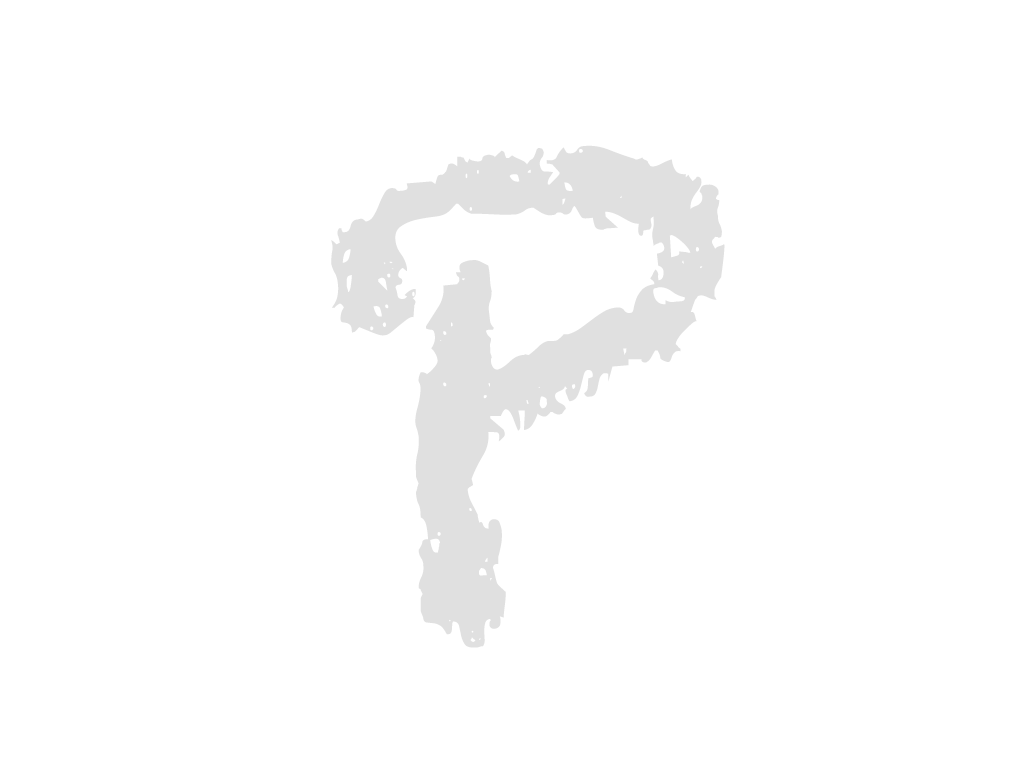
--- gradle/wrapper/gradle-wrapper.properties
+++ gradle/wrapper/gradle-wrapper.properties
... | ... | @@ -1,7 +1,6 @@ |
1 |
+#Wed Jul 24 13:48:54 KST 2024 |
|
2 |
+distributionUrl=https\://services.gradle.org/distributions/gradle-8.8-all.zip |
|
1 | 3 |
distributionBase=GRADLE_USER_HOME |
2 | 4 |
distributionPath=wrapper/dists |
3 |
-distributionUrl=https\://services.gradle.org/distributions/gradle-8.8-bin.zip |
|
4 |
-networkTimeout=10000 |
|
5 |
-validateDistributionUrl=true |
|
6 |
-zipStoreBase=GRADLE_USER_HOME |
|
7 | 5 |
zipStorePath=wrapper/dists |
6 |
+zipStoreBase=GRADLE_USER_HOME |
--- src/main/java/com/takensoft/ai_lms/common/confing/SecurityConfig.java
+++ src/main/java/com/takensoft/ai_lms/common/confing/SecurityConfig.java
... | ... | @@ -62,6 +62,7 @@ |
62 | 62 |
.requestMatchers( "/auth/**", "/auth/login.json").permitAll() // /user/** 경로는 모두 허용 |
63 | 63 |
.requestMatchers("/swagger-ui/**", "/v3/api-docs/**").permitAll() // swagger 진입 허용 |
64 | 64 |
.requestMatchers("/test/**").permitAll() |
65 |
+ .requestMatchers("/text/**").permitAll() |
|
65 | 66 |
.requestMatchers("/studentInfo/**").permitAll() // 학생 정보 진입 허용(민수) |
66 | 67 |
.requestMatchers("/board/**").permitAll() // 게시판 정보 진입 허용 |
67 | 68 |
.requestMatchers("/book/**").permitAll() // 교재 정보 진입 허용 |
+++ src/main/java/com/takensoft/ai_lms/lms/text/dao/TextDAO.java
... | ... | @@ -0,0 +1,34 @@ |
1 | +package com.takensoft.ai_lms.lms.text.dao; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.text.vo.TextVO; | |
4 | +import org.egovframe.rte.psl.dataaccess.mapper.Mapper; | |
5 | + | |
6 | +import java.util.HashMap; | |
7 | +import java.util.List; | |
8 | + | |
9 | +/** | |
10 | + * @author : 이은진 | |
11 | + * @since : 2024.07.25 | |
12 | + * | |
13 | + * 지문 관련 Mapper | |
14 | + */ | |
15 | +@Mapper("TextDAO") | |
16 | +public interface TextDAO { | |
17 | + // 지문 등록 | |
18 | + int insertText(TextVO TextVO) throws Exception; | |
19 | + | |
20 | + // 지문 리스트 조회 | |
21 | + List<HashMap<String, Object>> selectTextList(HashMap<String, Object> params) throws Exception; | |
22 | + | |
23 | + // 지문 수 조회 | |
24 | + int textCount(HashMap<String, Object> params) throws Exception; | |
25 | + | |
26 | + // 지문 출력 | |
27 | + List<HashMap<String, Object>> selectOneText(HashMap<String, Object> params) throws Exception; | |
28 | + | |
29 | + // 지문 수정 | |
30 | + int textUpdate(TextVO TextVO) throws Exception; | |
31 | + | |
32 | + // 지문 삭제 | |
33 | + int textDelete(String textId) throws Exception; | |
34 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/text/service/Impl/TextServiceImpl.java
... | ... | @@ -0,0 +1,83 @@ |
1 | +package com.takensoft.ai_lms.lms.text.service.Impl; | |
2 | + | |
3 | +import com.takensoft.ai_lms.common.idgen.service.IdgenService; | |
4 | +import com.takensoft.ai_lms.lms.text.dao.TextDAO; | |
5 | +import com.takensoft.ai_lms.lms.text.service.TextService; | |
6 | +import com.takensoft.ai_lms.lms.text.vo.TextVO; | |
7 | +import lombok.RequiredArgsConstructor; | |
8 | +import org.springframework.stereotype.Service; | |
9 | +import org.springframework.transaction.annotation.Transactional; | |
10 | +import org.springframework.web.servlet.ModelAndView; | |
11 | + | |
12 | +import java.util.HashMap; | |
13 | +import java.util.List; | |
14 | + | |
15 | +/** | |
16 | + * @author : 이은진 | |
17 | + * @since : 2024.07.25 | |
18 | + */ | |
19 | + | |
20 | +@Service("textService") | |
21 | +@RequiredArgsConstructor | |
22 | +public class TextServiceImpl implements TextService { | |
23 | + | |
24 | + private final TextDAO textDAO; | |
25 | + private final IdgenService textIdgn; | |
26 | + | |
27 | + /** | |
28 | + * 지문 등록 | |
29 | + */ | |
30 | + @Override | |
31 | + @Transactional | |
32 | + public int insertText(TextVO textVO) throws Exception { | |
33 | + String textID = textIdgn.getNextStringId(); | |
34 | + textVO.setTextId(textID); | |
35 | + return textDAO.insertText(textVO); | |
36 | + } | |
37 | + | |
38 | + /** | |
39 | + * 지문 리스트 조회 | |
40 | + */ | |
41 | + @Override | |
42 | + public List<HashMap<String, Object>> selectTextList(HashMap<String, Object> params) throws Exception { | |
43 | + int page = Integer.parseInt(params.get("page").toString()); | |
44 | + int pageSize = Integer.parseInt(params.get("pageSize").toString()); | |
45 | + | |
46 | + int startIndex = (page - 1) * pageSize; | |
47 | + params.put("startIndex", startIndex); | |
48 | + params.put("pageSize", pageSize); | |
49 | + return textDAO.selectTextList(params); | |
50 | + } | |
51 | + | |
52 | + /** | |
53 | + * 지문 수 조회 | |
54 | + */ | |
55 | + @Override | |
56 | + public int textCount(HashMap<String, Object> params) throws Exception { | |
57 | + return textDAO.textCount(params); | |
58 | + } | |
59 | + | |
60 | + /** | |
61 | + * 지문 출력 | |
62 | + */ | |
63 | + @Override | |
64 | + public List<HashMap<String, Object>> selectOneText(HashMap<String, Object> params) throws Exception { | |
65 | + return textDAO.selectOneText(params); | |
66 | + } | |
67 | + | |
68 | + /** | |
69 | + * 지문 수정 | |
70 | + */ | |
71 | + @Override | |
72 | + public int textUpdate(TextVO textVO) throws Exception { | |
73 | + return textDAO.textUpdate(textVO); | |
74 | + } | |
75 | + | |
76 | + /** | |
77 | + * 지문 삭제 | |
78 | + */ | |
79 | + @Override | |
80 | + public int textDelete(String textId) throws Exception { | |
81 | + return textDAO.textDelete(textId); | |
82 | + } | |
83 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/text/service/TextService.java
... | ... | @@ -0,0 +1,32 @@ |
1 | +package com.takensoft.ai_lms.lms.text.service; | |
2 | +import com.takensoft.ai_lms.lms.text.vo.TextVO; | |
3 | + | |
4 | +import java.util.HashMap; | |
5 | +import java.util.List; | |
6 | + | |
7 | +/** | |
8 | + * @author : 이은진 | |
9 | + * since : 2024.07.25 | |
10 | + * | |
11 | + * 지문 관련 인터페이스 | |
12 | + */ | |
13 | + | |
14 | +public interface TextService { | |
15 | + // 지문 등록 | |
16 | + int insertText(TextVO textVO) throws Exception; | |
17 | + | |
18 | + // 지문 리스트 조회 | |
19 | + List<HashMap<String, Object>> selectTextList(HashMap<String, Object> params) throws Exception; | |
20 | + | |
21 | + // 지문 수 조회 | |
22 | + int textCount(HashMap<String, Object> params) throws Exception; | |
23 | + | |
24 | + // 지문 출력 | |
25 | + List<HashMap<String, Object>> selectOneText(HashMap<String, Object> params) throws Exception; | |
26 | + | |
27 | + // 지문 수정 | |
28 | + int textUpdate(TextVO textVO) throws Exception; | |
29 | + | |
30 | + // 지문 삭제 | |
31 | + int textDelete(String textId) throws Exception; | |
32 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/text/vo/TextVO.java
... | ... | @@ -0,0 +1,34 @@ |
1 | +package com.takensoft.ai_lms.lms.text.vo; | |
2 | + | |
3 | +import lombok.AllArgsConstructor; | |
4 | +import lombok.Getter; | |
5 | +import lombok.NoArgsConstructor; | |
6 | +import lombok.Setter; | |
7 | + | |
8 | +import java.time.LocalDateTime; | |
9 | + | |
10 | +/** | |
11 | + * @author : 이은진 | |
12 | + * since : 2024.07.26 | |
13 | + * | |
14 | + * 지문 관련 VO | |
15 | + */ | |
16 | + | |
17 | +@Setter | |
18 | +@Getter | |
19 | +@NoArgsConstructor | |
20 | +@AllArgsConstructor | |
21 | +public class TextVO { | |
22 | + | |
23 | + // 지문 아이디 | |
24 | + private String textId; | |
25 | + // 지문 내용 | |
26 | + private String textCnt; | |
27 | + // 등록일 | |
28 | + private String regDt; | |
29 | + // 파일 관리 아이디 | |
30 | + private String fileMngId; | |
31 | + // 유형 아이디 | |
32 | + private String textTypeId; | |
33 | + | |
34 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/text/web/TextController.java
... | ... | @@ -0,0 +1,142 @@ |
1 | +package com.takensoft.ai_lms.lms.text.web; | |
2 | + | |
3 | +import com.google.gson.Gson; | |
4 | +import com.google.gson.JsonObject; | |
5 | +import com.takensoft.ai_lms.lms.text.service.TextService; | |
6 | +import com.takensoft.ai_lms.lms.text.vo.TextVO; | |
7 | +import io.swagger.v3.oas.annotations.Operation; | |
8 | +import lombok.RequiredArgsConstructor; | |
9 | +import lombok.extern.slf4j.Slf4j; | |
10 | +import org.springframework.http.HttpStatus; | |
11 | +import org.springframework.http.ResponseEntity; | |
12 | +import org.springframework.web.bind.annotation.*; | |
13 | +import org.springframework.web.servlet.ModelAndView; | |
14 | + | |
15 | +import java.util.HashMap; | |
16 | +import java.util.List; | |
17 | +import java.util.Map; | |
18 | + | |
19 | +/** | |
20 | + * @author 이은진 | |
21 | + * @since 2024.07.25 | |
22 | + */ | |
23 | + | |
24 | + | |
25 | +@RestController | |
26 | +@RequiredArgsConstructor | |
27 | +@Slf4j | |
28 | +@RequestMapping(value = "/text") | |
29 | +public class TextController { | |
30 | + | |
31 | + private final TextService TextService; | |
32 | + | |
33 | + /** | |
34 | + * 지문 등록 | |
35 | + */ | |
36 | + @PostMapping("/insertText.json") | |
37 | + @Operation(summary = "지문 등록") | |
38 | + public String insertText(@RequestBody TextVO textVO) throws Exception { | |
39 | + Gson gson = new Gson(); | |
40 | + JsonObject response = new JsonObject(); | |
41 | + | |
42 | + try { | |
43 | + int result = TextService.insertText(textVO); | |
44 | + if (result > 0) { | |
45 | + response.addProperty("status", "success"); | |
46 | + response.addProperty("message", "지문이 등록되었습니다."); | |
47 | + return gson.toJson(response); | |
48 | + } else { | |
49 | + response.addProperty("message", "지문 등록 실패"); | |
50 | + return gson.toJson(response); | |
51 | + } | |
52 | + } catch (Exception e) { | |
53 | + response.addProperty("status", "error"); | |
54 | + response.addProperty("message", e.getMessage()); | |
55 | + return gson.toJson(response); | |
56 | + } | |
57 | + } | |
58 | + | |
59 | + | |
60 | + /** | |
61 | + * 지문 리스트 조회 | |
62 | + */ | |
63 | + @GetMapping("/selectTextList.json") | |
64 | + @Operation(summary = "지문 리스트 조회") | |
65 | + public HashMap<String, Object> getTextList(@RequestBody HashMap<String, Object> params) throws Exception { | |
66 | + HashMap<String, Object> result = new HashMap<>(); | |
67 | + | |
68 | + int page = Integer.parseInt(params.get("page").toString()); | |
69 | + int pageSize = Integer.parseInt(params.get("pageSize").toString()); | |
70 | + | |
71 | + int totalText = TextService.textCount(params); | |
72 | + result.put("totalText", totalText); | |
73 | + result.put("texts", TextService.selectTextList(params)); | |
74 | + | |
75 | + return result; | |
76 | + } | |
77 | + | |
78 | + /** | |
79 | + * 지문 출력 | |
80 | + */ | |
81 | + @GetMapping("/selectOneText.json") | |
82 | + @Operation(summary = "지문 출력") | |
83 | + public List<HashMap<String, Object>> selectOneText(@RequestParam HashMap<String, Object> textId) throws Exception { | |
84 | + return TextService.selectOneText(textId); | |
85 | + } | |
86 | + | |
87 | + | |
88 | + /** | |
89 | + * 지문 수정 | |
90 | + */ | |
91 | + @PutMapping(value = "/textUpdate.json") | |
92 | + @Operation(summary = "지문 수정") | |
93 | + public String textUpdate(@RequestBody TextVO textVO) throws Exception { | |
94 | + Gson gson = new Gson(); | |
95 | + JsonObject response = new JsonObject(); | |
96 | + | |
97 | + try { | |
98 | + int result = TextService.textUpdate(textVO); | |
99 | + if (result > 0) { | |
100 | + response.addProperty("status", "success"); | |
101 | + response.addProperty("message", "지문이 수정되었습니다."); | |
102 | + return gson.toJson(response); | |
103 | + } else { | |
104 | + response.addProperty("message", "수정 실패"); | |
105 | + return gson.toJson(response); | |
106 | + } | |
107 | + } catch (Exception e) { | |
108 | + response.addProperty("status", "error"); | |
109 | + response.addProperty("message", e.getMessage()); | |
110 | + return gson.toJson(response); | |
111 | + } | |
112 | + } | |
113 | + | |
114 | + | |
115 | + /** | |
116 | + * 지문 삭제 | |
117 | + */ | |
118 | + @DeleteMapping(value = "/textDelete.json") | |
119 | + @Operation(summary = "지문 삭제") | |
120 | + public String textDelete(@RequestBody Map<String, String> request) throws Exception { | |
121 | + Gson gson = new Gson(); | |
122 | + JsonObject response = new JsonObject(); | |
123 | + | |
124 | + String textId = request.get("textId"); | |
125 | + int result = TextService.textDelete(textId); | |
126 | + try { | |
127 | + if (result > 0) { | |
128 | + response.addProperty("status", "success"); | |
129 | + response.addProperty("message", "사용자가 삭제되었습니다."); | |
130 | + return gson.toJson(response); | |
131 | + } else { | |
132 | + response.addProperty("message", "삭제 실패"); | |
133 | + return gson.toJson(response); | |
134 | + } | |
135 | + } catch (Exception e) { | |
136 | + response.addProperty("status", "error"); | |
137 | + response.addProperty("message", e.getMessage()); | |
138 | + return gson.toJson(response); | |
139 | + } | |
140 | + } | |
141 | +} | |
142 | + |
+++ src/main/resources/mybatis/mapper/lms/text-SQL.xml
... | ... | @@ -0,0 +1,100 @@ |
1 | +<?xml version="1.0" encoding="UTF-8"?> | |
2 | +<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> | |
3 | +<mapper namespace="com.takensoft.ai_lms.lms.text.dao.TextDAO"> | |
4 | + | |
5 | + <resultMap id="textMap" type="TextVO"> | |
6 | + <result property="textId" column="text_id"/> | |
7 | + <result property="textCnt" column="text_cnt"/> | |
8 | + <result property="regDt" column="reg_dt"/> | |
9 | + <result property="fileMngId" column="file_mng_id"/> | |
10 | + <result property="textTypeId" column="text_type_id"/> | |
11 | + </resultMap> | |
12 | + | |
13 | + | |
14 | + <!-- | |
15 | + 작성자 : 이은진 | |
16 | + 작성일 : 2024.07.26 | |
17 | + 내 용 : 지문 등록 | |
18 | + --> | |
19 | + <insert id="insertText" parameterType="TextVO"> | |
20 | + INSERT INTO text (text_id | |
21 | + ,text_cnt | |
22 | + ,reg_dt | |
23 | + ,file_mng_id | |
24 | + ,text_type_id | |
25 | + ) VALUES (#{textId} | |
26 | + ,#{textCnt} | |
27 | + ,now() | |
28 | + ,#{fileMngId} | |
29 | + ,#{textTypeId} | |
30 | + ); | |
31 | + </insert> | |
32 | + | |
33 | + <!-- | |
34 | + 작성자 : 이은진 | |
35 | + 작성일 : 2024.07.26 | |
36 | + 내 용 : 지문 수 조회 | |
37 | + --> | |
38 | + <select id="textCount" resultType="Integer"> | |
39 | + SELECT COUNT(*) | |
40 | + FROM text | |
41 | + </select> | |
42 | + | |
43 | + <!-- | |
44 | + 작성자 : 이은진 | |
45 | + 작성일 : 2024.07.26 | |
46 | + 내 용 : 지문 리스트 조회 | |
47 | + --> | |
48 | + <select id="selectTextList" resultMap="textMap"> | |
49 | + SELECT text_id | |
50 | + ,text_cnt | |
51 | + ,reg_dt | |
52 | + ,file_mng_id | |
53 | + ,text_type_id | |
54 | + FROM text | |
55 | + ORDER BY text_id DESC | |
56 | + LIMIT #{pageSize} | |
57 | + OFFSET #{startIndex} | |
58 | + </select> | |
59 | + | |
60 | + <!-- | |
61 | + 작성자 : 이은진 | |
62 | + 작성일 : 2024.07.26 | |
63 | + 내 용 : 지문 출력 | |
64 | + --> | |
65 | + <select id="selectOneText" parameterType="HashMap" resultType="HashMap"> | |
66 | + SELECT text_id | |
67 | + , text_cnt | |
68 | + , reg_dt | |
69 | + , file_mng_id | |
70 | + , text_type_id | |
71 | + | |
72 | + FROM text | |
73 | + WHERE text_id = #{textId} | |
74 | + ORDER BY text_id DESC | |
75 | + </select> | |
76 | + | |
77 | + <!-- | |
78 | + 작성자 : 이은진 | |
79 | + 작성일 : 2024.07.26 | |
80 | + 내 용 : 지문 수정 | |
81 | + --> | |
82 | + <update id="textUpdate" parameterType="TextVO"> | |
83 | + UPDATE text | |
84 | + SET text_cnt = #{textCnt} | |
85 | + , file_mng_id = #{fileMngId} | |
86 | + , text_type_id = #{textTypeId} | |
87 | + WHERE text_id = #{textId} | |
88 | + </update> | |
89 | + | |
90 | + <!-- | |
91 | + 작성자 : 이은진 | |
92 | + 작성일 : 2024.07.26 | |
93 | + 내 용 : 지문 삭제 | |
94 | + --> | |
95 | + <delete id="textDelete" parameterType="String"> | |
96 | + DELETE FROM text | |
97 | + WHERE text_id = #{textId} | |
98 | + </delete> | |
99 | + | |
100 | +</mapper> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?