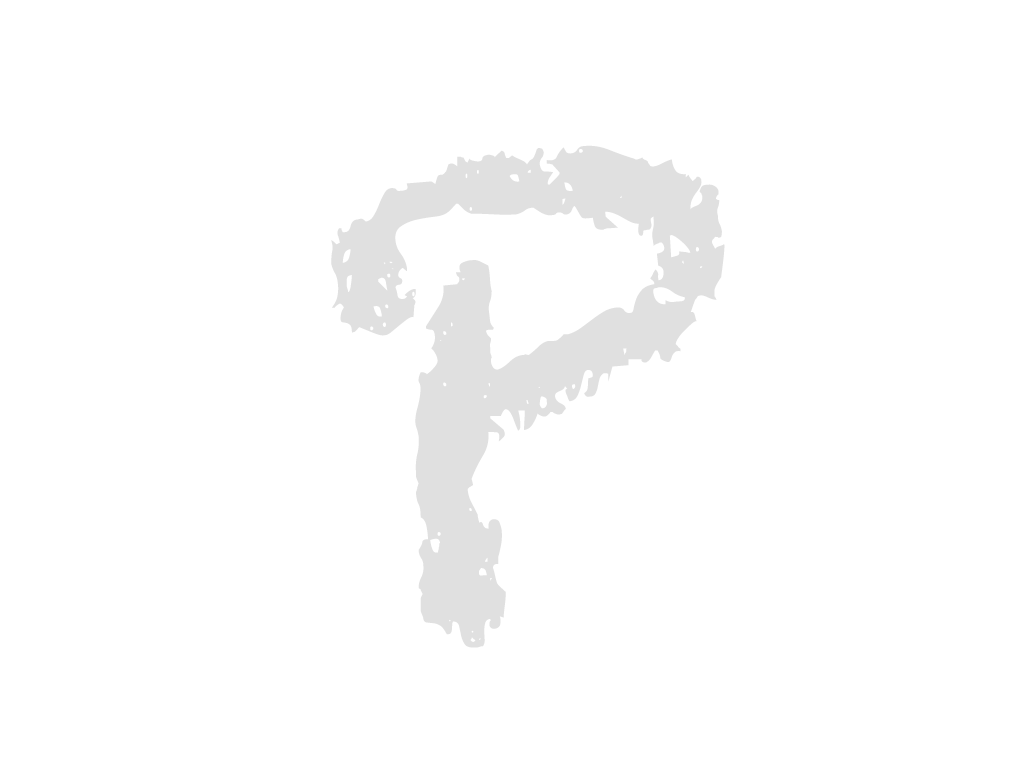
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import { createStore } from "vuex";
// Local storage에 상태를 저장하는 함수
function saveStateToLocalStorage(state) {
localStorage.setItem("vuexState", JSON.stringify(state));
}
// Local storage에서 상태를 불러오는 함수
function loadStateFromLocalStorage() {
const savedState = localStorage.getItem("vuexState");
if (savedState) {
return JSON.parse(savedState);
}
return {
token: null,
userId: null,
authcd: null,
stdId: null,
schdlId: null,
bookId: null,
unitId: null,
unitNm: null,
LearningId: null,
// 문제
currentLearningIds: [],
prblmTypeId: null,
currentLabel: null,
currentProblemIndex: 0,
allProblems: [],
allAnswers: [],
// 단어장
textId: null,
wdBookIdList: [],
currentWdBkIndex: 0,
// 순서
seqNum: null,
};
}
export default createStore({
state: loadStateFromLocalStorage(),
getters: {
getUserInfo(state) {
return {
userId: state.userId,
userNm: state.userNm,
authcd: state.authcd,
};
},
getStdId(state) {
return state.stdId;
},
getSchdlId(state) {
return state.schdlId;
},
getBookId(state) {
return state.bookId;
},
getUnitId(state) {
return state.unitId;
},
getLearningId(state) {
return state.learningId;
},
getTextId(state) {
return state.textId;
},
// 문제
currentLearningId(state) {
return state.currentLearningIds[state.currentProblemIndex];
},
currentLabel: (state) => state.currentLabel,
currentProblemIndex: (state) => state.currentProblemIndex,
seqNum: (state) => state.seqNum,
prblmTypeId: (state) => state.prblmTypeId,
getAllProblems(state) {
return state.allProblems;
},
getAllAnswers: state => state.allAnswers,
// 단어장
getWdBookIdList(state) {
return state.wdBookIdList;
},
getCurrentWdBkIndex: (state) => state.currentWdBkIndex,
},
mutations: {
setToken(state, token) {
state.token = token;
},
clearState(state) {
state.token = null;
state.userId = null;
state.userNm = null;
state.authcd = null;
state.stdId = null;
state.schdlId = null;
state.bookId = null;
state.unitId = null;
state.textId = null;
state.learningId = null;
state.currentLearningIds = [];
state.prblmTypeId = null;
state.currentLabel = null;
state.currentProblemIndex = null;
state.allProblems = [];
state.allAnswers = [];
state.wdBookIdList = [],
state.currentWdBkIndex = 0;
state.seqNum = null;
saveStateToLocalStorage(state);
},
setUser(state, userId) {
state.userId = userId;
saveStateToLocalStorage(state);
},
setUserNm(state, userNm) {
state.userNm = userNm;
saveStateToLocalStorage(state);
},
setAuthcd(state, authcd) {
state.authcd = authcd;
saveStateToLocalStorage(state);
},
setStdId(state, stdId) {
state.stdId = stdId;
saveStateToLocalStorage(state);
},
setSchdlId(state, schdlId) {
state.schdlId = schdlId;
saveStateToLocalStorage(state);
},
setBookId(state, bookId) {
state.bookId = bookId;
saveStateToLocalStorage(state);
},
setUnitId(state, unitId) {
state.unitId = unitId;
saveStateToLocalStorage(state);
},
setLearningId(state, learningId) {
state.learningId = learningId;
saveStateToLocalStorage(state);
},
setTextId(state, textId) {
state.textId = textId;
saveStateToLocalStorage(state);
},
// 순서
// setSeqNum(state, seqNum) {
// state.seqNum = seqNum;
// saveStateToLocalStorage(state);
// },
// 문제
setLearningData(state, payload) {
state.currentLearningIds = payload.learning_id; // Array of IDs
state.currentLabel = payload.label;
state.currentProblemIndex = 0; // Reset to the first problem
state.prblmTypeId = payload.prblmTypeId;
// 순서
state.seqNum = payload.seqNum;
saveStateToLocalStorage(state);
},
incrementProblemIndex(state) {
if (state.currentProblemIndex < state.currentLearningIds.length - 1) {
state.currentProblemIndex++;
saveStateToLocalStorage(state);
}
},
decrementProblemIndex(state) {
if (state.currentProblemIndex > 0) {
state.currentProblemIndex--;
saveStateToLocalStorage(state);
}
},
saveOrUpdateProblem(state, problemData) {
const existingProblemIndex = state.allProblems.findIndex(
(problem) => problem.prblmNumber === problemData.prblmNumber
);
if (existingProblemIndex !== -1) {
// 문제 데이터 업데이트
state.allProblems[existingProblemIndex] = problemData;
} else {
// 새로운 문제 추가
state.allProblems.push(problemData);
}
},
addOrUpdateProblemAttempt(state, answerData) {
if (!state.allAnswers) {
state.allAnswers = [];
}
const existingEntryIndex = state.allAnswers.findIndex(
answer => answer.prblmId === answerData.prblmId
);
if (existingEntryIndex !== -1) {
// 정답 데이터 업데이트
state.allAnswers[existingEntryIndex] = answerData;
} else {
// 새로운 정답 추가
state.allAnswers.push(answerData);
}
},
// 단어장
setWdBookIdList(state, wdBookIdList) {
state.wdBookIdList = wdBookIdList;
saveStateToLocalStorage(state);
},
setCurrentWdBkIndex(state, currentWdBkIndex) {
state.currentWdBkIndex = currentWdBkIndex;
saveStateToLocalStorage(state);
},
},
actions: {
login({ commit }, token) {
commit("setToken", token);
localStorage.setItem("token", token);
},
logout({ commit }) {
commit("clearState");
localStorage.removeItem("token");
localStorage.removeItem("vuexState");
},
updateStdId({ commit }, payload) {
return new Promise((resolve) => {
commit("setStdId", payload);
resolve();
});
},
updateSchdlId({ commit }, payload) {
return new Promise((resolve) => {
commit("setSchdlId", payload);
resolve();
});
},
updateBookId({ commit }, payload) {
return new Promise((resolve) => {
commit("setBookId", payload);
resolve();
});
},
updateUnitId({ commit }, payload) {
return new Promise((resolve) => {
commit("setUnitId", payload);
resolve();
});
},
updateLearningId({ commit }, learningId) {
commit("setLearningId", learningId);
},
updateLearningData({ commit }, payload) {
commit("setLearningData", payload);
},
updateTextId({ commit }, textId) {
commit("setTextId", textId);
},
// 문제
goToNextProblem({ commit }) {
commit("incrementProblemIndex");
},
goToPreviousProblem({ commit }) {
commit("decrementProblemIndex");
},
saveProblemData({ commit }, problemData) {
commit("saveOrUpdateProblem", problemData);
},
saveProblemAttempt({ commit }, answerData) {
commit('addOrUpdateProblemAttempt', answerData);
},
// 단어장
updateWdBookIdList({ commit }, wdBookIdList) {
commit("setWdBookIdList", wdBookIdList);
},
updateCurrentWdBkIndex({ commit }, currentWdBkIndex) {
commit("setCurrentWdBkIndex", currentWdBkIndex);
},
// 순서
// updateSeqNum({ commit }) {
// commit("setSeqNum", seqNum);
// },
},
});