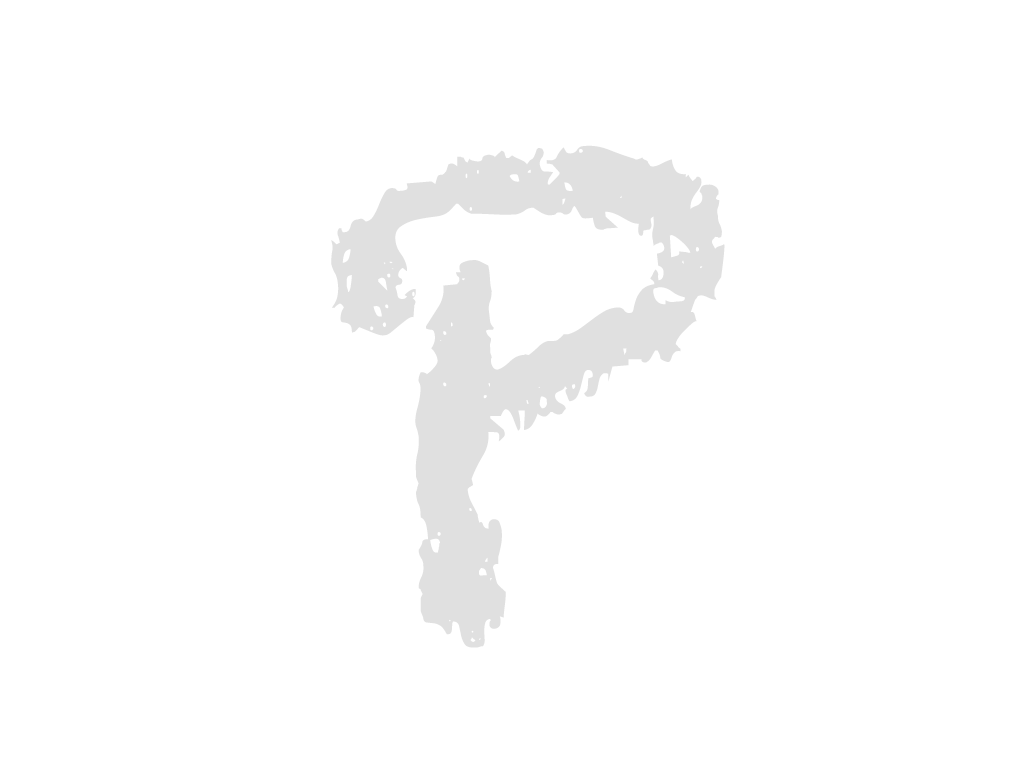
--- src/main/java/com/takensoft/ai_lms/common/confing/SecurityConfig.java
+++ src/main/java/com/takensoft/ai_lms/common/confing/SecurityConfig.java
... | ... | @@ -64,6 +64,7 @@ |
64 | 64 |
.requestMatchers("/test/**").permitAll() |
65 | 65 |
.requestMatchers("/studentInfo/**").permitAll() // 학생 정보 진입 허용(민수) |
66 | 66 |
.requestMatchers("/board/**").permitAll() // 게시판 정보 진입 허용 |
67 |
+ .requestMatchers("/file/**").permitAll() // 게시판 정보 진입 허용 |
|
67 | 68 |
.anyRequest().authenticated()); // 나머지 경로는 인증 필요 |
68 | 69 |
|
69 | 70 |
// jwt 필터 처리 적용 |
--- src/main/java/com/takensoft/ai_lms/lms/board/web/BoardController.java
+++ src/main/java/com/takensoft/ai_lms/lms/board/web/BoardController.java
... | ... | @@ -3,6 +3,7 @@ |
3 | 3 |
|
4 | 4 |
import com.takensoft.ai_lms.lms.board.service.BoardService; |
5 | 5 |
import com.takensoft.ai_lms.lms.board.vo.BoardVO; |
6 |
+import com.takensoft.ai_lms.lms.file.service.FileService; |
|
6 | 7 |
import lombok.RequiredArgsConstructor; |
7 | 8 |
import lombok.extern.slf4j.Slf4j; |
8 | 9 |
import org.springframework.http.HttpStatus; |
+++ src/main/java/com/takensoft/ai_lms/lms/file/dao/FileDAO.java
... | ... | @@ -0,0 +1,22 @@ |
1 | +package com.takensoft.ai_lms.lms.file.dao; | |
2 | + | |
3 | + | |
4 | +import org.egovframe.rte.psl.dataaccess.mapper.Mapper; | |
5 | + | |
6 | +import java.util.HashMap; | |
7 | +import java.util.List; | |
8 | + | |
9 | +/** | |
10 | + * @author : 홍아랑 | |
11 | + * since : 2024.07.26 | |
12 | + * | |
13 | + * 파일 관련 Mapper | |
14 | + */ | |
15 | + | |
16 | +@Mapper("FileDAO") | |
17 | + | |
18 | +public interface FileDAO { | |
19 | + | |
20 | + // 파일 정보 조회 | |
21 | + List<HashMap<String, Object>> findByFileId(HashMap<String, Object> params) throws Exception; | |
22 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/file/service/FileService.java
... | ... | @@ -0,0 +1,16 @@ |
1 | +package com.takensoft.ai_lms.lms.file.service; | |
2 | + | |
3 | + | |
4 | +import java.util.HashMap; | |
5 | +import java.util.List; | |
6 | + | |
7 | +/** | |
8 | + * @author : 홍아랑 | |
9 | + * since : 2024.07.26 | |
10 | + * | |
11 | + * 파일 관련 Service | |
12 | + */ | |
13 | +public interface FileService { | |
14 | + // 파일 정보 조회 | |
15 | + List<HashMap<String, Object>> findByFileId(HashMap<String, Object> params) throws Exception; | |
16 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/file/service/impl/FileServiceImpl.java
... | ... | @@ -0,0 +1,22 @@ |
1 | +package com.takensoft.ai_lms.lms.file.service.impl; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.file.dao.FileDAO; | |
4 | +import com.takensoft.ai_lms.lms.file.service.FileService; | |
5 | +import lombok.RequiredArgsConstructor; | |
6 | +import org.egovframe.rte.fdl.cmmn.EgovAbstractServiceImpl; | |
7 | +import org.springframework.stereotype.Service; | |
8 | + | |
9 | +import java.util.HashMap; | |
10 | +import java.util.List; | |
11 | + | |
12 | +@Service("FileService") | |
13 | +@RequiredArgsConstructor | |
14 | +public class FileServiceImpl extends EgovAbstractServiceImpl implements FileService { | |
15 | + private final FileDAO fileDAO; | |
16 | + | |
17 | + // 파일 정보 조회 | |
18 | + // @Override | |
19 | + public List<HashMap<String, Object>> findByFileId(HashMap<String, Object> params) throws Exception { | |
20 | + return fileDAO.findByFileId(params); | |
21 | + } | |
22 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/file/vo/FileVO.java
... | ... | @@ -0,0 +1,46 @@ |
1 | +package com.takensoft.ai_lms.lms.file.vo; | |
2 | + | |
3 | +import lombok.AllArgsConstructor; | |
4 | +import lombok.Getter; | |
5 | +import lombok.NoArgsConstructor; | |
6 | +import lombok.Setter; | |
7 | + | |
8 | +/** | |
9 | + * @author : 홍아랑 | |
10 | + * since : 2024.07.26 | |
11 | + * | |
12 | + * 파일 관련 VO | |
13 | + */ | |
14 | + | |
15 | + | |
16 | + | |
17 | +@Getter | |
18 | +@Setter | |
19 | +@AllArgsConstructor | |
20 | +@NoArgsConstructor | |
21 | +public class FileVO { | |
22 | + | |
23 | + // 파일 아이디 | |
24 | + private String fileId; | |
25 | + // 파일 관리 아이디 | |
26 | + private String fileMngId; | |
27 | + // 파일명 | |
28 | + private String fileNm; | |
29 | + // 마스크명 | |
30 | + private String fileEncptNm; | |
31 | + // 절대 경로 | |
32 | + private String fileApath; | |
33 | + // 상대 경로 | |
34 | + private String fileRpath; | |
35 | + // 확장자 | |
36 | + private String fileExtn; | |
37 | + // 파일 크기 | |
38 | + private String fileSz; | |
39 | + // 파일 타입 | |
40 | + private String fileClsf; | |
41 | + // 등록자 | |
42 | + private String reg; | |
43 | + // 등록 일자 | |
44 | + private String regDt; | |
45 | + | |
46 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/file/web/FileController.java
... | ... | @@ -0,0 +1,39 @@ |
1 | +package com.takensoft.ai_lms.lms.file.web; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.file.service.FileService; | |
4 | +import lombok.RequiredArgsConstructor; | |
5 | +import org.springframework.http.HttpStatus; | |
6 | +import org.springframework.http.ResponseEntity; | |
7 | +import org.springframework.web.bind.annotation.PostMapping; | |
8 | +import org.springframework.web.bind.annotation.RequestBody; | |
9 | +import org.springframework.web.bind.annotation.RequestMapping; | |
10 | +import org.springframework.web.bind.annotation.RestController; | |
11 | + | |
12 | +import java.util.HashMap; | |
13 | + | |
14 | +/** | |
15 | + * @author : 홍아랑 | |
16 | + * since : 2024.07.26 | |
17 | + * 파일 관련 Controller | |
18 | + */ | |
19 | +@RestController | |
20 | +//@Slf4j | |
21 | +@RequiredArgsConstructor | |
22 | +@RequestMapping(value = "/file") | |
23 | +public class FileController { | |
24 | + private final FileService fileService; | |
25 | + | |
26 | + /** | |
27 | + * @author 홍아랑 | |
28 | + * @since 2024.07.26 | |
29 | + * | |
30 | + * 파일 정보 조회 | |
31 | + */ | |
32 | + @PostMapping("/find.json") | |
33 | + public ResponseEntity<?> findByFileId(@RequestBody HashMap<String, Object> params) throws Exception { | |
34 | + HashMap<String, Object> result = new HashMap<>(); | |
35 | + | |
36 | + result.put("list", fileService.findByFileId(params)); | |
37 | + return new ResponseEntity<>(result, HttpStatus.OK); | |
38 | + } | |
39 | +} |
+++ src/main/resources/mybatis/mapper/lms/files-SQL.xml
... | ... | @@ -0,0 +1,45 @@ |
1 | +<?xml version="1.0" encoding="UTF-8"?> | |
2 | +<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> | |
3 | +<mapper namespace="com.takensoft.ai_lms.lms.file.dao.FileDAO"> | |
4 | + <!-- | |
5 | + 작 성 자 : 박민혁 | |
6 | + 작 성 일 : 2024.07.25 | |
7 | + 내 용 : 경로 생성을 위해 만들어 놓은 xml, | |
8 | + CRUD를 이용하는데 삭제하거나 수정해서 사용해주세요 | |
9 | + --> | |
10 | + <resultMap id="FileMap" type="FileVO"> | |
11 | + <result property="fileId" column="file_id"/> | |
12 | + <result property="fileMngId" column="file_mng_id"/> | |
13 | + <result property="fileNm" column="file_nm"/> | |
14 | + <result property="fileEncptNm" column="file_encpt_nm"/> | |
15 | + <result property="fileApath" column="file_apath"/> | |
16 | + <result property="fileRpath" column="file_rpath"/> | |
17 | + <result property="fileExtn" column="file_extn"/> | |
18 | + <result property="fileSz" column="file_sz"/> | |
19 | + <result property="fileClsf" column="file_clsf"/> | |
20 | + <result property="reg" column="reg"/> | |
21 | + <result property="regDt" column="reg_dt"/> | |
22 | + </resultMap> | |
23 | + | |
24 | + <!-- | |
25 | + 작성자 : 홍아랑 | |
26 | + 작성일 : 2024.07.26 | |
27 | + 내 용 : 파일 정보 조회 | |
28 | + --> | |
29 | + <select id="findByFileId" parameterType="FileVO" resultMap="FileMap"> | |
30 | + SELECT | |
31 | + file_id, | |
32 | + file_mng_id, | |
33 | + file_nm, | |
34 | + file_encpt_nm, | |
35 | + file_apath, | |
36 | + file_rpath, | |
37 | + file_extn, | |
38 | + file_sz, | |
39 | + file_clsf, | |
40 | + reg, | |
41 | + reg_dt | |
42 | + FROM cmmn_file | |
43 | + WHERE file_mng_id = #{file_mng_id} | |
44 | + </select> | |
45 | +</mapper>(파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?