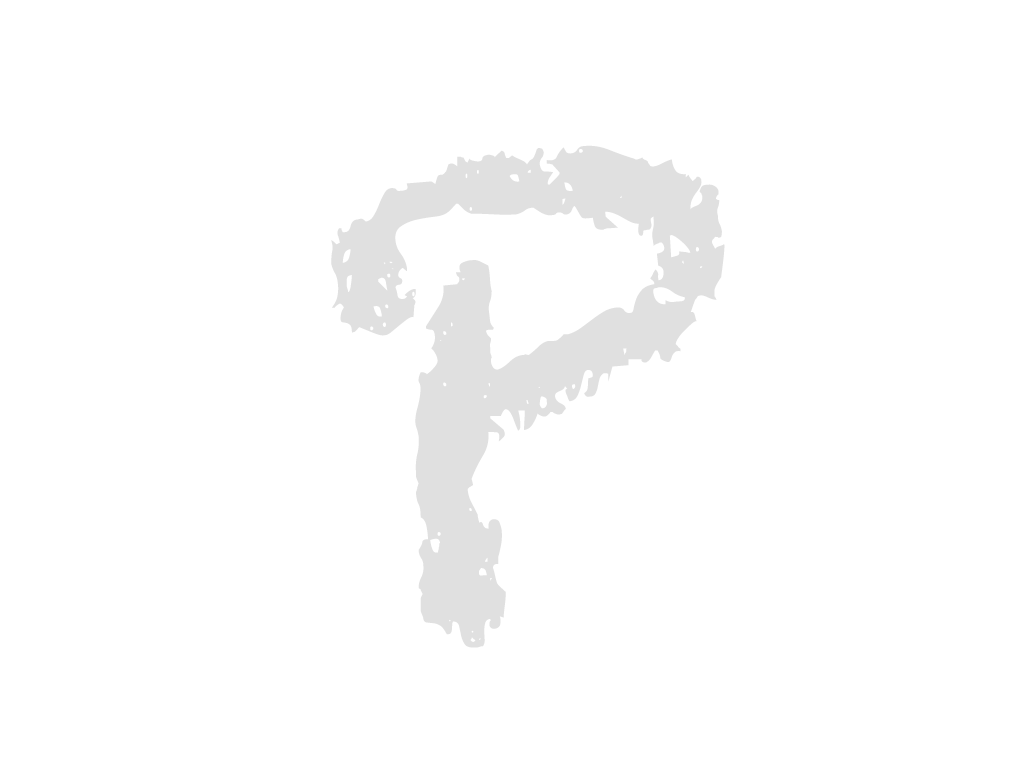
Merge branch 'admin' of http://210.180.118.83/yjryu/senior_care_system into admin
@0ec8a1431e24868c28e56e8054d84b06c07631b8
--- client/views/component/Modal_Guadian.jsx
+++ client/views/component/Modal_Guardian.jsx
... | ... | @@ -5,12 +5,13 @@ |
5 | 5 |
import Pagination from "./Pagination.jsx"; |
6 | 6 |
|
7 | 7 |
|
8 |
-export default function Modal({ open, close, header, useseniorId }) { |
|
9 |
- const seniorId = useseniorId; |
|
8 |
+export default function Modal_Guardian({ open, close, header, useSeniorId }) { |
|
9 |
+ |
|
10 |
+ const seniorId = useSeniorId; |
|
10 | 11 |
//대상자 - 보호자 매칭 리스트 |
11 | 12 |
const [sgMatchList, setSgMatchList] = React.useState([]); |
12 |
- const [addGuadian, setAddGuadian] = React.useState(true); //추가된 보호자가 있는지 확인 |
|
13 |
- |
|
13 |
+ const [guardianList, setGuardianList] = React.useState([]); |
|
14 |
+ const [addGuardian, setAddGuardian] = React.useState(true); //추가된 보호자가 있는지 확인 |
|
14 | 15 |
|
15 | 16 |
//사용자 등록 초기값 설정 |
16 | 17 |
const [userName, setUserName] = React.useState(""); |
... | ... | @@ -19,16 +20,17 @@ |
19 | 20 |
const [telNum, setTelNum] = React.useState(""); |
20 | 21 |
const [homeAddress, setHomeAddress] = React.useState(""); |
21 | 22 |
const [relationship, setRelationship] = React.useState(""); |
22 |
- const [matchState, setMatchState] = React.useState(true); |
|
23 |
+ const [guardianId, setGuardianId] = React.useState(""); |
|
23 | 24 |
|
24 | 25 |
//-------- 페이징 작업 설정 시작 --------// |
25 | 26 |
const limit = 5; // 페이지당 보여줄 공지 개수 |
26 | 27 |
const [page, setPage] = React.useState(1); //page index |
27 | 28 |
const offset = (page - 1) * limit; //게시물 위치 계산 |
28 |
- const [guadianTotal, setGuadianTotal] = React.useState(0); //최대길이 넣을 변수 |
|
29 |
+ const [guardianTotal, setGuardianTotal] = React.useState(0); //최대길이 넣을 변수 |
|
29 | 30 |
|
30 | 31 |
//-------- 변경되는 데이터 Handler 설정 --------// |
31 | 32 |
const handleUserName = (e) => { |
33 |
+ e.target.value = e.target.value.replace(/[^ㄱ-ㅎ|ㅏ-ㅣ|가-힣|a-z|A-Z]/g, ''); |
|
32 | 34 |
setUserName(e.target.value); |
33 | 35 |
}; |
34 | 36 |
const handleBrithday = (e) => { |
... | ... | @@ -41,15 +43,20 @@ |
41 | 43 |
const handelRelationship = (e) => { |
42 | 44 |
setRelationship(e.target.value); |
43 | 45 |
}; |
46 |
+ const handleGuarianId = (e) => { |
|
47 |
+ setGuardianId(e.target.value); |
|
48 |
+ }; |
|
49 |
+ |
|
44 | 50 |
// 데이터 초기화 함수 |
45 | 51 |
const dataReset = () => { |
52 |
+ setGuardianId(""); |
|
46 | 53 |
setUserName(""); |
47 | 54 |
setBrith(""); |
48 | 55 |
setTelNum(""); |
49 | 56 |
setRelationship(""); |
50 | 57 |
} |
51 | 58 |
// 매칭 리스트 출력 영역 |
52 |
- const getselectMatchList = () => { |
|
59 |
+ const getSelectMatchList = () => { |
|
53 | 60 |
fetch("/user/selectSeniorGuardianMatch.json", { |
54 | 61 |
method: "POST", |
55 | 62 |
headers: { |
... | ... | @@ -59,29 +66,50 @@ |
59 | 66 |
senior_id: seniorId, |
60 | 67 |
}), |
61 | 68 |
}).then((response) => response.json()).then((data) => { |
62 |
- console.log("getselectMatchList : ", data); |
|
69 |
+ console.log("getSelectMatchList : ", data); |
|
63 | 70 |
setSgMatchList(data); |
64 |
- setGuadianTotal(data.length); |
|
65 |
- setAddGuadian(false); |
|
71 |
+ setGuardianTotal(data.length); |
|
72 |
+ setAddGuardian(false); |
|
66 | 73 |
}).catch((error) => { |
67 |
- console.log('getselectMatchList() /user/selectSeniorGuardianMatch.json error : ', error); |
|
74 |
+ console.log('getSelectMatchList() /user/selectSeniorGuardianMatch.json error : ', error); |
|
68 | 75 |
}); |
69 | 76 |
}; |
77 |
+ |
|
78 |
+ // 보호자 리스트 불러오기 |
|
79 |
+ const getSelectGuardianList = () => { |
|
80 |
+ fetch("/user/selectUserList.json", { |
|
81 |
+ method: "POST", |
|
82 |
+ headers: { |
|
83 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
84 |
+ }, |
|
85 |
+ body: JSON.stringify({ |
|
86 |
+ agency_id: 'agency01', |
|
87 |
+ user_code: '3', |
|
88 |
+ }), |
|
89 |
+ }).then((response) => response.json()).then((data) => { |
|
90 |
+ const rowData = data; |
|
91 |
+ setGuardianList(rowData) |
|
92 |
+ |
|
93 |
+ }).catch((error) => { |
|
94 |
+ console.log('getSelectSeniorList() /user/selectUserList.json error : ', error); |
|
95 |
+ }); |
|
96 |
+ }; |
|
97 |
+ |
|
70 | 98 |
// 보호자 등록 영역 |
71 | 99 |
const insertUser = () => { |
72 |
- if(userName == ""){ |
|
100 |
+ if (userName == "") { |
|
73 | 101 |
alert("이름을 입력해 주세요."); |
74 |
- return ; |
|
75 |
- } else if(brith == "") { |
|
102 |
+ return; |
|
103 |
+ } else if (brith == "") { |
|
76 | 104 |
alert("생년월일을 선택해 주세요."); |
77 |
- return ; |
|
78 |
- } else if(telNum == "") { |
|
105 |
+ return; |
|
106 |
+ } else if (telNum == "") { |
|
79 | 107 |
alert("연락처를 입력해 선택해 주세요."); |
80 |
- return ; |
|
108 |
+ return; |
|
81 | 109 |
} |
82 | 110 |
var insertBtn = confirm("등록하시겠습니까?"); |
83 | 111 |
if (insertBtn) { |
84 |
- fetch("/user/insertSeniorData.json", { |
|
112 |
+ fetch("/user/insertUserData.json", { |
|
85 | 113 |
method: "POST", |
86 | 114 |
headers: { |
87 | 115 |
'Content-Type': 'application/json; charset=UTF-8' |
... | ... | @@ -101,13 +129,12 @@ |
101 | 129 |
console.log("보호자 등록"); |
102 | 130 |
insertGuadian(); |
103 | 131 |
}).catch((error) => { |
104 |
- console.log('insertUser() /user/insertSeniorData.json error : ', error); |
|
132 |
+ console.log('insertUser() /user/insertUserData.json error : ', error); |
|
105 | 133 |
}); |
106 | 134 |
} else { |
107 |
- return ; |
|
108 |
- } |
|
135 |
+ return; |
|
136 |
+ } |
|
109 | 137 |
} |
110 |
- |
|
111 | 138 |
const insertGuadian = () => { |
112 | 139 |
fetch("/user/insertGuardian.json", { |
113 | 140 |
method: "POST", |
... | ... | @@ -119,7 +146,7 @@ |
119 | 146 |
}), |
120 | 147 |
}).then((response) => response.json()).then((data) => { |
121 | 148 |
console.log("보호자 테이블 데이터 등록"); |
122 |
- matchSeniorGuadian(); |
|
149 |
+ matchSeniorGuadian2(); |
|
123 | 150 |
}).catch((error) => { |
124 | 151 |
console.log('insertGuadian() /user/insertGuardian.json error : ', error); |
125 | 152 |
}); |
... | ... | @@ -132,15 +159,34 @@ |
132 | 159 |
'Content-Type': 'application/json; charset=UTF-8' |
133 | 160 |
}, |
134 | 161 |
body: JSON.stringify({ |
135 |
- senior_id: useseniorId, |
|
136 |
- user_phonenumber: telNum, |
|
162 |
+ senior_id: seniorId, |
|
163 |
+ guardian_id: guardianId, |
|
137 | 164 |
senior_relationship: relationship, |
138 |
- guardian_match_state: matchState, |
|
139 | 165 |
}), |
140 | 166 |
}).then((response) => response.json()).then((data) => { |
141 | 167 |
console.log("매칭 등록"); |
142 | 168 |
dataReset(); |
143 |
- setAddGuadian(true); |
|
169 |
+ setAddGuardian(true); |
|
170 |
+ }).catch((error) => { |
|
171 |
+ console.log('matchSeniorGuadian() /user/insertSeniorGuardianMatch.json error : ', error); |
|
172 |
+ }); |
|
173 |
+ }; |
|
174 |
+ |
|
175 |
+ const matchSeniorGuadian2 = () => { |
|
176 |
+ fetch("/user/insertSeniorGuardianMatch.json", { |
|
177 |
+ method: "POST", |
|
178 |
+ headers: { |
|
179 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
180 |
+ }, |
|
181 |
+ body: JSON.stringify({ |
|
182 |
+ senior_id: seniorId, |
|
183 |
+ user_phonenumber: telNum, |
|
184 |
+ senior_relationship: relationship, |
|
185 |
+ }), |
|
186 |
+ }).then((response) => response.json()).then((data) => { |
|
187 |
+ console.log("매칭 등록"); |
|
188 |
+ dataReset(); |
|
189 |
+ setAddGuardian(true); |
|
144 | 190 |
}).catch((error) => { |
145 | 191 |
console.log('matchSeniorGuadian() /user/insertSeniorGuardianMatch.json error : ', error); |
146 | 192 |
}); |
... | ... | @@ -164,8 +210,9 @@ |
164 | 210 |
]; |
165 | 211 |
|
166 | 212 |
React.useEffect(() => { |
167 |
- getselectMatchList(); |
|
168 |
- }, [addGuadian]) |
|
213 |
+ getSelectGuardianList(); |
|
214 |
+ getSelectMatchList(); |
|
215 |
+ }, [addGuardian]) |
|
169 | 216 |
|
170 | 217 |
return ( |
171 | 218 |
<div class={open ? "openModal modal" : "modal"}> |
... | ... | @@ -177,6 +224,38 @@ |
177 | 224 |
</div> |
178 | 225 |
<div className="modal-main"> |
179 | 226 |
<div className="board-wrap"> |
227 |
+ <SubTitle explanation={"이미 등록된 사용자는 기존 ID,PW를 사용하시면 됩니다."} className="margin-bottom" /> |
|
228 |
+ <table className="margin-bottom2 senior-insert"> |
|
229 |
+ <tr> |
|
230 |
+ <th>검색</th> |
|
231 |
+ <input type="text" list="protectorlist" value={guardianId} onChange={handleGuarianId} /> |
|
232 |
+ <datalist id="protectorlist"> |
|
233 |
+ {/* <option value="가족1(ID)"></option> |
|
234 |
+ <option value="가족2(ID)"></option> */} |
|
235 |
+ {guardianList.map((item) => { |
|
236 |
+ // console.log('item : ',item) |
|
237 |
+ // setGaudianId(item['user_id']) |
|
238 |
+ // setTelNum(item['user_phonenumber']) |
|
239 |
+ return <option value={item['user_id']}>{item['user_name']}({item['user_id']})</option> |
|
240 |
+ })} |
|
241 |
+ </datalist> |
|
242 |
+ </tr> |
|
243 |
+ <tr> |
|
244 |
+ <th>대상자와의 관계</th> |
|
245 |
+ <td colSpan={3}> |
|
246 |
+ <input type="text" value={relationship} onChange={handelRelationship} /> |
|
247 |
+ </td> |
|
248 |
+ </tr> |
|
249 |
+ </table> |
|
250 |
+ <div className="btn-wrap flex-center margin-bottom5"> |
|
251 |
+ <Button |
|
252 |
+ className={"btn-small green-btn"} |
|
253 |
+ btnName={"등록"} |
|
254 |
+ onClick={() => { |
|
255 |
+ matchSeniorGuadian(); |
|
256 |
+ }} |
|
257 |
+ /> |
|
258 |
+ </div> |
|
180 | 259 |
<SubTitle explanation={"최초 ID는 연락처, PW는 생년월일 8자리입니다."} className="margin-bottom" /> |
181 | 260 |
<table className="margin-bottom2 senior-insert"> |
182 | 261 |
<tr> |
... | ... | @@ -204,26 +283,12 @@ |
204 | 283 |
</td> |
205 | 284 |
</tr> |
206 | 285 |
</table> |
207 |
- |
|
208 |
- <SubTitle explanation={"이미 등록된 사용자는 기존 ID,PW를 사용하시면 됩니다."} className="margin-bottom" /> |
|
209 |
- <div className="flex-start"> |
|
210 |
- <Button |
|
211 |
- className={"btn-small red-btn"} |
|
212 |
- btnName={"찾기"} |
|
213 |
- /> |
|
214 |
- <input type="text" list="protectorlist" /> |
|
215 |
- <datalist id="protectorlist"> |
|
216 |
- <option value="가족1(ID)"></option> |
|
217 |
- <option value="가족2(ID)"></option> |
|
218 |
- </datalist> |
|
219 |
- </div> |
|
220 | 286 |
<div className="btn-wrap flex-center margin-bottom5"> |
221 | 287 |
<Button |
222 |
- className={"btn-small red-btn"} |
|
288 |
+ className={"btn-small green-btn"} |
|
223 | 289 |
btnName={"추가"} |
224 | 290 |
onClick={() => { |
225 | 291 |
insertUser() |
226 |
- getselectMatchList(); |
|
227 | 292 |
}} |
228 | 293 |
/> |
229 | 294 |
</div> |
... | ... | @@ -239,7 +304,7 @@ |
239 | 304 |
/> |
240 | 305 |
</div> |
241 | 306 |
<div> |
242 |
- <Pagination total={guadianTotal} limit={limit} page={page} setPage={setPage} /> |
|
307 |
+ <Pagination total={guardianTotal} limit={limit} page={page} setPage={setPage} /> |
|
243 | 308 |
</div> |
244 | 309 |
</div> |
245 | 310 |
</div> |
... | ... | @@ -248,4 +313,4 @@ |
248 | 313 |
</div> |
249 | 314 |
|
250 | 315 |
); |
251 |
-} |
|
316 |
+}(파일 끝에 줄바꿈 문자 없음) |
--- client/views/component/Modal_SeniorInsert.jsx
+++ client/views/component/Modal_SeniorInsert.jsx
... | ... | @@ -4,6 +4,7 @@ |
4 | 4 |
|
5 | 5 |
export default function Modal({ open, close, header, setModalOpen3, setAddSenior }) { |
6 | 6 |
// 사용자 등록 시 초기값 세팅 |
7 |
+ // const [seniorId, setSeniorId] = React.useState(""); |
|
7 | 8 |
const [userName, setUserName] = React.useState(""); |
8 | 9 |
const [gender, setGender] = React.useState(""); |
9 | 10 |
const [brith, setBrith] = React.useState(""); |
... | ... | @@ -15,8 +16,15 @@ |
15 | 16 |
const [medication, setMedication] = React.useState(""); |
16 | 17 |
const [note, setNote] = React.useState(""); |
17 | 18 |
|
19 |
+ // //id blor 처리 진행 |
|
20 |
+ // const inputRef = React.useRef(); |
|
21 |
+ // const settingBlor = (e) => { |
|
22 |
+ // inputRef.current.blur(); |
|
23 |
+ // } |
|
24 |
+ |
|
18 | 25 |
// 등록 후 초기화 진행 |
19 | 26 |
const dataReset = () => { |
27 |
+ // setSeniorId(""); |
|
20 | 28 |
setUserName(""); |
21 | 29 |
setGender(""); |
22 | 30 |
setBrith(""); |
... | ... | @@ -29,7 +37,12 @@ |
29 | 37 |
setNote(""); |
30 | 38 |
} |
31 | 39 |
//-------- 변경되는 데이터 Handler 설정 --------// |
40 |
+ // const handleUserId = (e) => { |
|
41 |
+ // console.log(e.target.value); |
|
42 |
+ // setSeniorId(e.target.value); |
|
43 |
+ // }; |
|
32 | 44 |
const handleUserName = (e) => { |
45 |
+ e.target.value=e.target.value.replace(/[^ㄱ-ㅎ|ㅏ-ㅣ|가-힣|a-z|A-Z]/g, ''); |
|
33 | 46 |
setUserName(e.target.value); |
34 | 47 |
}; |
35 | 48 |
const handleGender = (e) => { |
... | ... | @@ -83,7 +96,7 @@ |
83 | 96 |
} |
84 | 97 |
var insertBtn = confirm("등록하시겠습니까?"); |
85 | 98 |
if (insertBtn) { |
86 |
- fetch("/user/insertSeniorData.json", { |
|
99 |
+ fetch("/user/insertUserData.json", { |
|
87 | 100 |
method: "POST", |
88 | 101 |
headers: { |
89 | 102 |
'Content-Type': 'application/json; charset=UTF-8' |
... | ... | @@ -102,7 +115,7 @@ |
102 | 115 |
console.log("대상자 정보 등록"); |
103 | 116 |
InsertUserPillData(); |
104 | 117 |
}).catch((error) => { |
105 |
- console.log('insertSeniorData() /user/insertSeniorData.json error : ', error); |
|
118 |
+ console.log('insertUserData() /user/insertUserData.json error : ', error); |
|
106 | 119 |
}); |
107 | 120 |
} else { |
108 | 121 |
return; |
... | ... | @@ -137,6 +150,7 @@ |
137 | 150 |
//-------- 등록 버튼 동작 수행 영역 끝 --------/ |
138 | 151 |
//-------- 모달 종료 --------> |
139 | 152 |
const noticeModalClose = () => { |
153 |
+ dataReset(); |
|
140 | 154 |
setModalOpen3(false); |
141 | 155 |
}; |
142 | 156 |
return ( |
... | ... | @@ -161,7 +175,13 @@ |
161 | 175 |
/> |
162 | 176 |
</td> |
163 | 177 |
</tr> */} |
164 |
- <tr> |
|
178 |
+ {/* <tr> |
|
179 |
+ <th>아이디</th> |
|
180 |
+ <td> |
|
181 |
+ <input type="text" value={`${telNum}_${userName}`.replace(/[-]/g,'')} onChange={handleUserId} onClick={(e) => settingBlor(e.target.value)} ref={inputRef} style={{backgroundColor : `#D3D3D3`}} readonly/> |
|
182 |
+ </td> |
|
183 |
+ </tr>*/} |
|
184 |
+ <tr> |
|
165 | 185 |
<th>이름</th> |
166 | 186 |
<td> |
167 | 187 |
<input type="text" value={userName} onChange={handleUserName} /> |
--- client/views/component/Table.jsx
+++ client/views/component/Table.jsx
... | ... | @@ -1,7 +1,7 @@ |
1 | 1 |
import React from "react"; |
2 | 2 |
import Button from "./Button.jsx"; |
3 | 3 |
import Modal_Matching from "./Modal_Matching.jsx"; |
4 |
-import Modal_Guadian from "./Modal_Guadian.jsx"; |
|
4 |
+import Modal_Guardian from "./Modal_Guardian.jsx"; |
|
5 | 5 |
import { useNavigate } from "react-router"; |
6 | 6 |
// import styled from "styled-components"; |
7 | 7 |
|
... | ... | @@ -19,7 +19,7 @@ |
19 | 19 |
// 모달 title에 대상자 명 출력을 위함 |
20 | 20 |
const [useName, setUseUserName] = React.useState(""); |
21 | 21 |
// 매칭을 위해 대상자 ID 값 전달을 위함 |
22 |
- const [useseniorId, setUseSeniorId] = React.useState(""); |
|
22 |
+ const [useSeniorId, setUseSeniorId] = React.useState(""); |
|
23 | 23 |
|
24 | 24 |
const [modalOpen, setModalOpen] = React.useState(false); |
25 | 25 |
const openModal = () => { |
... | ... | @@ -39,7 +39,34 @@ |
39 | 39 |
setModalOpen2(false); |
40 | 40 |
}; |
41 | 41 |
|
42 |
- const buttonPrint = (name, id) => { |
|
42 |
+ // 시니어 보호자 매칭 제거 |
|
43 |
+ const updateSeniorGuardianMatch = (useSeniorId,useGuardianId) => { |
|
44 |
+ console.log("useSeniorId",useSeniorId); |
|
45 |
+ console.log("useGuardianId",useGuardianId); |
|
46 |
+ var insertBtn = confirm("삭제하시겠습니까?"); |
|
47 |
+ if (insertBtn) { |
|
48 |
+ fetch("/user/updateGuardianMatchEnd.json", { |
|
49 |
+ method: "POST", |
|
50 |
+ headers: { |
|
51 |
+ 'Content-Type': 'application/json; charset=UTF-8' |
|
52 |
+ }, |
|
53 |
+ body: JSON.stringify({ |
|
54 |
+ senior_id: useSeniorId, |
|
55 |
+ guardian_id: useGuardianId, |
|
56 |
+ }), |
|
57 |
+ }).then((response) => response.json()).then((data) => { |
|
58 |
+ alert('삭제되었습니다.'); |
|
59 |
+ }).catch((error) => { |
|
60 |
+ console.log('updateSeniorGuardianMatch() /user/updateGuardianMatchEnd.json error : ', error); |
|
61 |
+ }); |
|
62 |
+ } else { |
|
63 |
+ return; |
|
64 |
+ } |
|
65 |
+ } |
|
66 |
+ |
|
67 |
+ |
|
68 |
+ |
|
69 |
+ const buttonPrint = (name, id, guardianId, seniorId) => { |
|
43 | 70 |
if (view == "mySenior") { |
44 | 71 |
return ( |
45 | 72 |
<td> |
... | ... | @@ -71,7 +98,7 @@ |
71 | 98 |
<Button |
72 | 99 |
className={"btn-small gray-btn"} |
73 | 100 |
btnName={"보기"} |
74 |
- onClick={(e) => { |
|
101 |
+ onClick={() => { |
|
75 | 102 |
setUseUserName(name); |
76 | 103 |
setUseSeniorId(id); |
77 | 104 |
openModal(); |
... | ... | @@ -82,6 +109,20 @@ |
82 | 109 |
); |
83 | 110 |
} else if (view == "qna") { |
84 | 111 |
return; |
112 |
+ } else if (view == "guadianMatch") { |
|
113 |
+ return ( |
|
114 |
+ <td> |
|
115 |
+ <Button |
|
116 |
+ className={"btn-small gray-btn"} |
|
117 |
+ btnName={"삭제"} |
|
118 |
+ onClick={() => { |
|
119 |
+ // setUseSeniorId(seniorId); |
|
120 |
+ // setUseGuardianId(guardianId); |
|
121 |
+ updateSeniorGuardianMatch(seniorId,guardianId,name,id); |
|
122 |
+ }} |
|
123 |
+ /> |
|
124 |
+ </td> |
|
125 |
+ ); |
|
85 | 126 |
} else { |
86 | 127 |
return ( |
87 | 128 |
<td> |
... | ... | @@ -96,7 +137,6 @@ |
96 | 137 |
); |
97 | 138 |
} |
98 | 139 |
}; |
99 |
- |
|
100 | 140 |
return ( |
101 | 141 |
<> |
102 | 142 |
{/* 담당자 보기 모달 */} |
... | ... | @@ -107,11 +147,11 @@ |
107 | 147 |
/> |
108 | 148 |
{/* 보호자 보기 모달창 */} |
109 | 149 |
{modalOpen ? ( |
110 |
- <Modal_Guadian |
|
150 |
+ <Modal_Guardian |
|
111 | 151 |
open={modalOpen} |
112 | 152 |
close={closeModal} |
113 | 153 |
header={useName + "님의 가족"} |
114 |
- useseniorId={useseniorId} |
|
154 |
+ useSeniorId={useSeniorId} |
|
115 | 155 |
/> |
116 | 156 |
) : null} |
117 | 157 |
<table className={className}> |
... | ... | @@ -126,6 +166,8 @@ |
126 | 166 |
{contents.slice(offset, offset + limit).map((i, index) => { |
127 | 167 |
const userName = i.user_name; |
128 | 168 |
const userId = i.user_id; |
169 |
+ const guardianId = i.guardian_id; |
|
170 |
+ const seniorId = i.senior_id; |
|
129 | 171 |
return ( |
130 | 172 |
<tr key={index}> |
131 | 173 |
{contentKey.map((kes) => { |
... | ... | @@ -150,7 +192,7 @@ |
150 | 192 |
</> |
151 | 193 |
); |
152 | 194 |
})} |
153 |
- {buttonPrint(userName, userId)} |
|
195 |
+ {buttonPrint(userName, userId, guardianId, seniorId)} |
|
154 | 196 |
</tr> |
155 | 197 |
); |
156 | 198 |
})} |
--- client/views/pages/callcenter/QuestionConfirm.jsx
+++ client/views/pages/callcenter/QuestionConfirm.jsx
... | ... | @@ -8,7 +8,38 @@ |
8 | 8 |
|
9 | 9 |
const navigate = useNavigate(); |
10 | 10 |
|
11 |
+ const [username, setUsername] = React.useState(""); |
|
12 |
+ const [qnaOne, setQnaOne] = React.useState([]); |
|
11 | 13 |
|
14 |
+ |
|
15 |
+ //qna 조회 |
|
16 |
+ const getQnaOne = () => { |
|
17 |
+ fetch("/qna/qnaSelectOne.json", { |
|
18 |
+ method: "POST", |
|
19 |
+ headers: { |
|
20 |
+ "Content-Type": 'application/json; charset=UTF-8', |
|
21 |
+ }, |
|
22 |
+ body: JSON.stringify({ |
|
23 |
+ qna_insert_user_name : username |
|
24 |
+ |
|
25 |
+ }), |
|
26 |
+ }) |
|
27 |
+ .then((response) => response.json()) |
|
28 |
+ .then((data) => { |
|
29 |
+ console.log(data[3]); |
|
30 |
+ setQnaOne(data[3]); |
|
31 |
+ }) |
|
32 |
+ .catch((error) => { |
|
33 |
+ console.log("qna error : ", error); |
|
34 |
+ }); |
|
35 |
+ }; |
|
36 |
+ |
|
37 |
+ React.useEffect(() => { |
|
38 |
+ |
|
39 |
+ getQnaOne(); |
|
40 |
+ |
|
41 |
+ }, []) |
|
42 |
+ |
|
12 | 43 |
return ( |
13 | 44 |
<main> |
14 | 45 |
<div className="content-wrap row"> |
... | ... | @@ -17,29 +48,23 @@ |
17 | 48 |
<table className="margin-bottom2 senior-insert"> |
18 | 49 |
<tr> |
19 | 50 |
<th>작성자</th> |
20 |
- <td> |
|
21 |
- 김가족 |
|
22 |
- </td> |
|
51 |
+ <td>{qnaOne.qna_insert_user_name}</td> |
|
23 | 52 |
</tr> |
24 | 53 |
|
25 | 54 |
<tr> |
26 | 55 |
<th>제목</th> |
27 |
- <td colSpan={3}> |
|
28 |
- 문의 |
|
29 |
- </td> |
|
56 |
+ <td colSpan={3}>{qnaOne.qna_title}</td> |
|
30 | 57 |
</tr> |
31 | 58 |
<tr> |
32 |
- <th>내용</th> |
|
33 |
- <td colSpan={3}> |
|
34 |
- 문의합니다. |
|
35 |
- </td> |
|
36 |
- </tr> |
|
37 |
- <tr> |
|
38 |
- <th>답변하기</th> |
|
39 |
- <td colSpan={3}> |
|
40 |
- <textarea className="medicine" cols="30" rows="2"></textarea> |
|
41 |
- </td> |
|
42 |
- </tr> |
|
59 |
+ <th>내용</th> |
|
60 |
+ <td colSpan={3}>{qnaOne.qna_content}</td> |
|
61 |
+ </tr> |
|
62 |
+ <tr> |
|
63 |
+ <th>답변하기</th> |
|
64 |
+ <td colSpan={3}> |
|
65 |
+ <textarea className="medicine" cols="30" rows="2"></textarea> |
|
66 |
+ </td> |
|
67 |
+ </tr> |
|
43 | 68 |
</table> |
44 | 69 |
|
45 | 70 |
<div className="btn-wrap flex-center"> |
... | ... | @@ -62,3 +87,114 @@ |
62 | 87 |
</main> |
63 | 88 |
); |
64 | 89 |
} |
90 |
+ |
|
91 |
+// import React from "react"; |
|
92 |
+// import Button from "../../component/Button.jsx"; |
|
93 |
+// import ContentTitle from "../../component/ContentTitle.jsx"; |
|
94 |
+// import SubTitle from "../../component/SubTitle.jsx"; |
|
95 |
+// import { useNavigate } from "react-router"; |
|
96 |
+// import { useParams } from "react-router"; |
|
97 |
+ |
|
98 |
+// export default function QuestionConfirm() { |
|
99 |
+// const navigate = useNavigate(); |
|
100 |
+ |
|
101 |
+ |
|
102 |
+// const [username, setUsername] = React.useState(""); |
|
103 |
+// const [qnaOne, setQnaOne] = React.useState([]); |
|
104 |
+ |
|
105 |
+ |
|
106 |
+// //qna 조회 |
|
107 |
+// const getQnaOne = () => { |
|
108 |
+// fetch("/qna/qnaSelectOne.json", { |
|
109 |
+// method: "POST", |
|
110 |
+// headers: { |
|
111 |
+// "Content-Type": 'application/json; charset=UTF-8', |
|
112 |
+// }, |
|
113 |
+// body: JSON.stringify({ |
|
114 |
+// qna_insert_user_name : username |
|
115 |
+ |
|
116 |
+// }), |
|
117 |
+// }) |
|
118 |
+// .then((response) => response.json()) |
|
119 |
+// .then((data) => { |
|
120 |
+// console.log(data[3]); |
|
121 |
+// setQnaOne(data[3]); |
|
122 |
+// }) |
|
123 |
+// .catch((error) => { |
|
124 |
+// console.log("qna error : ", error); |
|
125 |
+// }); |
|
126 |
+// }; |
|
127 |
+ |
|
128 |
+ |
|
129 |
+// // const getSeniorDataOne = () => { |
|
130 |
+// // fetch("/user/selectSeniorOne.json", { |
|
131 |
+// // method: "POST", |
|
132 |
+// // headers: { |
|
133 |
+// // 'Content-Type': 'application/json; charset=UTF-8' |
|
134 |
+// // }, |
|
135 |
+// // body: JSON.stringify({ |
|
136 |
+// // user_id: seniorId |
|
137 |
+// // }), |
|
138 |
+// // }).then((response) => response.json()).then((data) => { |
|
139 |
+// // console.log("data : ", data[0]); |
|
140 |
+// // setSeniorOne(data[0]); |
|
141 |
+ |
|
142 |
+// // }).catch((error) => { |
|
143 |
+// // console.log('getSeniorDataOne() /user/selectSeniorOne.json error : ', error); |
|
144 |
+// // }); |
|
145 |
+// // }; |
|
146 |
+ |
|
147 |
+// React.useEffect(() => { |
|
148 |
+ |
|
149 |
+// getQnaOne(); |
|
150 |
+ |
|
151 |
+// }, []) |
|
152 |
+ |
|
153 |
+ |
|
154 |
+// return ( |
|
155 |
+// <main> |
|
156 |
+// <div className="content-wrap row"> |
|
157 |
+// <ContentTitle contentTitle={"문의글 작성"} /> |
|
158 |
+// <SubTitle explanation={"작성자 정보"} /> |
|
159 |
+// <table className="margin-bottom2 senior-insert"> |
|
160 |
+// <tr> |
|
161 |
+// <th>작성자</th> |
|
162 |
+// <td>{qnaOne.qna_insert_user_name}</td> |
|
163 |
+// </tr> |
|
164 |
+ |
|
165 |
+// <tr> |
|
166 |
+// <th>제목</th> |
|
167 |
+// <td colSpan={3}>{qnaOne.qna_title}</td> |
|
168 |
+// </tr> |
|
169 |
+// <tr> |
|
170 |
+// <th>내용</th> |
|
171 |
+// <td colSpan={3}>{qnaOne.qna_content}</td> |
|
172 |
+// </tr> |
|
173 |
+// <tr> |
|
174 |
+// <th>답변하기</th> |
|
175 |
+// <td colSpan={3}> |
|
176 |
+// <textarea className="medicine" cols="30" rows="2"></textarea> |
|
177 |
+// </td> |
|
178 |
+// </tr> |
|
179 |
+// </table> |
|
180 |
+ |
|
181 |
+// <div className="btn-wrap flex-center"> |
|
182 |
+// <Button |
|
183 |
+// className={"btn-large gray-btn"} |
|
184 |
+// btnName={"이전"} |
|
185 |
+// onClick={() => { |
|
186 |
+// navigate("/QuestionSelect"); |
|
187 |
+// }} |
|
188 |
+// /> |
|
189 |
+// <Button |
|
190 |
+// className={"btn-large green-btn"} |
|
191 |
+// btnName={"등록"} |
|
192 |
+// onClick={() => { |
|
193 |
+// navigate("/QuestionSelect"); |
|
194 |
+// }} |
|
195 |
+// /> |
|
196 |
+// </div> |
|
197 |
+// </div> |
|
198 |
+// </main> |
|
199 |
+// ); |
|
200 |
+// } |
--- client/views/pages/senior_management/SeniorEdit.jsx
+++ client/views/pages/senior_management/SeniorEdit.jsx
... | ... | @@ -29,7 +29,7 @@ |
29 | 29 |
setMedicineL(data[0].lunch_medication_check) |
30 | 30 |
setMedicineD(data[0].dinner_medication_check) |
31 | 31 |
setMedication(data[0].medication_pill) |
32 |
- setNote(data[0].user_phonenumber) |
|
32 |
+ setNote(data[0].senior_note) |
|
33 | 33 |
|
34 | 34 |
}).catch((error) => { |
35 | 35 |
console.log('getSeniorDataOne() /user/selectSeniorOne.json error : ', error); |
--- client/views/pages/senior_management/SeniorSelectOne.jsx
+++ client/views/pages/senior_management/SeniorSelectOne.jsx
... | ... | @@ -108,9 +108,11 @@ |
108 | 108 |
}} |
109 | 109 |
/> |
110 | 110 |
<Button |
111 |
- className={"btn-large green-btn"} |
|
111 |
+ className={"btn-large red-btn"} |
|
112 | 112 |
btnName={"삭제"} |
113 |
- |
|
113 |
+ onClick={() => { |
|
114 |
+ alert("삭제 기능 만들거지롱."); |
|
115 |
+ }} |
|
114 | 116 |
/> |
115 | 117 |
</div> |
116 | 118 |
</div> |
--- client/views/pages/user_management/UserAuthoriySelect_agency.jsx
+++ client/views/pages/user_management/UserAuthoriySelect_agency.jsx
... | ... | @@ -47,12 +47,12 @@ |
47 | 47 |
}), |
48 | 48 |
}).then((response) => response.json()).then((data) => { |
49 | 49 |
const rowData = data; |
50 |
- if(addSenior || rowData.length !=data.length){ |
|
50 |
+ |
|
51 | 51 |
console.log(data); |
52 | 52 |
setSeniorList(rowData); |
53 | 53 |
setUserTotal(rowData.length); |
54 | 54 |
setAddSenior(false); |
55 |
- } |
|
55 |
+ |
|
56 | 56 |
|
57 | 57 |
}).catch((error) => { |
58 | 58 |
console.log('getSelectSeniorList() /user/selectUserList.json error : ', error); |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?