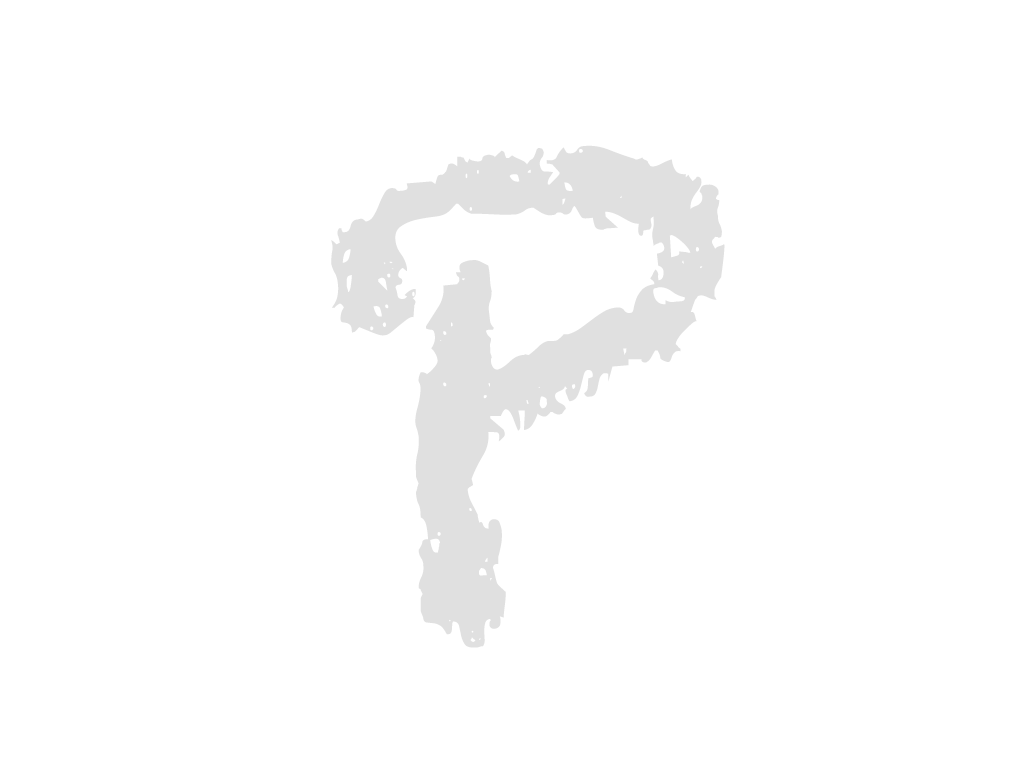
--- src/main/java/com/takensoft/ai_lms/lms/book/service/BookService.java
+++ src/main/java/com/takensoft/ai_lms/lms/book/service/BookService.java
... | ... | @@ -33,7 +33,7 @@ |
33 | 33 |
* |
34 | 34 |
* 교재 등록 |
35 | 35 |
*/ |
36 |
- int insertBook(BookVO book); |
|
36 |
+ int insertBook(BookVO bookVO); |
|
37 | 37 |
|
38 | 38 |
/** |
39 | 39 |
* @author : 구자현 |
... | ... | @@ -41,7 +41,7 @@ |
41 | 41 |
* |
42 | 42 |
* 교재 제목 수정 |
43 | 43 |
*/ |
44 |
- int updateBook(BookVO book); |
|
44 |
+ int updateBook(BookVO bookVO); |
|
45 | 45 |
|
46 | 46 |
/** |
47 | 47 |
* @author : 구자현 |
--- src/main/java/com/takensoft/ai_lms/lms/book/service/Impl/BookServiceImpl.java
+++ src/main/java/com/takensoft/ai_lms/lms/book/service/Impl/BookServiceImpl.java
... | ... | @@ -52,10 +52,11 @@ |
52 | 52 |
* 교재 등록 |
53 | 53 |
*/ |
54 | 54 |
@Override |
55 |
- public int insertBook(BookVO book) { |
|
55 |
+ public int insertBook(BookVO bookVO) { |
|
56 | 56 |
String book_id = bookIdgn.getNextStringId(); |
57 |
- book.setBook_id(book_id); |
|
58 |
- return bookDAO.insertBook(book); |
|
57 |
+ bookVO.setBook_id(book_id); |
|
58 |
+ return bookDAO.insertBook(bookVO); |
|
59 |
+ |
|
59 | 60 |
} |
60 | 61 |
|
61 | 62 |
/** |
... | ... | @@ -65,8 +66,8 @@ |
65 | 66 |
* 교재 제목 수정 |
66 | 67 |
*/ |
67 | 68 |
@Override |
68 |
- public int updateBook(BookVO book) { |
|
69 |
- return bookDAO.updateBook(book); |
|
69 |
+ public int updateBook(BookVO bookVO) { |
|
70 |
+ return bookDAO.updateBook(bookVO); |
|
70 | 71 |
} |
71 | 72 |
|
72 | 73 |
/** |
--- src/main/java/com/takensoft/ai_lms/lms/book/web/BookController.java
+++ src/main/java/com/takensoft/ai_lms/lms/book/web/BookController.java
... | ... | @@ -49,8 +49,8 @@ |
49 | 49 |
* 교재 등록 |
50 | 50 |
*/ |
51 | 51 |
@PostMapping("/insert.json") |
52 |
- public ResponseEntity<?> insertBook(@RequestBody BookVO book) { |
|
53 |
- return new ResponseEntity<>(bookServiceImpl.insertBook(book), HttpStatus.OK); |
|
52 |
+ public ResponseEntity<?> insertBook(@RequestBody BookVO bookVO) { |
|
53 |
+ return new ResponseEntity<>(bookServiceImpl.insertBook(bookVO), HttpStatus.OK); |
|
54 | 54 |
} |
55 | 55 |
|
56 | 56 |
/** |
... | ... | @@ -60,8 +60,8 @@ |
60 | 60 |
* 교재 제목 수정 |
61 | 61 |
*/ |
62 | 62 |
@PostMapping("/update.json") |
63 |
- public ResponseEntity<?> updateBook(@RequestBody BookVO book) { |
|
64 |
- return new ResponseEntity<>(bookServiceImpl.updateBook(book), HttpStatus.OK); |
|
63 |
+ public ResponseEntity<?> updateBook(@RequestBody BookVO bookVO) { |
|
64 |
+ return new ResponseEntity<>(bookServiceImpl.updateBook(bookVO), HttpStatus.OK); |
|
65 | 65 |
} |
66 | 66 |
|
67 | 67 |
/** |
--- src/main/java/com/takensoft/ai_lms/lms/unit_learning/service/Impl/UnitLearningServiceImpl.java
+++ src/main/java/com/takensoft/ai_lms/lms/unit_learning/service/Impl/UnitLearningServiceImpl.java
... | ... | @@ -1,5 +1,6 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.unit_learning.service.Impl; |
2 | 2 |
|
3 |
+import com.takensoft.ai_lms.common.idgen.service.IdgenService; |
|
3 | 4 |
import com.takensoft.ai_lms.lms.unit_learning.dao.UnitLearningDAO; |
4 | 5 |
import com.takensoft.ai_lms.lms.unit_learning.service.UnitLearningService; |
5 | 6 |
import com.takensoft.ai_lms.lms.unit_learning.vo.UnitLearningVO; |
... | ... | @@ -19,6 +20,8 @@ |
19 | 20 |
|
20 | 21 |
private final UnitLearningDAO unitLearningDAO; |
21 | 22 |
|
23 |
+ private final IdgenService unitLearningIdgn; |
|
24 |
+ |
|
22 | 25 |
/** |
23 | 26 |
* @author : 구자현 |
24 | 27 |
* @since : 2024.07.26 |
... | ... | @@ -37,8 +40,12 @@ |
37 | 40 |
* 로드맵 등록 |
38 | 41 |
*/ |
39 | 42 |
@Override |
40 |
- public int insertUnitLearning(List<UnitLearningVO> unitLearningVO) { |
|
41 |
- return unitLearningDAO.insertUnitLearning(unitLearningVO); |
|
43 |
+ public int insertUnitLearning(List<UnitLearningVO> unitLearningVOList) { |
|
44 |
+ for (UnitLearningVO unitLearningVO : unitLearningVOList) { |
|
45 |
+ String learning_id = unitLearningIdgn.getNextStringId(); |
|
46 |
+ unitLearningVO.setLearning_id(learning_id); |
|
47 |
+ } |
|
48 |
+ return unitLearningDAO.insertUnitLearning(unitLearningVOList); |
|
42 | 49 |
} |
43 | 50 |
|
44 | 51 |
/** |
--- src/main/java/com/takensoft/ai_lms/lms/unit_learning/vo/UnitLearningVO.java
+++ src/main/java/com/takensoft/ai_lms/lms/unit_learning/vo/UnitLearningVO.java
... | ... | @@ -16,6 +16,9 @@ |
16 | 16 |
@NoArgsConstructor |
17 | 17 |
@AllArgsConstructor |
18 | 18 |
public class UnitLearningVO { |
19 |
+ // 학습 로드맵 아이디 |
|
20 |
+ private String learning_id; |
|
21 |
+ |
|
19 | 22 |
// 단원 아이디 |
20 | 23 |
private String unit_id; |
21 | 24 |
|
+++ src/main/java/com/takensoft/ai_lms/lms/user_learning/dao/UserLearningDAO.java
... | ... | @@ -0,0 +1,39 @@ |
1 | +package com.takensoft.ai_lms.lms.user_learning.dao; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.user_learning.vo.UserLearningVO; | |
4 | +import org.egovframe.rte.psl.dataaccess.mapper.Mapper; | |
5 | + | |
6 | +/** | |
7 | + * @author : 구자현 | |
8 | + * @since : 2024.08.05 | |
9 | + * | |
10 | + * 로드맵 상태 관련 Mapper | |
11 | + */ | |
12 | +@Mapper("userLearningDAO") | |
13 | +public interface UserLearningDAO { | |
14 | + | |
15 | + /** | |
16 | + * @author : 구자현 | |
17 | + * @since : 2024.08.05 | |
18 | + * | |
19 | + * 로드맵의 상태 정보 | |
20 | + */ | |
21 | + UserLearningVO getUserLearningStatus(String learning_id); | |
22 | + | |
23 | + /** | |
24 | + * @author : 구자현 | |
25 | + * @since : 2024.08.05 | |
26 | + * | |
27 | + * 로드맵 상태 추가 | |
28 | + */ | |
29 | + int insertUserLearning(UserLearningVO userLearningVO); | |
30 | + | |
31 | + /** | |
32 | + * @author : 구자현 | |
33 | + * @since : 2024.08.05 | |
34 | + * | |
35 | + * 로드맵 상태 변경 | |
36 | + */ | |
37 | + int updateUserLearning(UserLearningVO userLearningVO); | |
38 | + | |
39 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/user_learning/service/Impl/UserLearningServiceImpl.java
... | ... | @@ -0,0 +1,54 @@ |
1 | +package com.takensoft.ai_lms.lms.user_learning.service.Impl; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.user_learning.dao.UserLearningDAO; | |
4 | +import com.takensoft.ai_lms.lms.user_learning.service.UserLearningService; | |
5 | +import com.takensoft.ai_lms.lms.user_learning.vo.UserLearningVO; | |
6 | +import lombok.RequiredArgsConstructor; | |
7 | +import org.springframework.stereotype.Service; | |
8 | + | |
9 | +/** | |
10 | + * @author : 구자현 | |
11 | + * @since : 2024.08.05 | |
12 | + * | |
13 | + * 로드맵 상태 관련 ServiceImpl 클래스 | |
14 | + */ | |
15 | +@Service | |
16 | +@RequiredArgsConstructor | |
17 | +public class UserLearningServiceImpl implements UserLearningService { | |
18 | + | |
19 | + private final UserLearningDAO userLearningDAO; | |
20 | + | |
21 | + /** | |
22 | + * @author : 구자현 | |
23 | + * @since : 2024.08.05 | |
24 | + * | |
25 | + * 로드맵의 상태 정보 | |
26 | + */ | |
27 | + @Override | |
28 | + public UserLearningVO getUserLearningStatus(String learning_id) { | |
29 | + return userLearningDAO.getUserLearningStatus(learning_id); | |
30 | + }; | |
31 | + | |
32 | + /** | |
33 | + * @author : 구자현 | |
34 | + * @since : 2024.08.05 | |
35 | + * | |
36 | + * 로드맵 상태 추가 | |
37 | + */ | |
38 | + @Override | |
39 | + public int insertUserLearning(UserLearningVO userLearningVO) { | |
40 | + return userLearningDAO.insertUserLearning(userLearningVO); | |
41 | + }; | |
42 | + | |
43 | + /** | |
44 | + * @author : 구자현 | |
45 | + * @since : 2024.08.05 | |
46 | + * | |
47 | + * 로드맵 상태 변경 | |
48 | + */ | |
49 | + @Override | |
50 | + public int updateUserLearning(UserLearningVO userLearningVO) { | |
51 | + return userLearningDAO.updateUserLearning(userLearningVO); | |
52 | + }; | |
53 | + | |
54 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/user_learning/service/UserLearningService.java
... | ... | @@ -0,0 +1,37 @@ |
1 | +package com.takensoft.ai_lms.lms.user_learning.service; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.user_learning.vo.UserLearningVO; | |
4 | + | |
5 | +/** | |
6 | + * @author : 구자현 | |
7 | + * @since : 2024.08.05 | |
8 | + * | |
9 | + * 로드맵 상태 관련 Service 클래스 | |
10 | + */ | |
11 | +public interface UserLearningService { | |
12 | + | |
13 | + /** | |
14 | + * @author : 구자현 | |
15 | + * @since : 2024.08.05 | |
16 | + * | |
17 | + * 로드맵의 상태 정보 | |
18 | + */ | |
19 | + UserLearningVO getUserLearningStatus(String learning_id); | |
20 | + | |
21 | + /** | |
22 | + * @author : 구자현 | |
23 | + * @since : 2024.08.05 | |
24 | + * | |
25 | + * 로드맵 상태 추가 | |
26 | + */ | |
27 | + int insertUserLearning(UserLearningVO userLearningVO); | |
28 | + | |
29 | + /** | |
30 | + * @author : 구자현 | |
31 | + * @since : 2024.08.05 | |
32 | + * | |
33 | + * 로드맵 상태 변경 | |
34 | + */ | |
35 | + int updateUserLearning(UserLearningVO userLearningVO); | |
36 | + | |
37 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/user_learning/vo/UserLearningVO.java
... | ... | @@ -0,0 +1,26 @@ |
1 | +package com.takensoft.ai_lms.lms.user_learning.vo; | |
2 | + | |
3 | +import lombok.AllArgsConstructor; | |
4 | +import lombok.Getter; | |
5 | +import lombok.NoArgsConstructor; | |
6 | +import lombok.Setter; | |
7 | + | |
8 | +/** | |
9 | + * @author : 구자현 | |
10 | + * @since : 2024.08.05 | |
11 | + * | |
12 | + * 로드맵 상태 관련 VO | |
13 | + */ | |
14 | +@Setter | |
15 | +@Getter | |
16 | +@NoArgsConstructor | |
17 | +@AllArgsConstructor | |
18 | +public class UserLearningVO { | |
19 | + | |
20 | + // 학습 로드맵 아이디 | |
21 | + private String learning_id; | |
22 | + // 학생 아이디 | |
23 | + private String std_id; | |
24 | + // 완료 여부 | |
25 | + private String learning_yn; | |
26 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/user_learning/web/UserLearningController.java
... | ... | @@ -0,0 +1,59 @@ |
1 | +package com.takensoft.ai_lms.lms.user_learning.web; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.user_learning.service.Impl.UserLearningServiceImpl; | |
4 | +import com.takensoft.ai_lms.lms.user_learning.vo.UserLearningVO; | |
5 | +import lombok.RequiredArgsConstructor; | |
6 | +import org.springframework.http.HttpStatus; | |
7 | +import org.springframework.http.ResponseEntity; | |
8 | +import org.springframework.web.bind.annotation.PostMapping; | |
9 | +import org.springframework.web.bind.annotation.RequestBody; | |
10 | +import org.springframework.web.bind.annotation.RequestMapping; | |
11 | +import org.springframework.web.bind.annotation.RestController; | |
12 | + | |
13 | +/** | |
14 | + * @author : 구자현 | |
15 | + * @since : 2024.08.05 | |
16 | + * | |
17 | + * 로드맵 상태 관련 Controller 클래스 | |
18 | + */ | |
19 | +@RequiredArgsConstructor | |
20 | +@RestController | |
21 | +@RequestMapping("/unitLearning/status") | |
22 | +public class UserLearningController { | |
23 | + | |
24 | + private final UserLearningServiceImpl userLearningServiceImpl; | |
25 | + | |
26 | + /** | |
27 | + * @author : 구자현 | |
28 | + * @since : 2024.08.05 | |
29 | + * | |
30 | + * 로드맵의 상태 정보 | |
31 | + */ | |
32 | + @PostMapping("/find.json") | |
33 | + public ResponseEntity<?> getUserLearningStatus(@RequestBody UserLearningVO userLearningVO) { | |
34 | + return new ResponseEntity<>(userLearningServiceImpl.getUserLearningStatus(userLearningVO.getLearning_id()), HttpStatus.OK); | |
35 | + }; | |
36 | + | |
37 | + /** | |
38 | + * @author : 구자현 | |
39 | + * @since : 2024.08.05 | |
40 | + * | |
41 | + * 로드맵 상태 추가 | |
42 | + */ | |
43 | + @PostMapping("/insert.json") | |
44 | + public ResponseEntity<?> insertUserLearning(@RequestBody UserLearningVO userLearningVO) { | |
45 | + return new ResponseEntity<>(userLearningServiceImpl.insertUserLearning(userLearningVO), HttpStatus.OK); | |
46 | + }; | |
47 | + | |
48 | + /** | |
49 | + * @author : 구자현 | |
50 | + * @since : 2024.08.05 | |
51 | + * | |
52 | + * 로드맵 상태 변경 | |
53 | + */ | |
54 | + @PostMapping("/update.json") | |
55 | + public ResponseEntity<?> updateUserLearning(@RequestBody UserLearningVO userLearningVO) { | |
56 | + return new ResponseEntity<>(userLearningServiceImpl.updateUserLearning(userLearningVO), HttpStatus.OK); | |
57 | + }; | |
58 | + | |
59 | +} |
--- src/main/resources/mybatis/mapper/lms/book-SQL.xml
+++ src/main/resources/mybatis/mapper/lms/book-SQL.xml
... | ... | @@ -40,7 +40,9 @@ |
40 | 40 |
--> |
41 | 41 |
<insert id="insertBook" parameterType="bookVO"> |
42 | 42 |
INSERT |
43 |
- INTO book (book_id, book_nm) |
|
43 |
+ INTO |
|
44 |
+ book |
|
45 |
+ (book_id, book_nm) |
|
44 | 46 |
VALUES (#{book_id}, #{book_nm}) |
45 | 47 |
</insert> |
46 | 48 |
|
--- src/main/resources/mybatis/mapper/lms/unit_learning-SQL.xml
+++ src/main/resources/mybatis/mapper/lms/unit_learning-SQL.xml
... | ... | @@ -3,6 +3,7 @@ |
3 | 3 |
<mapper namespace="com.takensoft.ai_lms.lms.unit_learning.dao.UnitLearningDAO"> |
4 | 4 |
|
5 | 5 |
<resultMap id="unitLearningMap" type="UnitLearningVO"> |
6 |
+ <result property="learning_id" column="learning_id"/> |
|
6 | 7 |
<result property="unit_id" column="unit_id"/> |
7 | 8 |
<result property="prblm_id" column="prblm_id"/> |
8 | 9 |
<result property="wd_book_id" column="wd_book_id"/> |
... | ... | @@ -18,6 +19,7 @@ |
18 | 19 |
--> |
19 | 20 |
<select id="getUnitLearning" parameterType="String" resultMap="unitLearningMap"> |
20 | 21 |
SELECT |
22 |
+ ul.learning_id, |
|
21 | 23 |
ul.unit_id, |
22 | 24 |
ul.prblm_id, |
23 | 25 |
ul.wd_book_id, |
... | ... | @@ -41,10 +43,10 @@ |
41 | 43 |
INSERT |
42 | 44 |
INTO |
43 | 45 |
unit_learning |
44 |
- (unit_id, prblm_id, wd_book_id, text_id, eval_id, seq) |
|
46 |
+ (learning_id, unit_id, prblm_id, wd_book_id, text_id, eval_id, seq) |
|
45 | 47 |
VALUES |
46 | 48 |
<foreach collection="list" item="item" separator=","> |
47 |
- (#{item.unit_id}, #{item.prblm_id}, #{item.wd_book_id}, #{item.text_id}, #{item.eval_id}, #{item.seq}) |
|
49 |
+ (#{item.learning_id}, #{item.unit_id}, #{item.prblm_id}, #{item.wd_book_id}, #{item.text_id}, #{item.eval_id}, #{item.seq}) |
|
48 | 50 |
</foreach> |
49 | 51 |
</insert> |
50 | 52 |
|
... | ... | @@ -53,7 +55,7 @@ |
53 | 55 |
작 성 일 : 2024.07.26 |
54 | 56 |
내 용 : 로드맵 수정 |
55 | 57 |
--> |
56 |
- <update id="updateUnitLearning" parameterType="unitLearningVO"> |
|
58 |
+ <update id="updateUnitLearning" parameterType="unitLearningVO"> |
|
57 | 59 |
UPDATE unit_learning |
58 | 60 |
SET seq = #{seq} |
59 | 61 |
WHERE unit_id = #{unit_id} |
+++ src/main/resources/mybatis/mapper/lms/user_learning-SQL.xml
... | ... | @@ -0,0 +1,49 @@ |
1 | +<?xml version="1.0" encoding="UTF-8"?> | |
2 | +<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> | |
3 | +<mapper namespace="com.takensoft.ai_lms.lms.user_learning.dao.UserLearningDAO"> | |
4 | + | |
5 | + <resultMap id="userLearningMap" type="UserLearningVO"> | |
6 | + <result property="learning_id" column="learning_id"/> | |
7 | + <result property="std_id" column="std_id"/> | |
8 | + <result property="learning_yn" column="learning_yn"/> | |
9 | + </resultMap> | |
10 | + | |
11 | + <!-- | |
12 | + 작 성 자 : 구자현 | |
13 | + 작 성 일 : 2024.08.05 | |
14 | + 내 용 : 로드맵의 상태 정보 | |
15 | + --> | |
16 | + <select id="getUserLearningStatus" parameterType="String" resultMap="userLearningMap"> | |
17 | + SELECT | |
18 | + learning_id, | |
19 | + std_id, | |
20 | + learning_yn | |
21 | + FROM user_learning | |
22 | + WHERE learning_id = #{learning_id} | |
23 | + </select> | |
24 | + | |
25 | + <!-- | |
26 | + 작 성 자 : 구자현 | |
27 | + 작 성 일 : 2024.08.05 | |
28 | + 내 용 : 로드맵 상태 추가 | |
29 | + --> | |
30 | + <insert id="insertUserLearning" parameterType="userLearningVO"> | |
31 | + INSERT | |
32 | + INTO | |
33 | + user_learning | |
34 | + (learning_id, std_id, learning_yn) | |
35 | + VALUES (#{learning_id}, #{std_id}, 'N') | |
36 | + </insert> | |
37 | + | |
38 | + <!-- | |
39 | + 작 성 자 : 구자현 | |
40 | + 작 성 일 : 2024.07.25 | |
41 | + 내 용 : 로드맵 상태 변경 | |
42 | + --> | |
43 | + <update id="updateUserLearning" parameterType="userLearningVO"> | |
44 | + UPDATE user_learning | |
45 | + SET learning_yn = #{learning_yn} | |
46 | + WHERE learning_id = #{learning_id} | |
47 | + </update> | |
48 | + | |
49 | +</mapper>(파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?