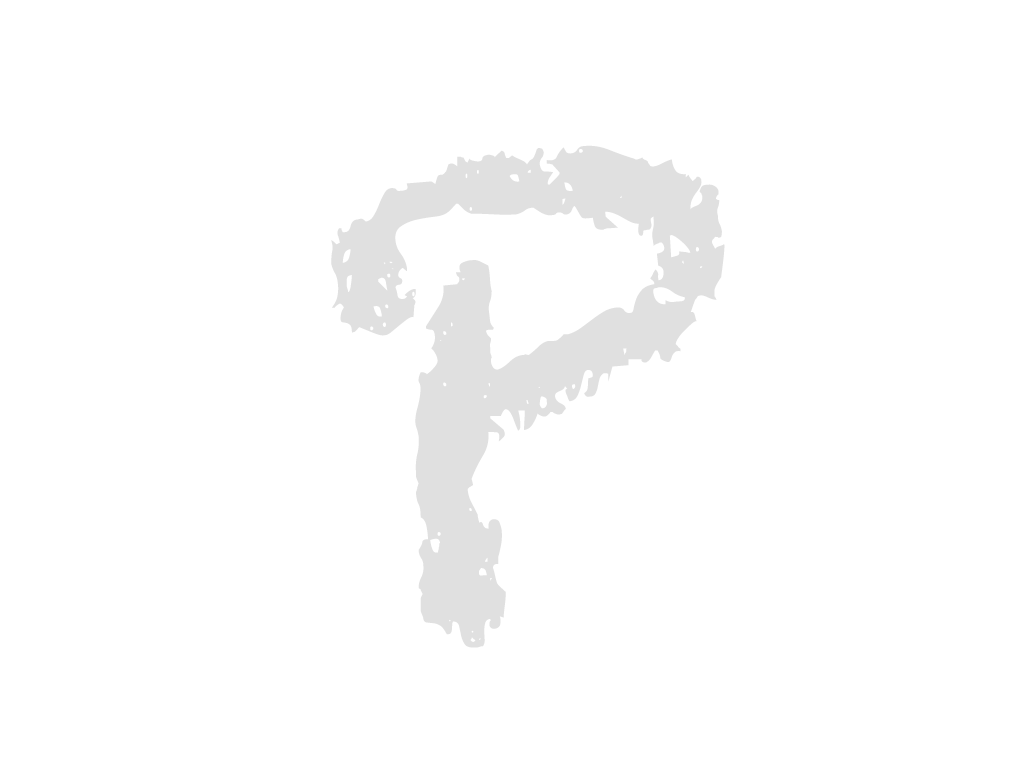
--- client/views/component/Modal_Guardian.jsx
+++ client/views/component/Modal_Guardian.jsx
... | ... | @@ -1,11 +1,118 @@ |
1 | 1 |
import React from "react"; |
2 |
-import Button from "./Button.jsx"; |
|
3 | 2 |
import SubTitle from "./SubTitle.jsx"; |
4 |
-import Table from "./Table.jsx"; |
|
5 |
-import Pagination from "./Pagination.jsx"; |
|
3 |
+import CommonUtil from "../../resources/js/CommonUtil.js"; |
|
4 |
+ |
|
5 |
+export default function Modal_Guardian({ open, close, guardianManagementCallback, seniorId, guardianListBySenior }) { |
|
6 |
+ |
|
7 |
+ //시니어 정보 |
|
8 |
+ const [guardian, setGuardian] = React.useState({ |
|
9 |
+ 'user_id': null, |
|
10 |
+ 'user_name': null, |
|
11 |
+ 'user_password': null, |
|
12 |
+ 'user_phonenumber': null, |
|
13 |
+ 'user_birth': null, |
|
14 |
+ 'user_gender': null, |
|
15 |
+ 'user_address': null, |
|
16 |
+ 'user_email': null, |
|
17 |
+ 'authority': 'ROLE_GUARDIAN', |
|
18 |
+ 'agency_id': null, |
|
19 |
+ 'government_id': null, |
|
20 |
+ |
|
21 |
+ 'senior_id': seniorId, |
|
22 |
+ 'senior_relationship': '자녀', |
|
23 |
+ 'senior_relationship_etc': '', |
|
24 |
+ }); |
|
25 |
+ |
|
26 |
+ //각 데이터별로 Dom 정보 담을 Ref 생성 |
|
27 |
+ const guardianRefInit = JSON.parse(JSON.stringify(guardian)); |
|
28 |
+ guardianRefInit['user_gender'] = {}; |
|
29 |
+ const guardianRef = React.useRef(guardianRefInit); |
|
30 |
+ |
|
31 |
+ //등록할 시니어 정보 변경 |
|
32 |
+ const guardianValueChange = (targetKey, value) => { |
|
33 |
+ let newGuardian = JSON.parse(JSON.stringify(guardian)); |
|
34 |
+ newGuardian[targetKey] = value; |
|
35 |
+ setGuardian(newGuardian); |
|
36 |
+ } |
|
37 |
+ |
|
38 |
+ //시니어 등록 유효성 검사 |
|
39 |
+ const guardianInsertValidation = () => { |
|
40 |
+ if (CommonUtil.isEmpty(guardian['user_name']) == true) { |
|
41 |
+ guardianRef.current['user_name'].focus(); |
|
42 |
+ alert("이름을 입력해 주세요."); |
|
43 |
+ return false; |
|
44 |
+ } |
|
45 |
+ if (CommonUtil.isEmpty(guardian['user_gender']) == true) { |
|
46 |
+ guardianRef.current['user_gender']['m'].focus(); |
|
47 |
+ alert("성별을 선택해 주세요."); |
|
48 |
+ return false; |
|
49 |
+ } |
|
50 |
+ if (CommonUtil.isEmpty(guardian['user_birth']) == true) { |
|
51 |
+ guardianRef.current['user_birth'].focus(); |
|
52 |
+ alert("생년월일을 선택해 주세요."); |
|
53 |
+ return false; |
|
54 |
+ } |
|
55 |
+ if (CommonUtil.isEmpty(guardian['user_phonenumber']) == true) { |
|
56 |
+ guardianRef.current['user_phonenumber'].focus(); |
|
57 |
+ alert("연락처를 입력해 주세요."); |
|
58 |
+ return false; |
|
59 |
+ } |
|
60 |
+ if (CommonUtil.isEmpty(guardian['user_address']) == true) { |
|
61 |
+ guardianRef.current['user_address'].focus(); |
|
62 |
+ alert("주소를 입력해 주세요."); |
|
63 |
+ return false; |
|
64 |
+ } |
|
65 |
+ if (CommonUtil.isEmpty(guardian['senior_relationship']) == true) { |
|
66 |
+ guardianRef.current['senior_relationship'].focus(); |
|
67 |
+ alert("대상자와의 관계를 선택해 주세요."); |
|
68 |
+ return false; |
|
69 |
+ } |
|
70 |
+ |
|
71 |
+ if (guardian['senior_relationship'] == '기타' && CommonUtil.isEmpty(guardian['senior_relationship_etc']) == true) { |
|
72 |
+ guardianRef.current['senior_relationship_etc'].focus(); |
|
73 |
+ alert("대상자와의 관계를 입력해 주세요."); |
|
74 |
+ return false; |
|
75 |
+ } |
|
76 |
+ |
|
77 |
+ return true; |
|
78 |
+ } |
|
79 |
+ //시니어 등록 |
|
80 |
+ const guardianInsert = () => { |
|
81 |
+ if (guardianInsertValidation() == false) { |
|
82 |
+ return; |
|
83 |
+ } |
|
84 |
+ |
|
85 |
+ fetch("/user/guardianInsert.json", { |
|
86 |
+ method: "POST", |
|
87 |
+ headers: { |
|
88 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
89 |
+ }, |
|
90 |
+ body: JSON.stringify(guardian), |
|
91 |
+ }).then((response) => response.json()).then((data) => { |
|
92 |
+ console.log("시니어 등록 결과(건수) : ", data); |
|
93 |
+ if (data > 0) { |
|
94 |
+ alert("등록완료"); |
|
95 |
+ guardianInsertCallback(); |
|
96 |
+ } else { |
|
97 |
+ alert("등록에 실패하였습니다. 관리자에게 문의바랍니다."); |
|
98 |
+ } |
|
99 |
+ }).catch((error) => { |
|
100 |
+ console.log('guardianInsert() /user/guardianInsert.json error : ', error); |
|
101 |
+ }); |
|
102 |
+ } |
|
6 | 103 |
|
7 | 104 |
|
8 |
-export default function Modal_Guardian({ open, close, seniorId }) { |
|
105 |
+ |
|
106 |
+ //Mounted |
|
107 |
+ React.useEffect(() => { |
|
108 |
+ const government_id = !defaultGovernmentId ? state.loginUser['government_id'] : defaultGovernmentId; |
|
109 |
+ const agency_id = !defaultAgencyId ? state.loginUser['agency_id'] : defaultAgencyId; |
|
110 |
+ |
|
111 |
+ let newSenior = JSON.parse(JSON.stringify(senior)); |
|
112 |
+ newSenior['government_id'] = government_id; |
|
113 |
+ newSenior['agency_id'] = agency_id; |
|
114 |
+ setSenior(newSenior); |
|
115 |
+ }, [defaultGovernmentId, defaultAgencyId]); |
|
9 | 116 |
|
10 | 117 |
return ( |
11 | 118 |
<div class={open ? "openModal modal" : "modal"}> |
... | ... | @@ -18,65 +125,95 @@ |
18 | 125 |
<div className="modal-main"> |
19 | 126 |
<div className="board-wrap"> |
20 | 127 |
|
21 |
- <SubTitle explanation={"OOO님의 보호자(가족)"} className="margin-bottom" /> |
|
22 |
- <div> |
|
23 |
- <table className='caregiver-user'> |
|
24 |
- <thead> |
|
25 |
- <tr> |
|
26 |
- <th>No</th> |
|
27 |
- <th>이름</th> |
|
28 |
- <th>대상자와의 관계</th> |
|
29 |
- <th>연락처</th> |
|
30 |
- <th>생년월일</th> |
|
31 |
- <th>삭제</th> |
|
32 |
- </tr> |
|
33 |
- </thead> |
|
34 |
- <tbody> |
|
35 |
- <tr> |
|
36 |
- <td>No</td> |
|
37 |
- <td>이름</td> |
|
38 |
- <td>대상자와의 관계</td> |
|
39 |
- <td>연락처</td> |
|
40 |
- <td>생년월일</td> |
|
41 |
- <td><button className={"btn-small gray-btn"} onClick={() => {}}>삭제</button></td> |
|
42 |
- </tr> |
|
43 |
- </tbody> |
|
44 |
- </table> |
|
45 |
- </div> |
|
46 |
- <div> |
|
47 |
- {/* <Pagination total={guardianTotal} limit={limit} page={page} setPage={setPage} /> */} |
|
48 |
- </div> |
|
49 |
- |
|
50 |
- |
|
51 | 128 |
<SubTitle explanation={"최초 ID는 연락처, PW는 생년월일 8자리입니다."} className="margin-bottom" /> |
52 | 129 |
<table className="margin-bottom2 senior-insert"> |
53 | 130 |
<tr> |
54 | 131 |
<th><span style={{color : "red"}}>*</span>이름</th> |
55 | 132 |
<td> |
56 |
- <input type="text"/> |
|
133 |
+ <input type="text" |
|
134 |
+ value={guardian['user_name']} |
|
135 |
+ onChange={(e) => {guardianValueChange('user_name', e.target.value)}} |
|
136 |
+ ref={el => guardianRef.current['user_name'] = el} |
|
137 |
+ /> |
|
57 | 138 |
</td> |
139 |
+ <th><span style={{color : "red"}}>*</span>성별</th> |
|
140 |
+ <td className=" gender"> |
|
141 |
+ <div className="flex-start"> |
|
142 |
+ <input type="radio" id="user_gender_m" name="user_gender" value="남" |
|
143 |
+ onChange={(e) => {e.target.checked ? guardianValueChange('user_gender', e.target.value) : null}} |
|
144 |
+ ref={el => guardianRef.current['user_gender']['m'] = el} |
|
145 |
+ /> |
|
146 |
+ <label for="user_gender_m">남</label> |
|
147 |
+ </div> |
|
148 |
+ <div className="flex-start"> |
|
149 |
+ <input type="radio" id="user_gender_f" name="user_gender" value="여" |
|
150 |
+ onChange={(e) => {e.target.checked ? guardianValueChange('user_gender', e.target.value) : null}} |
|
151 |
+ ref={el => guardianRef.current['user_gender']['f'] = el} |
|
152 |
+ /> |
|
153 |
+ <label for="user_gender_f">여</label> |
|
154 |
+ </div> |
|
155 |
+ </td> |
|
156 |
+ </tr> |
|
157 |
+ <tr> |
|
58 | 158 |
<th><span style={{color : "red"}}>*</span>생년월일</th> |
59 | 159 |
<td> |
60 | 160 |
<div className="flex"> |
61 |
- <input type='date' /> |
|
161 |
+ <input type='date' |
|
162 |
+ value={guardian['user_birth']} |
|
163 |
+ onChange={(e) => {guardianValueChange('user_birth', e.target.value)}} |
|
164 |
+ ref={el => guardianRef.current['user_birth'] = el} |
|
165 |
+ /> |
|
62 | 166 |
</div> |
63 | 167 |
</td> |
64 | 168 |
</tr> |
65 | 169 |
<tr> |
66 | 170 |
<th><span style={{color : "red"}}>*</span>연락처</th> |
67 | 171 |
<td colSpan={3}> |
68 |
- <input type="input" maxLength="13" /> |
|
172 |
+ <input type="number" maxLength="11" |
|
173 |
+ value={guardian['user_phonenumber']} |
|
174 |
+ onChange={(e) => {guardianValueChange('user_phonenumber', e.target.value)}} |
|
175 |
+ ref={el => guardianRef.current['user_phonenumber'] = el} |
|
176 |
+ /> |
|
69 | 177 |
</td> |
70 | 178 |
</tr> |
71 | 179 |
<tr> |
72 |
- <th>대상자와의 관계</th> |
|
180 |
+ <th><span style={{color : "red"}}>*</span>주소</th> |
|
73 | 181 |
<td colSpan={3}> |
74 |
- <input type="text" /> |
|
182 |
+ <input type="text" |
|
183 |
+ value={guardian['user_address']} |
|
184 |
+ onChange={(e) => {guardianValueChange('user_address', e.target.value)}} |
|
185 |
+ ref={el => guardianRef.current['user_address'] = el} |
|
186 |
+ /> |
|
187 |
+ </td> |
|
188 |
+ </tr> |
|
189 |
+ <tr> |
|
190 |
+ <th><span style={{color : "red"}}>*</span>대상자와의 관계</th> |
|
191 |
+ <td colSpan={3}> |
|
192 |
+ <select onChange={(e) => {guardianValueChange('senior_relationship', e.target.value)}} |
|
193 |
+ ref={el => guardianRef.current['senior_relationship'] = el}> |
|
194 |
+ <option>자녀</option> |
|
195 |
+ <option>손자녀</option> |
|
196 |
+ <option>배우자</option> |
|
197 |
+ <option>사위</option> |
|
198 |
+ <option>며느리</option> |
|
199 |
+ <option>형제</option> |
|
200 |
+ <option>자매</option> |
|
201 |
+ <option>남매</option> |
|
202 |
+ <option>친척</option> |
|
203 |
+ <option selected={guardian['senior_relationship'].indexOf('기타') > -1}>기타</option> |
|
204 |
+ </select> |
|
205 |
+ {guardian['senior_relationship'].indexOf('기타') > -1 ? ( |
|
206 |
+ <input type="text" |
|
207 |
+ value={guardian['senior_relationship_etc']} |
|
208 |
+ onChange={(e) => {guardianValueChange('senior_relationship_etc', e.target.value)}} |
|
209 |
+ ref={el => guardianRef.current['senior_relationship_etc'] = el} |
|
210 |
+ /> |
|
211 |
+ ) : null} |
|
75 | 212 |
</td> |
76 | 213 |
</tr> |
77 | 214 |
</table> |
78 | 215 |
<div className="btn-wrap flex-center margin-bottom5"> |
79 |
- <button className={"btn-small green-btn"} onClick={() => {}}>추가</button> |
|
216 |
+ <button className={"btn-small green-btn"} onClick={guardianInsert}>추가</button> |
|
80 | 217 |
</div> |
81 | 218 |
|
82 | 219 |
</div> |
--- client/views/component/Modal_SeniorInsert.jsx
+++ client/views/component/Modal_SeniorInsert.jsx
... | ... | @@ -1,6 +1,5 @@ |
1 | 1 |
import React, { useState, useRef } from "react"; |
2 | 2 |
import { useSelector } from "react-redux"; |
3 |
-import Button from "./Button.jsx"; |
|
4 | 3 |
import SubTitle from "./SubTitle.jsx"; |
5 | 4 |
|
6 | 5 |
import CommonUtil from "../../resources/js/CommonUtil.js"; |
--- client/views/pages/App.jsx
+++ client/views/pages/App.jsx
... | ... | @@ -55,7 +55,6 @@ |
55 | 55 |
|
56 | 56 |
//URL 변경 시, 발생 이벤트(hook) |
57 | 57 |
React.useEffect(() => { |
58 |
- console.log('location : ', location); |
|
59 | 58 |
loginUserSelectOne((loginResultData) => { |
60 | 59 |
//console.log('loginResultData : ', loginResultData); |
61 | 60 |
//console.log('isLogin : ', isLogin, ', authority : ', loginResultData['authority']); |
--- client/views/pages/senior_management/SeniorSelectOne.jsx
+++ client/views/pages/senior_management/SeniorSelectOne.jsx
... | ... | @@ -1,187 +1,225 @@ |
1 | 1 |
import React from "react"; |
2 |
-import Button from "../../component/Button.jsx"; |
|
2 |
+import { useNavigate, useLocation } from "react-router"; |
|
3 |
+ |
|
3 | 4 |
import SubTitle from "../../component/SubTitle.jsx"; |
4 |
-import { useNavigate } from "react-router"; |
|
5 |
-import ContentTitle from "../../component/ContentTitle.jsx"; |
|
6 |
-import PersonIcon from '@mui/icons-material/Person'; |
|
7 |
-import { useParams } from "react-router"; |
|
5 |
+import Modal_Guardian from "../../component/Modal_Guardian.jsx"; |
|
6 |
+ |
|
8 | 7 |
|
9 | 8 |
export default function SeniorSelectOne() { |
10 | 9 |
const navigate = useNavigate(); |
11 |
- let { seniorId } = useParams(); |
|
12 |
- console.log("seniorId : ", seniorId); |
|
10 |
+ const location = useLocation(); |
|
11 |
+ console.log('location.state : ', location.state); |
|
13 | 12 |
|
14 |
- const [seniortOne, setSeniorOne] = React.useState([]); |
|
15 |
- |
|
16 |
- //-------- 상세페이지 선택된 대상자의 정보 불러오기 --------// |
|
17 |
- const getSeniorDataOne = () => { |
|
18 |
- fetch("/user/selectSeniorOne.json", { |
|
19 |
- method: "POST", |
|
20 |
- headers: { |
|
21 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
22 |
- }, |
|
23 |
- body: JSON.stringify({ |
|
24 |
- user_id: seniorId |
|
25 |
- }), |
|
26 |
- }).then((response) => response.json()).then((data) => { |
|
27 |
- console.log("data : ", data[0]); |
|
28 |
- setSeniorOne(data[0]); |
|
29 |
- |
|
30 |
- }).catch((error) => { |
|
31 |
- console.log('getSeniorDataOne() /user/selectSeniorOne.json error : ', error); |
|
32 |
- }); |
|
13 |
+ //보호자 모달 오픈 여부 |
|
14 |
+ const [modalGuardianIsOpen, setModalGuardianIsOpen] = React.useState(false); |
|
15 |
+ //보호자 모달 오픈 |
|
16 |
+ const modalGuardianOpen = () => { |
|
17 |
+ setModalGuardianIsOpen(true); |
|
18 |
+ }; |
|
19 |
+ //보호자 모달 닫기 |
|
20 |
+ const modalGuardianClose = () => { |
|
21 |
+ setModalGuardianIsOpen(false); |
|
33 | 22 |
}; |
34 | 23 |
|
35 |
- // 시니어 보호자 매칭 제거 |
|
36 |
- const updateSeniorGuardianMatch = () => { |
|
37 |
- fetch("/user/updateGuardianMatchEnd.json", { |
|
24 |
+ |
|
25 |
+ const [medicationTimeCodeList, setMedicationTimeCodeList] = React.useState([]); |
|
26 |
+ //복약 시간 코드 목록 조회 |
|
27 |
+ const medicationTimeCodeSelectList = () => { |
|
28 |
+ fetch("/common/medicationTimeCodeSelectList.json", { |
|
38 | 29 |
method: "POST", |
39 | 30 |
headers: { |
40 | 31 |
'Content-Type': 'application/json; charset=UTF-8' |
41 | 32 |
}, |
42 |
- body: JSON.stringify({ |
|
43 |
- senior_id: seniorId, |
|
44 |
- }), |
|
33 |
+ body: JSON.stringify({}), |
|
45 | 34 |
}).then((response) => response.json()).then((data) => { |
46 |
- |
|
47 |
- }).catch((error) => { |
|
48 |
- console.log('updateSeniorGuardianMatch() /user/updateGuardianMatchEnd.json error : ', error); |
|
35 |
+ console.log("복약 시간 코드 목록 조회 : ", data); |
|
36 |
+ setMedicationTimeCodeList(data); |
|
37 |
+ setSenior(newSenior); |
|
38 |
+ }).catch((error) => { |
|
39 |
+ console.log('medicationTimeCodeSelectList() /common/medicationTimeCodeSelectList.json error : ', error); |
|
49 | 40 |
}); |
50 |
- } |
|
51 |
- // 시니어 - 담당자 매칭 종료 |
|
52 |
- const updateSeniorAgencyMatchEnd = () => { |
|
53 |
- |
|
54 |
- fetch("/user/updateWorkerMatchEnd.json", { |
|
55 |
- method: "POST", |
|
56 |
- headers: { |
|
57 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
58 |
- }, |
|
59 |
- body: JSON.stringify({ |
|
60 |
- senior_id: seniorId, |
|
61 |
- }), |
|
62 |
- }).then((response) => response.json()).then((data) => { |
|
63 |
- }).catch((error) => { |
|
64 |
- console.log('updateSeniorAgencyMatchEnd() /user/updateWorkerMatchEnd.json error : ', error); |
|
65 |
- }); |
|
66 |
- } |
|
67 |
- |
|
68 |
- // 시니어 삭제 (user_use : true > false) |
|
69 |
- const updateUserState = () => { |
|
70 |
- |
|
71 |
- fetch("/user/updateUserState.json", { |
|
72 |
- method: "POST", |
|
73 |
- headers: { |
|
74 |
- 'Content-Type': 'application/json; charset=UTF-8' |
|
75 |
- }, |
|
76 |
- body: JSON.stringify({ |
|
77 |
- user_id: seniorId, |
|
78 |
- }), |
|
79 |
- }).then((response) => response.json()).then((data) => { |
|
80 |
- }).catch((error) => { |
|
81 |
- console.log('updateUserState() /user/updateUserState.json error : ', error); |
|
82 |
- }); |
|
83 |
- |
|
84 |
-} |
|
85 |
- |
|
86 |
- const delSeniorData = () => { |
|
87 |
- let insertBtn = confirm("삭제하시겠습니까?"); |
|
88 |
- if (insertBtn) { |
|
89 |
- updateSeniorGuardianMatch(); |
|
90 |
- updateSeniorAgencyMatchEnd(); |
|
91 |
- updateUserState(); |
|
92 |
- alert('사용자가 삭제되었습니다.'); |
|
93 |
- navigate(`/UserAuthoriySelect_agency`); |
|
94 |
- } |
|
95 |
- else { |
|
96 |
- return ; |
|
97 |
- } |
|
98 | 41 |
} |
99 | 42 |
|
100 | 43 |
|
101 |
- React.useEffect(() => { |
|
102 |
- getSeniorDataOne(); |
|
44 |
+ //시니어 정보 |
|
45 |
+ const [senior, setSenior] = React.useState({ |
|
46 |
+ 'user_id': location.state['senior_id'], |
|
47 |
+ 'user_name': null, |
|
48 |
+ 'user_password': null, |
|
49 |
+ 'user_phonenumber': null, |
|
50 |
+ 'user_birth': null, |
|
51 |
+ 'user_gender': null, |
|
52 |
+ 'user_address': null, |
|
53 |
+ 'user_email': null, |
|
54 |
+ 'authority': 'ROLE_SENIOR', |
|
55 |
+ 'agency_id': null, |
|
56 |
+ 'government_id': null, |
|
103 | 57 |
|
58 |
+ 'senior_id': location.state['senior_id'], |
|
59 |
+ 'care_grade': null, |
|
60 |
+ 'medication_pill': null, |
|
61 |
+ 'underlie_disease': null, |
|
62 |
+ 'senior_note': null, |
|
63 |
+ |
|
64 |
+ 'seniorMedicationList': [], |
|
65 |
+ }); |
|
66 |
+ //시니어 상세 조회 |
|
67 |
+ const seniorSelectOne = () => { |
|
68 |
+ fetch("/user/seniorSelectOne.json", { |
|
69 |
+ method: "POST", |
|
70 |
+ headers: { |
|
71 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
72 |
+ }, |
|
73 |
+ body: JSON.stringify(senior), |
|
74 |
+ }).then((response) => response.json()).then((data) => { |
|
75 |
+ console.log("seniorSelectOne data : ", data); |
|
76 |
+ setSenior(data); |
|
77 |
+ }).catch((error) => { |
|
78 |
+ console.log('seniorSelectOne() /user/seniorSelectOne.json error : ', error); |
|
79 |
+ }); |
|
80 |
+ }; |
|
81 |
+ |
|
82 |
+ //보호자 상세 조회 |
|
83 |
+ const [guardianListBySenior, setGuardianListBySenior] = React.useState([]); |
|
84 |
+ const guardianSelectListBySenior = () => { |
|
85 |
+ fetch("/user/guardianSelectListBySenior.json", { |
|
86 |
+ method: "POST", |
|
87 |
+ headers: { |
|
88 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
89 |
+ }, |
|
90 |
+ body: JSON.stringify(senior), |
|
91 |
+ }).then((response) => response.json()).then((data) => { |
|
92 |
+ console.log("guardianSelectListBySenior data : ", data); |
|
93 |
+ setGuardianListBySenior(data); |
|
94 |
+ }).catch((error) => { |
|
95 |
+ console.log('guardianSelectListBySenior() /user/guardianSelectListBySenior.json error : ', error); |
|
96 |
+ }); |
|
97 |
+ }; |
|
98 |
+ //선택한 보호자 정보 |
|
99 |
+ const [guardianBySenior, setGuardianBySenior] = React.useState({}); |
|
100 |
+ |
|
101 |
+ //선택한 보호자 정보 관리 |
|
102 |
+ const guardianBySeniorManagement = (item) => { |
|
103 |
+ setGuardianBySenior(item); |
|
104 |
+ modalGuardianOpen(); |
|
105 |
+ } |
|
106 |
+ |
|
107 |
+ React.useEffect(() => { |
|
108 |
+ medicationTimeCodeSelectList(); |
|
109 |
+ seniorSelectOne(); |
|
110 |
+ guardianSelectListBySenior(); |
|
104 | 111 |
}, []) |
112 |
+ |
|
113 |
+ |
|
105 | 114 |
return ( |
106 | 115 |
<main> |
116 |
+ <Modal_Guardian open={modalGuardianIsOpen} close={modalGuardianClose} seniorId={location.state['senior_id']} |
|
117 |
+ guardianBySenior={guardianBySenior} |
|
118 |
+ guardianManagementCallback={() => {guardianSelectListBySenior(); modalGuardianClose()}}/> |
|
107 | 119 |
<div className="content-wrap row"> |
108 |
- <SubTitle explanation={"대상자 상세 프로필"} className="margin-bottom" /> |
|
120 |
+ <SubTitle explanation={"대상자 상세 프로필"} className="margin-bottom" /> |
|
109 | 121 |
<div> |
110 | 122 |
<table className="margin-bottom senior-detail"> |
111 | 123 |
<tbody> |
112 | 124 |
<tr> |
113 | 125 |
<th>아이디</th> |
114 | 126 |
<td> |
115 |
- <span>{seniortOne.user_id}</span> |
|
127 |
+ <span>{senior['user_id']}</span> |
|
116 | 128 |
</td> |
117 | 129 |
</tr> |
118 | 130 |
<tr> |
119 | 131 |
<th>이름</th> |
120 | 132 |
<td> |
121 |
- <span>{seniortOne.user_name}</span> |
|
133 |
+ <span>{senior['user_name']}</span> |
|
122 | 134 |
</td> |
123 | 135 |
<th>성별</th> |
124 | 136 |
<td> |
125 |
- <span>{seniortOne.user_gender}</span> |
|
137 |
+ <span>{senior['user_gender']}</span> |
|
126 | 138 |
</td> |
127 | 139 |
</tr> |
128 | 140 |
<tr> |
129 | 141 |
<th>생년월일</th> |
130 | 142 |
<td> |
131 |
- <span>{seniortOne.user_birth}</span> |
|
143 |
+ <span>{senior['user_birth']}</span> |
|
132 | 144 |
</td> |
133 | 145 |
<th>연락처</th> |
134 | 146 |
<td> |
135 |
- <span>{seniortOne.user_phonenumber}</span> |
|
147 |
+ <span>{senior['user_phonenumber']}</span> |
|
136 | 148 |
</td> |
137 | 149 |
</tr> |
138 | 150 |
<tr> |
139 | 151 |
<th>주소</th> |
140 | 152 |
<td> |
141 |
- <span>{seniortOne.user_address}</span> |
|
153 |
+ <span>{senior['user_address']}</span> |
|
142 | 154 |
</td> |
143 | 155 |
|
144 | 156 |
</tr> |
145 | 157 |
<tr> |
146 | 158 |
<th>필요복약</th> |
147 | 159 |
<td className="flex-start"> |
148 |
- {seniortOne.breakfast_medication_check ? <span>아침</span> : <></>} |
|
149 |
- {seniortOne.lunch_medication_check ? <span>점심</span> : <></>} |
|
150 |
- {seniortOne.dinner_medication_check ? <span>저녁</span> : <></>} |
|
160 |
+ {medicationTimeCodeList.filter(item => senior.seniorMedicationList.indexOf(item['medication_time_code']) > -1).map((item, idx) => { return ( |
|
161 |
+ <span>{item['medication_time_code_name']}</span> |
|
162 |
+ )})} |
|
151 | 163 |
</td> |
152 | 164 |
</tr> |
153 | 165 |
<tr> |
154 | 166 |
<th>복용중인 약</th> |
155 | 167 |
<td colSpan={3}> |
156 |
- <span className="medicine" cols="30" rows="2">{seniortOne.medication_pill}</span> |
|
168 |
+ <span className="medicine" cols="30" rows="2">{senior['medication_pill']}</span> |
|
157 | 169 |
</td> |
158 | 170 |
</tr> |
159 | 171 |
<tr> |
160 | 172 |
<th>비고</th> |
161 | 173 |
<td colSpan={3}> |
162 |
- <span className="note" cols="30" rows="2">{seniortOne.senior_note}</span> |
|
174 |
+ <span className="note" cols="30" rows="2">{senior['senior_note']}</span> |
|
163 | 175 |
</td> |
164 | 176 |
</tr> |
165 | 177 |
</tbody> |
166 | 178 |
</table> |
167 | 179 |
<div className="btn-wrap flex-center"> |
168 |
- <Button |
|
169 |
- className={"btn-large gray-btn"} |
|
170 |
- btnName={"수정"} |
|
171 |
- onClick={() => { |
|
172 |
- navigate(`/SeniorEdit/${seniorId}`); |
|
173 |
- }} |
|
174 |
- /> |
|
175 |
- <Button |
|
176 |
- className={"btn-large red-btn"} |
|
177 |
- btnName={"삭제"} |
|
178 |
- onClick={() => { |
|
179 |
- delSeniorData(); |
|
180 |
- }} |
|
181 |
- /> |
|
180 |
+ <button className={"btn-large green-btn"} onClick={() => modalGuardianOpen()}>보호자 추가</button> |
|
181 |
+ <button className={"btn-large gray-btn"} onClick={() => modalGuardianOpen()}>수정</button> |
|
182 |
+ <button className={"btn-large red-btn"} onClick={() => modalGuardianOpen()}>삭제</button> |
|
182 | 183 |
</div> |
183 | 184 |
</div> |
185 |
+ |
|
186 |
+ <SubTitle explanation={"대상자의 보호자"} className="margin-bottom" /> |
|
187 |
+ <div> |
|
188 |
+ <table className={"protector-user"}> |
|
189 |
+ <thead> |
|
190 |
+ <tr> |
|
191 |
+ <th>No</th> |
|
192 |
+ <th>이름</th> |
|
193 |
+ <th>관계</th> |
|
194 |
+ <th>연락처</th> |
|
195 |
+ <th>주소</th> |
|
196 |
+ <th>관리</th> |
|
197 |
+ </tr> |
|
198 |
+ </thead> |
|
199 |
+ <tbody> |
|
200 |
+ {guardianListBySenior.map((item, idx) => { return ( |
|
201 |
+ <tr key={idx}> |
|
202 |
+ <td>{idx + 1}</td> |
|
203 |
+ <td>{item['user_name']}</td> |
|
204 |
+ <td>{item['senior_relationship']}</td> |
|
205 |
+ <td>{item['user_phonenumber']}</td> |
|
206 |
+ <td>{item['user_address']}</td> |
|
207 |
+ <td> |
|
208 |
+ <button className={"btn-small lightgray-btn"} onClick={() => guardianBySeniorManagement(item)}>관리</button> |
|
209 |
+ </td> |
|
210 |
+ </tr> |
|
211 |
+ )})} |
|
212 |
+ {guardianListBySenior == null || guardianListBySenior.length == 0 ? |
|
213 |
+ <tr> |
|
214 |
+ <td colSpan={7}>조회된 데이터가 없습니다</td> |
|
215 |
+ </tr> |
|
216 |
+ : null} |
|
217 |
+ </tbody> |
|
218 |
+ </table> |
|
219 |
+ </div> |
|
220 |
+ |
|
184 | 221 |
</div> |
222 |
+ |
|
185 | 223 |
</main> |
186 | 224 |
); |
187 | 225 |
} |
+++ client/views/pages/senior_management/SeniorSelectOne_back_230404.jsx
... | ... | @@ -0,0 +1,187 @@ |
1 | +import React from "react"; | |
2 | +import Button from "../../component/Button.jsx"; | |
3 | +import SubTitle from "../../component/SubTitle.jsx"; | |
4 | +import { useNavigate } from "react-router"; | |
5 | +import ContentTitle from "../../component/ContentTitle.jsx"; | |
6 | +import PersonIcon from '@mui/icons-material/Person'; | |
7 | +import { useParams } from "react-router"; | |
8 | + | |
9 | +export default function SeniorSelectOne() { | |
10 | + const navigate = useNavigate(); | |
11 | + let { seniorId } = useParams(); | |
12 | + console.log("seniorId : ", seniorId); | |
13 | + | |
14 | + const [seniortOne, setSeniorOne] = React.useState([]); | |
15 | + | |
16 | + //-------- 상세페이지 선택된 대상자의 정보 불러오기 --------// | |
17 | + const getSeniorDataOne = () => { | |
18 | + fetch("/user/selectSeniorOne.json", { | |
19 | + method: "POST", | |
20 | + headers: { | |
21 | + 'Content-Type': 'application/json; charset=UTF-8' | |
22 | + }, | |
23 | + body: JSON.stringify({ | |
24 | + user_id: seniorId | |
25 | + }), | |
26 | + }).then((response) => response.json()).then((data) => { | |
27 | + console.log("data : ", data[0]); | |
28 | + setSeniorOne(data[0]); | |
29 | + | |
30 | + }).catch((error) => { | |
31 | + console.log('getSeniorDataOne() /user/selectSeniorOne.json error : ', error); | |
32 | + }); | |
33 | + }; | |
34 | + | |
35 | + // 시니어 보호자 매칭 제거 | |
36 | + const updateSeniorGuardianMatch = () => { | |
37 | + fetch("/user/updateGuardianMatchEnd.json", { | |
38 | + method: "POST", | |
39 | + headers: { | |
40 | + 'Content-Type': 'application/json; charset=UTF-8' | |
41 | + }, | |
42 | + body: JSON.stringify({ | |
43 | + senior_id: seniorId, | |
44 | + }), | |
45 | + }).then((response) => response.json()).then((data) => { | |
46 | + | |
47 | + }).catch((error) => { | |
48 | + console.log('updateSeniorGuardianMatch() /user/updateGuardianMatchEnd.json error : ', error); | |
49 | + }); | |
50 | + } | |
51 | + // 시니어 - 담당자 매칭 종료 | |
52 | + const updateSeniorAgencyMatchEnd = () => { | |
53 | + | |
54 | + fetch("/user/updateWorkerMatchEnd.json", { | |
55 | + method: "POST", | |
56 | + headers: { | |
57 | + 'Content-Type': 'application/json; charset=UTF-8' | |
58 | + }, | |
59 | + body: JSON.stringify({ | |
60 | + senior_id: seniorId, | |
61 | + }), | |
62 | + }).then((response) => response.json()).then((data) => { | |
63 | + }).catch((error) => { | |
64 | + console.log('updateSeniorAgencyMatchEnd() /user/updateWorkerMatchEnd.json error : ', error); | |
65 | + }); | |
66 | + } | |
67 | + | |
68 | + // 시니어 삭제 (user_use : true > false) | |
69 | + const updateUserState = () => { | |
70 | + | |
71 | + fetch("/user/updateUserState.json", { | |
72 | + method: "POST", | |
73 | + headers: { | |
74 | + 'Content-Type': 'application/json; charset=UTF-8' | |
75 | + }, | |
76 | + body: JSON.stringify({ | |
77 | + user_id: seniorId, | |
78 | + }), | |
79 | + }).then((response) => response.json()).then((data) => { | |
80 | + }).catch((error) => { | |
81 | + console.log('updateUserState() /user/updateUserState.json error : ', error); | |
82 | + }); | |
83 | + | |
84 | +} | |
85 | + | |
86 | + const delSeniorData = () => { | |
87 | + let insertBtn = confirm("삭제하시겠습니까?"); | |
88 | + if (insertBtn) { | |
89 | + updateSeniorGuardianMatch(); | |
90 | + updateSeniorAgencyMatchEnd(); | |
91 | + updateUserState(); | |
92 | + alert('사용자가 삭제되었습니다.'); | |
93 | + navigate(`/UserAuthoriySelect_agency`); | |
94 | + } | |
95 | + else { | |
96 | + return ; | |
97 | + } | |
98 | + } | |
99 | + | |
100 | + | |
101 | + React.useEffect(() => { | |
102 | + getSeniorDataOne(); | |
103 | + | |
104 | + }, []) | |
105 | + return ( | |
106 | + <main> | |
107 | + <div className="content-wrap row"> | |
108 | + <SubTitle explanation={"대상자 상세 프로필"} className="margin-bottom" /> | |
109 | + <div> | |
110 | + <table className="margin-bottom senior-detail"> | |
111 | + <tbody> | |
112 | + <tr> | |
113 | + <th>아이디</th> | |
114 | + <td> | |
115 | + <span>{seniortOne.user_id}</span> | |
116 | + </td> | |
117 | + </tr> | |
118 | + <tr> | |
119 | + <th>이름</th> | |
120 | + <td> | |
121 | + <span>{seniortOne.user_name}</span> | |
122 | + </td> | |
123 | + <th>성별</th> | |
124 | + <td> | |
125 | + <span>{seniortOne.user_gender}</span> | |
126 | + </td> | |
127 | + </tr> | |
128 | + <tr> | |
129 | + <th>생년월일</th> | |
130 | + <td> | |
131 | + <span>{seniortOne.user_birth}</span> | |
132 | + </td> | |
133 | + <th>연락처</th> | |
134 | + <td> | |
135 | + <span>{seniortOne.user_phonenumber}</span> | |
136 | + </td> | |
137 | + </tr> | |
138 | + <tr> | |
139 | + <th>주소</th> | |
140 | + <td> | |
141 | + <span>{seniortOne.user_address}</span> | |
142 | + </td> | |
143 | + | |
144 | + </tr> | |
145 | + <tr> | |
146 | + <th>필요복약</th> | |
147 | + <td className="flex-start"> | |
148 | + {seniortOne.breakfast_medication_check ? <span>아침</span> : <></>} | |
149 | + {seniortOne.lunch_medication_check ? <span>점심</span> : <></>} | |
150 | + {seniortOne.dinner_medication_check ? <span>저녁</span> : <></>} | |
151 | + </td> | |
152 | + </tr> | |
153 | + <tr> | |
154 | + <th>복용중인 약</th> | |
155 | + <td colSpan={3}> | |
156 | + <span className="medicine" cols="30" rows="2">{seniortOne.medication_pill}</span> | |
157 | + </td> | |
158 | + </tr> | |
159 | + <tr> | |
160 | + <th>비고</th> | |
161 | + <td colSpan={3}> | |
162 | + <span className="note" cols="30" rows="2">{seniortOne.senior_note}</span> | |
163 | + </td> | |
164 | + </tr> | |
165 | + </tbody> | |
166 | + </table> | |
167 | + <div className="btn-wrap flex-center"> | |
168 | + <Button | |
169 | + className={"btn-large gray-btn"} | |
170 | + btnName={"수정"} | |
171 | + onClick={() => { | |
172 | + navigate(`/SeniorEdit/${seniorId}`); | |
173 | + }} | |
174 | + /> | |
175 | + <Button | |
176 | + className={"btn-large red-btn"} | |
177 | + btnName={"삭제"} | |
178 | + onClick={() => { | |
179 | + delSeniorData(); | |
180 | + }} | |
181 | + /> | |
182 | + </div> | |
183 | + </div> | |
184 | + </div> | |
185 | + </main> | |
186 | + ); | |
187 | +} |
--- client/views/pages/user_management/UserAuthoriySelect.jsx
+++ client/views/pages/user_management/UserAuthoriySelect.jsx
... | ... | @@ -404,12 +404,11 @@ |
404 | 404 |
<th>성별</th> |
405 | 405 |
<th>연락처</th> |
406 | 406 |
<th>주소</th> |
407 |
- |
|
408 | 407 |
</tr> |
409 | 408 |
</thead> |
410 | 409 |
<tbody> |
411 | 410 |
{senior.userList.map((item, idx) => { return ( |
412 |
- <tr key={idx} onClick={() => {navigate("/SeniorSelectOne")}}> |
|
411 |
+ <tr key={idx} onClick={() => {navigate("/SeniorSelectOne", {state: {'senior_id': item['user_id']}})}}> |
|
413 | 412 |
<td>{senior.userListCount - idx - (senior.search.currentPage - 1) * senior.search.perPage}</td> |
414 | 413 |
<td>{item['agency_name']}</td> |
415 | 414 |
<td>{item['user_name']}</td> |
... | ... | @@ -471,8 +470,8 @@ |
471 | 470 |
<th>이름</th> |
472 | 471 |
<th>연락처</th> |
473 | 472 |
<th>이메일</th> |
474 |
- <th>담당 대상자(어르신)</th> |
|
475 | 473 |
<th>가입승인</th> |
474 |
+ <th>상세보기</th> |
|
476 | 475 |
</tr> |
477 | 476 |
</thead> |
478 | 477 |
<tbody> |
... | ... | @@ -485,12 +484,12 @@ |
485 | 484 |
<td>{item['user_phonenumber']}</td> |
486 | 485 |
<td>{item['user_email']}</td> |
487 | 486 |
<td> |
488 |
- <button className={"btn-small lightgray-btn"} onClick={() => modalGuardianOpen()}>담당 대상자(어르신) 보기</button> |
|
489 |
- </td> |
|
490 |
- <td> |
|
491 | 487 |
{item['is_accept'] ? "승인완료" : |
492 | 488 |
<button className={"btn-small red-btn"} onClick={() => {}}>가입승인</button> |
493 | 489 |
} |
490 |
+ </td> |
|
491 |
+ <td> |
|
492 |
+ <button className={"btn-small lightgray-btn"} onClick={() => modalGuardianOpen()}>상세 페이지 이동</button> |
|
494 | 493 |
</td> |
495 | 494 |
</tr> |
496 | 495 |
)})} |
... | ... | @@ -546,6 +545,7 @@ |
546 | 545 |
<th>연락처</th> |
547 | 546 |
<th>이메일</th> |
548 | 547 |
<th>가입승인</th> |
548 |
+ <th>관리</th> |
|
549 | 549 |
</tr> |
550 | 550 |
</thead> |
551 | 551 |
<tbody> |
... | ... | @@ -561,11 +561,14 @@ |
561 | 561 |
<button className={"btn-small red-btn"} onClick={() => {}}>가입승인</button> |
562 | 562 |
} |
563 | 563 |
</td> |
564 |
+ <td> |
|
565 |
+ <button className={"btn-small lightgray-btn"} onClick={() => modalGuardianOpen()}>정보 수정</button> |
|
566 |
+ </td> |
|
564 | 567 |
</tr> |
565 | 568 |
)})} |
566 | 569 |
{government.userList == null || government.userList.length == 0 ? |
567 | 570 |
<tr> |
568 |
- <td colSpan={6}>조회된 데이터가 없습니다</td> |
|
571 |
+ <td colSpan={7}>조회된 데이터가 없습니다</td> |
|
569 | 572 |
</tr> |
570 | 573 |
: null} |
571 | 574 |
</tbody> |
... | ... | @@ -608,6 +611,7 @@ |
608 | 611 |
<th>연락처</th> |
609 | 612 |
<th>이메일</th> |
610 | 613 |
<th>가입승인</th> |
614 |
+ <th>관리</th> |
|
611 | 615 |
</tr> |
612 | 616 |
</thead> |
613 | 617 |
<tbody> |
... | ... | @@ -622,11 +626,14 @@ |
622 | 626 |
<button className={"btn-small red-btn"} onClick={() => {}}>가입승인</button> |
623 | 627 |
} |
624 | 628 |
</td> |
629 |
+ <td> |
|
630 |
+ <button className={"btn-small lightgray-btn"} onClick={() => modalGuardianOpen()}>정보 수정</button> |
|
631 |
+ </td> |
|
625 | 632 |
</tr> |
626 | 633 |
)})} |
627 | 634 |
{admin.userList == null || admin.userList.length == 0 ? |
628 | 635 |
<tr> |
629 |
- <td colSpan={5}>조회된 데이터가 없습니다</td> |
|
636 |
+ <td colSpan={6}>조회된 데이터가 없습니다</td> |
|
630 | 637 |
</tr> |
631 | 638 |
: null} |
632 | 639 |
</tbody> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?