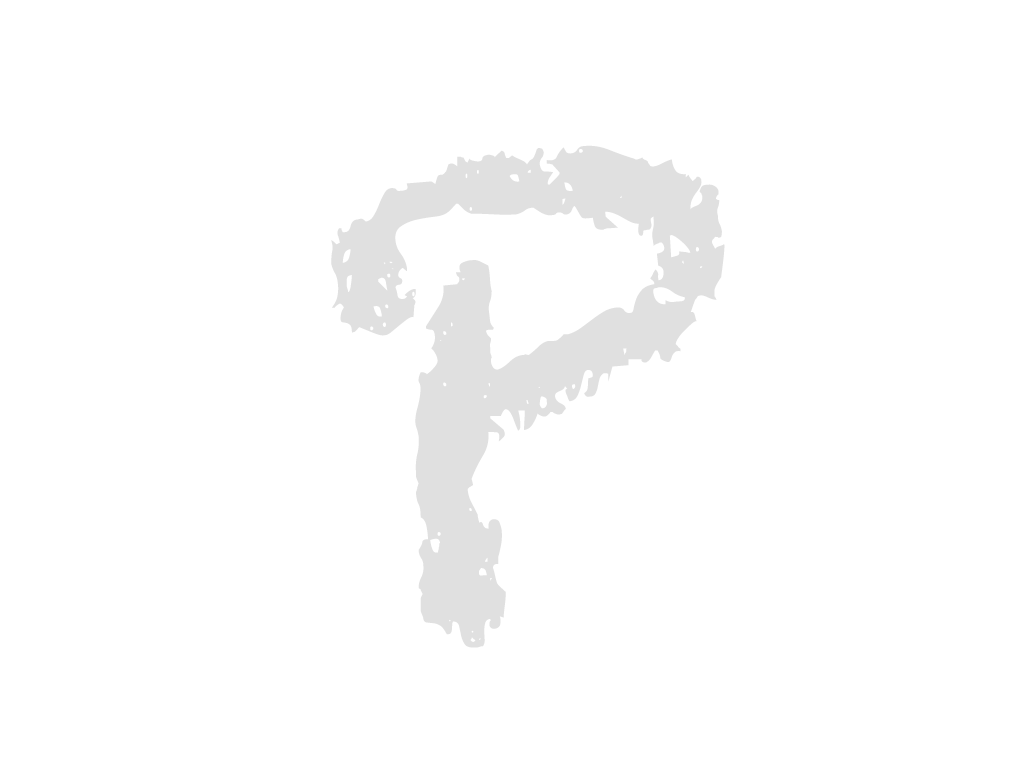
--- client/resources/css/common.css
+++ client/resources/css/common.css
... | ... | @@ -431,8 +431,8 @@ |
431 | 431 |
} |
432 | 432 |
|
433 | 433 |
/* 컬러 */ |
434 |
-.yellow{background: #f2db71;} |
|
435 |
-.sky{background-color: #d1e4e3;} |
|
436 |
-.grey{background-color: #8d8d8d;} |
|
437 |
-.green{background-color: #219102;} |
|
434 |
+.yellow{color: #f2db71;} |
|
435 |
+.sky{color: #68d1cc;} |
|
436 |
+.grey{color: #8d8d8d;} |
|
437 |
+.green{color: #209101;} |
|
438 | 438 |
|
--- client/views/component/Modal_SeniorInsert.jsx
+++ client/views/component/Modal_SeniorInsert.jsx
... | ... | @@ -1,10 +1,10 @@ |
1 |
-import React, { useState, useRef } from "react"; |
|
1 |
+import React from "react"; |
|
2 | 2 |
import { useSelector } from "react-redux"; |
3 | 3 |
import SubTitle from "./SubTitle.jsx"; |
4 | 4 |
|
5 | 5 |
import CommonUtil from "../../resources/js/CommonUtil.js"; |
6 | 6 |
|
7 |
-export default function Modal({ open, close, seniorInsertCallback, defaultAgencyId, defaultGovernmentId }) { |
|
7 |
+export default function Modal({ open, close, seniorInsertCallback, defaultAgentId, defaultAgencyId, defaultGovernmentId }) { |
|
8 | 8 |
|
9 | 9 |
//console.log(`seniorInsertModal - defaultGovernmentId:${defaultGovernmentId}, defaultAgencyId:${defaultAgencyId}`) |
10 | 10 |
|
... | ... | @@ -67,7 +67,7 @@ |
67 | 67 |
|
68 | 68 |
|
69 | 69 |
//등록할 시니어 정보 |
70 |
- const [senior, setSenior] = useState({ |
|
70 |
+ const [senior, setSenior] = React.useState({ |
|
71 | 71 |
'user_id': null, |
72 | 72 |
'user_name': null, |
73 | 73 |
'user_password': null, |
... | ... | @@ -87,13 +87,16 @@ |
87 | 87 |
'senior_note': null, |
88 | 88 |
|
89 | 89 |
'seniorMedicationList': [], |
90 |
+ |
|
91 |
+ 'agent_id': !defaultAgentId ? state.loginUser['agent_id'] : defaultAgentId, |
|
90 | 92 |
}); |
91 | 93 |
//console.log(`seniorInsertModal - senior:`, senior); |
92 | 94 |
|
93 | 95 |
//각 데이터별로 Dom 정보 담을 Ref 생성 |
94 | 96 |
const seniorRefInit = JSON.parse(JSON.stringify(senior)); |
95 | 97 |
seniorRefInit['user_gender'] = {}; |
96 |
- const seniorRef = useRef(seniorRefInit); |
|
98 |
+ seniorRefInit['user_id_check_button'] = null; |
|
99 |
+ const seniorRef = React.useRef(seniorRefInit); |
|
97 | 100 |
|
98 | 101 |
//등록할 시니어 정보 변경 |
99 | 102 |
const seniorValueChange = (targetKey, value) => { |
... | ... | @@ -137,7 +140,38 @@ |
137 | 140 |
} |
138 | 141 |
} |
139 | 142 |
|
143 |
+ //아이디 중복 확인 |
|
144 |
+ const [isIdCheck, setIsIdCheck] = React.useState(false); |
|
145 |
+ //로그인 아이디 중복 검사 |
|
146 |
+ const userIdCheck = () => { |
|
147 |
+ if (CommonUtil.isEmpty(senior['user_phonenumber']) == true) { |
|
148 |
+ seniorRef.current['user_phonenumber'].focus(); |
|
149 |
+ alert("연락처를 입력해 주세요."); |
|
150 |
+ return false; |
|
151 |
+ } |
|
140 | 152 |
|
153 |
+ senior['user_id'] = senior['user_phonenumber']; |
|
154 |
+ fetch("/user/userSelectOne.json", { |
|
155 |
+ method: "POST", |
|
156 |
+ headers: { |
|
157 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
158 |
+ }, |
|
159 |
+ body: JSON.stringify(senior), |
|
160 |
+ }).then((response) => response.json()).then((data) => { |
|
161 |
+ console.log("로그인 아이디 중복 검사(아이디를 통한 사용자 조회) : ", data); |
|
162 |
+ if (CommonUtil.isEmpty(data) == true) { |
|
163 |
+ setIsIdCheck(true); |
|
164 |
+ seniorRef.current['user_address'].focus(); |
|
165 |
+ alert("사용가능한 연락처 입니다."); |
|
166 |
+ } else { |
|
167 |
+ setIsIdCheck(false); |
|
168 |
+ seniorRef.current['user_phonenumber'].focus(); |
|
169 |
+ alert("이미 존재하는 연락처 입니다."); |
|
170 |
+ } |
|
171 |
+ }).catch((error) => { |
|
172 |
+ console.log('userIdCheck() /user/userSelectOne.json error : ', error); |
|
173 |
+ }); |
|
174 |
+ } |
|
141 | 175 |
//시니어 등록 유효성 검사 |
142 | 176 |
const seniorInsertValidation = () => { |
143 | 177 |
if (CommonUtil.isEmpty(senior['government_id']) == true) { |
... | ... | @@ -168,6 +202,10 @@ |
168 | 202 |
if (CommonUtil.isEmpty(senior['user_phonenumber']) == true) { |
169 | 203 |
seniorRef.current['user_phonenumber'].focus(); |
170 | 204 |
alert("연락처를 입력해 주세요."); |
205 |
+ return false; |
|
206 |
+ } |
|
207 |
+ if (isIdCheck == false) { |
|
208 |
+ alert("연락처 중복확인을 해주세요."); |
|
171 | 209 |
return false; |
172 | 210 |
} |
173 | 211 |
if (CommonUtil.isEmpty(senior['user_address']) == true) { |
... | ... | @@ -306,11 +344,15 @@ |
306 | 344 |
<tr> |
307 | 345 |
<th><span style={{color : "red"}}>*</span>연락처</th> |
308 | 346 |
<td colSpan={3}> |
309 |
- <input type="number" maxLength="11" |
|
347 |
+ <input type="number" maxLength="11" style={{width: 'calc(100% - 160px)'}} |
|
310 | 348 |
value={senior['user_phonenumber']} |
311 | 349 |
onChange={(e) => {seniorValueChange('user_phonenumber', e.target.value)}} |
312 | 350 |
ref={el => seniorRef.current['user_phonenumber'] = el} |
313 | 351 |
/> |
352 |
+ <button className={"red-btn btn-large"} onClick={userIdCheck} |
|
353 |
+ ref={el => seniorRef.current['user_id_check_button'] = el}> |
|
354 |
+ 중복확인 |
|
355 |
+ </button> |
|
314 | 356 |
</td> |
315 | 357 |
</tr> |
316 | 358 |
<tr> |
--- client/views/pages/AppRoute.jsx
+++ client/views/pages/AppRoute.jsx
... | ... | @@ -51,6 +51,9 @@ |
51 | 51 |
import Healthcare from "./healthcare/Healthcare.jsx" |
52 | 52 |
import Medicalcare from "./healthcare/Medicalcare.jsx" |
53 | 53 |
|
54 |
+import UserEdit from "./user_management/UserEdit.jsx"; |
|
55 |
+import AgentSelectOne from "./user_management/AgentSelectOne.jsx"; |
|
56 |
+ |
|
54 | 57 |
const AllAppMenuItems = [ |
55 | 58 |
{ |
56 | 59 |
title: "올잇메디", |
... | ... | @@ -461,7 +464,10 @@ |
461 | 464 |
<Route path="/QandAConfirm" element={<QandAConfirm />}></Route> |
462 | 465 |
<Route path="/Join" element={<Join />}></Route> |
463 | 466 |
<Route path="/SeniorEdit" element={<SeniorEdit />}></Route> |
464 |
- <Route path="/SeniorSelectOne" element={<SeniorSelectOne />}></Route> |
|
467 |
+ <Route path="/SeniorSelectOne" element={<SeniorSelectOne />}></Route> |
|
468 |
+ <Route path="/UserEdit" element={<UserEdit />}></Route> |
|
469 |
+ <Route path="/AgentSelectOne" element={<AgentSelectOne />}></Route> |
|
470 |
+ |
|
465 | 471 |
</Routes> |
466 | 472 |
); |
467 | 473 |
} |
... | ... | @@ -562,9 +568,12 @@ |
562 | 568 |
<Route path="/QandAConfirm" element={<QandAConfirm />}></Route> |
563 | 569 |
<Route path="/QandAInsert" element={<QandAInsert />}></Route> |
564 | 570 |
<Route path="/ProtectorSelect" element={<ProtectorSelect />}></Route> |
565 |
- <Route path="/SeniorEdit/:seniorId" element={<SeniorEdit />}></Route> |
|
566 | 571 |
<Route path="/AgencyInsert" element={<AgencyInsert />}></Route> |
567 | 572 |
<Route path="/Join" element={<Join />}></Route> |
573 |
+ <Route path="/SeniorEdit" element={<SeniorEdit />}></Route> |
|
574 |
+ <Route path="/SeniorSelectOne" element={<SeniorSelectOne />}></Route> |
|
575 |
+ <Route path="/UserEdit" element={<UserEdit />}></Route> |
|
576 |
+ <Route path="/AgentSelectOne" element={<AgentSelectOne />}></Route> |
|
568 | 577 |
</Routes> |
569 | 578 |
); |
570 | 579 |
} |
... | ... | @@ -632,8 +641,10 @@ |
632 | 641 |
|
633 | 642 |
<Route path="/Main" element={<Main_agency />}></Route> |
634 | 643 |
<Route path="/UserAuthoriySelect_agency" element={<UserAuthoriySelect_agency />}></Route> |
635 |
- <Route path="/SeniorEdit/:seniorId" element={<SeniorEdit />}></Route> |
|
636 |
- <Route path="/SeniorSelectOne/:seniorId" element={<SeniorSelectOne />}></Route> |
|
644 |
+ <Route path="/SeniorEdit" element={<SeniorEdit />}></Route> |
|
645 |
+ <Route path="/SeniorSelectOne" element={<SeniorSelectOne />}></Route> |
|
646 |
+ <Route path="/UserEdit" element={<UserEdit />}></Route> |
|
647 |
+ <Route path="/AgentSelectOne" element={<AgentSelectOne />}></Route> |
|
637 | 648 |
<Route |
638 | 649 |
path="/MedicineCareSelectOne" |
639 | 650 |
element={<MedicineCareSelectOne />} |
--- client/views/pages/join/Join.jsx
+++ client/views/pages/join/Join.jsx
... | ... | @@ -62,7 +62,7 @@ |
62 | 62 |
} |
63 | 63 |
/**** 기본 조회 데이터 (종료) ****/ |
64 | 64 |
|
65 |
- //로그인 중복 확인 |
|
65 |
+ //아이디 중복 확인 |
|
66 | 66 |
const [isIdCheck, setIsIdCheck] = React.useState(false); |
67 | 67 |
|
68 | 68 |
//등록할 사용자 정보 |
... | ... | @@ -123,6 +123,12 @@ |
123 | 123 |
} |
124 | 124 |
//로그인 아이디 중복 검사 |
125 | 125 |
const userIdCheck = () => { |
126 |
+ if (CommonUtil.isEmpty(user['user_id']) == true) { |
|
127 |
+ userRef.current['user_id'].focus(); |
|
128 |
+ alert("아이디를 입력해 주세요."); |
|
129 |
+ return; |
|
130 |
+ } |
|
131 |
+ |
|
126 | 132 |
fetch("/user/userSelectOne.json", { |
127 | 133 |
method: "POST", |
128 | 134 |
headers: { |
... | ... | @@ -172,7 +178,7 @@ |
172 | 178 |
return false; |
173 | 179 |
} |
174 | 180 |
if (isIdCheck == false) { |
175 |
- alert("아이디 중복 확인을 해주세요."); |
|
181 |
+ alert("아이디 중복확인을 해주세요."); |
|
176 | 182 |
return false; |
177 | 183 |
} |
178 | 184 |
if (CommonUtil.isEmpty(user['user_password']) == true) { |
... | ... | @@ -222,6 +228,31 @@ |
222 | 228 |
} |
223 | 229 |
}).catch((error) => { |
224 | 230 |
console.log('userInsert() /user/userInsert.json error : ', error); |
231 |
+ }); |
|
232 |
+ } |
|
233 |
+ |
|
234 |
+ //보호사 등록 |
|
235 |
+ const agentInsert = () => { |
|
236 |
+ if (userInsertValidation() == false) { |
|
237 |
+ return; |
|
238 |
+ } |
|
239 |
+ |
|
240 |
+ fetch("/user/agentInsert.json", { |
|
241 |
+ method: "POST", |
|
242 |
+ headers: { |
|
243 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
244 |
+ }, |
|
245 |
+ body: JSON.stringify(user), |
|
246 |
+ }).then((response) => response.json()).then((data) => { |
|
247 |
+ console.log("보호사 등록 결과(건수) : ", data); |
|
248 |
+ if (data > 0) { |
|
249 |
+ alert("계정생성완료"); |
|
250 |
+ navigate(-1); |
|
251 |
+ } else { |
|
252 |
+ alert("계정생성에 실패하였습니다. 관리자에게 문의바랍니다."); |
|
253 |
+ } |
|
254 |
+ }).catch((error) => { |
|
255 |
+ console.log('agentInsert() /user/agentInsert.json error : ', error); |
|
225 | 256 |
}); |
226 | 257 |
} |
227 | 258 |
|
... | ... | @@ -351,7 +382,10 @@ |
351 | 382 |
|
352 | 383 |
<div className="btn-wrap"> |
353 | 384 |
<button className={"gray-btn btn-large"} onClick={() => {navigate(-1)}}>취소</button> |
354 |
- <button className={"red-btn btn-large"} onClick={userInsert}>등록</button> |
|
385 |
+ {user['authority'] == "ROLE_AGENCY" |
|
386 |
+ ? <button className={"red-btn btn-large"} onClick={agentInsert}>등록</button> |
|
387 |
+ : <button className={"red-btn btn-large"} onClick={userInsert}>등록</button> |
|
388 |
+ } |
|
355 | 389 |
</div> |
356 | 390 |
|
357 | 391 |
</div> |
--- client/views/pages/senior_management/SeniorEdit.jsx
+++ client/views/pages/senior_management/SeniorEdit.jsx
... | ... | @@ -1,177 +1,353 @@ |
1 | 1 |
import React from "react"; |
2 |
-import Button from "../../component/Button.jsx"; |
|
3 |
-import ContentTitle from "../../component/ContentTitle.jsx"; |
|
4 |
-import { useNavigate } from "react-router"; |
|
2 |
+import { useNavigate, useLocation } from "react-router"; |
|
3 |
+import { useSelector } from "react-redux"; |
|
5 | 4 |
import SubTitle from "../../component/SubTitle.jsx"; |
6 |
-import { useParams } from "react-router"; |
|
5 |
+ |
|
6 |
+import CommonUtil from "../../../resources/js/CommonUtil.js"; |
|
7 | 7 |
|
8 | 8 |
export default function SeniorEdit() { |
9 | 9 |
const navigate = useNavigate(); |
10 |
- let { seniorId } = useParams(); |
|
10 |
+ const location = useLocation(); |
|
11 | 11 |
|
12 |
- const [seniortOne, setSeniorOne] = React.useState([]); |
|
12 |
+ /**** 기본 조회 데이터 (시작) ****/ |
|
13 |
+ //전역 변수 저장 객체 |
|
14 |
+ const state = useSelector((state) => {return state}); |
|
13 | 15 |
|
14 |
- //-------- 상세페이지 선택된 대상자의 정보 불러오기 --------// |
|
15 |
- const getSeniorDataOne = () => { |
|
16 |
- fetch("/user/selectSeniorOne.json", { |
|
16 |
+ //기관 계층 구조 목록 |
|
17 |
+ const [orgListOfHierarchy, setOrgListOfHierarchy] = React.useState([]); |
|
18 |
+ //기관(관리, 시행) 계층 구조 목록 조회 |
|
19 |
+ const orgSelectListOfHierarchy = () => { |
|
20 |
+ fetch("/org/orgSelectListOfHierarchy.json", { |
|
17 | 21 |
method: "POST", |
18 | 22 |
headers: { |
19 | 23 |
'Content-Type': 'application/json; charset=UTF-8' |
20 | 24 |
}, |
21 |
- body: JSON.stringify({ |
|
22 |
- user_id: seniorId |
|
23 |
- }), |
|
25 |
+ body: JSON.stringify({}), |
|
24 | 26 |
}).then((response) => response.json()).then((data) => { |
25 |
- setSeniorOne(data[0]); |
|
26 |
- setTelNum(data[0].user_phonenumber) |
|
27 |
- setHomeAddress(data[0].user_address) |
|
28 |
- setMedicineM(data[0].breakfast_medication_check) |
|
29 |
- setMedicineL(data[0].lunch_medication_check) |
|
30 |
- setMedicineD(data[0].dinner_medication_check) |
|
31 |
- setMedication(data[0].medication_pill) |
|
32 |
- setNote(data[0].senior_note) |
|
33 |
- |
|
27 |
+ console.log("기관(관리, 시행) 계층 구조 목록 조회 : ", data); |
|
28 |
+ setOrgListOfHierarchy(data); |
|
34 | 29 |
}).catch((error) => { |
35 |
- console.log('getSeniorDataOne() /user/selectSeniorOne.json error : ', error); |
|
30 |
+ console.log('orgSelectListOfHierarchy() /org/orgSelectListOfHierarchy.json error : ', error); |
|
36 | 31 |
}); |
37 | 32 |
}; |
33 |
+ const getAgencyList = () => { |
|
34 |
+ const government = orgListOfHierarchy.find(item => item['government_id'] == senior['government_id']); |
|
35 |
+ if (CommonUtil.isEmpty(government) || CommonUtil.isEmpty(government['agencyList'])) { |
|
36 |
+ return []; |
|
37 |
+ } else { |
|
38 |
+ return government['agencyList']; |
|
39 |
+ } |
|
40 |
+ } |
|
38 | 41 |
|
39 |
- //초기값 세팅 |
|
40 |
- const [telNum, setTelNum] = React.useState(""); |
|
41 |
- const [homeAddress, setHomeAddress] = React.useState(""); |
|
42 |
- const [medicineM, setMedicineM] = React.useState(false); |
|
43 |
- const [medicineL, setMedicineL] = React.useState(false); |
|
44 |
- const [medicineD, setMedicineD] = React.useState(false); |
|
45 |
- const [medication, setMedication] = React.useState(""); |
|
46 |
- const [note, setNote] = React.useState(""); |
|
47 |
- |
|
48 |
- //-------- 변경되는 데이터 Handler 설정 --------// |
|
49 |
- const handleTelNum = (e) => { |
|
50 |
- e.target.value = e.target.value.replace(/[^0-9]/g, '').replace(/^(\d{2,3})(\d{3,4})(\d{4})$/, `$1-$2-$3`); |
|
51 |
- setTelNum(e.target.value); |
|
52 |
- }; |
|
53 |
- const handleHomeAddress = (e) => { |
|
54 |
- setHomeAddress(e.target.value); |
|
55 |
- }; |
|
56 |
- const handleMedicineM = (e) => { |
|
57 |
- setMedicineM(e.target.checked); |
|
58 |
- }; |
|
59 |
- const handleMedicineL = (e) => { |
|
60 |
- setMedicineL(e.target.checked); |
|
61 |
- }; |
|
62 |
- const handleMedicineD = (e) => { |
|
63 |
- setMedicineD(e.target.checked); |
|
64 |
- }; |
|
65 |
- const handleMedication = (e) => { |
|
66 |
- setMedication(e.target.value); |
|
67 |
- }; |
|
68 |
- const handleNote = (e) => { |
|
69 |
- setNote(e.target.value); |
|
70 |
- }; |
|
71 |
- |
|
72 |
- const updateSeniorData = () => { |
|
73 |
- console.log("telNum : ", telNum); |
|
74 |
- console.log("homeAddress : ", homeAddress); |
|
75 |
- console.log("note : ", note); |
|
76 |
- console.log("medicineM : ", medicineM); |
|
77 |
- console.log("medicineL : ", medicineL); |
|
78 |
- console.log("medicineD : ", medicineD); |
|
79 |
- fetch("/user/updateSeniorMadication.json", { |
|
42 |
+ const [medicationTimeCodeList, setMedicationTimeCodeList] = React.useState([]); |
|
43 |
+ //복약 시간 코드 목록 조회 |
|
44 |
+ const medicationTimeCodeSelectList = () => { |
|
45 |
+ fetch("/common/medicationTimeCodeSelectList.json", { |
|
80 | 46 |
method: "POST", |
81 | 47 |
headers: { |
82 | 48 |
'Content-Type': 'application/json; charset=UTF-8' |
83 | 49 |
}, |
84 |
- body: JSON.stringify({ |
|
85 |
- user_id: seniorId, |
|
86 |
- user_phonenumber: telNum, |
|
87 |
- user_address: homeAddress, |
|
88 |
- breakfast_medication_check: medicineM, |
|
89 |
- lunch_medication_check: medicineL, |
|
90 |
- dinner_medication_check: medicineD, |
|
91 |
- medication_pill: medication , |
|
92 |
- senior_note: note , |
|
93 |
- }), |
|
50 |
+ body: JSON.stringify({}), |
|
94 | 51 |
}).then((response) => response.json()).then((data) => { |
95 |
- alert("수정 되었습니다."); |
|
96 |
- navigate(`/SeniorSelectOne/${seniorId}`); |
|
52 |
+ console.log("복약 시간 코드 목록 조회 : ", data); |
|
53 |
+ setMedicationTimeCodeList(data); |
|
54 |
+ |
|
55 |
+ //시니어 복약 정보 미리 세팅 |
|
56 |
+ let newSenior = JSON.parse(JSON.stringify(senior)); |
|
57 |
+ for (let i = 0; i < data.length; i++) { |
|
58 |
+ newSenior['seniorMedicationList'].push(data[i]['medication_time_code']); |
|
59 |
+ } |
|
60 |
+ setSenior(newSenior); |
|
97 | 61 |
}).catch((error) => { |
98 |
- console.log('updateSeniorData() /user/updateSeniorMadication.json error : ', error); |
|
62 |
+ console.log('medicationTimeCodeSelectList() /common/medicationTimeCodeSelectList.json error : ', error); |
|
63 |
+ }); |
|
64 |
+ } |
|
65 |
+ /**** 기본 조회 데이터 (종료) ****/ |
|
66 |
+ |
|
67 |
+ |
|
68 |
+ |
|
69 |
+ //시니어 정보 |
|
70 |
+ const [senior, setSenior] = React.useState({ |
|
71 |
+ 'user_id': location.state['senior_id'], |
|
72 |
+ 'user_name': null, |
|
73 |
+ 'user_password': null, |
|
74 |
+ 'user_phonenumber': null, |
|
75 |
+ 'user_birth': null, |
|
76 |
+ 'user_gender': null, |
|
77 |
+ 'user_address': null, |
|
78 |
+ 'user_email': null, |
|
79 |
+ 'authority': 'ROLE_SENIOR', |
|
80 |
+ 'agency_id': null, |
|
81 |
+ 'government_id': null, |
|
82 |
+ |
|
83 |
+ 'senior_id': location.state['senior_id'], |
|
84 |
+ 'care_grade': null, |
|
85 |
+ 'medication_pill': null, |
|
86 |
+ 'underlie_disease': null, |
|
87 |
+ 'senior_note': null, |
|
88 |
+ |
|
89 |
+ 'seniorMedicationList': [] |
|
90 |
+ }); |
|
91 |
+ //시니어 상세 조회 |
|
92 |
+ const seniorSelectOne = () => { |
|
93 |
+ fetch("/user/seniorSelectOne.json", { |
|
94 |
+ method: "POST", |
|
95 |
+ headers: { |
|
96 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
97 |
+ }, |
|
98 |
+ body: JSON.stringify(senior), |
|
99 |
+ }).then((response) => response.json()).then((data) => { |
|
100 |
+ console.log("seniorSelectOne data : ", data); |
|
101 |
+ setSenior(data); |
|
102 |
+ }).catch((error) => { |
|
103 |
+ console.log('seniorSelectOne() /user/seniorSelectOne.json error : ', error); |
|
99 | 104 |
}); |
100 | 105 |
}; |
101 | 106 |
|
107 |
+ //각 데이터별로 Dom 정보 담을 Ref 생성 |
|
108 |
+ const seniorRefInit = JSON.parse(JSON.stringify(senior)); |
|
109 |
+ seniorRefInit['user_gender'] = {}; |
|
110 |
+ const seniorRef = React.useRef(seniorRefInit); |
|
111 |
+ |
|
112 |
+ //등록할 시니어 정보 변경 |
|
113 |
+ const seniorValueChange = (targetKey, value) => { |
|
114 |
+ let newSenior = JSON.parse(JSON.stringify(senior)); |
|
115 |
+ newSenior[targetKey] = value; |
|
116 |
+ setSenior(newSenior); |
|
117 |
+ } |
|
118 |
+ //등록할 시니어의 관리기관 변경 |
|
119 |
+ const seniorGovernmentIdChange = (value) => { |
|
120 |
+ let newSenior = JSON.parse(JSON.stringify(senior)); |
|
121 |
+ if (CommonUtil.isEmpty(value) == true) { |
|
122 |
+ newSenior['government_id'] = null; |
|
123 |
+ } else { |
|
124 |
+ newSenior['government_id'] = value; |
|
125 |
+ } |
|
126 |
+ newSenior['agency_id'] = null; |
|
127 |
+ setSenior(newSenior); |
|
128 |
+ } |
|
129 |
+ //등록할 시니어의 시행기관 변경 |
|
130 |
+ const seniorAgencyIdChange = (value) => { |
|
131 |
+ let newSenior = JSON.parse(JSON.stringify(senior)); |
|
132 |
+ if (CommonUtil.isEmpty(value) == true) { |
|
133 |
+ newSenior['agency_id'] = null; |
|
134 |
+ } else { |
|
135 |
+ newSenior['agency_id'] = value; |
|
136 |
+ } |
|
137 |
+ setSenior(newSenior); |
|
138 |
+ } |
|
139 |
+ //등록할 시니어의 기본 복약 정보 변경 |
|
140 |
+ const seniorMedicationChange = (value, isChecked) => { |
|
141 |
+ let newSenior = JSON.parse(JSON.stringify(senior)); |
|
142 |
+ let index = newSenior['seniorMedicationList'].indexOf(value); |
|
143 |
+ if (isChecked == true && index == -1) { |
|
144 |
+ newSenior['seniorMedicationList'].push(value); |
|
145 |
+ setSenior(newSenior); |
|
146 |
+ } else if (isChecked == false && index > -1) { |
|
147 |
+ newSenior['seniorMedicationList'].splice(index, 1); |
|
148 |
+ setSenior(newSenior); |
|
149 |
+ } else { |
|
150 |
+ return; |
|
151 |
+ } |
|
152 |
+ } |
|
153 |
+ |
|
154 |
+ |
|
155 |
+ //시니어 등록 유효성 검사 |
|
156 |
+ const seniorUpdatetValidation = () => { |
|
157 |
+ if (CommonUtil.isEmpty(senior['government_id']) == true) { |
|
158 |
+ seniorRef.current['government_id'].focus(); |
|
159 |
+ alert("관리기관을 선택해 주세요."); |
|
160 |
+ return false; |
|
161 |
+ } |
|
162 |
+ if (CommonUtil.isEmpty(senior['agency_id']) == true) { |
|
163 |
+ seniorRef.current['agency_id'].focus(); |
|
164 |
+ alert("시행기관을 선택해 주세요."); |
|
165 |
+ return false; |
|
166 |
+ } |
|
167 |
+ if (CommonUtil.isEmpty(senior['user_name']) == true) { |
|
168 |
+ seniorRef.current['user_name'].focus(); |
|
169 |
+ alert("이름을 입력해 주세요."); |
|
170 |
+ return false; |
|
171 |
+ } |
|
172 |
+ if (CommonUtil.isEmpty(senior['user_gender']) == true) { |
|
173 |
+ seniorRef.current['user_gender']['m'].focus(); |
|
174 |
+ alert("성별을 선택해 주세요."); |
|
175 |
+ return false; |
|
176 |
+ } |
|
177 |
+ if (CommonUtil.isEmpty(senior['user_birth']) == true) { |
|
178 |
+ seniorRef.current['user_birth'].focus(); |
|
179 |
+ alert("생년월일을 선택해 주세요."); |
|
180 |
+ return false; |
|
181 |
+ } |
|
182 |
+ if (CommonUtil.isEmpty(senior['user_phonenumber']) == true) { |
|
183 |
+ seniorRef.current['user_phonenumber'].focus(); |
|
184 |
+ alert("연락처를 입력해 주세요."); |
|
185 |
+ return false; |
|
186 |
+ } |
|
187 |
+ if (CommonUtil.isEmpty(senior['user_address']) == true) { |
|
188 |
+ seniorRef.current['user_address'].focus(); |
|
189 |
+ alert("주소를 입력해 주세요."); |
|
190 |
+ return false; |
|
191 |
+ } |
|
192 |
+ |
|
193 |
+ return true; |
|
194 |
+ } |
|
195 |
+ //시니어 등록 |
|
196 |
+ const seniorUpdate = () => { |
|
197 |
+ if (seniorUpdatetValidation() == false) { |
|
198 |
+ return; |
|
199 |
+ } |
|
200 |
+ |
|
201 |
+ fetch("/user/seniorUpdate.json", { |
|
202 |
+ method: "POST", |
|
203 |
+ headers: { |
|
204 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
205 |
+ }, |
|
206 |
+ body: JSON.stringify(senior), |
|
207 |
+ }).then((response) => response.json()).then((data) => { |
|
208 |
+ console.log("시니어 수정 결과(건수) : ", data); |
|
209 |
+ if (data > 0) { |
|
210 |
+ alert("수정완료"); |
|
211 |
+ navigate(-1); |
|
212 |
+ } else { |
|
213 |
+ alert("등록에 실패하였습니다. 관리자에게 문의바랍니다."); |
|
214 |
+ } |
|
215 |
+ }).catch((error) => { |
|
216 |
+ console.log('seniorUpdate() /user/seniorUpdate.json error : ', error); |
|
217 |
+ }); |
|
218 |
+ } |
|
219 |
+ |
|
220 |
+ |
|
221 |
+ //Mounted |
|
102 | 222 |
React.useEffect(() => { |
103 |
- getSeniorDataOne(); |
|
104 |
- |
|
223 |
+ orgSelectListOfHierarchy(); |
|
224 |
+ medicationTimeCodeSelectList(); |
|
225 |
+ seniorSelectOne(); |
|
105 | 226 |
}, []) |
227 |
+ |
|
228 |
+ |
|
106 | 229 |
return ( |
107 | 230 |
<main> |
108 |
- <div className="board-wrap"> |
|
231 |
+ <div className="content-wrap row"> |
|
232 |
+ |
|
233 |
+ <div className="margin-bottom5"> |
|
109 | 234 |
<SubTitle explanation={"수정페이지"} className="margin-bottom" /> |
110 | 235 |
<table className="margin-bottom2 senior-detail"> |
111 |
- {/* <tr> |
|
112 |
- <th>대상자등록번호</th> |
|
113 |
- <td colSpan={3} className="flex"> |
|
114 |
- <input type="text" placeholder="생성하기 버튼 클릭 시 자동으로 생성됩니다."/> |
|
115 |
- <Button |
|
116 |
- className={"btn-large gray-btn"} |
|
117 |
- btnName={"생성하기"} |
|
118 |
- /> |
|
119 |
- </td> |
|
120 |
- </tr> */} |
|
121 | 236 |
<tr> |
122 |
- <th>아이디</th> |
|
237 |
+ <th><span style={{color : "red"}}>*</span>관리기관</th> |
|
123 | 238 |
<td> |
124 |
- <span>{seniortOne.user_id}</span> |
|
239 |
+ <select onChange={(e) => {seniorGovernmentIdChange(e.target.value)}} |
|
240 |
+ ref={el => seniorRef.current['government_id'] = el}> |
|
241 |
+ <option value={''} selected={senior['government_id'] == null}>관리기관선택</option> |
|
242 |
+ {orgListOfHierarchy.map((item, idx) => { return ( |
|
243 |
+ <option key={idx} value={item['government_id']} selected={senior['government_id'] == item['government_id']}> |
|
244 |
+ {item['government_name']} |
|
245 |
+ </option> |
|
246 |
+ )})} |
|
247 |
+ </select> |
|
248 |
+ </td> |
|
249 |
+ <th><span style={{color : "red"}}>*</span>시행기관</th> |
|
250 |
+ <td> |
|
251 |
+ <select onChange={(e) => {seniorAgencyIdChange(e.target.value)}} |
|
252 |
+ ref={el => seniorRef.current['agency_id'] = el}> |
|
253 |
+ <option value={''} selected={senior['agency_id'] == null}>시행기관선택</option> |
|
254 |
+ {getAgencyList().map((item, idx) => { return ( |
|
255 |
+ <option key={idx} value={item['agency_id']} selected={senior['agency_id'] == item['agency_id']}> |
|
256 |
+ {item['agency_name']} |
|
257 |
+ </option> |
|
258 |
+ )})} |
|
259 |
+ </select> |
|
125 | 260 |
</td> |
126 | 261 |
</tr> |
127 | 262 |
<tr> |
128 |
- <th>이름</th> |
|
263 |
+ <th><span style={{color : "red"}}>*</span>이름</th> |
|
129 | 264 |
<td> |
130 |
- <span>{seniortOne.user_name}</span> |
|
265 |
+ <input type="text" |
|
266 |
+ value={senior['user_name']} |
|
267 |
+ onChange={(e) => {seniorValueChange('user_name', e.target.value)}} |
|
268 |
+ ref={el => seniorRef.current['user_name'] = el} |
|
269 |
+ /> |
|
131 | 270 |
</td> |
132 |
- <th>성별</th> |
|
133 |
- <td> |
|
134 |
- <span>{seniortOne.user_gender}</span> |
|
271 |
+ <th><span style={{color : "red"}}>*</span>성별</th> |
|
272 |
+ <td className=""> |
|
273 |
+ <div className="gender flex-start"> |
|
274 |
+ <div className="flex-start"> |
|
275 |
+ <input type="radio" id="user_gender_m" name="user_gender" value="남" checked={senior['user_gender'] == '남'} |
|
276 |
+ onChange={(e) => {e.target.checked ? seniorValueChange('user_gender', e.target.value) : null}} |
|
277 |
+ ref={el => seniorRef.current['user_gender']['m'] = el} |
|
278 |
+ /> |
|
279 |
+ <label for="user_gender_m">남</label> |
|
280 |
+ </div> |
|
281 |
+ <div className="flex-start"> |
|
282 |
+ <input type="radio" id="user_gender_f" name="user_gender" value="여" checked={senior['user_gender'] == '여'} |
|
283 |
+ onChange={(e) => {e.target.checked ? seniorValueChange('user_gender', e.target.value) : null}} |
|
284 |
+ ref={el => seniorRef.current['user_gender']['f'] = el} |
|
285 |
+ /> |
|
286 |
+ <label for="user_gender_f">여</label> |
|
287 |
+ </div> |
|
288 |
+ </div > |
|
135 | 289 |
</td> |
136 | 290 |
</tr> |
137 | 291 |
<tr> |
138 |
- <th>생년월일</th> |
|
292 |
+ <th><span style={{color : "red"}}>*</span>생년월일</th> |
|
139 | 293 |
<td> |
140 |
- <span>{seniortOne.user_birth}</span> |
|
294 |
+ <div className="flex"> |
|
295 |
+ <input type='date' |
|
296 |
+ value={senior['user_birth']} |
|
297 |
+ onChange={(e) => {seniorValueChange('user_birth', e.target.value)}} |
|
298 |
+ ref={el => seniorRef.current['user_birth'] = el} |
|
299 |
+ /> |
|
300 |
+ </div> |
|
141 | 301 |
</td> |
142 | 302 |
</tr> |
143 | 303 |
<tr> |
144 |
- <th>연락처</th> |
|
304 |
+ <th><span style={{color : "red"}}>*</span>연락처</th> |
|
145 | 305 |
<td colSpan={3}> |
146 |
- <input type="text" maxLength="13" value={telNum} onChange={handleTelNum} /> |
|
306 |
+ <input type="number" maxLength="11" |
|
307 |
+ value={senior['user_phonenumber']} |
|
308 |
+ onChange={(e) => {seniorValueChange('user_phonenumber', e.target.value)}} |
|
309 |
+ ref={el => seniorRef.current['user_phonenumber'] = el} |
|
310 |
+ /> |
|
147 | 311 |
</td> |
148 | 312 |
</tr> |
149 | 313 |
<tr> |
150 |
- <th>주소</th> |
|
314 |
+ <th><span style={{color : "red"}}>*</span>주소</th> |
|
151 | 315 |
<td colSpan={3}> |
152 |
- <input type="text" value={homeAddress} onChange={handleHomeAddress} /> |
|
316 |
+ <input type="text" |
|
317 |
+ value={senior['user_address']} |
|
318 |
+ onChange={(e) => {seniorValueChange('user_address', e.target.value)}} |
|
319 |
+ ref={el => seniorRef.current['user_address'] = el} |
|
320 |
+ /> |
|
153 | 321 |
</td> |
154 | 322 |
</tr> |
155 | 323 |
<tr> |
156 | 324 |
<th>필요 복약</th> |
157 |
- <td> |
|
158 |
- <div className="flex-start"> |
|
159 |
- <input type="checkbox" name="medicationSelect" checked={medicineM} onClick={(e) => { handleMedicineM(e) }} /><label for="medicationTime">아침</label> |
|
160 |
- <input type="checkbox" name="medicationSelect" checked={medicineL} onClick={(e) => { handleMedicineL(e) }} /><label for="medicationTime">점심</label> |
|
161 |
- <input type="checkbox" name="medicationSelect" checked={medicineD} onClick={(e) => { handleMedicineD(e) }} /><label for="medicationTime">저녁</label> |
|
325 |
+ <td className="medicationTime-td"> |
|
326 |
+ <div className="flex"> |
|
327 |
+ {medicationTimeCodeList.map((item, idx) => { return ( |
|
328 |
+ <span key={idx}> |
|
329 |
+ <input type="checkbox" |
|
330 |
+ name="medicationTimeCodeList" |
|
331 |
+ id={item['medication_time_code']} |
|
332 |
+ value={item['medication_time_code']} |
|
333 |
+ onChange={(e) => {seniorMedicationChange(e.target.value, e.target.checked)}} |
|
334 |
+ checked={senior['seniorMedicationList'].indexOf(item['medication_time_code']) > -1}/> |
|
335 |
+ <label for={item['medication_time_code']}>{item['medication_time_code_name']}</label> |
|
336 |
+ </span> |
|
337 |
+ )})} |
|
162 | 338 |
</div> |
163 | 339 |
</td> |
164 | 340 |
</tr> |
165 | 341 |
<tr> |
166 | 342 |
<th>복용중인 약</th> |
167 | 343 |
<td colSpan={3}> |
168 |
- <textarea className="medicine" cols="30" rows="2" value={medication} onChange={handleMedication}></textarea> |
|
344 |
+ <textarea className="medicine" cols="30" rows="2" value={senior['medication_pill']} onChange={(e) => {seniorValueChange('medication_pill', e.target.value)}} /> |
|
169 | 345 |
</td> |
170 | 346 |
</tr> |
171 | 347 |
<tr> |
172 | 348 |
<th>비고</th> |
173 | 349 |
<td colSpan={3}> |
174 |
- <textarea className="note" cols="30" rows="2" value={note} onChange={handleNote}></textarea> |
|
350 |
+ <textarea className="note" cols="30" rows="2" value={senior['senior_note']} onChange={(e) => {seniorValueChange('senior_note', e.target.value)}} /> |
|
175 | 351 |
</td> |
176 | 352 |
</tr> |
177 | 353 |
|
... | ... | @@ -182,22 +358,14 @@ |
182 | 358 |
</td> |
183 | 359 |
</tr> */} |
184 | 360 |
</table> |
185 |
- <div className="btn-wrap flex-center"> |
|
186 |
- <Button |
|
187 |
- className={"btn-small gray-btn"} |
|
188 |
- btnName={"이전"} |
|
189 |
- onClick={() => {navigate(-1) |
|
190 |
- }} |
|
191 |
- /> |
|
192 |
- <Button |
|
193 |
- className={"btn-small gray-btn"} |
|
194 |
- btnName={"저장"} |
|
195 |
- onClick={() => { |
|
196 |
- updateSeniorData(); |
|
197 |
- }} |
|
198 |
- /> |
|
199 |
- </div> |
|
200 | 361 |
</div> |
362 |
+ |
|
363 |
+ <div className="btn-wrap flex-center"> |
|
364 |
+ <button className={"btn-large gray-btn"} onClick={() => navigate(-1)}>이전</button> |
|
365 |
+ <button className={"btn-large red-btn"} onClick={seniorUpdate}>수정</button> |
|
366 |
+ </div> |
|
367 |
+ |
|
368 |
+ </div> |
|
201 | 369 |
</main> |
202 | 370 |
); |
203 | 371 |
} |
--- client/views/pages/senior_management/SeniorSelectOne.jsx
+++ client/views/pages/senior_management/SeniorSelectOne.jsx
... | ... | @@ -8,7 +8,6 @@ |
8 | 8 |
export default function SeniorSelectOne() { |
9 | 9 |
const navigate = useNavigate(); |
10 | 10 |
const location = useLocation(); |
11 |
- console.log('location.state : ', location.state); |
|
12 | 11 |
|
13 | 12 |
//보호자 모달 오픈 여부 |
14 | 13 |
const [modalGuardianIsOpen, setModalGuardianIsOpen] = React.useState(false); |
... | ... | @@ -136,7 +135,7 @@ |
136 | 135 |
}, |
137 | 136 |
body: JSON.stringify(user), |
138 | 137 |
}).then((response) => response.json()).then((data) => { |
139 |
- console.log("시니어 등록 결과(건수) : ", data); |
|
138 |
+ console.log("가입승인 결과(건수) : ", data); |
|
140 | 139 |
if (data > 0) { |
141 | 140 |
alert("승인완료"); |
142 | 141 |
callback(); |
... | ... | @@ -195,9 +194,9 @@ |
195 | 194 |
</tr> |
196 | 195 |
|
197 | 196 |
<tr> |
198 |
- <th>생년월일</th> |
|
197 |
+ <th>연락처</th> |
|
199 | 198 |
<td> |
200 |
- <span>{senior['user_birth']}</span> |
|
199 |
+ <span>{senior['user_phonenumber']}</span> |
|
201 | 200 |
</td> |
202 | 201 |
</tr> |
203 | 202 |
|
... | ... | @@ -240,7 +239,7 @@ |
240 | 239 |
</tbody> |
241 | 240 |
</table> |
242 | 241 |
<div className="btn-wrap flex-center"> |
243 |
- <button className={"btn-large gray-btn"} onClick={() => {navigate("/SeniorEdit");}}>수정</button> |
|
242 |
+ <button className={"btn-large gray-btn"} onClick={() => {navigate("/SeniorEdit", {state: {'senior_id': senior['senior_id']}})}}>수정</button> |
|
244 | 243 |
<button className={"btn-large red-btn"} onClick={() => alert("삭제할 수 없습니다.")}>삭제</button> |
245 | 244 |
</div> |
246 | 245 |
</div> |
... | ... | @@ -320,7 +319,7 @@ |
320 | 319 |
<td>{item['agent_match_start_date']}</td> |
321 | 320 |
<td>{item['agent_match_end_date']}</td> |
322 | 321 |
<td> |
323 |
- <button className={"btn-small lightgray-btn"} onClick={() => {}}>상세 페이지 이동</button> |
|
322 |
+ <button className={"btn-small lightgray-btn"} onClick={() => {navigate("/AgentSelectOne", {state: {'agent_id': item['agent_id']}})}}>상세 페이지 이동</button> |
|
324 | 323 |
</td> |
325 | 324 |
</tr> |
326 | 325 |
)})} |
+++ client/views/pages/user_management/AgentSelectOne.jsx
... | ... | @@ -0,0 +1,267 @@ |
1 | +import React from "react"; | |
2 | +import { useNavigate, useLocation } from "react-router"; | |
3 | + | |
4 | +import SubTitle from "../../component/SubTitle.jsx"; | |
5 | +import Modal_Guardian from "../../component/Modal_Guardian.jsx"; | |
6 | +import Modal_SeniorInsert from "../../component/Modal_SeniorInsert.jsx"; | |
7 | + | |
8 | + | |
9 | +export default function AgentSelectOne() { | |
10 | + const navigate = useNavigate(); | |
11 | + const location = useLocation(); | |
12 | + | |
13 | + | |
14 | + //대상자(시니어) 등록 모달 오픈 여부 | |
15 | + const [modalSeniorInsertIsOpen, setModalSeniorInsertIsOpen] = React.useState(false); | |
16 | + //대상자(시니어) 등록 모달 오픈 | |
17 | + const modalSeniorInsertOpen = () => { | |
18 | + setModalSeniorInsertIsOpen(true); | |
19 | + }; | |
20 | + //대상자(시니어) 등록 모달 닫기 | |
21 | + const modalSeniorInsertClose = () => { | |
22 | + setModalSeniorInsertIsOpen(false); | |
23 | + }; | |
24 | + | |
25 | + | |
26 | + //시니어 정보 | |
27 | + const [agent, setAgent] = React.useState({ | |
28 | + 'user_id': location.state['agent_id'], | |
29 | + 'user_name': null, | |
30 | + 'user_password': null, | |
31 | + 'user_phonenumber': null, | |
32 | + 'user_birth': null, | |
33 | + 'user_gender': null, | |
34 | + 'user_address': null, | |
35 | + 'user_email': null, | |
36 | + 'authority': 'ROLE_AGENCY', | |
37 | + 'agency_id': null, | |
38 | + 'government_id': null, | |
39 | + | |
40 | + 'agent_id': location.state['agent_id'], | |
41 | + }); | |
42 | + //시니어 상세 조회 | |
43 | + const agentSelectOne = () => { | |
44 | + fetch("/user/userSelectOne.json", { | |
45 | + method: "POST", | |
46 | + headers: { | |
47 | + 'Content-Type': 'application/json; charset=UTF-8' | |
48 | + }, | |
49 | + body: JSON.stringify(agent), | |
50 | + }).then((response) => response.json()).then((data) => { | |
51 | + console.log("agentSelectOne data : ", data); | |
52 | + data['agent_id'] = location.state['agent_id']; | |
53 | + setAgent(data); | |
54 | + }).catch((error) => { | |
55 | + console.log('agentSelectOne() /user/userSelectOne.json error : ', error); | |
56 | + }); | |
57 | + }; | |
58 | + | |
59 | + //보호사의 대상자 목록 | |
60 | + const [seniorByAgent, setSeniorByAgent] = React.useState({seniorListByAgent: [], seniorListCountByAgent: 0}); | |
61 | + //보호사의 선택한 대상자 정보 | |
62 | + const [senior, setSenior] = React.useState({}); | |
63 | + //보호사의 대상자 목록 조회 | |
64 | + const seniorSelectListByAgent = () => { | |
65 | + fetch("/user/seniorSelectListByAgent.json", { | |
66 | + method: "POST", | |
67 | + headers: { | |
68 | + 'Content-Type': 'application/json; charset=UTF-8' | |
69 | + }, | |
70 | + body: JSON.stringify(agent), | |
71 | + }).then((response) => response.json()).then((data) => { | |
72 | + console.log("seniorSelectListByAgent data : ", data); | |
73 | + setSeniorByAgent(data); | |
74 | + }).catch((error) => { | |
75 | + console.log('seniorSelectListByAgent() /user/seniorSelectListByAgent.json error : ', error); | |
76 | + }); | |
77 | + }; | |
78 | + | |
79 | + //선택한 보호자 정보 관리 | |
80 | + const guardianByAgentManagement = (item) => { | |
81 | + setSenior(item); | |
82 | + } | |
83 | + | |
84 | + //가입승인 | |
85 | + const userUpdate = () => { | |
86 | + agent['is_accept'] = true; | |
87 | + | |
88 | + fetch("/user/userUpdate.json", { | |
89 | + method: "POST", | |
90 | + headers: { | |
91 | + 'Content-Type': 'application/json; charset=UTF-8' | |
92 | + }, | |
93 | + body: JSON.stringify(agent), | |
94 | + }).then((response) => response.json()).then((data) => { | |
95 | + console.log("가입승인 결과(건수) : ", data); | |
96 | + if (data > 0) { | |
97 | + alert("승인완료"); | |
98 | + agentSelectOne(); | |
99 | + } else { | |
100 | + alert("승인에 실패하였습니다. 관리자에게 문의바랍니다."); | |
101 | + } | |
102 | + }).catch((error) => { | |
103 | + console.log('userUpdate() /user/userUpdate.json error : ', error); | |
104 | + }); | |
105 | + } | |
106 | + | |
107 | + | |
108 | + | |
109 | + //보호자의 대상자 제거 | |
110 | + const agentSeniorDelete = (senior) => { | |
111 | + if (confirm('해당 보호대상자의 케어를 종료하시겠습니까?') == false) { | |
112 | + return; | |
113 | + } | |
114 | + | |
115 | + fetch("/user/agentSeniorDelete.json", { | |
116 | + method: "POST", | |
117 | + headers: { | |
118 | + 'Content-Type': 'application/json; charset=UTF-8' | |
119 | + }, | |
120 | + body: JSON.stringify(senior), | |
121 | + }).then((response) => response.json()).then((data) => { | |
122 | + console.log("보호자의 대상자 제거 결과(건수) : ", data); | |
123 | + if (data > 0) { | |
124 | + alert("케어종료완료"); | |
125 | + seniorSelectListByAgent(); | |
126 | + } else { | |
127 | + alert("케어종료에 실패하였습니다. 관리자에게 문의바랍니다."); | |
128 | + } | |
129 | + }).catch((error) => { | |
130 | + console.log('agentSeniorDelete() /user/agentSeniorDelete.json error : ', error); | |
131 | + }); | |
132 | + } | |
133 | + | |
134 | + React.useEffect(() => { | |
135 | + agentSelectOne(); | |
136 | + seniorSelectListByAgent(); | |
137 | + }, []) | |
138 | + | |
139 | + | |
140 | + return ( | |
141 | + <main> | |
142 | + <Modal_SeniorInsert | |
143 | + open={modalSeniorInsertIsOpen} | |
144 | + close={modalSeniorInsertClose} | |
145 | + seniorInsertCallback={() => {seniorSelectListByAgent(); modalSeniorInsertClose();}} | |
146 | + defaultAgentId={agent['agent_id']} | |
147 | + defaultAgencyId={agent['agency_id']} | |
148 | + defaultGovernmentId={agent['government_id']} | |
149 | + /> | |
150 | + | |
151 | + <div className="content-wrap row"> | |
152 | + <SubTitle explanation={"대상자 상세 프로필"} className="margin-bottom" /> | |
153 | + <div className="margin-bottom5"> | |
154 | + <table className="margin-bottom senior-detail"> | |
155 | + <tbody> | |
156 | + <tr> | |
157 | + <th>관리기관</th> | |
158 | + <td> | |
159 | + <span>{agent['government_name']}</span> | |
160 | + </td> | |
161 | + </tr> | |
162 | + | |
163 | + <tr> | |
164 | + <th>시행기관</th> | |
165 | + <td> | |
166 | + <span>{agent['agency_name']}</span> | |
167 | + </td> | |
168 | + </tr> | |
169 | + | |
170 | + <tr> | |
171 | + <th>이름(아이디)</th> | |
172 | + <td> | |
173 | + <span>{agent['user_name']}({agent['user_id']})</span> | |
174 | + </td> | |
175 | + </tr> | |
176 | + | |
177 | + <tr> | |
178 | + <th>연락처</th> | |
179 | + <td> | |
180 | + <span>{agent['user_phonenumber']}</span> | |
181 | + </td> | |
182 | + </tr> | |
183 | + | |
184 | + <tr> | |
185 | + <th>이메일</th> | |
186 | + <td> | |
187 | + <span>{agent['user_email']}</span> | |
188 | + </td> | |
189 | + </tr> | |
190 | + | |
191 | + <tr> | |
192 | + <th>가입승인관리</th> | |
193 | + <td> | |
194 | + {agent['is_accept'] ? <span>승인완료</span> : | |
195 | + <button className={"btn-small red-btn"} onClick={userUpdate}>가입승인</button> | |
196 | + } | |
197 | + </td> | |
198 | + </tr> | |
199 | + | |
200 | + </tbody> | |
201 | + </table> | |
202 | + <div className="btn-wrap flex-center"> | |
203 | + <button className={"btn-large gray-btn"} onClick={() => {navigate("/UserEdit", {state: {'user_id': agent['user_id']}})}}>수정</button> | |
204 | + <button className={"btn-large red-btn"} onClick={() => alert("삭제할 수 없습니다.")}>삭제</button> | |
205 | + </div> | |
206 | + </div> | |
207 | + | |
208 | + <SubTitle explanation={"보호사의 보호대상자"} className="margin-bottom display-inline-block" /> | |
209 | + <button className={"btn-small gray-btn display-inline-block float-right"} onClick={() => modalSeniorInsertOpen()}>보호대상자 추가</button> | |
210 | + <div className="margin-bottom5"> | |
211 | + <table className={"agent-user"}> | |
212 | + <thead> | |
213 | + <tr> | |
214 | + <th>No</th> | |
215 | + {/* <th>관리기관명</th> | |
216 | + <th>소속기관명</th> */} | |
217 | + <th>이름</th> | |
218 | + <th>연락처</th> | |
219 | + <th>생년월일</th> | |
220 | + <th>성별</th> | |
221 | + <th>상태</th> | |
222 | + <th>배정시작일</th> | |
223 | + <th>배정종료일</th> | |
224 | + <th>상세보기</th> | |
225 | + </tr> | |
226 | + </thead> | |
227 | + <tbody> | |
228 | + {seniorByAgent.seniorListByAgent.map((item, idx) => { return ( | |
229 | + <tr key={idx}> | |
230 | + <td>{idx + 1}</td> | |
231 | + {/* <td>{item['government_name']}</td> | |
232 | + <td>{item['agency_name']}</td> */} | |
233 | + <td>{item['user_name']}</td> | |
234 | + <td>{item['user_phonenumber']}</td> | |
235 | + <td>{item['user_birth']}</td> | |
236 | + <td>{item['user_gender']}</td> | |
237 | + <td>{item['agent_match_state'] ? <span className="green">케어중</span> : <span className="grey">케어완료</span>}</td> | |
238 | + <td>{item['agent_match_start_date']}</td> | |
239 | + <td> | |
240 | + {item['agent_match_state'] ? | |
241 | + <button className={"btn-small red-btn"} onClick={() => {agentSeniorDelete(item)}}>케어종료</button> | |
242 | + : item['agent_match_end_date'] | |
243 | + } | |
244 | + </td> | |
245 | + <td> | |
246 | + <button className={"btn-small lightgray-btn"} onClick={() => {navigate("/SeniorSelectOne", {state: {'senior_id': item['user_id']}})}}>상세 페이지 이동</button> | |
247 | + </td> | |
248 | + </tr> | |
249 | + )})} | |
250 | + {seniorByAgent.seniorListByAgent == null || seniorByAgent.seniorListByAgent.length == 0 ? | |
251 | + <tr> | |
252 | + <td colSpan={9}>조회된 데이터가 없습니다</td> | |
253 | + </tr> | |
254 | + : null} | |
255 | + </tbody> | |
256 | + </table> | |
257 | + </div> | |
258 | + | |
259 | + <div className="btn-wrap flex-center"> | |
260 | + <button className={"btn-large gray-btn"} onClick={() => navigate(-1)}>이전</button> | |
261 | + </div> | |
262 | + | |
263 | + </div> | |
264 | + | |
265 | + </main> | |
266 | + ); | |
267 | +} |
--- client/views/pages/user_management/UserAuthoriySelect.jsx
+++ client/views/pages/user_management/UserAuthoriySelect.jsx
... | ... | @@ -278,7 +278,6 @@ |
278 | 278 |
|
279 | 279 |
//사용자 등록 페이지 이동 |
280 | 280 |
const join = () => { |
281 |
- console.log('userSearch : ', userSearch); |
|
282 | 281 |
navigate("/Join", {state: { |
283 | 282 |
'government_id': userSearch['government_id'], |
284 | 283 |
'agency_id': userSearch['agency_id'], |
... | ... | @@ -416,8 +415,8 @@ |
416 | 415 |
</div> |
417 | 416 |
|
418 | 417 |
<div className="btn-wrap flex margin-bottom"> |
419 |
- <SubTitle explanation={"대상자 클릭 시 상세페이지로 이동합니다."} /> |
|
420 |
- <button className={"btn-small gray-btn"} onClick={() => modalSeniorInsertOpen()}>등록</button> |
|
418 |
+ <SubTitle explanation={"대상자 클릭 시 상세페이지로 이동합니다."} /> |
|
419 |
+ {/* <button className={"btn-small gray-btn"} onClick={() => modalSeniorInsertOpen()}>등록</button> */} |
|
421 | 420 |
</div> |
422 | 421 |
|
423 | 422 |
<table className={"protector-user"}> |
... | ... | @@ -515,7 +514,7 @@ |
515 | 514 |
} |
516 | 515 |
</td> |
517 | 516 |
<td> |
518 |
- <button className={"btn-small lightgray-btn"} onClick={() => modalGuardianOpen()}>상세 페이지 이동</button> |
|
517 |
+ <button className={"btn-small lightgray-btn"} onClick={() => {navigate("/AgentSelectOne", {state: {'agent_id': item['user_id']}})}}>상세 페이지 이동</button> |
|
519 | 518 |
</td> |
520 | 519 |
</tr> |
521 | 520 |
)})} |
... | ... | @@ -588,7 +587,7 @@ |
588 | 587 |
} |
589 | 588 |
</td> |
590 | 589 |
<td> |
591 |
- <button className={"btn-small lightgray-btn"} onClick={() => modalGuardianOpen()}>정보 수정</button> |
|
590 |
+ <button className={"btn-small lightgray-btn"} onClick={() => {navigate("/UserEdit", {state: {'user_id': item['user_id']}})}}>정보 수정</button> |
|
592 | 591 |
</td> |
593 | 592 |
</tr> |
594 | 593 |
)})} |
... | ... | @@ -653,7 +652,7 @@ |
653 | 652 |
} |
654 | 653 |
</td> |
655 | 654 |
<td> |
656 |
- <button className={"btn-small lightgray-btn"} onClick={() => modalGuardianOpen()}>정보 수정</button> |
|
655 |
+ <button className={"btn-small lightgray-btn"} onClick={() => {navigate("/UserEdit", {state: {'user_id': item['user_id']}})}}>정보 수정</button> |
|
657 | 656 |
</td> |
658 | 657 |
</tr> |
659 | 658 |
)})} |
+++ client/views/pages/user_management/UserEdit.jsx
... | ... | @@ -0,0 +1,362 @@ |
1 | +import React from "react"; | |
2 | +import { useNavigate, useLocation } from "react-router"; | |
3 | +import { useSelector } from "react-redux"; | |
4 | + | |
5 | +import CommonUtil from "../../../resources/js/CommonUtil.js"; | |
6 | + | |
7 | +export default function UserEdit() { | |
8 | + const navigate = useNavigate(); | |
9 | + const location = useLocation(); | |
10 | + | |
11 | + /**** 기본 조회 데이터 (시작) ****/ | |
12 | + //전역 변수 저장 객체 | |
13 | + const state = useSelector((state) => {return state}); | |
14 | + | |
15 | + //권한 타입 종류 | |
16 | + const [authorities, setAuthorities] = React.useState([]); | |
17 | + //권한 타입 종류 목록 조회 | |
18 | + const authoritiesSelect = () => { | |
19 | + fetch("/common/systemCode/authoritiesSelect.json", { | |
20 | + method: "POST", | |
21 | + headers: { | |
22 | + 'Content-Type': 'application/json; charset=UTF-8' | |
23 | + }, | |
24 | + body: JSON.stringify({}), | |
25 | + }).then((response) => response.json()).then((data) => { | |
26 | + console.log("권한 타입 종류 목록 조회 : ", data); | |
27 | + setAuthorities(data); | |
28 | + }).catch((error) => { | |
29 | + console.log('authoritiesSelect() /common/systemCode/authoritiesSelect.json error : ', error); | |
30 | + }); | |
31 | + }; | |
32 | + //기관 계층 구조 목록 | |
33 | + const [orgListOfHierarchy, setOrgListOfHierarchy] = React.useState([]); | |
34 | + //기관(관리, 시행) 계층 구조 목록 조회 | |
35 | + const orgSelectListOfHierarchy = () => { | |
36 | + fetch("/org/orgSelectListOfHierarchy.json", { | |
37 | + method: "POST", | |
38 | + headers: { | |
39 | + 'Content-Type': 'application/json; charset=UTF-8' | |
40 | + }, | |
41 | + body: JSON.stringify({}), | |
42 | + }).then((response) => response.json()).then((data) => { | |
43 | + console.log("기관(관리, 시행) 계층 구조 목록 조회 : ", data); | |
44 | + setOrgListOfHierarchy(data); | |
45 | + }).catch((error) => { | |
46 | + console.log('orgSelectListOfHierarchy() /org/orgSelectListOfHierarchy.json error : ', error); | |
47 | + }); | |
48 | + }; | |
49 | + const getAgencyList = () => { | |
50 | + const government = orgListOfHierarchy.find(item => item['government_id'] == user['government_id']); | |
51 | + if (CommonUtil.isEmpty(government) || CommonUtil.isEmpty(government['agencyList'])) { | |
52 | + return []; | |
53 | + } else { | |
54 | + return government['agencyList']; | |
55 | + } | |
56 | + } | |
57 | + /**** 기본 조회 데이터 (종료) ****/ | |
58 | + | |
59 | + //로그인 중복 확인 | |
60 | + const [isIdCheck, setIsIdCheck] = React.useState(true); | |
61 | + | |
62 | + //수정할 사용자 정보 | |
63 | + const [user, setUser] = React.useState({ | |
64 | + 'user_id': location.state['user_id'], | |
65 | + 'user_name': null, | |
66 | + 'user_password': null, | |
67 | + 'user_password_check': null, | |
68 | + 'user_phonenumber': null, | |
69 | + 'user_birth': null, | |
70 | + 'user_gender': null, | |
71 | + 'user_address': null, | |
72 | + 'user_email': null, | |
73 | + 'authority': null, | |
74 | + 'agency_id': null, | |
75 | + 'government_id': null, | |
76 | + }); | |
77 | + //각 데이터별로 Dom 정보 담을 Ref 생성 | |
78 | + const userRefInit = JSON.parse(JSON.stringify(user)); | |
79 | + userRefInit['user_gender'] = {}; | |
80 | + userRefInit['user_id_check_button'] = null; | |
81 | + const userRef = React.useRef(userRefInit); | |
82 | + | |
83 | + //사용자 정보 조회 | |
84 | + const userSelectOne = () => { | |
85 | + fetch("/user/userSelectOne.json", { | |
86 | + method: "POST", | |
87 | + headers: { | |
88 | + 'Content-Type': 'application/json; charset=UTF-8' | |
89 | + }, | |
90 | + body: JSON.stringify(user), | |
91 | + }).then((response) => response.json()).then((data) => { | |
92 | + console.log("사용자 정보 조회 : ", data); | |
93 | + setUser(data); | |
94 | + }).catch((error) => { | |
95 | + console.log('userSelectOne() /user/userSelectOne.json error : ', error); | |
96 | + }); | |
97 | + } | |
98 | + | |
99 | + //수정할 사용자 정보 변경 | |
100 | + const userValueChange = (targetKey, value) => { | |
101 | + let newUser = JSON.parse(JSON.stringify(user)); | |
102 | + newUser[targetKey] = value; | |
103 | + setUser(newUser); | |
104 | + } | |
105 | + //수정할 사용자의 관리기관 변경 | |
106 | + const userGovernmentIdChange = (value) => { | |
107 | + let newUser = JSON.parse(JSON.stringify(user)); | |
108 | + if (CommonUtil.isEmpty(value) == true) { | |
109 | + newUser['government_id'] = null; | |
110 | + } else { | |
111 | + newUser['government_id'] = value; | |
112 | + } | |
113 | + newUser['agency_id'] = null; | |
114 | + setUser(newUser); | |
115 | + } | |
116 | + //수정할 사용자의 시행기관 변경 | |
117 | + const userAgencyIdChange = (value) => { | |
118 | + let newUser = JSON.parse(JSON.stringify(user)); | |
119 | + if (CommonUtil.isEmpty(value) == true) { | |
120 | + newUser['agency_id'] = null; | |
121 | + } else { | |
122 | + newUser['agency_id'] = value; | |
123 | + } | |
124 | + setUser(newUser); | |
125 | + } | |
126 | + //로그인 아이디 중복 검사 | |
127 | + const userIdCheck = () => { | |
128 | + if (CommonUtil.isEmpty(user['user_id']) == true) { | |
129 | + userRef.current['user_id'].focus(); | |
130 | + alert("아이디를 입력해 주세요."); | |
131 | + return; | |
132 | + } | |
133 | + | |
134 | + fetch("/user/userSelectOne.json", { | |
135 | + method: "POST", | |
136 | + headers: { | |
137 | + 'Content-Type': 'application/json; charset=UTF-8' | |
138 | + }, | |
139 | + body: JSON.stringify(user), | |
140 | + }).then((response) => response.json()).then((data) => { | |
141 | + console.log("로그인 아이디 중복 검사(아이디를 통한 사용자 조회) : ", data); | |
142 | + if (CommonUtil.isEmpty(data) == true) { | |
143 | + setIsIdCheck(true); | |
144 | + userRef.current['user_password'].focus(); | |
145 | + alert("사용가능한 아이디 입니다."); | |
146 | + } else { | |
147 | + setIsIdCheck(false); | |
148 | + userRef.current['user_id'].focus(); | |
149 | + alert("이미 존재하는 아이디 입니다."); | |
150 | + } | |
151 | + }).catch((error) => { | |
152 | + console.log('userIdCheck() /user/userSelectOne.json error : ', error); | |
153 | + }); | |
154 | + } | |
155 | + | |
156 | + | |
157 | + //사용자 등록 유효성 검사 | |
158 | + const userUpdateValidation = () => { | |
159 | + if ((user['authority'] == 'ROLE_GOVERNMENT' || user['authority'] == 'ROLE_AGENCY') | |
160 | + && CommonUtil.isEmpty(user['government_id']) == true) { | |
161 | + userRef.current['government_id'].focus(); | |
162 | + alert("관리기관을 선택해 주세요."); | |
163 | + return false; | |
164 | + } | |
165 | + | |
166 | + if (user['authority'] == 'ROLE_AGENCY' && CommonUtil.isEmpty(user['agency_id']) == true) { | |
167 | + userRef.current['agency_id'].focus(); | |
168 | + alert("시행기관을 선택해 주세요."); | |
169 | + return false; | |
170 | + } | |
171 | + | |
172 | + if (CommonUtil.isEmpty(user['user_name']) == true) { | |
173 | + userRef.current['user_name'].focus(); | |
174 | + alert("이름을 입력해 주세요."); | |
175 | + return false; | |
176 | + } | |
177 | + if (CommonUtil.isEmpty(user['user_id']) == true) { | |
178 | + userRef.current['user_id'].focus(); | |
179 | + alert("아이디를 입력해 주세요."); | |
180 | + return false; | |
181 | + } | |
182 | + if (isIdCheck == false) { | |
183 | + alert("아이디 중복 확인을 해주세요."); | |
184 | + return false; | |
185 | + } | |
186 | + if (CommonUtil.isEmpty(user['user_password']) == false | |
187 | + && user['user_password'] != user['user_password_check']) { | |
188 | + userRef.current['user_password_check'].focus(); | |
189 | + alert("비밀번호가 일치하지 않습니다."); | |
190 | + return false; | |
191 | + } | |
192 | + if (CommonUtil.isEmpty(user['user_phonenumber']) == true) { | |
193 | + userRef.current['user_phonenumber'].focus(); | |
194 | + alert("연락처를 입력해 주세요."); | |
195 | + return false; | |
196 | + } | |
197 | + | |
198 | + /* if (CommonUtil.isEmpty(user['user_email']) == true) { | |
199 | + userRef.current['user_email'].focus(); | |
200 | + alert("이메일을 입력해 주세요."); | |
201 | + return false; | |
202 | + } */ | |
203 | + | |
204 | + return true; | |
205 | + } | |
206 | + | |
207 | + //사용자 등록 | |
208 | + const userUpdate = () => { | |
209 | + if (userUpdateValidation() == false) { | |
210 | + return; | |
211 | + } | |
212 | + | |
213 | + fetch("/user/userUpdate.json", { | |
214 | + method: "POST", | |
215 | + headers: { | |
216 | + 'Content-Type': 'application/json; charset=UTF-8' | |
217 | + }, | |
218 | + body: JSON.stringify(user), | |
219 | + }).then((response) => response.json()).then((data) => { | |
220 | + console.log("사용자 수정 결과(건수) : ", data); | |
221 | + if (data > 0) { | |
222 | + alert("계정수정완료"); | |
223 | + navigate(-1); | |
224 | + } else { | |
225 | + alert("계정수정에 실패하였습니다. 관리자에게 문의바랍니다."); | |
226 | + } | |
227 | + }).catch((error) => { | |
228 | + console.log('userUpdate() /user/userUpdate.json error : ', error); | |
229 | + }); | |
230 | + } | |
231 | + | |
232 | + //Mounted | |
233 | + React.useEffect(() => { | |
234 | + authoritiesSelect(); | |
235 | + orgSelectListOfHierarchy(); | |
236 | + userSelectOne(); | |
237 | + }, []); | |
238 | + | |
239 | + | |
240 | + return ( | |
241 | + <div className="container row flex-center join-login"> | |
242 | + <div className="join-group"> | |
243 | + <h3>계정수정</h3> | |
244 | + <div className="join-inner"> | |
245 | + <div> | |
246 | + <div className="flex-start margin-bottom2"> | |
247 | + <label className="flex25"><span style={{color : "red"}}>*</span>사용자구분</label> | |
248 | + {authorities[user['authority']]} | |
249 | + </div> | |
250 | + </div> | |
251 | + | |
252 | + {user['authority'] == 'ROLE_GOVERNMENT' || user['authority'] == 'ROLE_AGENCY' ? | |
253 | + <div> | |
254 | + <div className="flex-start margin-bottom2"> | |
255 | + <label className="flex25" htmlFor="name"><span style={{color : "red"}}>*</span>관리기관</label> | |
256 | + <select onChange={(e) => {userGovernmentIdChange(e.target.value)}} ref={el => userRef.current['government_id'] = el}> | |
257 | + <option value={''} selected={user['government_id'] == null}>관리기관선택</option> | |
258 | + {orgListOfHierarchy.map((item, idx) => { return ( | |
259 | + <option key={idx} value={item['government_id']} selected={user['government_id'] == item['government_id']}> | |
260 | + {item['government_name']} | |
261 | + </option> | |
262 | + )})} | |
263 | + </select> | |
264 | + </div> | |
265 | + </div> | |
266 | + : null} | |
267 | + | |
268 | + {user['authority'] == 'ROLE_AGENCY' ? | |
269 | + <div> | |
270 | + <div className="flex-start margin-bottom2"> | |
271 | + <label className="flex25" htmlFor="name"><span style={{color : "red"}}>*</span>시행기관</label> | |
272 | + <select onChange={(e) => {userAgencyIdChange(e.target.value)}} ref={el => userRef.current['agency_id'] = el}> | |
273 | + <option value={''} selected={user['agency_id'] == null}>시행기관선택</option> | |
274 | + {getAgencyList().map((item, idx) => { return ( | |
275 | + <option key={idx} value={item['agency_id']} selected={user['agency_id'] == item['agency_id']}> | |
276 | + {item['agency_name']} | |
277 | + </option> | |
278 | + )})} | |
279 | + </select> | |
280 | + </div> | |
281 | + </div> | |
282 | + : null} | |
283 | + | |
284 | + <div> | |
285 | + <div className="flex-start margin-bottom2"> | |
286 | + <label className="flex25" htmlFor="name"><span style={{color : "red"}}>*</span>이름</label> | |
287 | + <input type="text" | |
288 | + value={user['user_name']} | |
289 | + onChange={(e) => {userValueChange('user_name', e.target.value)}} | |
290 | + ref={el => userRef.current['user_name'] = el} | |
291 | + /> | |
292 | + </div> | |
293 | + </div> | |
294 | + | |
295 | + <div className="id"> | |
296 | + <div className="flex-start margin-bottom2"> | |
297 | + <label className="flex25" htmlFor="id"><span style={{color : "red"}}>*</span>아이디</label> | |
298 | + <input type="text" | |
299 | + value={user['user_id']} | |
300 | + onChange={(e) => {userValueChange('user_id', e.target.value); setIsIdCheck(false)}} | |
301 | + ref={el => userRef.current['user_id'] = el} | |
302 | + /> | |
303 | + <button className={"red-btn btn-large"} onClick={userIdCheck} | |
304 | + ref={el => userRef.current['user_id_check_button'] = el}> | |
305 | + 중복확인 | |
306 | + </button> | |
307 | + </div> | |
308 | + </div> | |
309 | + | |
310 | + <div> | |
311 | + <div className="flex-start margin-bottom2"> | |
312 | + <label className="flex25" htmlFor="password"><span style={{color : "red"}}>*</span>비밀번호</label> | |
313 | + <input type="password" | |
314 | + value={user['user_password']} | |
315 | + onChange={(e) => {userValueChange('user_password', e.target.value)}} | |
316 | + ref={el => userRef.current['user_password'] = el} | |
317 | + /> | |
318 | + </div> | |
319 | + </div> | |
320 | + <div> | |
321 | + <div className="flex-start margin-bottom2"> | |
322 | + <label className="flex25" htmlFor="password_check"><span style={{color : "red"}}>*</span>비밀번호 확인</label> | |
323 | + <input type="password" | |
324 | + value={user['user_password_check']} | |
325 | + onChange={(e) => {userValueChange('user_password_check', e.target.value)}} | |
326 | + ref={el => userRef.current['user_password_check'] = el} | |
327 | + /> | |
328 | + </div> | |
329 | + </div> | |
330 | + | |
331 | + <div> | |
332 | + <div className="flex margin-bottom2"> | |
333 | + <label className="flex25" htmlFor="phone_number"><span style={{color : "red"}}>*</span>전화번호</label> | |
334 | + <input type="number" maxLength="11" | |
335 | + value={user['user_phonenumber']} | |
336 | + onChange={(e) => {userValueChange('user_phonenumber', e.target.value)}} | |
337 | + ref={el => userRef.current['user_phonenumber'] = el} | |
338 | + /> | |
339 | + </div> | |
340 | + </div> | |
341 | + | |
342 | + <div> | |
343 | + <div className="flex-start margin-bottom2"> | |
344 | + <label className="flex25" htmlFor="password_check">이메일</label> | |
345 | + <input type="text" | |
346 | + value={user['user_email']} | |
347 | + onChange={(e) => {userValueChange('user_email', e.target.value)}} | |
348 | + ref={el => userRef.current['user_email'] = el} | |
349 | + /> | |
350 | + </div> | |
351 | + </div> | |
352 | + | |
353 | + <div className="btn-wrap"> | |
354 | + <button className={"gray-btn btn-large"} onClick={() => {navigate(-1)}}>취소</button> | |
355 | + <button className={"red-btn btn-large"} onClick={userUpdate}>수정</button> | |
356 | + </div> | |
357 | + | |
358 | + </div> | |
359 | + </div> | |
360 | + </div> | |
361 | + ); | |
362 | +} |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?