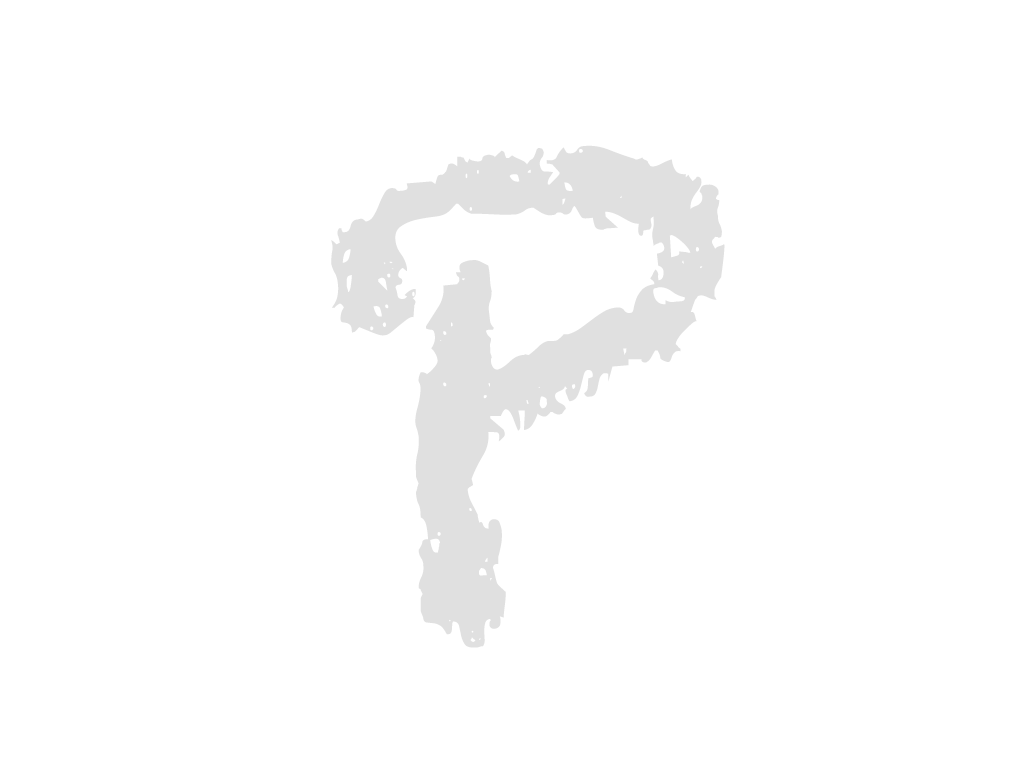
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import {interpolateTransformCss as interpolateTransform} from "d3-interpolate";
import {style} from "d3-selection";
import {set} from "./schedule.js";
import {tweenValue} from "./tween.js";
import interpolate from "./interpolate.js";
function styleNull(name, interpolate) {
var string00,
string10,
interpolate0;
return function() {
var string0 = style(this, name),
string1 = (this.style.removeProperty(name), style(this, name));
return string0 === string1 ? null
: string0 === string00 && string1 === string10 ? interpolate0
: interpolate0 = interpolate(string00 = string0, string10 = string1);
};
}
function styleRemove(name) {
return function() {
this.style.removeProperty(name);
};
}
function styleConstant(name, interpolate, value1) {
var string00,
string1 = value1 + "",
interpolate0;
return function() {
var string0 = style(this, name);
return string0 === string1 ? null
: string0 === string00 ? interpolate0
: interpolate0 = interpolate(string00 = string0, value1);
};
}
function styleFunction(name, interpolate, value) {
var string00,
string10,
interpolate0;
return function() {
var string0 = style(this, name),
value1 = value(this),
string1 = value1 + "";
if (value1 == null) string1 = value1 = (this.style.removeProperty(name), style(this, name));
return string0 === string1 ? null
: string0 === string00 && string1 === string10 ? interpolate0
: (string10 = string1, interpolate0 = interpolate(string00 = string0, value1));
};
}
function styleMaybeRemove(id, name) {
var on0, on1, listener0, key = "style." + name, event = "end." + key, remove;
return function() {
var schedule = set(this, id),
on = schedule.on,
listener = schedule.value[key] == null ? remove || (remove = styleRemove(name)) : undefined;
// If this node shared a dispatch with the previous node,
// just assign the updated shared dispatch and we’re done!
// Otherwise, copy-on-write.
if (on !== on0 || listener0 !== listener) (on1 = (on0 = on).copy()).on(event, listener0 = listener);
schedule.on = on1;
};
}
export default function(name, value, priority) {
var i = (name += "") === "transform" ? interpolateTransform : interpolate;
return value == null ? this
.styleTween(name, styleNull(name, i))
.on("end.style." + name, styleRemove(name))
: typeof value === "function" ? this
.styleTween(name, styleFunction(name, i, tweenValue(this, "style." + name, value)))
.each(styleMaybeRemove(this._id, name))
: this
.styleTween(name, styleConstant(name, i, value), priority)
.on("end.style." + name, null);
}