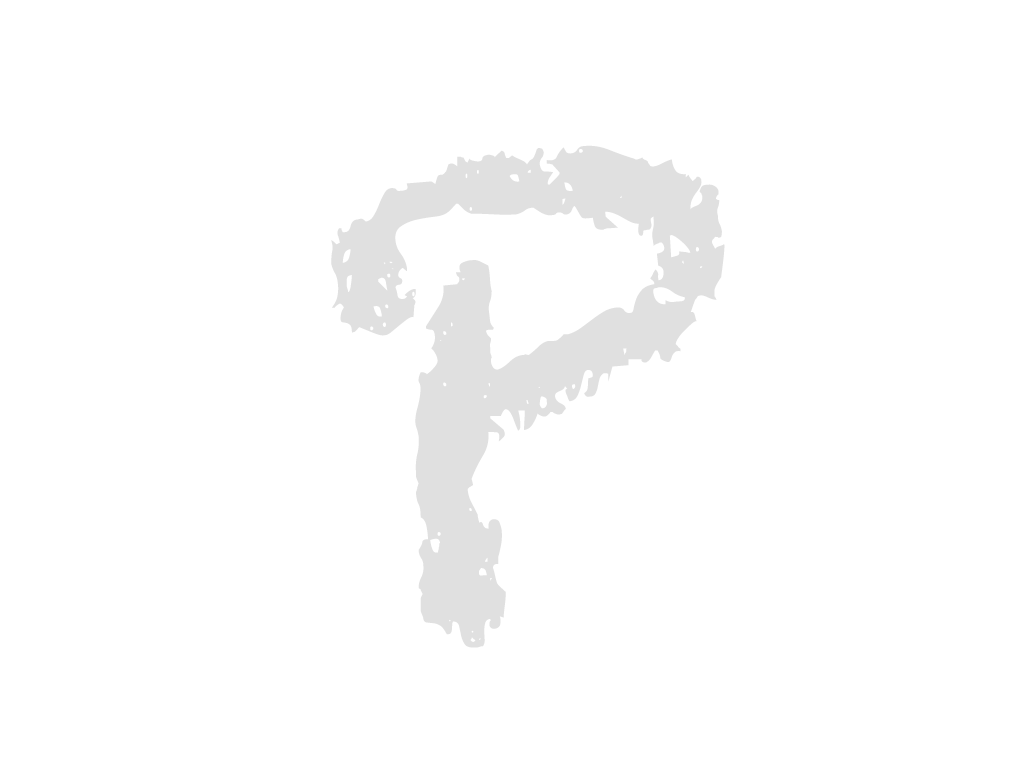
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import { createStore } from "vuex";
import createPersistedState from "vuex-persistedstate";
import { logOutProc } from "../../resources/api/logOut"
export default createStore({
plugins: [createPersistedState()],
state: {
authorization: null,
refresh: null,
mbrId: null,
mbrNm: null,
userType: "company",
menu: null,
menuList : null,
menuInfo: null,
pageNaviInfo : null,
currentMenu: null,
path: null,
authrtNm: null,
authrtTypeLvl: "9",
pdfid : null,
roles: [{authority: "ROLE_NONE"}],
pageAuth: null,
topScrollerFlag : true,
isLoading: false,
redirect: null,
routerFlag: false
},
getters: {
getAuthorization: function () {},
getRefresh: function () {},
getMbrNm: function () {},
getRoles: function () {},
},
mutations: {
setAuthorization(state, newValue) {
state.authorization = newValue;
},
setRefresh(state, newValue) {
state.refresh = newValue;
},
setMbrNm(state, newValue) {
state.mbrNm = newValue;
},
setMbrId(state, newValue) {
state.mbrId = newValue;
},
setAuthrtNm(state, newValue) {
state.authrtNm = newValue;
},
setAuthrtTypeLvl(state, newValue) {
state.authrtTypeLvl = newValue;
},
setRoles(state, newValue) {
state.roles = newValue;
},
setRoles(state, newValue) {
state.roles = newValue;
},
setUserType(state, newValue) {
state.userType = newValue;
},
setMenu(state, newValue) {
state.menu = newValue;
},
setMenuList(state, newValue) {
state.menuList = newValue;
},
setMenuInfo(state, newValue) {
state.menuInfo = newValue;
},
setPageNaviInfo(state, newValue) {
state.pageNaviInfo = newValue;
},
setCurrentMenu(state, newValue) {
state.currentMenu = newValue;
},
setTopScrollerFlag(state, newValue) {
state.topScrollerFlag = newValue;
},
setPath(state, newValue) {
state.path = newValue;
},
setStoreReset(state) {
state.authorization = null;
state.refresh = null;
state.mbrNm = null;
state.mbrId = null;
state.authrtTypeLvl = "9",
state.roles = [{authority: "ROLE_NONE"}];
state.menu = null;
state.currentMenu = null;
state.pageAuth = null;
},
setPageAuth(state, newValue) {
state.pageAuth = newValue;
},
setPdfId(state, newValue) {
state.pdfid = newValue;
},
setLoading(state,newValue){
state.isLoading = newValue;
},
setRedirect(state, newValue) {
state.redirect = newValue;
},
setRouterFlag(state, newValue) {
state.routerFlag = newValue;
},
},
actions: {
async logout({ commit }) {
const vm = this;
try {
const res = await logOutProc();
alert(res.data.message);
if (res.status == 200) {
commit("setStoreReset");
}
} catch(error) {
const errorData = error.response.data;
if (errorData.message != null && errorData.message != "") {
alert(error.response.data.message);
} else {
// alert("에러가 발생했습니다.\n관리자에게 문의해주세요.");
alert(vm.$getCmmnMessage('err005'));
}
}
},
setUserType({ commit }, userType) {
commit("setUserType", userType);
},
setMenu({ commit }, menu) {
commit("setMenu", menu);
},
setPath({ commit }, path) {
commit("setPath", path);
},
setPageAuth({ commit }, pageAuth) {
commit("setPageAuth", pageAuth);
},
setStoreReset({commit}) {
commit("setStoreReset");
},
setRedirect({commit}, redirect) {
commit("setRedirect", redirect)
}
},
});