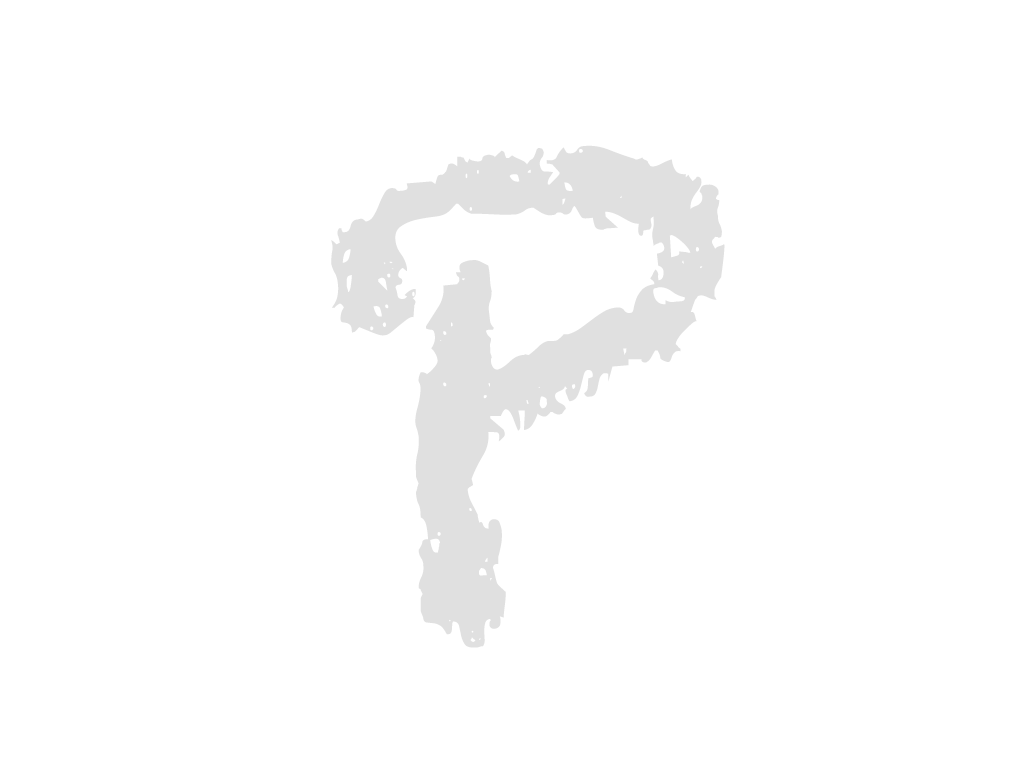
--- client/views/pages/equipment/EquipmentManagementSelect.jsx
+++ client/views/pages/equipment/EquipmentManagementSelect.jsx
... | ... | @@ -31,10 +31,48 @@ |
31 | 31 |
if (confirm('아니다') == false) { |
32 | 32 |
return; |
33 | 33 |
} |
34 |
- // 대상자 매칭 삭제 함수 사용하기 |
|
34 |
+ // 대상자 매칭 반납 함수 사용하기 |
|
35 | 35 |
}; |
36 | 36 |
|
37 | 37 |
const navigate = useNavigate(); |
38 |
+ |
|
39 |
+ // 대상자 목록 조회 |
|
40 |
+ const seniorSelectList = () => { |
|
41 |
+ console.log('seniorSelectList Function Run'); |
|
42 |
+ fetch("/user/userSeleteList.json", { |
|
43 |
+ method: "POST", |
|
44 |
+ headers: { |
|
45 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
46 |
+ }, |
|
47 |
+ body: JSON.stringify({ |
|
48 |
+ user_code: 'SENIOR01' |
|
49 |
+ }) |
|
50 |
+ }).then((response) => response.json()).then((data) => { |
|
51 |
+ console.log('seniorSelectList response : ', data); |
|
52 |
+ setSeniorList(data); |
|
53 |
+ }).catch((error) => { |
|
54 |
+ console.log('seniorSelectList error : ', error); |
|
55 |
+ }); |
|
56 |
+ } |
|
57 |
+ |
|
58 |
+ // 담당자 목록 조회 |
|
59 |
+ const workerSelectList = () => { |
|
60 |
+ console.log('workerSelectList Function Run'); |
|
61 |
+ fetch("/user/userSeleteList.json", { |
|
62 |
+ method: "POST", |
|
63 |
+ headers: { |
|
64 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
65 |
+ }, |
|
66 |
+ body: JSON.stringify({ |
|
67 |
+ user_code: 'WORKER01' |
|
68 |
+ }) |
|
69 |
+ }).then((response) => response.json()).then((data) => { |
|
70 |
+ console.log('workerSelectList response : ', data); |
|
71 |
+ setWorkerList(data); |
|
72 |
+ }).catch((error) => { |
|
73 |
+ console.log('workerSelectList error : ', error); |
|
74 |
+ }); |
|
75 |
+ } |
|
38 | 76 |
|
39 | 77 |
/********************************** 관리자 장비 (시작) **********************************/ |
40 | 78 |
// 관리자 전체 장비 목록 |
... | ... | @@ -182,8 +220,8 @@ |
182 | 220 |
const [seniorEquipment, setSeniorEquipment] = React.useState({}); |
183 | 221 |
// 대상자 장비 등록 모달창 |
184 | 222 |
const [seniorMatchInsertModal, setSeniorMatchInsertModal] = React.useState(false); |
185 |
- // 대상자 장비 삭제 모달창 |
|
186 |
- const [seniorMatchDeleteModal, setSeniorMatchDeleteModal] = React.useState(false); |
|
223 |
+ // 대상자 장비 반납 모달창 |
|
224 |
+ const [seniorMatchReturnModal, setSeniorMatchReturnModal] = React.useState(false); |
|
187 | 225 |
|
188 | 226 |
// 대상자 목록 |
189 | 227 |
const [seniorList, setSeniorList] = React.useState([]); |
... | ... | @@ -245,17 +283,21 @@ |
245 | 283 |
setSeniorEquipment(data); |
246 | 284 |
}; |
247 | 285 |
|
248 |
- // 대상자 장비 삭제 모달창 열기 |
|
249 |
- const seniorMatchDeleteModalOpen = (item) => { |
|
286 |
+ |
|
287 |
+ // 대상자 장비 반납 모달창 열기 |
|
288 |
+ const seniorMatchReturnModalOpen = (item) => { |
|
250 | 289 |
setSeniorEquipment(item); // 선택한 장비 데이터 저장 |
251 |
- setSeniorMatchDeleteModal(true); |
|
290 |
+ setSeniorMatchReturnModal(true); |
|
252 | 291 |
}; |
253 |
- // 대상자 장비 삭제 모달창 닫기 |
|
254 |
- const seniorMatchDeleteModalClose = () => { |
|
255 |
- setSeniorMatchDeleteModal(false); |
|
292 |
+ |
|
293 |
+ // 대상자 장비 반납 모달창 닫기 |
|
294 |
+ const seniorMatchReturnModalClose = () => { |
|
295 |
+ setSeniorMatchReturnModal(false); |
|
256 | 296 |
}; |
297 |
+ |
|
257 | 298 |
// 대상자 장비 - 반납일 |
258 | 299 |
const seniorEquipmentRentalReturnDateInsert = (e) => { |
300 |
+ console.log('@@@', e.target.value); |
|
259 | 301 |
// 원본 데이터 복사 및 수정 |
260 | 302 |
let data = { |
261 | 303 |
...seniorEquipment, |
... | ... | @@ -264,8 +306,10 @@ |
264 | 306 |
// 복사 데이터를 원본 데이터에 덮어쓰기 |
265 | 307 |
setSeniorEquipment(data); |
266 | 308 |
}; |
267 |
- // 대상자 장비 - 반납 관리자 |
|
309 |
+ |
|
310 |
+ // 대상자 장비 - 반납 담당자 |
|
268 | 311 |
const seniorEquipmentReturnUserIdInsert = (e) => { |
312 |
+ console.log('@@@@@', e.target.value); |
|
269 | 313 |
// 원본 데이터 복사 및 수정 |
270 | 314 |
let data = { |
271 | 315 |
...seniorEquipment, |
... | ... | @@ -277,27 +321,8 @@ |
277 | 321 |
|
278 | 322 |
|
279 | 323 |
// 시행기관 전체 장비 목록 조회 |
280 |
- // const agencyEquipmentSelectList = () => { |
|
281 |
- // console.log('agencyEquipmentSelectList Function Run'); |
|
282 |
- // fetch("/equipment/agencyEquipmentSelectList.json", { |
|
283 |
- // method: "POST", |
|
284 |
- // headers: { |
|
285 |
- // 'Content-Type': 'application/json; charset=UTF-8' |
|
286 |
- // }, |
|
287 |
- // body: JSON.stringify({ |
|
288 |
- // agency_id: 'AGENCY01', |
|
289 |
- // }), |
|
290 |
- // }).then((response) => response.json()).then((data) => { |
|
291 |
- // console.log('agencyEquipmentSelectList response : ', data); |
|
292 |
- // setAgencyEquipmentList(data); |
|
293 |
- // }).catch((error) => { |
|
294 |
- // console.log('agencyEquipmentSelectList error : ', error); |
|
295 |
- // }); |
|
296 |
- // } |
|
297 |
- |
|
298 |
- // 시행기관 전체 장비 목록 조회 |
|
299 | 324 |
const agencyEquipmentSelectList = () => { |
300 |
- console.log('equipmentSelectList Function Run'); |
|
325 |
+ console.log('agencyEquipmentSelectList Function Run'); |
|
301 | 326 |
fetch("/equipment/equipmentSelectList.json", { |
302 | 327 |
method: "POST", |
303 | 328 |
headers: { |
... | ... | @@ -308,10 +333,10 @@ |
308 | 333 |
searchAll: true |
309 | 334 |
}), |
310 | 335 |
}).then((response) => response.json()).then((data) => { |
311 |
- console.log('equipmentSelectList response : ', data); |
|
336 |
+ console.log('agencyEquipmentSelectList response : ', data); |
|
312 | 337 |
setAgencyEquipmentList(data); |
313 | 338 |
}).catch((error) => { |
314 |
- console.log('equipmentSelectList error : ', error); |
|
339 |
+ console.log('agencyEquipmentSelectList error : ', error); |
|
315 | 340 |
}); |
316 | 341 |
} |
317 | 342 |
|
... | ... | @@ -393,6 +418,12 @@ |
393 | 418 |
console.log('seniorEquipmentInsert response : ', data); |
394 | 419 |
// 대상자 장비 데이터 초기화 |
395 | 420 |
setSeniorEquipment({}); |
421 |
+ |
|
422 |
+ // 장비 목록 조회 |
|
423 |
+ agencyEquipmentSelectList(); |
|
424 |
+ agencySeniorEquipmentSelectList(); |
|
425 |
+ agencyStockEquipmentSelectList(); |
|
426 |
+ |
|
396 | 427 |
alert('등록이 완료됐습니다.'); |
397 | 428 |
}).catch((error) => { |
398 | 429 |
console.log('seniorEquipmentInsert error : ', error); |
... | ... | @@ -400,58 +431,64 @@ |
400 | 431 |
}); |
401 | 432 |
} |
402 | 433 |
|
403 |
- // 대상자 목록 조회 |
|
404 |
- const seniorSelectList = () => { |
|
405 |
- console.log('seniorSelectList Function Run'); |
|
406 |
- fetch("/user/selectSubjectList.json", { |
|
434 |
+ // 대상자 장비 반납 |
|
435 |
+ const seniorEquipmentReturn = () => { |
|
436 |
+ console.log('seniorEquipmentReturn Function Run'); |
|
437 |
+ |
|
438 |
+ // 담당자 아이디 유효성 검사 |
|
439 |
+ let check = 0; |
|
440 |
+ for (let i = 0; i < workerList.length; i++) { |
|
441 |
+ // 입력한 데이터가 담당자 목록에 있을 경우 |
|
442 |
+ if (workerList[i]['user_id'] == seniorEquipment['return_user_id']) { |
|
443 |
+ console.log('success: ', workerList[i]['user_id']); |
|
444 |
+ check = 1; |
|
445 |
+ } |
|
446 |
+ } |
|
447 |
+ // 입력한 데이터가 담당자 목록에 없을 경우 |
|
448 |
+ if (check != 1) { |
|
449 |
+ console.log('fail: ', workerList, seniorEquipment['return_user_id']); |
|
450 |
+ alert('존재하지 않는 담당자입니다.'); |
|
451 |
+ return; |
|
452 |
+ } |
|
453 |
+ |
|
454 |
+ fetch("/equipment/seniorEquipmentReturn.json", { |
|
407 | 455 |
method: "POST", |
408 | 456 |
headers: { |
409 | 457 |
'Content-Type': 'application/json; charset=UTF-8' |
410 | 458 |
}, |
411 |
- body: JSON.stringify({}) |
|
459 |
+ body: JSON.stringify(seniorEquipment), |
|
412 | 460 |
}).then((response) => response.json()).then((data) => { |
413 |
- console.log('seniorSelectList response : ', data); |
|
414 |
- setSeniorList(data); |
|
461 |
+ console.log('seniorEquipmentReturn response : ', data); |
|
462 |
+ // 대상자 장비 데이터 초기화 |
|
463 |
+ setSeniorEquipment({}); |
|
464 |
+ |
|
465 |
+ // 장비 목록 조회 |
|
466 |
+ agencyEquipmentSelectList(); |
|
467 |
+ agencySeniorEquipmentSelectList(); |
|
468 |
+ agencyStockEquipmentSelectList(); |
|
469 |
+ |
|
470 |
+ alert('반납이 완료됐습니다.'); |
|
415 | 471 |
}).catch((error) => { |
416 |
- console.log('seniorSelectList error : ', error); |
|
472 |
+ console.log('seniorEquipmentReturn error : ', error); |
|
473 |
+ alert('반납에 실패했습니다.'); |
|
417 | 474 |
}); |
418 | 475 |
} |
419 | 476 |
|
420 |
- // 담당자 목록 조회 |
|
421 |
- const workerSelectList = () => { |
|
422 |
- console.log('workerSelectList Function Run'); |
|
423 |
- fetch("/user/selectSubjectList.json", { |
|
424 |
- method: "POST", |
|
425 |
- headers: { |
|
426 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
427 |
- }, |
|
428 |
- body: JSON.stringify({}) |
|
429 |
- }).then((response) => response.json()).then((data) => { |
|
430 |
- console.log('workerSelectList response : ', data); |
|
431 |
- setSeniorList(data); |
|
432 |
- }).catch((error) => { |
|
433 |
- console.log('workerSelectList error : ', error); |
|
434 |
- }); |
|
435 |
- } |
|
436 | 477 |
|
437 | 478 |
// 시행기관 전체 장비 thead, key |
438 | 479 |
const thead11 = [ |
439 | 480 |
"No", |
440 | 481 |
"모델 명", |
441 | 482 |
"시리얼 넘버", |
442 |
- "상태", |
|
443 |
- "대상자", |
|
444 |
- "대여일", |
|
445 |
- "반납예정일", |
|
446 |
- "관리" |
|
483 |
+ "기기 상태", |
|
484 |
+ "대여 상태", |
|
485 |
+ "대상자 관리", |
|
447 | 486 |
]; |
448 | 487 |
const key11 = [ |
449 | 488 |
"equipment_name", |
450 | 489 |
"equipment_serial_number", |
451 | 490 |
"equipment_state", |
452 |
- "senior_name", |
|
453 |
- "equipment_rental_start_date", |
|
454 |
- "equipment_rental_end_date", |
|
491 |
+ "equipment_rental_state", |
|
455 | 492 |
]; |
456 | 493 |
|
457 | 494 |
// 시행기관 대상자 장비 thead, key |
... | ... | @@ -459,11 +496,11 @@ |
459 | 496 |
"No", |
460 | 497 |
"모델 명", |
461 | 498 |
"시리얼 넘버", |
462 |
- "상태", |
|
499 |
+ "기기 상태", |
|
463 | 500 |
"대상자", |
464 | 501 |
"대여일", |
465 | 502 |
"반납예정일", |
466 |
- "관리" |
|
503 |
+ "대상자 관리", |
|
467 | 504 |
]; |
468 | 505 |
const key22 = [ |
469 | 506 |
"equipment_name", |
... | ... | @@ -479,19 +516,13 @@ |
479 | 516 |
"No", |
480 | 517 |
"모델 명", |
481 | 518 |
"시리얼 넘버", |
482 |
- "상태", |
|
483 |
- "대상자", |
|
484 |
- "대여일", |
|
485 |
- "반납예정일", |
|
486 |
- "관리" |
|
519 |
+ "기기 상태", |
|
520 |
+ "대상자 관리", |
|
487 | 521 |
]; |
488 | 522 |
const key33 = [ |
489 | 523 |
"equipment_name", |
490 | 524 |
"equipment_serial_number", |
491 | 525 |
"equipment_state", |
492 |
- "senior_name", |
|
493 |
- "equipment_rental_start_date", |
|
494 |
- "equipment_rental_end_date", |
|
495 | 526 |
]; |
496 | 527 |
|
497 | 528 |
// 장비 대상자 등록 모달창 thead |
... | ... | @@ -502,15 +533,18 @@ |
502 | 533 |
"선택", |
503 | 534 |
]; |
504 | 535 |
|
505 |
- // 장비 대상자 삭제 모달창 thead |
|
536 |
+ // 장비 대상자 반납 모달창 thead |
|
506 | 537 |
const thead55 = [ |
507 | 538 |
"반납일", |
508 |
- "반납자", |
|
539 |
+ "반납 담당자", |
|
540 |
+ "선택", |
|
509 | 541 |
]; |
510 | 542 |
|
511 | 543 |
// 마운트 시 실행 함수 |
512 | 544 |
React.useEffect(() => { |
513 | 545 |
seniorSelectList(); |
546 |
+ workerSelectList(); |
|
547 |
+ |
|
514 | 548 |
//관리자 |
515 | 549 |
equipmentSelectList(); |
516 | 550 |
|
... | ... | @@ -602,7 +636,7 @@ |
602 | 636 |
/> |
603 | 637 |
: <Button |
604 | 638 |
className={"btn-small green-btn"} |
605 |
- btnName={"삭제"} |
|
639 |
+ btnName={"반납"} |
|
606 | 640 |
onClick={seniorMatchDelete} |
607 | 641 |
/> |
608 | 642 |
} |
... | ... | @@ -667,6 +701,7 @@ |
667 | 701 |
</thead> |
668 | 702 |
<tbody> |
669 | 703 |
{agencyEquipmentList.map((item, index) => { |
704 |
+ if(item) |
|
670 | 705 |
return ( |
671 | 706 |
<tr key={index}> |
672 | 707 |
<td>{agencyEquipmentList.length - index}</td> |
... | ... | @@ -675,7 +710,7 @@ |
675 | 710 |
})} |
676 | 711 |
<td> |
677 | 712 |
{ |
678 |
- item['senior_id'] == null |
|
713 |
+ item['equipment_rental_state'] == false |
|
679 | 714 |
? <Button |
680 | 715 |
className={"btn-small green-btn"} |
681 | 716 |
btnName={"등록"} |
... | ... | @@ -684,7 +719,7 @@ |
684 | 719 |
: <Button |
685 | 720 |
className={"btn-small green-btn"} |
686 | 721 |
btnName={"반납"} |
687 |
- onClick={() => seniorMatchDeleteModalOpen(item)} |
|
722 |
+ onClick={() => seniorMatchReturnModalOpen(item)} |
|
688 | 723 |
/> |
689 | 724 |
} |
690 | 725 |
</td> |
... | ... | @@ -725,7 +760,7 @@ |
725 | 760 |
<Button |
726 | 761 |
className={"btn-small green-btn"} |
727 | 762 |
btnName={"반납"} |
728 |
- onClick={() => seniorMatchDeleteModalOpen(item)} |
|
763 |
+ onClick={() => seniorMatchReturnModalOpen(item)} |
|
729 | 764 |
/> |
730 | 765 |
} |
731 | 766 |
</td> |
... | ... | @@ -891,7 +926,7 @@ |
891 | 926 |
</div> |
892 | 927 |
</Modal> |
893 | 928 |
|
894 |
- <Modal open={seniorMatchDeleteModal} close={seniorMatchDeleteModalClose} header="대상자 장비 반납"> |
|
929 |
+ <Modal open={seniorMatchReturnModal} close={seniorMatchReturnModalClose} header="대상자 장비 반납"> |
|
895 | 930 |
<div className="board-wrap"> |
896 | 931 |
<div> |
897 | 932 |
<table className={"caregiver-user"}> |
... | ... | @@ -908,12 +943,10 @@ |
908 | 943 |
<input type="date" onChange={seniorEquipmentRentalReturnDateInsert} /> |
909 | 944 |
</td> |
910 | 945 |
<td> |
911 |
- <input type="text" list="senior_list" placeholder="반납 관리자를 입력해 주세요" onChange={seniorEquipmentReturnUserIdInsert} /> |
|
946 |
+ <input type="text" list="senior_list" placeholder="반납 담당자를 입력해 주세요" onChange={seniorEquipmentReturnUserIdInsert} /> |
|
912 | 947 |
<datalist id="senior_list"> |
913 |
- {seniorList.map((item) => { |
|
914 |
- // return <option value={item['user_name'] + " (" + item['user_id'] + ")"}></option> |
|
948 |
+ {workerList.map((item) => { |
|
915 | 949 |
return <option value={item['user_id']}>{item['user_name']}</option> |
916 |
- |
|
917 | 950 |
})} |
918 | 951 |
</datalist> |
919 | 952 |
</td> |
... | ... | @@ -921,7 +954,7 @@ |
921 | 954 |
<Button |
922 | 955 |
className={"btn-small gray-btn"} |
923 | 956 |
btnName={"선택"} |
924 |
- onClick={seniorEquipmentInsert} |
|
957 |
+ onClick={seniorEquipmentReturn} |
|
925 | 958 |
/> |
926 | 959 |
</td> |
927 | 960 |
</tr> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?