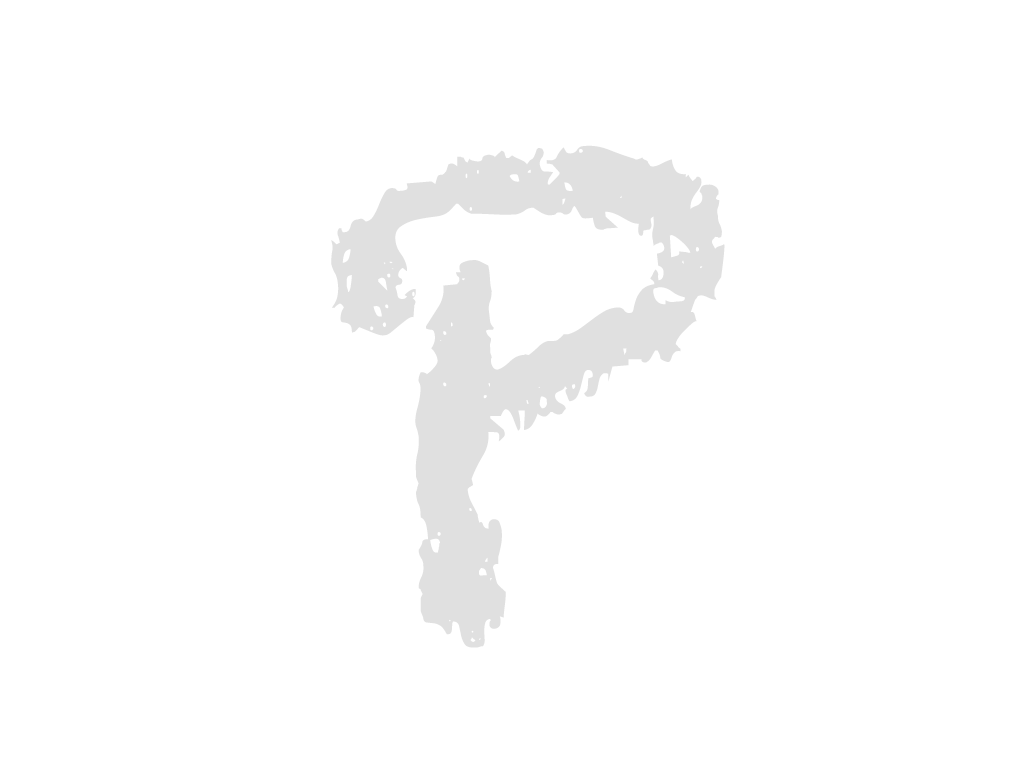
--- client/views/pages/main/Chapter/Chapter2_5.vue
+++ client/views/pages/main/Chapter/Chapter2_5.vue
... | ... | @@ -17,7 +17,7 @@ |
17 | 17 |
<button class="completeBtn" @click="complete"> 학습 종료 </button> |
18 | 18 |
</div> |
19 | 19 |
<div class="flex justify-between align-center"> |
20 |
- <div class="pre-btn" @click="goToPage('Chapter2_7')"> |
|
20 |
+ <div class="pre-btn" @click="previousProblem()"> |
|
21 | 21 |
<img src="../../../../resources/img/left.png" alt="" /> |
22 | 22 |
</div> |
23 | 23 |
<div class="content title-box"> |
... | ... | @@ -38,7 +38,7 @@ |
38 | 38 |
<!-- <p class="title1 mb40" style="color: #464749;">The sun rises in the east and sets in the west. It is very hot during summer.</p> |
39 | 39 |
<img src="../../../../resources/img/img65_37s.png" alt=""> --> |
40 | 40 |
|
41 |
- <img class="exampleImg" :src="imgSrc" alt="example img" /> |
|
41 |
+ <img class="exampleImg" :src="imgUrl" reloadable="true" alt="example img" /> |
|
42 | 42 |
|
43 | 43 |
<!-- 정답 칸 --> |
44 | 44 |
<div class="dropGroup flex align-center justify-center mt30"> |
... | ... | @@ -70,7 +70,7 @@ |
70 | 70 |
</div> |
71 | 71 |
</div> |
72 | 72 |
</div> |
73 |
- <div class="next-btn" @click="goToPage('Chapter2_6')"> |
|
73 |
+ <div class="next-btn" @click="nextProblem()"> |
|
74 | 74 |
<img src="../../../../resources/img/right.png" alt="" /> |
75 | 75 |
</div> |
76 | 76 |
</div> |
... | ... | @@ -78,25 +78,27 @@ |
78 | 78 |
</template> |
79 | 79 |
|
80 | 80 |
<script> |
81 |
+import axios from "axios"; |
|
81 | 82 |
export default { |
82 | 83 |
data() { |
83 | 84 |
return { |
84 | 85 |
imgSrc: "client/resources/img/jumpingRabbit.gif", |
85 |
- example: "a rabbit is/?/a guitar", |
|
86 |
+ example: "", |
|
86 | 87 |
beforeQuestion: "", |
87 | 88 |
afterQuestion: "", |
88 |
- choice: "ygnpali", |
|
89 |
- answer: "playing", |
|
89 |
+ choice: "", |
|
90 |
+ answer: "", |
|
90 | 91 |
answerLength: 0, // 초기화 시 0으로 설정 |
91 | 92 |
|
92 | 93 |
userAnswer: [], // 초기화 시 빈 배열로 설정 |
93 | 94 |
draggedChar: null, // 드래그한 문자를 임시로 저장 |
95 |
+ |
|
96 |
+ prblm_id: [], |
|
97 |
+ unit_id: null, |
|
98 |
+ dataList: [], |
|
94 | 99 |
}; |
95 | 100 |
}, |
96 | 101 |
computed: { |
97 |
- choiceCharacters() { |
|
98 |
- return this.choice.split(""); |
|
99 |
- }, |
|
100 | 102 |
}, |
101 | 103 |
methods: { |
102 | 104 |
complete() { |
... | ... | @@ -122,6 +124,7 @@ |
122 | 124 |
if (this.userAnswer[index] === "") { |
123 | 125 |
this.userAnswer.splice(index, 1, this.draggedChar); |
124 | 126 |
const charIndex = this.choiceCharacters.indexOf(this.draggedChar); |
127 |
+ console.log(charIndex) |
|
125 | 128 |
if (charIndex > -1) { |
126 | 129 |
this.choiceCharacters.splice(charIndex, 1); // 드래그한 문자를 choice에서 제거 |
127 | 130 |
} |
... | ... | @@ -148,10 +151,155 @@ |
148 | 151 |
returnPage() { |
149 | 152 |
window.location.reload(); |
150 | 153 |
}, |
154 |
+ async getProblem() { |
|
155 |
+ const prblmId = this.currentLearningId.prblm_id; |
|
156 |
+ |
|
157 |
+ try { |
|
158 |
+ const res = await axios.post("problem/problemInfo.json", { |
|
159 |
+ prblmId: prblmId, |
|
160 |
+ }, { |
|
161 |
+ headers: { |
|
162 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
163 |
+ }, |
|
164 |
+ }); |
|
165 |
+ |
|
166 |
+ console.log("problem - response : ", res.data); |
|
167 |
+ this.dataList = res.data.problem; |
|
168 |
+ this.problemDetail = res.data.problemDetail[0]; |
|
169 |
+ this.example = this.dataList.prblmExpln; |
|
170 |
+ this.answer = this.problemDetail.prblmDtlExpln; |
|
171 |
+ this.choice = this.answer ? this.shuffleString(this.answer) : ''; |
|
172 |
+ this.splitExample(); |
|
173 |
+ this.initializeUserAnswer(); |
|
174 |
+ this.choiceCharacters = this.choice.split(""); |
|
175 |
+ |
|
176 |
+ const fileInfo = await this.findFile(this.dataList.fileMngId); |
|
177 |
+ if (fileInfo) { |
|
178 |
+ this.imgUrl = "http://localhost:9080/" + fileInfo.fileRpath; |
|
179 |
+ console.log(this.imgUrl); |
|
180 |
+ } else { |
|
181 |
+ console.warn("No file found for the given fileMngId."); |
|
182 |
+ } |
|
183 |
+ } catch (error) { |
|
184 |
+ console.log("problem - error : ", error); |
|
185 |
+ } |
|
186 |
+ }, |
|
187 |
+ shuffleString(string) { |
|
188 |
+ const array = string.split(''); |
|
189 |
+ for (let i = array.length - 1; i > 0; i--) { |
|
190 |
+ const j = Math.floor(Math.random() * (i + 1)); |
|
191 |
+ [array[i], array[j]] = [array[j], array[i]]; |
|
192 |
+ } |
|
193 |
+ return array.join(''); |
|
194 |
+ }, |
|
195 |
+ async findFile(file_mng_id) { |
|
196 |
+ try { |
|
197 |
+ const res = await axios.post("/file/find.json", { |
|
198 |
+ file_mng_id: file_mng_id, |
|
199 |
+ }, { |
|
200 |
+ headers: { |
|
201 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
202 |
+ }, |
|
203 |
+ }); |
|
204 |
+ return res.data.list[0]; |
|
205 |
+ } catch (error) { |
|
206 |
+ console.log("result - error : ", error); |
|
207 |
+ return null; |
|
208 |
+ } |
|
209 |
+ }, |
|
210 |
+ nextProblem() { |
|
211 |
+ if (this.currentProblemIndex < this.$store.state.currentLearningIds.length - 1) { |
|
212 |
+ this.$store.dispatch('goToNextProblem'); |
|
213 |
+ this.handleProblemDetail(this.currentLearningId); |
|
214 |
+ this.goToPage(this.problemType); |
|
215 |
+ } else { |
|
216 |
+ // 마지막 문제면 이동 |
|
217 |
+ this.goToPage("Chapter4") |
|
218 |
+ } |
|
219 |
+ }, |
|
220 |
+ previousProblem() { |
|
221 |
+ if (this.currentProblemIndex > 0) { |
|
222 |
+ this.$store.dispatch('goToPreviousProblem'); |
|
223 |
+ this.handleProblemDetail(this.currentLearningId); |
|
224 |
+ this.goToPage(this.problemType); |
|
225 |
+ } |
|
226 |
+ }, |
|
227 |
+ |
|
228 |
+ handleProblemDetail(item) { |
|
229 |
+ if (item.prblm_type_id === "prblm_type_001") { |
|
230 |
+ this.problemType = "Chapter3"; |
|
231 |
+ } else if (item.prblm_type_id === "prblm_type_002") { |
|
232 |
+ this.problemType = "Chapter3_1"; |
|
233 |
+ } else if (item.prblm_type_id === "prblm_type_003") { |
|
234 |
+ this.problemType = "Chapter3_2"; |
|
235 |
+ } else if (item.prblm_type_id === "prblm_type_004") { |
|
236 |
+ this.problemType = "Chapter3_3"; |
|
237 |
+ } else if (item.prblm_type_id === "prblm_type_005") { |
|
238 |
+ this.problemType = "Chapter3_3_1"; |
|
239 |
+ } else if (item.prblm_type_id === "prblm_type_006") { |
|
240 |
+ this.problemType = "Chapter3_4"; |
|
241 |
+ } else if (item.prblm_type_id === "prblm_type_007") { |
|
242 |
+ this.problemType = "Chapter3_5"; |
|
243 |
+ } else if (item.prblm_type_id === "prblm_type_008") { |
|
244 |
+ this.problemType = "Chapter3_6"; |
|
245 |
+ } else if (item.prblm_type_id === "prblm_type_009") { |
|
246 |
+ this.problemType = "Chapter3_7"; |
|
247 |
+ } else if (item.prblm_type_id === "prblm_type_010") { |
|
248 |
+ this.problemType = "Chapter3_8"; |
|
249 |
+ } else if (item.prblm_type_id === "prblm_type_011") { |
|
250 |
+ this.problemType = "Chapter3_9"; |
|
251 |
+ } else if (item.prblm_type_id === "prblm_type_012") { |
|
252 |
+ this.problemType = "Chapter3_10"; |
|
253 |
+ } else if (item.prblm_type_id === "prblm_type_013") { |
|
254 |
+ this.problemType = "Chapter3_11"; |
|
255 |
+ } else if (item.prblm_type_id === "prblm_type_014") { |
|
256 |
+ this.problemType = "Chapter3_12"; |
|
257 |
+ } else if (item.prblm_type_id === "prblm_type_015") { |
|
258 |
+ this.problemType = "Chapter3_13"; |
|
259 |
+ } else if (item.prblm_type_id === "prblm_type_016") { |
|
260 |
+ this.problemType = "Chapter3_14"; |
|
261 |
+ } else if (item.prblm_type_id === "prblm_type_017") { |
|
262 |
+ this.problemType = "Chapter3_15"; |
|
263 |
+ } else if (item.prblm_type_id === "prblm_type_018") { |
|
264 |
+ this.problemType = "Chapter2_8"; |
|
265 |
+ } else if (item.prblm_type_id === "prblm_type_019") { |
|
266 |
+ this.problemType = "Chapter2_7"; |
|
267 |
+ } else if (item.prblm_type_id === "prblm_type_020") { |
|
268 |
+ this.problemType = "Chapter2_5"; |
|
269 |
+ } else if (item.prblm_type_id === "prblm_type_021") { |
|
270 |
+ this.problemType = "Chapter2_6"; |
|
271 |
+ } else if (item.prblm_type_id === "prblm_type_022") { |
|
272 |
+ this.problemType = "Chapter2_10"; |
|
273 |
+ } else if (item.prblm_type_id === "prblm_type_023") { |
|
274 |
+ this.problemType = "Chapter2_11"; |
|
275 |
+ } else if (item.prblm_type_id === "prblm_type_024") { |
|
276 |
+ this.problemType = "Chapter2_13"; |
|
277 |
+ } |
|
278 |
+ }, |
|
279 |
+ }, |
|
280 |
+ computed: { |
|
281 |
+ currentLearningId() { |
|
282 |
+ return this.$store.getters.currentLearningId; |
|
283 |
+ }, |
|
284 |
+ currentLabel() { |
|
285 |
+ return this.$store.getters.currentLabel; |
|
286 |
+ }, |
|
287 |
+ currentProblemIndex() { |
|
288 |
+ return this.$store.getters.currentProblemIndex; |
|
289 |
+ }, |
|
290 |
+ isPreviousButtonDisabled() { |
|
291 |
+ return this.currentProblemIndex === 0; |
|
292 |
+ } |
|
293 |
+ }, |
|
294 |
+ created() { |
|
295 |
+ console.log('Current Learning ID:', this.currentLearningId); |
|
296 |
+ console.log('Current Label:', this.currentLabel); |
|
297 |
+ console.log('Current Problem Index:', this.currentProblemIndex); |
|
298 |
+ |
|
299 |
+ // Fetch or process the current problem based on `currentLearningId` |
|
151 | 300 |
}, |
152 | 301 |
mounted() { |
153 |
- this.splitExample(); |
|
154 |
- this.initializeUserAnswer(); // 컴포넌트가 마운트될 때 userAnswer 초기화 |
|
302 |
+ this.getProblem() |
|
155 | 303 |
}, |
156 | 304 |
}; |
157 | 305 |
</script> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?