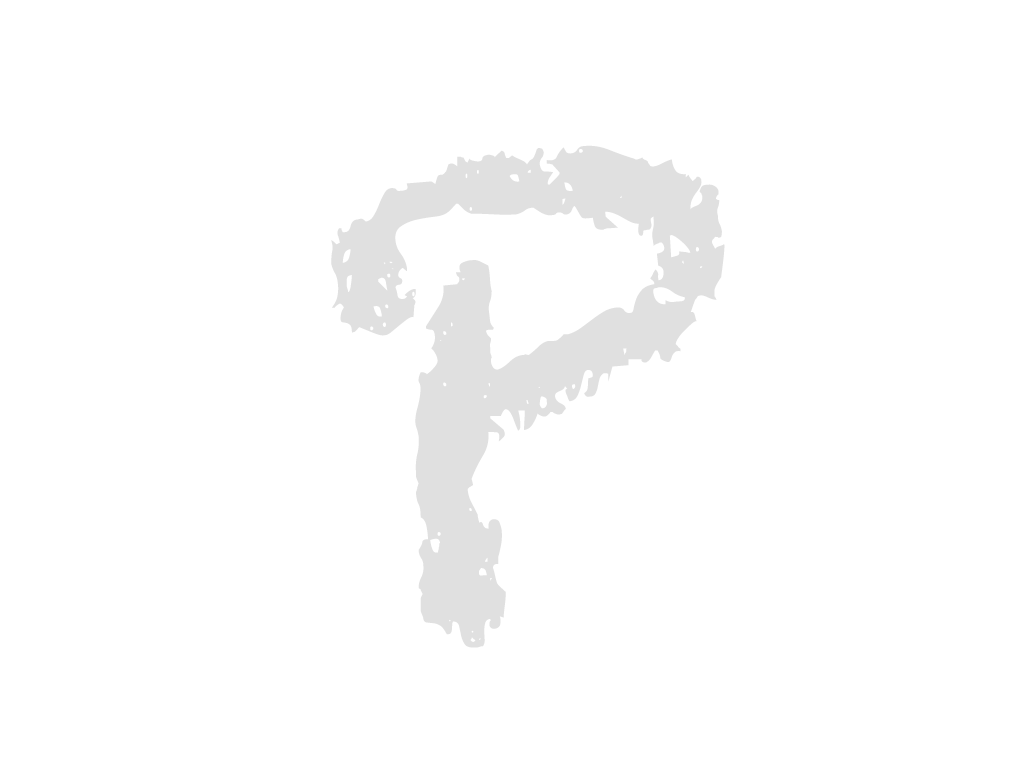
--- client/resources/css/common.css
+++ client/resources/css/common.css
... | ... | @@ -402,6 +402,29 @@ |
402 | 402 |
margin-left: 1rem; |
403 | 403 |
} |
404 | 404 |
|
405 |
+ |
|
406 |
+/* display */ |
|
407 |
+.display-inline-block { |
|
408 |
+ display: inline-block; |
|
409 |
+} |
|
410 |
+.display-inline { |
|
411 |
+ display: inline; |
|
412 |
+} |
|
413 |
+.display-block { |
|
414 |
+ display: block; |
|
415 |
+} |
|
416 |
+.display-none { |
|
417 |
+ display: none; |
|
418 |
+} |
|
419 |
+ |
|
420 |
+/* float */ |
|
421 |
+.float-left { |
|
422 |
+ float: left; |
|
423 |
+} |
|
424 |
+.float-right { |
|
425 |
+ float: right; |
|
426 |
+} |
|
427 |
+ |
|
405 | 428 |
hr { |
406 | 429 |
border-top: 1px solid #d1e4e3; |
407 | 430 |
margin-top: 2rem |
... | ... | @@ -410,4 +433,6 @@ |
410 | 433 |
/* 컬러 */ |
411 | 434 |
.yellow{background: #f2db71;} |
412 | 435 |
.sky{background-color: #d1e4e3;} |
436 |
+.grey{background-color: #8d8d8d;} |
|
437 |
+.green{background-color: #219102;} |
|
413 | 438 |
|
--- client/views/component/Modal_Guardian.jsx
+++ client/views/component/Modal_Guardian.jsx
... | ... | @@ -31,25 +31,25 @@ |
31 | 31 |
|
32 | 32 |
'senior_id': seniorId, |
33 | 33 |
'senior_relationship': '자녀', |
34 |
- 'senior_relationship_etc': '', |
|
35 | 34 |
}; |
36 | 35 |
|
37 |
- //시니어 정보 |
|
36 |
+ //보호자 정보 |
|
38 | 37 |
const [guardian, setGuardian] = React.useState(JSON.parse(JSON.stringify(guardianInit))); |
39 | 38 |
|
40 | 39 |
//각 데이터별로 Dom 정보 담을 Ref 생성 |
41 | 40 |
const guardianRefInit = JSON.parse(JSON.stringify(guardian)); |
42 | 41 |
guardianRefInit['user_gender'] = {}; |
42 |
+ guardianRefInit['senior_relationship_etc'] = null; |
|
43 | 43 |
const guardianRef = React.useRef(guardianRefInit); |
44 | 44 |
|
45 |
- //등록할 시니어 정보 변경 |
|
45 |
+ //등록할 보호자 정보 변경 |
|
46 | 46 |
const guardianValueChange = (targetKey, value) => { |
47 | 47 |
let newGuardian = JSON.parse(JSON.stringify(guardian)); |
48 | 48 |
newGuardian[targetKey] = value; |
49 | 49 |
setGuardian(newGuardian); |
50 | 50 |
} |
51 | 51 |
|
52 |
- //시니어 등록 유효성 검사 |
|
52 |
+ //보호자 등록 유효성 검사 |
|
53 | 53 |
const guardianInsertValidation = () => { |
54 | 54 |
if (CommonUtil.isEmpty(guardian['user_name']) == true) { |
55 | 55 |
guardianRef.current['user_name'].focus(); |
... | ... | @@ -77,14 +77,8 @@ |
77 | 77 |
return false; |
78 | 78 |
} |
79 | 79 |
if (CommonUtil.isEmpty(guardian['senior_relationship']) == true) { |
80 |
- guardianRef.current['senior_relationship'].focus(); |
|
81 |
- alert("대상자와의 관계를 선택해 주세요."); |
|
82 |
- return false; |
|
83 |
- } |
|
84 |
- |
|
85 |
- if (guardian['senior_relationship'] == '기타' && CommonUtil.isEmpty(guardian['senior_relationship_etc']) == true) { |
|
86 | 80 |
guardianRef.current['senior_relationship_etc'].focus(); |
87 |
- alert("대상자와의 관계를 입력해 주세요."); |
|
81 |
+ alert("대상자와의 관계를 입력 주세요."); |
|
88 | 82 |
return false; |
89 | 83 |
} |
90 | 84 |
|
... | ... | @@ -94,10 +88,6 @@ |
94 | 88 |
const guardianInsert = () => { |
95 | 89 |
if (guardianInsertValidation() == false) { |
96 | 90 |
return; |
97 |
- } |
|
98 |
- |
|
99 |
- if (guardian['senior_relationship'] == '기타') { |
|
100 |
- guardian['senior_relationship'] = guardian['senior_relationship_etc']; |
|
101 | 91 |
} |
102 | 92 |
|
103 | 93 |
fetch("/user/guardianInsert.json", { |
... | ... | @@ -119,7 +109,7 @@ |
119 | 109 |
}); |
120 | 110 |
} |
121 | 111 |
|
122 |
- //시니어 수정 |
|
112 |
+ //보호자 수정 |
|
123 | 113 |
const guardianUpdate = () => { |
124 | 114 |
if (guardianInsertValidation() == false) { |
125 | 115 |
return; |
... | ... | @@ -183,7 +173,7 @@ |
183 | 173 |
console.log('guardianBySenior : ', guardianBySenior); |
184 | 174 |
setGuardian(JSON.parse(JSON.stringify(guardianInit))); |
185 | 175 |
} |
186 |
- }, [guardianBySenior]); |
|
176 |
+ }, [open]); |
|
187 | 177 |
|
188 | 178 |
return ( |
189 | 179 |
<div class={open ? "openModal modal" : "modal"}> |
... | ... | @@ -265,12 +255,12 @@ |
265 | 255 |
{relationshipList.map((relationship, idx) => { return ( |
266 | 256 |
<option key={idx}>{relationship}</option> |
267 | 257 |
)})} |
268 |
- <option value="기타" selected={guardian['senior_relationship'] == '기타' || relationshipList.indexOf(guardian['senior_relationship']) == -1}>기타</option> |
|
258 |
+ <option value="" selected={relationshipList.indexOf(guardian['senior_relationship']) == -1}>기타</option> |
|
269 | 259 |
</select> |
270 |
- {guardian['senior_relationship'] == '기타' || relationshipList.indexOf(guardian['senior_relationship']) == -1 ? ( |
|
260 |
+ {relationshipList.indexOf(guardian['senior_relationship']) == -1 ? ( |
|
271 | 261 |
<input type="text" |
272 |
- value={guardian['senior_relationship_etc']} |
|
273 |
- onChange={(e) => {guardianValueChange('senior_relationship_etc', e.target.value)}} |
|
262 |
+ value={guardian['senior_relationship']} |
|
263 |
+ onChange={(e) => {guardianValueChange('senior_relationship', e.target.value)}} |
|
274 | 264 |
ref={el => guardianRef.current['senior_relationship_etc'] = el} |
275 | 265 |
/> |
276 | 266 |
) : null} |
--- client/views/component/Modal_SeniorInsert.jsx
+++ client/views/component/Modal_SeniorInsert.jsx
... | ... | @@ -140,6 +140,16 @@ |
140 | 140 |
|
141 | 141 |
//시니어 등록 유효성 검사 |
142 | 142 |
const seniorInsertValidation = () => { |
143 |
+ if (CommonUtil.isEmpty(senior['government_id']) == true) { |
|
144 |
+ seniorRef.current['government_id'].focus(); |
|
145 |
+ alert("관리기관을 선택해 주세요."); |
|
146 |
+ return false; |
|
147 |
+ } |
|
148 |
+ if (CommonUtil.isEmpty(senior['agency_id']) == true) { |
|
149 |
+ seniorRef.current['agency_id'].focus(); |
|
150 |
+ alert("시행기관을 선택해 주세요."); |
|
151 |
+ return false; |
|
152 |
+ } |
|
143 | 153 |
if (CommonUtil.isEmpty(senior['user_name']) == true) { |
144 | 154 |
seniorRef.current['user_name'].focus(); |
145 | 155 |
alert("이름을 입력해 주세요."); |
... | ... | @@ -227,9 +237,10 @@ |
227 | 237 |
<SubTitle explanation={"회원 등록 시 ID는 연락처, 패스워드는 생년월일 8자리입니다."} className="margin-bottom" /> |
228 | 238 |
<table className="margin-bottom2 senior-insert"> |
229 | 239 |
<tr> |
230 |
- <th>관리기관</th> |
|
240 |
+ <th><span style={{color : "red"}}>*</span>관리기관</th> |
|
231 | 241 |
<td> |
232 |
- <select onChange={(e) => {seniorGovernmentIdChange(e.target.value)}}> |
|
242 |
+ <select onChange={(e) => {seniorGovernmentIdChange(e.target.value)}} |
|
243 |
+ ref={el => seniorRef.current['government_id'] = el}> |
|
233 | 244 |
<option value={''} selected={senior['government_id'] == null}>관리기관선택</option> |
234 | 245 |
{orgListOfHierarchy.map((item, idx) => { return ( |
235 | 246 |
<option key={idx} value={item['government_id']} selected={senior['government_id'] == item['government_id']}> |
... | ... | @@ -238,9 +249,10 @@ |
238 | 249 |
)})} |
239 | 250 |
</select> |
240 | 251 |
</td> |
241 |
- <th>시행기관</th> |
|
252 |
+ <th><span style={{color : "red"}}>*</span>시행기관</th> |
|
242 | 253 |
<td> |
243 |
- <select onChange={(e) => {seniorAgencyIdChange(e.target.value)}}> |
|
254 |
+ <select onChange={(e) => {seniorAgencyIdChange(e.target.value)}} |
|
255 |
+ ref={el => seniorRef.current['agency_id'] = el}> |
|
244 | 256 |
<option value={''} selected={senior['agency_id'] == null}>시행기관선택</option> |
245 | 257 |
{getAgencyList().map((item, idx) => { return ( |
246 | 258 |
<option key={idx} value={item['agency_id']} selected={senior['agency_id'] == item['agency_id']}> |
--- client/views/pages/senior_management/SeniorSelectOne.jsx
+++ client/views/pages/senior_management/SeniorSelectOne.jsx
... | ... | @@ -60,7 +60,7 @@ |
60 | 60 |
'underlie_disease': null, |
61 | 61 |
'senior_note': null, |
62 | 62 |
|
63 |
- 'seniorMedicationList': [], |
|
63 |
+ 'seniorMedicationList': [] |
|
64 | 64 |
}); |
65 | 65 |
//시니어 상세 조회 |
66 | 66 |
const seniorSelectOne = () => { |
... | ... | @@ -78,8 +78,11 @@ |
78 | 78 |
}); |
79 | 79 |
}; |
80 | 80 |
|
81 |
- //보호자 상세 조회 |
|
81 |
+ //대상자의 보호자 목록 |
|
82 | 82 |
const [guardianListBySenior, setGuardianListBySenior] = React.useState([]); |
83 |
+ //대상자의 선택한 보호자 정보 |
|
84 |
+ const [guardianBySenior, setGuardianBySenior] = React.useState({}); |
|
85 |
+ //대상자의 보호자 목록 조회 |
|
83 | 86 |
const guardianSelectListBySenior = () => { |
84 | 87 |
fetch("/user/guardianSelectListBySenior.json", { |
85 | 88 |
method: "POST", |
... | ... | @@ -95,14 +98,31 @@ |
95 | 98 |
}); |
96 | 99 |
}; |
97 | 100 |
|
98 |
- //선택한 보호자 정보 |
|
99 |
- const [guardianBySenior, setGuardianBySenior] = React.useState({}); |
|
100 |
- |
|
101 | 101 |
//선택한 보호자 정보 관리 |
102 | 102 |
const guardianBySeniorManagement = (item) => { |
103 | 103 |
setGuardianBySenior(item); |
104 | 104 |
modalGuardianOpen(); |
105 | 105 |
} |
106 |
+ |
|
107 |
+ |
|
108 |
+ //대상자의 보호사 목록 |
|
109 |
+ const [agent, setAgent] = React.useState({agentListBySenior: [], agentListCountBySenior: 0}); |
|
110 |
+ //대상자의 보호사 목록 조회 |
|
111 |
+ const agentSelectListBySenior = () => { |
|
112 |
+ fetch("/user/agentSelectListBySenior.json", { |
|
113 |
+ method: "POST", |
|
114 |
+ headers: { |
|
115 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
116 |
+ }, |
|
117 |
+ body: JSON.stringify(senior), |
|
118 |
+ }).then((response) => response.json()).then((data) => { |
|
119 |
+ console.log("agentSelectListBySenior data : ", data); |
|
120 |
+ setAgent(data); |
|
121 |
+ }).catch((error) => { |
|
122 |
+ console.log('agentSelectListBySenior() /user/agentSelectListBySenior.json error : ', error); |
|
123 |
+ }); |
|
124 |
+ } |
|
125 |
+ |
|
106 | 126 |
|
107 | 127 |
|
108 | 128 |
//가입승인 |
... | ... | @@ -132,6 +152,7 @@ |
132 | 152 |
medicationTimeCodeSelectList(); |
133 | 153 |
seniorSelectOne(); |
134 | 154 |
guardianSelectListBySenior(); |
155 |
+ agentSelectListBySenior(); |
|
135 | 156 |
}, []) |
136 | 157 |
|
137 | 158 |
|
... | ... | @@ -142,56 +163,74 @@ |
142 | 163 |
guardianManagementCallback={() => {guardianSelectListBySenior(); modalGuardianClose(); setGuardianBySenior({})}}/> |
143 | 164 |
<div className="content-wrap row"> |
144 | 165 |
<SubTitle explanation={"대상자 상세 프로필"} className="margin-bottom" /> |
145 |
- <div> |
|
166 |
+ <div className="margin-bottom5"> |
|
146 | 167 |
<table className="margin-bottom senior-detail"> |
147 | 168 |
<tbody> |
148 |
- <tr> |
|
149 |
- <th>아이디</th> |
|
169 |
+ <tr> |
|
170 |
+ <th>관리기관</th> |
|
150 | 171 |
<td> |
151 |
- <span>{senior['user_id']}</span> |
|
172 |
+ <span>{senior['government_name']}</span> |
|
152 | 173 |
</td> |
153 | 174 |
</tr> |
175 |
+ |
|
154 | 176 |
<tr> |
155 |
- <th>이름</th> |
|
177 |
+ <th>시행기관</th> |
|
156 | 178 |
<td> |
157 |
- <span>{senior['user_name']}</span> |
|
158 |
- </td> |
|
179 |
+ <span>{senior['agency_name']}</span> |
|
180 |
+ </td> |
|
181 |
+ </tr> |
|
182 |
+ |
|
183 |
+ <tr> |
|
184 |
+ <th>이름(아이디)</th> |
|
185 |
+ <td> |
|
186 |
+ <span>{senior['user_name']}({senior['user_id']})</span> |
|
187 |
+ </td> |
|
188 |
+ </tr> |
|
189 |
+ |
|
190 |
+ <tr> |
|
159 | 191 |
<th>성별</th> |
160 | 192 |
<td> |
161 | 193 |
<span>{senior['user_gender']}</span> |
162 |
- </td> |
|
194 |
+ </td> |
|
163 | 195 |
</tr> |
196 |
+ |
|
164 | 197 |
<tr> |
165 |
- <th>생년월일</th> |
|
198 |
+ <th>생년월일</th> |
|
166 | 199 |
<td> |
167 | 200 |
<span>{senior['user_birth']}</span> |
168 | 201 |
</td> |
169 |
- <th>연락처</th> |
|
202 |
+ </tr> |
|
203 |
+ |
|
204 |
+ <tr> |
|
205 |
+ <th>생년월일</th> |
|
170 | 206 |
<td> |
171 |
- <span>{senior['user_phonenumber']}</span> |
|
207 |
+ <span>{senior['user_birth']}</span> |
|
172 | 208 |
</td> |
173 | 209 |
</tr> |
210 |
+ |
|
174 | 211 |
<tr> |
175 | 212 |
<th>주소</th> |
176 | 213 |
<td> |
177 | 214 |
<span>{senior['user_address']}</span> |
178 |
- </td> |
|
179 |
- |
|
215 |
+ </td> |
|
180 | 216 |
</tr> |
217 |
+ |
|
181 | 218 |
<tr> |
182 |
- <th>필요복약</th> |
|
219 |
+ <th>필요복약</th> |
|
183 | 220 |
<td className="flex-start"> |
184 | 221 |
{medicationTimeCodeList.filter(item => senior.seniorMedicationList.indexOf(item['medication_time_code']) > -1).map((item, idx) => { return ( |
185 | 222 |
<span>{item['medication_time_code_name']}</span> |
186 | 223 |
)})} |
187 | 224 |
</td> |
188 | 225 |
</tr> |
226 |
+ |
|
189 | 227 |
<tr> |
190 | 228 |
<th>복용중인 약</th> |
191 | 229 |
<td colSpan={3}> |
192 | 230 |
<span className="medicine" cols="30" rows="2">{senior['medication_pill']}</span> |
193 | 231 |
</td> |
194 | 232 |
</tr> |
233 |
+ |
|
195 | 234 |
<tr> |
196 | 235 |
<th>비고</th> |
197 | 236 |
<td colSpan={3}> |
... | ... | @@ -202,13 +241,14 @@ |
202 | 241 |
</table> |
203 | 242 |
<div className="btn-wrap flex-center"> |
204 | 243 |
<button className={"btn-large gray-btn"} onClick={() => modalGuardianOpen()}>수정</button> |
205 |
- <button className={"btn-large green-btn"} onClick={() => {setGuardianBySenior({}); modalGuardianOpen()}}>보호자 추가</button> |
|
244 |
+ |
|
206 | 245 |
<button className={"btn-large red-btn"} onClick={() => alert("삭제할 수 없습니다.")}>삭제</button> |
207 | 246 |
</div> |
208 | 247 |
</div> |
209 | 248 |
|
210 |
- <SubTitle explanation={"대상자의 보호자"} className="margin-bottom" /> |
|
211 |
- <div> |
|
249 |
+ <SubTitle explanation={"대상자의 보호자"} className="margin-bottom display-inline-block" /> |
|
250 |
+ <button className={"btn-small gray-btn display-inline-block float-right"} onClick={() => {setGuardianBySenior({}); modalGuardianOpen()}}>보호자 추가</button> |
|
251 |
+ <div className="margin-bottom5"> |
|
212 | 252 |
<table className={"protector-user"}> |
213 | 253 |
<thead> |
214 | 254 |
<tr> |
... | ... | @@ -218,8 +258,8 @@ |
218 | 258 |
<th>연락처</th> |
219 | 259 |
<th>생년월일</th> |
220 | 260 |
<th>주소</th> |
221 |
- <th>관리</th> |
|
222 | 261 |
<th>가입승인</th> |
262 |
+ <th>관리</th> |
|
223 | 263 |
</tr> |
224 | 264 |
</thead> |
225 | 265 |
<tbody> |
... | ... | @@ -237,7 +277,7 @@ |
237 | 277 |
} |
238 | 278 |
</td> |
239 | 279 |
<td> |
240 |
- <button className={"btn-small lightgray-btn"} onClick={() => guardianBySeniorManagement(item)}>관리</button> |
|
280 |
+ <button className={"btn-small lightgray-btn"} onClick={() => guardianBySeniorManagement(item)}>정보 수정</button> |
|
241 | 281 |
</td> |
242 | 282 |
</tr> |
243 | 283 |
)})} |
... | ... | @@ -250,6 +290,54 @@ |
250 | 290 |
</table> |
251 | 291 |
</div> |
252 | 292 |
|
293 |
+ |
|
294 |
+ <SubTitle explanation={"대상자의 보호사"} className="margin-bottom" /> |
|
295 |
+ <div className="margin-bottom5"> |
|
296 |
+ <table className={"senior-user"}> |
|
297 |
+ <thead> |
|
298 |
+ <tr> |
|
299 |
+ <th>No</th> |
|
300 |
+ <th>관리기관명</th> |
|
301 |
+ <th>소속기관명</th> |
|
302 |
+ <th>이름</th> |
|
303 |
+ <th>연락처</th> |
|
304 |
+ <th>이메일</th> |
|
305 |
+ <th>상태</th> |
|
306 |
+ <th>배정시작일</th> |
|
307 |
+ <th>배정종료일</th> |
|
308 |
+ <th>상세보기</th> |
|
309 |
+ </tr> |
|
310 |
+ </thead> |
|
311 |
+ <tbody> |
|
312 |
+ {agent.agentListBySenior.map((item, idx) => { return ( |
|
313 |
+ <tr key={idx}> |
|
314 |
+ <td>{idx + 1}</td> |
|
315 |
+ <td>{item['government_name']}</td> |
|
316 |
+ <td>{item['agency_name']}</td> |
|
317 |
+ <td>{item['user_name']}</td> |
|
318 |
+ <td>{item['user_phonenumber']}</td> |
|
319 |
+ <td>{item['user_email']}</td> |
|
320 |
+ <td>{item['agent_match_state'] ? <span className="green">현재 보호사</span> : <span className="grey">이전 보호사</span>}</td> |
|
321 |
+ <td>{item['agent_match_start_date']}</td> |
|
322 |
+ <td>{item['agent_match_end_date']}</td> |
|
323 |
+ <td> |
|
324 |
+ <button className={"btn-small lightgray-btn"} onClick={() => {}}>상세 페이지 이동</button> |
|
325 |
+ </td> |
|
326 |
+ </tr> |
|
327 |
+ )})} |
|
328 |
+ {agent.agentListBySenior == null || agent.agentListBySenior.length == 0 ? |
|
329 |
+ <tr> |
|
330 |
+ <td colSpan={10}>조회된 데이터가 없습니다</td> |
|
331 |
+ </tr> |
|
332 |
+ : null} |
|
333 |
+ </tbody> |
|
334 |
+ </table> |
|
335 |
+ </div> |
|
336 |
+ |
|
337 |
+ <div className="btn-wrap flex-center"> |
|
338 |
+ <button className={"btn-large gray-btn"} onClick={() => navigate(-1)}>이전</button> |
|
339 |
+ </div> |
|
340 |
+ |
|
253 | 341 |
</div> |
254 | 342 |
|
255 | 343 |
</main> |
--- client/views/pages/user_management/UserAuthoriySelect.jsx
+++ client/views/pages/user_management/UserAuthoriySelect.jsx
... | ... | @@ -385,7 +385,7 @@ |
385 | 385 |
{/* 탭 제목 */} |
386 | 386 |
<ul className="tab-menu flex-end" > |
387 | 387 |
<li onClick={() => setTabActiveByRoleType('ROLE_SENIOR')} className={tabActiveByRoleType == 'ROLE_SENIOR' ? "active" : null}>대상자(어르신) ({senior.userListCount})</li> |
388 |
- <li onClick={() => setTabActiveByRoleType('ROLE_AGENCY')} className={tabActiveByRoleType == 'ROLE_AGENCY' ? "active" : null}>담당자 ({agent.userListCount})</li> |
|
388 |
+ <li onClick={() => setTabActiveByRoleType('ROLE_AGENCY')} className={tabActiveByRoleType == 'ROLE_AGENCY' ? "active" : null}>보호사 ({agent.userListCount})</li> |
|
389 | 389 |
<li onClick={() => setTabActiveByRoleType('ROLE_GOVERNMENT')} className={tabActiveByRoleType == 'ROLE_GOVERNMENT' ? "active" : null}>기관 관리자 ({government.userListCount})</li> |
390 | 390 |
<li onClick={() => setTabActiveByRoleType('ROLE_ADMIN')} className={tabActiveByRoleType == 'ROLE_ADMIN' ? "active" : null}>시스템 관리자 ({admin.userListCount})</li> |
391 | 391 |
</ul> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?