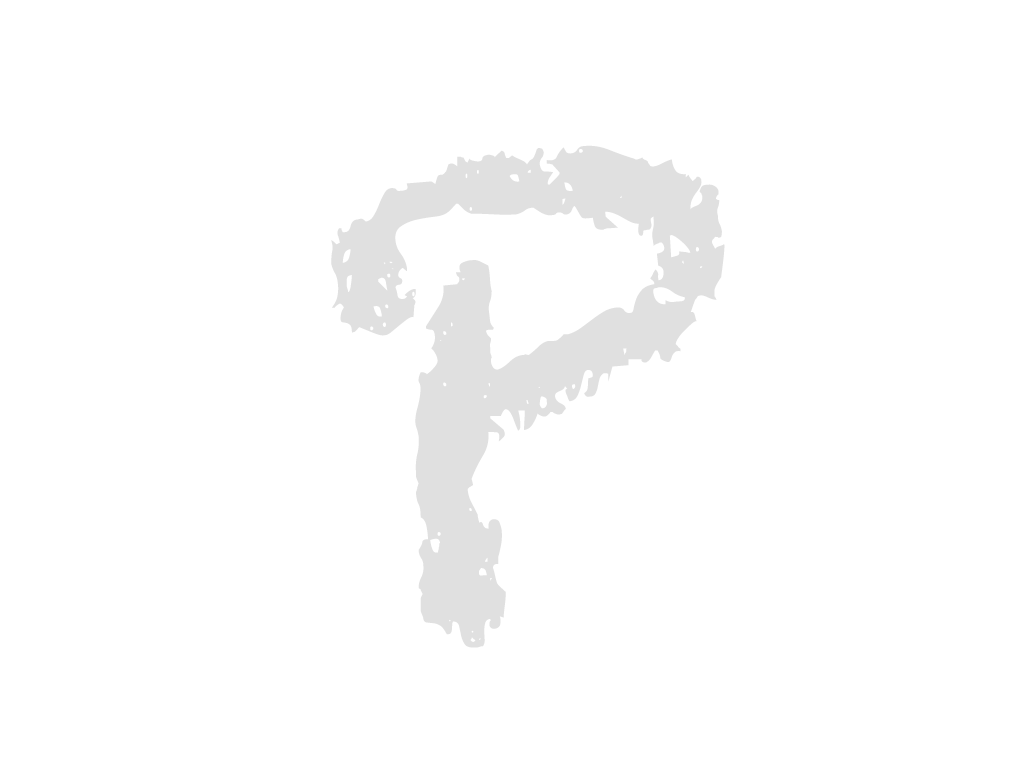
--- client/views/pages/equipment/EquipmentSelect.jsx
+++ client/views/pages/equipment/EquipmentSelect.jsx
... | ... | @@ -423,8 +423,10 @@ |
423 | 423 |
setStockEquipmentSearch({...stockEquipmentSearch}); |
424 | 424 |
setDeliveryEquipmentSearch({...deliveryEquipmentSearch}); |
425 | 425 |
|
426 |
+ newEquipmentSelectList(1); |
|
426 | 427 |
stockEquipmentSelectList(1); |
427 | 428 |
deliveryEquipmentSelectList(1); |
429 |
+ |
|
428 | 430 |
} |
429 | 431 |
const equipmentSearchingEnter = (key) => { |
430 | 432 |
if (key == 'Enter') { |
... | ... | @@ -432,6 +434,34 @@ |
432 | 434 |
} else { |
433 | 435 |
return; |
434 | 436 |
} |
437 |
+ } |
|
438 |
+ |
|
439 |
+ //재고 장비 검색 정보 |
|
440 |
+ const [newEquipmentSearch, setNewEquipmentSearch] = React.useState({ |
|
441 |
+ 'currentPage': 1, |
|
442 |
+ 'perPage': 10, |
|
443 |
+ }); |
|
444 |
+ //신규 장비 목록 |
|
445 |
+ const [newEquipment, setNewEquipment] = React.useState({equipmentList: [], equipmentListCount: 0}); |
|
446 |
+ //신규 장비 목록 조회 |
|
447 |
+ const newEquipmentSelectList = (currentPage) => { |
|
448 |
+ newEquipmentSearch.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; |
|
449 |
+ newEquipmentSearch['government_id'] = 'IS_NULL'; |
|
450 |
+ newEquipmentSearch['is_new_equipment'] = true; |
|
451 |
+ setNewEquipmentSearch({...newEquipmentSearch}); |
|
452 |
+ |
|
453 |
+ fetch("/equipment/equipmentSelectList.json", { |
|
454 |
+ method: "POST", |
|
455 |
+ headers: { |
|
456 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
457 |
+ }, |
|
458 |
+ body: JSON.stringify(newEquipmentSearch) |
|
459 |
+ }).then((response) => response.json()).then((data) => { |
|
460 |
+ console.log('newEquipmentSelectList response : ', data); |
|
461 |
+ setNewEquipment(data); |
|
462 |
+ }).catch((error) => { |
|
463 |
+ console.log('newEquipmentSelectList error : ', error); |
|
464 |
+ }); |
|
435 | 465 |
} |
436 | 466 |
|
437 | 467 |
//재고 장비 검색 정보 |
... | ... | @@ -445,6 +475,7 @@ |
445 | 475 |
const stockEquipmentSelectList = (currentPage) => { |
446 | 476 |
stockEquipmentSearch.currentPage = CommonUtil.isEmpty(currentPage) ? 1 : currentPage; |
447 | 477 |
stockEquipmentSearch['government_id'] = 'IS_NULL'; |
478 |
+ stockEquipmentSearch['is_new_equipment'] = false; |
|
448 | 479 |
const newStockEquipmentSearch = {...stockEquipmentSearch}; |
449 | 480 |
setStockEquipmentSearch(newStockEquipmentSearch); |
450 | 481 |
|
... | ... | @@ -504,6 +535,64 @@ |
504 | 535 |
const [tabIndex, setTabIndex] = React.useState(defaultTabIndex); |
505 | 536 |
//탭 초기화 |
506 | 537 |
const tab = [{ |
538 |
+ title: `신규 장비 (${newEquipment.equipmentListCount})`, |
|
539 |
+ content: ( |
|
540 |
+ <div> |
|
541 |
+ <div className="flex equip-tab"> |
|
542 |
+ <SubTitle explanation={"데이터 수집 정보로부터 신규 등록된 장비 목록입니다. ★장비 정보를 업데이트해주세요.★"} /> |
|
543 |
+ {/* <div className="btn-wrap flex-end margin-bottom "> |
|
544 |
+ {isEquipmentDelivery |
|
545 |
+ ? <> |
|
546 |
+ <button className={"btn-small green-btn"} onClick={modalDeliveryOpen}>장비납품</button> |
|
547 |
+ <button className={"btn-small red-btn"} onClick={() => {setIsEquipmentDelivery(false)}}>납품취소</button> |
|
548 |
+ </> |
|
549 |
+ : <> |
|
550 |
+ <button className={"btn-small gray-btn"} onClick={() => {setIsEquipmentDelivery(true)}}>납품장비선택</button> |
|
551 |
+ <button className={"btn-small gray-btn"} onClick={() => {modalEquipmentOpen()}}>등록</button> |
|
552 |
+ </> |
|
553 |
+ } |
|
554 |
+ </div> */} |
|
555 |
+ </div> |
|
556 |
+ <table class="caregiver-user protector-user"> |
|
557 |
+ <thead> |
|
558 |
+ <tr> |
|
559 |
+ <th>No</th> |
|
560 |
+ <th>시스템등록일시</th> |
|
561 |
+ <th>시리얼넘버</th> |
|
562 |
+ <th>상태</th> |
|
563 |
+ <th>관리</th> |
|
564 |
+ </tr> |
|
565 |
+ </thead> |
|
566 |
+ <tbody> |
|
567 |
+ {newEquipment.equipmentList.map((item, idx) => { return ( |
|
568 |
+ <tr> |
|
569 |
+ <td data-label="No">{newEquipment.equipmentListCount - idx - (newEquipmentSearch.currentPage - 1) * newEquipmentSearch.perPage}</td> |
|
570 |
+ <td data-label="시스템등록일시">{item['equipment_insert_datetime']}</td> |
|
571 |
+ <td data-label="시리얼넘버">{item['equipment_serial_number']}</td> |
|
572 |
+ <td data-label="상태">{equipmentStates[item['equipment_state']]}</td> |
|
573 |
+ <td data-label="관리"> |
|
574 |
+ <button className={"btn-small gray-btn"} onClick={() => modalEquipmentOpen(item)}>정보 수정</button> |
|
575 |
+ </td> |
|
576 |
+ </tr> |
|
577 |
+ )})} |
|
578 |
+ {CommonUtil.isEmpty(newEquipment.equipmentList) ? |
|
579 |
+ <tr> |
|
580 |
+ <td colSpan={8}>조회된 데이터가 없습니다</td> |
|
581 |
+ </tr> |
|
582 |
+ : null} |
|
583 |
+ |
|
584 |
+ </tbody> |
|
585 |
+ </table> |
|
586 |
+ <Pagination |
|
587 |
+ currentPage={newEquipmentSearch.currentPage} |
|
588 |
+ perPage={newEquipmentSearch.perPage} |
|
589 |
+ totalCount={newEquipment.equipmentListCount} |
|
590 |
+ maxRange={5} |
|
591 |
+ click={newEquipmentSelectList} |
|
592 |
+ /> |
|
593 |
+ </div> |
|
594 |
+ ) |
|
595 |
+ }, { |
|
507 | 596 |
title: `재고 장비 (${stockEquipment.equipmentListCount})`, |
508 | 597 |
content: ( |
509 | 598 |
<div> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?