198 |
+ }, |
|
199 |
+ body: JSON.stringify(seniorNum), |
|
200 |
+ }).then((response) => response.json()).then((data) => { |
|
201 |
+ console.log("seniorMedicationListByDay data : ", data); |
|
202 |
+ setSeniorMedicationListByDay(data); |
|
203 |
+ |
|
204 |
+ let showMedicationTimeCode = {}; |
|
205 |
+ for (let i = 0; i < seniorMedicationList.length; i++) { |
|
206 |
+ showMedicationTimeCode[seniorMedicationList[i]] = true; |
|
207 |
+ } |
|
208 |
+ setShowMedicationTimeCode(showMedicationTimeCode); |
|
209 |
+ console.log('showMedicationTimeCode : ', showMedicationTimeCode); |
|
210 |
+ |
|
211 |
+ if (CommonUtil.isEmpty(data) == false) { |
|
212 |
+ let _stackChartData = []; |
|
213 |
+ for (let i = 0; i < data.length; i++) { |
|
214 |
+ let sum = 0; // 실제 복약량 |
|
215 |
+ let counter = 0; // 복약해야하는 양 |
|
216 |
+ for (let j = 0; j < data[i]['medication_time_code_list'].length; j++) { |
|
217 |
+ if (CommonUtil.isEmpty(showMedicationTimeCode[data[i]['medication_time_code_list'][j]]) == false) { |
|
218 |
+ counter++; |
|
219 |
+ if (i > 0) { |
|
220 |
+ sum += data[i]['medication_time_code_count_list'][j]; |
|
221 |
+ } |
|
222 |
+ } else { |
|
223 |
+ continue; |
|
224 |
+ } |
|
225 |
+ } |
|
226 |
+ _stackChartData.push({ "xName": data[i]['medication_default_date'], "sum": sum, "total": counter }) |
|
227 |
+ } |
|
228 |
+ setStackChartData(_stackChartData); |
|
229 |
+ console.log('_stackChartData : ', _stackChartData); |
|
230 |
+ } |
|
231 |
+ |
|
232 |
+ }).catch((error) => { |
|
233 |
+ console.log('seniorMedicationSelectListByDay() /user/seniorMedicationSelectListByDay.json error : ', error); |
|
234 |
+ }); |
|
235 |
+ }; |
|
236 |
+ |
|
237 |
+ const seniorTemperatureList = ['02:00', '10:00', '14:00', '23:00'] |
|
238 |
+ |
|
239 |
+ //특정 대상자의 일별, 시간별 온도 목록 |
|
240 |
+ const [seniorTemperatureListByDay, setSeniorTemperatureListByDay] = React.useState([]); |
|
241 |
+ const [stackTemperatureData, setStackTemperatureData] = React.useState([]); |
|
242 |
+ //특정 대상자의 일별, 시간별 온도 목록 조회 |
|
243 |
+ const seniorTemperatureSelectListByDay = (seniorNum) => { |
|
244 |
+ fetch("/user/seniorTemperatureSelectListByDay.json", { |
|
245 |
+ method: "POST", |
|
246 |
+ headers: { |
|
247 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
248 |
+ }, |
|
249 |
+ body: JSON.stringify(seniorNum), |
|
250 |
+ }).then((response) => response.json()).then((data) => { |
|
251 |
+ console.log("seniorTemperatureListByDay data : ", data); |
|
252 |
+ setSeniorTemperatureListByDay(data); |
|
253 |
+ |
|
254 |
+ if (CommonUtil.isEmpty(data) == false) { |
|
255 |
+ let _stackTemperatureData = []; |
|
256 |
+ let chartData = {}; |
|
257 |
+ for (let i = 0; i < data.length; i++) { |
|
258 |
+ chartData = { |
|
259 |
+ xName: data[i]['temperature_date'], |
|
260 |
+ }; |
|
261 |
+ chartData['temperature'] = data[i]['temperature_data']; |
|
262 |
+ chartData['time'] = data[i]['temperature_time']; |
|
263 |
+ |
|
264 |
+ _stackTemperatureData.push(chartData); |
|
265 |
+ } |
|
266 |
+ setStackTemperatureData(_stackTemperatureData); |
|
267 |
+ console.log('_stackTemperatureData : ', _stackTemperatureData); |
|
268 |
+ } |
|
269 |
+ }).catch((error) => { |
|
270 |
+ console.log('seniorTemperatureSelectListByDay() /user/seniorTemperatureSelectListByDay.json error : ', error); |
|
271 |
+ }); |
|
272 |
+ }; |
|
273 |
+ |
|
274 |
+ //방문 기록 정보 |
|
275 |
+ const [visitRecordList, setVisitRecordList] = React.useState({}); |
|
276 |
+ //방문 기록 목록 조회 |
|
277 |
+ const visitRecordSelectList = (seniorNum) => { |
|
278 |
+ fetch("/welfare/visitRecordSelectList.json", { |
|
279 |
+ method: "POST", |
|
280 |
+ headers: { |
|
281 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
282 |
+ }, |
|
283 |
+ body: JSON.stringify(seniorNum), |
|
284 |
+ }).then((response) => response.json()).then((data) => { |
|
285 |
+ console.log("방문 기록 목록 조회 결과(건수) : ", data); |
|
286 |
+ let _stackVisitData = []; |
|
287 |
+ let chartData = {}; |
|
288 |
+ for (let i = 0; i < data['visitRecordList'].length; i++) { |
|
289 |
+ chartData = { |
|
290 |
+ xName: data['visitRecordList'][i]['visit_date'], |
|
291 |
+ }; |
|
292 |
+ chartData['reason'] = data['visitRecordList'][i]['visit_reason']; |
|
293 |
+ _stackVisitData.push(chartData); |
|
294 |
+ } |
|
295 |
+ setVisitRecordList(_stackVisitData); |
|
296 |
+ console.log('_stackVisitData : ', _stackVisitData); |
|
297 |
+ }).catch((error) => { |
|
298 |
+ console.log('visitRecordSelectList() /user/visitRecordSelectList.json error : ', error); |
|
299 |
+ }); |
|
300 |
+ } |
|
301 |
+ |
|
139 | 302 |
React.useEffect(() => { |
140 | 303 |
searching(); |
141 | 304 |
}, []); |
... | ... | @@ -143,97 +306,90 @@ |
143 | 306 |
return ( |
144 | 307 |
<> |
145 | 308 |
<main className="pink"> |
146 |
- {state['seniorList'].length > 1 ? ( |
|
147 |
- <select className="margin-bottom2" onChange={(value) => { setIsMySenior(value.target.value) }}> |
|
148 |
- {state['seniorList'].map((item, idx) => { |
|
149 |
- return ( |
|
150 |
- <option key={idx} value={idx}>{item['user_name']}</option> |
|
151 |
- ) |
|
152 |
- })} |
|
153 |
- </select>) : null} |
|
154 |
- |
|
309 |
+ <SelectBox /> |
|
155 | 310 |
<div className="flex-start main-guardian"><img src={Senior} alt="" /> |
156 | 311 |
<Title title={`${senior['user_name']} 어르신`} explanation={"방문, 복약, 온도, 배터리 현황을 확인하세요."} /> |
157 | 312 |
</div> |
158 |
- <div className="main-grid-guardian"> |
|
159 |
- <div className="combine-left combine-all-government battery-wrap "> |
|
160 |
- <div className="battery"> |
|
161 |
- <div className="flex-start"> |
|
162 |
- <img className="guardian-img" src={battery} alt="" /> |
|
163 |
- <div className="pc flex-start"><p>현재 스마트 약상자의 배터리가 <em className="red">28</em>% 입니다.</p><p className="red">※충전이 필요합니다.</p></div > |
|
164 |
- </div> |
|
165 |
- <div className="battery-img"> |
|
166 |
- {/* 0%일때 */} |
|
167 |
- <img src={zeropercent} alt="" /> |
|
168 |
- {/* 0~20%일때 */} |
|
169 |
- <img src={twentypercent} alt="" /> |
|
170 |
- {/* 20~40%일때 */} |
|
171 |
- <img src={fortypercent} alt="" className="show" /> |
|
172 |
- {/* 40~60%일때 */} |
|
173 |
- <img src={sixtytypercent} alt="" /> |
|
174 |
- {/* 60~80% 일때 */} |
|
175 |
- <img src={eightytypercent} alt="" /> |
|
176 |
- {/* 80~100%일때 */} |
|
177 |
- <img src={pullpercent} alt="" /> |
|
178 |
- </div> |
|
179 |
- </div> |
|
180 |
- <div className="battery-mobile"> |
|
181 |
- <div className="flex-start "><img className="guardian-img" src={battery} alt="" /><TitleSmall title={"배터리"} /><TitleSmall title={"28%"} /><p className="red">※충전이 필요합니다.</p></div > |
|
182 |
- <div className="battery-img"> |
|
183 |
- <img src={percent_m_0} alt="" /> |
|
184 |
- <img src={percent_m_20} alt="" /> |
|
185 |
- <img src={percent_m_40} alt="" className="show" /> |
|
186 |
- <img src={percent_m_60} alt="" /> |
|
187 |
- <img src={percent_m_80} alt="" /> |
|
188 |
- <img src={percent_m_100} alt="" /> |
|
189 |
- </div> |
|
190 |
- </div> |
|
191 |
- |
|
192 |
- </div> |
|
193 |
- <div className="statistics-guardian combine-right3"> |
|
194 |
- <div className="flex-start margin-bottom2"><img className="guardian-img" src={medicine} alt="" /><TitleSmall title={"복약체크"} explanation={"약을 잘 복용하고 계신지 체크해주세요."} /></div> |
|
195 |
- <ul className=""> |
|
196 |
- <li className="guardian-medicine smallbox"> |
|
197 |
- <DateDay /> |
|
198 |
- <ul className="flex"> |
|
199 |
- <li> |
|
200 |
- <img src={mornon} alt="" /> |
|
201 |
- <img src={mornoff} alt="" className="show" /> |
|
202 |
- <p className="medicine-title">아침</p> |
|
203 |
- </li> |
|
204 |
- <li> |
|
205 |
- <img src={lunchon} alt="" /> |
|
206 |
- <img src={lunchoff} alt="" className="show" /> |
|
207 |
- <p className="medicine-title">점심</p> |
|
208 |
- </li> |
|
209 |
- <li> |
|
210 |
- <img src={dinneron} alt="" /> |
|
211 |
- <img src={dinneroff} alt="" className="show" /> |
|
212 |
- <p className="medicine-title">저녁</p> |
|
213 |
- </li> |
|
214 |
- </ul> |
|
215 |
- </li> |
|
216 |
- </ul> |
|
217 |
- </div> |
|
218 |
- <div className=" statistics-guardian combine-right3"> |
|
219 |
- <div className="flex-start margin-bottom2 "><img className="guardian-img" src={temperature} alt="" /><TitleSmall title={"온도체크"} explanation={"댁내 온도가 적절한지 체크해보세요."} /></div> |
|
220 |
- <ul > |
|
221 |
- <li className="smallbox"> |
|
222 |
- <DateMonth /> |
|
223 |
- <Chart6 /> |
|
224 |
- </li> |
|
225 |
- </ul> |
|
226 |
- </div> |
|
227 |
- <div className=" statistics-guardian combine-left2 combine-middle-government"> |
|
228 |
- <div className="flex-start margin-bottom2 "><img className="guardian-img" src={calendarBig} alt="" /><TitleSmall title={"방문체크"} /></div> |
|
229 |
- <ul > |
|
230 |
- <li className="smallbox"> |
|
231 |
- <Calendar /> |
|
232 |
- </li> |
|
233 |
- </ul> |
|
234 |
- </div> |
|
235 |
- </div> |
|
313 |
+ <Calendar data={{ medication: stackChartData, temperature: stackTemperatureData, visit: visitRecordList }} /> |
|
236 | 314 |
</main> |
237 | 315 |
</> |
238 | 316 |
); |
239 | 317 |
} |
318 |
+ |
|
319 |
+{/* <main className="pink"> |
|
320 |
+ <SelectBox /> |
|
321 |
+ <div className="flex-start main-guardian"><img src={Senior} alt="" /> |
|
322 |
+ <Title title={`${senior['user_name']} 어르신`} explanation={"방문, 복약, 온도, 배터리 현황을 확인하세요."} /> |
|
323 |
+ </div> |
|
324 |
+ <div className="main-grid-guardian"> |
|
325 |
+ <div className="combine-left combine-all-government battery-wrap "> |
|
326 |
+ <div className="battery"> |
|
327 |
+ <div className="flex-start"> |
|
328 |
+ <img className="guardian-img" src={battery} alt="" /> |
|
329 |
+ <div className="pc flex-start"><p>현재 스마트 약상자의 배터리가 <em className="red">28</em>% 입니다.</p><p className="red">※충전이 필요합니다.</p></div > |
|
330 |
+ </div> |
|
331 |
+ <div className="battery-img"> |
|
332 |
+ <img src={zeropercent} alt="" /> |
|
333 |
+ <img src={twentypercent} alt="" /> |
|
334 |
+ <img src={fortypercent} alt="" className="show" /> |
|
335 |
+ <img src={sixtytypercent} alt="" /> |
|
336 |
+ <img src={eightytypercent} alt="" /> |
|
337 |
+ <img src={pullpercent} alt="" /> |
|
338 |
+ </div> |
|
339 |
+ </div> |
|
340 |
+ <div className="battery-mobile"> |
|
341 |
+ <div className="flex-start "><img className="guardian-img" src={battery} alt="" /><TitleSmall title={"배터리"} /><TitleSmall title={"28%"} /><p className="red">※충전이 필요합니다.</p></div > |
|
342 |
+ <div className="battery-img"> |
|
343 |
+ <img src={percent_m_0} alt="" /> |
|
344 |
+ <img src={percent_m_20} alt="" /> |
|
345 |
+ <img src={percent_m_40} alt="" className="show" /> |
|
346 |
+ <img src={percent_m_60} alt="" /> |
|
347 |
+ <img src={percent_m_80} alt="" /> |
|
348 |
+ <img src={percent_m_100} alt="" /> |
|
349 |
+ </div> |
|
350 |
+ </div> |
|
351 |
+ </div> |
|
352 |
+ <div className="statistics-guardian combine-right3"> |
|
353 |
+ <div className="flex-start margin-bottom2"><img className="guardian-img" src={medicine} alt="" /><TitleSmall title={"복약체크"} explanation={"약을 잘 복용하고 계신지 체크해주세요."} /></div> |
|
354 |
+ <ul className=""> |
|
355 |
+ <li className="guardian-medicine smallbox"> |
|
356 |
+ <DateDay /> |
|
357 |
+ <ul className="flex"> |
|
358 |
+ <li> |
|
359 |
+ <img src={mornon} alt="" /> |
|
360 |
+ <img src={mornoff} alt="" className="show" /> |
|
361 |
+ <p className="medicine-title">아침</p> |
|
362 |
+ </li> |
|
363 |
+ <li> |
|
364 |
+ <img src={lunchon} alt="" /> |
|
365 |
+ <img src={lunchoff} alt="" className="show" /> |
|
366 |
+ <p className="medicine-title">점심</p> |
|
367 |
+ </li> |
|
368 |
+ <li> |
|
369 |
+ <img src={dinneron} alt="" /> |
|
370 |
+ <img src={dinneroff} alt="" className="show" /> |
|
371 |
+ <p className="medicine-title">저녁</p> |
|
372 |
+ </li> |
|
373 |
+ </ul> |
|
374 |
+ </li> |
|
375 |
+ </ul> |
|
376 |
+ </div> |
|
377 |
+ <div className=" statistics-guardian combine-right3"> |
|
378 |
+ <div className="flex-start margin-bottom2 "><img className="guardian-img" src={temperature} alt="" /><TitleSmall title={"온도체크"} explanation={"댁내 온도가 적절한지 체크해보세요."} /></div> |
|
379 |
+ <ul > |
|
380 |
+ <li className="smallbox"> |
|
381 |
+ <DateMonth /> |
|
382 |
+ <Chart6 /> |
|
383 |
+ </li> |
|
384 |
+ </ul> |
|
385 |
+ </div> |
|
386 |
+ <div className=" statistics-guardian combine-left2 combine-middle-government"> |
|
387 |
+ <div className="flex-start margin-bottom2 "><img className="guardian-img" src={calendarBig} alt="" /><TitleSmall title={"방문체크"} /></div> |
|
388 |
+ <ul > |
|
389 |
+ <li className="smallbox"> |
|
390 |
+ <Calendar /> |
|
391 |
+ </li> |
|
392 |
+ </ul> |
|
393 |
+ </div> |
|
394 |
+ </div> |
|
395 |
+</main> */}(No newline at end of file) |
+++ client/views/pages/user_management/AgencyAdminSeniorSelect.jsx
... | ... | @@ -0,0 +1,372 @@ |
1 | +import React from "react"; | |
2 | +import { useNavigate, useLocation } from "react-router"; | |
3 | +import { useSelector } from "react-redux"; | |
4 | + | |
5 | +import SubTitle from "../../component/SubTitle.jsx"; | |
6 | +import Pagination from "../../component/Pagination.jsx"; | |
7 | +import House from "../../../resources/files/icon/house.png"; | |
8 | +import Arrow from "../../../resources/files/icon/arrow.png"; | |
9 | + | |
10 | +import Modal_SeniorInsert from "../../component/Modal_SeniorInsert.jsx"; | |
11 | + | |
12 | +import CommonUtil from "../../../resources/js/CommonUtil.js"; | |
13 | + | |
14 | +export default function AgencyAdminSeniorSelect() { | |
15 | + const navigate = useNavigate(); | |
16 | + const location = useLocation(); | |
17 | + | |
18 | + //대상자(시니어) 등록 모달 오픈 여부 | |
19 | + const [modalSeniorInsertIsOpen, setModalSeniorInsertIsOpen] = React.useState(false); | |
20 | + //대상자(시니어) 등록 모달 오픈 | |
21 | + const modalSeniorInsertOpen = () => { | |
22 | + setModalSeniorInsertIsOpen(true); | |
23 | + }; | |
24 | + //대상자(시니어) 등록 모달 닫기 | |
25 | + const modalSeniorInsertClose = () => { | |
26 | + setModalSeniorInsertIsOpen(false); | |
27 | + }; | |
28 | + | |
29 | + //전역 변수 저장 객체 | |
30 | + const state = useSelector((state) => { return state }); | |
31 | + | |
32 | + | |
33 | + | |
34 | + //시행기관 담당자 목록 | |
35 | + const [agent, setAgent] = React.useState({ | |
36 | + userList: [], userListCount: 0, search: { | |
37 | + 'government_id': state.loginUser['government_id'], | |
38 | + 'agency_id': state.loginUser['agency_id'], | |
39 | + 'authority': 'ROLE_AGENCY', | |
40 | + } | |
41 | + }); | |
42 | + //시행기관 담당자 목록 조회 | |
43 | + const agentSelectList = () => { | |
44 | + fetch("/user/userSelectList.json", { | |
45 | + method: "POST", | |
46 | + headers: { | |
47 | + 'Content-Type': 'application/json; charset=UTF-8' | |
48 | + }, | |
49 | + body: JSON.stringify(agent.search), | |
50 | + }).then((response) => response.json()).then((data) => { | |
51 | + data.search = agent.search; | |
52 | + console.log("시행기관 담당자 목록 조회 : ", data); | |
53 | + setAgent(data); | |
54 | + }).catch((error) => { | |
55 | + console.log('agentSelectList() /user/userSelectList.json error : ', error); | |
56 | + }); | |
57 | + } | |
58 | + | |
59 | + | |
60 | + | |
61 | + | |
62 | + //검색 변수 (초기화값) | |
63 | + const [search, setSearch] = React.useState({ | |
64 | + 'searchType': null, | |
65 | + 'searchText': null, | |
66 | + 'currentPage': 1, | |
67 | + 'perPage': 10, | |
68 | + }); | |
69 | + const searchingEnter = (key) => { | |
70 | + if (key == 'Enter') { | |
71 | + searching(); | |
72 | + } else { | |
73 | + return; | |
74 | + } | |
75 | + } | |
76 | + const searching = () => { | |
77 | + mySenior.search['searchType'] = search['searchType']; | |
78 | + mySenior.search['searchText'] = search['searchText']; | |
79 | + mySenior.search['agent_id'] = state.loginUser['user_id']; | |
80 | + setMySenior({ ...mySenior }); | |
81 | + | |
82 | + agencySenior.search['searchType'] = search['searchType']; | |
83 | + agencySenior.search['searchText'] = search['searchText']; | |
84 | + agencySenior.search['agency_id'] = state.loginUser['agency_id']; | |
85 | + setAgencySenior({ ...agencySenior }); | |
86 | + | |
87 | + mySeniorSelectList(1); | |
88 | + agencySeniorSelectList(1); | |
89 | + } | |
90 | + | |
91 | + | |
92 | + //보호사(간호사)의 돌봄 대상자(시니어) | |
93 | + const [mySenior, setMySenior] = React.useState({ seniorList: [], seniorListCount: 0, search: JSON.parse(JSON.stringify(search)) }); | |
94 | + //보호사(간호사)의 돌봄 대상자(시니어) 목록 조회 | |
95 | + const mySeniorSelectList = (currentPage) => { | |
96 | + mySenior.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; | |
97 | + | |
98 | + fetch("/user/seniorSelectList.json", { | |
99 | + method: "POST", | |
100 | + headers: { | |
101 | + 'Content-Type': 'application/json; charset=UTF-8' | |
102 | + }, | |
103 | + body: JSON.stringify(mySenior.search), | |
104 | + }).then((response) => response.json()).then((data) => { | |
105 | + data.search = mySenior.search; | |
106 | + console.log("보호사(간호사)의 돌봄 대상자(시니어) 목록 조회 : ", data); | |
107 | + setMySenior(data); | |
108 | + }).catch((error) => { | |
109 | + console.log('seniorSelectList() /user/seniorSelectList.json error : ', error); | |
110 | + }); | |
111 | + } | |
112 | + | |
113 | + | |
114 | + | |
115 | + //시행기관의 대상자(시니어) | |
116 | + const [agencySenior, setAgencySenior] = React.useState({ seniorList: [], seniorListCount: 0, search: JSON.parse(JSON.stringify(search)) }); | |
117 | + //시행기관의 대상자(시니어) 목록 조회 | |
118 | + const agencySeniorSelectList = (currentPage) => { | |
119 | + agencySenior.search.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; | |
120 | + | |
121 | + fetch("/user/seniorSelectList.json", { | |
122 | + method: "POST", | |
123 | + headers: { | |
124 | + 'Content-Type': 'application/json; charset=UTF-8' | |
125 | + }, | |
126 | + body: JSON.stringify(agencySenior.search), | |
127 | + }).then((response) => response.json()).then((data) => { | |
128 | + data.search = agencySenior.search; | |
129 | + console.log("시행기관의 대상자(시니어) 목록 조회 : ", data); | |
130 | + setAgencySenior(data); | |
131 | + }).catch((error) => { | |
132 | + console.log('agencySeniorSelectList() /user/seniorSelectList.json error : ', error); | |
133 | + }); | |
134 | + } | |
135 | + //보호사 선택 -> 시행기관의 대상자(시니어) 목록 조회 | |
136 | + const agentChange = (userId) => { | |
137 | + agencySenior.search['agent_id'] = userId; | |
138 | + setAgencySenior({ ...agencySenior }); | |
139 | + agencySeniorSelectList(1); | |
140 | + } | |
141 | + //선택한 보호사의 이름 | |
142 | + const getSearchAgentName = () => { | |
143 | + for (let i = 0; i < agent.userList.length; i++) { | |
144 | + if (agent.userList[i]['user_id'] == agencySenior.search['agent_id']) { | |
145 | + return agent.userList[i]['user_name']; | |
146 | + } | |
147 | + } | |
148 | + return '보호사'; | |
149 | + } | |
150 | + | |
151 | + | |
152 | + | |
153 | + //보호사의 대상자 등록 | |
154 | + const agentSeniorInsert = (senior, agentId) => { | |
155 | + if (confirm(`${senior['user_name']}님을 돌봄 대상자로 추가하시겠습니까?`) == false) { | |
156 | + return; | |
157 | + } else { | |
158 | + senior['agent_id'] = state.loginUser['user_id']; | |
159 | + } | |
160 | + fetch("/user/agentSeniorInsert.json", { | |
161 | + method: "POST", | |
162 | + headers: { | |
163 | + 'Content-Type': 'application/json; charset=UTF-8' | |
164 | + }, | |
165 | + body: JSON.stringify(senior), | |
166 | + }).then((response) => response.json()).then((data) => { | |
167 | + if (data > 0) { | |
168 | + setTabIndex(0); | |
169 | + search.searchText = ''; | |
170 | + search.searchType = ''; | |
171 | + searching(); | |
172 | + alert("추가완료"); | |
173 | + } else { | |
174 | + alert("추가에 실패하였습니다. 관리자에게 문의바랍니다."); | |
175 | + } | |
176 | + }).catch((error) => { | |
177 | + console.log('agencySeniorSelectList() /user/seniorSelectList.json error : ', error); | |
178 | + }); | |
179 | + } | |
180 | + | |
181 | + | |
182 | + React.useEffect(() => { | |
183 | + agentSelectList(); | |
184 | + searching(); | |
185 | + }, []); | |
186 | + | |
187 | + | |
188 | + //현재 탭 Index | |
189 | + const [tabIndex, setTabIndex] = React.useState(0); | |
190 | + //탭 초기화 | |
191 | + const tab = [{ | |
192 | + title: `우리기관 돌봄 대상자 (${agencySenior.seniorListCount})`, | |
193 | + content: ( | |
194 | + <div> | |
195 | + <div className="flex-align-start userauthoriylist gap5"> | |
196 | + <div className="left"> | |
197 | + <SubTitle explanation={"우리기관 보호사"} /> | |
198 | + <div style={{ fontSize: '16px', marginTop: '0px' }} className="category"> | |
199 | + <a className="active" onClick={() => { agentChange(null) }}> | |
200 | + {state.loginUser['government_name']} | |
201 | + </a> | |
202 | + <ul style={{ marginLeft: '15px' }}> | |
203 | + <li style={{ margin: '10px 0px' }}> | |
204 | + <span style={{ marginRight: '5px' }}><img src={House} alt="" /></span> | |
205 | + <a className="active" onClick={() => { agentChange(null) }}> | |
206 | + {state.loginUser['agency_name']} | |
207 | + </a> | |
208 | + <ul style={{ marginLeft: '15px' }}> | |
209 | + {agent.userList.map((user, idx) => { | |
210 | + return ( | |
211 | + <li style={{ margin: '10px 0px' }} key={idx}> | |
212 | + <span style={{ marginRight: '5px' }}><img src={Arrow} alt="" /></span> | |
213 | + <a className={user['user_id'] == agencySenior.search['agent_id'] ? "active" : ""} | |
214 | + onClick={() => { agentChange(user['user_id']) }}> | |
215 | + {user['user_name']} | |
216 | + {state.loginUser['user_id'] == user['user_id'] | |
217 | + ? '(나)' | |
218 | + : `(${user['user_id']})` | |
219 | + } | |
220 | + </a> | |
221 | + </li> | |
222 | + ) | |
223 | + })} | |
224 | + <li style={{ margin: '10px 0px' }}> | |
225 | + <span style={{ marginRight: '5px' }}><img src={Arrow} alt="" /></span> | |
226 | + <a className={'IS_NULL' == agencySenior.search['agent_id'] ? "active" : ""} | |
227 | + onClick={() => { agentChange('IS_NULL') }}> | |
228 | + 미배정 | |
229 | + </a> | |
230 | + </li> | |
231 | + </ul> | |
232 | + </li> | |
233 | + </ul> | |
234 | + </div> | |
235 | + </div> | |
236 | + <div className="right"> | |
237 | + <div className="flex equip-tab"> | |
238 | + <SubTitle explanation={CommonUtil.isEmpty(agencySenior.search['agent_id']) | |
239 | + ? '현재 기관에서 관리중인 돌봄 대상자 목록입니다.' | |
240 | + : (agencySenior.search['agent_id'] == 'IS_NULL' | |
241 | + ? '담당자가 배정되지 않은 돌봄 대상자 목록입니다.' | |
242 | + : `${getSearchAgentName()}님의 돌봄 대상자 목록입니다.` | |
243 | + ) | |
244 | + } /> | |
245 | + <div className="btn-wrap flex-end margin-bottom "> | |
246 | + {/* <button className={"btn-small gray-btn"} onClick={() => {modalEquipmentOpen()}}>등록</button> */} | |
247 | + </div> | |
248 | + </div> | |
249 | + <table className={"protector-user"}> | |
250 | + <thead> | |
251 | + <tr> | |
252 | + <th>No</th> | |
253 | + <th>소속기관명</th> | |
254 | + <th>이름</th> | |
255 | + <th>생년월일</th> | |
256 | + <th>성별</th> | |
257 | + <th>연락처</th> | |
258 | + <th>보호자</th> | |
259 | + <th>보호사</th> | |
260 | + <th>대상자관리</th> | |
261 | + </tr> | |
262 | + </thead> | |
263 | + <tbody> | |
264 | + {agencySenior.seniorList.map((item, idx) => { | |
265 | + return ( | |
266 | + <tr key={idx}> | |
267 | + <td data-label="No">{agencySenior.seniorListCount - idx - (agencySenior.search.currentPage - 1) * agencySenior.search.perPage}</td> | |
268 | + <td data-label="소속기관명">{item['agency_name']}</td> | |
269 | + <td data-label="이름">{item['user_name']}</td> | |
270 | + <td data-label="생년월일">{item['user_birth']}</td> | |
271 | + <td data-label="성별">{item['user_gender']}</td> | |
272 | + <td data-label="연락처">{item['user_phonenumber']}</td> | |
273 | + <td data-label="보호자"> | |
274 | + {CommonUtil.isEmpty(item['guardian_user_names']) | |
275 | + ? '없음' | |
276 | + : item['guardian_user_names'] | |
277 | + } | |
278 | + </td> | |
279 | + <td data-label="보호사"> | |
280 | + {CommonUtil.isEmpty(item['agent_user_names']) | |
281 | + ? <button className="btn-small gray-btn" onClick={() => agentSeniorInsert(item)}>내 돌봄 대상자로 추가</button> | |
282 | + : item['agent_user_names'] | |
283 | + } | |
284 | + </td> | |
285 | + <td data-label="대상자관리"> | |
286 | + <button className="btn-small gray-btn" onClick={() => { | |
287 | + navigate("/SeniorSelectOne", { | |
288 | + state: { | |
289 | + 'senior_id': item['senior_id'], | |
290 | + 'agency_id': item['agency_id'], | |
291 | + 'government_id': item['government_id'] | |
292 | + } | |
293 | + }) | |
294 | + }}>대상자관리</button> | |
295 | + </td> | |
296 | + </tr> | |
297 | + ) | |
298 | + })} | |
299 | + {CommonUtil.isEmpty(agencySenior.seniorList) ? | |
300 | + <tr> | |
301 | + <td colSpan={9}>조회된 데이터가 없습니다</td> | |
302 | + </tr> | |
303 | + : null} | |
304 | + </tbody> | |
305 | + </table> | |
306 | + <Pagination | |
307 | + currentPage={agencySenior.search.currentPage} | |
308 | + perPage={agencySenior.search.perPage} | |
309 | + totalCount={agencySenior.seniorListCount} | |
310 | + maxRange={5} | |
311 | + click={agencySeniorSelectList} | |
312 | + /> | |
313 | + </div> | |
314 | + </div> | |
315 | + </div> | |
316 | + ) | |
317 | + } | |
318 | + ]; | |
319 | + | |
320 | + | |
321 | + return ( | |
322 | + <main> | |
323 | + <Modal_SeniorInsert | |
324 | + open={modalSeniorInsertIsOpen} | |
325 | + close={modalSeniorInsertClose} | |
326 | + seniorInsertCallback={() => { | |
327 | + search.searchText = ''; | |
328 | + search.searchType = ''; | |
329 | + searching(); | |
330 | + modalSeniorInsertClose(); | |
331 | + }} | |
332 | + defaultAgentId={state.loginUser['user_id']} | |
333 | + defaultAgencyId={state.loginUser['agency_id']} | |
334 | + defaultGovernmentId={state.loginUser['government_id']} | |
335 | + /> | |
336 | + <div className="tab-container"> | |
337 | + | |
338 | + <ul className="tab-menu flex-start"> | |
339 | + {tab.map((item, idx) => { | |
340 | + return ( | |
341 | + <li onClick={() => setTabIndex(idx)} className={idx == tabIndex ? 'active' : null}> | |
342 | + {item.title} | |
343 | + </li> | |
344 | + ) | |
345 | + })} | |
346 | + </ul> | |
347 | + | |
348 | + <div className="content-wrap"> | |
349 | + <div className="search-management flex-start margin-bottom2"> | |
350 | + <select style={{ maxWidth: '150px' }} | |
351 | + onChange={(e) => { search.searchType = e.target.value; setSearch({ ...search }); }}> | |
352 | + <option value="" selected={CommonUtil.isEmpty(search.searchType)}>전체</option> | |
353 | + <option value="user_name" selected={search.searchType == 'user_name'}>이름</option> | |
354 | + <option value="user_id" selected={search.searchType == 'user_id'}>아이디</option> | |
355 | + <option value="user_phonenumber" selected={search.searchType == 'user_phonenumber'}>연락처</option> | |
356 | + </select> | |
357 | + <input type="text" className="senior-search" value={search.searchText} | |
358 | + onChange={(e) => { search.searchText = e.target.value; setSearch({ ...search }); }} | |
359 | + onKeyUp={(e) => searchingEnter(e.key)} /> | |
360 | + <button className="btn-small gray-btn" onClick={searching}>검색</button> | |
361 | + </div> | |
362 | + | |
363 | + <ul className="tab-content"> | |
364 | + <li> | |
365 | + {tab[tabIndex].content} | |
366 | + </li> | |
367 | + </ul> | |
368 | + </div> | |
369 | + </div> | |
370 | + </main> | |
371 | + ); | |
372 | +} |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?