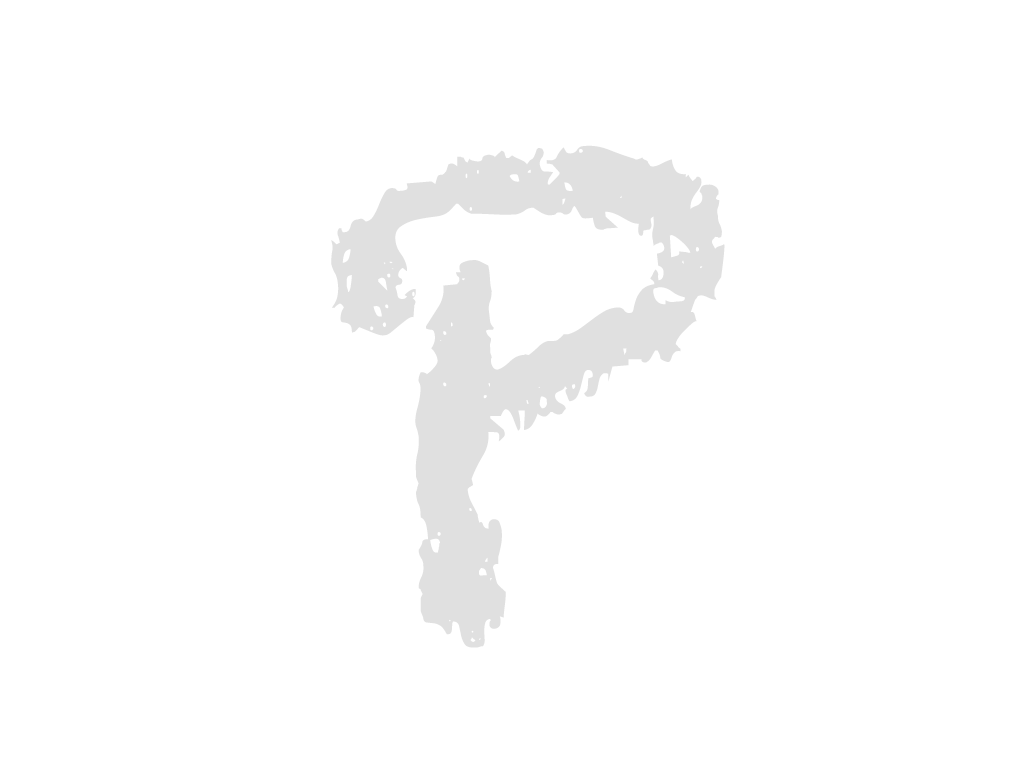
--- client/views/component/Modal_Guardian.jsx
+++ client/views/component/Modal_Guardian.jsx
... | ... | @@ -2,26 +2,28 @@ |
2 | 2 |
import SubTitle from "./SubTitle.jsx"; |
3 | 3 |
import CommonUtil from "../../resources/js/CommonUtil.js"; |
4 | 4 |
|
5 |
-export default function Modal_Guardian({ open, close, guardianManagementCallback, seniorId, guardianListBySenior }) { |
|
5 |
+export default function Modal_Guardian({ open, close, guardianManagementCallback, seniorId, guardianBySenior }) { |
|
6 | 6 |
|
7 |
- //시니어 정보 |
|
8 |
- const [guardian, setGuardian] = React.useState({ |
|
9 |
- 'user_id': null, |
|
10 |
- 'user_name': null, |
|
11 |
- 'user_password': null, |
|
12 |
- 'user_phonenumber': null, |
|
13 |
- 'user_birth': null, |
|
14 |
- 'user_gender': null, |
|
15 |
- 'user_address': null, |
|
16 |
- 'user_email': null, |
|
7 |
+ const guardianInit = { |
|
8 |
+ 'user_id': '', |
|
9 |
+ 'user_name': '', |
|
10 |
+ 'user_password': '', |
|
11 |
+ 'user_phonenumber': '', |
|
12 |
+ 'user_birth': '', |
|
13 |
+ 'user_gender': '', |
|
14 |
+ 'user_address': '', |
|
15 |
+ 'user_email': '', |
|
17 | 16 |
'authority': 'ROLE_GUARDIAN', |
18 |
- 'agency_id': null, |
|
19 |
- 'government_id': null, |
|
17 |
+ 'agency_id': '', |
|
18 |
+ 'government_id': '', |
|
20 | 19 |
|
21 | 20 |
'senior_id': seniorId, |
22 | 21 |
'senior_relationship': '자녀', |
23 | 22 |
'senior_relationship_etc': '', |
24 |
- }); |
|
23 |
+ }; |
|
24 |
+ |
|
25 |
+ //시니어 정보 |
|
26 |
+ const [guardian, setGuardian] = React.useState(JSON.parse(JSON.stringify(guardianInit))); |
|
25 | 27 |
|
26 | 28 |
//각 데이터별로 Dom 정보 담을 Ref 생성 |
27 | 29 |
const guardianRefInit = JSON.parse(JSON.stringify(guardian)); |
... | ... | @@ -76,7 +78,7 @@ |
76 | 78 |
|
77 | 79 |
return true; |
78 | 80 |
} |
79 |
- //시니어 등록 |
|
81 |
+ //보호자 등록 |
|
80 | 82 |
const guardianInsert = () => { |
81 | 83 |
if (guardianInsertValidation() == false) { |
82 | 84 |
return; |
... | ... | @@ -92,9 +94,62 @@ |
92 | 94 |
console.log("시니어 등록 결과(건수) : ", data); |
93 | 95 |
if (data > 0) { |
94 | 96 |
alert("등록완료"); |
95 |
- guardianInsertCallback(); |
|
97 |
+ guardianManagementCallback(); |
|
96 | 98 |
} else { |
97 | 99 |
alert("등록에 실패하였습니다. 관리자에게 문의바랍니다."); |
100 |
+ } |
|
101 |
+ }).catch((error) => { |
|
102 |
+ console.log('guardianInsert() /user/guardianInsert.json error : ', error); |
|
103 |
+ }); |
|
104 |
+ } |
|
105 |
+ |
|
106 |
+ //시니어 수정 |
|
107 |
+ const guardianUpdate = () => { |
|
108 |
+ if (guardianInsertValidation() == false) { |
|
109 |
+ return; |
|
110 |
+ } |
|
111 |
+ |
|
112 |
+ fetch("/user/guardianUpdate.json", { |
|
113 |
+ method: "POST", |
|
114 |
+ headers: { |
|
115 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
116 |
+ }, |
|
117 |
+ body: JSON.stringify(guardian), |
|
118 |
+ }).then((response) => response.json()).then((data) => { |
|
119 |
+ console.log("시니어 수정 결과(건수) : ", data); |
|
120 |
+ if (data > 0) { |
|
121 |
+ alert("수정완료"); |
|
122 |
+ guardianManagementCallback(); |
|
123 |
+ } else { |
|
124 |
+ alert("수정에 실패하였습니다. 관리자에게 문의바랍니다."); |
|
125 |
+ } |
|
126 |
+ }).catch((error) => { |
|
127 |
+ console.log('guardianInsert() /user/guardianInsert.json error : ', error); |
|
128 |
+ }); |
|
129 |
+ } |
|
130 |
+ |
|
131 |
+ //보호자 삭제 |
|
132 |
+ const guardianDelete = () => { |
|
133 |
+ if (confirm("보호자를 삭제하시겠습니까?") == false) { |
|
134 |
+ return; |
|
135 |
+ } |
|
136 |
+ if (guardianInsertValidation() == false) { |
|
137 |
+ return; |
|
138 |
+ } |
|
139 |
+ |
|
140 |
+ fetch("/user/guardianDelete.json", { |
|
141 |
+ method: "POST", |
|
142 |
+ headers: { |
|
143 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
144 |
+ }, |
|
145 |
+ body: JSON.stringify(guardian), |
|
146 |
+ }).then((response) => response.json()).then((data) => { |
|
147 |
+ console.log("시니어 삭제 결과(건수) : ", data); |
|
148 |
+ if (data > 0) { |
|
149 |
+ alert("삭제완료"); |
|
150 |
+ guardianManagementCallback(); |
|
151 |
+ } else { |
|
152 |
+ alert("삭제에 실패하였습니다. 관리자에게 문의바랍니다."); |
|
98 | 153 |
} |
99 | 154 |
}).catch((error) => { |
100 | 155 |
console.log('guardianInsert() /user/guardianInsert.json error : ', error); |
... | ... | @@ -105,14 +160,14 @@ |
105 | 160 |
|
106 | 161 |
//Mounted |
107 | 162 |
React.useEffect(() => { |
108 |
- const government_id = !defaultGovernmentId ? state.loginUser['government_id'] : defaultGovernmentId; |
|
109 |
- const agency_id = !defaultAgencyId ? state.loginUser['agency_id'] : defaultAgencyId; |
|
110 |
- |
|
111 |
- let newSenior = JSON.parse(JSON.stringify(senior)); |
|
112 |
- newSenior['government_id'] = government_id; |
|
113 |
- newSenior['agency_id'] = agency_id; |
|
114 |
- setSenior(newSenior); |
|
115 |
- }, [defaultGovernmentId, defaultAgencyId]); |
|
163 |
+ console.log('guardianBySenior : ', guardianBySenior); |
|
164 |
+ if (CommonUtil.isEmpty(guardianBySenior) == false) { |
|
165 |
+ setGuardian(guardianBySenior); |
|
166 |
+ } else { |
|
167 |
+ console.log('guardianBySenior : ', guardianBySenior); |
|
168 |
+ setGuardian(JSON.parse(JSON.stringify(guardianInit))); |
|
169 |
+ } |
|
170 |
+ }, [guardianBySenior]); |
|
116 | 171 |
|
117 | 172 |
return ( |
118 | 173 |
<div class={open ? "openModal modal" : "modal"}> |
... | ... | @@ -213,7 +268,12 @@ |
213 | 268 |
</tr> |
214 | 269 |
</table> |
215 | 270 |
<div className="btn-wrap flex-center margin-bottom5"> |
216 |
- <button className={"btn-small green-btn"} onClick={guardianInsert}>추가</button> |
|
271 |
+ {CommonUtil.isEmpty(guardian['guardian_id']) |
|
272 |
+ ? <button className={"btn-small gray-btn"} onClick={guardianInsert}>추가</button> |
|
273 |
+ : <> |
|
274 |
+ <button className={"btn-small gray-btn"} onClick={guardianUpdate}>수정</button> |
|
275 |
+ <button className={"btn-small red-btn"} onClick={guardianDelete}>삭제</button> |
|
276 |
+ </>} |
|
217 | 277 |
</div> |
218 | 278 |
|
219 | 279 |
</div> |
--- client/views/component/Modal_SeniorInsert.jsx
+++ client/views/component/Modal_SeniorInsert.jsx
... | ... | @@ -262,14 +262,14 @@ |
262 | 262 |
<th><span style={{color : "red"}}>*</span>성별</th> |
263 | 263 |
<td className=" gender"> |
264 | 264 |
<div className="flex-start"> |
265 |
- <input type="radio" id="user_gender_m" name="user_gender" value="남" |
|
265 |
+ <input type="radio" id="user_gender_m" name="user_gender" value="남" checked={senior['user_gender'] == '남'} |
|
266 | 266 |
onChange={(e) => {e.target.checked ? seniorValueChange('user_gender', e.target.value) : null}} |
267 | 267 |
ref={el => seniorRef.current['user_gender']['m'] = el} |
268 | 268 |
/> |
269 | 269 |
<label for="user_gender_m">남</label> |
270 | 270 |
</div> |
271 | 271 |
<div className="flex-start"> |
272 |
- <input type="radio" id="user_gender_f" name="user_gender" value="여" |
|
272 |
+ <input type="radio" id="user_gender_f" name="user_gender" value="여" checked={senior['user_gender'] == '여'} |
|
273 | 273 |
onChange={(e) => {e.target.checked ? seniorValueChange('user_gender', e.target.value) : null}} |
274 | 274 |
ref={el => seniorRef.current['user_gender']['f'] = el} |
275 | 275 |
/> |
--- client/views/pages/senior_management/SeniorSelectOne.jsx
+++ client/views/pages/senior_management/SeniorSelectOne.jsx
... | ... | @@ -34,7 +34,6 @@ |
34 | 34 |
}).then((response) => response.json()).then((data) => { |
35 | 35 |
console.log("복약 시간 코드 목록 조회 : ", data); |
36 | 36 |
setMedicationTimeCodeList(data); |
37 |
- setSenior(newSenior); |
|
38 | 37 |
}).catch((error) => { |
39 | 38 |
console.log('medicationTimeCodeSelectList() /common/medicationTimeCodeSelectList.json error : ', error); |
40 | 39 |
}); |
... | ... | @@ -95,6 +94,7 @@ |
95 | 94 |
console.log('guardianSelectListBySenior() /user/guardianSelectListBySenior.json error : ', error); |
96 | 95 |
}); |
97 | 96 |
}; |
97 |
+ |
|
98 | 98 |
//선택한 보호자 정보 |
99 | 99 |
const [guardianBySenior, setGuardianBySenior] = React.useState({}); |
100 | 100 |
|
... | ... | @@ -102,6 +102,30 @@ |
102 | 102 |
const guardianBySeniorManagement = (item) => { |
103 | 103 |
setGuardianBySenior(item); |
104 | 104 |
modalGuardianOpen(); |
105 |
+ } |
|
106 |
+ |
|
107 |
+ |
|
108 |
+ //가입승인 |
|
109 |
+ const userUpdate = (user, callback) => { |
|
110 |
+ user['is_accept'] = true; |
|
111 |
+ |
|
112 |
+ fetch("/user/userUpdate.json", { |
|
113 |
+ method: "POST", |
|
114 |
+ headers: { |
|
115 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
116 |
+ }, |
|
117 |
+ body: JSON.stringify(user), |
|
118 |
+ }).then((response) => response.json()).then((data) => { |
|
119 |
+ console.log("시니어 등록 결과(건수) : ", data); |
|
120 |
+ if (data > 0) { |
|
121 |
+ alert("승인완료"); |
|
122 |
+ callback(); |
|
123 |
+ } else { |
|
124 |
+ alert("승인에 실패하였습니다. 관리자에게 문의바랍니다."); |
|
125 |
+ } |
|
126 |
+ }).catch((error) => { |
|
127 |
+ console.log('userUpdate() /user/userUpdate.json error : ', error); |
|
128 |
+ }); |
|
105 | 129 |
} |
106 | 130 |
|
107 | 131 |
React.useEffect(() => { |
... | ... | @@ -115,7 +139,7 @@ |
115 | 139 |
<main> |
116 | 140 |
<Modal_Guardian open={modalGuardianIsOpen} close={modalGuardianClose} seniorId={location.state['senior_id']} |
117 | 141 |
guardianBySenior={guardianBySenior} |
118 |
- guardianManagementCallback={() => {guardianSelectListBySenior(); modalGuardianClose()}}/> |
|
142 |
+ guardianManagementCallback={() => {guardianSelectListBySenior(); modalGuardianClose(); setGuardianBySenior({})}}/> |
|
119 | 143 |
<div className="content-wrap row"> |
120 | 144 |
<SubTitle explanation={"대상자 상세 프로필"} className="margin-bottom" /> |
121 | 145 |
<div> |
... | ... | @@ -177,9 +201,9 @@ |
177 | 201 |
</tbody> |
178 | 202 |
</table> |
179 | 203 |
<div className="btn-wrap flex-center"> |
180 |
- <button className={"btn-large green-btn"} onClick={() => modalGuardianOpen()}>보호자 추가</button> |
|
181 | 204 |
<button className={"btn-large gray-btn"} onClick={() => modalGuardianOpen()}>수정</button> |
182 |
- <button className={"btn-large red-btn"} onClick={() => modalGuardianOpen()}>삭제</button> |
|
205 |
+ <button className={"btn-large green-btn"} onClick={() => {setGuardianBySenior({}); modalGuardianOpen()}}>보호자 추가</button> |
|
206 |
+ <button className={"btn-large red-btn"} onClick={() => alert("삭제할 수 없습니다.")}>삭제</button> |
|
183 | 207 |
</div> |
184 | 208 |
</div> |
185 | 209 |
|
... | ... | @@ -194,6 +218,7 @@ |
194 | 218 |
<th>연락처</th> |
195 | 219 |
<th>주소</th> |
196 | 220 |
<th>관리</th> |
221 |
+ <th>가입승인</th> |
|
197 | 222 |
</tr> |
198 | 223 |
</thead> |
199 | 224 |
<tbody> |
... | ... | @@ -205,6 +230,11 @@ |
205 | 230 |
<td>{item['user_phonenumber']}</td> |
206 | 231 |
<td>{item['user_address']}</td> |
207 | 232 |
<td> |
233 |
+ {item['is_accept'] ? "승인완료" : |
|
234 |
+ <button className={"btn-small red-btn"} onClick={() => {userUpdate(item, guardianSelectListBySenior)}}>가입승인</button> |
|
235 |
+ } |
|
236 |
+ </td> |
|
237 |
+ <td> |
|
208 | 238 |
<button className={"btn-small lightgray-btn"} onClick={() => guardianBySeniorManagement(item)}>관리</button> |
209 | 239 |
</td> |
210 | 240 |
</tr> |
--- client/views/pages/user_management/UserAuthoriySelect.jsx
+++ client/views/pages/user_management/UserAuthoriySelect.jsx
... | ... | @@ -283,6 +283,29 @@ |
283 | 283 |
}}); |
284 | 284 |
} |
285 | 285 |
|
286 |
+ //가입승인 |
|
287 |
+ const userUpdate = (user, callback) => { |
|
288 |
+ user['is_accept'] = true; |
|
289 |
+ |
|
290 |
+ fetch("/user/userUpdate.json", { |
|
291 |
+ method: "POST", |
|
292 |
+ headers: { |
|
293 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
294 |
+ }, |
|
295 |
+ body: JSON.stringify(user), |
|
296 |
+ }).then((response) => response.json()).then((data) => { |
|
297 |
+ console.log("시니어 등록 결과(건수) : ", data); |
|
298 |
+ if (data > 0) { |
|
299 |
+ alert("승인완료"); |
|
300 |
+ callback(); |
|
301 |
+ } else { |
|
302 |
+ alert("승인에 실패하였습니다. 관리자에게 문의바랍니다."); |
|
303 |
+ } |
|
304 |
+ }).catch((error) => { |
|
305 |
+ console.log('userUpdate() /user/userUpdate.json error : ', error); |
|
306 |
+ }); |
|
307 |
+ } |
|
308 |
+ |
|
286 | 309 |
|
287 | 310 |
//Mounted |
288 | 311 |
React.useEffect(() => { |
... | ... | @@ -485,7 +508,7 @@ |
485 | 508 |
<td>{item['user_email']}</td> |
486 | 509 |
<td> |
487 | 510 |
{item['is_accept'] ? "승인완료" : |
488 |
- <button className={"btn-small red-btn"} onClick={() => {}}>가입승인</button> |
|
511 |
+ <button className={"btn-small red-btn"} onClick={() => {userUpdate(item, agentSelectList)}}>가입승인</button> |
|
489 | 512 |
} |
490 | 513 |
</td> |
491 | 514 |
<td> |
... | ... | @@ -558,7 +581,7 @@ |
558 | 581 |
<td>{item['user_email']}</td> |
559 | 582 |
<td> |
560 | 583 |
{item['is_accept'] ? "승인완료" : |
561 |
- <button className={"btn-small red-btn"} onClick={() => {}}>가입승인</button> |
|
584 |
+ <button className={"btn-small red-btn"} onClick={() => {userUpdate(item, governmentSelectList)}}>가입승인</button> |
|
562 | 585 |
} |
563 | 586 |
</td> |
564 | 587 |
<td> |
... | ... | @@ -623,7 +646,7 @@ |
623 | 646 |
<td>{item['user_email']}</td> |
624 | 647 |
<td> |
625 | 648 |
{item['is_accept'] ? "승인완료" : |
626 |
- <button className={"btn-small red-btn"} onClick={() => {}}>가입승인</button> |
|
649 |
+ <button className={"btn-small red-btn"} onClick={() => {userUpdate(item, adminSelectList)}}>가입승인</button> |
|
627 | 650 |
} |
628 | 651 |
</td> |
629 | 652 |
<td> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?