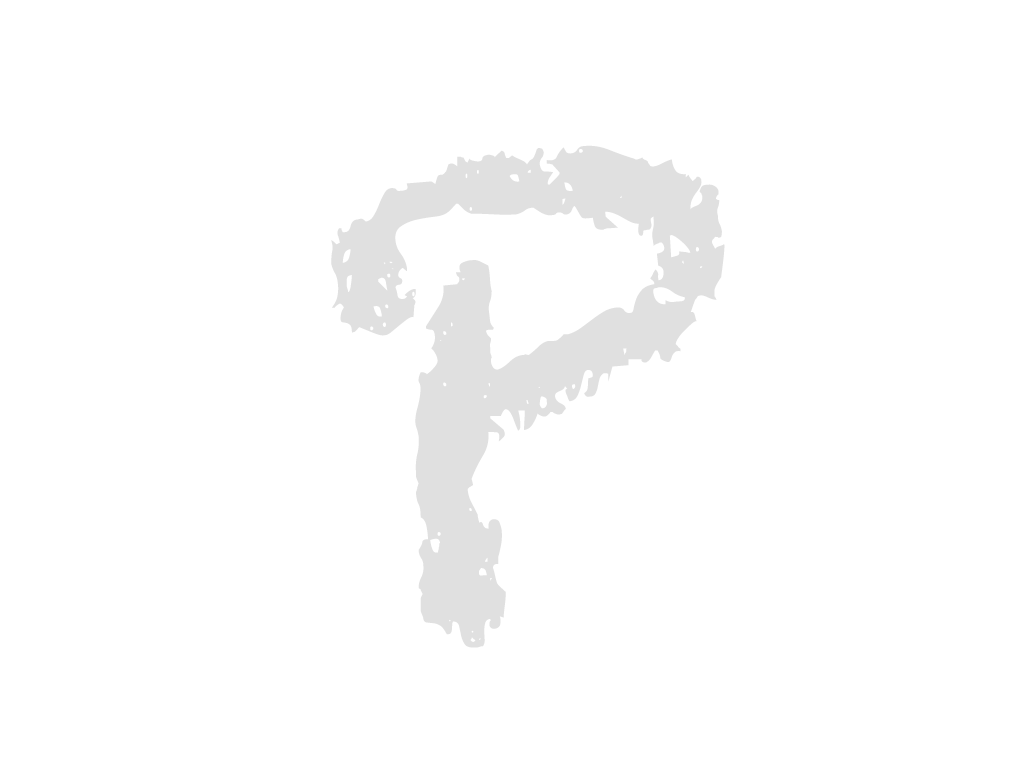
--- src/main/java/com/takensoft/ai_lms/lms/file/dao/FileDAO.java
+++ src/main/java/com/takensoft/ai_lms/lms/file/dao/FileDAO.java
... | ... | @@ -1,6 +1,7 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.file.dao; |
2 | 2 |
|
3 | 3 |
|
4 |
+import com.takensoft.ai_lms.lms.file.vo.FileVO; |
|
4 | 5 |
import org.egovframe.rte.psl.dataaccess.mapper.Mapper; |
5 | 6 |
|
6 | 7 |
import java.util.HashMap; |
... | ... | @@ -19,4 +20,20 @@ |
19 | 20 |
|
20 | 21 |
// 파일 정보 조회 |
21 | 22 |
List<HashMap<String, Object>> findByFileId(HashMap<String, Object> params) throws Exception; |
23 |
+ |
|
24 |
+ /* |
|
25 |
+ * @author : 박민혁 |
|
26 |
+ * since : 2024.08.01 |
|
27 |
+ * |
|
28 |
+ * 파일 매니지 아이디 insert |
|
29 |
+ */ |
|
30 |
+ int fileManageInsert(String fileMngId) throws Exception; |
|
31 |
+ |
|
32 |
+ /* |
|
33 |
+ * @author : 박민혁 |
|
34 |
+ * since : 2024.08.01 |
|
35 |
+ * |
|
36 |
+ * 파일 정보 insert |
|
37 |
+ */ |
|
38 |
+ int fileInsert(FileVO fileVO) throws Exception; |
|
22 | 39 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/file/service/FileService.java
+++ src/main/java/com/takensoft/ai_lms/lms/file/service/FileService.java
... | ... | @@ -1,6 +1,8 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.file.service; |
2 | 2 |
|
3 | 3 |
|
4 |
+import org.springframework.web.multipart.MultipartFile; |
|
5 |
+ |
|
4 | 6 |
import java.util.HashMap; |
5 | 7 |
import java.util.List; |
6 | 8 |
|
... | ... | @@ -13,4 +15,13 @@ |
13 | 15 |
public interface FileService { |
14 | 16 |
// 파일 정보 조회 |
15 | 17 |
List<HashMap<String, Object>> findByFileId(HashMap<String, Object> params) throws Exception; |
18 |
+ |
|
19 |
+ /* |
|
20 |
+ * @author : 박민혁 |
|
21 |
+ * since : 2024.08.01 |
|
22 |
+ * |
|
23 |
+ * 파일 업로드 기능 (파일 매니지 아이디 및 파일 정보 올리면서) |
|
24 |
+ */ |
|
25 |
+ String fileUpload(MultipartFile[] files) throws Exception; |
|
26 |
+ |
|
16 | 27 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/file/service/impl/FileServiceImpl.java
+++ src/main/java/com/takensoft/ai_lms/lms/file/service/impl/FileServiceImpl.java
... | ... | @@ -1,22 +1,87 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.file.service.impl; |
2 | 2 |
|
3 |
+import com.takensoft.ai_lms.common.idgen.service.IdgenService; |
|
3 | 4 |
import com.takensoft.ai_lms.lms.file.dao.FileDAO; |
4 | 5 |
import com.takensoft.ai_lms.lms.file.service.FileService; |
6 |
+import com.takensoft.ai_lms.lms.file.vo.FileManageVO; |
|
7 |
+import com.takensoft.ai_lms.lms.file.vo.FileVO; |
|
5 | 8 |
import lombok.RequiredArgsConstructor; |
6 | 9 |
import org.egovframe.rte.fdl.cmmn.EgovAbstractServiceImpl; |
7 | 10 |
import org.springframework.stereotype.Service; |
11 |
+import org.springframework.web.multipart.MultipartFile; |
|
8 | 12 |
|
13 |
+import java.io.File; |
|
14 |
+import java.nio.file.Files; |
|
15 |
+import java.nio.file.Path; |
|
16 |
+import java.nio.file.Paths; |
|
9 | 17 |
import java.util.HashMap; |
10 | 18 |
import java.util.List; |
19 |
+import java.util.UUID; |
|
11 | 20 |
|
12 | 21 |
@Service("FileService") |
13 | 22 |
@RequiredArgsConstructor |
14 | 23 |
public class FileServiceImpl extends EgovAbstractServiceImpl implements FileService { |
15 | 24 |
private final FileDAO fileDAO; |
16 | 25 |
|
26 |
+ private final IdgenService fileMngIdgn; |
|
27 |
+ private final IdgenService fileIdgn; |
|
28 |
+ |
|
17 | 29 |
// 파일 정보 조회 |
18 | 30 |
// @Override |
19 | 31 |
public List<HashMap<String, Object>> findByFileId(HashMap<String, Object> params) throws Exception { |
20 | 32 |
return fileDAO.findByFileId(params); |
21 | 33 |
} |
34 |
+ |
|
35 |
+ /* |
|
36 |
+ * @author : 박민혁 |
|
37 |
+ * since : 2024.08.01 |
|
38 |
+ * |
|
39 |
+ * 파일 업로드 기능 (파일 매니지 아이디 및 파일 정보 올리면서) |
|
40 |
+ */ |
|
41 |
+ public String fileUpload(MultipartFile[] files) throws Exception { |
|
42 |
+ FileManageVO fileManageVO = new FileManageVO(); |
|
43 |
+ String fileMngId = fileMngIdgn.getNextStringId(); |
|
44 |
+ fileManageVO.setFileMngId(fileMngId); |
|
45 |
+ |
|
46 |
+ // 파일 매니지 저장 |
|
47 |
+ fileDAO.fileManageInsert(fileMngId); |
|
48 |
+ |
|
49 |
+ String rootPath = "C:\\ai_lms\\cmmmfile\\"; |
|
50 |
+ |
|
51 |
+ for (MultipartFile file : files) { |
|
52 |
+ String originalFileName = file.getOriginalFilename(); |
|
53 |
+ String fileName = UUID.randomUUID().toString() + "_" + originalFileName; |
|
54 |
+ |
|
55 |
+ File tempDir = new File(rootPath); |
|
56 |
+ if (!tempDir.exists()) { |
|
57 |
+ tempDir.mkdirs(); |
|
58 |
+ } |
|
59 |
+ |
|
60 |
+ Path savePath = Paths.get(rootPath + fileName); |
|
61 |
+ |
|
62 |
+ // 파일 저장 |
|
63 |
+ Files.copy(file.getInputStream(), savePath); |
|
64 |
+ |
|
65 |
+ // 단일 파일들 저장 |
|
66 |
+ FileVO fileVO = new FileVO(); |
|
67 |
+ |
|
68 |
+ String fileId = fileIdgn.getNextStringId(); |
|
69 |
+ fileVO.setFileId(fileId); |
|
70 |
+ fileVO.setFileMngId(fileMngId); |
|
71 |
+ fileVO.setFileNm(originalFileName); |
|
72 |
+ fileVO.setFileEncptNm(fileName); |
|
73 |
+ fileVO.setFileSz(String.valueOf(file.getSize())); |
|
74 |
+ fileVO.setFileClsf(null); |
|
75 |
+ fileVO.setFileApath(savePath.toString()); |
|
76 |
+ fileVO.setFileExtn(fileName.substring(fileName.lastIndexOf(".") + 1)); |
|
77 |
+ |
|
78 |
+ // 상대 경로 설정 |
|
79 |
+ String relativePath = savePath.toString().replace(rootPath, ""); |
|
80 |
+ fileVO.setFileRpath(relativePath); |
|
81 |
+ |
|
82 |
+ fileDAO.fileInsert(fileVO); |
|
83 |
+ } |
|
84 |
+ |
|
85 |
+ return fileMngId; |
|
86 |
+ } |
|
22 | 87 |
} |
+++ src/main/java/com/takensoft/ai_lms/lms/file/vo/FileManageVO.java
... | ... | @@ -0,0 +1,24 @@ |
1 | +package com.takensoft.ai_lms.lms.file.vo; | |
2 | + | |
3 | +import lombok.AllArgsConstructor; | |
4 | +import lombok.Getter; | |
5 | +import lombok.NoArgsConstructor; | |
6 | +import lombok.Setter; | |
7 | + | |
8 | +/** | |
9 | + * @author : 박민혁 | |
10 | + * since : 2024.08.01 | |
11 | + * | |
12 | + * 파일 매니지 VO | |
13 | + */ | |
14 | + | |
15 | + | |
16 | + | |
17 | +@Getter | |
18 | +@Setter | |
19 | +@AllArgsConstructor | |
20 | +@NoArgsConstructor | |
21 | +public class FileManageVO { | |
22 | + // 파일 매니저 아이디 | |
23 | + private String fileMngId; | |
24 | +} |
--- src/main/java/com/takensoft/ai_lms/lms/file/web/FileController.java
+++ src/main/java/com/takensoft/ai_lms/lms/file/web/FileController.java
... | ... | @@ -4,10 +4,8 @@ |
4 | 4 |
import lombok.RequiredArgsConstructor; |
5 | 5 |
import org.springframework.http.HttpStatus; |
6 | 6 |
import org.springframework.http.ResponseEntity; |
7 |
-import org.springframework.web.bind.annotation.PostMapping; |
|
8 |
-import org.springframework.web.bind.annotation.RequestBody; |
|
9 |
-import org.springframework.web.bind.annotation.RequestMapping; |
|
10 |
-import org.springframework.web.bind.annotation.RestController; |
|
7 |
+import org.springframework.web.bind.annotation.*; |
|
8 |
+import org.springframework.web.multipart.MultipartFile; |
|
11 | 9 |
|
12 | 10 |
import java.util.HashMap; |
13 | 11 |
|
... | ... | @@ -36,4 +34,18 @@ |
36 | 34 |
result.put("list", fileService.findByFileId(params)); |
37 | 35 |
return new ResponseEntity<>(result, HttpStatus.OK); |
38 | 36 |
} |
37 |
+ |
|
38 |
+ /** |
|
39 |
+ * @author 박민혁 |
|
40 |
+ * @since 2024.08.01 |
|
41 |
+ * |
|
42 |
+ * 파일 업로드 기능 |
|
43 |
+ */ |
|
44 |
+ @PostMapping("/upload.json") |
|
45 |
+ public ResponseEntity<?> fileUpload (@RequestParam("files") MultipartFile[] files) throws Exception { |
|
46 |
+ HashMap<String, Object> result = new HashMap<>(); |
|
47 |
+ |
|
48 |
+ result.put("fileMngId", fileService.fileUpload(files)); |
|
49 |
+ return new ResponseEntity<>(result, HttpStatus.OK); |
|
50 |
+ } |
|
39 | 51 |
} |
--- src/main/resources/mybatis/mapper/lms/files-SQL.xml
+++ src/main/resources/mybatis/mapper/lms/files-SQL.xml
... | ... | @@ -42,4 +42,49 @@ |
42 | 42 |
FROM cmmn_file |
43 | 43 |
WHERE file_mng_id = #{file_mng_id} |
44 | 44 |
</select> |
45 |
+ |
|
46 |
+ <!-- |
|
47 |
+ 작성자 : 박민혁 |
|
48 |
+ 작성일 : 2024.08.01 |
|
49 |
+ 내 용 : 파일 매니지 정보 넣기 |
|
50 |
+ --> |
|
51 |
+ <select id="fileManageInsert" parameterType="String"> |
|
52 |
+ INSERT INTO cmmn_file_manage |
|
53 |
+ file_mng_id |
|
54 |
+ VALUES (#{fileMngId}) |
|
55 |
+ </select> |
|
56 |
+ <!-- |
|
57 |
+ 작성자 : 박민혁 |
|
58 |
+ 작성일 : 2024.08.01 |
|
59 |
+ 내 용 : 파일 매니지 정보 넣기 |
|
60 |
+ --> |
|
61 |
+ <select id="fileInsert" parameterType="FileVO"> |
|
62 |
+ INSERT INTO cmmn_file( |
|
63 |
+ file_id, |
|
64 |
+ file_mng_id, |
|
65 |
+ file_nm, |
|
66 |
+ file_encpt_nm, |
|
67 |
+ file_apath, |
|
68 |
+ file_rpath, |
|
69 |
+ file_extn, |
|
70 |
+ file_sz, |
|
71 |
+ file_clsf, |
|
72 |
+ reg, |
|
73 |
+ reg_dt |
|
74 |
+ ) |
|
75 |
+ VALUES ( |
|
76 |
+ #{fileId}, |
|
77 |
+ #{fileMngId}, |
|
78 |
+ #{fileNm}, |
|
79 |
+ #{fileEncptNm}, |
|
80 |
+ #{fileApath}, |
|
81 |
+ #{fileRpath}, |
|
82 |
+ #{fileExtn}, |
|
83 |
+ #{fileSz}, |
|
84 |
+ #{fileClsf}, |
|
85 |
+ #{reg}, |
|
86 |
+ now() |
|
87 |
+ ) |
|
88 |
+ </select> |
|
89 |
+ |
|
45 | 90 |
</mapper>(파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?