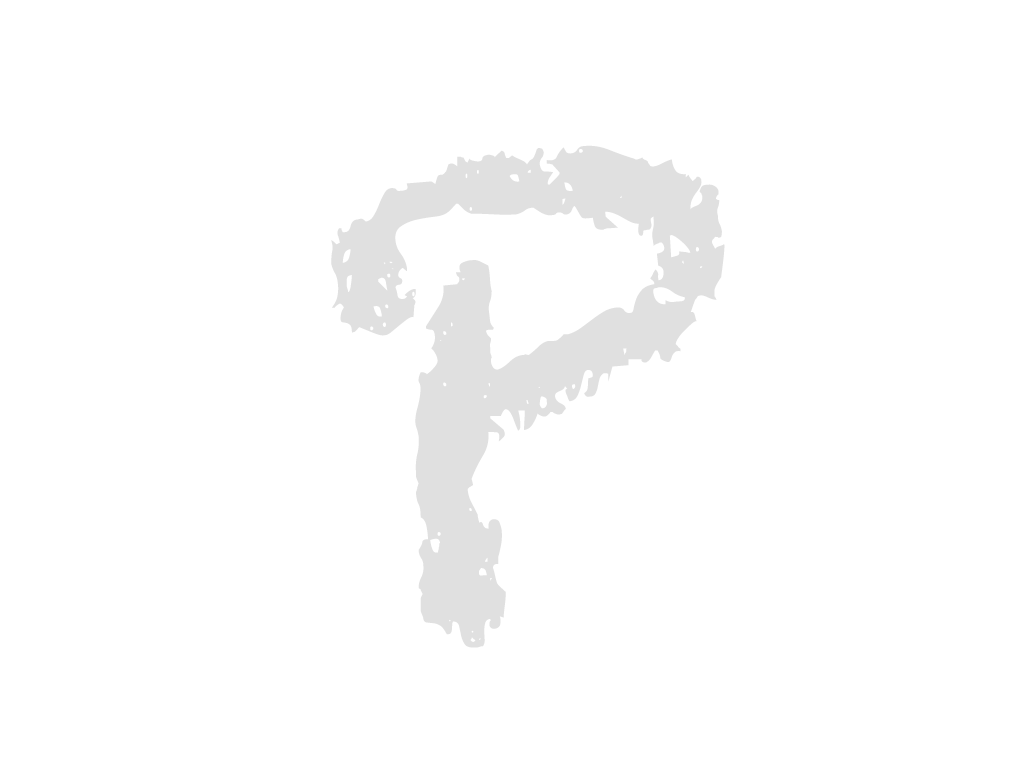
--- client/views/pages/equipment/EquipmentManagementSelect.jsx
+++ client/views/pages/equipment/EquipmentManagementSelect.jsx
... | ... | @@ -9,6 +9,11 @@ |
9 | 9 |
|
10 | 10 |
export default function EquipmentManagementSelect() { |
11 | 11 |
|
12 |
+ // 시스템 코드 - 장비 상태 |
|
13 |
+ const [equipmentStates, setEquipmentStates] = React.useState({}); |
|
14 |
+ // 시스템 코드 - 장비 대여 상태 |
|
15 |
+ const [equipmentRentalStates, setEquipmentRentalStates] = React.useState({}); |
|
16 |
+ |
|
12 | 17 |
const [modalOpen, setModalOpen] = React.useState(false); |
13 | 18 |
const openModal = () => { |
14 | 19 |
setModalOpen(true); |
... | ... | @@ -37,78 +42,97 @@ |
37 | 42 |
const navigate = useNavigate(); |
38 | 43 |
|
39 | 44 |
// 시스템 코드 - 장비 대여 상태 조회 |
40 |
- const equipmentRentalStatesSelect = () => { |
|
41 |
- // console.log('equipmentRentalStatesSelect Function Run'); |
|
42 |
- // axios.get('/common/systemCode/equipmentRentalStatesSelect.json') |
|
43 |
- // .then(function (response) { |
|
44 |
- // console.log('equipmentRentalStatesSelect : ', response.data); |
|
45 |
- // }).catch(function (error) { |
|
46 |
- // console.log("equipmentRentalStatesSelect - error : ", error); |
|
47 |
- // }); |
|
45 |
+ const equipmentStatesSelect = () => { |
|
46 |
+ console.log('equipmentStatesSelect Function Run'); |
|
48 | 47 |
|
49 |
- // fetch('/common/systemCode/equipmentRentalStatesSelect.json', { |
|
50 |
- // method: "GET", |
|
51 |
- // }) |
|
52 |
- // .then((response) => response.json()) |
|
53 |
- // .then((data) => { |
|
54 |
- // console.log('equipmentRentalStatesSelect response : ', data); |
|
55 |
- // }).catch((error) => { |
|
56 |
- // console.log('equipmentRentalStatesSelect error : ', error); |
|
57 |
- // }); |
|
58 |
- |
|
59 |
- // fetch('/common/systemCode/equipmentRentalStatesSelect.json', { |
|
60 |
- // // headers: { |
|
61 |
- // // Accept: "application / json", |
|
62 |
- // // }, |
|
63 |
- // method: "GET", |
|
64 |
- // }) |
|
65 |
- // .then((response) => response.json()) |
|
66 |
- // .then((data) => console.log(data)); |
|
67 |
- |
|
68 |
- // fetch('https://dummyjson.com/products/1') |
|
69 |
- // .then(res => res.json()) |
|
70 |
- // .then(json => console.log(json)) |
|
48 |
+ //fetch post |
|
49 |
+ fetch("/common/systemCode/equipmentStatesSelect.json", { |
|
50 |
+ method: "POST", |
|
51 |
+ headers: { |
|
52 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
53 |
+ }, |
|
54 |
+ body: JSON.stringify({}) |
|
55 |
+ }).then((response) => response.json()).then((data) => { |
|
56 |
+ console.log('equipmentStatesSelect response : ', data); |
|
57 |
+ setEquipmentStates(data); |
|
58 |
+ }).catch((error) => { |
|
59 |
+ console.log('equipmentStatesSelect error : ', error); |
|
60 |
+ }); |
|
71 | 61 |
} |
72 | 62 |
|
73 |
- // fetch("https://jsonplaceholder.typicode.com/posts/1") |
|
74 |
- // .then((response) => response.json()) |
|
75 |
- // .then((data) => console.log(data)) |
|
63 |
+ // 시스템 코드 - 장비 대여 상태 조회 |
|
64 |
+ const equipmentRentalStatesSelect = () => { |
|
65 |
+ console.log('equipmentRentalStatesSelect Function Run'); |
|
76 | 66 |
|
77 |
- // fetch("https://jsonplaceholder.typicode.com/posts/1", { |
|
78 |
- // method : "POST", |
|
79 |
- // headers : {"Content-Type": "application/json",}, |
|
80 |
- // body: JSON.stringify({ |
|
81 |
- // title: "Test", |
|
82 |
- // body: "I am testing!", |
|
83 |
- // userId: 1, |
|
84 |
- // }), |
|
85 |
- // }) |
|
86 |
- // .then((response) => response.json()) |
|
87 |
- // .then((json) => console.log(json)) |
|
67 |
+ //fetch post |
|
68 |
+ fetch("/common/systemCode/equipmentRentalStatesSelect.json", { |
|
69 |
+ method: "POST", |
|
70 |
+ headers: { |
|
71 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
72 |
+ }, |
|
73 |
+ body: JSON.stringify({}) |
|
74 |
+ }).then((response) => response.json()).then((data) => { |
|
75 |
+ console.log('equipmentRentalStatesSelect response : ', data); |
|
76 |
+ setEquipmentRentalStates(data); |
|
77 |
+ }).catch((error) => { |
|
78 |
+ console.log('equipmentRentalStatesSelect error : ', error); |
|
79 |
+ }); |
|
88 | 80 |
|
89 |
- // 대상자 목록 조회 |
|
90 |
- const seniorSelectList = () => { |
|
81 |
+ //fetch get |
|
82 |
+ /* fetch('/common/systemCode/equipmentRentalStatesSelect.json') |
|
83 |
+ .then((response) => response.json()).then((data) => { |
|
84 |
+ console.log('equipmentRentalStatesSelect response : ', data); |
|
85 |
+ }).catch((error) => { |
|
86 |
+ console.log('equipmentRentalStatesSelect error : ', error); |
|
87 |
+ }); */ |
|
88 |
+ |
|
89 |
+ //axios get |
|
90 |
+ /* axios.get('/common/systemCode/equipmentRentalStatesSelect.json') |
|
91 |
+ .then(function (response) { |
|
92 |
+ console.log('equipmentRentalStatesSelect : ', response.data); |
|
93 |
+ }).catch(function (error) { |
|
94 |
+ console.log("equipmentRentalStatesSelect - error : ", error); |
|
95 |
+ }); */ |
|
96 |
+ |
|
97 |
+ //axios post |
|
98 |
+ /* axios({ |
|
99 |
+ url: '/common/systemCode/equipmentRentalStatesSelect.json', |
|
100 |
+ method: 'post', |
|
101 |
+ headers: { |
|
102 |
+ 'Content-Type': "application/json; charset=UTF-8", |
|
103 |
+ }, |
|
104 |
+ data: {} |
|
105 |
+ }).then(function (response) { |
|
106 |
+ console.log("equipmentRentalStatesSelect - response : ", response.data); |
|
107 |
+ }).catch(function (error) { |
|
108 |
+ console.log("equipmentRentalStatesSelect - error : ", error); |
|
109 |
+ }); */ |
|
110 |
+ } |
|
111 |
+ |
|
112 |
+ // 장비 비매칭 대상자 목록 조회 |
|
113 |
+ const equipmentNotMatchSeniorSelectList = () => { |
|
91 | 114 |
console.log('seniorSelectList Function Run'); |
92 |
- fetch("/user/userSeleteList.json", { |
|
115 |
+ fetch("/user/equipmentMatchStateSelectListBySenior.json", { |
|
93 | 116 |
method: "POST", |
94 | 117 |
headers: { |
95 | 118 |
'Content-Type': 'application/json; charset=UTF-8' |
96 | 119 |
}, |
97 | 120 |
body: JSON.stringify({ |
98 |
- user_code: 'SENIOR01' |
|
121 |
+ agency_id: 'AGENCY01', |
|
122 |
+ matchState: false |
|
99 | 123 |
}) |
100 | 124 |
}).then((response) => response.json()).then((data) => { |
101 |
- console.log('seniorSelectList response : ', data); |
|
102 |
- setSeniorList(data); |
|
125 |
+ console.log('equipmentNotMatchSeniorSelectList response : ', data); |
|
126 |
+ setEquipmentNotMatchSeniorList(data); |
|
103 | 127 |
}).catch((error) => { |
104 |
- console.log('seniorSelectList error : ', error); |
|
128 |
+ console.log('equipmentNotMatchSeniorSelectList error : ', error); |
|
105 | 129 |
}); |
106 | 130 |
} |
107 | 131 |
|
108 | 132 |
// 담당자 목록 조회 |
109 | 133 |
const workerSelectList = () => { |
110 | 134 |
console.log('workerSelectList Function Run'); |
111 |
- fetch("/user/userSeleteList.json", { |
|
135 |
+ fetch("/user/selectUserList.json", { |
|
112 | 136 |
method: "POST", |
113 | 137 |
headers: { |
114 | 138 |
'Content-Type': 'application/json; charset=UTF-8' |
... | ... | @@ -257,7 +281,7 @@ |
257 | 281 |
/********************************** 시행기관 장비 (시작) **********************************/ |
258 | 282 |
//로그 확인 |
259 | 283 |
const logCheck = () => { |
260 |
- console.log('seniorList - change: ', seniorList); |
|
284 |
+ console.log('equipmentNotMatchSeniorList - change: ', equipmentNotMatchSeniorList); |
|
261 | 285 |
}; |
262 | 286 |
|
263 | 287 |
// 시행기관 전체 장비 목록 |
... | ... | @@ -273,8 +297,8 @@ |
273 | 297 |
// 대상자 장비 반납 모달창 |
274 | 298 |
const [seniorMatchReturnModal, setSeniorMatchReturnModal] = React.useState(false); |
275 | 299 |
|
276 |
- // 대상자 목록 |
|
277 |
- const [seniorList, setSeniorList] = React.useState([]); |
|
300 |
+ // 장비 비매칭 대상자 목록 |
|
301 |
+ const [equipmentNotMatchSeniorList, setEquipmentNotMatchSeniorList] = React.useState([]); |
|
278 | 302 |
// 담당자 목록 |
279 | 303 |
const [workerList, setWorkerList] = React.useState([]); |
280 | 304 |
|
... | ... | @@ -436,14 +460,14 @@ |
436 | 460 |
|
437 | 461 |
// 대상자 아이디 유효성 검사 |
438 | 462 |
let check = 0; |
439 |
- for (let i = 0; i < seniorList.length; i++) { |
|
440 |
- // 입력한 데이터가 대상자 목록에 있을 경우 |
|
441 |
- if (seniorList[i]['user_id'] == seniorEquipment['senior_id']) { |
|
442 |
- console.log('success: ', seniorList[i]['user_id']); |
|
463 |
+ for (let i = 0; i < equipmentNotMatchSeniorList.length; i++) { |
|
464 |
+ // 입력한 대상자 아이디가 대상자 목록에 있을 경우 |
|
465 |
+ if (equipmentNotMatchSeniorList[i]['senior_id'] == seniorEquipment['senior_id']) { |
|
466 |
+ console.log('success: ', equipmentNotMatchSeniorList[i]['senior_id']); |
|
443 | 467 |
// 원본 데이터 복사 및 수정 |
444 | 468 |
let data = { |
445 | 469 |
...seniorEquipment, |
446 |
- user_name: seniorList[i]['user_name'] |
|
470 |
+ user_name: equipmentNotMatchSeniorList[i]['user_name'], |
|
447 | 471 |
} |
448 | 472 |
// 복사 데이터를 원본 데이터에 덮어쓰기 |
449 | 473 |
setSeniorEquipment(data); |
... | ... | @@ -451,12 +475,24 @@ |
451 | 475 |
check = 1; |
452 | 476 |
} |
453 | 477 |
} |
454 |
- // 입력한 데이터가 대상자 목록에 없을 경우 |
|
478 |
+ // 입력한 대상자 아이디가 대상자 목록에 없을 경우 |
|
455 | 479 |
if (check != 1) { |
456 |
- console.log('fail: ', seniorList, seniorEquipment['senior_id']); |
|
480 |
+ console.log('fail: ', equipmentNotMatchSeniorList, seniorEquipment['senior_id']); |
|
457 | 481 |
alert('존재하지 않는 대상자입니다.'); |
458 | 482 |
return; |
459 | 483 |
} |
484 |
+ |
|
485 |
+ // 대여일 유효성 검사 |
|
486 |
+ /* if(seniorEquipment['equipment_rental_start_date'] == null) { |
|
487 |
+ alert('대여일을 입력해 주세요'); |
|
488 |
+ return; |
|
489 |
+ } */ |
|
490 |
+ |
|
491 |
+ // 반납 예정일 유효성 검사 |
|
492 |
+ /* if(seniorEquipment['equipment_rental_end_date'] == null) { |
|
493 |
+ alert('반납 예정일 입력해 주세요'); |
|
494 |
+ return; |
|
495 |
+ } */ |
|
460 | 496 |
|
461 | 497 |
fetch("/equipment/seniorEquipmentInsert.json", { |
462 | 498 |
method: "POST", |
... | ... | @@ -468,11 +504,14 @@ |
468 | 504 |
console.log('seniorEquipmentInsert response : ', data); |
469 | 505 |
// 대상자 장비 데이터 초기화 |
470 | 506 |
setSeniorEquipment({}); |
471 |
- |
|
507 |
+ //장비 비매칭 대상자 목록 조회 |
|
508 |
+ equipmentNotMatchSeniorSelectList(); |
|
472 | 509 |
// 장비 목록 조회 |
473 | 510 |
agencyEquipmentSelectList(); |
474 | 511 |
agencySeniorEquipmentSelectList(); |
475 | 512 |
agencyStockEquipmentSelectList(); |
513 |
+ // 모달창 닫기 |
|
514 |
+ setSeniorMatchInsertModal(false); |
|
476 | 515 |
|
477 | 516 |
alert('등록이 완료됐습니다.'); |
478 | 517 |
}).catch((error) => { |
... | ... | @@ -488,18 +527,24 @@ |
488 | 527 |
// 담당자 아이디 유효성 검사 |
489 | 528 |
let check = 0; |
490 | 529 |
for (let i = 0; i < workerList.length; i++) { |
491 |
- // 입력한 데이터가 담당자 목록에 있을 경우 |
|
530 |
+ // 입력한 담당자 아이디가 담당자 목록에 있을 경우 |
|
492 | 531 |
if (workerList[i]['user_id'] == seniorEquipment['return_user_id']) { |
493 | 532 |
console.log('success: ', workerList[i]['user_id']); |
494 | 533 |
check = 1; |
495 | 534 |
} |
496 | 535 |
} |
497 |
- // 입력한 데이터가 담당자 목록에 없을 경우 |
|
536 |
+ // 입력한 담당자 아이디가 담당자 목록에 없을 경우 |
|
498 | 537 |
if (check != 1) { |
499 | 538 |
console.log('fail: ', workerList, seniorEquipment['return_user_id']); |
500 | 539 |
alert('존재하지 않는 담당자입니다.'); |
501 | 540 |
return; |
502 | 541 |
} |
542 |
+ |
|
543 |
+ // 반납 예정일 유효성 검사 |
|
544 |
+ /* if(seniorEquipment['equipment_rental_return_date'] == null) { |
|
545 |
+ alert('반납일 입력해 주세요'); |
|
546 |
+ return; |
|
547 |
+ } */ |
|
503 | 548 |
|
504 | 549 |
fetch("/equipment/seniorEquipmentReturn.json", { |
505 | 550 |
method: "POST", |
... | ... | @@ -511,11 +556,14 @@ |
511 | 556 |
console.log('seniorEquipmentReturn response : ', data); |
512 | 557 |
// 대상자 장비 데이터 초기화 |
513 | 558 |
setSeniorEquipment({}); |
514 |
- |
|
559 |
+ //장비 비매칭 대상자 목록 조회 |
|
560 |
+ equipmentNotMatchSeniorSelectList(); |
|
515 | 561 |
// 장비 목록 조회 |
516 | 562 |
agencyEquipmentSelectList(); |
517 | 563 |
agencySeniorEquipmentSelectList(); |
518 | 564 |
agencyStockEquipmentSelectList(); |
565 |
+ // 모달창 닫기 |
|
566 |
+ setSeniorMatchReturnModal(false); |
|
519 | 567 |
|
520 | 568 |
alert('반납이 완료됐습니다.'); |
521 | 569 |
}).catch((error) => { |
... | ... | @@ -532,6 +580,7 @@ |
532 | 580 |
"시리얼 넘버", |
533 | 581 |
"기기 상태", |
534 | 582 |
"대여 상태", |
583 |
+ "동일 기기 매칭 순번", |
|
535 | 584 |
"대상자 관리", |
536 | 585 |
]; |
537 | 586 |
const key11 = [ |
... | ... | @@ -550,6 +599,7 @@ |
550 | 599 |
"대상자", |
551 | 600 |
"대여일", |
552 | 601 |
"반납예정일", |
602 |
+ "동일 기기 매칭 순번", |
|
553 | 603 |
"대상자 관리", |
554 | 604 |
]; |
555 | 605 |
const key22 = [ |
... | ... | @@ -559,6 +609,7 @@ |
559 | 609 |
"senior_name", |
560 | 610 |
"equipment_rental_start_date", |
561 | 611 |
"equipment_rental_end_date", |
612 |
+ "equipment_match_idx", |
|
562 | 613 |
]; |
563 | 614 |
|
564 | 615 |
// 시행기관 재고 장비 thead, key |
... | ... | @@ -592,8 +643,9 @@ |
592 | 643 |
|
593 | 644 |
// 마운트 시 실행 함수 |
594 | 645 |
React.useEffect(() => { |
646 |
+ equipmentStatesSelect(); |
|
595 | 647 |
equipmentRentalStatesSelect(); |
596 |
- seniorSelectList(); |
|
648 |
+ equipmentNotMatchSeniorSelectList(); |
|
597 | 649 |
workerSelectList(); |
598 | 650 |
|
599 | 651 |
//관리자 |
... | ... | @@ -756,12 +808,30 @@ |
756 | 808 |
return ( |
757 | 809 |
<tr key={index}> |
758 | 810 |
<td>{agencyEquipmentList.length - index}</td> |
759 |
- {key11.map((kes) => { |
|
811 |
+ {/* {key11.map((kes) => { |
|
812 |
+ if(kes == "equipment_rental_state") { |
|
813 |
+ for(let i = 0; i < Object.keys(equipmentRentalStates).length; i++) { |
|
814 |
+ if(item[kes].toString().toUpperCase() == Object.keys(equipmentRentalStates)[i]) { |
|
815 |
+ item[kes] = Object.values(equipmentRentalStates)[i]; |
|
816 |
+ } |
|
817 |
+ } |
|
818 |
+ } |
|
760 | 819 |
return <td>{item[kes]}</td> |
820 |
+ })} */} |
|
821 |
+ <td>{item['equipment_name']}</td> |
|
822 |
+ <td>{item['equipment_serial_number']}</td> |
|
823 |
+ {Object.keys(equipmentStates).map((key, idx) => { |
|
824 |
+ if(item['equipment_state'] == key) |
|
825 |
+ return <td>{Object.values(equipmentStates)[idx]}</td> |
|
761 | 826 |
})} |
827 |
+ {Object.keys(equipmentRentalStates).map((key, idx) => { |
|
828 |
+ if(item['equipment_rental_state'] == key) |
|
829 |
+ return <td>{Object.values(equipmentRentalStates)[idx]}</td> |
|
830 |
+ })} |
|
831 |
+ <td>{item['equipment_match_idx']}</td> |
|
762 | 832 |
<td> |
763 | 833 |
{ |
764 |
- item['equipment_rental_state'] == false |
|
834 |
+ item['equipment_rental_state'] == 'RENTABLE' |
|
765 | 835 |
? <Button |
766 | 836 |
className={"btn-small green-btn"} |
767 | 837 |
btnName={"등록"} |
... | ... | @@ -943,9 +1013,9 @@ |
943 | 1013 |
<td> |
944 | 1014 |
<input type="text" list="senior_list" placeholder="대상자를 입력해 주세요" onChange={seniorEquipmentSeniorInsert} /> |
945 | 1015 |
<datalist id="senior_list"> |
946 |
- {seniorList.map((item) => { |
|
947 |
- // return <option value={item['user_name'] + " (" + item['user_id'] + ")"}></option> |
|
948 |
- return <option value={item['user_id']}>{item['user_name']}</option> |
|
1016 |
+ {equipmentNotMatchSeniorList.map((item) => { |
|
1017 |
+ // return <option value={item['user_name'] + " (" + item['senior_id'] + ")"}></option> |
|
1018 |
+ return <option value={item['senior_id']}>{item['user_name']}</option> |
|
949 | 1019 |
|
950 | 1020 |
})} |
951 | 1021 |
</datalist> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?