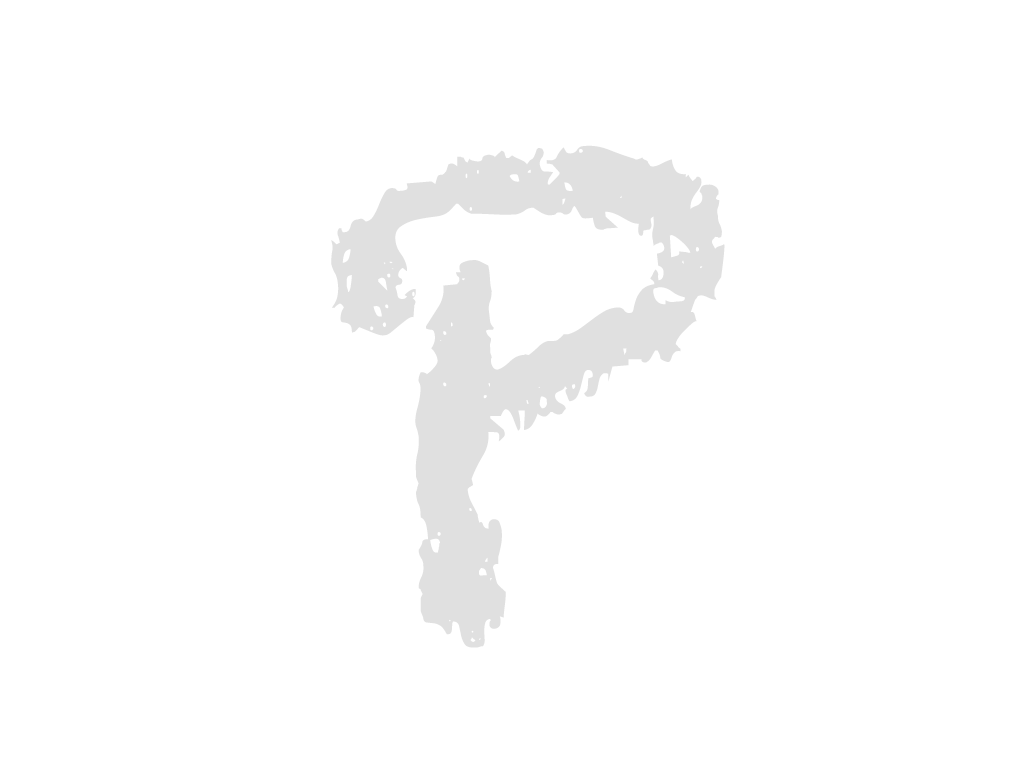
--- src/main/java/com/takensoft/ai_lms/common/confing/SecurityConfig.java
+++ src/main/java/com/takensoft/ai_lms/common/confing/SecurityConfig.java
... | ... | @@ -62,7 +62,8 @@ |
62 | 62 |
.requestMatchers( "/auth/**", "/auth/login.json").permitAll() // /user/** 경로는 모두 허용 |
63 | 63 |
.requestMatchers("/swagger-ui/**", "/v3/api-docs/**").permitAll() // swagger 진입 허용 |
64 | 64 |
.requestMatchers("/test/**").permitAll() |
65 |
- .requestMatchers("/text/**").permitAll() |
|
65 |
+ .requestMatchers("/text/**").permitAll() // 지문 정보 진입 허용 |
|
66 |
+ .requestMatchers("/schedule/**").permitAll() // 학습일정 정보 진입 허용 |
|
66 | 67 |
.requestMatchers("/studentInfo/**").permitAll() // 학생 정보 진입 허용(민수) |
67 | 68 |
.requestMatchers("/board/**").permitAll() // 게시판 정보 진입 허용 |
68 | 69 |
.requestMatchers("/book/**").permitAll() // 교재 정보 진입 허용 |
--- src/main/java/com/takensoft/ai_lms/lms/text/dao/TextDAO.java
+++ src/main/java/com/takensoft/ai_lms/lms/text/dao/TextDAO.java
... | ... | @@ -31,4 +31,7 @@ |
31 | 31 |
|
32 | 32 |
// 지문 삭제 |
33 | 33 |
int textDelete(String textId) throws Exception; |
34 |
+ |
|
35 |
+ // 지문 검색 |
|
36 |
+ List<HashMap<String, Object>> searchText(HashMap<String, Object> params) throws Exception; |
|
34 | 37 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/text/service/Impl/TextServiceImpl.java
+++ src/main/java/com/takensoft/ai_lms/lms/text/service/Impl/TextServiceImpl.java
... | ... | @@ -80,4 +80,13 @@ |
80 | 80 |
public int textDelete(String textId) throws Exception { |
81 | 81 |
return textDAO.textDelete(textId); |
82 | 82 |
} |
83 |
+ |
|
84 |
+ |
|
85 |
+ /** |
|
86 |
+ * 지문 검색 |
|
87 |
+ */ |
|
88 |
+ @Override |
|
89 |
+ public List<HashMap<String, Object>> searchText(HashMap<String, Object> params) throws Exception { |
|
90 |
+ return textDAO.searchText(params); |
|
91 |
+ } |
|
83 | 92 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/text/service/TextService.java
+++ src/main/java/com/takensoft/ai_lms/lms/text/service/TextService.java
... | ... | @@ -29,4 +29,7 @@ |
29 | 29 |
|
30 | 30 |
// 지문 삭제 |
31 | 31 |
int textDelete(String textId) throws Exception; |
32 |
+ |
|
33 |
+ // 지문 검색 |
|
34 |
+ public List<HashMap<String, Object>> searchText(HashMap<String, Object> params) throws Exception; |
|
32 | 35 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/text/web/TextController.java
+++ src/main/java/com/takensoft/ai_lms/lms/text/web/TextController.java
... | ... | @@ -138,5 +138,19 @@ |
138 | 138 |
return gson.toJson(response); |
139 | 139 |
} |
140 | 140 |
} |
141 |
+ |
|
142 |
+ |
|
143 |
+ /** |
|
144 |
+ * 지문 검색 |
|
145 |
+ */ |
|
146 |
+ @GetMapping("/textSearch.json") |
|
147 |
+ @Operation(summary = "지문 검색") |
|
148 |
+ public HashMap<String, Object> searchText(@RequestBody HashMap<String, Object> params) throws Exception { |
|
149 |
+ HashMap<String, Object> result = new HashMap<>(); |
|
150 |
+ List<HashMap<String, Object>> textList = TextService.searchText(params); |
|
151 |
+ result.put("list", textList); |
|
152 |
+ return result; |
|
153 |
+ } |
|
154 |
+ |
|
141 | 155 |
} |
142 | 156 |
|
--- src/main/resources/mybatis/mapper/lms/text-SQL.xml
+++ src/main/resources/mybatis/mapper/lms/text-SQL.xml
... | ... | @@ -97,4 +97,31 @@ |
97 | 97 |
WHERE text_id = #{textId} |
98 | 98 |
</delete> |
99 | 99 |
|
100 |
+ |
|
101 |
+ <!-- |
|
102 |
+ 작성자 : 이은진 |
|
103 |
+ 작성일 : 2024.07.26 |
|
104 |
+ 내 용 : 지문 검색 |
|
105 |
+ --> |
|
106 |
+ <select id="searchText" parameterType="TextVO" resultMap="textMap"> |
|
107 |
+ SELECT * |
|
108 |
+ FROM text |
|
109 |
+ WHERE 1 = 1 |
|
110 |
+ <if test="option != null and keyword != null"> |
|
111 |
+ <choose> |
|
112 |
+ <when test="option == 'textId'"> |
|
113 |
+ AND text_id LIKE CONCAT('%', #{keyword}, '%') |
|
114 |
+ </when> |
|
115 |
+ <when test="option == 'textCnt'"> |
|
116 |
+ AND text_cnt LIKE CONCAT('%', #{keyword}, '%') |
|
117 |
+ </when> |
|
118 |
+ <when test="option == 'regDt'"> |
|
119 |
+ AND TO_CHAR(reg_dt, 'YYYY-MM-DD HH24:MI:SS') LIKE CONCAT('%', #{keyword}, '%') |
|
120 |
+ </when> |
|
121 |
+ </choose> |
|
122 |
+ </if> |
|
123 |
+ ORDER BY text_id DESC |
|
124 |
+ LIMIT #{pageSize} OFFSET #{startIndex} |
|
125 |
+ </select> |
|
126 |
+ |
|
100 | 127 |
</mapper> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?