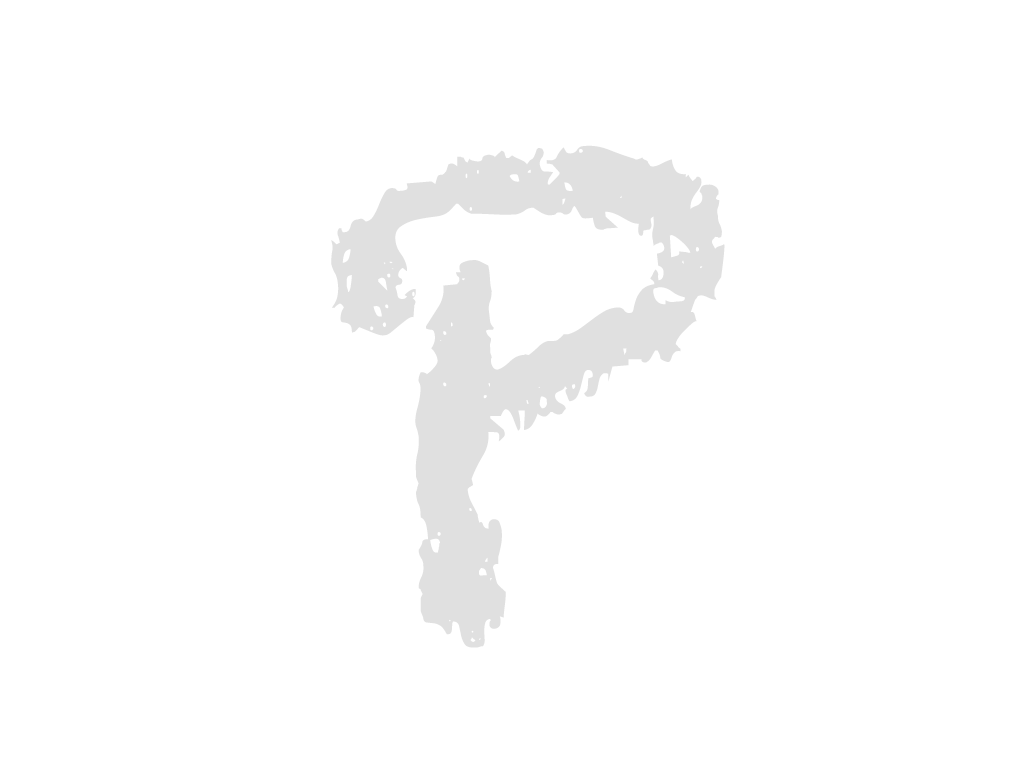
Merge branch 'admin' of http://210.180.118.83/yjryu/senior_care_system into admin
@0f8fe44dcad495800d7fbb305458d9cfff82b72d
--- client/views/component/QnAModal.jsx
+++ client/views/component/QnAModal.jsx
... | ... | @@ -4,45 +4,106 @@ |
4 | 4 |
import SubTitle from "./SubTitle.jsx"; |
5 | 5 |
import { useNavigate } from "react-router"; |
6 | 6 |
|
7 |
-export default function QaAModal({ open, close, }) { |
|
7 |
+export default function QnAModal({ open, close }) { |
|
8 |
+ const [qnaRegisteringList, setQnaRegisteringList] = React.useState([]); |
|
9 |
+ |
|
10 |
+ const [idx, setIdx] = React.useState(); |
|
11 |
+ const [title, setTitle] = React.useState(""); |
|
12 |
+ const [datetime, setDatetime] = React.useState(""); |
|
13 |
+ const [contents, setContents] = React.useState(""); |
|
14 |
+ |
|
15 |
+ //qna등록 |
|
16 |
+ const getQnaRegisteringList = () => { |
|
17 |
+ fetch("/qna/qnaInsert.json", { |
|
18 |
+ method: "POST", |
|
19 |
+ headers: { |
|
20 |
+ "Content-Type": "application/json; charset=UTF-8;", |
|
21 |
+ }, |
|
22 |
+ body: JSON.stringify({ |
|
23 |
+ qna_idx: idx, |
|
24 |
+ qna_title: title, |
|
25 |
+ qna_insert_user_id: "gym1004", |
|
26 |
+ qna_insert_datetime: datetime, |
|
27 |
+ }), |
|
28 |
+ }) |
|
29 |
+ .then((response) => response.json()) |
|
30 |
+ .then((data) => { |
|
31 |
+ if (data > 0) { |
|
32 |
+ console.log(data); |
|
33 |
+ alert("등록완료"); |
|
34 |
+ close(true); |
|
35 |
+ } else { |
|
36 |
+ alert("등록실패, 관리자에게 문의해주세요."); |
|
37 |
+ } |
|
38 |
+ }) |
|
39 |
+ .catch((error) => { |
|
40 |
+ console.log("qna error : ", error); |
|
41 |
+ }); |
|
42 |
+ }; |
|
43 |
+ |
|
44 |
+ //게시판 |
|
45 |
+ const thead = ["제목", "작성자", "작성일자"]; |
|
46 |
+ const key = ["qna_title", "qna_insert_user_name", "qna_insert_datetime"]; |
|
47 |
+ |
|
8 | 48 |
return ( |
9 | 49 |
<div class={open ? "openModal modal" : "modal"}> |
10 |
- {open ? ( |
|
11 |
- <div className="modal-inner"> |
|
12 |
- <div className="modal-header flex"> |
|
13 |
- Q&A등록 |
|
14 |
- <Button className={"close"} onClick={close} btnName={"X"} /> |
|
15 |
- </div> |
|
16 |
- <div className="modal-main"><div className="board-wrap"> |
|
17 |
- <div> |
|
18 |
- <SubTitle explanation={"작성자 정보"} /> |
|
19 |
- <table className="margin-bottom2 qna-insert"> |
|
20 |
- <tr> |
|
21 |
- <th>작성자</th> |
|
22 |
- <td> |
|
23 |
- <input type="text" placeholder="자동입력부분" /> |
|
24 |
- </td> |
|
25 |
- </tr> |
|
50 |
+ {open ? ( |
|
51 |
+ <div className="modal-inner"> |
|
52 |
+ <div className="modal-header flex"> |
|
53 |
+ Q&A등록 |
|
54 |
+ <Button className={"close"} onClick={close} btnName={"X"} /> |
|
55 |
+ </div> |
|
56 |
+ <div className="modal-main"> |
|
57 |
+ <div className="board-wrap"> |
|
58 |
+ <div> |
|
59 |
+ <SubTitle explanation={"작성자 정보"} /> |
|
60 |
+ <table className="margin-bottom2 qna-insert"> |
|
61 |
+ <tr> |
|
62 |
+ <th>작성자</th> |
|
63 |
+ <td> |
|
64 |
+ <input type="text" placeholder="자동입력부분" /> |
|
65 |
+ </td> |
|
66 |
+ </tr> |
|
26 | 67 |
|
27 |
- <tr> |
|
28 |
- <th>제목</th> |
|
29 |
- <td colSpan={3}> |
|
30 |
- <input type="text" /> |
|
31 |
- </td> |
|
32 |
- </tr> |
|
33 |
- <tr> |
|
34 |
- <th>내용</th> |
|
35 |
- <td colSpan={3}> |
|
36 |
- <textarea className="medicine" cols="30" rows="2"></textarea> |
|
37 |
- </td> |
|
38 |
- </tr> |
|
39 |
- </table> |
|
68 |
+ <tr> |
|
69 |
+ <th>제목</th> |
|
70 |
+ <td colSpan={3}> |
|
71 |
+ <input |
|
72 |
+ type="text" |
|
73 |
+ value={title} |
|
74 |
+ onInput={(e) => { |
|
75 |
+ setTitle(e.target.value); |
|
76 |
+ }} |
|
77 |
+ /> |
|
78 |
+ </td> |
|
79 |
+ </tr> |
|
80 |
+ <tr> |
|
81 |
+ <th>내용</th> |
|
82 |
+ <td colSpan={3}> |
|
83 |
+ <textarea |
|
84 |
+ className="medicine" |
|
85 |
+ cols="30" |
|
86 |
+ rows="2" |
|
87 |
+ value={contents} |
|
88 |
+ onInput={(e) => { |
|
89 |
+ setContents(e.target.value); |
|
90 |
+ }} |
|
91 |
+ ></textarea> |
|
92 |
+ </td> |
|
93 |
+ </tr> |
|
94 |
+ </table> |
|
95 |
+ </div> |
|
96 |
+ <div className="flex-center"> |
|
97 |
+ <Button |
|
98 |
+ className={"btn-small red-btn"} |
|
99 |
+ btnName={"저장"} |
|
100 |
+ onClick={() => getQnaRegisteringList()} |
|
101 |
+ /> |
|
102 |
+ </div> |
|
103 |
+ </div> |
|
104 |
+ </div> |
|
40 | 105 |
</div> |
41 |
- <div className="flex-center"><Button className={"btn-small red-btn"} btnName={"저장"} /></div > |
|
42 |
- </div></div> |
|
43 |
- </div> |
|
44 |
- ) : null} |
|
45 |
- </div> |
|
46 |
- |
|
106 |
+ ) : null} |
|
107 |
+ </div> |
|
47 | 108 |
); |
48 | 109 |
} |
--- client/views/pages/App.jsx
+++ client/views/pages/App.jsx
... | ... | @@ -26,8 +26,8 @@ |
26 | 26 |
}; |
27 | 27 |
|
28 | 28 |
|
29 |
- const menuItems = GuardianApp.menuItems; //AdminApp, GovernmentApp, AllApp, AgencyApp, GuardianApp |
|
30 |
- const AppRoute = GuardianApp.AppRoute; |
|
29 |
+ const menuItems = AllApp.menuItems; //AdminApp, GovernmentApp, AllApp, AgencyApp, GuardianApp |
|
30 |
+ const AppRoute = AllApp.AppRoute; |
|
31 | 31 |
|
32 | 32 |
|
33 | 33 |
const { title } = menuItems.find( |
--- client/views/pages/callcenter/QuestionConfirm.jsx
+++ client/views/pages/callcenter/QuestionConfirm.jsx
... | ... | @@ -5,7 +5,10 @@ |
5 | 5 |
import { useNavigate } from "react-router"; |
6 | 6 |
|
7 | 7 |
export default function QuestionConfirm() { |
8 |
+ |
|
8 | 9 |
const navigate = useNavigate(); |
10 |
+ |
|
11 |
+ |
|
9 | 12 |
return ( |
10 | 13 |
<main> |
11 | 14 |
<div className="content-wrap row"> |
... | ... | @@ -44,14 +47,14 @@ |
44 | 47 |
className={"btn-large gray-btn"} |
45 | 48 |
btnName={"이전"} |
46 | 49 |
onClick={() => { |
47 |
- navigate("/QandASelect"); |
|
50 |
+ navigate("/QuestionSelect"); |
|
48 | 51 |
}} |
49 | 52 |
/> |
50 | 53 |
<Button |
51 | 54 |
className={"btn-large green-btn"} |
52 | 55 |
btnName={"등록"} |
53 | 56 |
onClick={() => { |
54 |
- navigate("/QandASelect"); |
|
57 |
+ navigate("/QuestionSelect"); |
|
55 | 58 |
}} |
56 | 59 |
/> |
57 | 60 |
</div> |
--- client/views/pages/callcenter/QuestionSelect.jsx
+++ client/views/pages/callcenter/QuestionSelect.jsx
... | ... | @@ -13,16 +13,16 @@ |
13 | 13 |
const openModal = () => { |
14 | 14 |
setModalOpen(true); |
15 | 15 |
}; |
16 |
- const closeModal = () => { |
|
16 |
+ const closeModal = (isInsertComplete) => { |
|
17 | 17 |
setModalOpen(false); |
18 |
+ if (isInsertComplete == true) { |
|
19 |
+ getQnaList(); |
|
20 |
+ } |
|
18 | 21 |
}; |
19 | 22 |
|
20 |
- const [qnaList, setQnaList] = React.useState(); |
|
23 |
+ const [qnaList, setQnaList] = React.useState([]); |
|
21 | 24 |
|
22 |
- const [state, setState] = React.useState(); |
|
23 |
- const [title, setTitle] = React.useState(); |
|
24 |
- const [username, setUsername] = React.useState(); |
|
25 |
- const [datetime, setDatetime] = React.useState(); |
|
25 |
+ const [idx, setIdx] = React.useState(); |
|
26 | 26 |
|
27 | 27 |
//-------- 페이징 작업 설정 시작 --------// |
28 | 28 |
const limit = 15; // 페이지당 보여줄 공지 개수 |
... | ... | @@ -39,17 +39,15 @@ |
39 | 39 |
}, |
40 | 40 |
body: JSON.stringify({ |
41 | 41 |
|
42 |
- qna_state: state, |
|
43 |
- qna_title: title, |
|
44 |
- qna_insert_user_id: username, |
|
45 |
- qna_insert_datetime: datetime, |
|
42 |
+ qna_idx: idx, |
|
43 |
+ |
|
46 | 44 |
}), |
47 | 45 |
}) |
48 | 46 |
.then((response) => response.json()) |
49 | 47 |
.then((data) => { |
50 | 48 |
console.log(data); |
51 | 49 |
setQnaList(data); |
52 |
- // setMyQnaTotal(data.length); |
|
50 |
+ setMyQnaTotal(data.length); |
|
53 | 51 |
}) |
54 | 52 |
.catch((error) => { |
55 | 53 |
console.log( |
... | ... | @@ -61,25 +59,8 @@ |
61 | 59 |
|
62 | 60 |
//게시판 |
63 | 61 |
const thead = ["No", "답변상태", "제목", "작성자", "작성일자"]; |
64 |
- const key = ["idx", "state", "title", "username", "datetime"]; |
|
65 |
- const content = [ |
|
66 |
- { |
|
67 |
- No: 1, |
|
68 |
- answer: "답변완료", |
|
69 |
- title: ( |
|
70 |
- <div |
|
71 |
- className="title" |
|
72 |
- onClick={() => { |
|
73 |
- navigate("/QuestionConfirm"); |
|
74 |
- }} |
|
75 |
- > |
|
76 |
- 담당자 바꿔주세요 |
|
77 |
- </div> |
|
78 |
- ), |
|
79 |
- writer: "홍길동", |
|
80 |
- date: "2023-01-27", |
|
81 |
- }, |
|
82 |
- ]; |
|
62 |
+ const key = ["qna_idx", "qna_state", "qna_title", "qna_insert_user_name", "qna_insert_datetime"]; |
|
63 |
+ |
|
83 | 64 |
|
84 | 65 |
React.useEffect(() => { |
85 | 66 |
|
... | ... | @@ -114,7 +95,7 @@ |
114 | 95 |
<Table |
115 | 96 |
className="equipment-detail" |
116 | 97 |
head={thead} |
117 |
- contents={content} |
|
98 |
+ contents={qnaList} |
|
118 | 99 |
contentKey={key} |
119 | 100 |
view={"qna"} |
120 | 101 |
offset={offset} |
--- client/views/pages/equipment/EquipmentManagementSelect.jsx
+++ client/views/pages/equipment/EquipmentManagementSelect.jsx
... | ... | @@ -149,7 +149,7 @@ |
149 | 149 |
} |
150 | 150 |
|
151 | 151 |
|
152 |
- // 관리자 전체 장비 목록 |
|
152 |
+ // 전체 장비 목록 |
|
153 | 153 |
const [equipmentList, setEquipmentList] = React.useState([]); |
154 | 154 |
// 장비 |
155 | 155 |
const [equipment, setEquipment] = React.useState({ |
... | ... | @@ -162,20 +162,10 @@ |
162 | 162 |
}); |
163 | 163 |
// 장비 등록 모달창 |
164 | 164 |
const [equipmentInsertModal, setEquipmentInsertModal] = React.useState(false); |
165 |
+ // 장비 상세 조회 모달창 |
|
166 |
+ const [equipmentOneModal, setEquipmentOneModal] = React.useState(false); |
|
165 | 167 |
|
166 |
- // 장비 등록 모달창 열기 |
|
167 |
- const equipmentInsertModalOpen = () => { |
|
168 |
- setEquipmentInsertModal(true); |
|
169 |
- }; |
|
170 |
- // 장비 등록 모달창 닫기 |
|
171 |
- const equipmentInsertModalClose = () => { |
|
172 |
- // 장비 초기화 |
|
173 |
- setEquipment(equipment_); |
|
174 |
- setEquipmentInsertModal(false); |
|
175 |
- }; |
|
176 |
- |
|
177 |
- |
|
178 |
- // 관리자 전체 장비 목록 조회 |
|
168 |
+ // 전체 장비 목록 조회 |
|
179 | 169 |
const equipmentSelectList = () => { |
180 | 170 |
console.log('equipmentSelectList Function Run'); |
181 | 171 |
fetch("/equipment/equipmentSelectList.json", { |
... | ... | @@ -192,9 +182,20 @@ |
192 | 182 |
}); |
193 | 183 |
} |
194 | 184 |
|
185 |
+ // 장비 등록 모달창 열기 |
|
186 |
+ const equipmentInsertModalOpen = () => { |
|
187 |
+ setEquipmentInsertModal(true); |
|
188 |
+ }; |
|
189 |
+ // 장비 등록 모달창 닫기 |
|
190 |
+ const equipmentInsertModalClose = () => { |
|
191 |
+ // 장비 초기화 |
|
192 |
+ setEquipment(equipment_); |
|
193 |
+ setEquipmentInsertModal(false); |
|
194 |
+ }; |
|
195 |
+ |
|
195 | 196 |
// 장비 임시 데이터 |
196 | 197 |
const equipmentChange = (e) => { |
197 |
- console.log('equipmentChange: ', e.target.name, e.target.value); |
|
198 |
+ // console.log('equipmentChange: ', e.target.name, e.target.value); |
|
198 | 199 |
|
199 | 200 |
// 원본 데이터 복사 및 수정 |
200 | 201 |
let data = {}; |
... | ... | @@ -227,6 +228,14 @@ |
227 | 228 |
return; |
228 | 229 |
} |
229 | 230 |
} |
231 |
+ |
|
232 |
+ // 시리얼 넘버 중복검사 |
|
233 |
+ for(let i = 0; i < equipmentList.length; i++) { |
|
234 |
+ if(equipment["equipment_serial_number"] == equipmentList[i]["equipment_serial_number"]) { |
|
235 |
+ alert('이미 등록된 시리얼 넘버입니다.'); |
|
236 |
+ return; |
|
237 |
+ } |
|
238 |
+ } |
|
230 | 239 |
|
231 | 240 |
fetch("/equipment/equipmentInsert.json", { |
232 | 241 |
method: "POST", |
... | ... | @@ -249,6 +258,84 @@ |
249 | 258 |
alert('등록에 실패했습니다.'); |
250 | 259 |
}); |
251 | 260 |
} |
261 |
+ |
|
262 |
+ // 장비 상세 조회 모달창 열기 |
|
263 |
+ const equipmentOneModalOpen = (item) => { |
|
264 |
+ setEquipment(item); |
|
265 |
+ setEquipmentOneModal(true); |
|
266 |
+ }; |
|
267 |
+ // 장비 상세 조회 모달창 닫기 |
|
268 |
+ const equipmentOneModalClose = () => { |
|
269 |
+ // 장비 초기화 |
|
270 |
+ setEquipment(equipment_); |
|
271 |
+ setEquipmentOneModal(false); |
|
272 |
+ }; |
|
273 |
+ |
|
274 |
+ // 장비 수정 |
|
275 |
+ const equipmentUpdate = () => { |
|
276 |
+ console.log('equipmentUpdate Function Run'); |
|
277 |
+ |
|
278 |
+ // 유효성 검사 |
|
279 |
+ for(let i = 0; i < equipmentInsertHead.length; i++) { |
|
280 |
+ if(equipment[equipmentInsertKey[i]] == null) { |
|
281 |
+ alert(equipmentInsertHead[i] +'를 선택해 주세요'); |
|
282 |
+ return; |
|
283 |
+ } |
|
284 |
+ } |
|
285 |
+ |
|
286 |
+ fetch("/equipment/equipmentUpdate.json", { |
|
287 |
+ method: "POST", |
|
288 |
+ headers: { |
|
289 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
290 |
+ }, |
|
291 |
+ body: JSON.stringify(equipment), |
|
292 |
+ }).then((response) => response.json()).then((data) => { |
|
293 |
+ console.log('equipmentUpdate response : ', data); |
|
294 |
+ // 장비 목록 조회 |
|
295 |
+ equipmentSelectList(); |
|
296 |
+ // 장비 초기화 |
|
297 |
+ setEquipment(equipment_); |
|
298 |
+ // 모달창 닫기 |
|
299 |
+ setEquipmentOneModal(false); |
|
300 |
+ |
|
301 |
+ alert('수정이 완료됐습니다.'); |
|
302 |
+ }).catch((error) => { |
|
303 |
+ console.log('equipmentUpdate error : ', error); |
|
304 |
+ alert('수정에 실패했습니다.'); |
|
305 |
+ }); |
|
306 |
+ } |
|
307 |
+ |
|
308 |
+ // 장비 삭제 |
|
309 |
+ const equipmentDelete = () => { |
|
310 |
+ console.log('equipmentDelete Function Run'); |
|
311 |
+ |
|
312 |
+ // 삭제 확인 팝업 |
|
313 |
+ if (confirm('정말 삭제하시겠습니까?') == false) { |
|
314 |
+ return; |
|
315 |
+ } |
|
316 |
+ |
|
317 |
+ fetch("/equipment/equipmentDelete.json", { |
|
318 |
+ method: "POST", |
|
319 |
+ headers: { |
|
320 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
321 |
+ }, |
|
322 |
+ body: JSON.stringify(equipment), |
|
323 |
+ }).then((response) => response.json()).then((data) => { |
|
324 |
+ console.log('equipmentDelete response : ', data); |
|
325 |
+ // 장비 목록 조회 |
|
326 |
+ equipmentSelectList(); |
|
327 |
+ // 장비 초기화 |
|
328 |
+ setEquipment(equipment_); |
|
329 |
+ // 모달창 닫기 |
|
330 |
+ setEquipmentOneModal(false); |
|
331 |
+ |
|
332 |
+ alert('삭제가 완료됐습니다.'); |
|
333 |
+ }).catch((error) => { |
|
334 |
+ console.log('equipmentDelete error : ', error); |
|
335 |
+ alert('삭제에 실패했습니다.'); |
|
336 |
+ }); |
|
337 |
+ } |
|
338 |
+ |
|
252 | 339 |
|
253 | 340 |
// 장비 등록 th |
254 | 341 |
const equipmentInsertHead = [ |
... | ... | @@ -278,7 +365,7 @@ |
278 | 365 |
"입고 일자", |
279 | 366 |
"상태", |
280 | 367 |
"보유 기관", |
281 |
- "시행기관 관리", |
|
368 |
+ "기관 관리", |
|
282 | 369 |
]; |
283 | 370 |
const key1 = [ |
284 | 371 |
"equipment_name", |
... | ... | @@ -882,7 +969,7 @@ |
882 | 969 |
{equipmentList.length > 0 |
883 | 970 |
? equipmentList.map((item, index) => { |
884 | 971 |
return ( |
885 |
- <tr key={index}> |
|
972 |
+ <tr key={index} onClick={() => equipmentOneModalOpen(item)}> |
|
886 | 973 |
<td>{equipmentList.length - index}</td> |
887 | 974 |
{key1.map((kes) => { |
888 | 975 |
if(kes == "equipment_state") { |
... | ... | @@ -894,7 +981,7 @@ |
894 | 981 |
} |
895 | 982 |
return <td>{item[kes]}</td> |
896 | 983 |
})} |
897 |
- <td> |
|
984 |
+ <td onClick={(e) => e.stopPropagation()}> |
|
898 | 985 |
{ |
899 | 986 |
item['senior_id'] == null |
900 | 987 |
? <Button |
... | ... | @@ -908,6 +995,11 @@ |
908 | 995 |
onClick={seniorMatchDelete} |
909 | 996 |
/> |
910 | 997 |
} |
998 |
+ <Button |
|
999 |
+ className={"btn-small gray-btn"} |
|
1000 |
+ btnName={"삭제"} |
|
1001 |
+ onClick={() => seniorMatchInsertModalOpen(item)} |
|
1002 |
+ /> |
|
911 | 1003 |
</td> |
912 | 1004 |
</tr> |
913 | 1005 |
); |
... | ... | @@ -1176,6 +1268,101 @@ |
1176 | 1268 |
</div> |
1177 | 1269 |
</Modal> |
1178 | 1270 |
|
1271 |
+ <Modal open={equipmentOneModal} close={equipmentOneModalClose} header="장비 상세 조회"> |
|
1272 |
+ <div className="board-wrap"> |
|
1273 |
+ <div> |
|
1274 |
+ <table className="flex70 margin-bottom"> |
|
1275 |
+ <tbody className="equipment-insert"> |
|
1276 |
+ <tr> |
|
1277 |
+ <th>종류</th> |
|
1278 |
+ <td colSpan={5}> |
|
1279 |
+ <select value={equipment['equipment_type']} name="equipment_type" onChange={equipmentChange}> |
|
1280 |
+ <option value="" selected disabled>선택</option> |
|
1281 |
+ <option value="DIGITAL_MEDIBOX">디지털 약상자</option> |
|
1282 |
+ <option value="ECG" disabled>심전도계</option> |
|
1283 |
+ <option value="SPHYGMOMANOMETER" disabled>혈압계</option> |
|
1284 |
+ </select> |
|
1285 |
+ </td> |
|
1286 |
+ </tr> |
|
1287 |
+ <tr> |
|
1288 |
+ <th>모델 명</th> |
|
1289 |
+ <td colSpan={5}> |
|
1290 |
+ <input type="text" value={equipment['equipment_name']} id="equipmentName" name="equipment_name" placeholder="모델 명을 입력해 주세요" onChange={equipmentChange}></input> |
|
1291 |
+ {/* {smartMediBoxNameList.map((item) => { |
|
1292 |
+ return ( |
|
1293 |
+ <span> |
|
1294 |
+ <input type="radio" id={item} name="equipment_name" value={item}></input> |
|
1295 |
+ <label for={item}>{item}</label> |
|
1296 |
+ </span> |
|
1297 |
+ ) |
|
1298 |
+ })} */} |
|
1299 |
+ </td> |
|
1300 |
+ </tr> |
|
1301 |
+ <tr> |
|
1302 |
+ <th>시리얼 넘버</th> |
|
1303 |
+ <td colSpan={5}> |
|
1304 |
+ <input type="text" value={equipment['equipment_serial_number']} name="equipment_serial_number" placeholder="S/N을 입력해 주세요" disabled onChange={equipmentChange}/> |
|
1305 |
+ </td> |
|
1306 |
+ </tr> |
|
1307 |
+ <tr> |
|
1308 |
+ <th>생산 일자</th> |
|
1309 |
+ <td colSpan={5}> |
|
1310 |
+ <input type="date" value={equipment['equipment_product_date']} name="equipment_product_date" onChange={equipmentChange}/> |
|
1311 |
+ </td> |
|
1312 |
+ </tr> |
|
1313 |
+ <tr> |
|
1314 |
+ <th>입고 일자</th> |
|
1315 |
+ <td colSpan={5}> |
|
1316 |
+ <input type="date" value={equipment['equipment_stock_date']} name="equipment_stock_date" onChange={equipmentChange}/> |
|
1317 |
+ </td> |
|
1318 |
+ </tr> |
|
1319 |
+ </tbody> |
|
1320 |
+ </table> |
|
1321 |
+ |
|
1322 |
+ <table className={"caregiver-user"}> |
|
1323 |
+ <thead> |
|
1324 |
+ <tr> |
|
1325 |
+ {thead66.map((i) => { |
|
1326 |
+ return <th>{i}</th>; |
|
1327 |
+ })} |
|
1328 |
+ </tr> |
|
1329 |
+ </thead> |
|
1330 |
+ <tbody> |
|
1331 |
+ {seniorMatchListByEquipment.length > 0 |
|
1332 |
+ ? seniorMatchListByEquipment.map((item, index) => { |
|
1333 |
+ return ( |
|
1334 |
+ <tr> |
|
1335 |
+ {key66.map((kes) => { |
|
1336 |
+ return <td>{item[kes]}</td> |
|
1337 |
+ })} |
|
1338 |
+ </tr> |
|
1339 |
+ ); |
|
1340 |
+ }) |
|
1341 |
+ : <td colSpan={4}>조회된 데이터가 없습니다.</td> |
|
1342 |
+ } |
|
1343 |
+ </tbody> |
|
1344 |
+ </table> |
|
1345 |
+ </div> |
|
1346 |
+ <div justify-content="center"> |
|
1347 |
+ <Button |
|
1348 |
+ className={"btn-small gray-btn"} |
|
1349 |
+ btnName={"수정"} |
|
1350 |
+ onClick={equipmentUpdate} |
|
1351 |
+ /> |
|
1352 |
+ <Button |
|
1353 |
+ className={"btn-small gray-btn"} |
|
1354 |
+ btnName={"삭제"} |
|
1355 |
+ onClick={equipmentDelete} |
|
1356 |
+ /> |
|
1357 |
+ <Button |
|
1358 |
+ className={"btn-small gray-btn"} |
|
1359 |
+ btnName={"닫기"} |
|
1360 |
+ onClick={equipmentOneModalClose} |
|
1361 |
+ /> |
|
1362 |
+ </div> |
|
1363 |
+ </div> |
|
1364 |
+ </Modal> |
|
1365 |
+ |
|
1179 | 1366 |
<Modal open={modalOpen} close={closeModal} header="납품 기관 선택"> |
1180 | 1367 |
<div className="board-wrap"> |
1181 | 1368 |
<div> |
... | ... | @@ -1291,7 +1478,7 @@ |
1291 | 1478 |
</div> |
1292 | 1479 |
</Modal> |
1293 | 1480 |
|
1294 |
- <Modal open={agencyEquipmentOneModal} close={agencyEquipmentOneModalClose} header="장비 상세 조회"> |
|
1481 |
+ <Modal open={agencyEquipmentOneModal} close={agencyEquipmentOneModalClose} header="대상자 장비 상세 조회"> |
|
1295 | 1482 |
<div className="board-wrap"> |
1296 | 1483 |
<div> |
1297 | 1484 |
<table className={"caregiver-user"}> |
--- server/modules/web/Server.js
+++ server/modules/web/Server.js
... | ... | @@ -96,17 +96,19 @@ |
96 | 96 |
webServer.get('*', function (request, response, next) { |
97 | 97 |
response.sendFile(`${BASE_DIR}/client/views/index.html`); |
98 | 98 |
}) |
99 |
+ |
|
99 | 100 |
/** |
100 | 101 |
+ * @author : 방선주 |
101 | 102 |
+ * @since : 2023.02.14 |
102 | 103 |
+ * @dscription : REST API 서버에 데이터 요청 보내기(Proxy) |
103 | 104 |
+ */ |
104 |
-+webServer.use('*.json', expressProxy(API_SERVER_HOST, { |
|
105 |
- proxyReqPathResolver: function (request) { |
|
106 |
- //console.log('request : ', request.url, request.params[0]); |
|
107 |
- return `${request.params['0']}.json`; |
|
108 |
- } |
|
109 |
- })); |
|
105 |
+webServer.use('*.json', expressProxy(API_SERVER_HOST, { |
|
106 |
+ proxyReqPathResolver: function (request) { |
|
107 |
+ //console.log('request : ', request.url, request.params[0]); |
|
108 |
+ return `${request.params['0']}.json`; |
|
109 |
+ } |
|
110 |
+})); |
|
111 |
+ |
|
110 | 112 |
/** |
111 | 113 |
* @author : 최정우 |
112 | 114 |
* @since : 2022.09.21 |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?