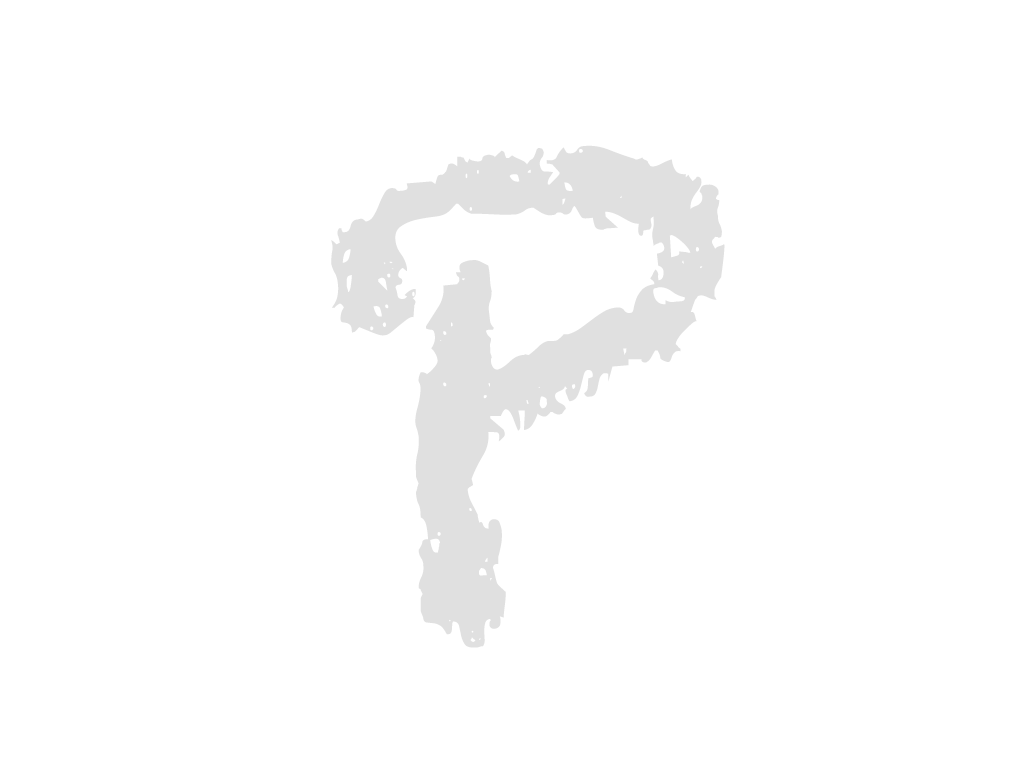
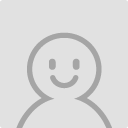
240729 권지수 단원 평가 CRUD, 문제 검색 추가
@28a82e8240b4148cf1045389645fbde0fa9ca70f
--- src/main/java/com/takensoft/ai_lms/common/confing/SecurityConfig.java
+++ src/main/java/com/takensoft/ai_lms/common/confing/SecurityConfig.java
... | ... | @@ -70,6 +70,9 @@ |
70 | 70 |
.requestMatchers("/file/**").permitAll() // 파일 정보 진입 허용 |
71 | 71 |
.requestMatchers("/classes/**").permitAll() // 반 정보 진입 허용 |
72 | 72 |
.requestMatchers("/classBook/**").permitAll() // 반 - 책 정보 진입 허용 |
73 |
+ .requestMatchers("/problem/**").permitAll() // 문제 정보 진입 허용 |
|
74 |
+ .requestMatchers("/evaluation/**").permitAll() // 단원 평가 정보 진입 허용 |
|
75 |
+ .requestMatchers("/evalProblem/**").permitAll() // 단원 평가 문제 진입 허용 |
|
73 | 76 |
.requestMatchers("/unitLearning/**").permitAll() // 로드맵 정보 진입 허용 |
74 | 77 |
.requestMatchers("/unit/**").permitAll() |
75 | 78 |
.requestMatchers("/photo/**").permitAll() |
+++ src/main/java/com/takensoft/ai_lms/lms/eval_problem/dao/EvalProblemDAO.java
... | ... | @@ -0,0 +1,32 @@ |
1 | +package com.takensoft.ai_lms.lms.eval_problem.dao; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.eval_problem.vo.EvalProblemVO; | |
4 | +import com.takensoft.ai_lms.lms.evaluation.vo.EvaluationVO; | |
5 | +import org.egovframe.rte.psl.dataaccess.mapper.Mapper; | |
6 | + | |
7 | +/** | |
8 | + * @author 권지수 | |
9 | + * @since 2024.07.29 | |
10 | + * | |
11 | + * 단원 평가 문제 DAO 클래스 | |
12 | + */ | |
13 | + | |
14 | +@Mapper("evalProblemDAO") | |
15 | +public interface EvalProblemDAO { | |
16 | + | |
17 | + /** | |
18 | + * @author 권지수 | |
19 | + * @since 2024.07.29 | |
20 | + * | |
21 | + * 단원 평가 문제 정보 입력 | |
22 | + */ | |
23 | + int insertEvalProblem(EvalProblemVO evalProblemVO) throws Exception; | |
24 | + | |
25 | + /** | |
26 | + * @author 권지수 | |
27 | + * @since 2024.07.30 | |
28 | + * | |
29 | + * 특정 단원의 문제 수 조회 | |
30 | + */ | |
31 | + int unitProblemNum(EvalProblemVO evalProblemVO) throws Exception; | |
32 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/eval_problem/service/EvalProblemService.java
... | ... | @@ -0,0 +1,28 @@ |
1 | +package com.takensoft.ai_lms.lms.eval_problem.service; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.eval_problem.vo.EvalProblemVO; | |
4 | + | |
5 | +/** | |
6 | + * @author 권지수 | |
7 | + * @since 2024.07.29 | |
8 | + * | |
9 | + * 단원 평가 문제 Service 클래스 | |
10 | + */ | |
11 | +public interface EvalProblemService { | |
12 | + | |
13 | + /** | |
14 | + * @author 권지수 | |
15 | + * @since 2024.07.29 | |
16 | + * | |
17 | + * 단원 평가 문제 정보 입력 | |
18 | + */ | |
19 | + int insertEvalProblem(EvalProblemVO evalProblemVO) throws Exception; | |
20 | + | |
21 | + /** | |
22 | + * @author 권지수 | |
23 | + * @since 2024.07.30 | |
24 | + * | |
25 | + * 특정 단원의 문제 수 조회 | |
26 | + */ | |
27 | + int unitProblemNum(EvalProblemVO evalProblemVO) throws Exception; | |
28 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/eval_problem/service/Impl/EvalProblemImpl.java
... | ... | @@ -0,0 +1,42 @@ |
1 | +package com.takensoft.ai_lms.lms.eval_problem.service.Impl; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.eval_problem.dao.EvalProblemDAO; | |
4 | +import com.takensoft.ai_lms.lms.eval_problem.service.EvalProblemService; | |
5 | +import com.takensoft.ai_lms.lms.eval_problem.vo.EvalProblemVO; | |
6 | +import lombok.RequiredArgsConstructor; | |
7 | +import org.egovframe.rte.fdl.cmmn.EgovAbstractServiceImpl; | |
8 | +import org.springframework.stereotype.Service; | |
9 | + | |
10 | +/** | |
11 | + * @author 권지수 | |
12 | + * @since 2024.07.29 | |
13 | + * | |
14 | + * 단원 평가 문제 ServiceImpl 클래스 | |
15 | + */ | |
16 | + | |
17 | +@Service("evalProblemService") | |
18 | +@RequiredArgsConstructor | |
19 | +public class EvalProblemImpl extends EgovAbstractServiceImpl implements EvalProblemService { | |
20 | + | |
21 | + private final EvalProblemDAO evalProblemDAO; | |
22 | + | |
23 | + /** | |
24 | + * @author 권지수 | |
25 | + * @since 2024.07.29 | |
26 | + * | |
27 | + * 단원 평가 문제 정보 입력 | |
28 | + */ | |
29 | + public int insertEvalProblem(EvalProblemVO evalProblemVO) throws Exception { | |
30 | + return evalProblemDAO.insertEvalProblem(evalProblemVO); | |
31 | + } | |
32 | + | |
33 | + /** | |
34 | + * @author 권지수 | |
35 | + * @since 2024.07.30 | |
36 | + * | |
37 | + * 특정 단원의 문제 수 조회 | |
38 | + */ | |
39 | + public int unitProblemNum(EvalProblemVO evalProblemVO) throws Exception { | |
40 | + return evalProblemDAO.unitProblemNum(evalProblemVO); | |
41 | + } | |
42 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/eval_problem/vo/EvalProblemVO.java
... | ... | @@ -0,0 +1,17 @@ |
1 | +package com.takensoft.ai_lms.lms.eval_problem.vo; | |
2 | + | |
3 | +import lombok.AllArgsConstructor; | |
4 | +import lombok.Getter; | |
5 | +import lombok.NoArgsConstructor; | |
6 | +import lombok.Setter; | |
7 | + | |
8 | +@Getter | |
9 | +@Setter | |
10 | +@AllArgsConstructor | |
11 | +@NoArgsConstructor | |
12 | +public class EvalProblemVO { | |
13 | + // 단원 평가 아이디 | |
14 | + private String evalId; | |
15 | + // 문제 아이디 | |
16 | + private String prblmId; | |
17 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/eval_problem/web/EvalProblemController.java
... | ... | @@ -0,0 +1,55 @@ |
1 | +package com.takensoft.ai_lms.lms.eval_problem.web; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.eval_problem.service.EvalProblemService; | |
4 | +import com.takensoft.ai_lms.lms.eval_problem.vo.EvalProblemVO; | |
5 | +import com.takensoft.ai_lms.lms.evaluation.vo.EvaluationVO; | |
6 | +import lombok.RequiredArgsConstructor; | |
7 | +import lombok.extern.slf4j.Slf4j; | |
8 | +import org.springframework.http.HttpStatus; | |
9 | +import org.springframework.http.ResponseEntity; | |
10 | +import org.springframework.web.bind.annotation.PostMapping; | |
11 | +import org.springframework.web.bind.annotation.RequestBody; | |
12 | +import org.springframework.web.bind.annotation.RequestMapping; | |
13 | +import org.springframework.web.bind.annotation.RestController; | |
14 | + | |
15 | +/** | |
16 | + * @author 권지수 | |
17 | + * @since 2024.07.29 | |
18 | + * | |
19 | + * 단원 평가 Controller 클래스 | |
20 | + */ | |
21 | + | |
22 | +@RestController | |
23 | +@RequiredArgsConstructor | |
24 | +@Slf4j | |
25 | +@RequestMapping(value = "/evalProblem") | |
26 | +public class EvalProblemController { | |
27 | + | |
28 | + private final EvalProblemService evalProblemService; | |
29 | + | |
30 | + /** | |
31 | + * @author 권지수 | |
32 | + * @since 2024.07.29 | |
33 | + * | |
34 | + * 단원 평가 문제 입력 | |
35 | + */ | |
36 | + @PostMapping(path = "/insertEvalProblem.json") | |
37 | + public ResponseEntity<?> insertEvalProblem(@RequestBody EvalProblemVO evalProblemVO) throws Exception { | |
38 | + int result = evalProblemService.insertEvalProblem(evalProblemVO); | |
39 | + | |
40 | + return new ResponseEntity<>(result, HttpStatus.OK); | |
41 | + } | |
42 | + | |
43 | + /** | |
44 | + * @author 권지수 | |
45 | + * @since 2024.07.30 | |
46 | + * | |
47 | + * 특정 단원의 문제 수 조회 | |
48 | + */ | |
49 | + @PostMapping(path = "/unitProblemNum.json") | |
50 | + public ResponseEntity<?> unitProblemNum(@RequestBody EvalProblemVO evalProblemVO) throws Exception { | |
51 | + int result = evalProblemService.unitProblemNum(evalProblemVO); | |
52 | + | |
53 | + return new ResponseEntity<>(result, HttpStatus.OK); | |
54 | + } | |
55 | +} |
--- src/main/java/com/takensoft/ai_lms/lms/evaluation/dao/EvaluationDAO.java
+++ src/main/java/com/takensoft/ai_lms/lms/evaluation/dao/EvaluationDAO.java
... | ... | @@ -1,4 +1,59 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.evaluation.dao; |
2 | 2 |
|
3 |
+import com.takensoft.ai_lms.lms.evaluation.vo.EvaluationVO; |
|
4 |
+import com.takensoft.ai_lms.lms.problem.vo.SearchVO; |
|
5 |
+import org.egovframe.rte.psl.dataaccess.mapper.Mapper; |
|
6 |
+ |
|
7 |
+import java.util.List; |
|
8 |
+ |
|
9 |
+/** |
|
10 |
+ * @author 권지수 |
|
11 |
+ * @since 2024.07.29 |
|
12 |
+ * |
|
13 |
+ * 단원 평가 DAO 클래스 |
|
14 |
+ */ |
|
15 |
+ |
|
16 |
+@Mapper("evaluationDAO") |
|
3 | 17 |
public interface EvaluationDAO { |
18 |
+ |
|
19 |
+ /** |
|
20 |
+ * @author 권지수 |
|
21 |
+ * @since 2024.07.29 |
|
22 |
+ * |
|
23 |
+ * 단원 평가 정보 입력 |
|
24 |
+ */ |
|
25 |
+ int insertEvaluation(EvaluationVO evaluationVO) throws Exception; |
|
26 |
+ |
|
27 |
+ /** |
|
28 |
+ * @author 권지수 |
|
29 |
+ * @since 2024.07.29 |
|
30 |
+ * |
|
31 |
+ * 단원 평가 정보 조회 |
|
32 |
+ */ |
|
33 |
+ EvaluationVO evaluationInfo(EvaluationVO evaluationVO) throws Exception; |
|
34 |
+ |
|
35 |
+ /** |
|
36 |
+ * @author 권지수 |
|
37 |
+ * @since 2024.07.29 |
|
38 |
+ * |
|
39 |
+ * 특정 단원 평가 정보 조회 |
|
40 |
+ */ |
|
41 |
+ List<EvaluationVO> evaluationUnitList(SearchVO searchVO) throws Exception; |
|
42 |
+ |
|
43 |
+ /** |
|
44 |
+ * @author 권지수 |
|
45 |
+ * @since 2024.07.29 |
|
46 |
+ * |
|
47 |
+ * 단원 평가 정보 수정 |
|
48 |
+ */ |
|
49 |
+ int updateEvaluation(EvaluationVO evaluationVO) throws Exception; |
|
50 |
+ |
|
51 |
+ /** |
|
52 |
+ * @author 권지수 |
|
53 |
+ * @since 2024.07.29 |
|
54 |
+ * |
|
55 |
+ * 단원 평가 정보 삭제 |
|
56 |
+ */ |
|
57 |
+ int deleteEvaluation(EvaluationVO evaluationVO) throws Exception; |
|
58 |
+ |
|
4 | 59 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/evaluation/service/EvaluationService.java
+++ src/main/java/com/takensoft/ai_lms/lms/evaluation/service/EvaluationService.java
... | ... | @@ -1,4 +1,58 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.evaluation.service; |
2 | 2 |
|
3 |
+import com.takensoft.ai_lms.lms.evaluation.vo.EvaluationVO; |
|
4 |
+import com.takensoft.ai_lms.lms.problem.vo.SearchVO; |
|
5 |
+import org.egovframe.rte.psl.dataaccess.mapper.Mapper; |
|
6 |
+ |
|
7 |
+import java.util.List; |
|
8 |
+ |
|
9 |
+/** |
|
10 |
+ * @author 권지수 |
|
11 |
+ * @since 2024.07.29 |
|
12 |
+ * |
|
13 |
+ * 단원 평가 Service 클래스 |
|
14 |
+ */ |
|
15 |
+ |
|
3 | 16 |
public interface EvaluationService { |
17 |
+ |
|
18 |
+ /** |
|
19 |
+ * @author 권지수 |
|
20 |
+ * @since 2024.07.29 |
|
21 |
+ * |
|
22 |
+ * 단원 평가 정보 입력 |
|
23 |
+ */ |
|
24 |
+ int insertEvaluation(EvaluationVO evaluationVO) throws Exception; |
|
25 |
+ |
|
26 |
+ /** |
|
27 |
+ * @author 권지수 |
|
28 |
+ * @since 2024.07.29 |
|
29 |
+ * |
|
30 |
+ * 단원 평가 정보 조회 |
|
31 |
+ */ |
|
32 |
+ EvaluationVO evaluationInfo(EvaluationVO evaluationVO) throws Exception; |
|
33 |
+ |
|
34 |
+ /** |
|
35 |
+ * @author 권지수 |
|
36 |
+ * @since 2024.07.29 |
|
37 |
+ * |
|
38 |
+ * 특정 단원 평가 정보 조회 |
|
39 |
+ */ |
|
40 |
+ List<EvaluationVO> evaluationUnitList(SearchVO searchVO) throws Exception; |
|
41 |
+ |
|
42 |
+ /** |
|
43 |
+ * @author 권지수 |
|
44 |
+ * @since 2024.07.29 |
|
45 |
+ * |
|
46 |
+ * 단원 평가 정보 수정 |
|
47 |
+ */ |
|
48 |
+ int updateEvaluation(EvaluationVO evaluationVO) throws Exception; |
|
49 |
+ |
|
50 |
+ /** |
|
51 |
+ * @author 권지수 |
|
52 |
+ * @since 2024.07.29 |
|
53 |
+ * |
|
54 |
+ * 단원 평가 정보 삭제 |
|
55 |
+ */ |
|
56 |
+ int deleteEvaluation(EvaluationVO evaluationVO) throws Exception; |
|
57 |
+ |
|
4 | 58 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/evaluation/service/Impl/EvaluationService.java
... | ... | @@ -1,4 +0,0 @@ |
1 | -package com.takensoft.ai_lms.lms.evaluation.service.Impl; | |
2 | - | |
3 | -public class EvaluationService { | |
4 | -} |
+++ src/main/java/com/takensoft/ai_lms/lms/evaluation/service/Impl/EvaluationServiceImpl.java
... | ... | @@ -0,0 +1,81 @@ |
1 | +package com.takensoft.ai_lms.lms.evaluation.service.Impl; | |
2 | + | |
3 | +import com.takensoft.ai_lms.common.idgen.service.IdgenService; | |
4 | +import com.takensoft.ai_lms.lms.evaluation.dao.EvaluationDAO; | |
5 | +import com.takensoft.ai_lms.lms.evaluation.service.EvaluationService; | |
6 | +import com.takensoft.ai_lms.lms.evaluation.vo.EvaluationVO; | |
7 | +import com.takensoft.ai_lms.lms.problem.vo.SearchVO; | |
8 | +import lombok.RequiredArgsConstructor; | |
9 | +import org.egovframe.rte.fdl.cmmn.EgovAbstractServiceImpl; | |
10 | +import org.springframework.stereotype.Service; | |
11 | + | |
12 | +import java.util.List; | |
13 | + | |
14 | +/** | |
15 | + * @author 권지수 | |
16 | + * @since 2024.07.29 | |
17 | + * | |
18 | + * 단원 평가 ServiceImpl 클래스 | |
19 | + */ | |
20 | + | |
21 | +@Service("evaluationService") | |
22 | +@RequiredArgsConstructor | |
23 | +public class EvaluationServiceImpl extends EgovAbstractServiceImpl implements EvaluationService { | |
24 | + | |
25 | + private final EvaluationDAO evaluationDAO; | |
26 | + private final IdgenService evalIdgn; | |
27 | + | |
28 | + /** | |
29 | + * @author 권지수 | |
30 | + * @since 2024.07.29 | |
31 | + * | |
32 | + * 단원 평가 정보 입력 | |
33 | + */ | |
34 | + public int insertEvaluation(EvaluationVO evaluationVO) throws Exception { | |
35 | + String evaluationId = evalIdgn.getNextStringId(); | |
36 | + evaluationVO.setEvalId(evaluationId); | |
37 | + | |
38 | + return evaluationDAO.insertEvaluation(evaluationVO); | |
39 | + } | |
40 | + | |
41 | + /** | |
42 | + * @author 권지수 | |
43 | + * @since 2024.07.29 | |
44 | + * | |
45 | + * 단원 평가 정보 조회 | |
46 | + */ | |
47 | + public EvaluationVO evaluationInfo(EvaluationVO evaluationVO) throws Exception { | |
48 | + return evaluationDAO.evaluationInfo(evaluationVO); | |
49 | + } | |
50 | + | |
51 | + /** | |
52 | + * @author 권지수 | |
53 | + * @since 2024.07.29 | |
54 | + * | |
55 | + * 특정 단원 평가 정보 조회 | |
56 | + */ | |
57 | + public List<EvaluationVO> evaluationUnitList(SearchVO searchVO) throws Exception { | |
58 | + return evaluationDAO.evaluationUnitList(searchVO); | |
59 | + } | |
60 | + | |
61 | + /** | |
62 | + * @author 권지수 | |
63 | + * @since 2024.07.29 | |
64 | + * | |
65 | + * 단원 평가 정보 수정 | |
66 | + */ | |
67 | + public int updateEvaluation(EvaluationVO evaluationVO) throws Exception { | |
68 | + return evaluationDAO.updateEvaluation(evaluationVO); | |
69 | + } | |
70 | + | |
71 | + /** | |
72 | + * @author 권지수 | |
73 | + * @since 2024.07.29 | |
74 | + * | |
75 | + * 단원 평가 정보 삭제 | |
76 | + */ | |
77 | + public int deleteEvaluation(EvaluationVO evaluationVO) throws Exception { | |
78 | + return evaluationDAO.deleteEvaluation(evaluationVO); | |
79 | + } | |
80 | + | |
81 | +} |
--- src/main/java/com/takensoft/ai_lms/lms/evaluation/vo/EvaluationVO.java
+++ src/main/java/com/takensoft/ai_lms/lms/evaluation/vo/EvaluationVO.java
... | ... | @@ -1,4 +1,19 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.evaluation.vo; |
2 | 2 |
|
3 |
+import lombok.AllArgsConstructor; |
|
4 |
+import lombok.Getter; |
|
5 |
+import lombok.NoArgsConstructor; |
|
6 |
+import lombok.Setter; |
|
7 |
+ |
|
8 |
+@Getter |
|
9 |
+@Setter |
|
10 |
+@AllArgsConstructor |
|
11 |
+@NoArgsConstructor |
|
3 | 12 |
public class EvaluationVO { |
13 |
+ // 단원 평가 아이디 |
|
14 |
+ private String evalId; |
|
15 |
+ // 단원 평가 유형 |
|
16 |
+ private String evalType; |
|
17 |
+ // 단원 아이디 |
|
18 |
+ private String unitId; |
|
4 | 19 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/evaluation/web/EvaluationController.java
+++ src/main/java/com/takensoft/ai_lms/lms/evaluation/web/EvaluationController.java
... | ... | @@ -1,4 +1,97 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.evaluation.web; |
2 | 2 |
|
3 |
+import com.takensoft.ai_lms.lms.evaluation.service.EvaluationService; |
|
4 |
+import com.takensoft.ai_lms.lms.evaluation.vo.EvaluationVO; |
|
5 |
+import com.takensoft.ai_lms.lms.problem.vo.ProblemVO; |
|
6 |
+import com.takensoft.ai_lms.lms.problem.vo.SearchVO; |
|
7 |
+import lombok.RequiredArgsConstructor; |
|
8 |
+import lombok.extern.slf4j.Slf4j; |
|
9 |
+import org.springframework.http.HttpStatus; |
|
10 |
+import org.springframework.http.ResponseEntity; |
|
11 |
+import org.springframework.web.bind.annotation.PostMapping; |
|
12 |
+import org.springframework.web.bind.annotation.RequestBody; |
|
13 |
+import org.springframework.web.bind.annotation.RequestMapping; |
|
14 |
+import org.springframework.web.bind.annotation.RestController; |
|
15 |
+ |
|
16 |
+import java.util.List; |
|
17 |
+ |
|
18 |
+/** |
|
19 |
+ * @author 권지수 |
|
20 |
+ * @since 2024.07.29 |
|
21 |
+ * |
|
22 |
+ * 단원 평가 Controller 클래스 |
|
23 |
+ */ |
|
24 |
+ |
|
25 |
+@RestController |
|
26 |
+@RequiredArgsConstructor |
|
27 |
+@Slf4j |
|
28 |
+@RequestMapping(value = "/evaluation") |
|
3 | 29 |
public class EvaluationController { |
30 |
+ |
|
31 |
+ private final EvaluationService evaluationService; |
|
32 |
+ |
|
33 |
+ /** |
|
34 |
+ * @author 권지수 |
|
35 |
+ * @since 2024.07.29 |
|
36 |
+ * |
|
37 |
+ * 단원 평가 정보 입력 |
|
38 |
+ */ |
|
39 |
+ @PostMapping(path = "/insertEvaluation.json") |
|
40 |
+ public ResponseEntity<?> insertEvaluation(@RequestBody EvaluationVO evaluationVO) throws Exception { |
|
41 |
+ int result = evaluationService.insertEvaluation(evaluationVO); |
|
42 |
+ |
|
43 |
+ return new ResponseEntity<>(result, HttpStatus.OK); |
|
44 |
+ } |
|
45 |
+ |
|
46 |
+ /** |
|
47 |
+ * @author 권지수 |
|
48 |
+ * @since 2024.07.29 |
|
49 |
+ * |
|
50 |
+ * 단원 평가 정보 조회 |
|
51 |
+ */ |
|
52 |
+ @PostMapping(path = "/evaluationInfo.json") |
|
53 |
+ public ResponseEntity<?> evaluationInfo(@RequestBody EvaluationVO evaluationVO) throws Exception { |
|
54 |
+ EvaluationVO result = evaluationService.evaluationInfo(evaluationVO); |
|
55 |
+ |
|
56 |
+ return new ResponseEntity<>(result, HttpStatus.OK); |
|
57 |
+ } |
|
58 |
+ |
|
59 |
+ /** |
|
60 |
+ * @author 권지수 |
|
61 |
+ * @since 2024.07.29 |
|
62 |
+ * |
|
63 |
+ * 특정 단원 평가 정보 조회 |
|
64 |
+ */ |
|
65 |
+ @PostMapping(path = "/evaluationUnitList.json") |
|
66 |
+ public ResponseEntity<?> evaluationUnitList(@RequestBody SearchVO searchVO) throws Exception { |
|
67 |
+ List<EvaluationVO> result = evaluationService.evaluationUnitList(searchVO); |
|
68 |
+ |
|
69 |
+ return new ResponseEntity<>(result, HttpStatus.OK); |
|
70 |
+ } |
|
71 |
+ |
|
72 |
+ /** |
|
73 |
+ * @author 권지수 |
|
74 |
+ * @since 2024.07.29 |
|
75 |
+ * |
|
76 |
+ * 단원 평가 정보 수정 |
|
77 |
+ */ |
|
78 |
+ @PostMapping(path = "/updateEvaluation.json") |
|
79 |
+ public ResponseEntity<?> updateEvaluation(@RequestBody EvaluationVO evaluationVO) throws Exception { |
|
80 |
+ int result = evaluationService.updateEvaluation(evaluationVO); |
|
81 |
+ |
|
82 |
+ return new ResponseEntity<>(result, HttpStatus.OK); |
|
83 |
+ } |
|
84 |
+ |
|
85 |
+ /** |
|
86 |
+ * @author 권지수 |
|
87 |
+ * @since 2024.07.29 |
|
88 |
+ * |
|
89 |
+ * 단원 평가 정보 삭제 |
|
90 |
+ */ |
|
91 |
+ @PostMapping(path = "/deleteEvaluation.json") |
|
92 |
+ public ResponseEntity<?> deleteEvaluation(@RequestBody EvaluationVO evaluationVO) throws Exception { |
|
93 |
+ int result = evaluationService.deleteEvaluation(evaluationVO); |
|
94 |
+ |
|
95 |
+ return new ResponseEntity<>(result, HttpStatus.OK); |
|
96 |
+ } |
|
4 | 97 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/problem/dao/ProblemDAO.java
+++ src/main/java/com/takensoft/ai_lms/lms/problem/dao/ProblemDAO.java
... | ... | @@ -1,7 +1,9 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.problem.dao; |
2 | 2 |
|
3 |
+import com.takensoft.ai_lms.lms.eval_problem.vo.EvalProblemVO; |
|
3 | 4 |
import com.takensoft.ai_lms.lms.problem.vo.ProblemDetailVO; |
4 | 5 |
import com.takensoft.ai_lms.lms.problem.vo.ProblemVO; |
6 |
+import com.takensoft.ai_lms.lms.problem.vo.SearchVO; |
|
5 | 7 |
import org.egovframe.rte.psl.dataaccess.mapper.Mapper; |
6 | 8 |
|
7 | 9 |
import java.util.List; |
... | ... | @@ -72,4 +74,20 @@ |
72 | 74 |
*/ |
73 | 75 |
int deleteProblem(ProblemVO problemVO) throws Exception; |
74 | 76 |
|
77 |
+ /** |
|
78 |
+ * @author 권지수 |
|
79 |
+ * @since 2024.07.30 |
|
80 |
+ * |
|
81 |
+ * 전체 문제 목록 조회 |
|
82 |
+ */ |
|
83 |
+ List<ProblemVO> problemList(SearchVO searchVO) throws Exception; |
|
84 |
+ |
|
85 |
+ /** |
|
86 |
+ * @author 권지수 |
|
87 |
+ * @since 2024.07.30 |
|
88 |
+ * |
|
89 |
+ * 특정 단원 평가의 문제 목록 조회 |
|
90 |
+ */ |
|
91 |
+ List<ProblemVO> evaluationProblemList(SearchVO searchVO) throws Exception; |
|
92 |
+ |
|
75 | 93 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/problem/service/Impl/ProblemServiceImpl.java
+++ src/main/java/com/takensoft/ai_lms/lms/problem/service/Impl/ProblemServiceImpl.java
... | ... | @@ -1,10 +1,12 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.problem.service.Impl; |
2 | 2 |
|
3 | 3 |
import com.takensoft.ai_lms.common.idgen.service.IdgenService; |
4 |
+import com.takensoft.ai_lms.lms.eval_problem.vo.EvalProblemVO; |
|
4 | 5 |
import com.takensoft.ai_lms.lms.problem.dao.ProblemDAO; |
5 | 6 |
import com.takensoft.ai_lms.lms.problem.service.ProblemService; |
6 | 7 |
import com.takensoft.ai_lms.lms.problem.vo.ProblemDetailVO; |
7 | 8 |
import com.takensoft.ai_lms.lms.problem.vo.ProblemVO; |
9 |
+import com.takensoft.ai_lms.lms.problem.vo.SearchVO; |
|
8 | 10 |
import lombok.RequiredArgsConstructor; |
9 | 11 |
import org.egovframe.rte.fdl.cmmn.EgovAbstractServiceImpl; |
10 | 12 |
import org.springframework.stereotype.Service; |
... | ... | @@ -106,4 +108,24 @@ |
106 | 108 |
public int deleteProblem(ProblemVO problemVO) throws Exception { |
107 | 109 |
return problemDAO.deleteProblem(problemVO); |
108 | 110 |
} |
111 |
+ |
|
112 |
+ /** |
|
113 |
+ * @author 권지수 |
|
114 |
+ * @since 2024.07.30 |
|
115 |
+ * |
|
116 |
+ * 전체 문제 목록 조회 |
|
117 |
+ */ |
|
118 |
+ public List<ProblemVO> problemList(SearchVO searchVO) throws Exception { |
|
119 |
+ return problemDAO.problemList(searchVO); |
|
120 |
+ } |
|
121 |
+ |
|
122 |
+ /** |
|
123 |
+ * @author 권지수 |
|
124 |
+ * @since 2024.07.30 |
|
125 |
+ * |
|
126 |
+ * 특정 단원 평가의 문제 목록 조회 |
|
127 |
+ */ |
|
128 |
+ public List<ProblemVO> evaluationProblemList(SearchVO searchVO) throws Exception { |
|
129 |
+ return problemDAO.evaluationProblemList(searchVO); |
|
130 |
+ } |
|
109 | 131 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/problem/service/ProblemService.java
+++ src/main/java/com/takensoft/ai_lms/lms/problem/service/ProblemService.java
... | ... | @@ -1,7 +1,9 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.problem.service; |
2 | 2 |
|
3 |
+import com.takensoft.ai_lms.lms.eval_problem.vo.EvalProblemVO; |
|
3 | 4 |
import com.takensoft.ai_lms.lms.problem.vo.ProblemDetailVO; |
4 | 5 |
import com.takensoft.ai_lms.lms.problem.vo.ProblemVO; |
6 |
+import com.takensoft.ai_lms.lms.problem.vo.SearchVO; |
|
5 | 7 |
|
6 | 8 |
import java.util.HashMap; |
7 | 9 |
import java.util.List; |
... | ... | @@ -70,4 +72,20 @@ |
70 | 72 |
* 문제 정보 삭제 |
71 | 73 |
*/ |
72 | 74 |
int deleteProblem(ProblemVO problemVO) throws Exception; |
75 |
+ |
|
76 |
+ /** |
|
77 |
+ * @author 권지수 |
|
78 |
+ * @since 2024.07.30 |
|
79 |
+ * |
|
80 |
+ * 전체 문제 목록 조회 |
|
81 |
+ */ |
|
82 |
+ List<ProblemVO> problemList(SearchVO searchVO) throws Exception; |
|
83 |
+ |
|
84 |
+ /** |
|
85 |
+ * @author 권지수 |
|
86 |
+ * @since 2024.07.30 |
|
87 |
+ * |
|
88 |
+ * 특정 단원 평가의 문제 목록 조회 |
|
89 |
+ */ |
|
90 |
+ List<ProblemVO> evaluationProblemList(SearchVO searchVO) throws Exception; |
|
73 | 91 |
} |
+++ src/main/java/com/takensoft/ai_lms/lms/problem/vo/SearchVO.java
... | ... | @@ -0,0 +1,25 @@ |
1 | +package com.takensoft.ai_lms.lms.problem.vo; | |
2 | + | |
3 | +import lombok.AllArgsConstructor; | |
4 | +import lombok.Getter; | |
5 | +import lombok.NoArgsConstructor; | |
6 | +import lombok.Setter; | |
7 | + | |
8 | +@Getter | |
9 | +@Setter | |
10 | +@AllArgsConstructor | |
11 | +@NoArgsConstructor | |
12 | +public class SearchVO { | |
13 | + // 검색 옵션 | |
14 | + private String option; | |
15 | + // 검색 키워드 | |
16 | + private String keyword; | |
17 | + // 페이지 크기 | |
18 | + private int pageSize; | |
19 | + // 시작 인덱스 | |
20 | + private int startIndex; | |
21 | + // 단원 평가 아이디 | |
22 | + private String evalId; | |
23 | + // 단원 아이디 | |
24 | + private String unitId; | |
25 | +} |
--- src/main/java/com/takensoft/ai_lms/lms/problem/web/ProblemController.java
+++ src/main/java/com/takensoft/ai_lms/lms/problem/web/ProblemController.java
... | ... | @@ -1,9 +1,11 @@ |
1 | 1 |
package com.takensoft.ai_lms.lms.problem.web; |
2 | 2 |
|
3 | 3 |
import com.takensoft.ai_lms.lms.auth.vo.UserVO; |
4 |
+import com.takensoft.ai_lms.lms.eval_problem.vo.EvalProblemVO; |
|
4 | 5 |
import com.takensoft.ai_lms.lms.problem.service.ProblemService; |
5 | 6 |
import com.takensoft.ai_lms.lms.problem.vo.ProblemDetailVO; |
6 | 7 |
import com.takensoft.ai_lms.lms.problem.vo.ProblemVO; |
8 |
+import com.takensoft.ai_lms.lms.problem.vo.SearchVO; |
|
7 | 9 |
import lombok.RequiredArgsConstructor; |
8 | 10 |
import lombok.extern.slf4j.Slf4j; |
9 | 11 |
import org.springframework.http.HttpStatus; |
... | ... | @@ -136,4 +138,30 @@ |
136 | 138 |
|
137 | 139 |
return new ResponseEntity<>(result, HttpStatus.OK); |
138 | 140 |
} |
141 |
+ |
|
142 |
+ /** |
|
143 |
+ * @author 권지수 |
|
144 |
+ * @since 2024.07.26 |
|
145 |
+ * |
|
146 |
+ * 전체 문제 목록 조회 |
|
147 |
+ */ |
|
148 |
+ @PostMapping(path = "/problemList.json") |
|
149 |
+ public ResponseEntity<?> problemList(@RequestBody SearchVO searchVO) throws Exception { |
|
150 |
+ List<ProblemVO> result = problemService.problemList(searchVO); |
|
151 |
+ |
|
152 |
+ return new ResponseEntity<>(result, HttpStatus.OK); |
|
153 |
+ } |
|
154 |
+ |
|
155 |
+ /** |
|
156 |
+ * @author 권지수 |
|
157 |
+ * @since 2024.07.26 |
|
158 |
+ * |
|
159 |
+ * 특정 단원 평가의 문제 목록 조회 |
|
160 |
+ */ |
|
161 |
+ @PostMapping(path = "/evaluationProblemList.json") |
|
162 |
+ public ResponseEntity<?> evaluationProblemList(@RequestBody SearchVO searchVO) throws Exception { |
|
163 |
+ List<ProblemVO> result = problemService.evaluationProblemList(searchVO); |
|
164 |
+ |
|
165 |
+ return new ResponseEntity<>(result, HttpStatus.OK); |
|
166 |
+ } |
|
139 | 167 |
} |
+++ src/main/resources/mybatis/mapper/lms/eval_problem-SQL.xml
... | ... | @@ -0,0 +1,32 @@ |
1 | +<?xml version="1.0" encoding="UTF-8"?> | |
2 | +<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> | |
3 | +<mapper namespace="com.takensoft.ai_lms.lms.eval_problem.dao.EvalProblemDAO"> | |
4 | + | |
5 | + <!-- | |
6 | + 작 성 자 : 권지수 | |
7 | + 작 성 일 : 2024.07.29 | |
8 | + 내 용 : 단원 평가 문제 insert | |
9 | + --> | |
10 | + <insert id="insertEvalProblem" parameterType="EvalProblemVO"> | |
11 | + INSERT INTO eval_problem ( | |
12 | + eval_id, | |
13 | + prblm_id | |
14 | + ) VALUES ( | |
15 | + #{evalId}, | |
16 | + #{prblmId} | |
17 | + ) | |
18 | + </insert> | |
19 | + | |
20 | + <!-- | |
21 | + 작 성 자 : 권지수 | |
22 | + 작 성 일 : 2024.07.30 | |
23 | + 내 용 : 특정 단원의 문제 수 조회 | |
24 | + --> | |
25 | + <select id="unitProblemNum" parameterType="EvaluationVO" resultType="int"> | |
26 | + SELECT | |
27 | + COUNT(*) | |
28 | + FROM eval_problem | |
29 | + WHERE eval_id = #{evalId} | |
30 | + </select> | |
31 | + | |
32 | +</mapper>(파일 끝에 줄바꿈 문자 없음) |
--- src/main/resources/mybatis/mapper/lms/evaluation-SQL.xml
+++ src/main/resources/mybatis/mapper/lms/evaluation-SQL.xml
... | ... | @@ -2,6 +2,89 @@ |
2 | 2 |
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
3 | 3 |
<mapper namespace="com.takensoft.ai_lms.lms.evaluation.dao.EvaluationDAO"> |
4 | 4 |
|
5 |
+ <!-- |
|
6 |
+ 작 성 자 : 권지수 |
|
7 |
+ 작 성 일 : 2024.07.29 |
|
8 |
+ 내 용 : 단원 평가 정보 insert |
|
9 |
+ --> |
|
10 |
+ <insert id="insertEvaluation" parameterType="EvaluationVO"> |
|
11 |
+ INSERT INTO evaluation ( |
|
12 |
+ eval_id, |
|
13 |
+ eval_type, |
|
14 |
+ unit_id |
|
15 |
+ ) VALUES ( |
|
16 |
+ #{evalId}, |
|
17 |
+ #{evalType}, |
|
18 |
+ #{unitId} |
|
19 |
+ ) |
|
20 |
+ </insert> |
|
21 |
+ |
|
22 |
+ <!-- |
|
23 |
+ 작 성 자 : 권지수 |
|
24 |
+ 작 성 일 : 2024.07.29 |
|
25 |
+ 내 용 : 단원 평가 정보 read |
|
26 |
+ --> |
|
27 |
+ <select id="evaluationInfo" parameterType="EvaluationVO" resultType="EvaluationVO"> |
|
28 |
+ SELECT |
|
29 |
+ eval_id, |
|
30 |
+ eval_type, |
|
31 |
+ unit_id |
|
32 |
+ FROM evaluation |
|
33 |
+ WHERE eval_id = #{evalId} |
|
34 |
+ </select> |
|
35 |
+ |
|
36 |
+ <!-- |
|
37 |
+ 작 성 자 : 권지수 |
|
38 |
+ 작 성 일 : 2024.07.29 |
|
39 |
+ 내 용 : 특정 단원 평가 정보 read |
|
40 |
+ --> |
|
41 |
+ <select id="evaluationUnitList" parameterType="SearchVO" resultType="EvaluationVO"> |
|
42 |
+ SELECT |
|
43 |
+ eval_id, |
|
44 |
+ eval_type, |
|
45 |
+ unit_id |
|
46 |
+ FROM evaluation |
|
47 |
+ WHERE unit_id = #{unitId} |
|
48 |
+ <if test="option != null and keyword != null"> |
|
49 |
+ <choose> |
|
50 |
+ <when test="option == 'eval_type'"> |
|
51 |
+ AND eval_type LIKE CONCAT('%', #{keyword}, '%') |
|
52 |
+ </when> |
|
53 |
+ <when test="option == 'eval_id'"> |
|
54 |
+ AND eval_id = #{keyword} |
|
55 |
+ </when> |
|
56 |
+ <otherwise> |
|
57 |
+ AND (eval_type LIKE CONCAT('%', #{keyword}, '%') |
|
58 |
+ OR eval_id = #{keyword}) |
|
59 |
+ </otherwise> |
|
60 |
+ </choose> |
|
61 |
+ </if> |
|
62 |
+ LIMIT #{pageSize} OFFSET #{startIndex} |
|
63 |
+ </select> |
|
64 |
+ |
|
65 |
+ <!-- |
|
66 |
+ 작 성 자 : 권지수 |
|
67 |
+ 작 성 일 : 2024.07.29 |
|
68 |
+ 내 용 : 단원 평가 정보 update |
|
69 |
+ --> |
|
70 |
+ <update id="updateEvaluation" parameterType="EvaluationVO"> |
|
71 |
+ UPDATE evaluation |
|
72 |
+ SET |
|
73 |
+ eval_id = #{evalId}, |
|
74 |
+ eval_type = #{evalType}, |
|
75 |
+ unit_id = #{unitId} |
|
76 |
+ WHERE eval_id = #{evalId} |
|
77 |
+ </update> |
|
78 |
+ |
|
79 |
+ <!-- |
|
80 |
+ 작 성 자 : 권지수 |
|
81 |
+ 작 성 일 : 2024.07.29 |
|
82 |
+ 내 용 : 단원 평가 정보 delete |
|
83 |
+ --> |
|
84 |
+ <delete id="deleteEvaluation" parameterType="EvaluationVO"> |
|
85 |
+ DELETE FROM evaluation |
|
86 |
+ WHERE eval_id = #{evalId} |
|
87 |
+ </delete> |
|
5 | 88 |
|
6 | 89 |
|
7 | 90 |
</mapper>(파일 끝에 줄바꿈 문자 없음) |
--- src/main/resources/mybatis/mapper/lms/problem-SQL.xml
+++ src/main/resources/mybatis/mapper/lms/problem-SQL.xml
... | ... | @@ -2,6 +2,17 @@ |
2 | 2 |
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
3 | 3 |
<mapper namespace="com.takensoft.ai_lms.lms.problem.dao.ProblemDAO"> |
4 | 4 |
|
5 |
+ <!--resultMap id="problemMap" type="ProblemVO"> |
|
6 |
+ <result property="prblmId" column="prblm_id"/> |
|
7 |
+ <result property="prblmExpln" column="prblm_expln"/> |
|
8 |
+ <result property="prblmScr" column="prblm_scr"/> |
|
9 |
+ <result property="prblmHint" column="prblm_hint"/> |
|
10 |
+ <result property="prblmCmmt" column="prblm_cmmt"/> |
|
11 |
+ <result property="fileMngId" column="file_mng_id"/> |
|
12 |
+ <result property="prblmTypeId" column="prblm_type_id"/> |
|
13 |
+ <result property="prblmCtgryId" column="prblm_ctgry_id"/> |
|
14 |
+ </resultMap--> |
|
15 |
+ |
|
5 | 16 |
<!-- |
6 | 17 |
작 성 자 : 권지수 |
7 | 18 |
작 성 일 : 2024.07.25 |
... | ... | @@ -127,5 +138,85 @@ |
127 | 138 |
WHERE prblm_id = #{prblmId} |
128 | 139 |
</delete> |
129 | 140 |
|
141 |
+ <!-- |
|
142 |
+ 작 성 자 : 권지수 |
|
143 |
+ 작 성 일 : 2024.07.30 |
|
144 |
+ 내 용 : 전체 문제 목록 조회 |
|
145 |
+ --> |
|
146 |
+ <select id="problemList" parameterType="SearchVO" resultType="ProblemVO"> |
|
147 |
+ SELECT |
|
148 |
+ prblm_id, |
|
149 |
+ prblm_expln, |
|
150 |
+ prblm_scr, |
|
151 |
+ prblm_hint, |
|
152 |
+ prblm_cmmt, |
|
153 |
+ file_mng_id, |
|
154 |
+ prblm_type_id, |
|
155 |
+ prblm_ctgry_id |
|
156 |
+ FROM problem |
|
157 |
+ WHERE 1 = 1 |
|
158 |
+ <if test="option != null and keyword != null"> |
|
159 |
+ <choose> |
|
160 |
+ <when test="option == 'prblm_expln'"> |
|
161 |
+ AND prblm_expln LIKE CONCAT('%', #{keyword}, '%') |
|
162 |
+ </when> |
|
163 |
+ <when test="option == 'prblm_type_id'"> |
|
164 |
+ AND prblm_type_id = #{keyword} |
|
165 |
+ </when> |
|
166 |
+ <when test="option == 'prblm_ctgry_id'"> |
|
167 |
+ AND prblm_ctgry_id = #{keyword} |
|
168 |
+ </when> |
|
169 |
+ <otherwise> |
|
170 |
+ AND (prblm_expln LIKE CONCAT('%', #{keyword}, '%') |
|
171 |
+ OR prblm_type_id = #{keyword} |
|
172 |
+ OR prblm_ctgry_id = #{keyword}) |
|
173 |
+ </otherwise> |
|
174 |
+ </choose> |
|
175 |
+ </if> |
|
176 |
+ LIMIT #{pageSize} OFFSET #{startIndex} |
|
177 |
+ |
|
178 |
+ </select> |
|
179 |
+ |
|
180 |
+ <!-- |
|
181 |
+ 작 성 자 : 권지수 |
|
182 |
+ 작 성 일 : 2024.07.25 |
|
183 |
+ 내 용 : 특정 단원 평가의 문제 목록 조회 |
|
184 |
+ --> |
|
185 |
+ <select id="evaluationProblemList" parameterType="SearchVO" resultType="ProblemVO"> |
|
186 |
+ SELECT |
|
187 |
+ p.prblm_id AS prblmId, |
|
188 |
+ p.prblm_expln AS prblmExpln, |
|
189 |
+ p.prblm_scr AS prblmScr, |
|
190 |
+ p.prblm_hint AS prblmHint, |
|
191 |
+ p.prblm_cmmt AS prblmCmmt, |
|
192 |
+ p.file_mng_id AS fileMngId, |
|
193 |
+ p.prblm_type_id AS prblmTypeId, |
|
194 |
+ p.prblm_ctgry_id AS prblmCtgryId |
|
195 |
+ FROM |
|
196 |
+ eval_problem ep |
|
197 |
+ JOIN |
|
198 |
+ problem p ON ep.prblm_id = p.prblm_id |
|
199 |
+ WHERE |
|
200 |
+ ep.eval_id = #{evalId} |
|
201 |
+ <if test="option != null and keyword != null"> |
|
202 |
+ <choose> |
|
203 |
+ <when test="option == 'prblm_expln'"> |
|
204 |
+ AND prblm_expln LIKE CONCAT('%', #{keyword}, '%') |
|
205 |
+ </when> |
|
206 |
+ <when test="option == 'prblm_type_id'"> |
|
207 |
+ AND prblm_type_id = #{keyword} |
|
208 |
+ </when> |
|
209 |
+ <when test="option == 'prblm_ctgry_id'"> |
|
210 |
+ AND prblm_ctgry_id = #{keyword} |
|
211 |
+ </when> |
|
212 |
+ <otherwise> |
|
213 |
+ AND (prblm_expln LIKE CONCAT('%', #{keyword}, '%') |
|
214 |
+ OR prblm_type_id = #{keyword} |
|
215 |
+ OR prblm_ctgry_id = #{keyword}) |
|
216 |
+ </otherwise> |
|
217 |
+ </choose> |
|
218 |
+ </if> |
|
219 |
+ </select> |
|
220 |
+ |
|
130 | 221 |
|
131 | 222 |
</mapper>(파일 끝에 줄바꿈 문자 없음) |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?