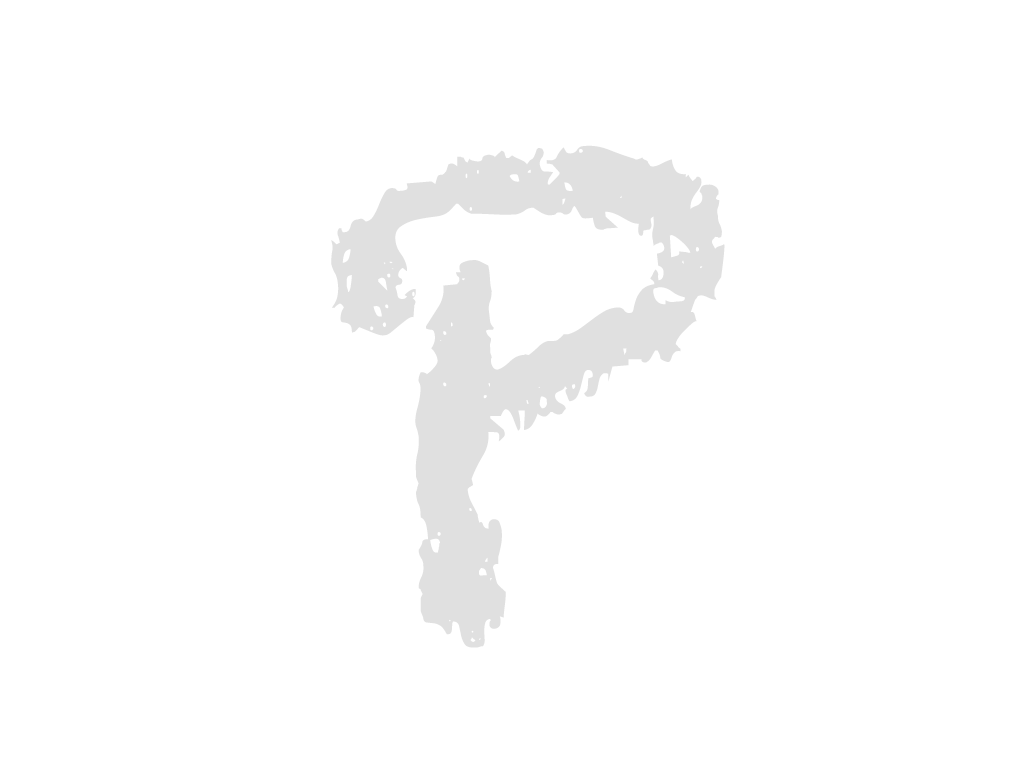
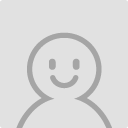
학생 정보 받아오고 학생 질문 수정하는 기능 추가
@8955dc479b7dc5e077c6c87d1f41ad603b1c7e3f
--- src/main/java/com/takensoft/ai_lms/common/confing/SecurityConfig.java
+++ src/main/java/com/takensoft/ai_lms/common/confing/SecurityConfig.java
... | ... | @@ -62,6 +62,7 @@ |
62 | 62 |
.requestMatchers( "/auth/**", "/auth/login.json").permitAll() // /user/** 경로는 모두 허용 |
63 | 63 |
.requestMatchers("/swagger-ui/**", "/v3/api-docs/**").permitAll() // swagger 진입 허용 |
64 | 64 |
.requestMatchers("/test/**").permitAll() |
65 |
+ .requestMatchers("/studentInfo/**").permitAll() // 학생 정보 진입 허용(민수) |
|
65 | 66 |
.anyRequest().authenticated()); // 나머지 경로는 인증 필요 |
66 | 67 |
|
67 | 68 |
// jwt 필터 처리 적용 |
+++ src/main/java/com/takensoft/ai_lms/lms/student/dao/StudentInfoDAO.java
... | ... | @@ -0,0 +1,30 @@ |
1 | +package com.takensoft.ai_lms.lms.student.dao; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.student.vo.StudentInfoVO; | |
4 | +import org.apache.ibatis.annotations.Param; | |
5 | +import org.egovframe.rte.psl.dataaccess.mapper.Mapper; | |
6 | + | |
7 | +/** | |
8 | + * @author 권민수 | |
9 | + * @since 2024.07.25 | |
10 | + * | |
11 | + * 학생 정보 DAO 클래스 | |
12 | + */ | |
13 | + | |
14 | +@Mapper("studentInfoDAO") | |
15 | +public interface StudentInfoDAO { | |
16 | + | |
17 | + // 학생 정보 불러오기 | |
18 | + StudentInfoVO getStudentInfo(@Param("userId") String userId); | |
19 | + | |
20 | + // 학생 질문 사항 수정하기 | |
21 | + void updateStudentQuestion(@Param("userId") String userId, @Param("studentQuestion") String studentQuestion); | |
22 | + | |
23 | +} | |
24 | + | |
25 | +// users(user_id) - user_nm(사용자 이름), | |
26 | +// users(user_id) -> institution(ednst_id) - ednst_nm(기관명), | |
27 | +// user_class(user_id) -> class(scls_id) -> grade(sgrd_id) - grd_no(학년), | |
28 | +// user_class(user_id) -> class(scls_id) - scls_nm(반 이름), | |
29 | +// user_class(user_id) - stn_qna(학생 질문), | |
30 | +// + 오늘의 공부, 최근 학습 히스토리(파일 끝에 줄바꿈 문자 없음) |
+++ src/main/java/com/takensoft/ai_lms/lms/student/dto/UpdateStudentQuestionDTO.java
... | ... | @@ -0,0 +1,18 @@ |
1 | +package com.takensoft.ai_lms.lms.student.dto; | |
2 | + | |
3 | +import lombok.Getter; | |
4 | +import lombok.Setter; | |
5 | + | |
6 | +/** | |
7 | + * @author 권민수 | |
8 | + * @since 2024.07.25 | |
9 | + * | |
10 | + * 학생 질문 수정용 DTO 클래스 | |
11 | + */ | |
12 | + | |
13 | +@Getter | |
14 | +@Setter | |
15 | +public class UpdateStudentQuestionDTO { | |
16 | + private String userId; | |
17 | + private String studentQuestion; | |
18 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/student/service/Impl/StudentInfoServiceImpl.java
... | ... | @@ -0,0 +1,31 @@ |
1 | +package com.takensoft.ai_lms.lms.student.service.Impl; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.student.dao.StudentInfoDAO; | |
4 | +import com.takensoft.ai_lms.lms.student.service.StudentInfoService; | |
5 | +import com.takensoft.ai_lms.lms.student.vo.StudentInfoVO; | |
6 | +import lombok.RequiredArgsConstructor; | |
7 | +import org.springframework.stereotype.Service; | |
8 | + | |
9 | +/** | |
10 | + * @author 권민수 | |
11 | + * @since 2024.07.25 | |
12 | + * | |
13 | + * 학생 정보 관련 서비스 | |
14 | + */ | |
15 | + | |
16 | +@Service | |
17 | +@RequiredArgsConstructor | |
18 | +public class StudentInfoServiceImpl implements StudentInfoService { | |
19 | + | |
20 | + private final StudentInfoDAO studentInfoDAO; | |
21 | + | |
22 | + @Override | |
23 | + public StudentInfoVO getStudentInfo(String userId) { | |
24 | + return studentInfoDAO.getStudentInfo(userId); | |
25 | + } | |
26 | + | |
27 | + @Override | |
28 | + public void updateStudentQuestion(String userId, String studentQuestion) { | |
29 | + studentInfoDAO.updateStudentQuestion(userId, studentQuestion); | |
30 | + } | |
31 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/student/service/StudentInfoService.java
... | ... | @@ -0,0 +1,20 @@ |
1 | +package com.takensoft.ai_lms.lms.student.service; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.student.vo.StudentInfoVO; | |
4 | + | |
5 | +/** | |
6 | + * @author 권민수 | |
7 | + * @since 2024.07.25 | |
8 | + * | |
9 | + * 학생 정보 관련 인터페이스 | |
10 | + */ | |
11 | + | |
12 | +public interface StudentInfoService { | |
13 | + | |
14 | + // 학생 정보 불러오기 | |
15 | + StudentInfoVO getStudentInfo(String userId); | |
16 | + | |
17 | + // 학생 질문 사항 수정하기 | |
18 | + void updateStudentQuestion(String userId, String studentQuestion); | |
19 | + | |
20 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/student/vo/StudentInfoVO.java
... | ... | @@ -0,0 +1,23 @@ |
1 | +package com.takensoft.ai_lms.lms.student.vo; | |
2 | + | |
3 | +import lombok.Getter; | |
4 | +import lombok.Setter; | |
5 | + | |
6 | +/** | |
7 | + * @author 권민수 | |
8 | + * @since 2024.07.25 | |
9 | + * | |
10 | + * 학생 불러오는 정보 VO 클래스 | |
11 | + */ | |
12 | + | |
13 | +@Setter | |
14 | +@Getter | |
15 | +public class StudentInfoVO { | |
16 | + private String studentName; | |
17 | + private String institutionName; | |
18 | + private String grade; | |
19 | + private String className; | |
20 | + private String studentQuestion; | |
21 | + | |
22 | +} | |
23 | + |
+++ src/main/java/com/takensoft/ai_lms/lms/student/web/StudentInfoController.java
... | ... | @@ -0,0 +1,37 @@ |
1 | +package com.takensoft.ai_lms.lms.student.web; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.student.dto.UpdateStudentQuestionDTO; | |
4 | +import com.takensoft.ai_lms.lms.student.service.StudentInfoService; | |
5 | +import com.takensoft.ai_lms.lms.student.vo.StudentInfoVO; | |
6 | +import lombok.RequiredArgsConstructor; | |
7 | +import lombok.extern.slf4j.Slf4j; | |
8 | +import org.springframework.web.bind.annotation.*; | |
9 | + | |
10 | +/** | |
11 | + * @author 권민수 | |
12 | + * @since 2024.07.25 | |
13 | + * | |
14 | + * 학생 정보 관련 컨트롤러 클래스 | |
15 | + */ | |
16 | + | |
17 | +@RestController | |
18 | +@RequiredArgsConstructor | |
19 | +@Slf4j | |
20 | +@RequestMapping("/studentInfo") | |
21 | +public class StudentInfoController { | |
22 | + | |
23 | + private final StudentInfoService studentInfoService; | |
24 | + | |
25 | + // 학생 정보 불러오는 api | |
26 | + @GetMapping("/student/{userId}") | |
27 | + public StudentInfoVO getStudentInfo(@PathVariable String userId) { | |
28 | + return studentInfoService.getStudentInfo(userId); | |
29 | + } | |
30 | + | |
31 | + // 학생 질문 사항 수정하는 api | |
32 | + @PostMapping("/student/updateQuestion") | |
33 | + public void updateStudentQuestion(@RequestBody UpdateStudentQuestionDTO request) { | |
34 | + studentInfoService.updateStudentQuestion(request.getUserId(), request.getStudentQuestion()); | |
35 | + } | |
36 | + | |
37 | +} |
+++ src/main/resources/mybatis/mapper/lms/student-SQL.xml
... | ... | @@ -0,0 +1,40 @@ |
1 | +<?xml version="1.0" encoding="UTF-8"?> | |
2 | +<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> | |
3 | +<mapper namespace="com.takensoft.ai_lms.lms.student.dao.StudentInfoDAO"> | |
4 | + | |
5 | + <!-- | |
6 | + 작 성 자 : 권민수 | |
7 | + 작 성 일 : 2024.07.25 | |
8 | + 내 용 : 학생 정보 관련 sql 매핑 xml 문서 | |
9 | + --> | |
10 | + | |
11 | + <resultMap id="StudentInfoResultMap" type="com.takensoft.ai_lms.lms.student.vo.StudentInfoVO"> | |
12 | + <result property="studentName" column="studentName"/> | |
13 | + <result property="institutionName" column="institutionName"/> | |
14 | + <result property="grade" column="grade"/> | |
15 | + <result property="className" column="className"/> | |
16 | + <result property="studentQuestion" column="studentQuestion"/> | |
17 | + </resultMap> | |
18 | + | |
19 | + <select id="getStudentInfo" resultType="com.takensoft.ai_lms.lms.student.vo.StudentInfoVO"> | |
20 | + SELECT | |
21 | + u.user_nm AS studentName, | |
22 | + i.ednst_nm AS institutionName, | |
23 | + g.grd_no AS grade, | |
24 | + c.scls_nm AS className, | |
25 | + uc.stn_qna AS studentQuestion | |
26 | + FROM users u | |
27 | + JOIN institution i ON u.ednst_id = i.ednst_id | |
28 | + JOIN user_class uc ON u.user_id = uc.user_id | |
29 | + JOIN class c ON uc.scls_id = c.scls_id | |
30 | + JOIN grade g ON c.sgrd_id = g.sgrd_id | |
31 | + WHERE u.user_id = #{userId} | |
32 | + </select> | |
33 | + | |
34 | + <update id="updateStudentQuestion"> | |
35 | + UPDATE user_class | |
36 | + SET stn_qna = #{studentQuestion} | |
37 | + WHERE user_id = #{userId} | |
38 | + </update> | |
39 | + | |
40 | +</mapper>(파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?