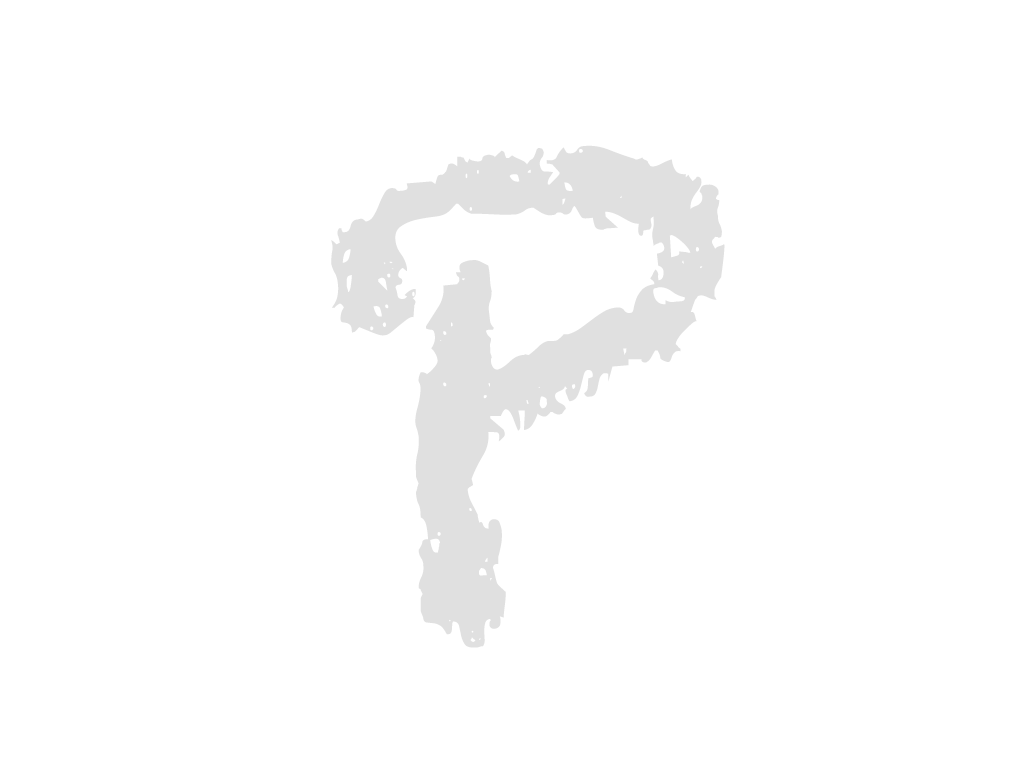
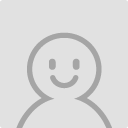
230302 김동준 qna 조회 일부 수정
@991021546b1e82c411bc98eaca64eaecc60cad32
--- client/views/pages/callcenter/QuestionSelect.jsx
+++ client/views/pages/callcenter/QuestionSelect.jsx
... | ... | @@ -1,11 +1,11 @@ |
1 |
-import React, {useState } from "react"; |
|
1 |
+import React from "react"; |
|
2 | 2 |
import Button from "../../component/Button.jsx"; |
3 | 3 |
import Table from "../../component/Table.jsx"; |
4 | 4 |
import QnAModal from "../../component/QnAModal.jsx"; |
5 | 5 |
import { useNavigate } from "react-router"; |
6 | 6 |
import ContentTitle from "../../component/ContentTitle.jsx"; |
7 |
-import RestoreFromTrashIcon from '@mui/icons-material/RestoreFromTrash'; |
|
8 |
- |
|
7 |
+import RestoreFromTrashIcon from "@mui/icons-material/RestoreFromTrash"; |
|
8 |
+import Pagination from "../../component/Pagination.jsx"; |
|
9 | 9 |
|
10 | 10 |
export default function QuestionSelect() { |
11 | 11 |
const navigate = useNavigate(); |
... | ... | @@ -16,95 +16,100 @@ |
16 | 16 |
const closeModal = () => { |
17 | 17 |
setModalOpen(false); |
18 | 18 |
}; |
19 |
- |
|
20 |
- const [qnalist, setQnaList] = useState(); |
|
21 | 19 |
|
22 |
- const [state, setState] = useState(); |
|
23 |
- const [title, setTitle] = useState(); |
|
24 |
- const [username, setUsername] = useState(); |
|
25 |
- const [datetime, setDatetime] = useState(); |
|
20 |
+ const [qnaList, setQnaList] = React.useState(); |
|
26 | 21 |
|
27 |
- //-------- 페이징 작업 설정 시작 --------// |
|
28 |
- const limit = 15; // 페이지당 보여줄 공지 개수 |
|
29 |
- const [page, setPage] = React.useState(1); //page index |
|
30 |
- const offset = (page - 1) * limit; //게시물 위치 계산 |
|
31 |
- const [myQnaTotal, setMyQnaTotal] = React.useState(0); //최대길이 넣을 변수 |
|
32 |
- |
|
22 |
+ const [state, setState] = React.useState(); |
|
23 |
+ const [title, setTitle] = React.useState(); |
|
24 |
+ const [username, setUsername] = React.useState(); |
|
25 |
+ const [datetime, setDatetime] = React.useState(); |
|
26 |
+ |
|
27 |
+ //-------- 페이징 작업 설정 시작 --------// |
|
28 |
+ const limit = 15; // 페이지당 보여줄 공지 개수 |
|
29 |
+ const [page, setPage] = React.useState(1); //page index |
|
30 |
+ const offset = (page - 1) * limit; //게시물 위치 계산 |
|
31 |
+ const [myQnaTotal, setMyQnaTotal] = React.useState(0); //최대길이 넣을 변수 |
|
32 |
+ |
|
33 | 33 |
//qna 조회 |
34 |
- const getQnaList = () => { |
|
35 |
- fetch("/qna/qnaSelectList.json", { |
|
36 |
- method: "POST", |
|
37 |
- headers: { |
|
38 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
39 |
- }, |
|
40 |
- body: JSON.stringify({ |
|
41 |
- |
|
42 |
- qna_state : state, |
|
43 |
- qna_title : title, |
|
44 |
- qna_insert_user_id : username, |
|
45 |
- qna_insert_datetime : datetime |
|
34 |
+ const getQnaList = () => { |
|
35 |
+ fetch("/qna/qnaSelectList.json", { |
|
36 |
+ method: "POST", |
|
37 |
+ headers: { |
|
38 |
+ "Content-Type": "application/json; charset=UTF-8;" |
|
39 |
+ }, |
|
40 |
+ body: JSON.stringify({ |
|
46 | 41 |
|
47 |
- }), |
|
48 |
- }).then((response) => response.json()).then((data) => { |
|
49 |
- |
|
50 |
- console.log(data); |
|
51 |
- setQnaList(data); |
|
52 |
- setMyQnaTotal(data.length); |
|
53 |
- |
|
54 |
- |
|
55 |
- }).catch((error) => { |
|
56 |
- console.log('getSelectSeniorList() /user/selectUserList.json error : ', error); |
|
42 |
+ qna_state: state, |
|
43 |
+ qna_title: title, |
|
44 |
+ qna_insert_user_id: username, |
|
45 |
+ qna_insert_datetime: datetime, |
|
46 |
+ }), |
|
47 |
+ }) |
|
48 |
+ .then((response) => response.json()) |
|
49 |
+ .then((data) => { |
|
50 |
+ console.log(data); |
|
51 |
+ setQnaList(data); |
|
52 |
+ // setMyQnaTotal(data.length); |
|
53 |
+ }) |
|
54 |
+ .catch((error) => { |
|
55 |
+ console.log( |
|
56 |
+ "qna error : ", |
|
57 |
+ error |
|
58 |
+ ); |
|
57 | 59 |
}); |
58 |
- }; |
|
59 |
- |
|
60 |
+ }; |
|
61 |
+ |
|
60 | 62 |
//게시판 |
61 |
- const thead = [ |
|
62 |
- "No", |
|
63 |
- "답변상태", |
|
64 |
- "제목", |
|
65 |
- "작성자", |
|
66 |
- "작성일자", |
|
67 |
- ]; |
|
68 |
- const key = [ |
|
69 |
- "No", |
|
70 |
- "answer", |
|
71 |
- "title", |
|
72 |
- "writer", |
|
73 |
- "date", |
|
74 |
- ]; |
|
63 |
+ const thead = ["No", "답변상태", "제목", "작성자", "작성일자"]; |
|
64 |
+ const key = ["idx", "state", "title", "username", "datetime"]; |
|
75 | 65 |
const content = [ |
76 | 66 |
{ |
77 | 67 |
No: 1, |
78 | 68 |
answer: "답변완료", |
79 | 69 |
title: ( |
80 |
- <div className="title" onClick={() => { |
|
81 |
- navigate("/QuestionConfirm"); |
|
82 |
- }}>담당자 바꿔주세요</div> |
|
70 |
+ <div |
|
71 |
+ className="title" |
|
72 |
+ onClick={() => { |
|
73 |
+ navigate("/QuestionConfirm"); |
|
74 |
+ }} |
|
75 |
+ > |
|
76 |
+ 담당자 바꿔주세요 |
|
77 |
+ </div> |
|
83 | 78 |
), |
84 | 79 |
writer: "홍길동", |
85 | 80 |
date: "2023-01-27", |
86 | 81 |
}, |
87 |
- |
|
88 | 82 |
]; |
83 |
+ |
|
84 |
+ React.useEffect(() => { |
|
85 |
+ |
|
86 |
+ getQnaList(); |
|
87 |
+ |
|
88 |
+ }, []) |
|
89 |
+ |
|
89 | 90 |
return ( |
90 | 91 |
<main> |
91 |
- <QnAModal open={modalOpen} close={closeModal} header="기관 등록"> |
|
92 |
- </QnAModal> |
|
92 |
+ <QnAModal |
|
93 |
+ open={modalOpen} |
|
94 |
+ close={closeModal} |
|
95 |
+ header="기관 등록" |
|
96 |
+ ></QnAModal> |
|
93 | 97 |
<div className="content-wrap"> |
94 | 98 |
<ContentTitle contentTitle={"Q&A"} /> |
95 | 99 |
<div className="board-wrap"> |
96 | 100 |
<div className="btn-wrap flex-end margin-bottom question-select"> |
97 |
- <input type="checkbox" /><label htmlFor="">내 Q&A 보기</label> |
|
98 |
- <select name="" id=""> |
|
99 |
- <option value="답변상태">답변상태</option> |
|
100 |
- <option value="미답변">미답변</option> |
|
101 |
- <option value="답변완료">답변완료</option> |
|
102 |
- </select> |
|
103 |
- <Button |
|
104 |
- className={"btn-small gray-btn"} |
|
105 |
- btnName={"작성하기"} |
|
106 |
- onClick={openModal} |
|
107 |
- /> |
|
101 |
+ <input type="checkbox" /> |
|
102 |
+ <label htmlFor="">내 Q&A 보기</label> |
|
103 |
+ <select name="" id=""> |
|
104 |
+ <option value="답변상태">답변상태</option> |
|
105 |
+ <option value="미답변">미답변</option> |
|
106 |
+ <option value="답변완료">답변완료</option> |
|
107 |
+ </select> |
|
108 |
+ <Button |
|
109 |
+ className={"btn-small gray-btn"} |
|
110 |
+ btnName={"작성하기"} |
|
111 |
+ onClick={openModal} |
|
112 |
+ /> |
|
108 | 113 |
</div> |
109 | 114 |
</div> |
110 | 115 |
<Table |
... | ... | @@ -114,7 +119,16 @@ |
114 | 119 |
contentKey={key} |
115 | 120 |
view={"qna"} |
116 | 121 |
offset={offset} |
117 |
- limit={limit}/> |
|
122 |
+ limit={limit} |
|
123 |
+ /> |
|
124 |
+ </div> |
|
125 |
+ <div> |
|
126 |
+ <Pagination |
|
127 |
+ total={myQnaTotal} |
|
128 |
+ limit={limit} |
|
129 |
+ page={page} |
|
130 |
+ setPage={setPage} |
|
131 |
+ /> |
|
118 | 132 |
</div> |
119 | 133 |
</main> |
120 | 134 |
); |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?