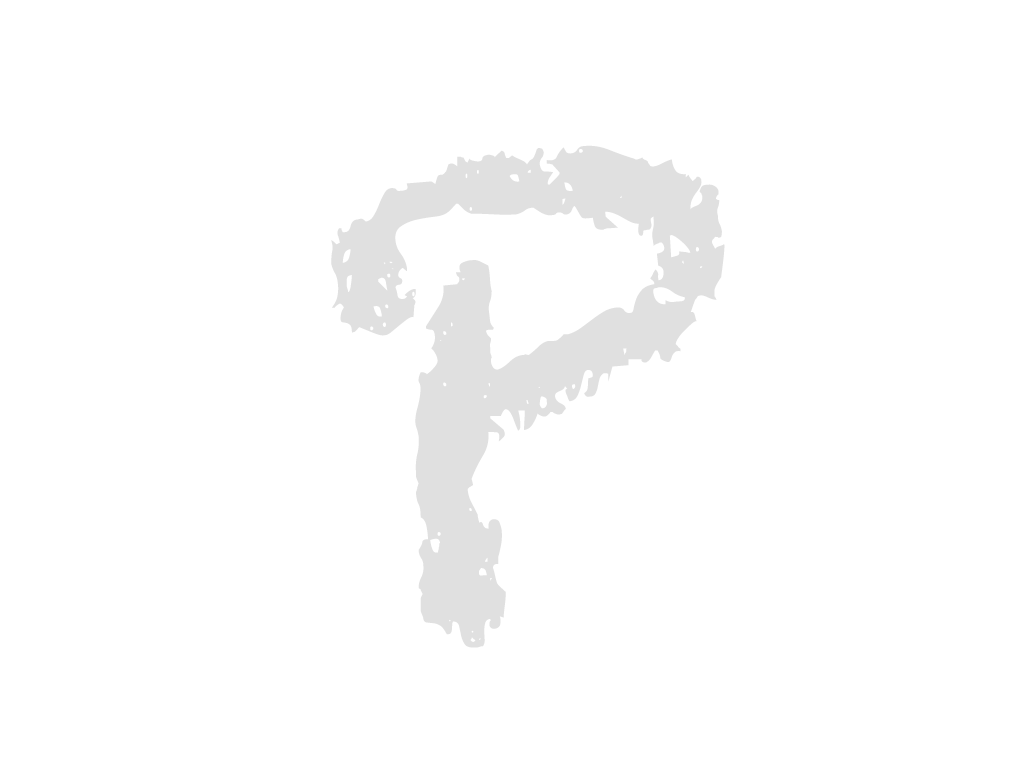
File name
Commit message
Commit date
File name
Commit message
Commit date
File name
Commit message
Commit date
import React, {useEffect} from 'react';
import {View, Text} from 'react-native';
import Modal from 'react-native-modal';
import {BLACK, RED, WHITE} from '../color';
const ModalComponent = ({isVisible, toggleModal, alertTitle, alertMessage}) => {
useEffect(() => {
if (isVisible) {
const timeoutId = setTimeout(() => {
toggleModal();
}, 2000);
// 컴포넌트가 언마운트될 때 clearTimeout
return () => clearTimeout(timeoutId);
}
}, [isVisible, toggleModal]);
return (
<Modal
isVisible={isVisible}
style={{
position: 'absolute',
bottom: 40,
left: 0,
width: '90%',
borderColor: RED,
borderWidth: 2,
borderRadius: 10,
overflow: 'hidden',
}}
backdropOpacity={0}>
<View
style={{
backgroundColor: WHITE,
padding: 22,
borderRadius: 10,
alignItems: 'center',
justifyContent: 'center',
}}>
<Text style={{color: RED, fontSize: 20}}>{alertTitle}</Text>
<Text style={{color: BLACK, fontSize: 15, marginTop: 5}}>
{alertMessage} 안전운행하세요.
</Text>
</View>
</Modal>
);
};
export default ModalComponent;