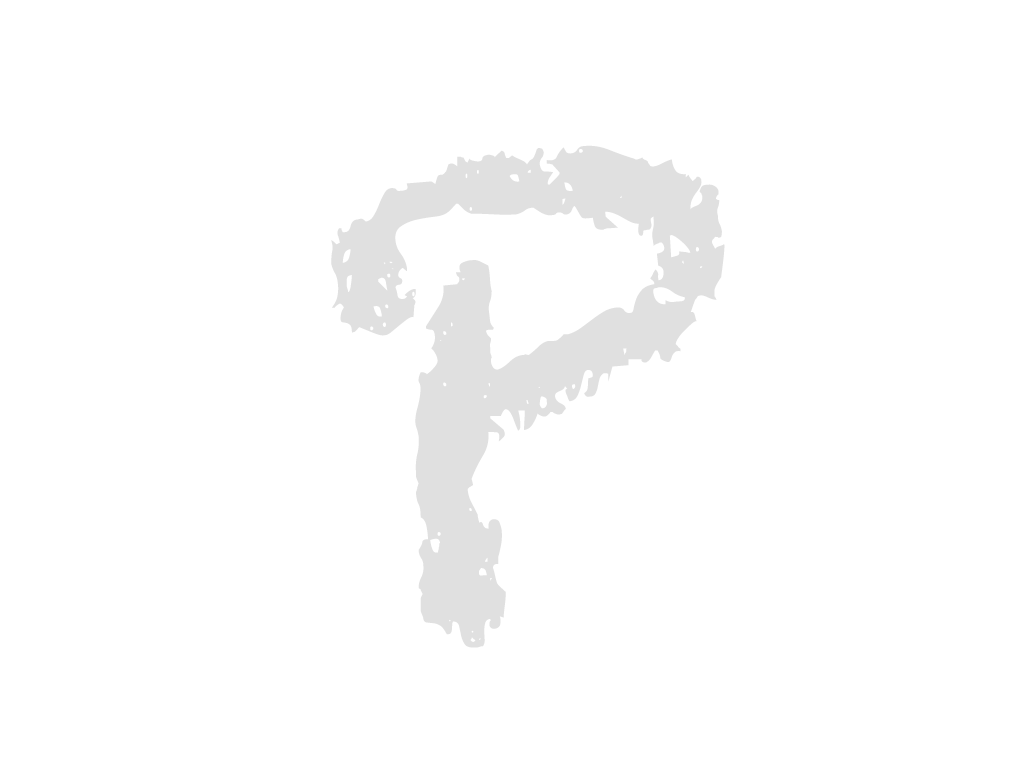
+++ src/main/java/com/takensoft/ai_lms/lms/schedule/dao/ScheduleDAO.java
... | ... | @@ -0,0 +1,25 @@ |
1 | +package com.takensoft.ai_lms.lms.schedule.dao; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.schedule.vo.ScheduleVO; | |
4 | +import java.util.HashMap; | |
5 | +import java.util.List; | |
6 | + | |
7 | +/** | |
8 | + * @author : 이은진 | |
9 | + * @since : 2024.07.29 | |
10 | + * | |
11 | + * 학습 일정 관련 Mapper | |
12 | + */ | |
13 | +public interface ScheduleDAO { | |
14 | + //학습 일정 등록 | |
15 | + int insertSchedule(ScheduleVO scheduleVO) throws Exception; | |
16 | + | |
17 | + // 학습 일정 출력 | |
18 | + List<HashMap<String, Object>> selectSchedule(HashMap<String, Object> params) throws Exception; | |
19 | + | |
20 | + // 학습 일정 수정 | |
21 | + int scheduleUpdate(ScheduleVO scheduleVO) throws Exception; | |
22 | + | |
23 | + // 학습 일정 삭제 | |
24 | + int scheduleDelete(String scheduleID) throws Exception; | |
25 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/schedule/service/Impl/ScheduleServiceImpl.java
... | ... | @@ -0,0 +1,58 @@ |
1 | +package com.takensoft.ai_lms.lms.schedule.service.Impl; | |
2 | + | |
3 | +import com.takensoft.ai_lms.common.idgen.service.IdgenService; | |
4 | +import com.takensoft.ai_lms.lms.schedule.dao.ScheduleDAO; | |
5 | +import com.takensoft.ai_lms.lms.schedule.service.ScheduleService; | |
6 | +import com.takensoft.ai_lms.lms.schedule.vo.ScheduleVO; | |
7 | +import lombok.RequiredArgsConstructor; | |
8 | +import org.springframework.stereotype.Service; | |
9 | +import java.util.HashMap; | |
10 | +import java.util.List; | |
11 | + | |
12 | + | |
13 | +/** | |
14 | + * @author : 이은진 | |
15 | + * @since : 2024.07.29 | |
16 | + */ | |
17 | + | |
18 | +@Service("scheduleService") | |
19 | +@RequiredArgsConstructor | |
20 | +public class ScheduleServiceImpl implements ScheduleService { | |
21 | + | |
22 | + private final ScheduleDAO scheduleDAO; | |
23 | + private final IdgenService scheduleIdgn; | |
24 | + | |
25 | + /** | |
26 | + * 학습 일정 등록 | |
27 | + */ | |
28 | + @Override | |
29 | + public int insertSchedule(ScheduleVO scheduleVO) throws Exception { | |
30 | + String scheduleId = scheduleIdgn.getNextStringId(); | |
31 | + scheduleVO.setScheduleId(scheduleId); | |
32 | + return scheduleDAO.insertSchedule(scheduleVO); | |
33 | + } | |
34 | + | |
35 | + /** | |
36 | + * 학습 일정 출력 | |
37 | + */ | |
38 | + @Override | |
39 | + public List<HashMap<String, Object>> selectSchedule(HashMap<String, Object> params) throws Exception { | |
40 | + return scheduleDAO.selectSchedule(params); | |
41 | + } | |
42 | + | |
43 | + /** | |
44 | + * 학습 일정 수정 | |
45 | + */ | |
46 | + @Override | |
47 | + public int scheduleUpdate(ScheduleVO scheduleVO) throws Exception { | |
48 | + return scheduleDAO.scheduleUpdate(scheduleVO); | |
49 | + } | |
50 | + | |
51 | + /** | |
52 | + * 학습 일정 삭제 | |
53 | + */ | |
54 | + @Override | |
55 | + public int scheduleDelete(String scheduleId) throws Exception { | |
56 | + return scheduleDAO.scheduleDelete(scheduleId); | |
57 | + } | |
58 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/schedule/service/ScheduleService.java
... | ... | @@ -0,0 +1,26 @@ |
1 | +package com.takensoft.ai_lms.lms.schedule.service; | |
2 | + | |
3 | +import com.takensoft.ai_lms.lms.schedule.vo.ScheduleVO; | |
4 | +import java.util.HashMap; | |
5 | +import java.util.List; | |
6 | + | |
7 | +/** | |
8 | + * @author : 이은진 | |
9 | + * since : 2024.07.29 | |
10 | + * | |
11 | + * 학습 일정 관련 인터페이스 | |
12 | + */ | |
13 | +public interface ScheduleService { | |
14 | + | |
15 | + // 학습 일정 등록 | |
16 | + int insertSchedule(ScheduleVO scheduleVO) throws Exception; | |
17 | + | |
18 | + // 학습 일정 출력 | |
19 | + List<HashMap<String, Object>> selectSchedule(HashMap<String, Object> params) throws Exception; | |
20 | + | |
21 | + // 학습 일정 수정 | |
22 | + int scheduleUpdate(ScheduleVO scheduleVO) throws Exception; | |
23 | + | |
24 | + // 학습 일정 삭제 | |
25 | + int scheduleDelete(String scheduleId) throws Exception; | |
26 | +} |
+++ src/main/java/com/takensoft/ai_lms/lms/schedule/vo/ScheduleVO.java
... | ... | @@ -0,0 +1,30 @@ |
1 | +package com.takensoft.ai_lms.lms.schedule.vo; | |
2 | + | |
3 | +import lombok.AllArgsConstructor; | |
4 | +import lombok.Getter; | |
5 | +import lombok.NoArgsConstructor; | |
6 | +import lombok.Setter; | |
7 | + | |
8 | +/** | |
9 | + * @author : 이은진 | |
10 | + * since : 2024.07.29 | |
11 | + * | |
12 | + * 스케줄 관련 VO | |
13 | + */ | |
14 | + | |
15 | +@Setter | |
16 | +@Getter | |
17 | +@NoArgsConstructor | |
18 | +@AllArgsConstructor | |
19 | +public class ScheduleVO { | |
20 | + // 학습 일정 아이디 | |
21 | + private String scheduleId; | |
22 | + // 학습 날짜 | |
23 | + private String scheduleDt; | |
24 | + // 학습 교시 | |
25 | + private String scheduleUnit; | |
26 | + // 책 아이디 | |
27 | + private String bookId; | |
28 | + // 학생 아이디 | |
29 | + private String stdId; | |
30 | +}(파일 끝에 줄바꿈 문자 없음) |
+++ src/main/java/com/takensoft/ai_lms/lms/schedule/web/ScheduleController.java
... | ... | @@ -0,0 +1,118 @@ |
1 | +package com.takensoft.ai_lms.lms.schedule.web; | |
2 | + | |
3 | +import com.google.gson.Gson; | |
4 | +import com.google.gson.JsonObject; | |
5 | +import com.takensoft.ai_lms.lms.schedule.service.ScheduleService; | |
6 | +import com.takensoft.ai_lms.lms.schedule.vo.ScheduleVO; | |
7 | +import io.swagger.v3.oas.annotations.Operation; | |
8 | +import lombok.RequiredArgsConstructor; | |
9 | +import lombok.extern.slf4j.Slf4j; | |
10 | +import org.springframework.web.bind.annotation.*; | |
11 | + | |
12 | +import java.util.HashMap; | |
13 | +import java.util.List; | |
14 | +import java.util.Map; | |
15 | + | |
16 | +/** | |
17 | + * @author 이은진 | |
18 | + * @since 2024.07.29 | |
19 | + */ | |
20 | + | |
21 | +@RestController | |
22 | +@RequiredArgsConstructor | |
23 | +@Slf4j | |
24 | +@RequestMapping(value = "/schedule") | |
25 | +public class ScheduleController { | |
26 | + | |
27 | + private final ScheduleService scheduleService; | |
28 | + | |
29 | + /** | |
30 | + * 학습 일정 등록 | |
31 | + */ | |
32 | + @PostMapping("/insertSchedule.json") | |
33 | + @Operation(summary = "스케줄 등록") | |
34 | + public String insertSchedule(@RequestBody ScheduleVO schedulevO) throws Exception { | |
35 | + Gson gson = new Gson(); | |
36 | + JsonObject response = new JsonObject(); | |
37 | + | |
38 | + try { | |
39 | + int result = scheduleService.insertSchedule(schedulevO); | |
40 | + if (result > 0) { | |
41 | + response.addProperty("status", "success"); | |
42 | + response.addProperty("message", "학습 일정이 등록되었습니다."); | |
43 | + return gson.toJson(response); | |
44 | + } else { | |
45 | + response.addProperty("message", "학습 일정 등록 실패"); | |
46 | + return gson.toJson(response); | |
47 | + } | |
48 | + } catch (Exception e) { | |
49 | + response.addProperty("status", "error"); | |
50 | + response.addProperty("message", e.getMessage()); | |
51 | + return gson.toJson(response); | |
52 | + } | |
53 | + } | |
54 | + | |
55 | + /** | |
56 | + * 학습 일정 출력 | |
57 | + */ | |
58 | + @GetMapping("/selectSchedule.json") | |
59 | + @Operation(summary = "스케줄 출력") | |
60 | + public List<HashMap<String, Object>> selectSchedule(@RequestParam HashMap<String, Object> scheduleId) throws Exception { | |
61 | + return scheduleService.selectSchedule(scheduleId); | |
62 | + } | |
63 | + | |
64 | + | |
65 | + /** | |
66 | + * 학습 일정 수정 | |
67 | + */ | |
68 | + @PutMapping(value = "/scheduleUpdate.json") | |
69 | + @Operation(summary = "스케줄 수정") | |
70 | + public String scheduleUpdate(@RequestBody ScheduleVO scheduleVO) throws Exception { | |
71 | + Gson gson = new Gson(); | |
72 | + JsonObject response = new JsonObject(); | |
73 | + | |
74 | + try { | |
75 | + int result = scheduleService.scheduleUpdate(scheduleVO); | |
76 | + if (result > 0) { | |
77 | + response.addProperty("status", "success"); | |
78 | + response.addProperty("message", "학습 일정이 수정되었습니다."); | |
79 | + return gson.toJson(response); | |
80 | + } else { | |
81 | + response.addProperty("message", "학습 일정 수정 실패"); | |
82 | + return gson.toJson(response); | |
83 | + } | |
84 | + } catch (Exception e) { | |
85 | + response.addProperty("status", "error"); | |
86 | + response.addProperty("message", e.getMessage()); | |
87 | + return gson.toJson(response); | |
88 | + } | |
89 | + } | |
90 | + | |
91 | + | |
92 | + /** | |
93 | + * 학습 일정 삭제 | |
94 | + */ | |
95 | + @DeleteMapping(value = "/scheduleDelete.json") | |
96 | + @Operation(summary = "스케줄 삭제") | |
97 | + public String scheduleDelete(@RequestBody Map<String, String> request) throws Exception { | |
98 | + Gson gson = new Gson(); | |
99 | + JsonObject response = new JsonObject(); | |
100 | + | |
101 | + String scheduleId = request.get("scheduleId"); | |
102 | + int result = scheduleService.scheduleDelete(scheduleId); | |
103 | + try { | |
104 | + if (result > 0) { | |
105 | + response.addProperty("status", "success"); | |
106 | + response.addProperty("message", "학습 일정이 삭제되었습니다."); | |
107 | + return gson.toJson(response); | |
108 | + } else { | |
109 | + response.addProperty("message", "학습 일정 삭제 실패"); | |
110 | + return gson.toJson(response); | |
111 | + } | |
112 | + } catch (Exception e) { | |
113 | + response.addProperty("status", "error"); | |
114 | + response.addProperty("message", e.getMessage()); | |
115 | + return gson.toJson(response); | |
116 | + } | |
117 | + } | |
118 | +} |
--- src/main/java/com/takensoft/ai_lms/lms/text/vo/TextVO.java
+++ src/main/java/com/takensoft/ai_lms/lms/text/vo/TextVO.java
... | ... | @@ -5,7 +5,6 @@ |
5 | 5 |
import lombok.NoArgsConstructor; |
6 | 6 |
import lombok.Setter; |
7 | 7 |
|
8 |
-import java.time.LocalDateTime; |
|
9 | 8 |
|
10 | 9 |
/** |
11 | 10 |
* @author : 이은진 |
... | ... | @@ -22,13 +21,19 @@ |
22 | 21 |
|
23 | 22 |
// 지문 아이디 |
24 | 23 |
private String textId; |
24 |
+ // 지문 제목 |
|
25 |
+ private String textTtl; |
|
25 | 26 |
// 지문 내용 |
26 | 27 |
private String textCnt; |
28 |
+ // 지문 url |
|
29 |
+ private String textUrl; |
|
27 | 30 |
// 등록일 |
28 | 31 |
private String regDt; |
29 | 32 |
// 파일 관리 아이디 |
30 | 33 |
private String fileMngId; |
31 | 34 |
// 유형 아이디 |
32 | 35 |
private String textTypeId; |
36 |
+ // 사용자 아이디(선생님) |
|
37 |
+ private String userId; |
|
33 | 38 |
|
34 | 39 |
} |
--- src/main/java/com/takensoft/ai_lms/lms/text/web/TextController.java
+++ src/main/java/com/takensoft/ai_lms/lms/text/web/TextController.java
... | ... | @@ -80,7 +80,7 @@ |
80 | 80 |
*/ |
81 | 81 |
@GetMapping("/selectOneText.json") |
82 | 82 |
@Operation(summary = "지문 출력") |
83 |
- public List<HashMap<String, Object>> selectOneText(@RequestParam HashMap<String, Object> textId) throws Exception { |
|
83 |
+ public List<HashMap<String, Object>> selectOneText(@RequestBody HashMap<String, Object> textId) throws Exception { |
|
84 | 84 |
return TextService.selectOneText(textId); |
85 | 85 |
} |
86 | 86 |
|
+++ src/main/resources/mybatis/mapper/lms/schedule-SQL.xml
... | ... | @@ -0,0 +1,72 @@ |
1 | +<?xml version="1.0" encoding="UTF-8"?> | |
2 | +<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> | |
3 | +<mapper namespace="com.takensoft.ai_lms.lms.schedule.dao.ScheduleDAO"> | |
4 | + | |
5 | + <resultMap id="scheduleMap" type="ScheduleVO"> | |
6 | + <result property="scheduleId" column="schdl_id"/> | |
7 | + <result property="scheduleDt" column="schdl_dt"/> | |
8 | + <result property="scheduleUnit" column="schdl_unit"/> | |
9 | + <result property="bookId" column="book_id"/> | |
10 | + <result property="stdId" column="std_id"/> | |
11 | + </resultMap> | |
12 | + | |
13 | + | |
14 | + <!-- | |
15 | + 작성자 : 이은진 | |
16 | + 작성일 : 2024.07.29 | |
17 | + 내 용 : 스케줄 등록 | |
18 | + --> | |
19 | + <insert id="insertSchedule" parameterType="ScheduleVO"> | |
20 | + INSERT INTO schedule (schdl_id | |
21 | + ,schdl_dt | |
22 | + ,schdl_unit | |
23 | + ,book_id | |
24 | + ,std_id | |
25 | + ) VALUES (#{scheduleId} | |
26 | + ,now() | |
27 | + ,#{scheduleUnit} | |
28 | + ,#{bookId} | |
29 | + ,#{stdId} | |
30 | + ); | |
31 | + </insert> | |
32 | + | |
33 | + <!-- | |
34 | + 작성자 : 이은진 | |
35 | + 작성일 : 2024.07.29 | |
36 | + 내 용 : 스케줄 출력 | |
37 | + --> | |
38 | + <select id="selectSchedule" parameterType="HashMap" resultType="HashMap"> | |
39 | + SELECT schdl_id | |
40 | + , schdl_dt | |
41 | + , schdl_unit | |
42 | + , book_id | |
43 | + , std_id | |
44 | + | |
45 | + FROM schedule | |
46 | + ORDER BY schdl_dt DESC | |
47 | + </select> | |
48 | + | |
49 | + <!-- | |
50 | + 작성자 : 이은진 | |
51 | + 작성일 : 2024.07.29 | |
52 | + 내 용 : 스케줄 수정 | |
53 | + --> | |
54 | + <update id="scheduleUpdate" parameterType="ScheduleVO"> | |
55 | + UPDATE schedule | |
56 | + SET schdl_dt = #{scheduleDt} | |
57 | + , schdl_unit = #{scheduleUnit} | |
58 | + , book_id = #{bookId} | |
59 | + WHERE schdl_id = #{scheduleId} | |
60 | + </update> | |
61 | + | |
62 | + <!-- | |
63 | + 작성자 : 이은진 | |
64 | + 작성일 : 2024.07.29 | |
65 | + 내 용 : 스케줄 삭제 | |
66 | + --> | |
67 | + <delete id="scheduleDelete" parameterType="String"> | |
68 | + DELETE FROM schedule | |
69 | + WHERE schdl_id = #{scheduleId} | |
70 | + </delete> | |
71 | + | |
72 | +</mapper> |
--- src/main/resources/mybatis/mapper/lms/text-SQL.xml
+++ src/main/resources/mybatis/mapper/lms/text-SQL.xml
... | ... | @@ -4,10 +4,13 @@ |
4 | 4 |
|
5 | 5 |
<resultMap id="textMap" type="TextVO"> |
6 | 6 |
<result property="textId" column="text_id"/> |
7 |
+ <result property="textTtl" column="text_ttl"/> |
|
7 | 8 |
<result property="textCnt" column="text_cnt"/> |
9 |
+ <result property="textUrl" column="text_url"/> |
|
8 | 10 |
<result property="regDt" column="reg_dt"/> |
9 | 11 |
<result property="fileMngId" column="file_mng_id"/> |
10 | 12 |
<result property="textTypeId" column="text_type_id"/> |
13 |
+ <result property="userId" column="user_id"/> |
|
11 | 14 |
</resultMap> |
12 | 15 |
|
13 | 16 |
|
... | ... | @@ -18,15 +21,21 @@ |
18 | 21 |
--> |
19 | 22 |
<insert id="insertText" parameterType="TextVO"> |
20 | 23 |
INSERT INTO text (text_id |
24 |
+ ,text_ttl |
|
21 | 25 |
,text_cnt |
26 |
+ ,text_url |
|
22 | 27 |
,reg_dt |
23 | 28 |
,file_mng_id |
24 | 29 |
,text_type_id |
30 |
+ ,user_id |
|
25 | 31 |
) VALUES (#{textId} |
32 |
+ ,#{textTtl} |
|
26 | 33 |
,#{textCnt} |
34 |
+ ,#{textUrl} |
|
27 | 35 |
,now() |
28 | 36 |
,#{fileMngId} |
29 | 37 |
,#{textTypeId} |
38 |
+ ,#{userId} |
|
30 | 39 |
); |
31 | 40 |
</insert> |
32 | 41 |
|
... | ... | @@ -47,10 +56,13 @@ |
47 | 56 |
--> |
48 | 57 |
<select id="selectTextList" resultMap="textMap"> |
49 | 58 |
SELECT text_id |
59 |
+ ,text_ttl |
|
50 | 60 |
,text_cnt |
61 |
+ ,text_url |
|
51 | 62 |
,reg_dt |
52 | 63 |
,file_mng_id |
53 | 64 |
,text_type_id |
65 |
+ ,user_id |
|
54 | 66 |
FROM text |
55 | 67 |
ORDER BY text_id DESC |
56 | 68 |
LIMIT #{pageSize} |
... | ... | @@ -64,11 +76,13 @@ |
64 | 76 |
--> |
65 | 77 |
<select id="selectOneText" parameterType="HashMap" resultType="HashMap"> |
66 | 78 |
SELECT text_id |
79 |
+ ,text_ttl |
|
67 | 80 |
, text_cnt |
81 |
+ ,text_url |
|
68 | 82 |
, reg_dt |
69 | 83 |
, file_mng_id |
70 | 84 |
, text_type_id |
71 |
- |
|
85 |
+ ,user_id |
|
72 | 86 |
FROM text |
73 | 87 |
WHERE text_id = #{textId} |
74 | 88 |
ORDER BY text_id DESC |
... | ... | @@ -81,7 +95,9 @@ |
81 | 95 |
--> |
82 | 96 |
<update id="textUpdate" parameterType="TextVO"> |
83 | 97 |
UPDATE text |
84 |
- SET text_cnt = #{textCnt} |
|
98 |
+ SET text_ttl = #{textTtl} |
|
99 |
+ , text_cnt = #{textCnt} |
|
100 |
+ , text_url = #{textUrl} |
|
85 | 101 |
, file_mng_id = #{fileMngId} |
86 | 102 |
, text_type_id = #{textTypeId} |
87 | 103 |
WHERE text_id = #{textId} |
... | ... | @@ -112,9 +128,15 @@ |
112 | 128 |
<when test="option == 'textId'"> |
113 | 129 |
AND text_id LIKE CONCAT('%', #{keyword}, '%') |
114 | 130 |
</when> |
131 |
+ <when test="option == 'textTtl'"> |
|
132 |
+ AND text_ttl LIKE CONCAT('%', #{keyword}, '%') |
|
133 |
+ </when> |
|
115 | 134 |
<when test="option == 'textCnt'"> |
116 | 135 |
AND text_cnt LIKE CONCAT('%', #{keyword}, '%') |
117 | 136 |
</when> |
137 |
+ <when test="option == 'userId'"> |
|
138 |
+ AND user_id LIKE CONCAT('%', #{keyword}, '%') |
|
139 |
+ </when> |
|
118 | 140 |
<when test="option == 'regDt'"> |
119 | 141 |
AND TO_CHAR(reg_dt, 'YYYY-MM-DD HH24:MI:SS') LIKE CONCAT('%', #{keyword}, '%') |
120 | 142 |
</when> |
Add a comment
Delete comment
Once you delete this comment, you won't be able to recover it. Are you sure you want to delete this comment?