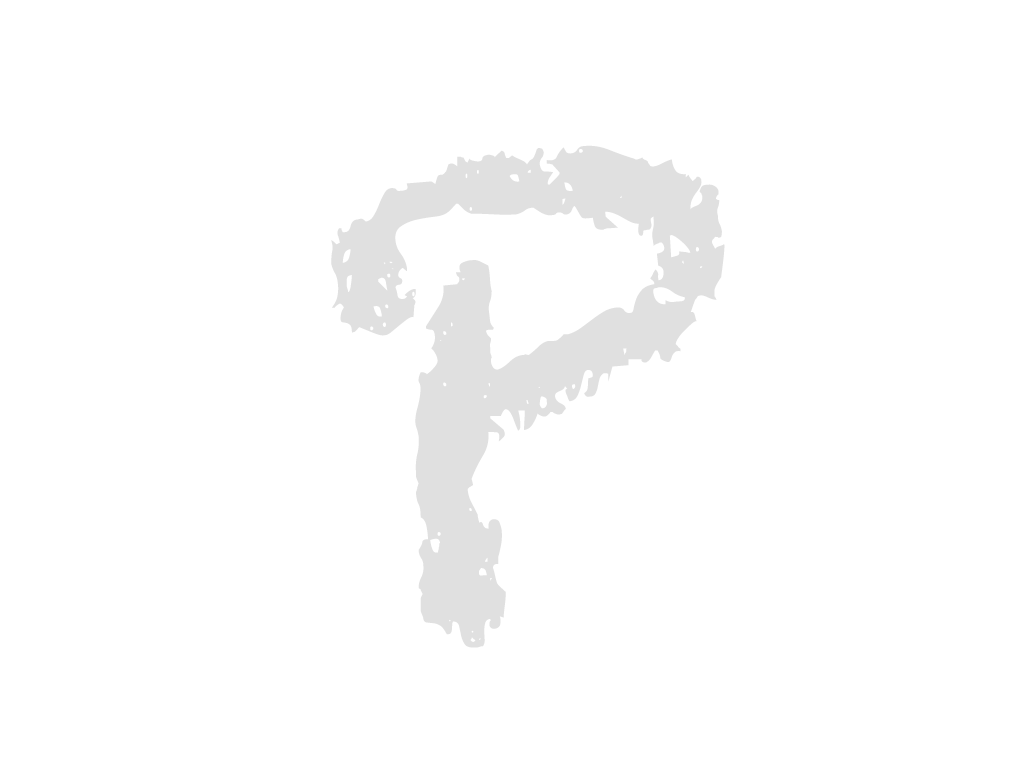
--- client/views/Login.vue
+++ client/views/Login.vue
... | ... | @@ -185,8 +185,13 @@ |
185 | 185 |
password: this.password, |
186 | 186 |
}, |
187 | 187 |
}).then(function (response) { |
188 |
+ |
|
188 | 189 |
const token = response.headers.get('Authorization'); |
190 |
+ const Refreshtoken = response.headers.get('RefreshToken'); |
|
191 |
+ |
|
189 | 192 |
store.dispatch('login', token); |
193 |
+ localStorage.setItem("Refreshtoken", Refreshtoken); |
|
194 |
+ |
|
190 | 195 |
vm.branchPage(); |
191 | 196 |
}).catch(function (error) { |
192 | 197 |
alert("아이디나 비밀번호가 잘못됐습니다 !"); |
... | ... | @@ -197,37 +202,49 @@ |
197 | 202 |
branchPage() { |
198 | 203 |
const vm = this; |
199 | 204 |
const token = localStorage.getItem('token'); |
200 |
- axios.post('/auth/validateToken.json', {}, { |
|
201 |
- headers: { |
|
202 |
- Authorization: token |
|
203 |
- } |
|
204 |
- }).then(response => { |
|
205 |
- if (response.data.status === 'success') { |
|
206 |
- const userInfo = response.data.userInfo; |
|
205 |
+ |
|
206 |
+ if (token) { |
|
207 |
+ // JWT 토큰을 '.'로 분리 |
|
208 |
+ const tokenParts = token.split('.'); |
|
209 |
+ if (tokenParts.length === 3) { |
|
210 |
+ const payload = tokenParts[1]; |
|
211 |
+ |
|
212 |
+ const base64 = payload.replace(/-/g, '+').replace(/_/g, '/'); |
|
213 |
+ |
|
214 |
+ // Base64 디코딩 후 UTF-8로 변환 |
|
215 |
+ const jsonPayload = decodeURIComponent( |
|
216 |
+ Array.prototype.map.call(atob(base64), c => { |
|
217 |
+ return '%' + ('00' + c.charCodeAt(0).toString(16)).slice(-2); |
|
218 |
+ }).join('') |
|
219 |
+ ); |
|
220 |
+ |
|
221 |
+ const userInfo = JSON.parse(jsonPayload); |
|
222 |
+ |
|
223 |
+ // Vuex 스토어에 사용자 정보 저장 |
|
207 | 224 |
store.commit('setToken', token); |
208 | 225 |
store.commit('setUser', userInfo.usid); |
209 |
- store.commit('setAuthcd', userInfo.author[0].authorCode); |
|
210 |
- const roles = userInfo.author.map(role => role.authorCode); |
|
226 |
+ store.commit('setUserNm', userInfo.userNm); |
|
227 |
+ store.commit('setAuthcd', userInfo.author[0].authority); |
|
228 |
+ const roles = userInfo.author.map(role => role.authority); |
|
211 | 229 |
|
212 |
- // 학생은 Main_t로 접근할 수 없음 |
|
230 |
+ console.log(userInfo); |
|
231 |
+ |
|
232 |
+ // 역할에 따라 페이지 이동 |
|
213 | 233 |
if (roles.includes("STUDENT")) { |
214 | 234 |
vm.goToPage('Dashboard'); |
215 |
- } |
|
216 |
- // 선생님은 Main으로 접근할 수 없음 |
|
217 |
- else if (roles.includes("TEACHER")) { |
|
235 |
+ } else if (roles.includes("TEACHER")) { |
|
218 | 236 |
vm.goToPage('Board'); |
219 |
- } |
|
220 |
- // 부모님은 Main으로 접근할 수 없음 |
|
221 |
- else if (roles.includes("PARENT")) { |
|
237 |
+ } else if (roles.includes("PARENT")) { |
|
222 | 238 |
vm.goToPage('Main_p'); |
223 |
- } |
|
224 |
- else if (roles.includes("ADMIN")) { |
|
239 |
+ } else if (roles.includes("ADMIN")) { |
|
225 | 240 |
vm.goToPage('Dashboard'); |
226 | 241 |
} |
242 |
+ } else { |
|
243 |
+ console.error('Invalid token structure'); |
|
227 | 244 |
} |
228 |
- }).catch(error => { |
|
229 |
- console.error(error); |
|
230 |
- }); |
|
245 |
+ } else { |
|
246 |
+ console.error('No token found'); |
|
247 |
+ } |
|
231 | 248 |
}, |
232 | 249 |
closeModal() { |
233 | 250 |
this.showModal = false; |
--- client/views/pages/AppStore.js
+++ client/views/pages/AppStore.js
... | ... | @@ -9,6 +9,7 @@ |
9 | 9 |
schdlId: null, |
10 | 10 |
bookId: null, |
11 | 11 |
unitId: null, |
12 |
+ unitNm: null, |
|
12 | 13 |
LearningId: null, |
13 | 14 |
currentLearningIds: [], |
14 | 15 |
prblmTypeId: null, |
... | ... | @@ -23,6 +24,7 @@ |
23 | 24 |
getUserInfo(state) { |
24 | 25 |
return { |
25 | 26 |
userId: state.userId, |
27 |
+ userNm: state.userNm, |
|
26 | 28 |
authcd: state.authcd, |
27 | 29 |
}; |
28 | 30 |
}, |
... | ... | @@ -58,6 +60,7 @@ |
58 | 60 |
clearToken(state) { |
59 | 61 |
state.token = null; |
60 | 62 |
state.userId = null; |
63 |
+ state.userNm = null; |
|
61 | 64 |
state.authcd = null; |
62 | 65 |
state.stdId = null; |
63 | 66 |
state.schdlId = null; |
... | ... | @@ -68,6 +71,9 @@ |
68 | 71 |
setUser(state, userId) { |
69 | 72 |
state.userId = userId; |
70 | 73 |
}, |
74 |
+ setUserNm(state, userNm) { |
|
75 |
+ state.userNm = userNm; |
|
76 |
+ }, |
|
71 | 77 |
setAuthcd(state, authcd) { |
72 | 78 |
state.authcd = authcd; |
73 | 79 |
}, |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?