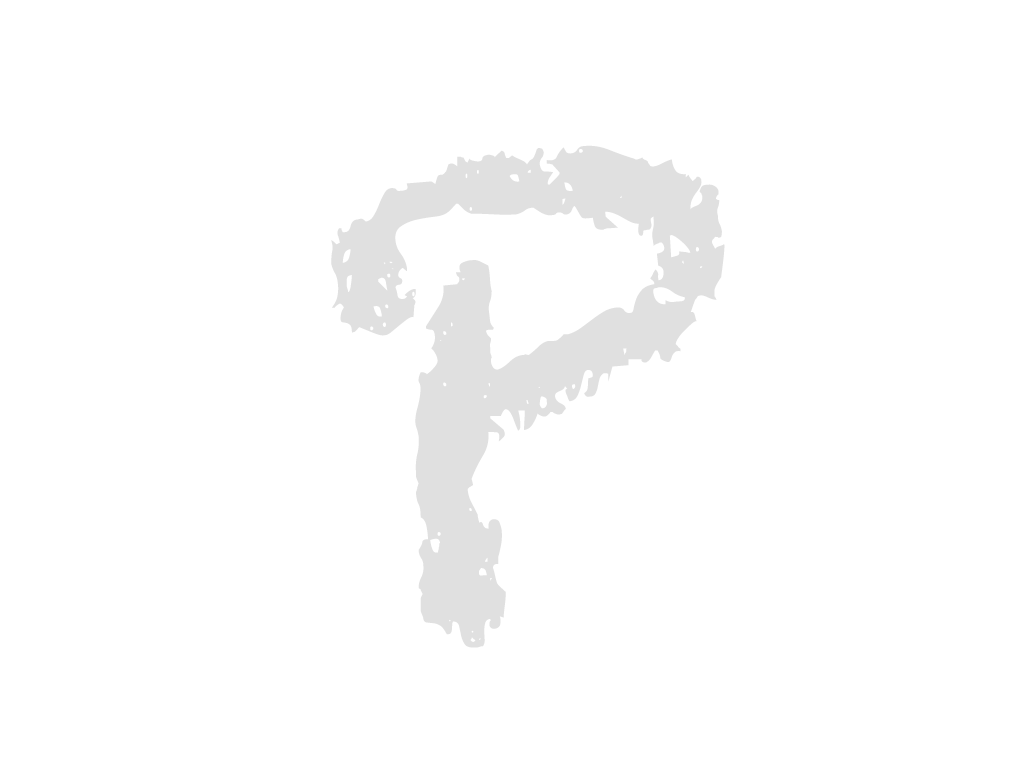
--- client/views/pages/AppRouter.js
+++ client/views/pages/AppRouter.js
... | ... | @@ -81,6 +81,7 @@ |
81 | 81 |
import ClassDetail from "./teacher/ClassDetail.vue"; |
82 | 82 |
import TextList from "./teacher/TextList.vue"; |
83 | 83 |
import TextInsert from "./teacher/TextInsert.vue"; |
84 |
+import TextDetail from "./teacher/TextDetail.vue"; |
|
84 | 85 |
import QuestionList from "./teacher/QuestionList.vue"; |
85 | 86 |
import QuestionInsert from "./teacher/QuestionInsert.vue"; |
86 | 87 |
import VocaList from "./teacher/VocaList.vue"; |
... | ... | @@ -199,6 +200,8 @@ |
199 | 200 |
{ path: '/C_ExamInsert.page', name: 'C_ExamInsert', component: C_ExamInsert }, |
200 | 201 |
|
201 | 202 |
{ path: '/RoadMap.page', name: 'RoadMap', component: RoadMap }, |
203 |
+ |
|
204 |
+ { path: '/TextDetail.page', name: 'TextDetail', component: TextDetail }, |
|
202 | 205 |
], |
203 | 206 |
}, |
204 | 207 |
]; |
+++ client/views/pages/teacher/TextDetail.vue
... | ... | @@ -0,0 +1,167 @@ |
1 | +<template> | |
2 | + <div class="title-box flex justify-between mb40"> | |
3 | + <p class="title">지문 등록</p> | |
4 | + </div> | |
5 | + <div class="board-wrap"> | |
6 | + <div class="flex align-center mb20"> | |
7 | + <label for="" class="title2">제목</label> | |
8 | + <div v-if="isEditing"> | |
9 | + <input type="text" v-model="newData.textTtl" class="data-wrap"> | |
10 | + </div> | |
11 | + <div v-else> | |
12 | + <p class="data-wrap">{{ post.text_ttl }}</p> | |
13 | + </div> | |
14 | + </div> | |
15 | + <div class="flex align-center mb20"> | |
16 | + <label for="" class="title2">URL</label> | |
17 | + <div v-if="isEditing"> | |
18 | + <input type="text" v-model="newData.textUrl" class="data-wrap"> | |
19 | + </div> | |
20 | + <div v-else> | |
21 | + <p class="data-wrap">{{ post.text_url }}</p> | |
22 | + </div> | |
23 | + </div> | |
24 | + <div class="flex align-center"> | |
25 | + <label class="title2">형식</label> | |
26 | + <label class="title2"> | |
27 | + <input type="radio" v-model="radio" value="1" class="data-wrap" :disabled="!isEditing"> 일반 | |
28 | + </label> | |
29 | + <label class="title2"> | |
30 | + <input type="radio" v-model="radio" value="2" class="data-wrap" :disabled="!isEditing"> 대화 | |
31 | + </label> | |
32 | + <label class="title2"> | |
33 | + <input type="radio" v-model="radio" value="3" class="data-wrap" :disabled="!isEditing"> 책 리스닝 | |
34 | + </label> | |
35 | + </div> | |
36 | + <hr> | |
37 | + <div class="flex align-center"> | |
38 | + <label for="" class="title2">스크립트</label> | |
39 | + <div v-if="isEditing"> | |
40 | + <textarea v-model="newData.textCnt" class="data-wrap"></textarea> | |
41 | + </div> | |
42 | + <div v-else> | |
43 | + <p class="data-wrap">{{ post.text_cnt }}</p> | |
44 | + </div> | |
45 | + </div> | |
46 | + </div> | |
47 | + <div class="flex justify-between mt50"> | |
48 | + <button type="button" title="목록으로" class="new-btn" @click="goToPage('TextList')"> | |
49 | + 목록 | |
50 | + </button> | |
51 | + <div class="flex"> | |
52 | + <button type="button" title="수정" class="new-btn mr10" @click="toggleEdit"> | |
53 | + {{ isEditing ? '저장' : '수정' }} | |
54 | + </button> | |
55 | + <button type="button" title="삭제" class="new-btn" @click="deletePost"> | |
56 | + 삭제 | |
57 | + </button> | |
58 | + </div> | |
59 | + </div> | |
60 | +</template> | |
61 | + | |
62 | +<script> | |
63 | +import axios from "axios"; | |
64 | +import SvgIcon from '@jamescoyle/vue-icon'; | |
65 | +import { mdiMagnify } from '@mdi/js'; | |
66 | + | |
67 | +export default { | |
68 | + data() { | |
69 | + return { | |
70 | + mdiMagnify: mdiMagnify, | |
71 | + post: {}, | |
72 | + newData: { | |
73 | + "textId": "", | |
74 | + "textTtl": "", | |
75 | + "textCnt": "", | |
76 | + "textUrl": "", | |
77 | + "textTypeId": "" | |
78 | + }, | |
79 | + radio: null, | |
80 | + isEditing: false // Add this property to toggle edit mode | |
81 | + }; | |
82 | + }, | |
83 | + computed: { | |
84 | + textId() { | |
85 | + return this.$route.query.textId; | |
86 | + } | |
87 | + }, | |
88 | + methods: { | |
89 | + goToPage(page) { | |
90 | + this.$router.push({ name: page }); | |
91 | + }, | |
92 | + fetchPostDetail() { | |
93 | + const textId = this.$route.query.textId; | |
94 | + axios.post('/text/selectOneText.json', { textId }, { | |
95 | + headers: { | |
96 | + "Content-Type": "application/json; charset=UTF-8", | |
97 | + } | |
98 | + }) | |
99 | + .then(response => { | |
100 | + if (response.data && response.data[0]) { | |
101 | + this.post = response.data[0]; | |
102 | + this.radio = this.post.text_type_id; | |
103 | + } else { | |
104 | + this.error = "Failed to fetch post details."; | |
105 | + } | |
106 | + }) | |
107 | + .catch(error => { | |
108 | + console.error("Error fetching post detail:", error); | |
109 | + this.error = "Failed to fetch post details."; | |
110 | + }); | |
111 | + }, | |
112 | + dataInsert() { | |
113 | + this.newData.textId=this.textId; | |
114 | + axios.post("/text/textUpdate.json", this.newData, { | |
115 | + headers: { | |
116 | + "Content-Type": "application/json; charset=UTF-8", | |
117 | + } | |
118 | + }) | |
119 | + .then(response => { | |
120 | + alert(response.data.message); | |
121 | + this.fetchPostDetail(); | |
122 | + }) | |
123 | + .catch(error => { | |
124 | + console.log("dataInsert - error : ", error.response.data); | |
125 | + alert("게시글 등록에 오류가 발생했습니다."); | |
126 | + }); | |
127 | + }, | |
128 | + toggleEdit() { | |
129 | + if (this.isEditing) { | |
130 | + // Save changes if in edit mode | |
131 | + this.newData.textTypeId = this.radio; | |
132 | + this.dataInsert(); | |
133 | + this.isEditing = false; | |
134 | + } else { | |
135 | + // Enter edit mode | |
136 | + this.isEditing = true; | |
137 | + } | |
138 | + }, | |
139 | + deletePost() { | |
140 | + axios.post("/text/textDelete.json", { textId: this.textId }, { | |
141 | + headers: { | |
142 | + "Content-Type": "application/json; charset=UTF-8", | |
143 | + } | |
144 | + }) | |
145 | + .then(response => { | |
146 | + alert(response.data.message); | |
147 | + this.goToPage('TextList'); | |
148 | + }) | |
149 | + .catch(error => { | |
150 | + console.error("Error deleting post:", error); | |
151 | + alert("게시글 삭제에 오류가 발생했습니다."); | |
152 | + }); | |
153 | + } | |
154 | + }, | |
155 | + mounted() { | |
156 | + this.fetchPostDetail(); | |
157 | + console.log('Main2 mounted'); | |
158 | + }, | |
159 | + components: { | |
160 | + SvgIcon | |
161 | + } | |
162 | +}; | |
163 | +</script> | |
164 | + | |
165 | +<style scoped> | |
166 | +/* Add any necessary styles here */ | |
167 | +</style> |
--- client/views/pages/teacher/TextInsert.vue
+++ client/views/pages/teacher/TextInsert.vue
... | ... | @@ -1,63 +1,143 @@ |
1 |
+<!-- userid, bookid, unitid 추가해야함. filemng는? 모르겟음 --> |
|
1 | 2 |
<template> |
2 | 3 |
<div class="title-box flex justify-between mb40"> |
3 | 4 |
<p class="title">지문 등록</p> |
4 | 5 |
</div> |
5 |
- <div class="board-wrap"> |
|
6 |
- <div class="flex align-center mb20"> |
|
7 |
- <label for="" class="title2">제목</label> |
|
8 |
- <input type="text" class="data-wrap"> |
|
9 |
- </div> |
|
10 |
- <div class="flex align-center"> |
|
11 |
- <label for="" class="title2">URL</label> |
|
12 |
- <input type="text" class="data-wrap"> |
|
13 |
- </div> |
|
14 |
- <hr> |
|
15 |
- <div class="flex align-center"> |
|
16 |
- <label for="" class="title2">스크립트</label> |
|
17 |
- <textarea name="" id="" class="data-wrap"></textarea> |
|
18 |
- </div> |
|
6 |
+ <div class="board-wrap"> |
|
7 |
+ <div class="flex align-center mb20"> |
|
8 |
+ <label for="" class="title2">제목</label> |
|
9 |
+ <input type="text" class="data-wrap" v-model="newData.textTtl"> |
|
19 | 10 |
</div> |
20 |
- <div class="flex justify-between mt50"> |
|
21 |
- <button type="button" title="글쓰기" class="new-btn" @click="goToPage('TextList')"> |
|
22 |
- 목록 |
|
23 |
- </button> |
|
24 |
- <div class="flex"> |
|
25 |
- <button type="button" title="글쓰기" class="new-btn mr10" > |
|
26 |
- 수정 |
|
27 |
- </button> |
|
28 |
- <button type="button" title="글쓰기" class="new-btn" > |
|
29 |
- 삭제 |
|
30 |
- </button> |
|
31 |
- </div> |
|
11 |
+ <div class="flex align-center mb20"> |
|
12 |
+ <label for="" class="title2">URL</label> |
|
13 |
+ <input type="text" class="data-wrap" v-model="newData.textUrl"> |
|
32 | 14 |
</div> |
15 |
+ <div class="flex align-center"> |
|
16 |
+ <label class="title2">형식</label> |
|
17 |
+ <label class="title2"> |
|
18 |
+ <input type="radio" v-model="newData.textTypeId" value="1" class="data-wrap"> 일반 |
|
19 |
+ </label> |
|
20 |
+ <label class="title2"> |
|
21 |
+ <input type="radio" v-model="newData.textTypeId" value="2" class="data-wrap"> 대화 |
|
22 |
+ </label> |
|
23 |
+ <label class="title2"> |
|
24 |
+ <input type="radio" v-model="newData.textTypeId" value="3" class="data-wrap"> 책 리스닝 |
|
25 |
+ </label> |
|
26 |
+ </div> |
|
27 |
+ <hr> |
|
28 |
+ <div class="flex align-center"> |
|
29 |
+ <label for="" class="title2">스크립트</label> |
|
30 |
+ <textarea name="" id="" class="data-wrap" v-model="newData.textCnt"></textarea> |
|
31 |
+ </div> |
|
32 |
+ </div> |
|
33 |
+ <div class="flex justify-between mt50"> |
|
34 |
+ <button type="button" title="글쓰기" class="new-btn" @click="goToPage('TextList')"> |
|
35 |
+ 목록 |
|
36 |
+ </button> |
|
37 |
+ <div class="flex"> |
|
38 |
+ <button type="button" title="글쓰기" class="new-btn mr10" @click="handleButtonAction"> |
|
39 |
+ 작성 |
|
40 |
+ </button> |
|
41 |
+ </div> |
|
42 |
+ </div> |
|
33 | 43 |
</template> |
34 | 44 |
|
35 | 45 |
<script> |
46 |
+import axios from "axios"; |
|
36 | 47 |
import SvgIcon from '@jamescoyle/vue-icon'; |
37 |
-import { mdiMagnify} from '@mdi/js'; |
|
48 |
+import { mdiMagnify } from '@mdi/js'; |
|
38 | 49 |
|
39 | 50 |
|
40 | 51 |
export default { |
41 |
- data () { |
|
52 |
+ computed: { |
|
53 |
+ textId() { |
|
54 |
+ return this.$route.query.textId; |
|
55 |
+ } |
|
56 |
+ }, |
|
57 |
+ data() { |
|
42 | 58 |
return { |
43 | 59 |
mdiMagnify: mdiMagnify, |
60 |
+ post: null, |
|
61 |
+ newData: { |
|
62 |
+ textId: "", |
|
63 |
+ textTtl: "", |
|
64 |
+ textCnt: "", |
|
65 |
+ textUrl: "", |
|
66 |
+ textTypeId: "" |
|
67 |
+ }, |
|
44 | 68 |
} |
45 | 69 |
}, |
46 | 70 |
methods: { |
47 | 71 |
goToPage(page) { |
48 |
- this.$router.push({ name: page }); |
|
49 |
- }, |
|
72 |
+ this.$router.push({ name: page }); |
|
73 |
+ }, fetchPostDetail() { |
|
74 |
+ const textId=this.$route.query.textId |
|
75 |
+ axios({ |
|
76 |
+ url: `/text/selectOneText.json`, |
|
77 |
+ method: "post", |
|
78 |
+ headers: { |
|
79 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
80 |
+ }, |
|
81 |
+ data: { "textId": textId } |
|
82 |
+ }) |
|
83 |
+ .then(response => { |
|
84 |
+ if (response.data && response.data[0]) { |
|
85 |
+ this.post = response.data[0]; |
|
86 |
+ this.editTitle = this.post.title; |
|
87 |
+ this.editContent = this.post.content; |
|
88 |
+ console.log(this.post); |
|
89 |
+ } else { |
|
90 |
+ this.error = "Failed to fetch post details."; |
|
91 |
+ } |
|
92 |
+ }) |
|
93 |
+ .catch(error => { |
|
94 |
+ console.error("Error fetching post detail:", error); |
|
95 |
+ this.error = "Failed to fetch post details."; |
|
96 |
+ }); |
|
97 |
+ }, dataInsert() { |
|
98 |
+ const vm = this; |
|
99 |
+ axios({ |
|
100 |
+ url: "/text/insertText.json", |
|
101 |
+ method: "post", |
|
102 |
+ headers: { |
|
103 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
104 |
+ }, |
|
105 |
+ data: vm.newData, |
|
106 |
+ }) |
|
107 |
+ .then(response => { |
|
108 |
+ alert(response.data.message); |
|
109 |
+ this.goToPage('TextList') |
|
110 |
+ }) |
|
111 |
+ .catch(error => { |
|
112 |
+ console.log("dataInsert - error : ", error.response.data); |
|
113 |
+ alert("게시글 등록에 오류가 발생했습니다."); |
|
114 |
+ }); |
|
115 |
+ }, |
|
116 |
+ handleButtonAction() { |
|
117 |
+ if (!this.newData.textTtl) { |
|
118 |
+ alert("제목을 입력해 주세요."); |
|
119 |
+ return; |
|
120 |
+ } |
|
121 |
+ |
|
122 |
+ if (!this.newData.textCnt) { |
|
123 |
+ alert("내용을 입력해 주세요."); |
|
124 |
+ return; |
|
125 |
+ } |
|
126 |
+ |
|
127 |
+ this.dataInsert(); |
|
128 |
+ } |
|
50 | 129 |
}, |
51 | 130 |
watch: { |
52 | 131 |
|
53 | 132 |
}, |
54 | 133 |
computed: { |
55 |
- |
|
134 |
+ |
|
56 | 135 |
}, |
57 |
- components:{ |
|
136 |
+ components: { |
|
58 | 137 |
SvgIcon |
59 | 138 |
}, |
60 | 139 |
mounted() { |
140 |
+ this.fetchPostDetail(); |
|
61 | 141 |
console.log('Main2 mounted'); |
62 | 142 |
} |
63 | 143 |
} |
--- client/views/pages/teacher/TextList.vue
+++ client/views/pages/teacher/TextList.vue
... | ... | @@ -6,64 +6,88 @@ |
6 | 6 |
</select> |
7 | 7 |
</div> |
8 | 8 |
<div class="search-wrap flex justify-end mb20"> |
9 |
- <select name="" id="" class="mr10 data-wrap"> |
|
10 |
- <option value="">전체</option> |
|
11 |
- </select> |
|
12 |
- <input type="text" placeholder="검색하세요."> |
|
13 |
- <button type="button" title="위원회 검색"> |
|
14 |
- <img src="../../../resources/img/look_t.png" alt=""> |
|
15 |
- </button> |
|
16 |
- </div> |
|
17 |
- <div class="table-wrap"> |
|
18 |
- <table> |
|
19 |
- <thead> |
|
9 |
+ <select name="" id="" class="mr10 data-wrap" style="width: 15rem;" v-model="option"> |
|
10 |
+ <option value="" disabled>검색유형</option> |
|
11 |
+ <option v-for="g in optionList" :key="g.value" :value="g.value">{{ g.text }}</option> |
|
12 |
+ </select> |
|
13 |
+ <input type="text" placeholder="검색하세요." v-model="keyword"> |
|
14 |
+ <button type="button" title="위원회 검색" @click="search"> |
|
15 |
+ <img src="../../../resources/img/look_t.png" alt=""> |
|
16 |
+ </button> |
|
17 |
+ </div> |
|
18 |
+ <div class="table-wrap"> |
|
19 |
+ <table> |
|
20 |
+ <thead> |
|
21 |
+ <tr> |
|
20 | 22 |
<td>No.</td> |
21 | 23 |
<td>제목</td> |
22 | 24 |
<td>내용</td> |
23 | 25 |
<td>작성자</td> |
24 | 26 |
<td>등록일</td> |
25 |
- </thead> |
|
26 |
- <tbody> |
|
27 |
- <tr @click="goToPage('TextInsert')"> |
|
28 |
- <td></td> |
|
29 |
- <td></td> |
|
30 |
- <td></td> |
|
31 |
- <td></td> |
|
32 |
- <td></td> |
|
33 |
- </tr> |
|
34 |
- </tbody> |
|
35 |
- </table> |
|
36 |
- <article class="table-pagination flex justify-center align-center mb20 mt30" style="gap: 10px;"> |
|
37 |
- <button><img src="../../../resources/img/btn27_90t_normal.png" alt=""></button> |
|
38 |
- <button class="selected-btn">1</button> |
|
39 |
- <button>2</button> |
|
40 |
- <button>3</button> |
|
41 |
- <button><img src="../../../resources/img/btn28_90t_normal.png" alt=""></button> |
|
42 |
- </article> |
|
43 |
- <div class="flex justify-end "> |
|
44 |
- <button type="button" title="등록" class="new-btn" @click="goToPage('TextInsert')"> |
|
45 |
- 등록 |
|
46 |
- </button> |
|
27 |
+ </tr> |
|
28 |
+ </thead> |
|
29 |
+ <tbody> |
|
30 |
+ <tr v-for="(post, index) in posts" :key="index" class="post" |
|
31 |
+ @click="goToPage('TextDetail', post.textId)"> |
|
32 |
+ <td>{{ index + 1 + (currentPage - 1) * pageSize }}</td> |
|
33 |
+ <td>{{ post.textTtl.slice(0, 20) }}{{ post.textTtl.length > 20 ? '...' : '' }}</td> |
|
34 |
+ <td>{{ post.textCnt.slice(0, 20) }}{{ post.textCnt.length > 20 ? '...' : '' }}</td> |
|
35 |
+ <td>{{ post.userId }}</td> |
|
36 |
+ <td>{{ post.regDt }}</td> |
|
37 |
+ </tr> |
|
38 |
+ </tbody> |
|
39 |
+ </table> |
|
40 |
+ <article class="table-pagination flex justify-center align-center mb20 mt30" style="gap: 10px;"> |
|
41 |
+ <button @click="changePage(currentPage - 1)" :disabled="currentPage === 1"> |
|
42 |
+ <img src="../../../resources/img/btn27_90t_normal.png" alt="Previous"> |
|
43 |
+ </button> |
|
44 |
+ <button v-for="page in totalPages" :key="page" @click="changePage(page)" |
|
45 |
+ :class="{ 'selected-btn': currentPage === page }"> |
|
46 |
+ {{ page }} |
|
47 |
+ </button> |
|
48 |
+ <button @click="changePage(currentPage + 1)" :disabled="currentPage === totalPages"> |
|
49 |
+ <img src="../../../resources/img/btn28_90t_normal.png" alt="Next"> |
|
50 |
+ </button> |
|
51 |
+ </article> |
|
52 |
+ <div class="flex justify-end"> |
|
53 |
+ <button type="button" title="등록" class="new-btn" @click="goToPage('TextInsert')"> |
|
54 |
+ 등록 |
|
55 |
+ </button> |
|
47 | 56 |
</div> |
48 |
- </div> |
|
57 |
+ </div> |
|
49 | 58 |
</template> |
50 | 59 |
|
51 | 60 |
<script> |
61 |
+import axios from "axios"; |
|
52 | 62 |
import SvgIcon from '@jamescoyle/vue-icon'; |
53 |
-import { mdiMagnify} from '@mdi/js'; |
|
54 |
- |
|
63 |
+import { mdiMagnify } from '@mdi/js'; |
|
55 | 64 |
|
56 | 65 |
export default { |
57 |
- data () { |
|
66 |
+ data() { |
|
58 | 67 |
return { |
59 | 68 |
mdiMagnify: mdiMagnify, |
60 |
- } |
|
69 |
+ posts: [], |
|
70 |
+ currentPage: 1, |
|
71 |
+ pageSize: 10, |
|
72 |
+ totalPosts: 0, |
|
73 |
+ option: "", |
|
74 |
+ keyword: "", |
|
75 |
+ optionList: [ |
|
76 |
+ { text: "번호", value: "textId" }, |
|
77 |
+ { text: "제목", value: "textTtl" }, |
|
78 |
+ { text: "내용", value: "textCnt" }, |
|
79 |
+ { text: "작성자", value: "userId" }, |
|
80 |
+ { text: "등록일", value: "regDt" }, |
|
81 |
+ ], |
|
82 |
+ searching: false, |
|
83 |
+ |
|
84 |
+ }; |
|
61 | 85 |
}, |
62 | 86 |
methods: { |
63 |
- goToPage(page) { |
|
64 |
- this.$router.push({ name: page }); |
|
65 |
- }, |
|
66 |
- showConfirm(type) { |
|
87 |
+ goToPage(page, textId) { |
|
88 |
+ this.$router.push({ name: page, query: { textId } }); |
|
89 |
+ }, |
|
90 |
+ showConfirm(type) { |
|
67 | 91 |
let message = ''; |
68 | 92 |
if (type === 'cancel') { |
69 | 93 |
message = '삭제하시겠습니까?'; |
... | ... | @@ -77,19 +101,64 @@ |
77 | 101 |
this.goBack(); |
78 | 102 |
} |
79 | 103 |
}, |
80 |
- |
|
81 |
- }, |
|
82 |
- watch: { |
|
83 |
- |
|
84 |
- }, |
|
85 |
- computed: { |
|
86 |
- |
|
87 |
- }, |
|
88 |
- components:{ |
|
89 |
- SvgIcon |
|
90 |
- }, |
|
91 |
- mounted() { |
|
92 |
- console.log('Main2 mounted'); |
|
93 |
- } |
|
94 |
-} |
|
95 |
-</script>(No newline at end of file) |
|
104 |
+ search() { |
|
105 |
+ if (!this.option) { |
|
106 |
+ alert("검색유형을 선택해 주세요") |
|
107 |
+ } else { |
|
108 |
+ this.currentPage = 1; // Reset to first page on new search |
|
109 |
+ this.searching = true; |
|
110 |
+ this.fetchData(); // Call the method to fetch search results} |
|
111 |
+ }}, |
|
112 |
+ fetchData() { |
|
113 |
+ const idx = (this.currentPage - 1) * this.pageSize; |
|
114 |
+ axios({ |
|
115 |
+ url: "/text/textSearch.json", |
|
116 |
+ method: "post", |
|
117 |
+ headers: { |
|
118 |
+ "Content-Type": "application/json; charset=UTF-8", |
|
119 |
+ }, |
|
120 |
+ data: { |
|
121 |
+ "option": this.option, |
|
122 |
+ "keyword": this.keyword, |
|
123 |
+ "pageSize": this.pageSize, |
|
124 |
+ "startIndex": idx |
|
125 |
+ }, |
|
126 |
+ }) |
|
127 |
+ .then(response => { |
|
128 |
+ this.posts = response.data.list; |
|
129 |
+ if (!this.searching || this.keyword == "") { |
|
130 |
+ this.totalPosts = response.data.totalText; |
|
131 |
+ } |
|
132 |
+ else if (this.searching) |
|
133 |
+ this.totalPosts = response.data.resultCount; |
|
134 |
+ this.posts.forEach(post => { |
|
135 |
+ let regDt = post.regDt; |
|
136 |
+ let date = new Date(regDt); |
|
137 |
+ post.regDt = date.toISOString().split('T')[0]; |
|
138 |
+ }); |
|
139 |
+ }) |
|
140 |
+ .catch(error => { |
|
141 |
+ console.error("fetchData - error: ", error); |
|
142 |
+ alert("검색 중 오류가 발생했습니다."); |
|
143 |
+ }); |
|
144 |
+ }, |
|
145 |
+ changePage(page) { |
|
146 |
+ if (page > 0 && page <= this.totalPages) { |
|
147 |
+ this.currentPage = page; |
|
148 |
+ this.fetchData(); |
|
149 |
+ } |
|
150 |
+ } |
|
151 |
+ }, |
|
152 |
+ computed: { |
|
153 |
+ totalPages() { |
|
154 |
+ return Math.ceil(this.totalPosts / this.pageSize); |
|
155 |
+ } |
|
156 |
+ }, |
|
157 |
+ components: { |
|
158 |
+ SvgIcon |
|
159 |
+ }, |
|
160 |
+ mounted() { |
|
161 |
+ this.fetchData(); |
|
162 |
+ } |
|
163 |
+ }; |
|
164 |
+</script> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?