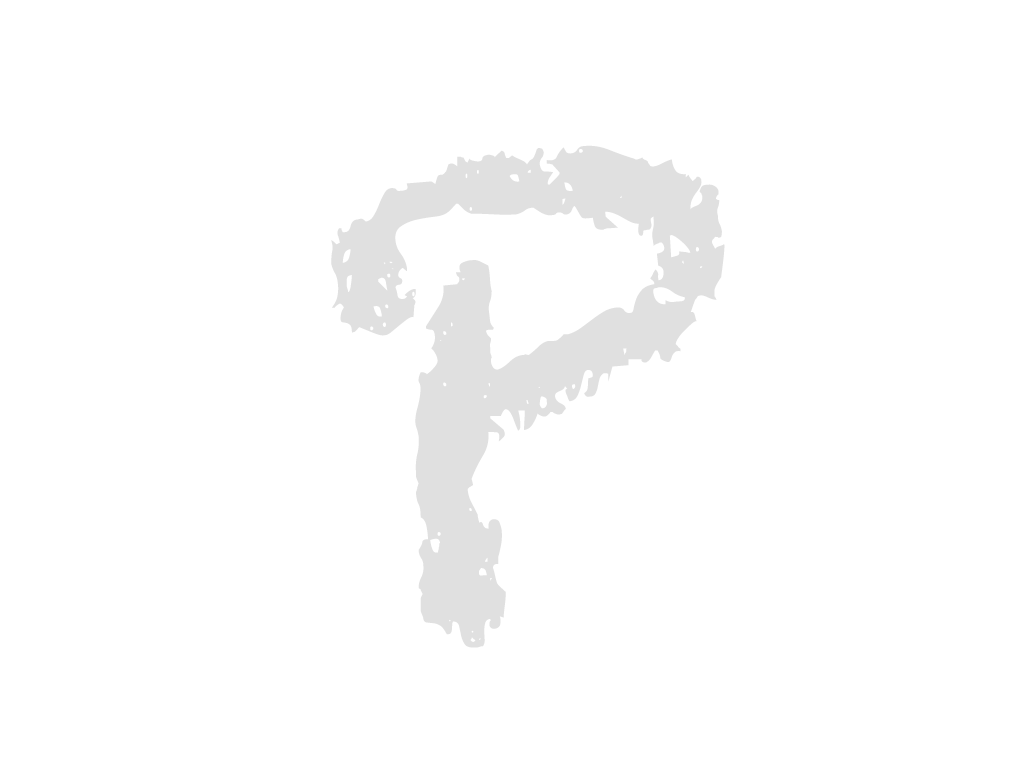
--- client/views/component/Calendar.jsx
+++ client/views/component/Calendar.jsx
... | ... | @@ -28,9 +28,21 @@ |
28 | 28 |
todayTemperature.push(item['temperature']); |
29 | 29 |
} |
30 | 30 |
}) |
31 |
- return ( |
|
32 |
- <p>{todayTemperature[0]}</p> |
|
33 |
- ) |
|
31 |
+ if (todayTemperature.length > 0) { |
|
32 |
+ if (moment(date['date']).format("MM") >= 11 || moment(date['date']).format("MM") <= 2) { |
|
33 |
+ return ( |
|
34 |
+ <p>{Math.min.apply(null, todayTemperature)}</p> |
|
35 |
+ ) |
|
36 |
+ } else { |
|
37 |
+ return ( |
|
38 |
+ <p>{Math.max.apply(null, todayTemperature)}</p> |
|
39 |
+ ) |
|
40 |
+ } |
|
41 |
+ } else { |
|
42 |
+ return ( |
|
43 |
+ <p>-</p> |
|
44 |
+ ) |
|
45 |
+ } |
|
34 | 46 |
} |
35 | 47 |
|
36 | 48 |
const mark = []; |
--- client/views/component/chart/Chart9.jsx
+++ client/views/component/chart/Chart9.jsx
... | ... | @@ -2,13 +2,14 @@ |
2 | 2 |
import * as am5 from "@amcharts/amcharts5"; |
3 | 3 |
import * as am5xy from "@amcharts/amcharts5/xy"; |
4 | 4 |
import am5themes_Animated from "@amcharts/amcharts5/themes/Animated"; |
5 |
+import CommonUtil from "../../../resources/js/CommonUtil"; |
|
5 | 6 |
|
6 |
-class Chart9 extends Component { |
|
7 |
- componentDidMount() { |
|
8 |
- /* Chart code */ |
|
9 |
- // Create root element |
|
10 |
- // https://www.amcharts.com/docs/v5/getting-started/#Root_element |
|
11 |
- let root = am5.Root.new("chart9"); |
|
7 |
+export default function Chart9({ data }) { |
|
8 |
+ const createChart = () => { |
|
9 |
+ console.log('createChart9 data : ', data); |
|
10 |
+ |
|
11 |
+ let root = am5.Root.new("Chart9"); |
|
12 |
+ root._logo.dispose(); |
|
12 | 13 |
|
13 | 14 |
|
14 | 15 |
// Set themes |
... | ... | @@ -37,33 +38,9 @@ |
37 | 38 |
cursor.lineY.set("visible", false); |
38 | 39 |
|
39 | 40 |
|
40 |
- // Generate random data |
|
41 |
- let date = new Date(); |
|
42 |
- date.setHours(0, 0, 0, 0); |
|
43 |
- let value = 0; |
|
44 |
- |
|
45 |
- function generateData() { |
|
46 |
- value = Math.round((Math.random() * 100) + 1); |
|
47 |
- am5.time.add(date, "day", 1); |
|
48 |
- return { |
|
49 |
- date: date.getTime(), |
|
50 |
- value: value |
|
51 |
- }; |
|
52 |
- } |
|
53 |
- |
|
54 |
- function generateDatas(count) { |
|
55 |
- let data = []; |
|
56 |
- for (var i = 0; i < count; ++i) { |
|
57 |
- data.push(generateData()); |
|
58 |
- } |
|
59 |
- return data; |
|
60 |
- } |
|
61 |
- |
|
62 |
- |
|
63 | 41 |
// Create axes |
64 | 42 |
// https://www.amcharts.com/docs/v5/charts/xy-chart/axes/ |
65 | 43 |
let xAxis = chart.xAxes.push(am5xy.DateAxis.new(root, { |
66 |
- maxDeviation: 0.2, |
|
67 | 44 |
baseInterval: { |
68 | 45 |
timeUnit: "day", |
69 | 46 |
count: 1 |
... | ... | @@ -73,6 +50,8 @@ |
73 | 50 |
})); |
74 | 51 |
|
75 | 52 |
let yAxis = chart.yAxes.push(am5xy.ValueAxis.new(root, { |
53 |
+ min: 0, |
|
54 |
+ max: 100, |
|
76 | 55 |
renderer: am5xy.AxisRendererY.new(root, {}) |
77 | 56 |
})); |
78 | 57 |
|
... | ... | @@ -83,12 +62,16 @@ |
83 | 62 |
name: "Series", |
84 | 63 |
xAxis: xAxis, |
85 | 64 |
yAxis: yAxis, |
86 |
- valueYField: "value", |
|
87 |
- valueXField: "date", |
|
65 |
+ valueYField: "percent", |
|
66 |
+ valueXField: "xName", |
|
88 | 67 |
tooltip: am5.Tooltip.new(root, { |
89 | 68 |
labelText: "{valueY}" |
90 | 69 |
}) |
91 | 70 |
})); |
71 |
+ series.strokes.template.setAll({ |
|
72 |
+ strokeWidth: 2, |
|
73 |
+ strokeDasharray: [3, 3], |
|
74 |
+ }); |
|
92 | 75 |
|
93 | 76 |
|
94 | 77 |
// Add scrollbar |
... | ... | @@ -99,7 +82,6 @@ |
99 | 82 |
|
100 | 83 |
|
101 | 84 |
// Set data |
102 |
- let data = generateDatas(120); |
|
103 | 85 |
series.data.setAll(data); |
104 | 86 |
|
105 | 87 |
|
... | ... | @@ -107,11 +89,17 @@ |
107 | 89 |
// https://www.amcharts.com/docs/v5/concepts/animations/ |
108 | 90 |
series.appear(1000); |
109 | 91 |
chart.appear(1000, 100); |
92 |
+ |
|
110 | 93 |
} |
111 | 94 |
|
112 |
- render() { |
|
113 |
- return <div id="chart9" style={{ width: "100%", height: "100%" }}></div>; |
|
114 |
- } |
|
115 |
-} |
|
95 |
+ React.useEffect(() => { |
|
96 |
+ console.log('React.useEffect chart2 data : ', data); |
|
97 |
+ if (CommonUtil.isEmpty(data) == false) { |
|
98 |
+ createChart(); |
|
99 |
+ } |
|
100 |
+ }, [data]) |
|
116 | 101 |
|
117 |
-export default Chart9; |
|
102 |
+ return ( |
|
103 |
+ <div id="Chart9" style={{ width: "100%", height: "100%" }}></div> |
|
104 |
+ ) |
|
105 |
+}(No newline at end of file) |
--- client/views/pages/equipment/AgencyEquipmentSelect.jsx
+++ client/views/pages/equipment/AgencyEquipmentSelect.jsx
... | ... | @@ -247,7 +247,11 @@ |
247 | 247 |
<div className="board-wrap" style={{ marginTop: "3rem" }} > |
248 | 248 |
<div className="btn-wrap margin-bottom"> |
249 | 249 |
<div className="btn-wrap flex-end margin-bottom "> |
250 |
- <button className={"btn-small gray-btn"} onClick={() => navigate("/AgencyAdminSeniorSelect")}>대상자 관리</button> |
|
250 |
+ {CommonUtil.isEmpty(state.loginUser) && state.loginUser['authority'] == 'ROLE_AGENCYADMIN' ? ( |
|
251 |
+ <button className={"btn-small gray-btn"} onClick={() => navigate("/AgencyAdminSeniorSelect")}>대상자 관리</button> |
|
252 |
+ ) : ( |
|
253 |
+ <button className={"btn-small gray-btn"} onClick={() => navigate("/AgencySeniorSelect")}>대상자 관리</button> |
|
254 |
+ )} |
|
251 | 255 |
</div> |
252 | 256 |
{rentalContent} |
253 | 257 |
</div> |
--- client/views/pages/healthcare/Healthcare.jsx
+++ client/views/pages/healthcare/Healthcare.jsx
... | ... | @@ -171,9 +171,9 @@ |
171 | 171 |
chartData['time'] = data[i]['temperature_time']; |
172 | 172 |
|
173 | 173 |
if (data[i]['temperature_date'].substr(5, 2) >= 11 || data[i]['temperature_date'].substr(5, 2) <= 2) { |
174 |
- _stackTemperatureData.push(chartData); |
|
175 |
- } else { |
|
176 | 174 |
_stackTemperatureData.unshift(chartData); |
175 |
+ } else { |
|
176 |
+ _stackTemperatureData.push(chartData); |
|
177 | 177 |
} |
178 | 178 |
} |
179 | 179 |
|
--- client/views/pages/healthcare/HealthcareSelectOne.jsx
+++ client/views/pages/healthcare/HealthcareSelectOne.jsx
... | ... | @@ -95,6 +95,7 @@ |
95 | 95 |
const [seniorMedicationListByDay, setSeniorMedicationListByDay] = React.useState([]); |
96 | 96 |
const [stackChartData, setStackChartData] = React.useState([]); |
97 | 97 |
const [medicationData, setMedicationData] = React.useState([]); |
98 |
+ const [medicationStats, setMedicationStats] = React.useState([]); |
|
98 | 99 |
//특정 대상자의 일별, 복약시간별 복약 목록 조회 |
99 | 100 |
const seniorMedicationSelectListByDay = (seniorMedicationList) => { |
100 | 101 |
fetch("/user/seniorMedicationSelectListByDay.json", { |
... | ... | @@ -117,9 +118,11 @@ |
117 | 118 |
if (CommonUtil.isEmpty(data) == false) { |
118 | 119 |
let _stackChartData = []; |
119 | 120 |
let _medicationData = []; |
121 |
+ let _medicationStats = []; |
|
120 | 122 |
for (let i = 0; i < data.length; i++) { |
121 | 123 |
let sum = 0; // 실제 복약량 |
122 | 124 |
let counter = 0; // 복약해야하는 양 |
125 |
+ let percent = 0; |
|
123 | 126 |
let chartData = { |
124 | 127 |
xName: data[i]['medication_default_date'], |
125 | 128 |
}; |
... | ... | @@ -134,11 +137,17 @@ |
134 | 137 |
continue; |
135 | 138 |
} |
136 | 139 |
} |
140 |
+ if (sum != 0 && counter != 0) { |
|
141 |
+ percent = Math.floor((sum / counter) * 100) |
|
142 |
+ } |
|
137 | 143 |
_medicationData.push(chartData); |
138 | 144 |
_stackChartData.push({ "xName": data[i]['medication_default_date'], "sum": sum, "total": counter }); |
145 |
+ _medicationStats.push({ "xName": new Date(data[i]['medication_default_date']).getTime(), "percent": percent }); |
|
139 | 146 |
} |
147 |
+ console.log("_medicationStats: ", _medicationStats); |
|
140 | 148 |
setMedicationData(_medicationData); |
141 | 149 |
setStackChartData(_stackChartData); |
150 |
+ setMedicationStats(_medicationStats); |
|
142 | 151 |
} |
143 | 152 |
|
144 | 153 |
}).catch((error) => { |
... | ... | @@ -173,8 +182,13 @@ |
173 | 182 |
chartData['temperature'] = data[i]['temperature_data']; |
174 | 183 |
chartData['time'] = data[i]['temperature_time']; |
175 | 184 |
|
176 |
- _stackTemperatureData.push(chartData); |
|
185 |
+ if (data[i]['temperature_date'].substr(5, 2) >= 11 || data[i]['temperature_date'].substr(5, 2) <= 2) { |
|
186 |
+ _stackTemperatureData.unshift(chartData); |
|
187 |
+ } else { |
|
188 |
+ _stackTemperatureData.push(chartData); |
|
189 |
+ } |
|
177 | 190 |
} |
191 |
+ |
|
178 | 192 |
setStackTemperatureData(_stackTemperatureData); |
179 | 193 |
console.log('_stackTemperatureData : ', _stackTemperatureData); |
180 | 194 |
} |
... | ... | @@ -455,7 +469,7 @@ |
455 | 469 |
|
456 | 470 |
seniorBatteryListByDay.map((item, idx) => { |
457 | 471 |
if (item['battery_date'] == visitDate) { |
458 |
- clcickDayBattery = item['battery_power_data']; |
|
472 |
+ clcickDayBattery = item['battery_power_data'] + '%'; |
|
459 | 473 |
} else { |
460 | 474 |
return |
461 | 475 |
} |
... | ... | @@ -495,7 +509,7 @@ |
495 | 509 |
<div className="content-wrap margin-top"> |
496 | 510 |
<DetailTitle contentTitle={"대상자의 복용률을 확인 할 수 있습니다."} /> |
497 | 511 |
<div className="main-guardian-chart margin-top"> |
498 |
- <Chart9 /> |
|
512 |
+ <Chart9 data={medicationStats} /> |
|
499 | 513 |
</div> |
500 | 514 |
</div> |
501 | 515 |
|
--- client/views/pages/main/Main_guardian.jsx
+++ client/views/pages/main/Main_guardian.jsx
... | ... | @@ -37,6 +37,7 @@ |
37 | 37 |
import percent_m_60 from '../../../resources/files/images/percent_m_60.png'; |
38 | 38 |
import percent_m_80 from '../../../resources/files/images/percent_m_80.png'; |
39 | 39 |
import percent_m_100 from '../../../resources/files/images/percent_m_100.png'; |
40 |
+import Chart9 from "../../component/chart/Chart9.jsx"; |
|
40 | 41 |
|
41 | 42 |
import CommonUtil from "../../../resources/js/CommonUtil.js"; |
42 | 43 |
|
... | ... | @@ -62,6 +63,7 @@ |
62 | 63 |
seniorSelectOne(); |
63 | 64 |
seniorMedicationSelectList(); |
64 | 65 |
seniorTemperatureSelectListByDay(); |
66 |
+ seniorBatterySelectListByDay(); |
|
65 | 67 |
visitRecordSelectList(); |
66 | 68 |
} else { |
67 | 69 |
return; |
... | ... | @@ -122,6 +124,7 @@ |
122 | 124 |
const [seniorMedicationListByDay, setSeniorMedicationListByDay] = React.useState([]); |
123 | 125 |
const [stackChartData, setStackChartData] = React.useState([]); |
124 | 126 |
const [medicationData, setMedicationData] = React.useState([]); |
127 |
+ const [medicationStats, setMedicationStats] = React.useState([]); |
|
125 | 128 |
//특정 대상자의 일별, 복약시간별 복약 목록 조회 |
126 | 129 |
const seniorMedicationSelectListByDay = (seniorMedicationList) => { |
127 | 130 |
fetch("/user/seniorMedicationSelectListByDay.json", { |
... | ... | @@ -140,9 +143,11 @@ |
140 | 143 |
if (CommonUtil.isEmpty(data) == false) { |
141 | 144 |
let _stackChartData = []; |
142 | 145 |
let _medicationData = []; |
146 |
+ let _medicationStats = []; |
|
143 | 147 |
for (let i = 0; i < data.length; i++) { |
144 | 148 |
let sum = 0; // 실제 복약량 |
145 | 149 |
let counter = 0; // 복약해야하는 양 |
150 |
+ let percent = 0; |
|
146 | 151 |
let chartData = { |
147 | 152 |
xName: data[i]['medication_default_date'], |
148 | 153 |
}; |
... | ... | @@ -157,11 +162,17 @@ |
157 | 162 |
continue; |
158 | 163 |
} |
159 | 164 |
} |
165 |
+ if (sum != 0 && counter != 0) { |
|
166 |
+ percent = Math.floor((sum / counter) * 100) |
|
167 |
+ } |
|
160 | 168 |
_medicationData.push(chartData); |
161 | 169 |
_stackChartData.push({ "xName": data[i]['medication_default_date'], "sum": sum, "total": counter }); |
170 |
+ _medicationStats.push({ "xName": new Date(data[i]['medication_default_date']).getTime(), "percent": percent }); |
|
162 | 171 |
} |
172 |
+ console.log("_medicationStats: ", _medicationStats); |
|
163 | 173 |
setMedicationData(_medicationData); |
164 | 174 |
setStackChartData(_stackChartData); |
175 |
+ setMedicationStats(_medicationStats); |
|
165 | 176 |
} |
166 | 177 |
}).catch((error) => { |
167 | 178 |
console.log('seniorMedicationSelectListByDay() /user/seniorMedicationSelectListByDay.json error : ', error); |
... | ... | @@ -209,13 +220,13 @@ |
209 | 220 |
//특정 대상자의 일별 배터리 목록 |
210 | 221 |
const [seniorBatteryListByDay, setSeniorBatteryListByDay] = React.useState([]); |
211 | 222 |
//특정 대상자의 일별 배터리 목록 조회 |
212 |
- const seniorBatterySelectListByDay = (seniorNum) => { |
|
223 |
+ const seniorBatterySelectListByDay = () => { |
|
213 | 224 |
fetch("/user/seniorBatterySelectListByDay.json", { |
214 | 225 |
method: "POST", |
215 | 226 |
headers: { |
216 | 227 |
'Content-Type': 'application/json; charset=UTF-8' |
217 | 228 |
}, |
218 |
- body: JSON.stringify(seniorNum), |
|
229 |
+ body: JSON.stringify(seniorSearch), |
|
219 | 230 |
}).then((response) => response.json()).then((data) => { |
220 | 231 |
console.log("seniorBatteryListByDay data : ", data); |
221 | 232 |
setSeniorBatteryListByDay(data); |
... | ... | @@ -359,7 +370,7 @@ |
359 | 370 |
|
360 | 371 |
seniorBatteryListByDay.map((item, idx) => { |
361 | 372 |
if (item['battery_date'] == visitDate) { |
362 |
- clcickDayBattery = item['battery_power_data']; |
|
373 |
+ clcickDayBattery = item['battery_power_data'] + '%'; |
|
363 | 374 |
} else { |
364 | 375 |
return |
365 | 376 |
} |
... | ... | @@ -416,6 +427,12 @@ |
416 | 427 |
</div> |
417 | 428 |
<Calendar data={{ senior: senior, medication: stackChartData, temperature: stackTemperatureData, visitRecordList: visitRecordList, onClick: calerndarClick }} /> |
418 | 429 |
|
430 |
+ <div className="content-wrap margin-top"> |
|
431 |
+ <div className="main-guardian-chart margin-top"> |
|
432 |
+ <Chart9 data={medicationStats} /> |
|
433 |
+ </div> |
|
434 |
+ </div> |
|
435 |
+ |
|
419 | 436 |
<Modal open={modalOpen} close={closeModal} header="시니어 정보 관리"> |
420 | 437 |
<div className="modal-visit board-wrap"> |
421 | 438 |
<table className="margin-bottom"> |
--- client/views/pages/user_management/UserSelect.jsx
+++ client/views/pages/user_management/UserSelect.jsx
... | ... | @@ -71,12 +71,39 @@ |
71 | 71 |
'government_id': state.loginUser['government_id'], |
72 | 72 |
'agency_id': state.loginUser['agency_id'], |
73 | 73 |
'authority': null, |
74 |
+ 'user_use': null, |
|
74 | 75 |
|
75 | 76 |
'searchType': null, |
76 | 77 |
'searchText': null, |
77 | 78 |
'currentPage': 1, |
78 | 79 |
'perPage': 10, |
79 | 80 |
}); |
81 |
+ |
|
82 |
+ const useStateChange = () => { |
|
83 |
+ console.log("isViewType:", isViewType) |
|
84 |
+ senior.search.user_use = isViewType ? null : false; |
|
85 |
+ orgSelectListOfHierarchy(); |
|
86 |
+ |
|
87 |
+ if (CommonUtil.isEmpty(location.state) == false) { |
|
88 |
+ const param = location.state; |
|
89 |
+ if (CommonUtil.isEmpty(param['tabActiveByRoleType']) == false) { |
|
90 |
+ setTabActiveByRoleType(param['tabActiveByRoleType']); |
|
91 |
+ } |
|
92 |
+ governmentChange(param['government_id']); |
|
93 |
+ agencyChange(param['government_id'], param['agency_id']); |
|
94 |
+ } else { |
|
95 |
+ seniorSelectList(); |
|
96 |
+ agentSelectList(); |
|
97 |
+ agentAdminSelectList(); |
|
98 |
+ if (state.loginUser['authority'] == 'ROLE_ADMIN' || state.loginUser['authority'] == 'ROLE_GOVERNMENT') { |
|
99 |
+ governmentSelectList(); |
|
100 |
+ } |
|
101 |
+ } |
|
102 |
+ |
|
103 |
+ if (state.loginUser['authority'] == 'ROLE_ADMIN') { |
|
104 |
+ adminSelectList(); |
|
105 |
+ } |
|
106 |
+ } |
|
80 | 107 |
|
81 | 108 |
//대상자(시니어) 목록 및 페이징 정보 |
82 | 109 |
userSearch['authority'] = 'ROLE_SENIOR'; |
... | ... | @@ -376,6 +403,10 @@ |
376 | 403 |
} |
377 | 404 |
}, []); |
378 | 405 |
|
406 |
+ React.useEffect(() => { |
|
407 |
+ useStateChange(); |
|
408 |
+ }, [isViewType]) |
|
409 |
+ |
|
379 | 410 |
|
380 | 411 |
return ( |
381 | 412 |
<main> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?