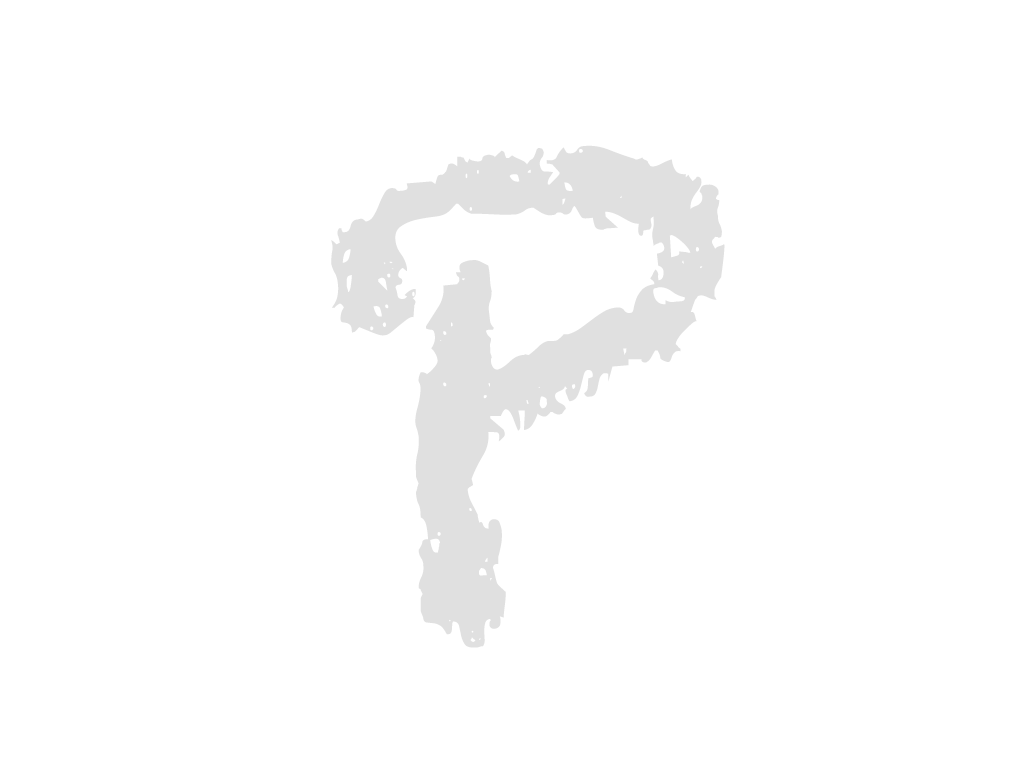
--- app/src/main/java/co/kr/ajinpaper/salesTask/MainActivity.java
+++ app/src/main/java/co/kr/ajinpaper/salesTask/MainActivity.java
... | ... | @@ -1,5 +1,6 @@ |
1 | 1 |
package co.kr.ajinpaper.salesTask; |
2 | 2 |
|
3 |
+import androidx.activity.OnBackPressedCallback; |
|
3 | 4 |
import androidx.activity.result.ActivityResultLauncher; |
4 | 5 |
import androidx.activity.result.contract.ActivityResultContracts; |
5 | 6 |
import androidx.annotation.NonNull; |
... | ... | @@ -31,8 +32,9 @@ |
31 | 32 |
public class MainActivity extends AppCompatActivity { |
32 | 33 |
private ValueCallback<Uri[]> mUploadMessage; |
33 | 34 |
private Uri mCapturedImageURI = null; |
34 |
- |
|
35 | 35 |
private PermissionManager permission; |
36 |
+ private long lastBackPressedTime = 0; // 마지막으로 뒤로 가기 버튼이 눌린 시간을 기록하는 변수 |
|
37 |
+ private static final int BACK_PRESS_INTERVAL = 2000; // 2초 간격 |
|
36 | 38 |
|
37 | 39 |
@SuppressLint("SetJavaScriptEnabled") |
38 | 40 |
@Override |
... | ... | @@ -51,6 +53,24 @@ |
51 | 53 |
SharedPreferences.Editor editor = sharedPreferences.edit(); |
52 | 54 |
editor.putString("pushTitle", title).apply(); |
53 | 55 |
} |
56 |
+ |
|
57 |
+ // 뒤로 가기 버튼으로 뒤로 가기 구현 |
|
58 |
+ OnBackPressedCallback callback = new OnBackPressedCallback(true) { |
|
59 |
+ @Override |
|
60 |
+ public void handleOnBackPressed() { |
|
61 |
+ if (myWebView.canGoBack()) { |
|
62 |
+ myWebView.goBack(); |
|
63 |
+ } else { |
|
64 |
+ if (lastBackPressedTime + BACK_PRESS_INTERVAL > System.currentTimeMillis()) { |
|
65 |
+ finish(); |
|
66 |
+ } else { |
|
67 |
+ Toast.makeText(MainActivity.this, "뒤로 가기 버튼을 한 번 더 누르면 종료됩니다.", Toast.LENGTH_SHORT).show(); |
|
68 |
+ } |
|
69 |
+ lastBackPressedTime = System.currentTimeMillis(); |
|
70 |
+ } |
|
71 |
+ } |
|
72 |
+ }; |
|
73 |
+ getOnBackPressedDispatcher().addCallback(this, callback); |
|
54 | 74 |
|
55 | 75 |
WebSettings webSettings = myWebView.getSettings(); |
56 | 76 |
webSettings.setJavaScriptEnabled(true); |
... | ... | @@ -89,7 +109,7 @@ |
89 | 109 |
} |
90 | 110 |
} |
91 | 111 |
|
92 |
- Intent contentSelectionIntent = new Intent(Intent.ACTION_OPEN_DOCUMENT); // 변경된 부분 |
|
112 |
+ Intent contentSelectionIntent = new Intent(Intent.ACTION_OPEN_DOCUMENT); |
|
93 | 113 |
contentSelectionIntent.addCategory(Intent.CATEGORY_OPENABLE); |
94 | 114 |
contentSelectionIntent.setType("image/*"); |
95 | 115 |
contentSelectionIntent.putExtra(Intent.EXTRA_ALLOW_MULTIPLE, true); |
... | ... | @@ -106,7 +126,7 @@ |
106 | 126 |
chooserIntent.putExtra(Intent.EXTRA_TITLE, "Image Chooser"); |
107 | 127 |
chooserIntent.putExtra(Intent.EXTRA_INITIAL_INTENTS, intentArray); |
108 | 128 |
|
109 |
- mFileChooser.launch(chooserIntent); // 수정된 부분 |
|
129 |
+ mFileChooser.launch(chooserIntent); |
|
110 | 130 |
|
111 | 131 |
return true; |
112 | 132 |
} |
... | ... | @@ -117,6 +137,7 @@ |
117 | 137 |
|
118 | 138 |
} |
119 | 139 |
|
140 |
+ // 이미지 파일 등록 가능하도록 |
|
120 | 141 |
private final ActivityResultLauncher<Intent> mFileChooser = registerForActivityResult( |
121 | 142 |
new ActivityResultContracts.StartActivityForResult(), |
122 | 143 |
result -> { |
... | ... | @@ -167,6 +188,7 @@ |
167 | 188 |
} |
168 | 189 |
} |
169 | 190 |
|
191 |
+ // 권함 알림 메소드 |
|
170 | 192 |
private void permissionCheck(){ |
171 | 193 |
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.TIRAMISU) { |
172 | 194 |
permission = new PermissionManager(this, this); |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?