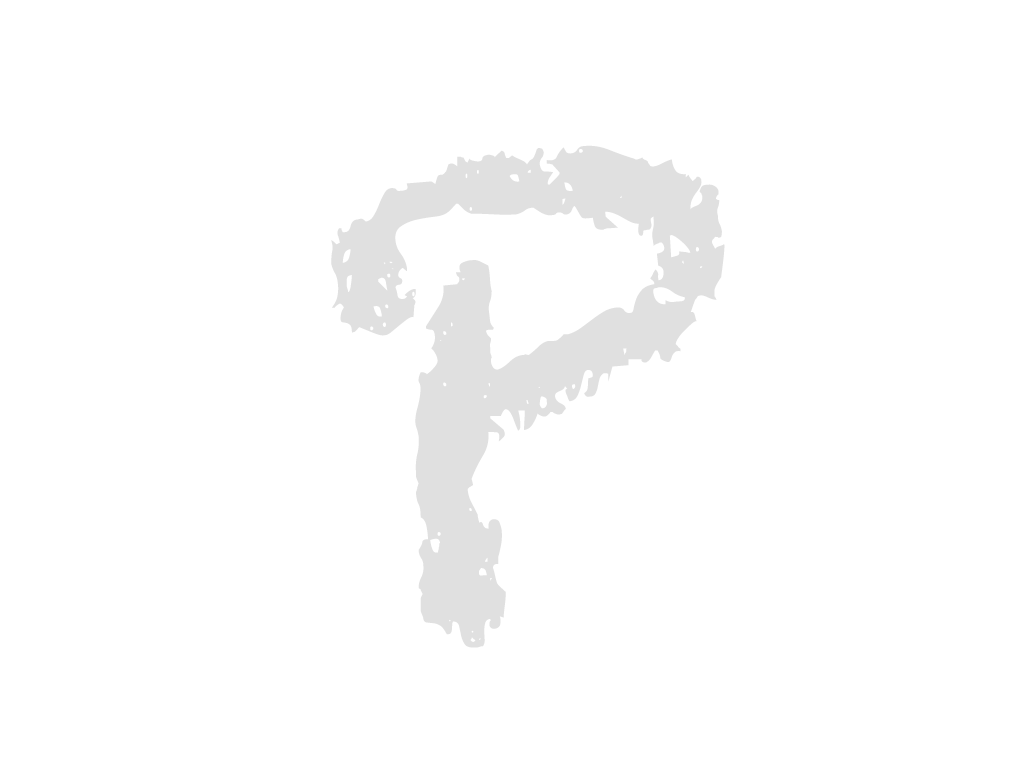
--- src/main/java/com/takensoft/ai_lms/lms/book/dao/BookDAO.java
+++ src/main/java/com/takensoft/ai_lms/lms/book/dao/BookDAO.java
... | ... | @@ -36,7 +36,7 @@ |
36 | 36 |
* |
37 | 37 |
* 교재 등록 |
38 | 38 |
*/ |
39 |
- void insertBook(BookVO book); |
|
39 |
+ int insertBook(BookVO bookVO); |
|
40 | 40 |
|
41 | 41 |
/** |
42 | 42 |
* @author : 구자현 |
... | ... | @@ -44,7 +44,7 @@ |
44 | 44 |
* |
45 | 45 |
* 교재 제목 수정 |
46 | 46 |
*/ |
47 |
- void updateBook(BookVO book); |
|
47 |
+ int updateBook(BookVO bookVO); |
|
48 | 48 |
|
49 | 49 |
/** |
50 | 50 |
* @author : 구자현 |
... | ... | @@ -52,5 +52,6 @@ |
52 | 52 |
* |
53 | 53 |
* 교재 삭제 |
54 | 54 |
*/ |
55 |
- void deleteBook(String book_id); |
|
55 |
+ int deleteBook(String book_id); |
|
56 |
+ |
|
56 | 57 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/book/service/BookService.java
+++ src/main/java/com/takensoft/ai_lms/lms/book/service/BookService.java
... | ... | @@ -33,7 +33,7 @@ |
33 | 33 |
* |
34 | 34 |
* 교재 등록 |
35 | 35 |
*/ |
36 |
- void insertBook(BookVO book); |
|
36 |
+ int insertBook(BookVO book); |
|
37 | 37 |
|
38 | 38 |
/** |
39 | 39 |
* @author : 구자현 |
... | ... | @@ -41,7 +41,7 @@ |
41 | 41 |
* |
42 | 42 |
* 교재 제목 수정 |
43 | 43 |
*/ |
44 |
- void updateBook(BookVO book); |
|
44 |
+ int updateBook(BookVO book); |
|
45 | 45 |
|
46 | 46 |
/** |
47 | 47 |
* @author : 구자현 |
... | ... | @@ -49,5 +49,6 @@ |
49 | 49 |
* |
50 | 50 |
* 교재 삭제 |
51 | 51 |
*/ |
52 |
- void deleteBook(String book_id); |
|
52 |
+ int deleteBook(String book_id); |
|
53 |
+ |
|
53 | 54 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/book/service/Impl/BookServiceImpl.java
+++ src/main/java/com/takensoft/ai_lms/lms/book/service/Impl/BookServiceImpl.java
... | ... | @@ -52,10 +52,10 @@ |
52 | 52 |
* 교재 등록 |
53 | 53 |
*/ |
54 | 54 |
@Override |
55 |
- public void insertBook(BookVO book) { |
|
55 |
+ public int insertBook(BookVO book) { |
|
56 | 56 |
String book_id = bookIdgn.getNextStringId(); |
57 | 57 |
book.setBook_id(book_id); |
58 |
- bookDAO.insertBook(book); |
|
58 |
+ return bookDAO.insertBook(book); |
|
59 | 59 |
} |
60 | 60 |
|
61 | 61 |
/** |
... | ... | @@ -65,8 +65,8 @@ |
65 | 65 |
* 교재 제목 수정 |
66 | 66 |
*/ |
67 | 67 |
@Override |
68 |
- public void updateBook(BookVO book) { |
|
69 |
- bookDAO.updateBook(book); |
|
68 |
+ public int updateBook(BookVO book) { |
|
69 |
+ return bookDAO.updateBook(book); |
|
70 | 70 |
} |
71 | 71 |
|
72 | 72 |
/** |
... | ... | @@ -76,7 +76,8 @@ |
76 | 76 |
* 교재 삭제 |
77 | 77 |
*/ |
78 | 78 |
@Override |
79 |
- public void deleteBook(String book_id) { |
|
80 |
- bookDAO.deleteBook(book_id); |
|
79 |
+ public int deleteBook(String book_id) { |
|
80 |
+ return bookDAO.deleteBook(book_id); |
|
81 | 81 |
} |
82 |
+ |
|
82 | 83 |
}(파일 끝에 줄바꿈 문자 없음) |
--- src/main/java/com/takensoft/ai_lms/lms/book/web/BookController.java
+++ src/main/java/com/takensoft/ai_lms/lms/book/web/BookController.java
... | ... | @@ -3,9 +3,9 @@ |
3 | 3 |
import com.takensoft.ai_lms.lms.book.service.Impl.BookServiceImpl; |
4 | 4 |
import com.takensoft.ai_lms.lms.book.vo.BookVO; |
5 | 5 |
import lombok.RequiredArgsConstructor; |
6 |
+import org.springframework.http.HttpStatus; |
|
7 |
+import org.springframework.http.ResponseEntity; |
|
6 | 8 |
import org.springframework.web.bind.annotation.*; |
7 |
- |
|
8 |
-import java.util.List; |
|
9 | 9 |
|
10 | 10 |
/** |
11 | 11 |
* @author : 구자현 |
... | ... | @@ -26,9 +26,9 @@ |
26 | 26 |
* |
27 | 27 |
* 전체 교재 목록 출력 |
28 | 28 |
*/ |
29 |
- @GetMapping("/findAll.json") |
|
30 |
- public List<BookVO> getAllBooks() { |
|
31 |
- return bookServiceImpl.getAllBooks(); |
|
29 |
+ @PostMapping("/findAll.json") |
|
30 |
+ public ResponseEntity<?> getAllBooks() { |
|
31 |
+ return new ResponseEntity<>(bookServiceImpl.getAllBooks(), HttpStatus.OK); |
|
32 | 32 |
} |
33 | 33 |
|
34 | 34 |
/** |
... | ... | @@ -37,9 +37,9 @@ |
37 | 37 |
* |
38 | 38 |
* 책의 상세 정보 |
39 | 39 |
*/ |
40 |
- @GetMapping("/find.json") |
|
41 |
- public BookVO getBookById(@RequestBody BookVO bookVO) { |
|
42 |
- return bookServiceImpl.getBookById(bookVO.getBook_id()); |
|
40 |
+ @PostMapping("/find.json") |
|
41 |
+ public ResponseEntity<?> getBookById(@RequestBody BookVO bookVO) { |
|
42 |
+ return new ResponseEntity<>(bookServiceImpl.getBookById(bookVO.getBook_id()), HttpStatus.OK); |
|
43 | 43 |
} |
44 | 44 |
|
45 | 45 |
/** |
... | ... | @@ -49,8 +49,8 @@ |
49 | 49 |
* 교재 등록 |
50 | 50 |
*/ |
51 | 51 |
@PostMapping("/insert.json") |
52 |
- public void insertBook(@RequestBody BookVO book) { |
|
53 |
- bookServiceImpl.insertBook(book); |
|
52 |
+ public ResponseEntity<?> insertBook(@RequestBody BookVO book) { |
|
53 |
+ return new ResponseEntity<>(bookServiceImpl.insertBook(book), HttpStatus.OK); |
|
54 | 54 |
} |
55 | 55 |
|
56 | 56 |
/** |
... | ... | @@ -59,9 +59,9 @@ |
59 | 59 |
* |
60 | 60 |
* 교재 제목 수정 |
61 | 61 |
*/ |
62 |
- @PutMapping("/update.json") |
|
63 |
- public void updateBook(@RequestBody BookVO book) { |
|
64 |
- bookServiceImpl.updateBook(book); |
|
62 |
+ @PostMapping("/update.json") |
|
63 |
+ public ResponseEntity<?> updateBook(@RequestBody BookVO book) { |
|
64 |
+ return new ResponseEntity<>(bookServiceImpl.updateBook(book), HttpStatus.OK); |
|
65 | 65 |
} |
66 | 66 |
|
67 | 67 |
/** |
... | ... | @@ -70,9 +70,9 @@ |
70 | 70 |
* |
71 | 71 |
* 교재 삭제 |
72 | 72 |
*/ |
73 |
- @DeleteMapping("/delete.json") |
|
74 |
- public void deleteBook(@RequestBody BookVO bookVO) { |
|
75 |
- bookServiceImpl.deleteBook(bookVO.getBook_id()); |
|
73 |
+ @PostMapping("/delete.json") |
|
74 |
+ public ResponseEntity<?> deleteBook(@RequestBody BookVO bookVO) { |
|
75 |
+ return new ResponseEntity<>(bookServiceImpl.deleteBook(bookVO.getBook_id()), HttpStatus.OK); |
|
76 | 76 |
} |
77 | 77 |
|
78 | 78 |
}(파일 끝에 줄바꿈 문자 없음) |
--- src/main/java/com/takensoft/ai_lms/lms/unit_learning/dao/UnitLearningDAO.java
+++ src/main/java/com/takensoft/ai_lms/lms/unit_learning/dao/UnitLearningDAO.java
... | ... | @@ -18,17 +18,9 @@ |
18 | 18 |
* @author : 구자현 |
19 | 19 |
* @since : 2024.07.26 |
20 | 20 |
* |
21 |
- * 전체 로드맵 목록 출력 |
|
22 |
- */ |
|
23 |
- List<UnitLearningVO> getAllUnitLearning(); |
|
24 |
- |
|
25 |
- /** |
|
26 |
- * @author : 구자현 |
|
27 |
- * @since : 2024.07.26 |
|
28 |
- * |
|
29 | 21 |
* 로드맵의 상세 정보 |
30 | 22 |
*/ |
31 |
- UnitLearningVO getUnitLearningBySeq(int seq); |
|
23 |
+ List<UnitLearningVO> getUnitLearning(String unit_id, String book_id); |
|
32 | 24 |
|
33 | 25 |
/** |
34 | 26 |
* @author : 구자현 |
... | ... | @@ -36,7 +28,7 @@ |
36 | 28 |
* |
37 | 29 |
* 로드맵 등록 |
38 | 30 |
*/ |
39 |
- void insertUnitLearning(UnitLearningVO scheduleVO); |
|
31 |
+ int insertUnitLearning(List<UnitLearningVO> unitLearningVO); |
|
40 | 32 |
|
41 | 33 |
/** |
42 | 34 |
* @author : 구자현 |
... | ... | @@ -44,7 +36,7 @@ |
44 | 36 |
* |
45 | 37 |
* 로드맵 순서 수정 |
46 | 38 |
*/ |
47 |
- void updateUnitLearning(UnitLearningVO scheduleVO); |
|
39 |
+ int updateUnitLearning(UnitLearningVO unitLearningVO); |
|
48 | 40 |
|
49 | 41 |
/** |
50 | 42 |
* @author : 구자현 |
... | ... | @@ -52,5 +44,6 @@ |
52 | 44 |
* |
53 | 45 |
* 로드맵 삭제 |
54 | 46 |
*/ |
55 |
- void deleteUnitLearning(int seq); |
|
47 |
+ int deleteUnitLearning(String unit_id); |
|
48 |
+ |
|
56 | 49 |
} |
+++ src/main/java/com/takensoft/ai_lms/lms/unit_learning/dto/UnitLearningDTO.java
... | ... | @@ -0,0 +1,19 @@ |
1 | +package com.takensoft.ai_lms.lms.unit_learning.dto; | |
2 | + | |
3 | +import lombok.Getter; | |
4 | +import lombok.Setter; | |
5 | + | |
6 | +/** | |
7 | + * @author 구자현 | |
8 | + * @since 2024.07.31 | |
9 | + * | |
10 | + * 로드맵 조회용 DTO 클래스 | |
11 | + */ | |
12 | + | |
13 | +@Getter | |
14 | +@Setter | |
15 | +public class UnitLearningDTO { | |
16 | + private String book_id; | |
17 | + private String unit_id; | |
18 | +} | |
19 | + |
--- src/main/java/com/takensoft/ai_lms/lms/unit_learning/service/Impl/UnitLearningServiceImpl.java
+++ src/main/java/com/takensoft/ai_lms/lms/unit_learning/service/Impl/UnitLearningServiceImpl.java
... | ... | @@ -23,22 +23,11 @@ |
23 | 23 |
* @author : 구자현 |
24 | 24 |
* @since : 2024.07.26 |
25 | 25 |
* |
26 |
- * 전체 로드맵 목록 출력 |
|
27 |
- */ |
|
28 |
- @Override |
|
29 |
- public List<UnitLearningVO> getAllUnitLearning() { |
|
30 |
- return unitLearningDAO.getAllUnitLearning(); |
|
31 |
- }; |
|
32 |
- |
|
33 |
- /** |
|
34 |
- * @author : 구자현 |
|
35 |
- * @since : 2024.07.26 |
|
36 |
- * |
|
37 | 26 |
* 로드맵의 상세 정보 |
38 | 27 |
*/ |
39 | 28 |
@Override |
40 |
- public UnitLearningVO getUnitLearningBySeq(int seq) { |
|
41 |
- return unitLearningDAO.getUnitLearningBySeq(seq); |
|
29 |
+ public List<UnitLearningVO> getUnitLearning(String unit_id, String book_id) { |
|
30 |
+ return unitLearningDAO.getUnitLearning(unit_id, book_id); |
|
42 | 31 |
}; |
43 | 32 |
|
44 | 33 |
/** |
... | ... | @@ -48,9 +37,9 @@ |
48 | 37 |
* 로드맵 등록 |
49 | 38 |
*/ |
50 | 39 |
@Override |
51 |
- public void insertUnitLearning(UnitLearningVO unitLearningVO) { |
|
52 |
- unitLearningDAO.insertUnitLearning(unitLearningVO); |
|
53 |
- }; |
|
40 |
+ public int insertUnitLearning(List<UnitLearningVO> unitLearningVO) { |
|
41 |
+ return unitLearningDAO.insertUnitLearning(unitLearningVO); |
|
42 |
+ } |
|
54 | 43 |
|
55 | 44 |
/** |
56 | 45 |
* @author : 구자현 |
... | ... | @@ -59,8 +48,8 @@ |
59 | 48 |
* 로드맵 순서 수정 |
60 | 49 |
*/ |
61 | 50 |
@Override |
62 |
- public void updateUnitLearning(UnitLearningVO unitLearningVO) { |
|
63 |
- unitLearningDAO.updateUnitLearning(unitLearningVO); |
|
51 |
+ public int updateUnitLearning(UnitLearningVO unitLearningVO) { |
|
52 |
+ return unitLearningDAO.updateUnitLearning(unitLearningVO); |
|
64 | 53 |
}; |
65 | 54 |
|
66 | 55 |
/** |
... | ... | @@ -70,7 +59,8 @@ |
70 | 59 |
* 로드맵 삭제 |
71 | 60 |
*/ |
72 | 61 |
@Override |
73 |
- public void deleteUnitLearning(int seq) { |
|
74 |
- unitLearningDAO.deleteUnitLearning(seq); |
|
62 |
+ public int deleteUnitLearning(String unit_id) { |
|
63 |
+ return unitLearningDAO.deleteUnitLearning(unit_id); |
|
75 | 64 |
}; |
65 |
+ |
|
76 | 66 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/unit_learning/service/UnitLearningService.java
+++ src/main/java/com/takensoft/ai_lms/lms/unit_learning/service/UnitLearningService.java
... | ... | @@ -16,17 +16,9 @@ |
16 | 16 |
* @author : 구자현 |
17 | 17 |
* @since : 2024.07.26 |
18 | 18 |
* |
19 |
- * 전체 로드맵 목록 출력 |
|
20 |
- */ |
|
21 |
- List<UnitLearningVO> getAllUnitLearning(); |
|
22 |
- |
|
23 |
- /** |
|
24 |
- * @author : 구자현 |
|
25 |
- * @since : 2024.07.26 |
|
26 |
- * |
|
27 | 19 |
* 로드맵의 상세 정보 |
28 | 20 |
*/ |
29 |
- UnitLearningVO getUnitLearningBySeq(int seq); |
|
21 |
+ List<UnitLearningVO> getUnitLearning(String unit_id, String book_id); |
|
30 | 22 |
|
31 | 23 |
/** |
32 | 24 |
* @author : 구자현 |
... | ... | @@ -34,7 +26,7 @@ |
34 | 26 |
* |
35 | 27 |
* 로드맵 등록 |
36 | 28 |
*/ |
37 |
- void insertUnitLearning(UnitLearningVO unitLearningVO); |
|
29 |
+ int insertUnitLearning(List<UnitLearningVO> unitLearningList); |
|
38 | 30 |
|
39 | 31 |
/** |
40 | 32 |
* @author : 구자현 |
... | ... | @@ -42,7 +34,7 @@ |
42 | 34 |
* |
43 | 35 |
* 로드맵 순서 수정 |
44 | 36 |
*/ |
45 |
- void updateUnitLearning(UnitLearningVO unitLearningVO); |
|
37 |
+ int updateUnitLearning(UnitLearningVO unitLearningVO); |
|
46 | 38 |
|
47 | 39 |
/** |
48 | 40 |
* @author : 구자현 |
... | ... | @@ -50,5 +42,6 @@ |
50 | 42 |
* |
51 | 43 |
* 로드맵 삭제 |
52 | 44 |
*/ |
53 |
- void deleteUnitLearning(int seq); |
|
45 |
+ int deleteUnitLearning(String unit_id); |
|
46 |
+ |
|
54 | 47 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/unit_learning/web/UnitLearningController.java
+++ src/main/java/com/takensoft/ai_lms/lms/unit_learning/web/UnitLearningController.java
... | ... | @@ -1,8 +1,11 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.unit_learning.web; |
2 | 2 |
|
3 |
+import com.takensoft.ai_lms.lms.unit_learning.dto.UnitLearningDTO; |
|
3 | 4 |
import com.takensoft.ai_lms.lms.unit_learning.service.Impl.UnitLearningServiceImpl; |
4 | 5 |
import com.takensoft.ai_lms.lms.unit_learning.vo.UnitLearningVO; |
5 | 6 |
import lombok.RequiredArgsConstructor; |
7 |
+import org.springframework.http.HttpStatus; |
|
8 |
+import org.springframework.http.ResponseEntity; |
|
6 | 9 |
import org.springframework.web.bind.annotation.*; |
7 | 10 |
|
8 | 11 |
import java.util.List; |
... | ... | @@ -18,22 +21,11 @@ |
18 | 21 |
* @author : 구자현 |
19 | 22 |
* @since : 2024.07.26 |
20 | 23 |
* |
21 |
- * 전체 로드맵 목록 출력 |
|
22 |
- */ |
|
23 |
- @GetMapping("/findAll.json") |
|
24 |
- public List<UnitLearningVO> getAllUnitLearning(){ |
|
25 |
- return unitLearningServiceImpl.getAllUnitLearning(); |
|
26 |
- } |
|
27 |
- |
|
28 |
- /** |
|
29 |
- * @author : 구자현 |
|
30 |
- * @since : 2024.07.26 |
|
31 |
- * |
|
32 | 24 |
* 로드맵의 상세 정보 |
33 | 25 |
*/ |
34 |
- @GetMapping("/find.json") |
|
35 |
- public UnitLearningVO getUnitLearningBySeq(@RequestBody UnitLearningVO unitLearningVO){ |
|
36 |
- return unitLearningServiceImpl.getUnitLearningBySeq(unitLearningVO.getSeq()); |
|
26 |
+ @PostMapping("/find.json") |
|
27 |
+ public ResponseEntity<?> getUnitLearning(@RequestBody UnitLearningDTO unitLearningDTO){ |
|
28 |
+ return new ResponseEntity<>(unitLearningServiceImpl.getUnitLearning(unitLearningDTO.getUnit_id(), unitLearningDTO.getBook_id()), HttpStatus.OK); |
|
37 | 29 |
} |
38 | 30 |
|
39 | 31 |
/** |
... | ... | @@ -43,8 +35,8 @@ |
43 | 35 |
* 로드맵 등록 |
44 | 36 |
*/ |
45 | 37 |
@PostMapping("/insert.json") |
46 |
- public void insertUnitLearning(@RequestBody UnitLearningVO unitLearningVO) { |
|
47 |
- unitLearningServiceImpl.insertUnitLearning(unitLearningVO); |
|
38 |
+ public ResponseEntity<?> insertUnitLearning(@RequestBody List<UnitLearningVO> unitLearning) { |
|
39 |
+ return new ResponseEntity<>(unitLearningServiceImpl.insertUnitLearning(unitLearning), HttpStatus.OK); |
|
48 | 40 |
} |
49 | 41 |
|
50 | 42 |
/** |
... | ... | @@ -53,9 +45,9 @@ |
53 | 45 |
* |
54 | 46 |
* 로드맵 순서 수정 |
55 | 47 |
*/ |
56 |
- @PutMapping("/update.json") |
|
57 |
- public void updateUnitLearning(@RequestBody UnitLearningVO unitLearningVO) { |
|
58 |
- unitLearningServiceImpl.updateUnitLearning(unitLearningVO); |
|
48 |
+ @PostMapping("/update.json") |
|
49 |
+ public ResponseEntity<?> updateUnitLearning(@RequestBody UnitLearningVO unitLearningVO) { |
|
50 |
+ return new ResponseEntity<>(unitLearningServiceImpl.updateUnitLearning(unitLearningVO), HttpStatus.OK); |
|
59 | 51 |
} |
60 | 52 |
|
61 | 53 |
/** |
... | ... | @@ -64,8 +56,9 @@ |
64 | 56 |
* |
65 | 57 |
* 로드맵 삭제 |
66 | 58 |
*/ |
67 |
- @DeleteMapping("/delete.json") |
|
68 |
- public void deleteUnitLearning(@RequestBody UnitLearningVO unitLearningVO) { |
|
69 |
- unitLearningServiceImpl.deleteUnitLearning(unitLearningVO.getSeq()); |
|
59 |
+ @PostMapping("/delete.json") |
|
60 |
+ public ResponseEntity<?> deleteUnitLearning(@RequestBody UnitLearningVO unitLearningVO) { |
|
61 |
+ return new ResponseEntity<>(unitLearningServiceImpl.deleteUnitLearning(unitLearningVO.getUnit_id()), HttpStatus.OK); |
|
70 | 62 |
} |
63 |
+ |
|
71 | 64 |
} |
--- src/main/resources/mybatis/mapper/lms/book-SQL.xml
+++ src/main/resources/mybatis/mapper/lms/book-SQL.xml
... | ... | @@ -38,8 +38,9 @@ |
38 | 38 |
내 용 : 교재 등록 |
39 | 39 |
--> |
40 | 40 |
<insert id="insertBook" parameterType="bookVO"> |
41 |
- INSERT INTO book (book_id, book_nm) |
|
42 |
- VALUES (#{book_id}, #{book_nm}) |
|
41 |
+ INSERT |
|
42 |
+ INTO book (book_id, book_nm) |
|
43 |
+ VALUES (#{book_id}, #{book_nm}) |
|
43 | 44 |
</insert> |
44 | 45 |
|
45 | 46 |
<!-- |
... | ... | @@ -59,7 +60,9 @@ |
59 | 60 |
내 용 : 교재 삭제 |
60 | 61 |
--> |
61 | 62 |
<delete id="deleteBook" parameterType="String"> |
62 |
- DELETE FROM book |
|
63 |
+ DELETE |
|
64 |
+ FROM book |
|
63 | 65 |
WHERE book_id = #{book_id} |
64 | 66 |
</delete> |
67 |
+ |
|
65 | 68 |
</mapper>(파일 끝에 줄바꿈 문자 없음) |
--- src/main/resources/mybatis/mapper/lms/unit_learning-SQL.xml
+++ src/main/resources/mybatis/mapper/lms/unit_learning-SQL.xml
... | ... | @@ -14,60 +14,37 @@ |
14 | 14 |
<!-- |
15 | 15 |
작 성 자 : 구자현 |
16 | 16 |
작 성 일 : 2024.07.26 |
17 |
- 내 용 : 전체 로드맵 목록 출력 |
|
18 |
- --> |
|
19 |
- <select id="getAllUnitLearning" resultMap="unitLearningMap"> |
|
20 |
- SELECT |
|
21 |
- unit_id, |
|
22 |
- prblm_id, |
|
23 |
- wd_book_id, |
|
24 |
- text_id, |
|
25 |
- eval_id, |
|
26 |
- seq |
|
27 |
- FROM unit_learning |
|
28 |
- </select> |
|
29 |
- |
|
30 |
- <!-- |
|
31 |
- 작 성 자 : 구자현 |
|
32 |
- 작 성 일 : 2024.07.26 |
|
33 | 17 |
내 용 : 로드맵의 상세 정보 |
34 | 18 |
--> |
35 |
- <select id="getUnitLearningBySeq" parameterType="int" resultMap="unitLearningMap"> |
|
19 |
+ <select id="getUnitLearning" parameterType="String" resultMap="unitLearningMap"> |
|
36 | 20 |
SELECT |
37 |
- unit_id, |
|
38 |
- prblm_id, |
|
39 |
- wd_book_id, |
|
40 |
- text_id, |
|
41 |
- eval_id, |
|
42 |
- seq |
|
43 |
- FROM unit_learning |
|
44 |
- WHERE seq = #{seq} |
|
21 |
+ ul.unit_id, |
|
22 |
+ ul.prblm_id, |
|
23 |
+ ul.wd_book_id, |
|
24 |
+ ul.text_id, |
|
25 |
+ ul.eval_id, |
|
26 |
+ ul.seq |
|
27 |
+ FROM unit_learning ul |
|
28 |
+ JOIN unit u |
|
29 |
+ ON ul.unit_id = u.unit_id |
|
30 |
+ WHERE ul.unit_id = #{unit_id} AND u.book_id = #{book_id} |
|
45 | 31 |
</select> |
46 | 32 |
|
47 | 33 |
<!-- |
48 | 34 |
작 성 자 : 구자현 |
49 | 35 |
작 성 일 : 2024.07.26 |
50 | 36 |
내 용 : 로드맵 등록 |
37 |
+ |
|
51 | 38 |
--> |
52 |
- <insert id="insertUnitLearning" parameterType="unitLearningVO"> |
|
53 |
- INSERT INTO unit_learning |
|
54 |
- ( |
|
55 |
- unit_id, |
|
56 |
- prblm_id, |
|
57 |
- wd_book_id, |
|
58 |
- text_id, |
|
59 |
- eval_id, |
|
60 |
- seq |
|
61 |
- ) |
|
62 |
- VALUES |
|
63 |
- ( |
|
64 |
- #{unit_id}, |
|
65 |
- #{prblm_id}, |
|
66 |
- #{wd_book_id}, |
|
67 |
- #{text_id}, |
|
68 |
- #{eval_id}, |
|
69 |
- #{seq} |
|
70 |
- ) |
|
39 |
+ <insert id="insertUnitLearning" parameterType="list"> |
|
40 |
+ INSERT |
|
41 |
+ INTO |
|
42 |
+ unit_learning |
|
43 |
+ (unit_id, prblm_id, wd_book_id, text_id, eval_id, seq) |
|
44 |
+ VALUES |
|
45 |
+ <foreach collection="list" item="item" separator=","> |
|
46 |
+ (#{item.unit_id}, #{item.prblm_id}, #{item.wd_book_id}, #{item.text_id}, #{item.eval_id}, #{item.seq}) |
|
47 |
+ </foreach> |
|
71 | 48 |
</insert> |
72 | 49 |
|
73 | 50 |
<!-- |
... | ... | @@ -75,14 +52,22 @@ |
75 | 52 |
작 성 일 : 2024.07.26 |
76 | 53 |
내 용 : 로드맵 수정 |
77 | 54 |
--> |
78 |
- <update id="updateUnitLearning" parameterType="unitLearningVO"> |
|
55 |
+ <update id="updateUnitLearning" parameterType="unitLearningVO"> |
|
79 | 56 |
UPDATE unit_learning |
80 | 57 |
SET seq = #{seq} |
81 | 58 |
WHERE unit_id = #{unit_id} |
82 |
- AND prblm_id = #{prblm_id} |
|
83 |
- AND wd_book_id = #{wd_book_id} |
|
84 |
- AND text_id = #{text_id} |
|
85 |
- AND eval_id = #{eval_id} |
|
59 |
+ <if test="prblm_id != null"> |
|
60 |
+ AND prblm_id = #{prblm_id} |
|
61 |
+ </if> |
|
62 |
+ <if test="wd_book_id != null"> |
|
63 |
+ AND wd_book_id = #{wd_book_id} |
|
64 |
+ </if> |
|
65 |
+ <if test="text_id != null"> |
|
66 |
+ AND text_id = #{text_id} |
|
67 |
+ </if> |
|
68 |
+ <if test="eval_id != null"> |
|
69 |
+ AND eval_id = #{eval_id} |
|
70 |
+ </if> |
|
86 | 71 |
</update> |
87 | 72 |
|
88 | 73 |
<!-- |
... | ... | @@ -90,8 +75,10 @@ |
90 | 75 |
작 성 일 : 2024.07.26 |
91 | 76 |
내 용 : 로드맵 삭제 |
92 | 77 |
--> |
93 |
- <delete id="deleteUnitLearning" parameterType="int"> |
|
94 |
- DELETE FROM unit_learning |
|
95 |
- WHERE seq = #{seq} |
|
78 |
+ <delete id="deleteUnitLearning" parameterType="unitLearningVO"> |
|
79 |
+ DELETE |
|
80 |
+ FROM unit_learning |
|
81 |
+ WHERE unit_id = #{unit_id} |
|
96 | 82 |
</delete> |
83 |
+ |
|
97 | 84 |
</mapper>(파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?